Differentiation in MATLAB can be easily performed using the `diff` function, which computes the difference between adjacent elements of a vector or the symbolic derivative of a function.
Here’s a code snippet demonstrating both numerical and symbolic differentiation in MATLAB:
% Numerical differentiation
x = 0:0.1:10; % Define a range of x values
y = sin(x); % Define a function y = sin(x)
dy_dx = diff(y)./diff(x); % Calculate the numerical derivative
% Symbolic differentiation
syms z; % Declare a symbolic variable
f = sin(z); % Define a symbolic function
df_dz = diff(f, z); % Compute the symbolic derivative
Understanding Differentiation
What is Differentiation?
Differentiation is a fundamental concept in calculus that deals with the rate at which a function changes. In simpler terms, it helps to determine the slope of a function at any given point. The derivative provides critical insights into the function's behavior, such as finding its maxima, minima, and points of inflection.
In engineering and data analysis, differentiation serves various purposes—such as modeling physical systems, optimizing processes, and analyzing trends in datasets.
Types of Differentiation
Differentiation can be categorized into two main types:
- Symbolic Differentiation: This involves obtaining an algebraic expression for the derivative. In MATLAB, this can be efficiently achieved using the Symbolic Math Toolbox.
- Numeric Differentiation: This type approximates the derivative using discrete data points. MATLAB offers various methods to carry out this process, such as finite difference methods.
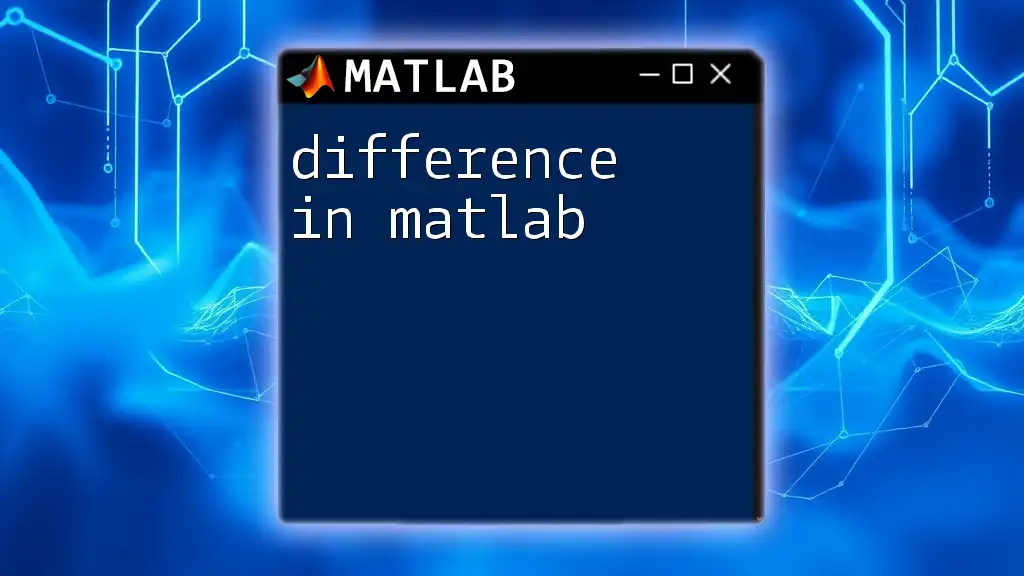
Getting Started with MATLAB
Setting Up Your MATLAB Environment
Before diving into differentiation on MATLAB, it's vital to install and configure your MATLAB environment. Download and install MATLAB from the official MathWorks website. Familiarize yourself with the MATLAB interface, focusing on the Command Window and the Editor.
Basic MATLAB Commands
Start your journey with basic commands that help keep your workspace organized:
- `clc`: Clears the command window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all open figures.
