An integrator in MATLAB is a tool that allows users to compute the integral of a function over a specified interval, commonly used in numerical analysis and simulations.
Here’s a simple example of how to use the `integral` function in MATLAB to compute the integral of the sine function from 0 to π:
result = integral(@(x) sin(x), 0, pi);
Understanding Integrators in MATLAB
What is an Integrator?
An integrator is a fundamental tool in numerical analysis that computes the integral of a function, which can represent various physical quantities, such as areas under curves or cumulative sums. Understanding integrators is crucial in fields ranging from engineering to physics, where they are employed to solve differential equations, perform statistical analyses, or model dynamic systems.
Why Use MATLAB for Integration?
MATLAB offers a powerful environment for performing integration tasks due to its extensive computing capabilities and straightforward syntax. Unlike many other programming languages or software tools, MATLAB provides built-in functions that simplify complex mathematical operations. It streamlines numerical and symbolic integrations, making it accessible for both beginners and advanced users alike, enabling them to focus more on solving problems rather than coding intricacies.
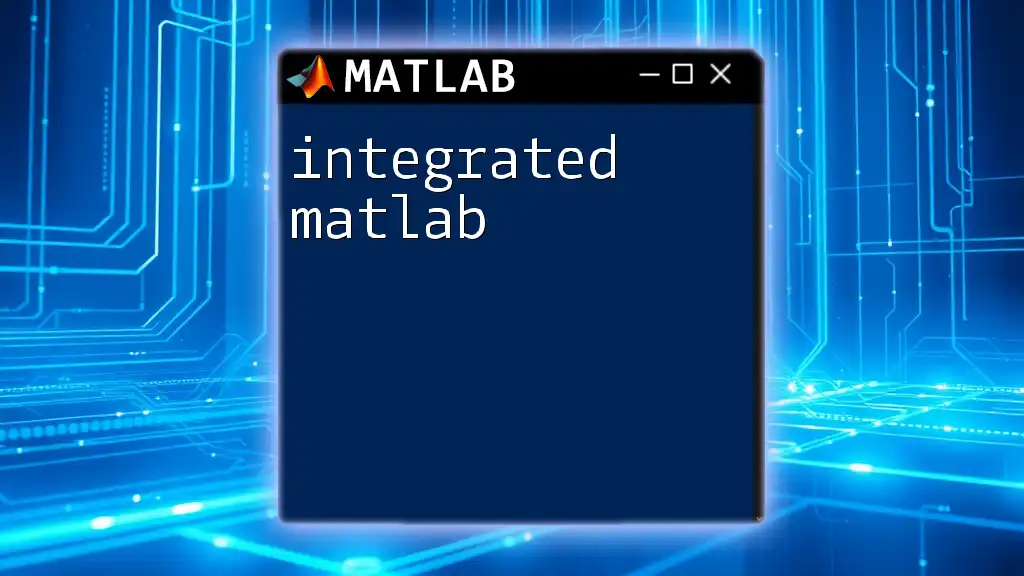
Types of Integration in MATLAB
Numerical Integration
Numerical integration refers to techniques for approximating the value of an integral when an analytical solution is difficult or impossible to obtain. It is widely used in practice due to its effectiveness in handling irregular or complex functions. The most common numerical methods include:
- Trapezoidal Rule: This method approximates the area under a curve by dividing it into small trapezoids.
- Simpson's Rule: This technique uses parabolic segments to achieve higher accuracy than the trapezoidal rule.
Symbolic Integration
On the other hand, symbolic integration allows users to determine an integral in its exact form, providing an analytical solution rather than an approximation. This is particularly useful when dealing with functions that can be expressed algebraically.
