In MATLAB, you can compute the definite integral of a function using the `integral` function, which efficiently calculates the area under the curve for a specified interval.
Here’s a code snippet to demonstrate this:
% Define the function as an anonymous function
f = @(x) x.^2;
% Calculate the definite integral from 0 to 1
result = integral(f, 0, 1);
% Display the result
disp(result);
Understanding Integrals
What is an Integral?
An integral represents a fundamental concept in calculus that allows us to compute areas under curves and to find accumulated quantities. There are two primary types of integrals: definite integrals and indefinite integrals. A definite integral computes the area under a curve between two specified limits, while an indefinite integral finds a function whose derivative provides the original function.
Types of Integrals in MATLAB
MATLAB offers capabilities for both numerical and symbolic integration. Choosing between these methods depends on the nature of the problem at hand. Numerical integration is typically used for functions where an analytical form isn't available or is extremely complex. In contrast, symbolic integration is valuable when an exact solution is needed.
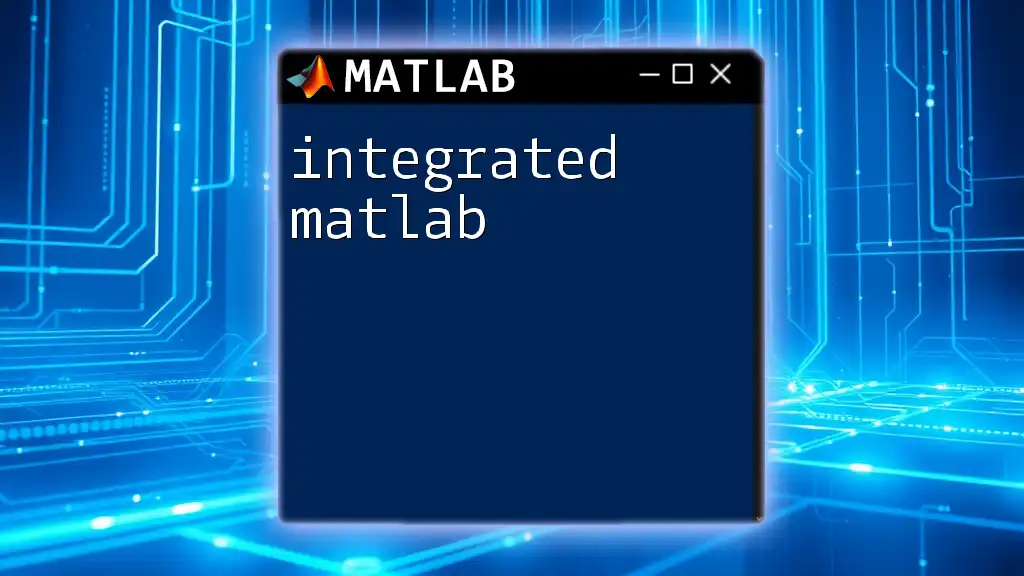
Setting Up Your MATLAB Environment
Installing MATLAB
Getting started with MATLAB is straightforward. Ensure you download and install the software from the official MathWorks website. Follow the installation prompts, and once installed, launch the MATLAB interface.
Loading Required Toolboxes
For symbolic integration, you will need the Symbolic Math Toolbox. To check if your toolbox is installed, you can use the command:
ver
This will display a list of all installed toolboxes. If the Symbolic Math Toolbox is not present, you can purchase and install it through the MathWorks website.
