The `corr` function in MATLAB computes the correlation coefficient between two datasets, helping you measure the strength and direction of their linear relationship.
% Example: Calculate the correlation coefficient between two vectors x and y
x = [1, 2, 3, 4, 5];
y = [2, 3, 4, 5, 6];
r = corr(x', y'); % Note: transpose vectors to make them column vectors
disp(r); % Display the correlation coefficient
Understanding Correlation
What is Correlation?
Correlation is a statistical measure that describes the extent to which two variables are related. It is typically quantified using a correlation coefficient, which can range from -1 to +1. A coefficient close to 1 indicates a strong positive correlation—meaning as one variable increases, the other variable tends to increase as well. Conversely, a coefficient close to -1 indicates a strong negative correlation—where an increase in one variable leads to a decrease in the other variable. A coefficient around 0 indicates no correlation.
Importance of Correlation in Data Analysis
Correlation plays a crucial role in data analysis across various fields, such as finance, where it helps analysts understand the relationship between asset prices; in science, where it can uncover relationships between biological factors; and in engineering, where it aids in quality control processes. Understanding the correlation between variables can significantly improve decision-making, predictive modeling, and statistical analysis.
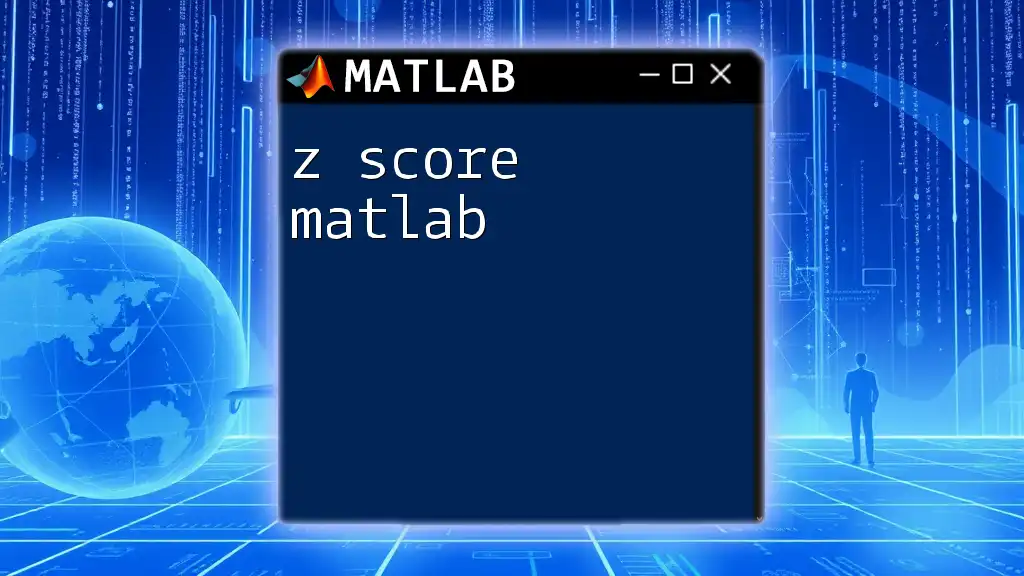
Introduction to the `corr` Function in MATLAB
What is the `corr` Function?
In MATLAB, the `corr` function is a powerful tool used to compute correlation coefficients between pairs of data. It can be applied to matrices, where each row represents an observation and each column represents a variable. The `corr` function facilitates the analysis of relationships between multiple variables efficiently.
Syntax of the `corr` Function
The basic syntax of the `corr` function is:
R = corr(X)
- X: The input matrix. If X is an m-by-n matrix, R will be an n-by-n matrix containing correlation coefficients between each pair of variables.
There are additional optional arguments to specify how to calculate the correlation and handle missing data.
Example of Basic Syntax
To calculate a correlation matrix from random data, you might use the following code:
A = rand(100, 3); % Generates a 100x3 matrix of random numbers
R = corr(A); % Computes the correlation coefficients
In this example, R will contain the correlation coefficients for the three columns of the matrix A.
