"Electronic MATLAB" refers to the application of MATLAB for designing, simulating, and analyzing electronic systems and circuits efficiently.
Here’s a simple example of using MATLAB to plot a basic sine wave:
t = 0:0.01:2*pi; % Time vector from 0 to 2π
y = sin(t); % Sine wave calculation
plot(t, y); % Plot the sine wave
xlabel('Time (seconds)'); % X-axis label
ylabel('Amplitude'); % Y-axis label
title('Sine Wave'); % Title of the plot
grid on; % Add grid
Getting Started with MATLAB
Installation and Setup
To kick off your journey with electronic MATLAB, you first need to install it on your computer. Download it from the official MathWorks website and follow the setup instructions provided. Once installed, you will be greeted by a user-friendly interface that includes:
- Command Window: Where you can type commands and view results in real-time.
- Workspace: Displays all variables created during the session.
- Editor: Where you can create scripts for automation and reuse.
Basic MATLAB Commands
Familiarizing yourself with essential commands will enhance your workflow in electronic MATLAB. Three fundamental commands that every user should know are:
- `clc`: Clears the Command Window.
- `clear`: Removes all variables from the workspace.
- `close all`: Closes all open figure windows.
You can integrate these commands at the beginning of your scripts to ensure a clean workspace:
clc; % Clear Command Window
clear; % Clear Workspace
close all; % Close all figures
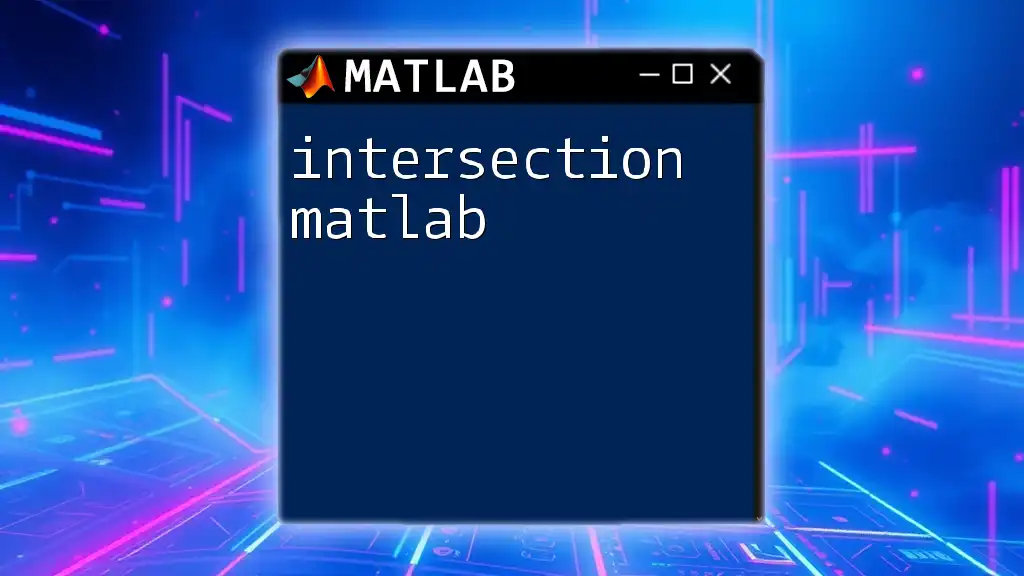
Understanding Electrical Circuits with MATLAB
Circuit Analysis Techniques
In the realm of electronics, understanding circuit analysis techniques is crucial. Key principles include Ohm’s Law, which relates voltage, current, and resistance, and Kirchhoff’s Laws, which aid in analyzing complex circuits. These laws enable you to set up equations that can be solved using MATLAB, helping visualize circuit behavior through simulations.
Example: Simple RLC Circuit
A common project for beginners is modeling a simple RLC circuit, where R (Resistance), L (Inductance), and C (Capacitance) come together to form a circuit. The following MATLAB code illustrates how to analyze its step response:
R = 10; % Resistance in Ohms
L = 0.1; % Inductance in Henrys
C = 1e-6; % Capacitance in Farads
s = tf('s');
H = 1/(R + 1/(s*L) + s*C); % Transfer function
step(H); % Plot step response
In this example, we create a transfer function `H` representing the RLC circuit and use the `step()` function to visualize the response. The step response is pivotal for evaluating the stability and behavior of the system.
