ANOVA (Analysis of Variance) in MATLAB is a statistical method used to determine whether there are any statistically significant differences between the means of three or more independent groups.
Here’s a simple example of how to perform a one-way ANOVA in MATLAB:
% Sample data
group1 = [23, 21, 22, 24, 25];
group2 = [30, 29, 31, 32, 28];
group3 = [22, 25, 24, 23, 21];
% Combine data into a matrix
data = [group1'; group2'; group3'];
% Perform one-way ANOVA
p = anova1(data);
Understanding ANOVA
What is ANOVA?
ANOVA, or Analysis of Variance, is a statistical method used to determine if there are any statistically significant differences between the means of three or more independent (unrelated) groups. The primary objective of ANOVA is to test for differences between means and to ascertain whether these differences can be attributed to the effects of various independent variables.
ANOVA is widely utilized in diverse fields, including psychology, medicine, and business analytics. For example, researchers might use ANOVA when comparing the effectiveness of different treatments among multiple patient groups.
Types of ANOVA
One-Way ANOVA
One-Way ANOVA tests the impact of a single factor on a dependent variable. It assesses whether there are any statistically significant means differences among the groups formed by the factor's levels.
You would typically use One-Way ANOVA when you want to compare the means of three or more unrelated groups. For example, comparing the test scores of students from different classrooms.
Two-Way ANOVA
Two-Way ANOVA extends the One-Way ANOVA. It examines the influence of two different categorical independent variables on a continuous dependent variable, as well as any interaction effects between the two factors.
This method is particularly useful when exploring complex designs that involve multiple factors. For instance, analyzing the interaction between gender and teaching method on student performance could be assessed using Two-Way ANOVA.
Repeated Measures ANOVA
Repeated Measures ANOVA is applied when the same subjects are measured multiple times under different conditions or over time. This method is essential for detecting differences in means when observations are correlated.
For instance, if you measure a group of patients' blood pressure before and after a treatment, Repeated Measures ANOVA would be the appropriate choice.
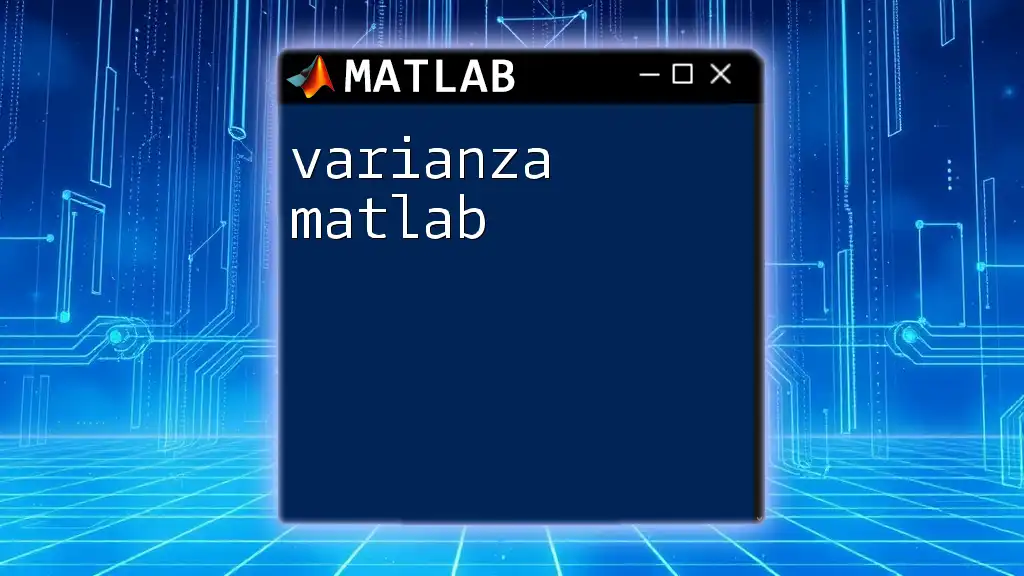
Setting Up MATLAB for ANOVA
Installing MATLAB
To get started with ANOVA in MATLAB, the first step is to ensure that MATLAB is installed on your system. You can download the latest version of MATLAB from the MathWorks official website. For beginners, it’s also helpful to explore MATLAB’s built-in tutorials to get familiar with its programming environment and capabilities.
