In MATLAB, the `cov` function calculates the covariance matrix of a given dataset, helping you understand the relationships between different variables.
% Example of using the cov function in MATLAB
data = [1, 2; 3, 4; 5, 6]; % Sample data
covarianceMatrix = cov(data); % Calculate the covariance matrix
disp(covarianceMatrix); % Display the covariance matrix
Understanding Covariance
What is Covariance?
Covariance is a statistical measure that indicates the extent to which two variables change in tandem. If both variables tend to increase together, the covariance is positive. If one variable tends to increase as the other decreases, the covariance is negative. Covariance provides insight into the relationship between datasets, which is essential for data analysis and statistics.
The Mathematical Formula
The mathematical definition of covariance is expressed as:
Cov(X,Y) = E[(X - μX)(Y - μY)]
- X and Y are the two variables.
- μX is the mean of X.
- μY is the mean of Y.
- E represents the expected value.
This formula highlights that covariance is based on how much the individual deviations of each variable from their respective means multiply together.
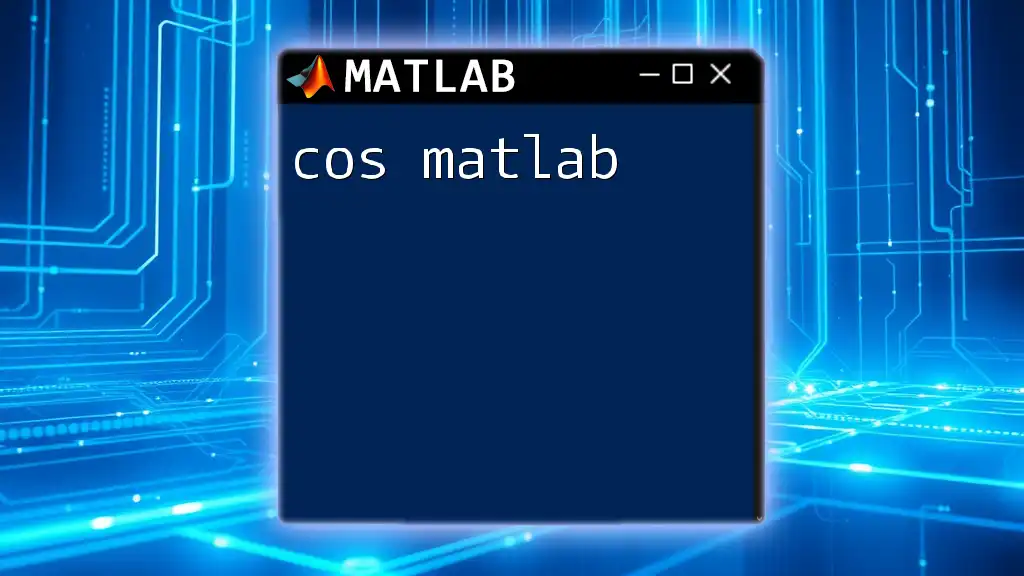
Using the cov Function in MATLAB
Basic Syntax of cov
In MATLAB, the covariance can be calculated using the cov function. The syntax varies slightly depending on the input:
C = cov(X)
C = cov(X,Y)
- X is a matrix where each column represents a variable and each row represents an observation.
- When using two variables (X and Y), cov computes the covariance between them, returning a covariance matrix.
Examples of Basic Covariance Calculation
Example: Covariance of a Single Dataset
data = [1 2; 3 4; 5 6];
covarianceMatrix = cov(data);
disp(covarianceMatrix);
Here, the output matrix will show the covariance of the two dimensions represented in the `data` array. A positive value indicates that both dimensions tend to increase together.
Example: Covariance of Two Datasets
X = [1 2 3];
Y = [4 5 6];
covarianceXY = cov(X, Y);
disp(covarianceXY);
This code snippet computes the covariance between the two vectors X and Y. The resulting output illustrates how the changes in one vector are associated with changes in the other.
