The "for" loop in MATLAB is used to execute a group of statements a specific number of times, iterating over a range of values.
Here's a simple example:
for i = 1:5
fprintf('Iteration number: %d\n', i);
end
Understanding the Basics of the "for" Loop in MATLAB
What is a Loop?
In programming, a loop is a fundamental structure that repeats a block of code multiple times based on certain conditions. This repetition allows for tasks that would be tedious and error-prone if performed manually. Loops are essential in programming because they enable automation, which is crucial for handling large datasets, performing repetitive calculations, and simplifying code structure.
Structure of the "for" Loop in MATLAB
In MATLAB, the "for" loop has a specific syntax that makes it straightforward to understand:
for index = startValue:endValue
% Code to execute
end
- index: This variable takes on each value in a range defined by startValue to endValue.
- startValue and endValue: These two values determine the range of the loop. The loop starts at startValue and terminates after reaching endValue.
This concise syntax makes it easy to iterate over a sequence of values.
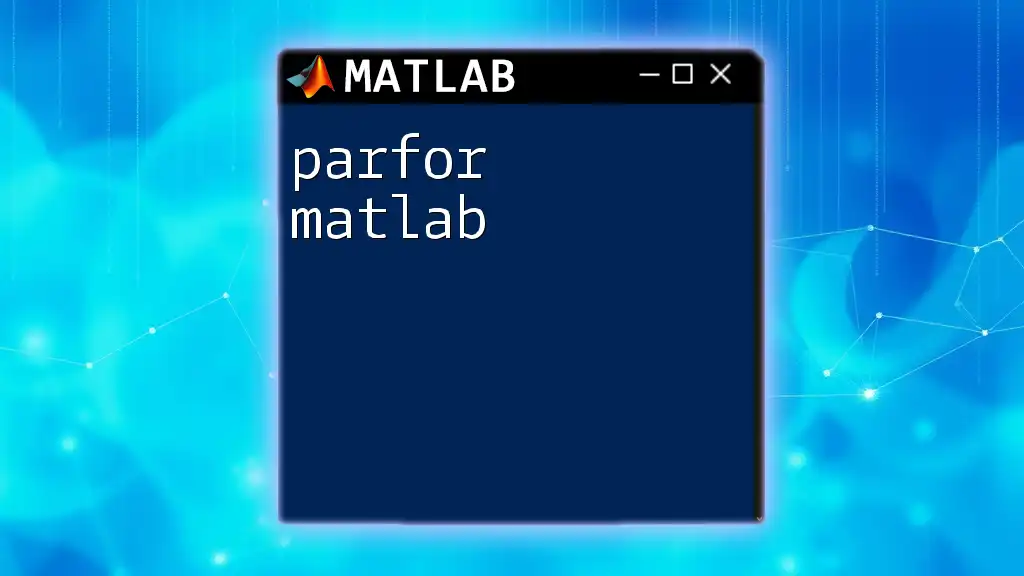
How the "for" Loop Works in MATLAB
Iteration Explained
During each iteration of a "for" loop, the code inside the loop executes for the current value of the index. For example, consider the following code snippet:
for i = 1:5
disp(i)
end
This loop executes five times, displaying the numbers 1 through 5 in order. With each iteration, the variable `i` takes on a new value, allowing for dynamic operations based on the current index.
Using Arrays with the "for" Loop
Loops become even more powerful when combined with arrays. You can traverse each element of an array and perform computations. Here’s an example where we sum the elements of an array:
arr = [1, 2, 3, 4, 5];
sum = 0;
for i = 1:length(arr)
sum = sum + arr(i);
end
disp(sum)
In this code, we use `length(arr)` to determine how many times to iterate, ensuring that we process each element. The total sum of the array elements (in this case 15) is calculated and displayed.
Nested "for" Loops
Nested loops allow more complex iteration structures, such as performing operations on two arrays or creating matrices. A common usage is generating a multiplication table. Below is an example of nested "for" loops:
for i = 1:5
for j = 1:5
result = i * j;
fprintf('%d x %d = %d\n', i, j, result);
end
end
Here, the outer loop iterates through values 1 to 5, while the inner loop does the same, effectively multiplying each pair of values together and displaying the output in a formatted way. This approach demonstrates how to generate tabulated data efficiently.