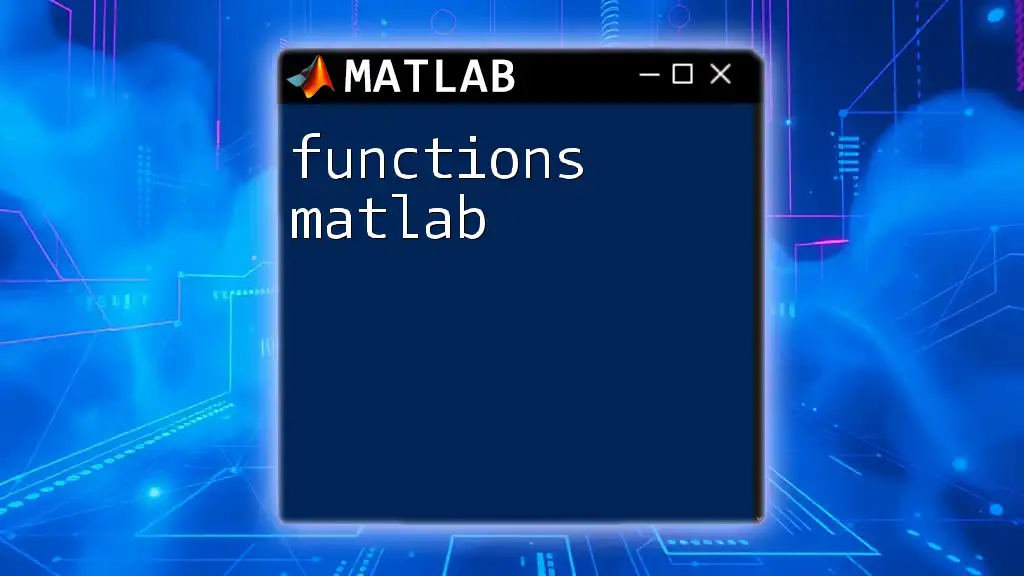
Simulating Electronic Circuits with Simulink
Introduction to Simulink
Simulink is a MATLAB add-on that allows models to be created graphically. It is particularly useful for simulating dynamic systems in electronics. Users can drag and drop components, making it accessible for beginners and effective for professionals in the industry.
Building a Simulink Model for Electronic Systems
Creating a model in Simulink involves:
- Launching Simulink: Type `simulink` in the Command Window to open the Simulink library.
- Selecting Components: Choose from the blocks available in the library, such as resistors, capacitors, and voltage sources.
- Connecting Blocks: Drag the chosen components onto the canvas and connect them as required.
Example: Buck Converter
A buck converter is a DC-DC converter that steps down voltage. To model this in Simulink, you can utilize the following components:
- Voltage Source: Simulates the input voltage.
- Switch: Represents the switching element.
- Inductor and Capacitor: Storage elements in the converter.
Simulink allows you to visualize the voltage and current waveforms at different points in the circuit, providing insights into efficiency and performance.
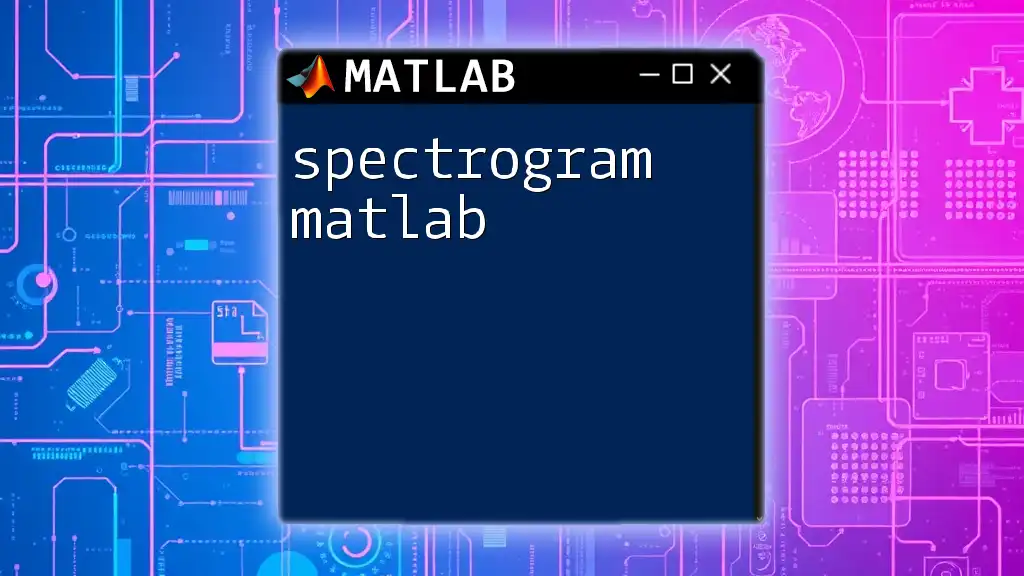
Control Systems in Electronics
Basics of Control Systems
Control systems involve regulating the behavior of electronic devices or processes. Two fundamental types are SISO (Single Input, Single Output) and MIMO (Multiple Input, Multiple Output). Mastering concepts in this area is vital for any electronic engineer.