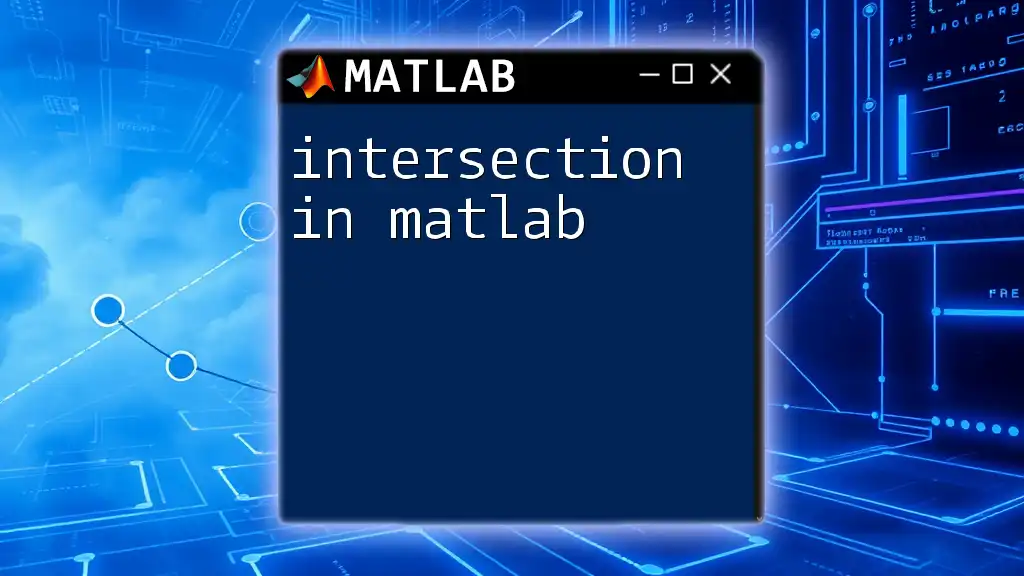
MATLAB Functions for Differentiation
Symbolic Differentiation
Utilizing the Symbolic Math Toolbox in MATLAB enables you to perform symbolic differentiation efficiently. Here’s how:
syms x
f = x^2 + 3*x + 5; % Define the function
df = diff(f, x); % Differentiate the function
In this code, `syms` creates a symbolic variable, while `diff` computes the derivative of the defined function `f`. The result, `df`, will provide a polynomial representing the derivative of `f`, which in this case is 2x + 3. Symbolic differentiation is particularly useful for analytical work and obtaining exact derivatives.
Numeric Differentiation
Numeric differentiation is essential when working with empirical data or when symbolic derivatives are too complex to compute analytically.
- Central Difference Method: A popular method for numerical differentiation involves computing the central difference. Here’s an example:
x = 0:0.1:10; % Define the range
f = sin(x); % Sample function
df = gradient(f); % Numeric differentiation
In this snippet, `gradient` computes the derivative of the sine function across the given range. It's particularly effective for uniformly spaced data and will yield an approximation of the derivative function.
- Using the `diff` function: Another approach for numeric differentiation is applying the `diff` function directly to data vectors.
x = [1, 2, 3, 4]; % Sample data points
f = [1, 4, 9, 16]; % Corresponding function values
df = diff(f) ./ diff(x); % Compute derivative
This code computes the differences in function values and divides them by the differences in corresponding x-values, producing an approximate derivative. It's particularly useful when dealing with discrete datasets.