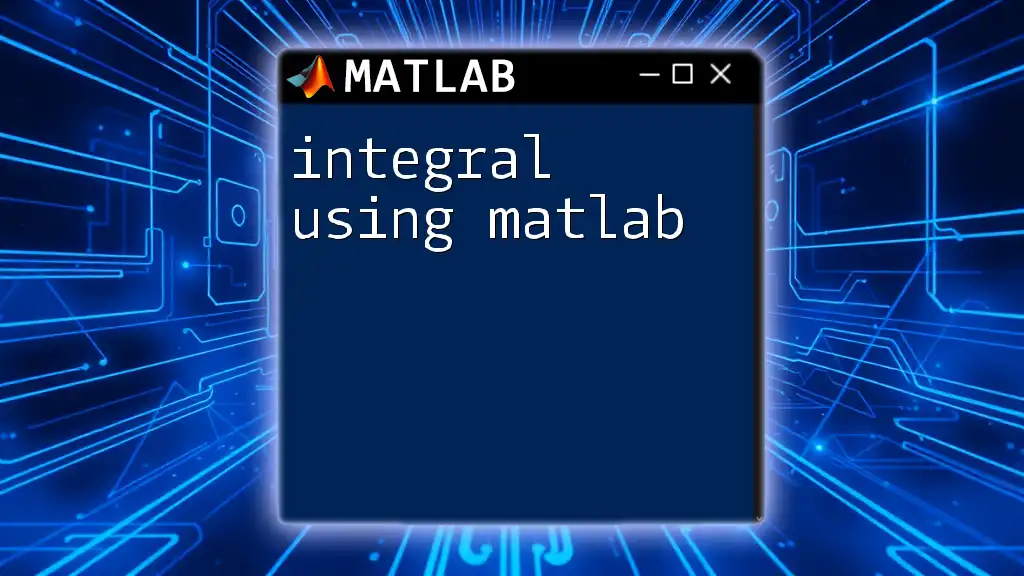
Using MATLAB for Numerical Integration
Integral Function for Definite Integrals
In MATLAB, the `integral` function is essential for calculating definite integrals. The syntax follows the form:
integral(function, a, b)
Here, `function` is a function handle representing the mathematical function to be integrated, while `a` and `b` are the limits of integration.
Example: To compute the definite integral of the function \( f(x) = x^2 \) from 0 to 1, you can use the following code:
f = @(x) x.^2; % define the function
result = integral(f, 0, 1); % compute the integral from 0 to 1
disp(result); % display the result
This code snippet defines the function \( f(x) \), calculates the integral within the specified limits, and then displays the result. The expected output will be \( \frac{1}{3} \) or approximately 0.3333.
Using Integral2 for Double Integrals
When dealing with functions of two variables, the `integral2` function comes into play. Its syntax is:
integral2(function, a, b, c, d)
- `function` represents a function handle for \( f(x,y) \).
- `a` and `b` specify the limits for \( x \), while `c` and `d` specify the limits for \( y \).
Example: To compute the double integral \( \int_0^1 \int_0^1 xy \, dy \, dx \):
f = @(x,y) x.*y; % define the 2D function
result = integral2(f, 0, 1, 0, 1); % compute double integral
disp(result);
This shows how to work with two-dimensional integration, yielding a result that is pivotal in areas such as physics and engineering.
Using Integral3 for Triple Integrals
For functions of three variables, the `integral3` function is used:
integral3(function, a, b, c, d, e, f)
Each parameter corresponds to the integration limits for the respective variables \( x, y, \) and \( z \).
Example: For the triple integral \( \int_0^1 \int_0^1 \int_0^1 xyz \, dz \, dy \, dx \):
f = @(x,y,z) x.*y.*z; % define the 3D function
result = integral3(f, 0, 1, 0, 1, 0, 1); % compute triple integral
disp(result);
This demonstrates computing triple integrals, which is useful in advanced applications such as fluid dynamics.
