In MATLAB, you can define the length of an array using the `length` function, which returns the number of elements in the largest array dimension.
Here’s the code snippet:
array = [1, 2, 3, 4, 5]; % Sample array
len = length(array); % Determine the length of the array
disp(len); % Display the length
Understanding Array Length in MATLAB
What is an Array?
In MATLAB, an array is a structured collection of data elements that can be accessed using indices. Arrays come in various types, including 1D arrays (vectors), 2D arrays (matrices), and even higher-dimensional arrays. Understanding how to manipulate and analyze arrays is fundamental to efficiently solving mathematical problems and managing data.
Why is Array Length Important?
Knowing how to define the length of an array in MATLAB is essential for a variety of reasons:
- Memory Management: Accurate knowledge of array size can help optimize memory usage during program execution.
- Algorithm Efficiency: Many algorithms rely on array dimensions; failure to utilize the correct length can lead to inefficiencies or errors in code.
- Use Cases: Length is significant in mathematical computations, statistical analysis, and visualizations.
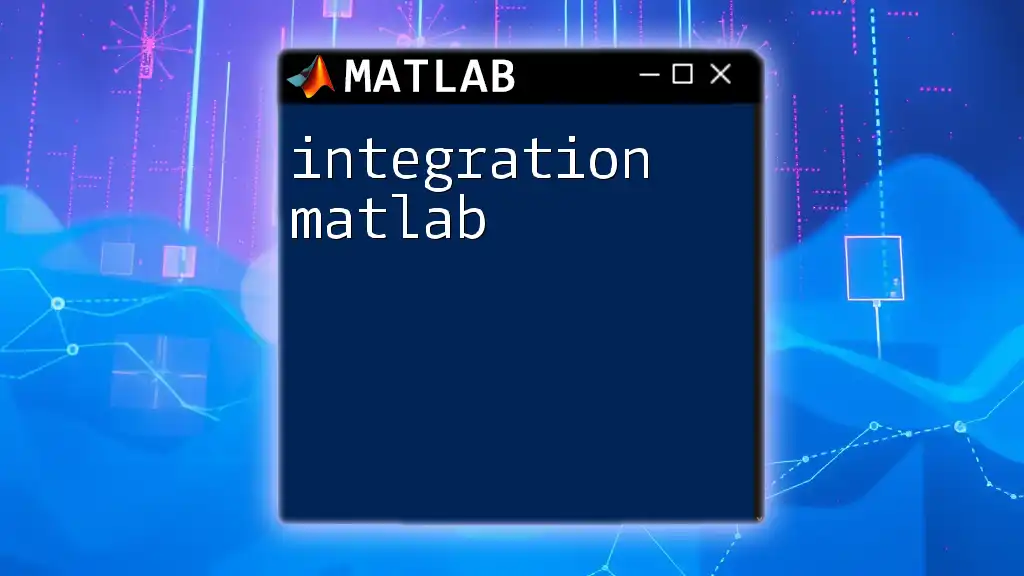
Methods to Determine Array Length in MATLAB
Using the `length` Function
MATLAB provides a built-in function, `length`, which is one of the easiest ways to find the length of an array.
Syntax:
L = length(A)
Example:
A = [1, 2, 3, 4, 5];
L = length(A); % L will be 5
In this case, `length` will return 5 because the array A has five elements. The `length` function computes the largest dimension of the array. For a 1D array, it directly returns the number of elements, while for a 2D array, it returns the size of the largest dimension.
Using the `size` Function
The `size` function offers a more detailed approach by returning both the number of rows and columns in an array.
Syntax:
[rows, cols] = size(A)
Example:
B = [1, 2, 3; 4, 5, 6];
[rows, cols] = size(B); % rows will be 2, cols will be 3
For array B, `size` will tell us it has 2 rows and 3 columns. This function is particularly useful when working with matrices where knowing both dimensions matters.
Using the `numel` Function
The `numel` function is another valuable function in MATLAB that provides the total number of elements in an array, regardless of its dimensions.
Syntax:
N = numel(A)
Example:
C = [1, 2; 3, 4; 5, 6];
N = numel(C); % N will be 6
Here, `numel` for array C will return 6, indicating that there are six individual elements in the array. Use `numel` when you need to know the total number of elements, not just the length along one dimension.