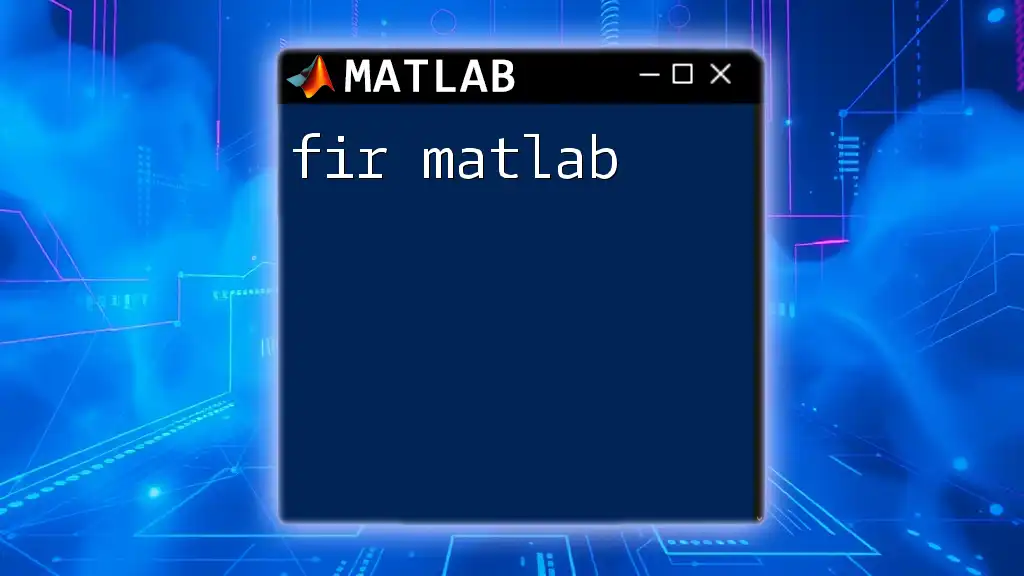
Advanced Use-Cases for the "for" Loop
Conditional Statements within a "for" Loop
You can also integrate conditional logic within a "for" loop using if-else statements. This allows for dynamic behavior based on the conditions met during loop iteration. For example, check if a number is even or odd:
for i = 1:10
if mod(i, 2) == 0
fprintf('%d is even\n', i);
else
fprintf('%d is odd\n', i);
end
end
This loop will evaluate each number from 1 to 10, providing a simple yet effective demonstration of how to use conditions to govern the flow of your loop logic.
Using "for" Loops with Functions
You can encapsulate a "for" loop within a function to promote code reusability. Here's how to define a function that calculates and displays the square of each integer up to a specified number:
function squareValues(n)
for i = 1:n
fprintf('The square of %d is %d\n', i, i^2);
end
end
This modular approach separates the logic of squaring numbers from the rest of your code, making it cleaner and easier to manage. You simply call `squareValues(5)` to execute the loop.
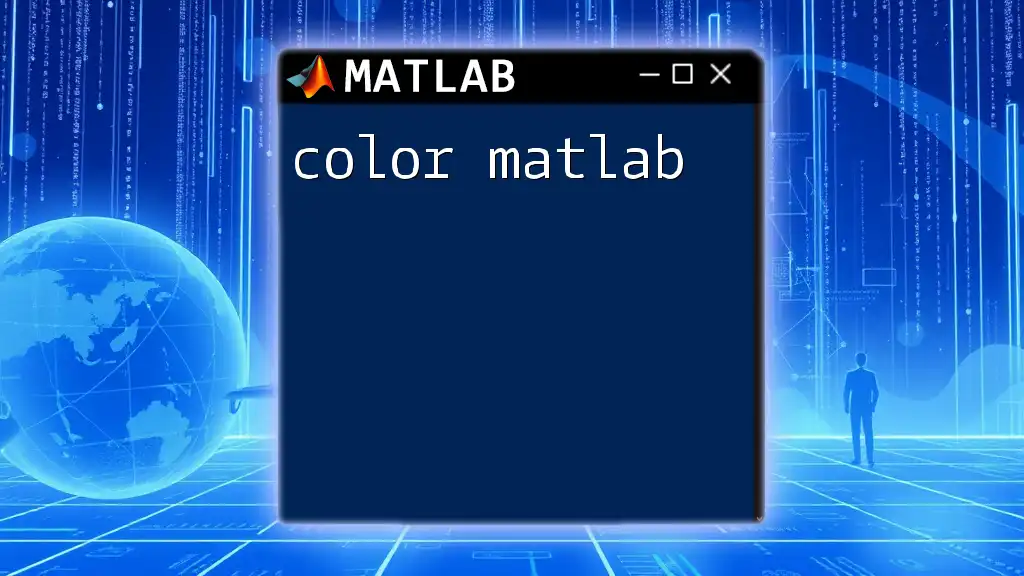
Best Practices for Using "for" Loops
Keep Code Readable
Writing clear and concise code is essential for maintainability and efficiency. Use meaningful variable names instead of single letters, and always include comments to explain your intent. For instance, instead of using `i`, `j`, or `k`, you might use `row` or `column` if the context requires it.
