The `c2d` function in MATLAB is used to convert continuous-time system models to discrete-time models using a specified sampling time.
Here's a code snippet demonstrating its use:
% Define a continuous-time transfer function
sys_c = tf([1], [1, 2, 1]);
% Convert the continuous-time system to a discrete-time system with a sampling time of 0.1 seconds
sys_d = c2d(sys_c, 0.1);
Understanding `c2d` Function
Definition
The `c2d` command in MATLAB stands for "Continuous to Discrete." This function is essential for engineers and researchers who work with control systems. It facilitates the transformation of a continuous-time system into a discrete-time system, enabling the implementation and analysis of systems in digital environments, such as microcontrollers and digital signal processors.
Syntax of `c2d`
The basic syntax for the `c2d` function is as follows:
sysd = c2d(sys, Ts, method)
Parameter Explanations:
- `sys`: This represents the continuous-time system you want to convert, which can be defined in various forms, such as transfer functions, state-space models, and zero-pole-gain representations.
- `Ts`: This parameter indicates the sampling time, crucial for determining the discrete time step at which the continuous signal will be sampled.
- `method`: This optional parameter allows you to specify the conversion method, which can significantly affect the resulting discrete-time system.
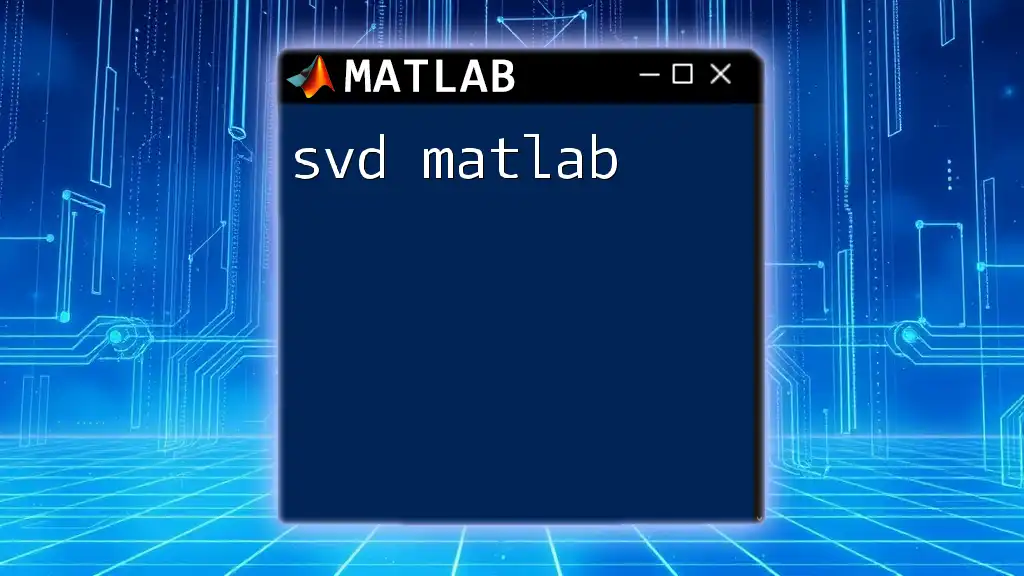
Why Use `c2d`?
Applications in Control Systems
Engineers often need to simulate real-world systems that operate in discrete time, particularly in digital controllers and digital signal processing applications. The ability to convert a continuous system into discrete form allows for easier implementation on digital hardware, making `c2d` an important tool in control engineering.
Benefits of Discrete Models
Using discrete models indicates enhanced computational efficiency. This is particularly true when simulating systems or implementing algorithms that involve repeated calculations where continuous systems would take a toll on processor resources. Furthermore, discrete systems can lead to simplifications in the design and analysis processes and can improve accuracy in specific applications through effective sampling strategies.