Importing Data into MATLAB
To conduct ANOVA, you first need to import your dataset into MATLAB. The most common data format for analysis is Excel, which can be imported easily using the `readtable` function.
Here’s a quick example of how to import data:
data = readtable('datafile.xlsx');
In this code snippet, replace `'datafile.xlsx'` with your actual file name. Upon execution, MATLAB reads your data and stores it as a table object that can be manipulated for analysis.
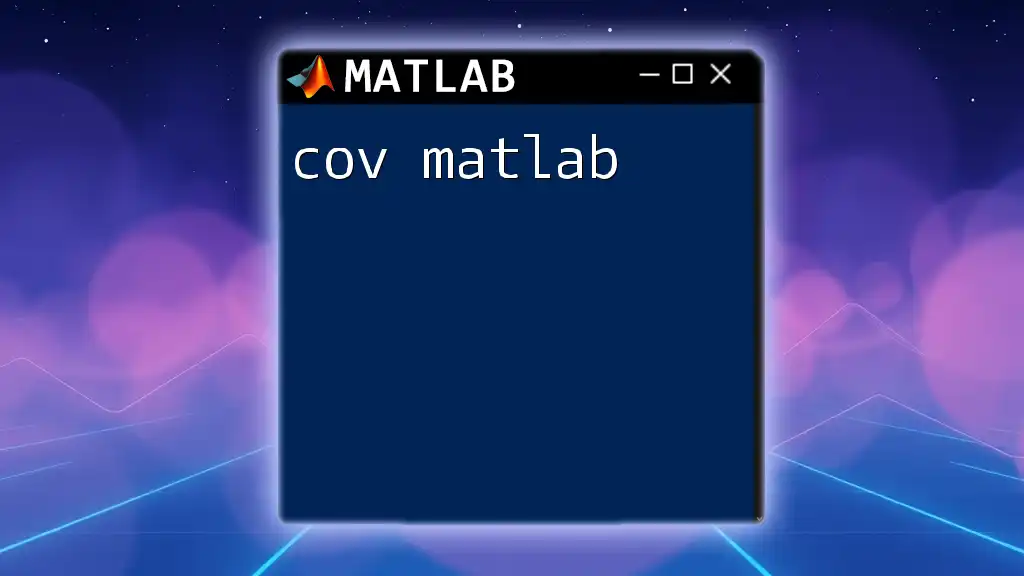
Performing One-Way ANOVA in MATLAB
Basic Steps to Conduct One-Way ANOVA
Conducting One-Way ANOVA in MATLAB requires a specific data structure, where the dependent variable (the outcome you’re measuring) is numeric, and the independent variable (the group factor) is categorical.
It's important to ensure your data is clean and properly organized to avoid any complications during analysis.
Example Use Case
Assume you have a dataset where students from different classrooms are tested, and scores are recorded in a variable called `ResponseVariable`, while a categorical variable called `Group` indicates the classroom. You can perform One-Way ANOVA with the following command:
[p, tbl, stats] = anova1(data.ResponseVariable, data.Group);
Explanation:
- `p` is the p-value that indicates the significance of your ANOVA results.
- `tbl` is the ANOVA table that lists the sources of variation.
- `stats` contains information for post-hoc analysis.
Interpreting Results
When you run the ANOVA model, MATLAB provides an ANOVA table. The key components to look for include the p-value and the F-statistic. A low p-value (typically less than 0.05) suggests that there are significant differences between your group means.