Avoid Unnecessary Computations
Optimize "for" loops to prevent performance issues. Ensure that computations inside a loop are necessary and not repetitive without need. For example, if a condition doesn't change within the loop, calculate it outside before the loop starts.
threshold = 10; % Example threshold check outside the loop
for i = 1:100
if i > threshold
% Do something useful
end
end
Choose Appropriate Data Structures
Understanding when to use arrays, matrices, or cell arrays can significantly affect performance. Use arrays when you need homogenous data types and matrices for mathematical operations. Cell arrays allow for different data types but may require more processing time.
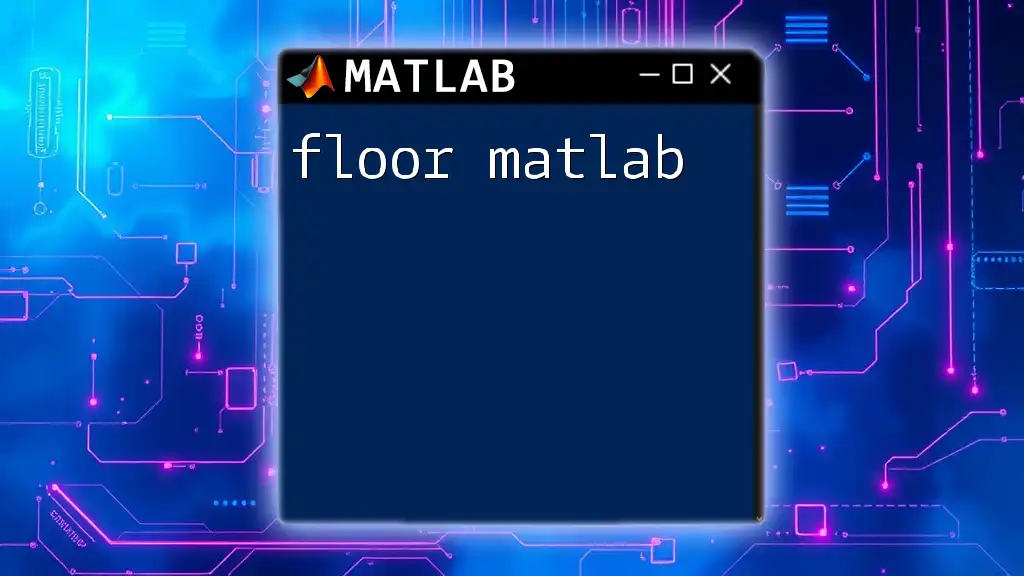
Common Errors and Troubleshooting
Syntax Errors
Syntax errors often occur when a "for" loop is misconfigured. Understanding error messages and their context can help identify these mistakes quickly. Here's a typical syntax error example:
for i = 1:5
disp(i;
end
The error is caused by missing the closing parenthesis, which MATLAB will highlight in the command window.
Infinite Loops
An infinite loop occurs when the loop condition never evaluates to false. This can disrupt the execution of your program. For example, if you forget to increment the loop variable:
for i = 1:10
% Missing increment step
end
This loop will run indefinitely, causing your program to hang. Always make sure your loop variable changes within the loop or ensure that conditions lead to proper termination.
