The `fileparts` function in MATLAB is used to split a full file path into its components: the folder path, the file name, and the file extension.
filepath = 'C:\Users\Example\Documents\file.txt';
[folder, name, ext] = fileparts(filepath);
Understanding the `fileparts` Function
What is `fileparts`?
`fileparts` is a built-in function in MATLAB that serves a straightforward yet essential purpose: it extracts components of a file path. By breaking down a full file name into its constituent elements—directory path, file name, and file extension—`fileparts` makes file manipulation tasks more intuitive.
Why Use `fileparts`?
Using `fileparts` provides numerous advantages, particularly in coding efficiency and readability. It is especially useful for script developers who need to process files dynamically based on their properties. Common use cases include:
- Generating new file names while preserving original directory structures.
- Extracting extensions for condition-based file processing.
- Simplifying the integration of file paths in larger applications.
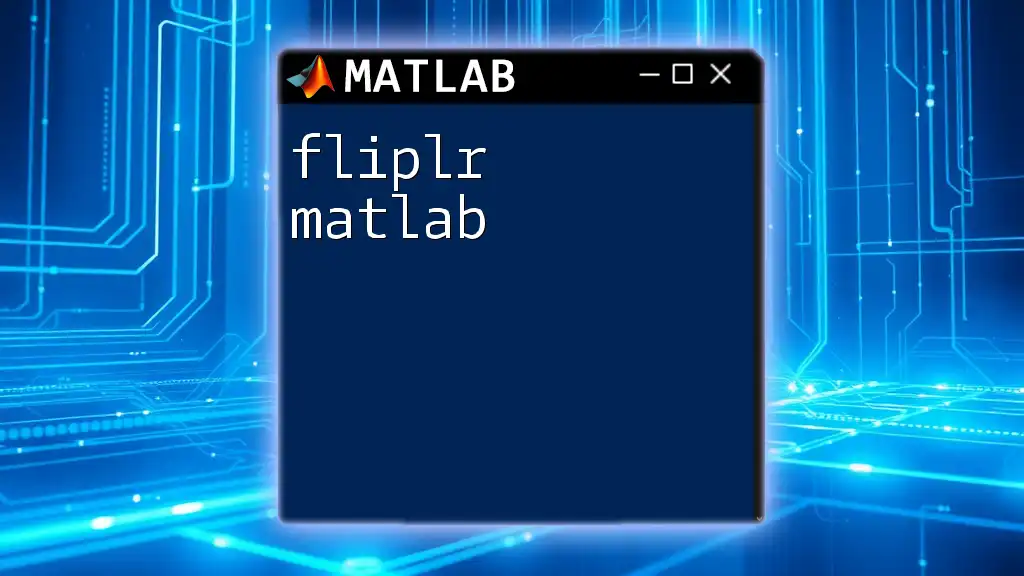
Syntax of `fileparts`
Basic Syntax
The syntax for `fileparts` is both simple and intuitive:
[filepath, name, ext] = fileparts(filename);
Input Argument Breakdown
filename is the single input argument that can accept various formats—this includes absolute paths, relative paths, and even just the file name. For instance:
filename = 'C:\Users\Documents\example.txt';
Output Variables Explained
File Path
The `filepath` output demonstrates the significance of separating the path component of the file. This can be particularly helpful when constructing new paths or moving files.
[filepath, ~, ~] = fileparts(filename);
disp(filepath); % Output: C:\Users\Documents
File Name
The `name` output gives you the file name without the extension, which is essential when performing operations like appending or modifying names.
[, name, ~] = fileparts(filename);
disp(name); % Output: example
File Extension
The `ext` output represents the file's extension, critical for file type identification.