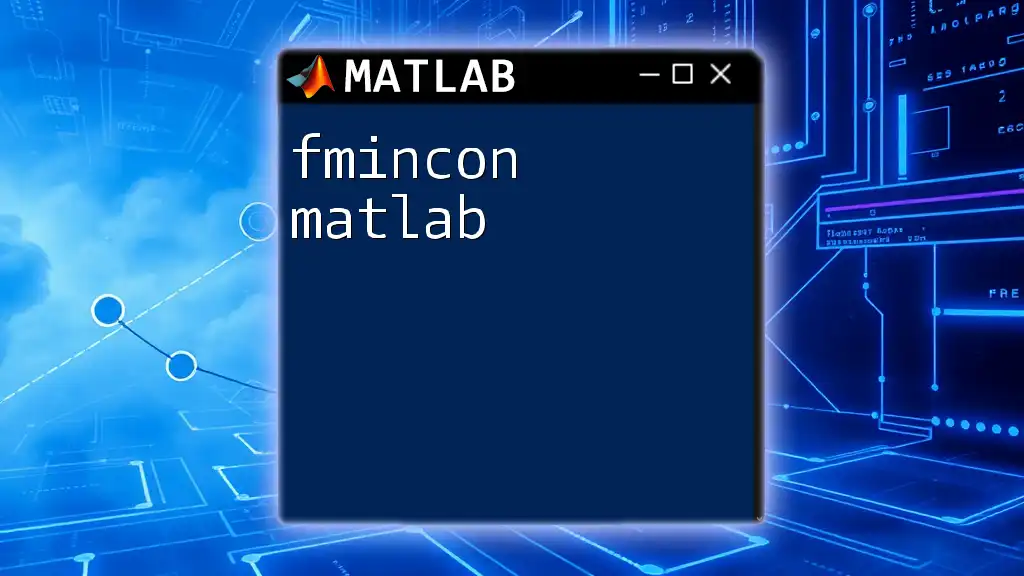
Practical Applications of cov in MATLAB
Financial Data Analysis
Covariance is frequently used in finance to understand the relationship between the return rates of different assets. For example, if you have stock prices for two companies, you can analyze how they move together.
% Sample stock prices
stock1 = [100, 102, 101, 104];
stock2 = [98, 97, 95, 96];
covarianceStocks = cov(stock1, stock2);
The output helps in identifying whether the stocks exhibit similar movements, which is beneficial for portfolio construction and risk assessment.
Machine Learning and Data Science
Covariance is also vital in machine learning for feature selection. When features in a dataset are highly correlated (high covariance), they may not provide additional information. Recognizing and eliminating such redundancy can improve the model's performance and accuracy.
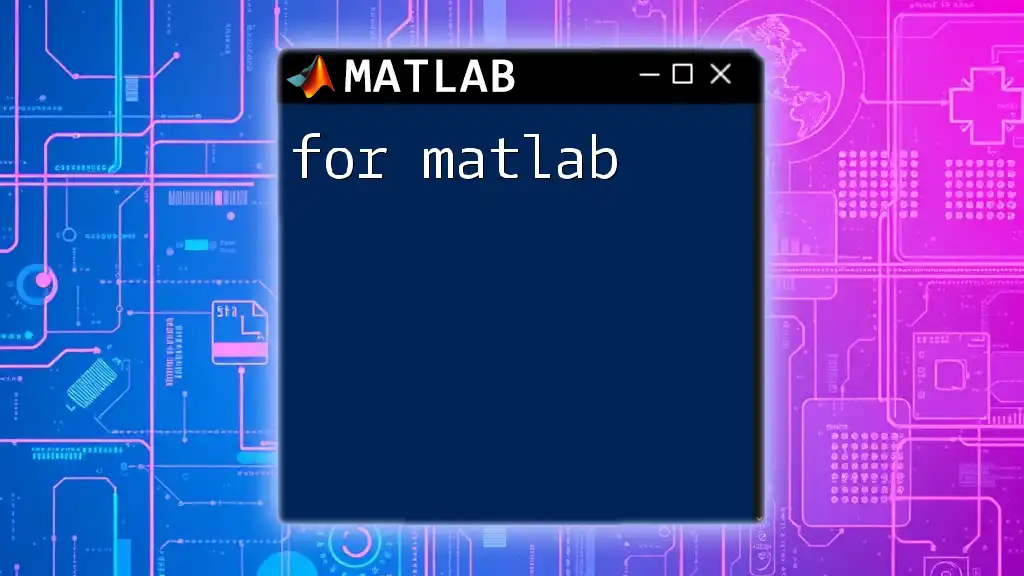
Handling Multidimensional Data
Covariance of Multidimensional Arrays
When working with multidimensional data, it's essential to know how to compute covariance beyond simple matrices. The cov function can handle higher dimensions, but you may need to reshape your data.