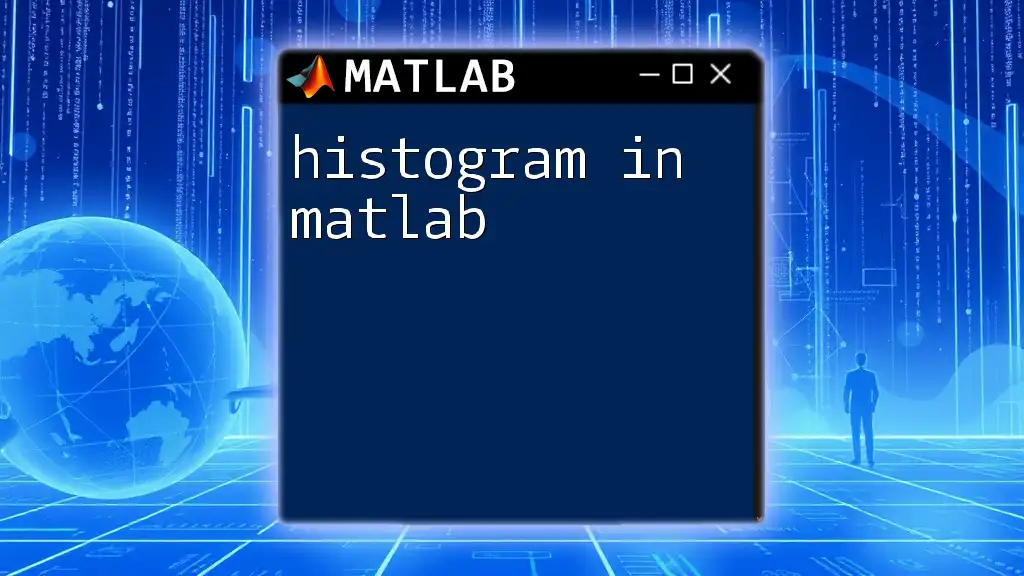
Using MATLAB for Symbolic Integration
Introduction to Symbolic Math Toolbox
The Symbolic Math Toolbox allows for analytical computations that provide exact results. Here, integration can be approached differently than in numerical scenarios, often yielding expressions instead of numerical values.
Symbolic Indefinite Integrals
To compute an indefinite integral, you would use the `int` function. The syntax is:
int(f, x)
Example: For the integral of function \( f(x) = x^2 \):
syms x; % declare symbolic variable
result = int(x^2, x); % compute the indefinite integral
disp(result);
This will produce \( \frac{x^3}{3} + C \), where \( C \) is the constant of integration.
Symbolic Definite Integrals
For a definite integral, the syntax extends to include limits:
int(f, x, a, b)
Example: To compute the definite integral from 0 to 1 of \( f(x) = x^2 \):
syms x;
result = int(x^2, x, 0, 1); % compute the definite integral
disp(result);
This will provide the exact result \( \frac{1}{3} \).
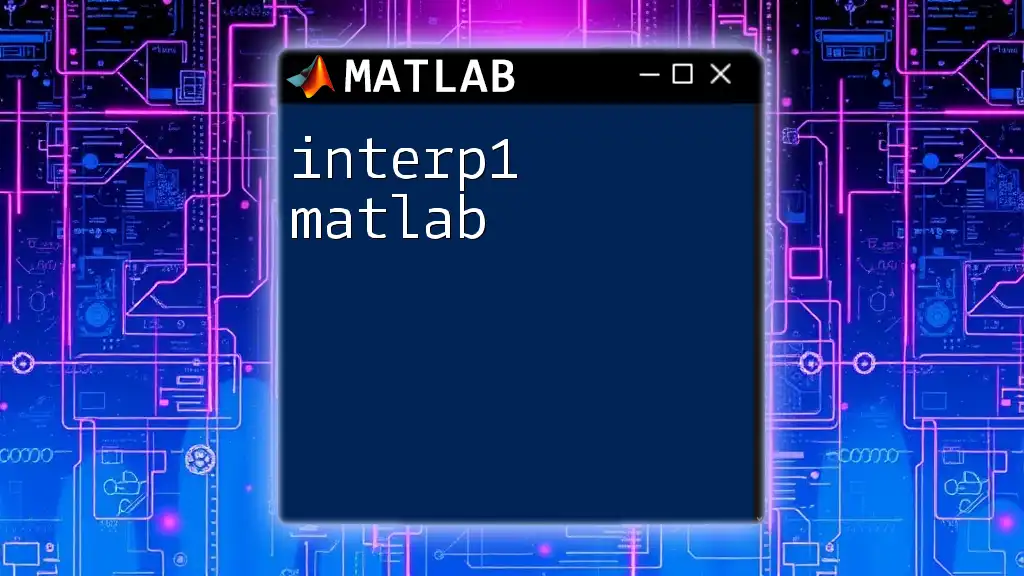
Common Pitfalls and Troubleshooting
Handling Errors
Error messages often arise from improperly defined functions or integration limits. It’s crucial to ensure that the function is properly vectorized for numerical integration. MATLAB will provide warnings if the computation runs into difficulties such as insufficient accuracy.