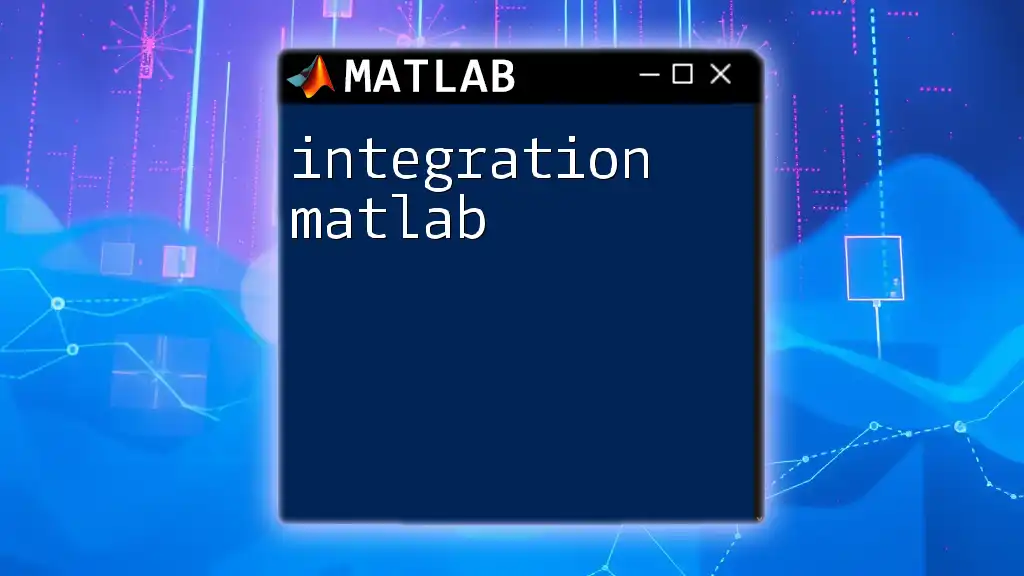
Using Integrator Commands in MATLAB
The `integral` Function
The `integral` function in MATLAB is designed for numerical integration. It efficiently computes definite integrals using adaptive quadrature methods that automatically adjust the computational effort based on the function's properties.
The syntax for the `integral` function is as follows:
integral(fun, a, b)
Here, `fun` is the function to integrate, while `a` and `b` define the limits of integration.
Example:
To integrate the function \( f(x) = x^2 \) from 0 to 1, you can use the following MATLAB code:
% Example: Integrate f(x) = x^2 from 0 to 1
f = @(x) x.^2;
result = integral(f, 0, 1);
disp(result); % Output will be 0.3333
This output implies that the area under the curve of \( x^2 \) from 0 to 1 is \( \frac{1}{3} \).
The `integral2` Function
For cases that require double integrals, MATLAB provides the `integral2` function. This function allows integration over two dimensions.
The syntax is as follows:
integral2(fun, xmin, xmax, ymin, ymax)
Example:
To integrate the function \( f(x,y) = xy \) over the rectangle defined by \( [0,1] \times [0,1] \):
% Example: Integrate f(x,y) = xy over the region [0,1] x [0,1]
fun = @(x,y) x.*y;
result = integral2(fun, 0, 1, 0, 1);
disp(result); % Output: 0.1667
This indicates the area obtained by the integration over the specified limits.
The `integral3` Function
For triple integrals, the `integral3` function expands upon the concept, enabling computations in three-dimensional space.
The syntax for this function is:
integral3(fun, xmin, xmax, ymin, ymax, zmin, zmax)
Example:
To carry out the triple integration of \( f(x,y,z) = xyz \) over the unit cube \( [0,1]^3 \):
% Example: Integrate f(x,y,z) = xyz over the cube [0,1]^3
fun = @(x,y,z) x.*y.*z;
result = integral3(fun, 0, 1, 0, 1, 0, 1);
disp(result); % Output: 0.0417
This value represents the volume under the specified function within the defined region.
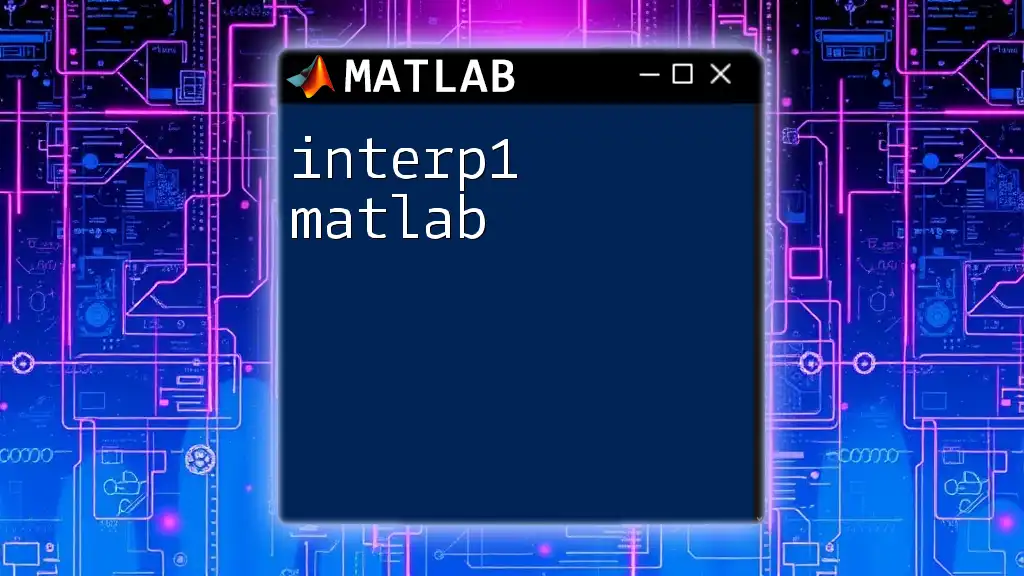
Advanced Integration Techniques
Adaptive Quadrature
The adaptive quadrature method used within the `integral` function enhances the accuracy of the numerical solutions. It divides the integration process into smaller segments and adjusts the number of points in areas where the function has high variability. This approach yields precise results without unnecessary computational costs.