data3D = rand(4,3,2); % Generating random 3D data
cov3D = cov(reshape(data3D, [], 3)); % Reshaping and calculating covariance
disp(cov3D);
This snippet reshapes the 3D data for covariance calculation, enabling the analysis of relationships across multiple dimensions.
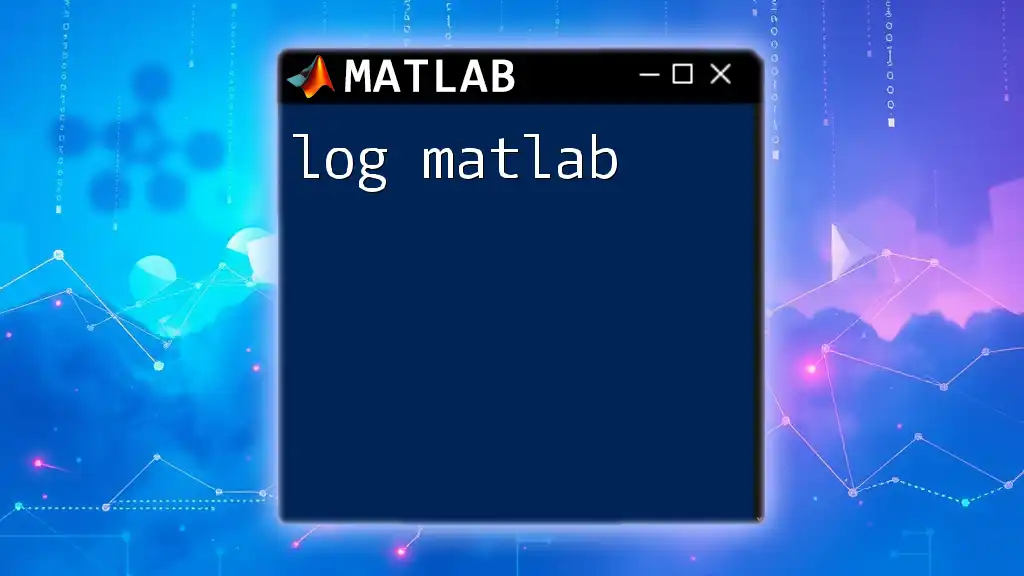
Advanced Techniques
Normalization of Data Before Covariance Calculation
To improve the effectiveness of covariance analysis, normalizing data is often recommended. This ensures that the variables are on the same scale, making the covariance more meaningful.
data = rand(10,2); % Generating random data
normalizedData = (data - mean(data))./std(data);
covNormalized = cov(normalizedData);
Normalization mitigates the risk of large value ranges influencing the calculation, leading to clearer insights.
Covariance with Missing Data
Handling missing data is crucial in statistical computations. The cov function in MATLAB can accommodate such scenarios.
dataWithNaN = [1, 2; NaN, 4; 5, 6];
covWithNaN = cov(dataWithNaN, 'omitrows');
The 'omitrows' option allows you to skip any rows containing NaN values, ensuring that the covariance is computed based only on the complete data.