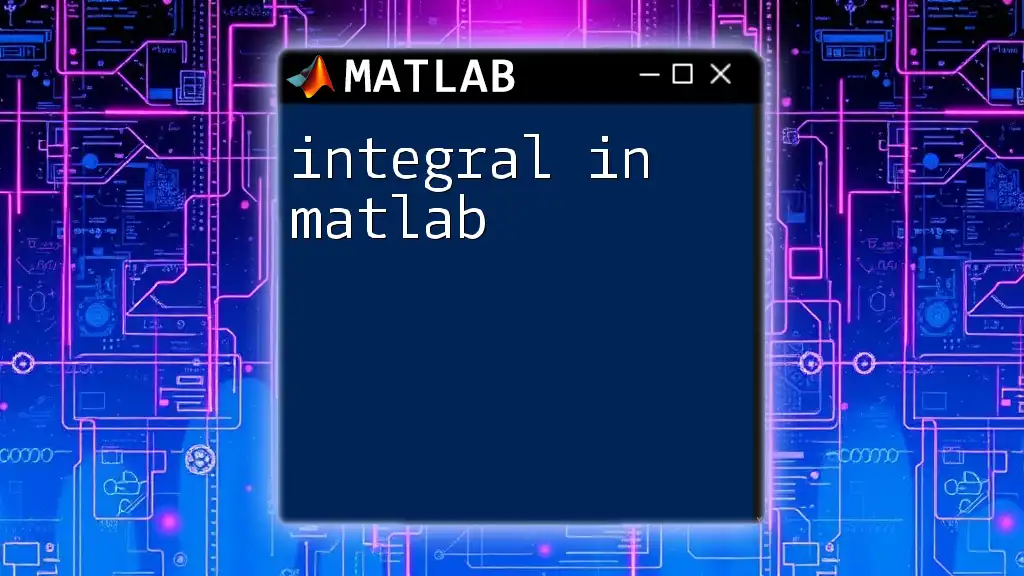
Visually Understanding Array Dimensions
Graphical Representation of Arrays
Visualizing the structure of your arrays can significantly improve your understanding of the data you are dealing with and the way functions apply to them. Visual representations highlight differences in dimensionality, helping to clarify concepts like length and size.
Practice Code for Understanding Dimensions
One practical way to gain insight into how MATLAB handles arrays is to visualize 1D and 2D arrays. Here is some code to help facilitate that understanding.
figure;
subplot(1, 2, 1);
histogram(A); % 1D visualization
subplot(1, 2, 2);
imagesc(B); % 2D visualization
The first subplot displays a histogram of the 1D array A, illustrating its elements. The second subplot employs the `imagesc` function to visualize the 2D array B, allowing analysts to see the data structure in a more intuitive format.
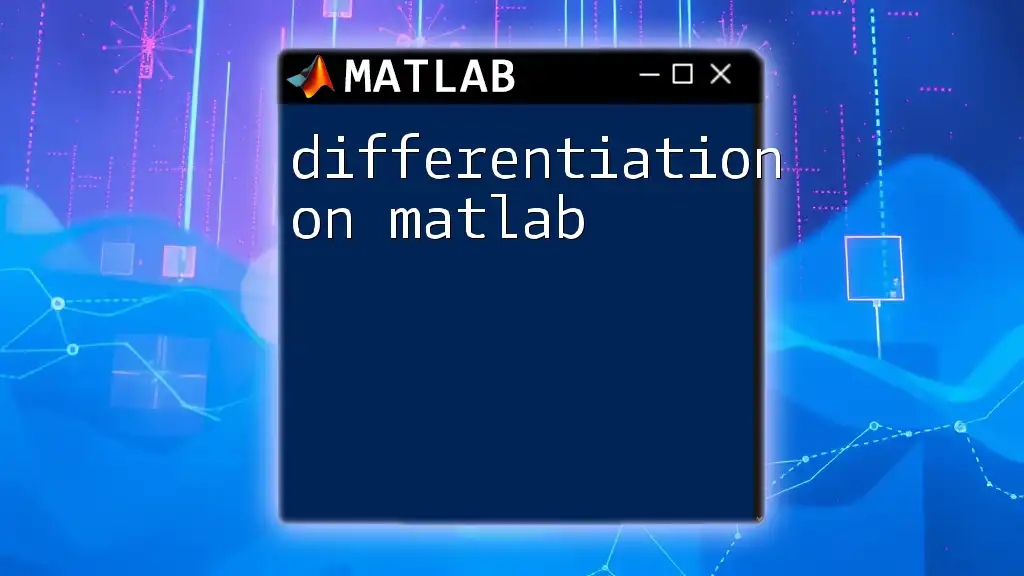
Common Pitfalls and Troubleshooting
Misconceptions About Array Length
Many programmers, especially those new to MATLAB, misunderstand how length should be utilized. A common misconception is equating length to the total number of elements in all dimensions, which is incorrect. `length` specifically provides a measure based on the largest dimension.
Troubleshooting Tips
Sometimes, it may appear that an array has no elements, yet it still shows dimensions. For instance, an array initialized as follows:
D = [];
lengthD = length(D); % lengthD will be 0
If `D` is empty—in this case—the `length` function returns 0. Always check for emptiness using the `isempty` function before applying length-related functions to avoid confusion.