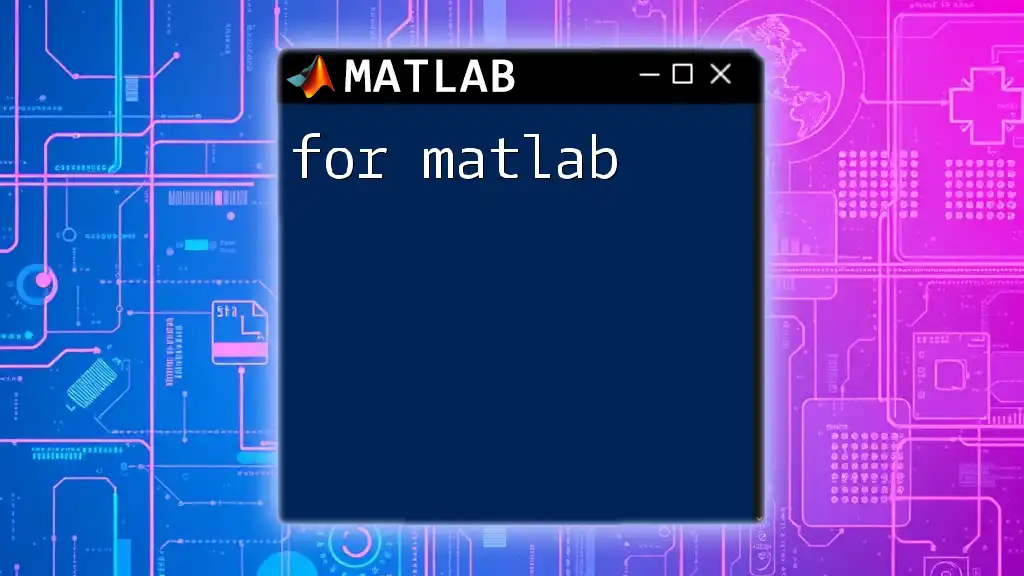
Types of Correlation Coefficients
Pearson Correlation Coefficient
The Pearson correlation coefficient measures the linear relationship between two continuous variables. It is the most widely used correlation coefficient. The formula for Pearson correlation uses covariance, and can be computed as follows:
R = corr(A, 'Rows', 'pairwise', 'type', 'Pearson');
This code computes the Pearson correlation matrix for the matrix A while allowing for pairwise deletion of missing data.
Spearman's Rank Correlation Coefficient
Spearman's rank correlation assesses how well the relationship between two variables can be described using a monotonic function. This can be particularly useful when the data is not normally distributed. To calculate it in MATLAB, you can use:
R = corr(A, 'type', 'Spearman');
Unlike Pearson, Spearman's coefficient considers the ranks of the data rather than their actual values, making it resilient to outliers and non-parametric.
Kendall’s Tau
Kendall’s Tau is another measure that assesses the strength of association between two variables. It works by counting the number of concordant and discordant pairs. To perform this calculation in MATLAB, you can use:
R = corr(A, 'type', 'Kendall');
Kendall’s Tau is often preferred in ordinal data analysis due to its robustness against non-normal distributions.