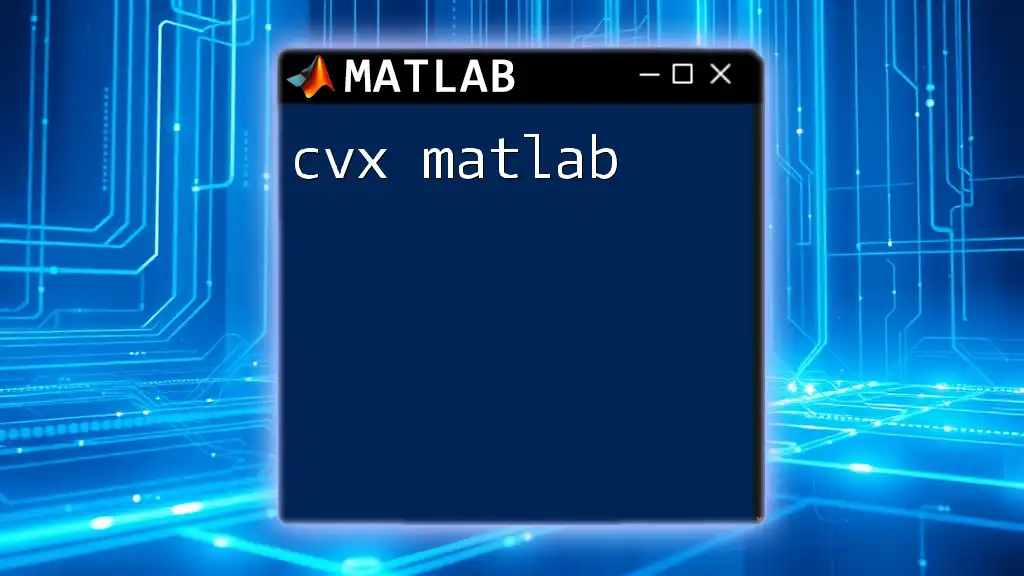
Visualizing Covariance
Heatmaps and Matrices
Visual representation of covariance can greatly enhance understanding. Heatmaps provide intuitive visual cues about relationships between various variables.
heatmap(covarianceMatrix);
This generates a graphical representation of the covariance values, where varying colors can signal the strength and direction of relationships, aiding in quick analysis.
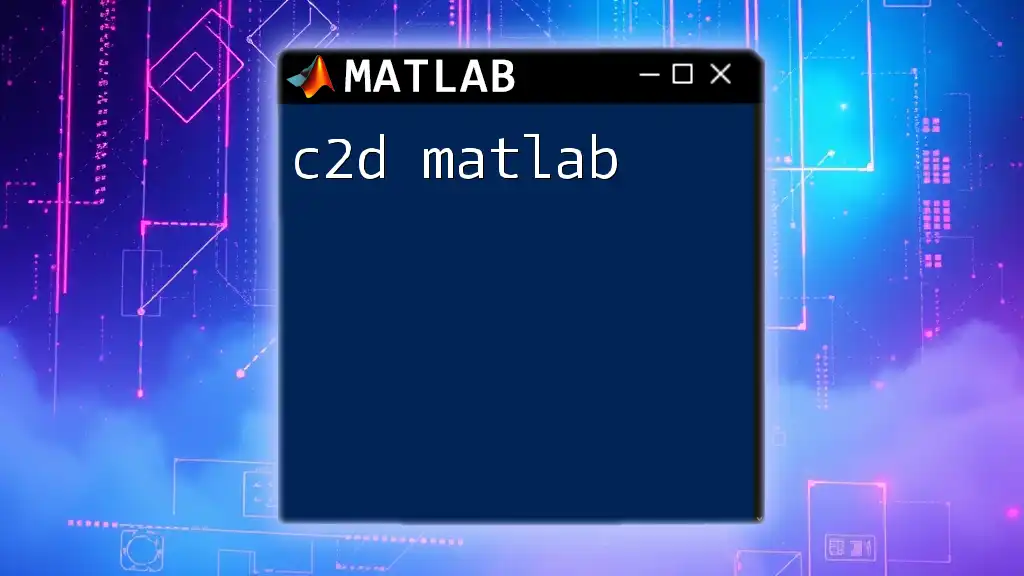
Common Mistakes and Troubleshooting
Understanding Output Matrix
A common misunderstanding when interpreting the output of the cov function is the layout of the covariance matrix. Remember that the diagonal elements represent the variance of each variable, while the off-diagonal elements represent the covariance between pairs of variables.
Troubleshooting Common Errors
Errors often arise due to dimension mismatches or incompatible data types. Always ensure that the input data is properly formatted; each variable should be in a column, and observations should be in rows.