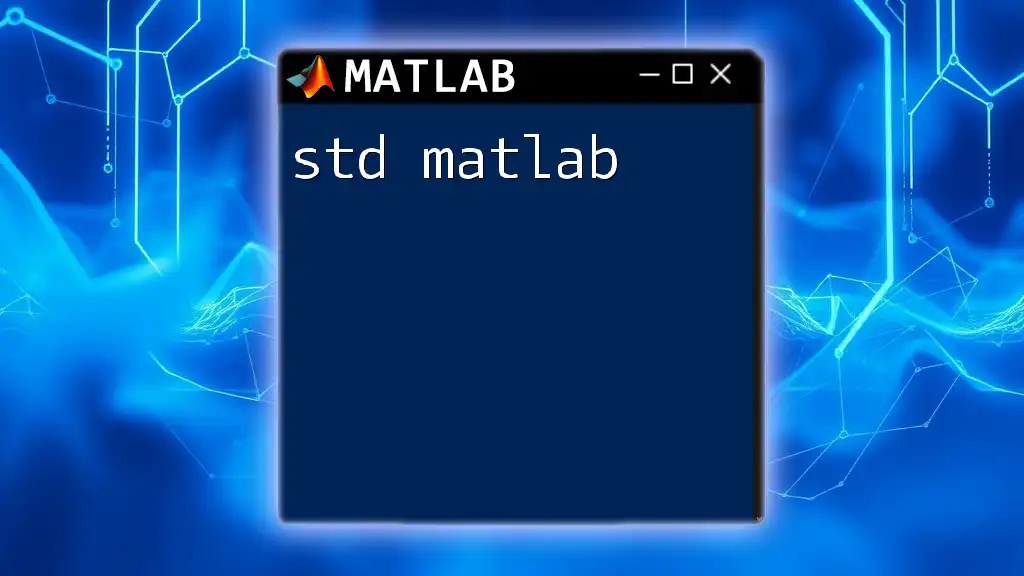
Methods of Discretization in `c2d`
Overview of Discretization Methods
When employing `c2d`, it is vital to understand the common methods of discretization to select the one that best fits your specific needs. Common methods include Zero-Order Hold (ZOH), First-Order Hold (FOH), and Matched Zeros/Poles. Each method has its own applications, advantages, and disadvantages, which will be discussed below.
Zero-Order Hold (ZOH)
The Zero-Order Hold method is the most commonly used technique when discretizing continuous systems. In this approach, it is assumed that the input signal is held constant over each sampling period. When to use ZOH? It is particularly beneficial when your system output is influenced by the continuous input, such as in digital-to-analog converters.
To use ZOH in MATLAB, you can use the following command:
sys = tf([1], [1, 2, 1]); % Define a continuous-time system
Ts = 0.1; % Sampling time
sysd = c2d(sys, Ts, 'zoh'); % Convert using Zero-Order Hold
First-Order Hold (FOH)
The First-Order Hold method is a bit more complex than ZOH. In this method, the output of the continuous system is approximated using linear interpolation between sampled points. This approach is beneficial when trying to maintain a smoother output, especially in high-frequency systems.
To convert a continuous system using FOH, the following code can be used:
sysd = c2d(sys, Ts, 'foh'); % Convert using First-Order Hold
Matched Zeros/Poles Method
The Matched Zeros/Poles method seeks a discrete system that maintains the same poles and zeros as the continuous system. This method is typically used when a more accurate representation of the continuous system is required in the discrete domain.