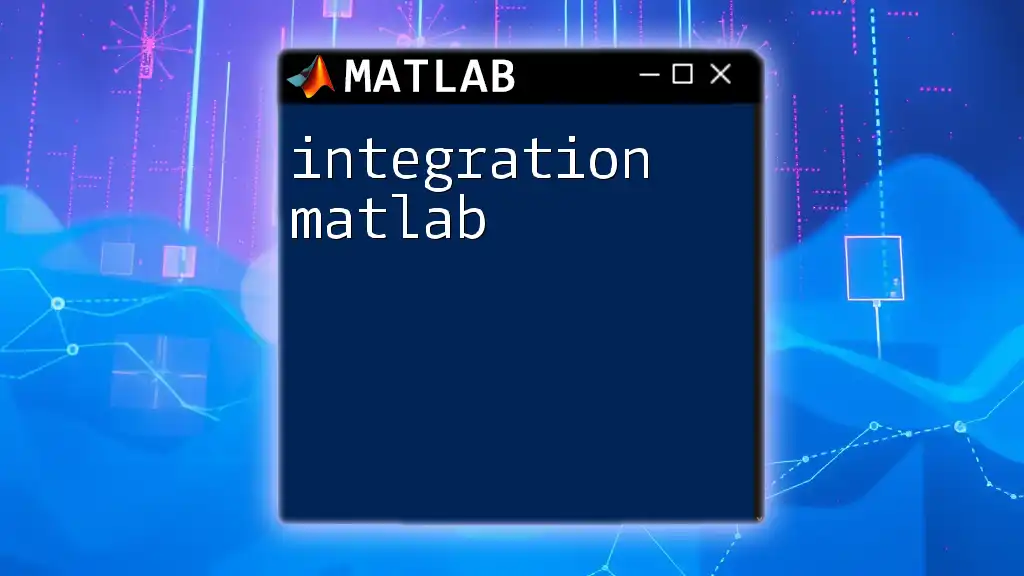
Practical Applications of Differentiation
Understanding derivatives allows for deeper analysis of function behavior. Derivatives can help identify the nature of a function at critical points, such as when it is increasing or decreasing.
Analyzing Function Behavior
One application of differentiation in MATLAB is to find the maximum and minimum values of a function. By setting the derivative equal to zero, we can locate critical points, which can indicate local maxima, minima, or points of inflection.
critical_points = solve(diff(f, x) == 0, x); % Find critical points
This code uses the `solve` function to find values of `x` that make the derivative equal to zero.
Using Derivatives in Data Fitting
Derivatives also play a vital role in data fitting and optimization, allowing for better model fits in various applications. For instance:
data = [1, 2, 3, 4, 5]; % Sample data
f = @(x) x.^2; % Quadratic fitting function
fit_params = polyfit(data, f(data), 2); % Fit a polynomial
Here, the `polyfit` function estimates the parameters of a polynomial that best fits the provided data points. Analyzing the fit can often involve examining the derivatives to understand how well the model captures changes in the data.
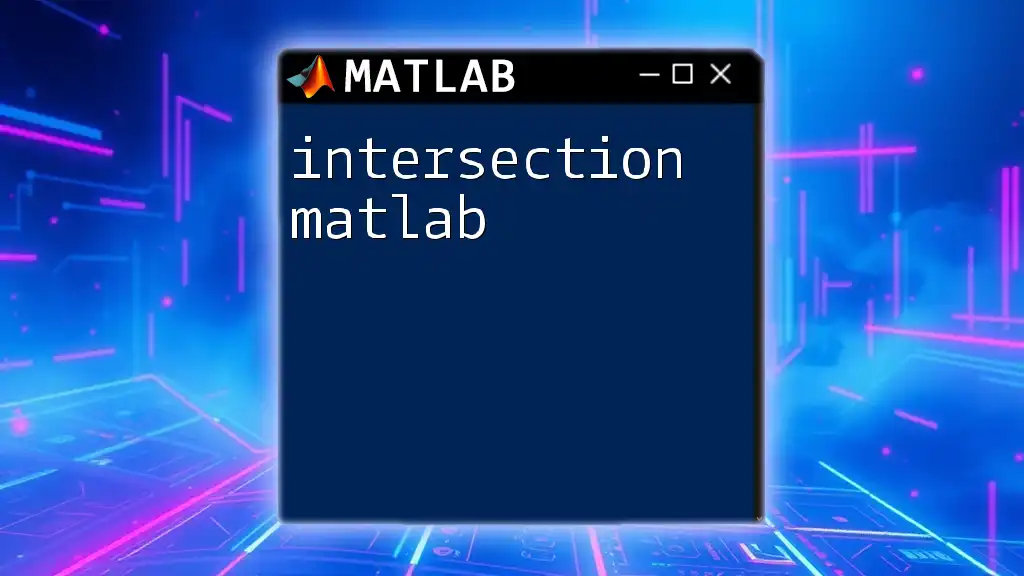
Visualizing Derivatives
A powerful way to understand differentiation is through visualization. Plotting a function alongside its derivative can provide insights into how changes in the function relate to changes in the underlying variable.