Optimization Techniques
To enhance performance, especially for complex functions, you can adjust the relative tolerance (`RelTol`) and absolute tolerance (`AbsTol`) parameters in the `integral` function.
For example:
result = integral(f, 0, 1, 'RelTol',1e-6, 'AbsTol',1e-12);
These adjustments can significantly affect the speed and accuracy of the integration process.
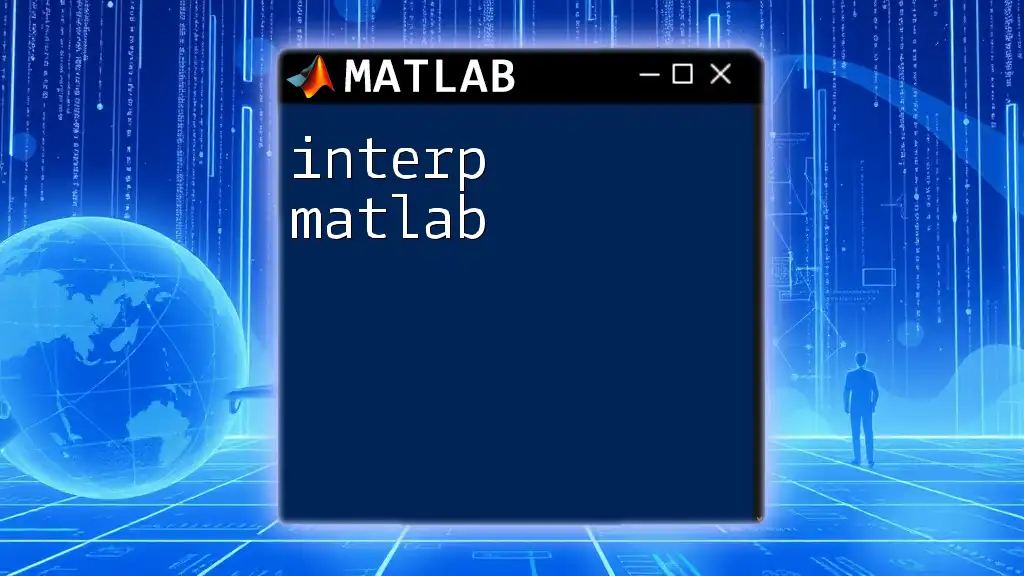
Practical Applications of Integration in MATLAB
Real-World Applications
Integration has vast applications in fields such as engineering, physics, and economics. Common implementations include calculating areas, volumes, and cumulative distributions in data analysis.
Case Study: Solving a Real-World Problem
Consider a scenario where you need to find the total area beneath a curve representing velocity over time. You can define a function that represents the velocity, compute the integral over a specified time interval, and interpret the result as the total displacement.
Example:
v = @(t) 3*t.^2 + 2*t + 1; % velocity function
displacement = integral(v, 0, 5); % total displacement from t=0 to t=5
disp(displacement); % results in total displacement
In this scenario, such calculations are pivotal for analyzing motion and can support various engineering projects.