You can use this command to implement the Matched Zeros/Poles method:
sysd = c2d(sys, Ts, 'matched'); % Convert using Matched Zeros/Poles
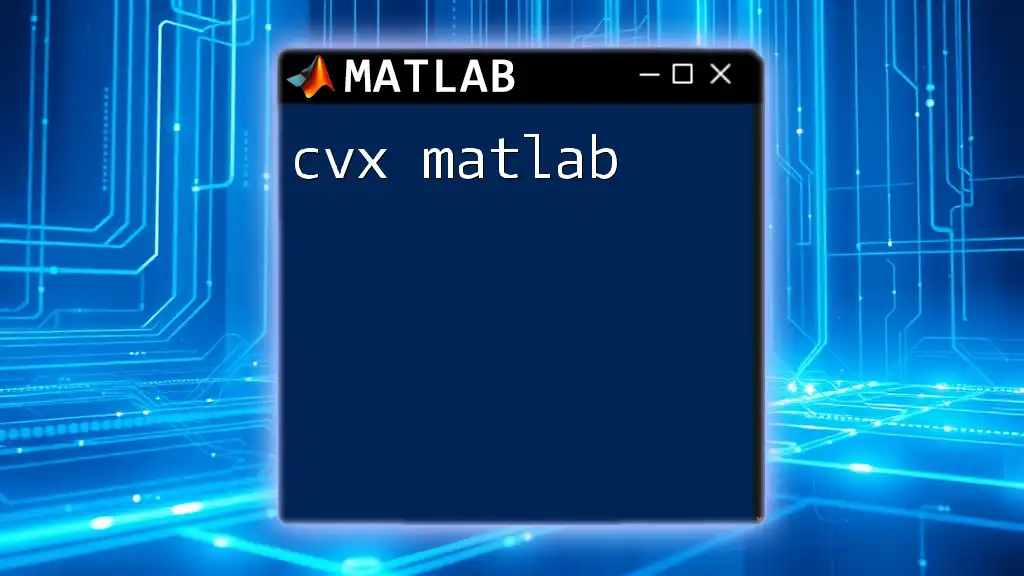
Practical Examples
Example of Continuous to Discrete Conversion
To illustrate the conversion process, let’s define a continuous-time transfer function first:
sys = tf([2], [1, 2, 3]); % Example continuous system
Next, we can convert this continuous system to a discrete one with a specified sampling time. For instance:
Ts = 0.2; % Sampling time
sysd = c2d(sys, Ts, 'zoh'); % Use ZOH method
Analyzing the Resulting Discrete System
Once you have your discrete system, it is essential to analyze and validate its behavior. This can be achieved using MATLAB’s built-in functions. For instance, you can inspect the step response and frequency response of the newly created discrete-time system using the following commands:
step(sysd); % Step response of discrete system
bode(sysd); % Bode plot for frequency analysis
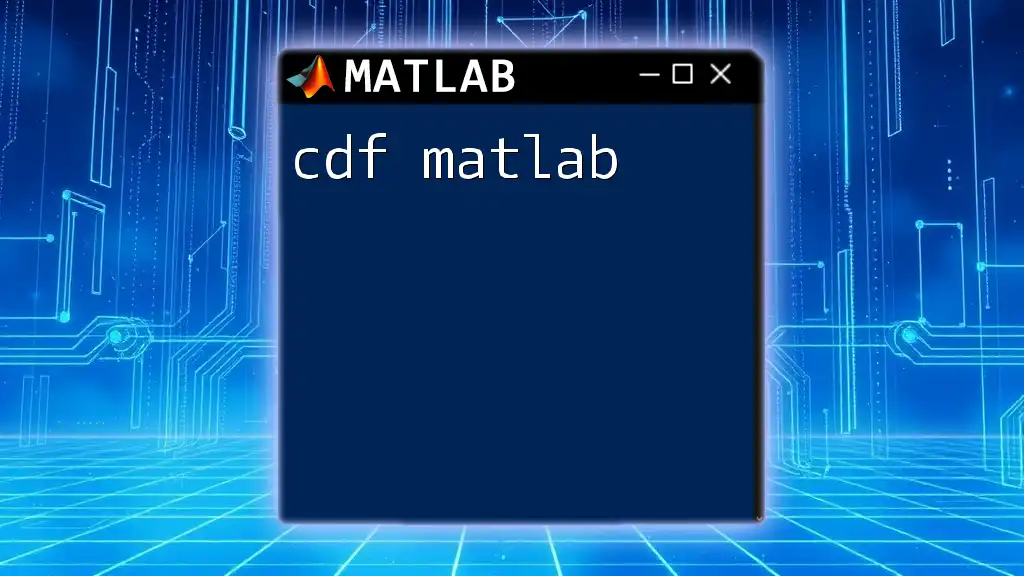
Common Issues and Troubleshooting
Common Errors in Using `c2d`
While using the `c2d` command can significantly ease system modeling, new users often run into potential pitfalls. Common errors include incorrect sampling times, which lead to inaccurate representations of the system, or misaligned input-output dimensions, resulting in conversion failures.