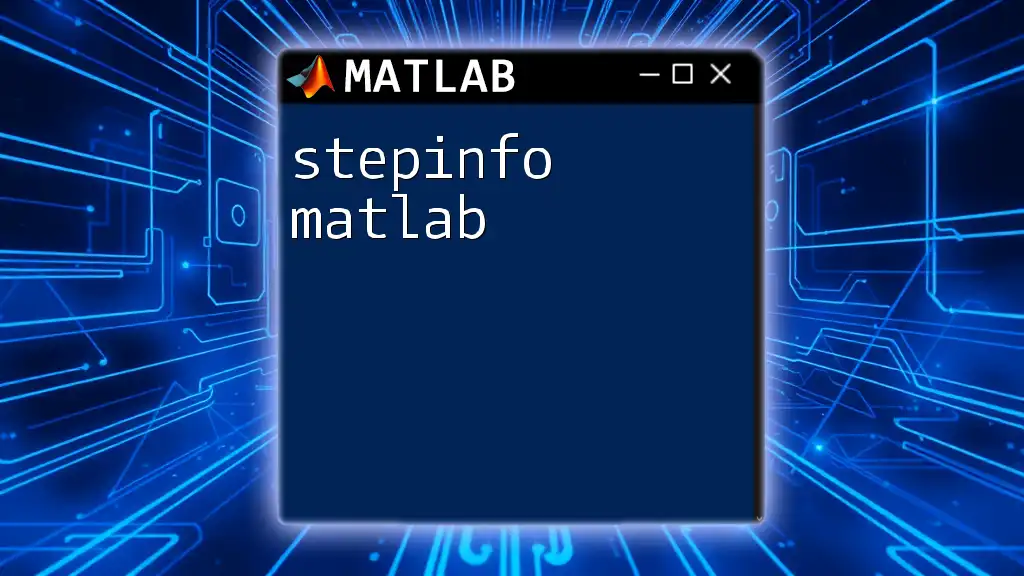
Conclusion
The "for" loop in MATLAB is a powerful and versatile tool that can significantly enhance your programming capabilities. It automates repetitive tasks, making your code not only shorter but also more efficient. Practicing with "for" loops in real-world projects will deepen your understanding and mastery of MATLAB programming.
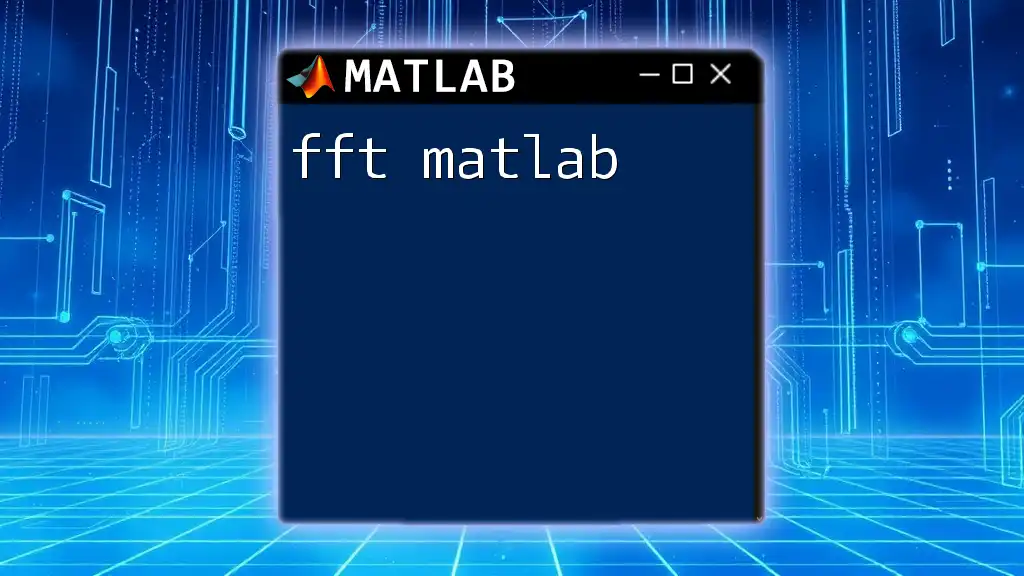
Call to Action
If you’re eager to further develop your MATLAB skills, consider joining our MATLAB workshop or participating in our online tutorials. We offer a range of resources for enthusiasts from beginners to advanced users. Stay updated with the latest trends in MATLAB programming for continuous improvement!