[, , ext] = fileparts(filename);
disp(ext); % Output: .txt
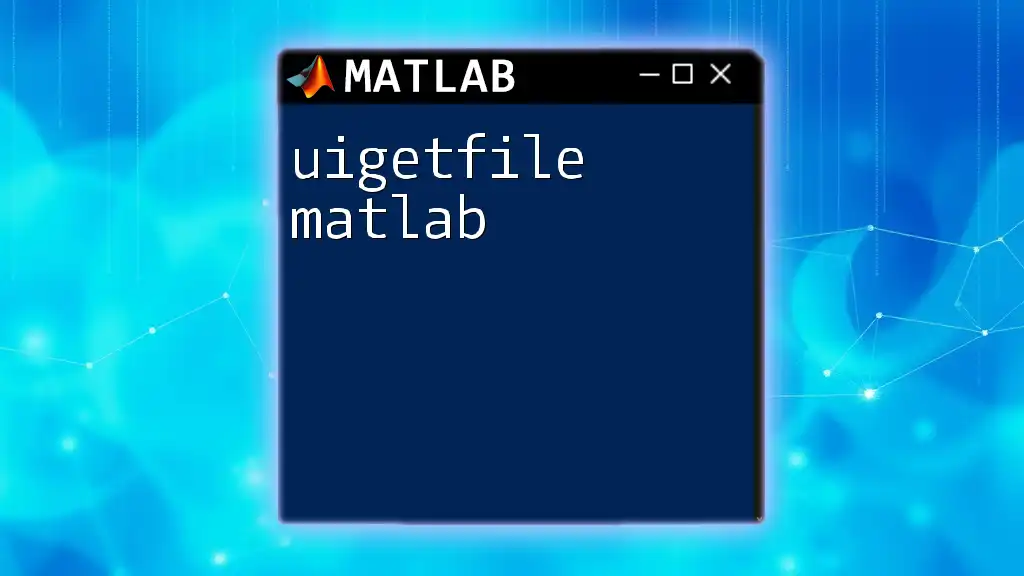
Practical Applications of `fileparts`
Separating File Components
There are numerous scenarios where separating file components can greatly enhance workflow. For example, in a project that involves generating reports, you might need to save different versions of a file.
complex_filename = '/home/user/docs/report_data.csv';
[fp, n, e] = fileparts(complex_filename);
disp(['Path: ' fp ', Name: ' n ', Extension: ' e]);
Dynamic File Manipulation
`fileparts` can be utilized for dynamic file name generation. For instance, if you want to create a backup of the original file, the following code illustrates how to achieve that:
new_filename = fullfile(filepath, [name, '_backup', ext]);
disp(new_filename); % Output: C:\Users\Documents\example_backup.txt
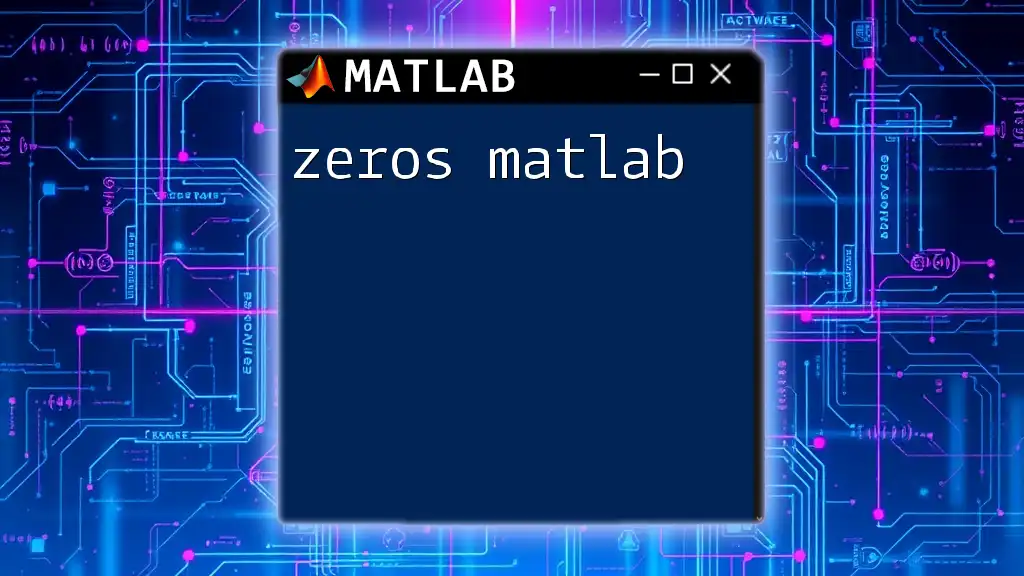
Advanced Usage of `fileparts`
Handling Multiple Files
`fileparts` can also be leveraged in loops to process multiple files quickly. This is particularly useful for batch processing large datasets. Below is an example showcasing this:
fileList = {'file1.txt', 'file2.mat', 'file3.csv'};
for i = 1:length(fileList)
[fp, n, e] = fileparts(fileList{i});
fprintf('File: %s, Path: %s, Name: %s, Ext: %s\n', fileList{i}, fp, n, e);
end
Combining with Other Functions
Integrating `fileparts` with other MATLAB functions like `fullfile` and `strcat` can produce robust solutions. For example, the workflow below showcases how to efficiently create a new filename with "_new" appended:
[fp, n, e] = fileparts(filename);
new_file = fullfile(fp, [n '_new' e]);
disp(new_file); % Output: C:\Users\Documents\example_new.txt
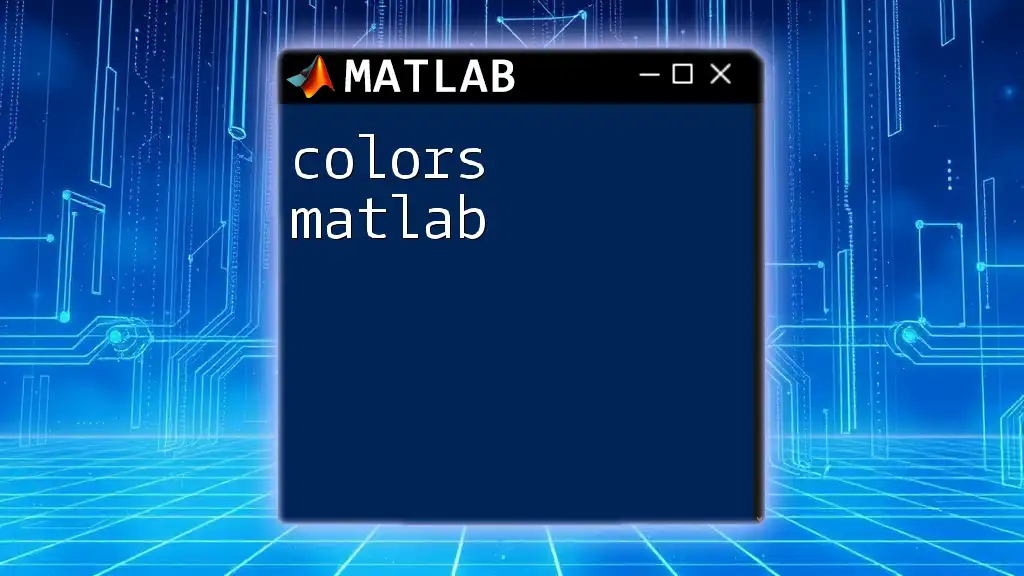
Best Practices when Using `fileparts`
Handling Edge Cases
When working with file paths, edge cases like invalid file names or empty strings can lead to errors. It’s essential to incorporate error handling when utilizing `fileparts`. For example:
try
[fp, n, e] = fileparts(''); % Passing an empty string
catch ME
disp('Error: Invalid filename provided.');
end
Performance Considerations
To optimize code when managing large datasets or numerous file operations, it's important to consider performance. Efficient batch processing in scripts can save significant computation time. Implement best practices like pre-defining file lists or avoiding unnecessary string manipulations.
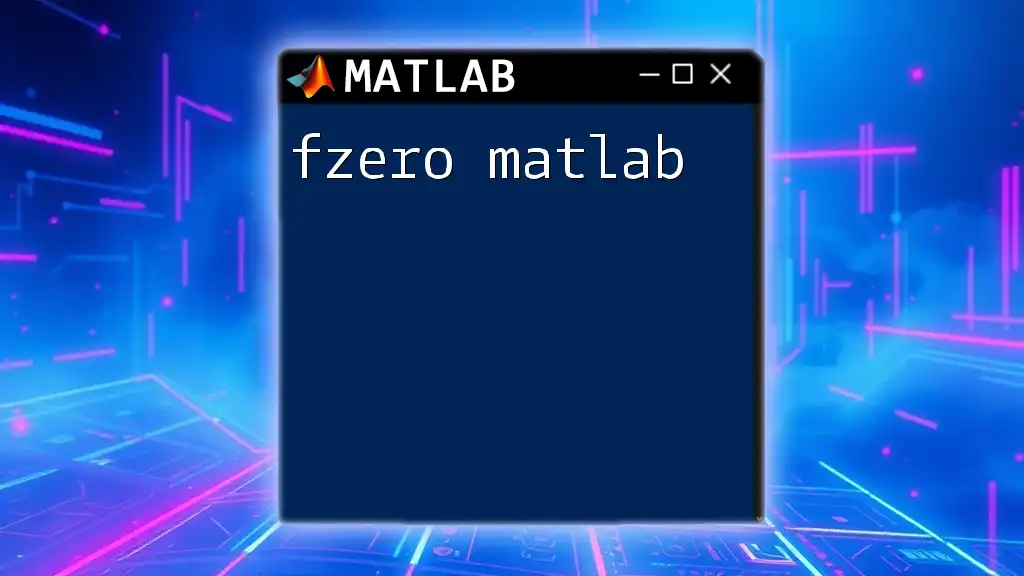
Conclusion
This comprehensive guide has walked you through the intricacies of `fileparts` in MATLAB, showcasing its utility in file management tasks. By using `fileparts`, you can easily enhance both the functionality and clarity of your code, making it an invaluable tool in your MATLAB programming toolkit. Don't hesitate to explore more complex file path manipulations to expand your skills even further!
Further Resources
For additional information and advanced techniques, check out the official MATLAB documentation on `fileparts`, and consider delving into related topics to bolster your understanding of file handling in MATLAB.