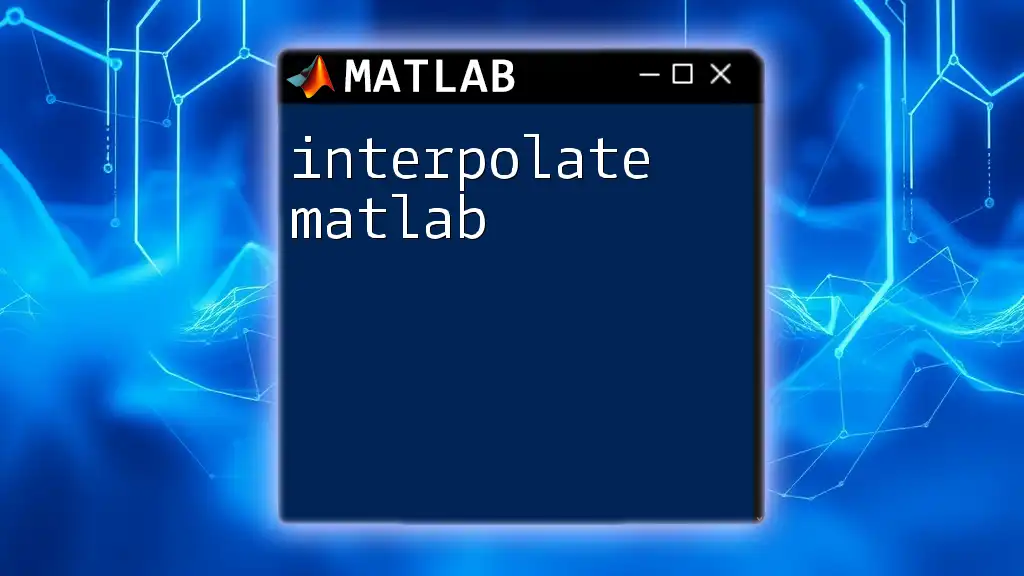
Conclusion
Summary of Key Points
Mastering the integral in MATLAB is essential for both numerical and symbolic calculations. Understanding when to use numerical versus symbolic tools can greatly enhance your computational efficiency and accuracy in solving problems.
Next Steps
To further develop your proficiency, consider exploring additional resources such as textbooks on numerical analysis, courses focused on MATLAB programming, or online platforms offering interactive MATLAB exercises.
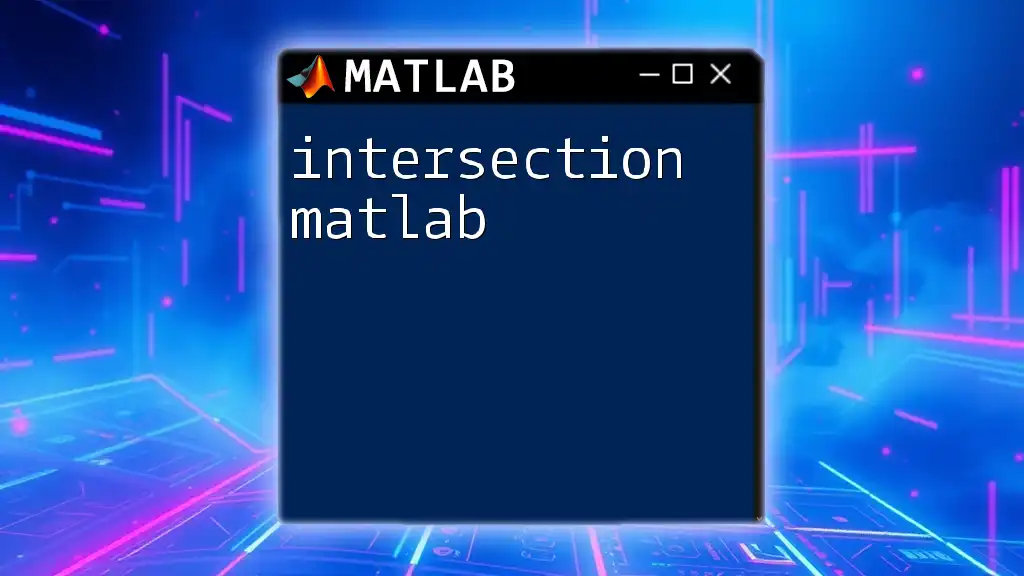
FAQs
How do I integrate a complex function?
You can define complex functions using MATLAB's anonymous functions, just like simpler functions. Ensure to account for any multi-dimensional variables appropriately.
What should I do if I get a warning about insufficient accuracy?
You may need to refine your function or adjust the `RelTol` and `AbsTol` parameters to improve the estimate of the integral.
Can I visualize the integral?
While MATLAB does not directly plot integrals, you can plot the function and shade the area under the curve to illustrate the integral visually. This can aid in understanding the concept better.
With this comprehensive guide on using the integral in MATLAB, you are well-equipped to tackle various problems using both numerical and symbolic methods. Happy coding!