To visualize your results, you can create a box plot:
boxplot(data.ResponseVariable, data.Group);
title('Box Plot of Response Variable by Group');
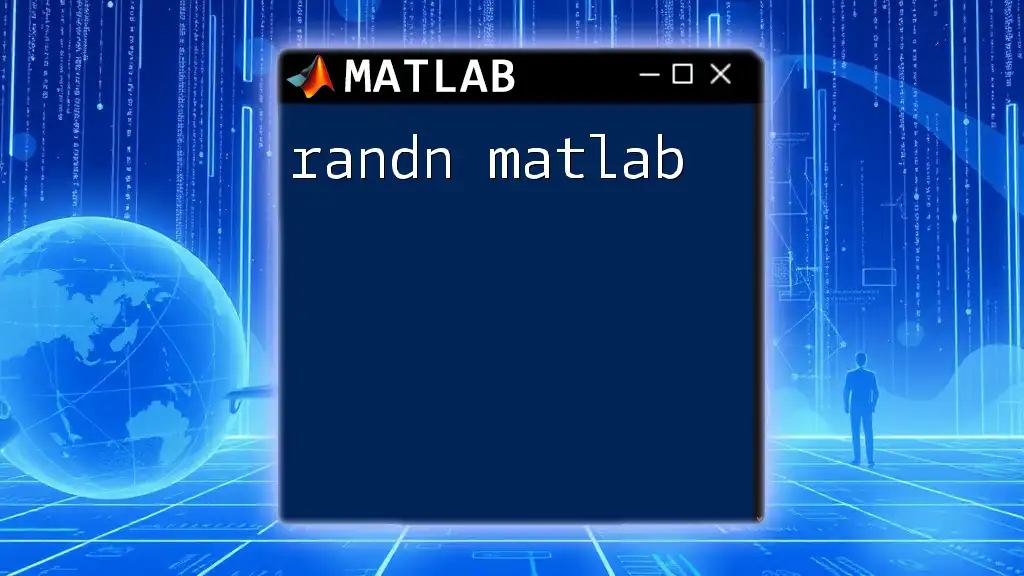
Performing Two-Way ANOVA in MATLAB
Requirements for Two-Way ANOVA
To conduct a Two-Way ANOVA, your data should include two categorical independent variables and one continuous dependent variable. Each combination of the factors will form a unique group for comparison.
Example Use Case
Let’s say you have collected data on student performance that includes two factors: `Gender` and `TeachingMethod`. To perform Two-Way ANOVA in MATLAB, you might set up your command like this:
[p, tbl, stats] = anova2(data.ResponseMatrix, numberOfRows, 'off');
Here, `ResponseMatrix` is a matrix where each row represents a subject and each column represents different conditions or groups.
Visualizing Two-Way ANOVA Results
You can visualize interaction effects between your factors through an interaction plot:
interactionplot(data.ResponseVariable, data.Gender, data.TeachingMethod);
title('Interaction Plot of Factors');
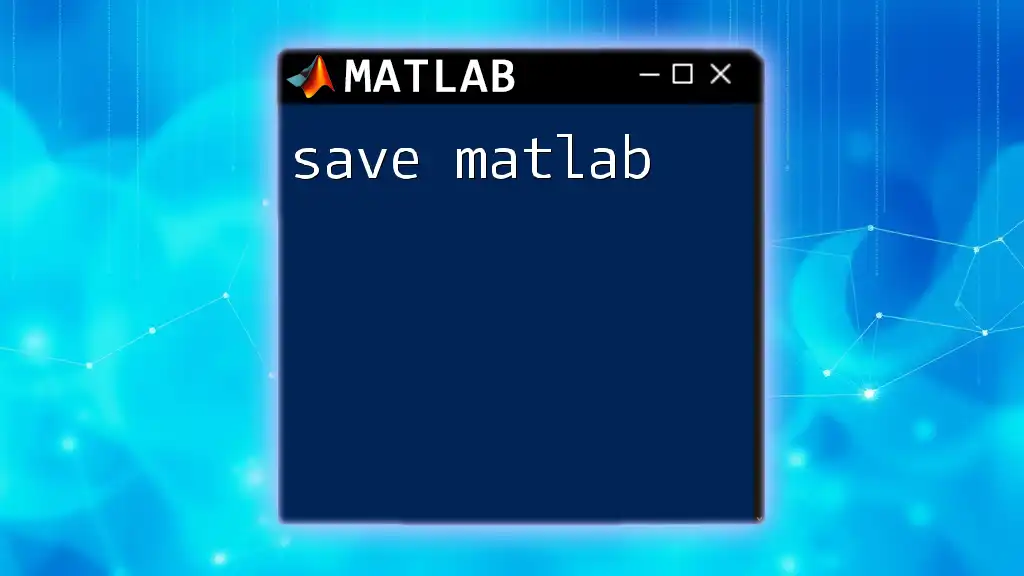
Conducting Repeated Measures ANOVA
Why Use Repeated Measures ANOVA?