f = @(x) x.^3 - 6*x.^2 + 9*x; % Define a function
df = @(x) 3*x.^2 - 12*x + 9; % Define its derivative
fplot(f, [-1, 10], 'b'); % Plot function
hold on;
fplot(df, [-1, 10], 'r--'); % Plot derivative
legend('Function', 'Derivative');
title('Function and Its Derivative');
In this example, `fplot` generates plots of both the function and its derivative across a specified range. The function is plotted in blue, and the derivative is plotted as a dashed red line, providing a clear visual distinction between them.
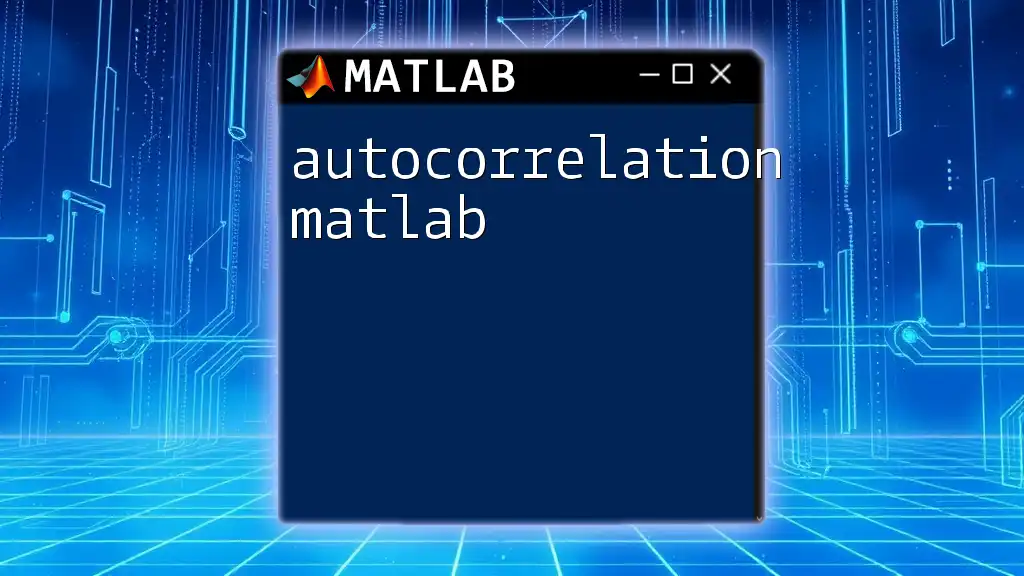
Common Issues and Troubleshooting
While working with differentiation in MATLAB, you may encounter some common errors:
-
Symbolic vs. Numeric Differentiation Issues: Be aware that symbolic differentiation can sometimes lead to complex results that may not correlate well with numeric approximations, especially if your function involves piecewise definitions or discontinuities.
-
Matrix-Related Errors: Ensure that your vectors are properly dimensioned when using operations like `diff`, as MATLAB requires inputs to conform to specific sizes.
Debugging Tips
If you run into issues, use MATLAB's debugging tools. The editor allows you to set breakpoints, step through your code, and examine variable values at each stage, further aiding you in identifying where errors may occur.