Best Practices
To avoid issues during the conversion process, always choose a sampling rate that meets the Nyquist criterion, which suggests that the sampling frequency should be at least twice that of the highest frequency content of the continuous signal. Moreover, method selection should be guided by the specific application and the characteristics of the system you are working with.
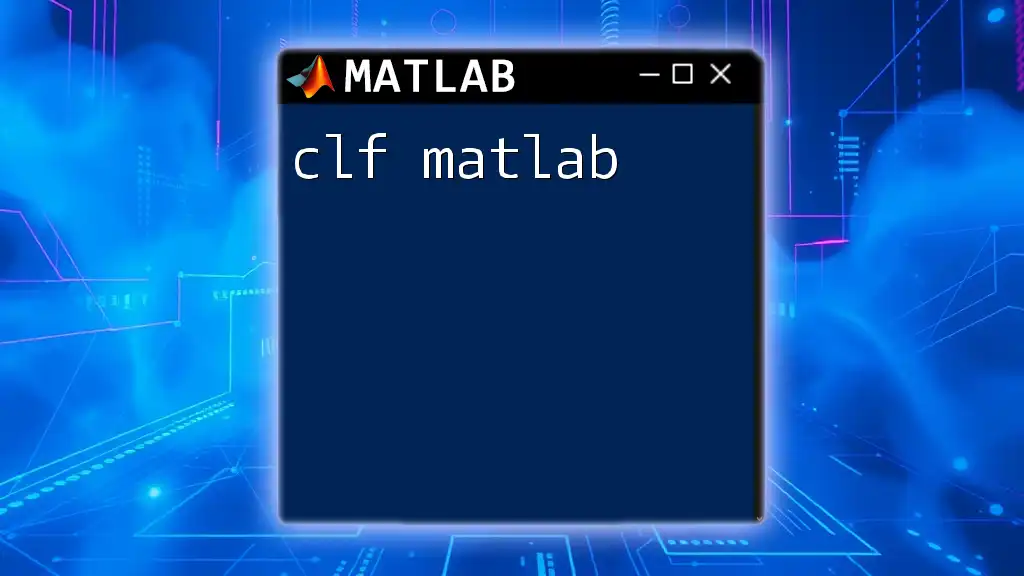
Conclusion
The `c2d` function within MATLAB is a powerful tool that converts continuous systems to discrete forms, essential for modern control solutions. Through understanding its syntactical structure, utilizing appropriate methods, and recognizing common pitfalls, you can effectively harness the capabilities of `c2d`. Feel encouraged to explore the examples provided and experiment with the function on your systems.