Repeated Measures ANOVA is ideal when examining changes over time within the same subjects. This method is critical for studies where individual differences could potentially confound results across multiple conditions.
Example Scenario
Imagine you are measuring a patient’s health parameter at various time points. Your dataset should be structured with each subject's multiple measurements. Using the `fitrm` and `ranova` functions, you can perform the analysis:
rm = fitrm(data, 'ResponseVariable1-ResponseVariable3 ~ 1', 'WithinDesign', designMatrix);
ranova(rm);
Here, `ResponseVariable1-ResponseVariable3` denotes the range of measurements taken.
Analyzing Repeated Measures Results
After executing the analysis, you will receive output describing whether there are significant differences across time points or conditions. Pay attention to p-values for each effect and interaction tested.
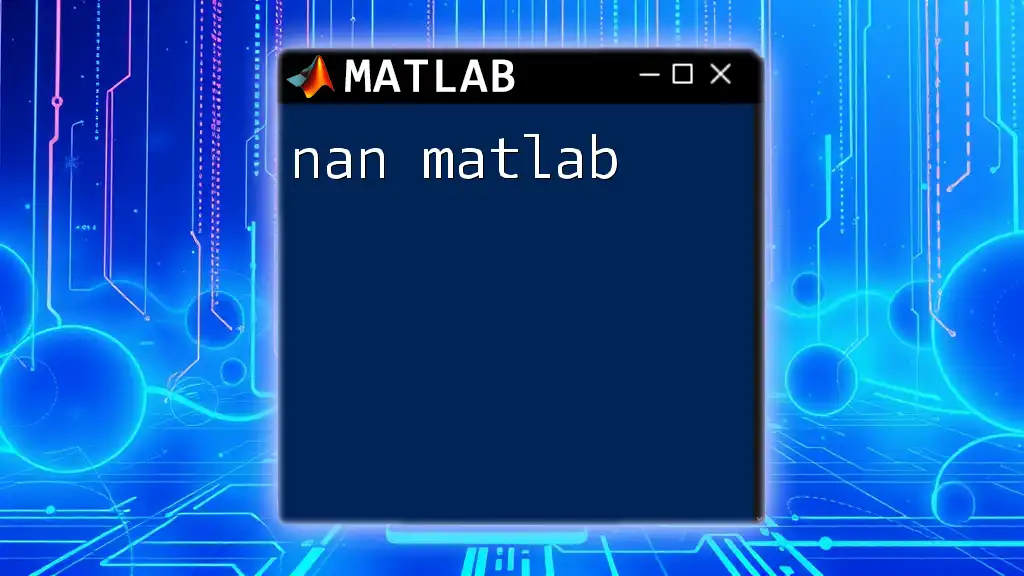
Post-ANOVA Analysis
Multiple Comparisons
After finding significant differences, it may be important to identify which specific groups are different. This is where post-hoc tests come into play, such as Tukey's Honestly Significant Difference (HSD). Execute the following to perform multiple comparisons:
[c, m, h, nms] = multcompare(stats);
Reporting Results
When documenting your findings from ANOVA, it is crucial to clearly communicate your results. Include numerical values, such as means and standard deviations, and graphical representations. An effective report enhances clarity and ensures that your audience can interpret the data accurately.