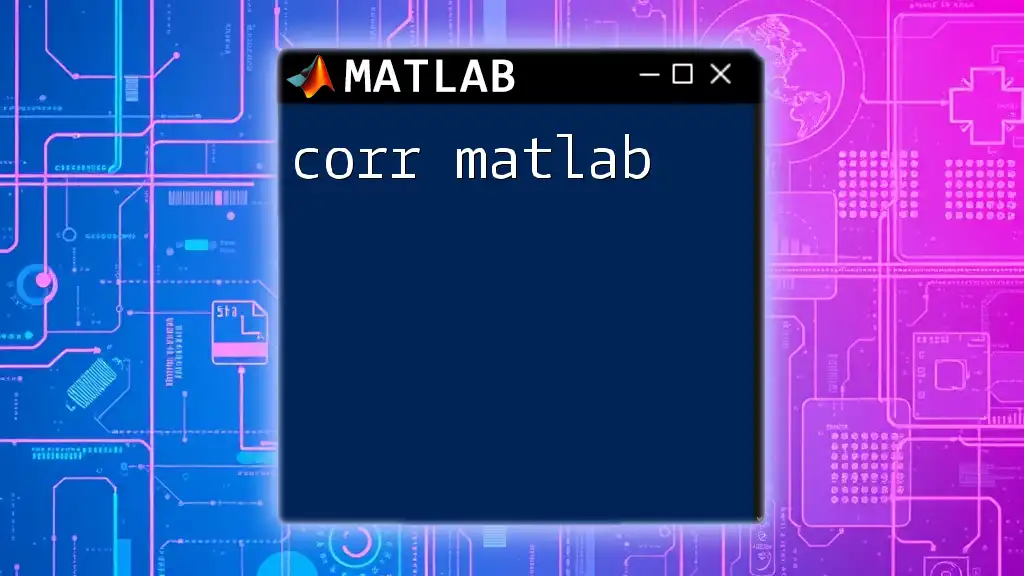
Conclusion
In summary, covariance is a foundational concept in statistics and data analysis, crucial for effectively interpreting relationships between datasets. The cov function in MATLAB equips users with the tools to compute covariance efficiently, whether for single datasets or pairs of variables. By mastering this function, one can uncover valuable insights that drive decision-making in various fields, from finance to machine learning.
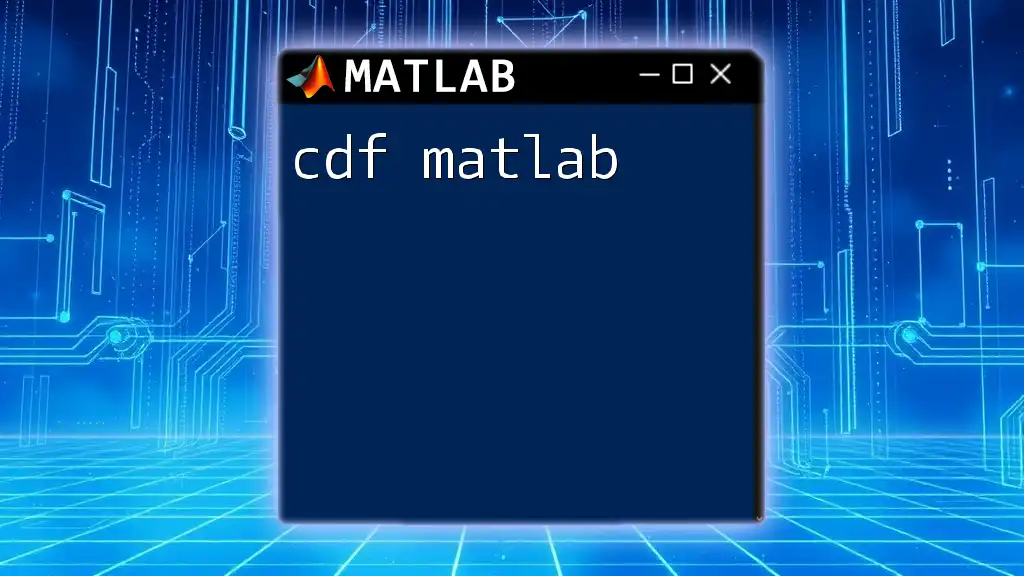
Additional Resources
For deeper dives, refer to MATLAB's official documentation on the cov function. Additionally, consider exploring online courses and textbooks that focus on statistical analysis and MATLAB programming to enhance your understanding of these concepts.