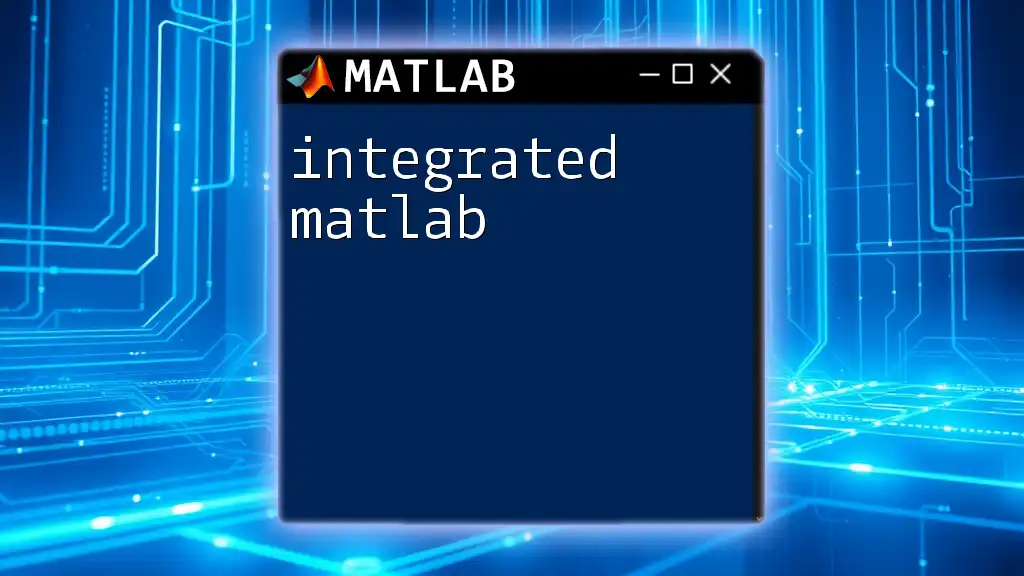
Conclusion
Recap of Key Points
Understanding how to effectively define the length of an array in MATLAB is crucial for any data analysis task. The three core functions—`length`, `size`, and `numel`—each serve unique purposes and provide flexibility depending on your needs. Familiarity with these concepts will improve your programming efficiency and accuracy.
Call to Action
I encourage you to practice these functions and experiment with various types of arrays. For a deeper dive into MATLAB's functionality and techniques, consider checking out our concise tutorials that will facilitate your learning journey.
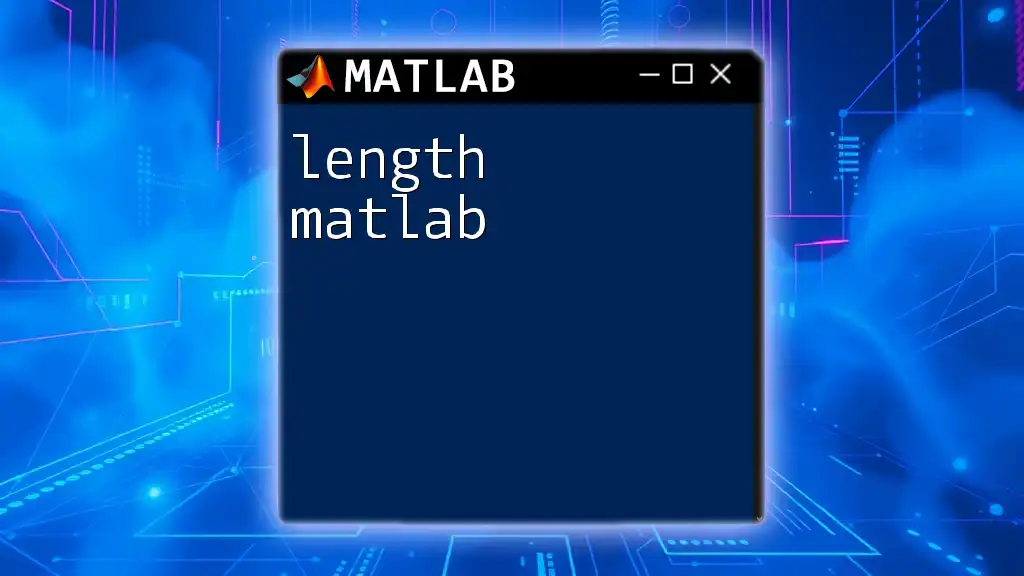
Additional Resources
Further Reading and Exploration
Explore the official MATLAB documentation for a detailed understanding of functionalities related to arrays. Additionally, enrolling in online courses or tutorials can help enhance your MATLAB expertise.
Community Engagement
Feel free to share your experiences or questions in the comments section. Connect with fellow MATLAB enthusiasts in forums or social media groups to expand your network and knowledge base.