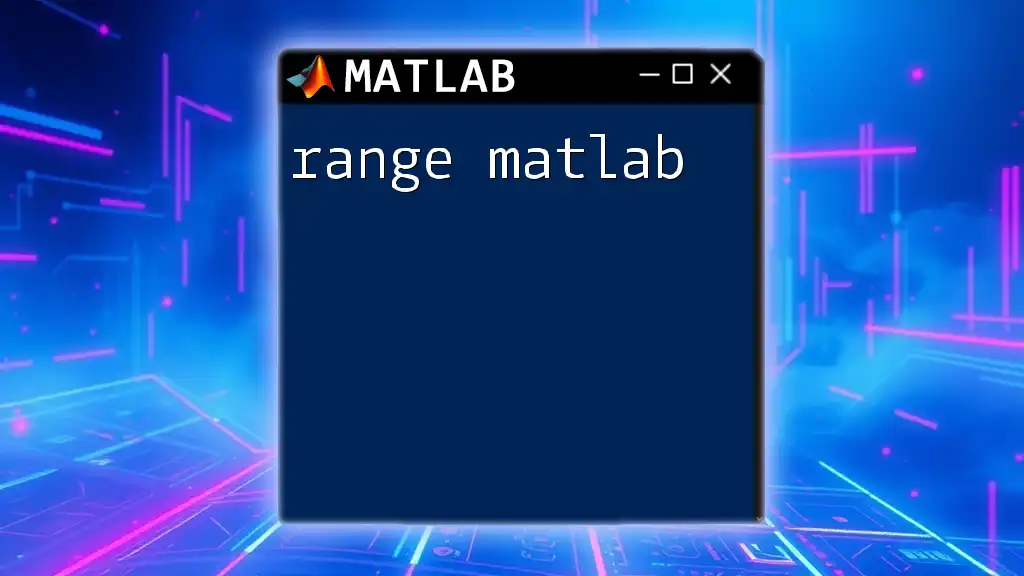
Conclusion
Recap of ANOVA's Importance
ANOVA provides an invaluable tool for researchers and analysts seeking to understand differences among group means. From simple One-Way ANOVA to complex Two-Way and Repeated Measures ANOVA, this technique is essential in making informed statistical decisions.
Resources for Further Learning
For those looking to deepen their understanding of ANOVA in MATLAB, consider exploring online courses or resources available through MathWorks and various educational platforms. Supplementing practical experience with theoretical knowledge can enhance your analytical capabilities significantly.
Encouragement to Practice
To master ANOVA techniques in MATLAB, practice is key. Work with different datasets and scenarios to familiarize yourself with the methods and interpretation of results. Becoming adept at using MATLAB for statistical analyses can greatly enhance your skills and job prospects in data analysis.
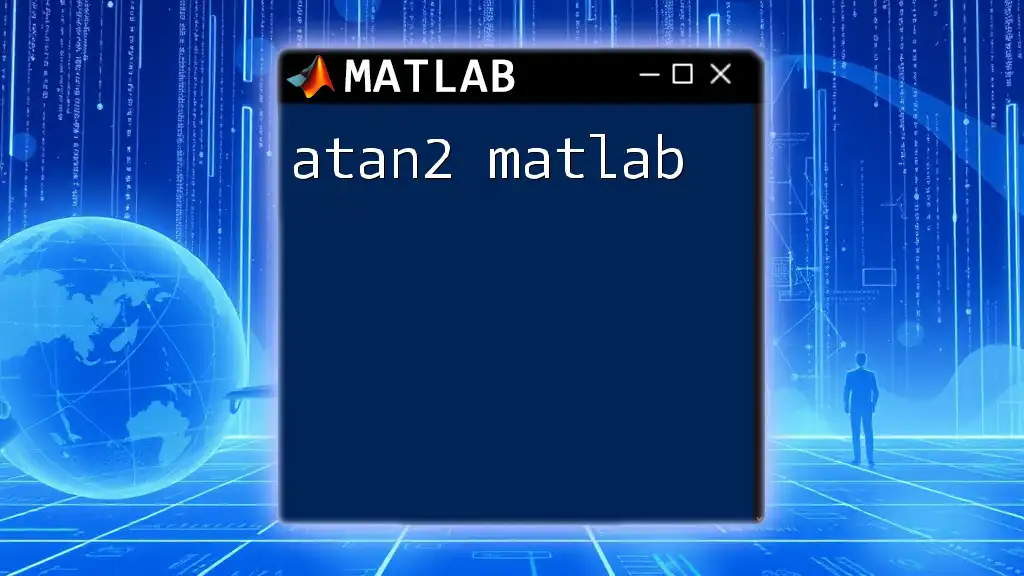
Call to Action
If you're looking for more personalized guidance or wish to enhance your MATLAB skills further, feel free to reach out or enroll in our upcoming courses. We are here to help you on your journey to becoming proficient with MATLAB commands!