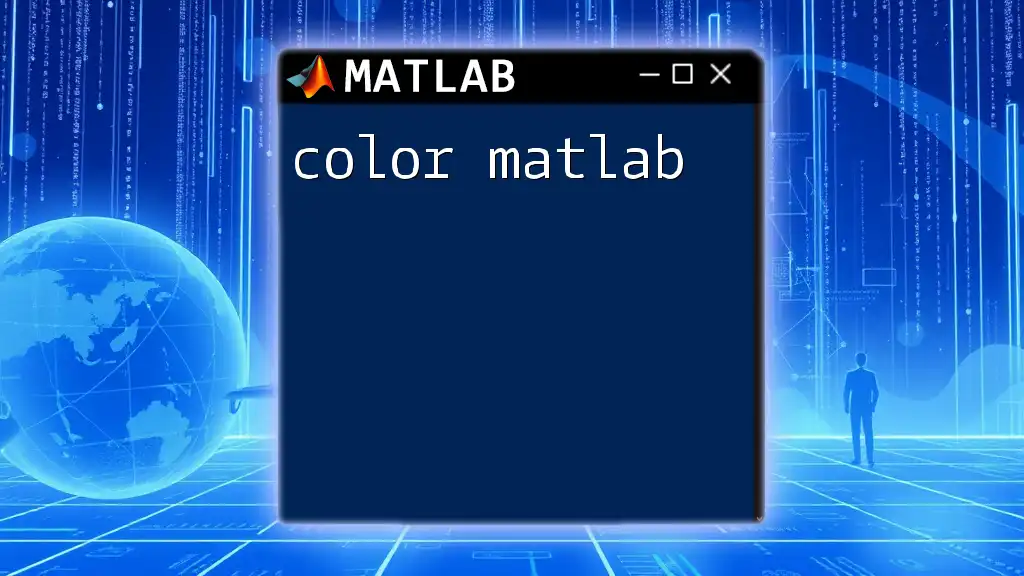
How to Handle Missing Data
Understanding Missing Data in MATLAB
In real-world applications, datasets often contain missing values represented by NaN (Not a Number). The presence of missing data can skew correlation results, making it crucial to address this before performing any analysis.
Using the `Rows` Argument
The Rows argument in the `corr` function allows you to specify how to handle missing data.
- 'all': Default option that uses all data, leading to the risk of skewed results if any missing values exist.
- 'complete': Uses only complete pairs of data, discarding any rows that contain NaN values.
- 'pairwise': Allows pairwise deletion, which calculates correlation using only the available data pairs for computation.
Here’s how you can implement this:
R = corr(A, 'Rows', 'pairwise');
This snippet will compute the correlation matrix, using available pairs of data and ignoring rows with any missing values.
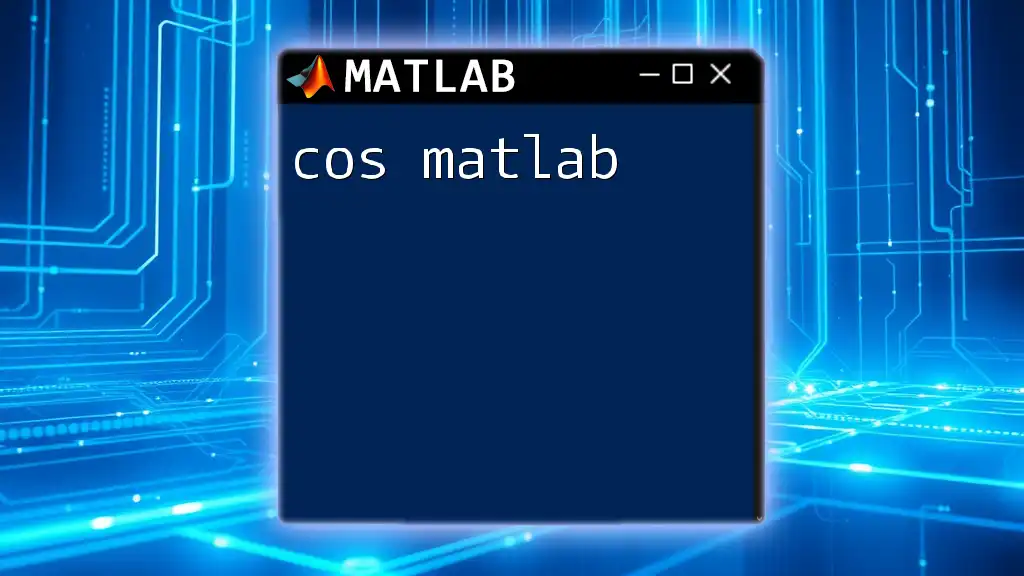
Visualizing Correlation
Plotting Correlation Matrix
Visualizing correlation data can significantly aid in interpreting results. A heatmap is one effective way to visualize a correlation matrix. For example:
imagesc(R);
colorbar;
title('Correlation Heatmap');
This code snippet generates a heatmap of the correlation matrix R and adds a color bar for reference.