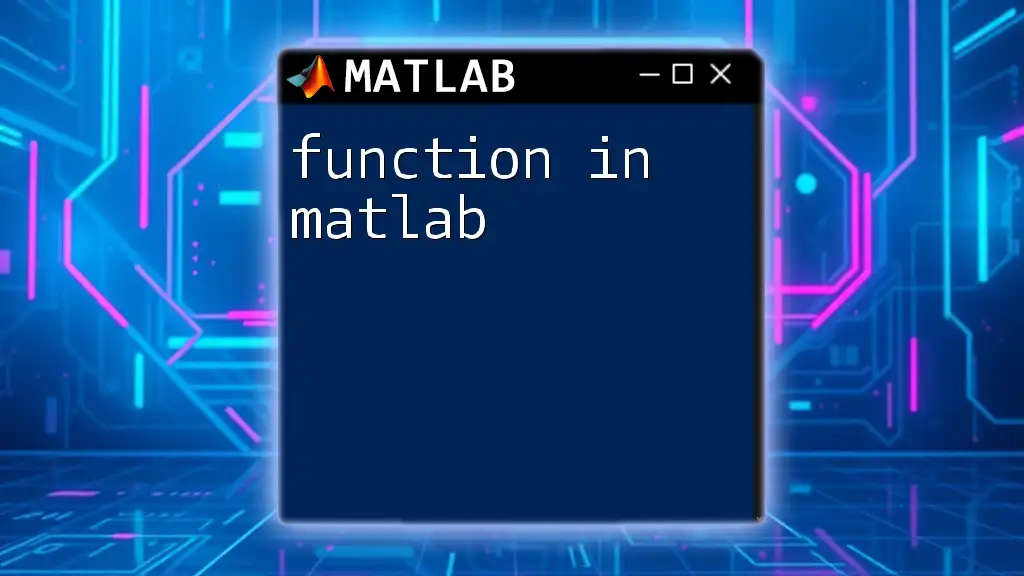
Conclusion
Differentiation on MATLAB offers a robust set of tools to explore and analyze functions mathematically and numerically. Whether you're employing symbolic methods for precise analytical results or using numeric differentiation for data-driven insights, understanding these concepts can significantly enhance your computational capabilities.
Mastering differentiation in MATLAB aids in unlocking advanced analytical techniques, optimizing solutions, and visualizing functions effectively. Start experimenting with these tools and incorporate differentiation techniques into your projects to deepen your understanding of the underlying principles.
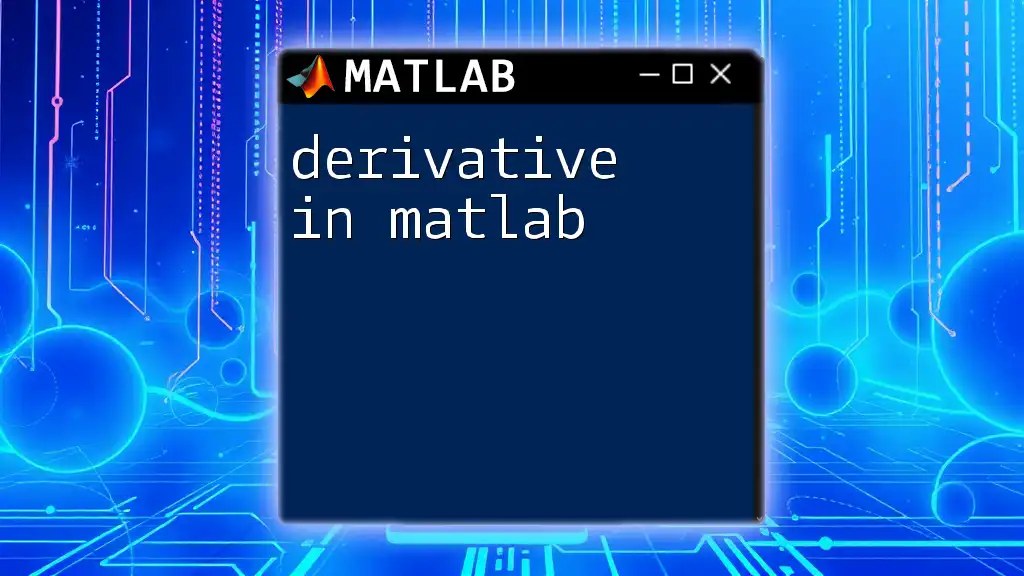
Additional Resources
To further your knowledge on differentiation in MATLAB, consider exploring MATLAB's official documentation and engaging with online tutorials. Practicing with various exercises will also enhance your proficiency in utilizing differentiation tools and functions in MATLAB effectively.