Error Estimation
In numerical integration, understanding potential errors is vital. MATLAB allows users to specify `AbsTol` (absolute tolerance) and `RelTol` (relative tolerance) parameters, enabling effective control over error estimates. By fine-tuning these parameters, users can achieve a balance between accuracy and computation time.
Using Custom Functions
Creating custom functions for integration is straightforward. Users can define any mathematical function using function handles.
Example:
To integrate a user-defined function such as \( f(x) = \sin(x^2) \):
% Custom function example
custom_fun = @(x) sin(x.^2);
result = integral(custom_fun, 0, pi);
This command will compute the integral of the sine function squared from 0 to \( \pi \), illustrating MATLAB's flexibility in handling diverse mathematical tasks.
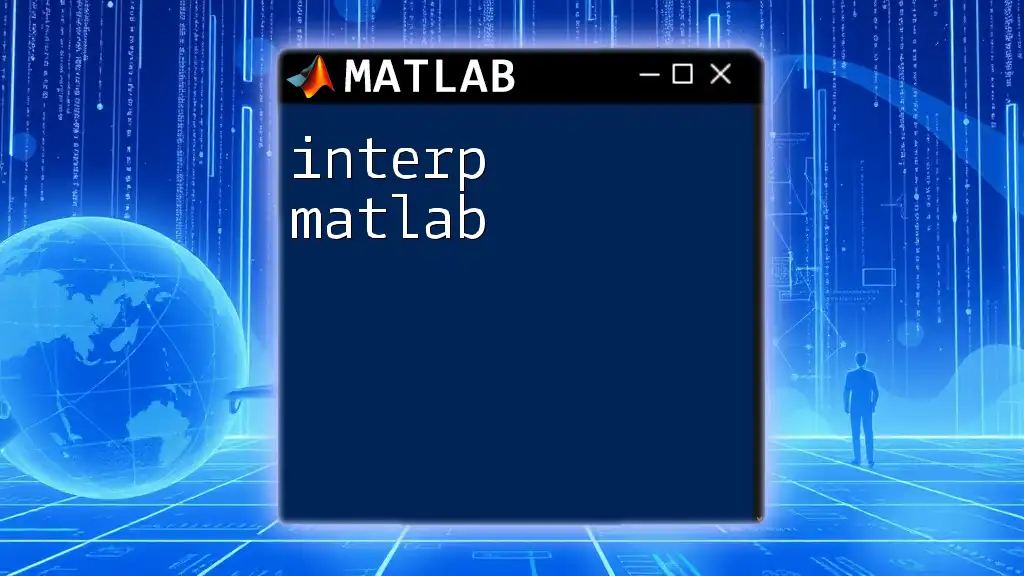
Real-World Applications of Integration in MATLAB
Applications in Physics
In physics, integration plays a crucial role in calculating the area under curves (representing work done by a force), determining displacement from velocity, or evaluating total charge from charge density distributions. MATLAB’s robust integration functions streamline these calculations.
Applications in Engineering
In engineering, integrators are extensively used for control systems design (to understand system dynamics), fluid mechanics (to analyze flow rates), and structural analyses (to determine stress distributions). MATLAB provides tools that integrate these principles conveniently, fostering innovation and precise modeling.