Scatter Plot to Visualize Relationships
Creating scatter plots can also help in understanding relationships between individual pairs of variables. For instance:
scatter(A(:,1), A(:,2));
xlabel('Variable 1');
ylabel('Variable 2');
title('Scatter Plot of Variable 1 vs. Variable 2');
This graph illustrates the relationship between the first two variables, allowing you to visually assess the strength and direction of their correlation.
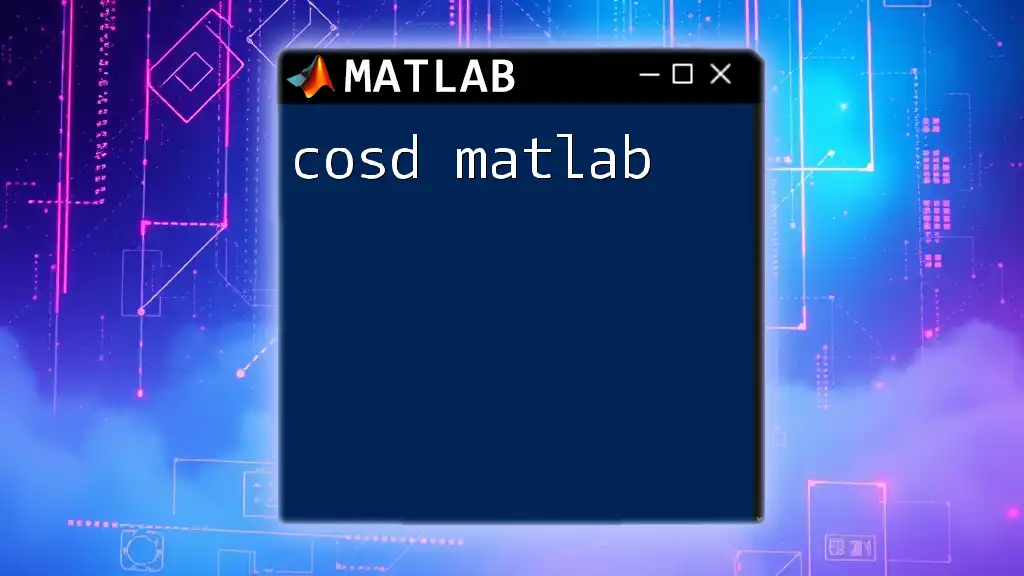
Advanced Uses of the `corr` Function
Correlating with Conditionals
You can enhance your analysis by applying logical indexing to filter your data before computing correlation. For example:
filtered_A = A(A(:,3) > 0.5, :); % Filters rows where the third variable is greater than 0.5
R_filtered = corr(filtered_A);
This code computes the correlation matrix only for those observations where the third variable satisfies the filtering condition.
Correlation in Time Series Data
In time series analysis, you might want to analyze the correlation between lagged versions of a dataset. For example, you can compare current values with their previous values:
R_lagged = corr(A(1:end-1,:), A(2:end,:));
This snippet shifts the data to compute correlations between current and previous observations, providing insights into time-dependent relationships.