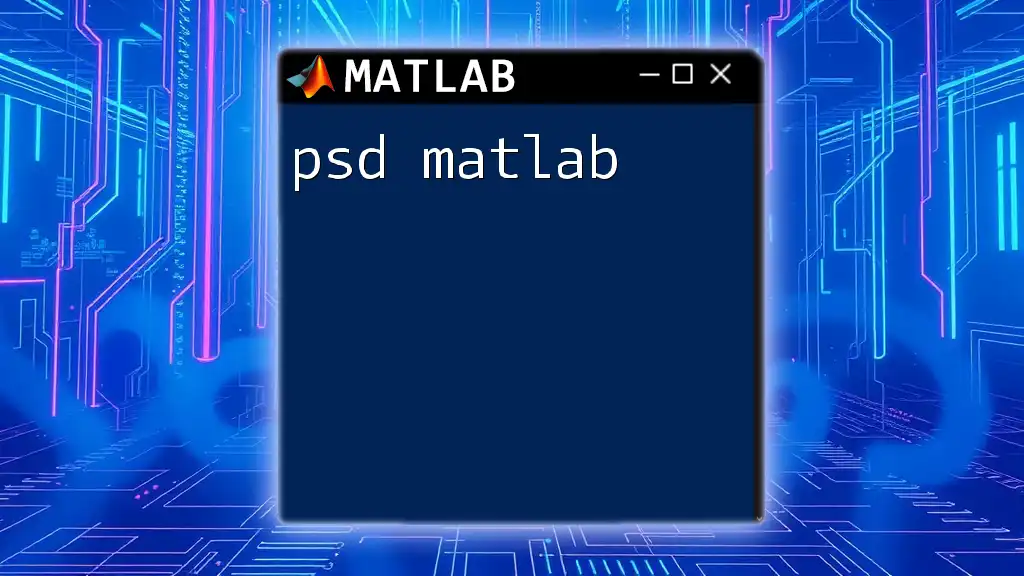
Further Reading and Resources
For those looking to deepen their knowledge beyond the `c2d` command, consider exploring literature focused on control systems and MATLAB programming. Many online resources, including tutorials and forums, can also provide insights and shared experiences that further illuminate the intricacies of control system modeling.
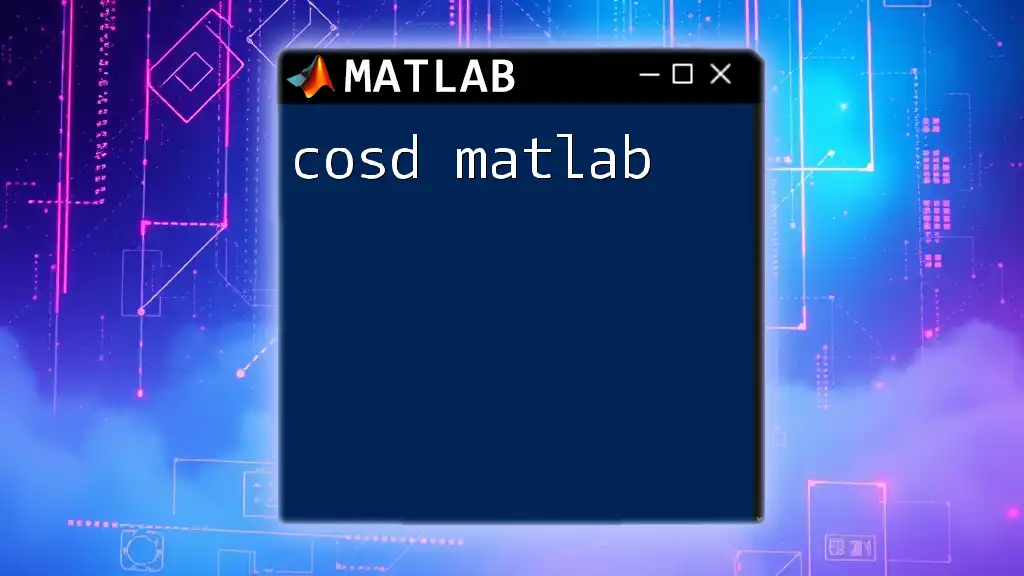
Call to Action
If you found this guide helpful, we invite you to subscribe to our services for more in-depth tutorials and resources focused on mastering essential MATLAB commands like `c2d` and beyond. Stay tuned for our upcoming posts that will cover other important functionalities in MATLAB that can enhance your knowledge and practice!