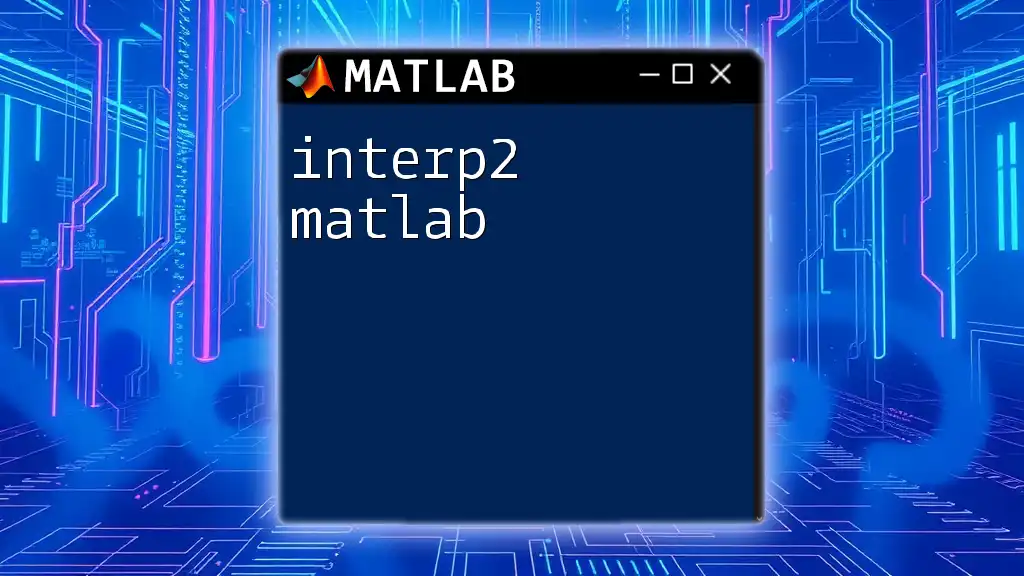
Conclusion
Recap of Key Points
In this guide, we explored the essentials of integration in MATLAB, including the use of various integral functions, advanced techniques like adaptive quadrature and error estimation, and practical applications across physics and engineering.
Additional Resources
For further reading, refer to the official MATLAB documentation, which provides an in-depth analysis of integrators and their applications. Moreover, consider online courses or tutorials that focus on mastering MATLAB for numerical computations.
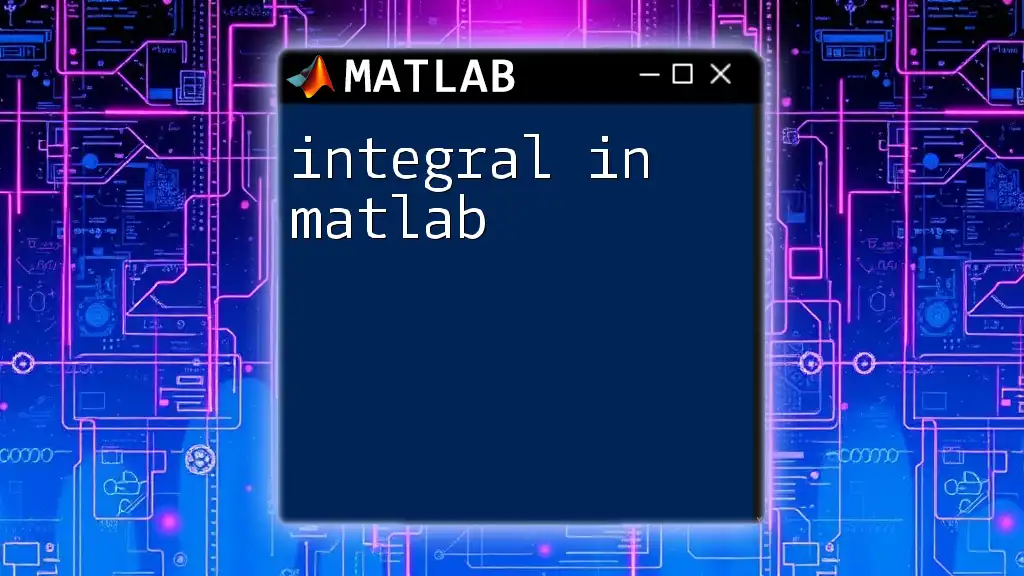
Call to Action
We invite you to explore more topics related to MATLAB on our website. Have you tried using MATLAB for integration? Share your experiences in the comments section!