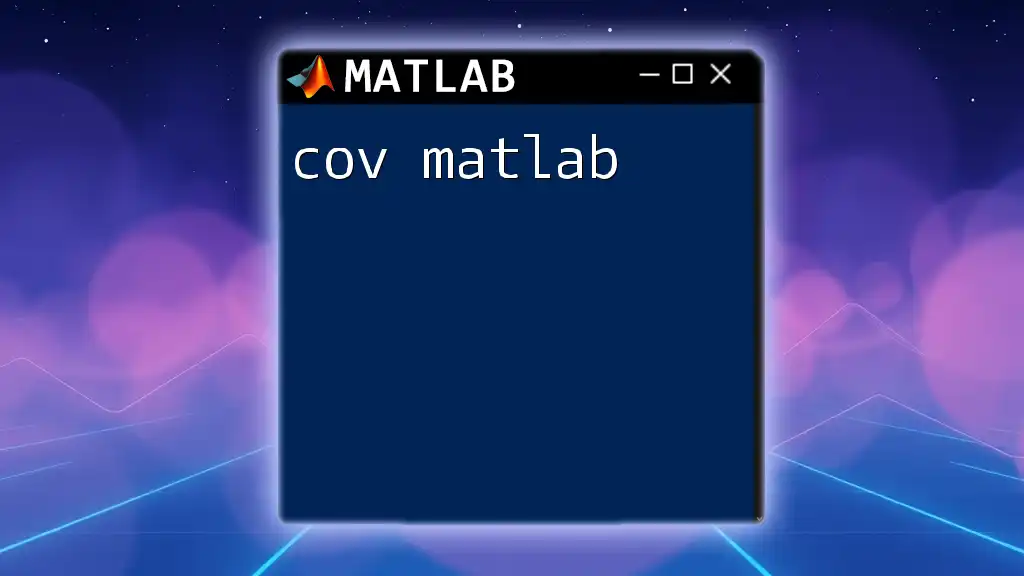
Common Pitfalls and Troubleshooting
Misinterpreting Correlation
It is crucial to remember that correlation does not imply causation. A high correlation between two variables does not indicate that one variable causes changes in the other. Misinterpreting correlation is a common pitfall, especially in fields like finance and healthcare, where spurious relationships can lead to incorrect conclusions.
Errors and Debugging `corr` in MATLAB
Some common errors you might encounter when using the `corr` function in MATLAB include dimensional mismatches—where your matrix does not conform to include the right number of observations. If you see an error message, double-check your data dimensions to ensure they are compatible with correlation analysis.
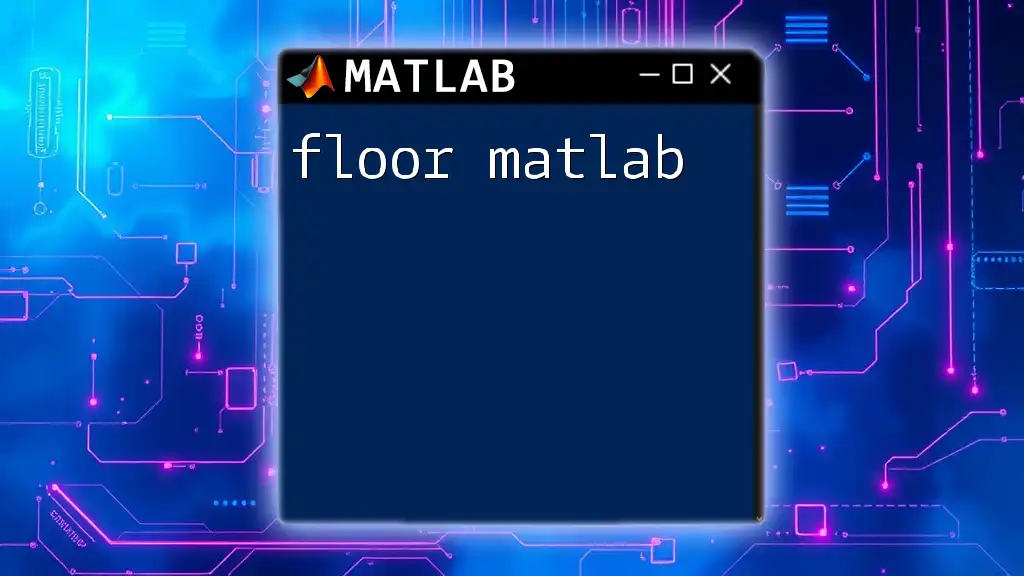
Conclusion
The `corr` function in MATLAB is an essential tool for exploring the relationships between variables in your data. By understanding the various types of correlation coefficients and applying the function correctly, you can reveal significant insights in your analysis, from basic statistics to complex data interactions. Additionally, remember to visualize your results for better interpretation and avoid misinterpretations that could lead to incorrect conclusions. The analysis of correlation is a gateway to deeper statistical insights, enabling smarter, data-driven decisions.