Example: Designing a PID Controller
One of the most common control strategies in electronics is the PID (Proportional-Integral-Derivative) controller, which adjusts the output based on the error between a desired setpoint and a measured variable. The following code initializes a PID controller in MATLAB:
Kp = 1; Ki = 0.1; Kd = 0.01; % PID coefficients
C = pid(Kp, Ki, Kd); % Create PID controller
H = tf(1, [1 2 1]); % Placeholder system transfer function
T = feedback(C*H, 1); % Create closed-loop system
step(T); % Plot step response
In this code, we define the PID coefficients and create a closed-loop transfer function `T`, which allows us to visualize the effects of our PID controller on system dynamics through the step response.
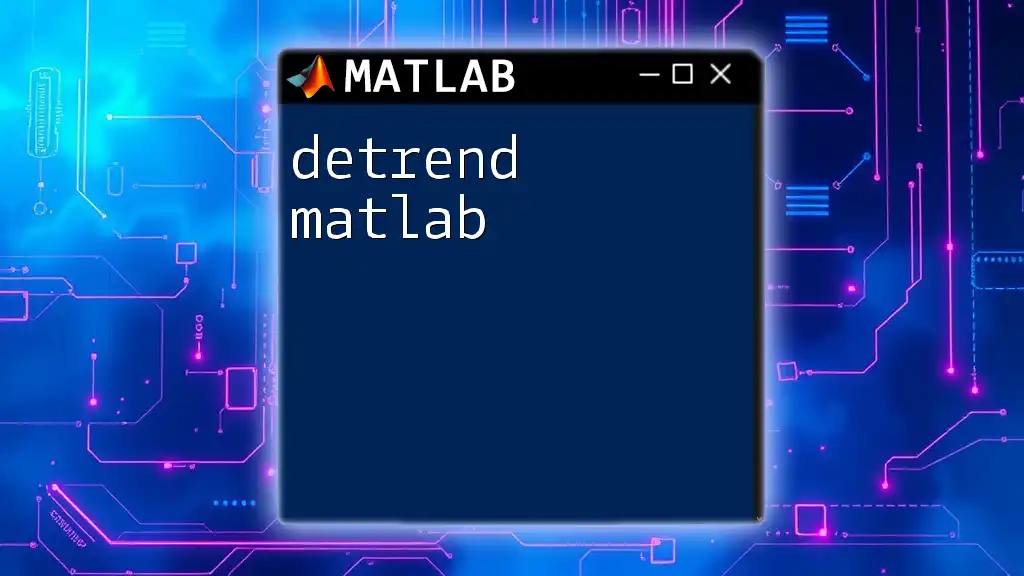
Signal Processing with MATLAB
Fundamentals of Signal Processing
Signal processing is crucial for analyzing and manipulating signals. Fundamental concepts include sampling (converting continuous signals to discrete) and filtering (removing unwanted frequencies).
Example: Filter Design
Designing filters is a key application in electronic MATLAB. The following code snippet creates a low-pass Butterworth filter:
Fs = 1000; % Sampling Frequency
Fc = 100; % Cut-off Frequency
[b,a] = butter(5, Fc/(Fs/2)); % 5th order Butterworth filter
fvtool(b, a); % Visualize the filter
In this case, `butter()` generates filter coefficients, which you can analyze using `fvtool()`. Understanding filter design and response characteristics is essential for applications like audio processing, communications, and more.
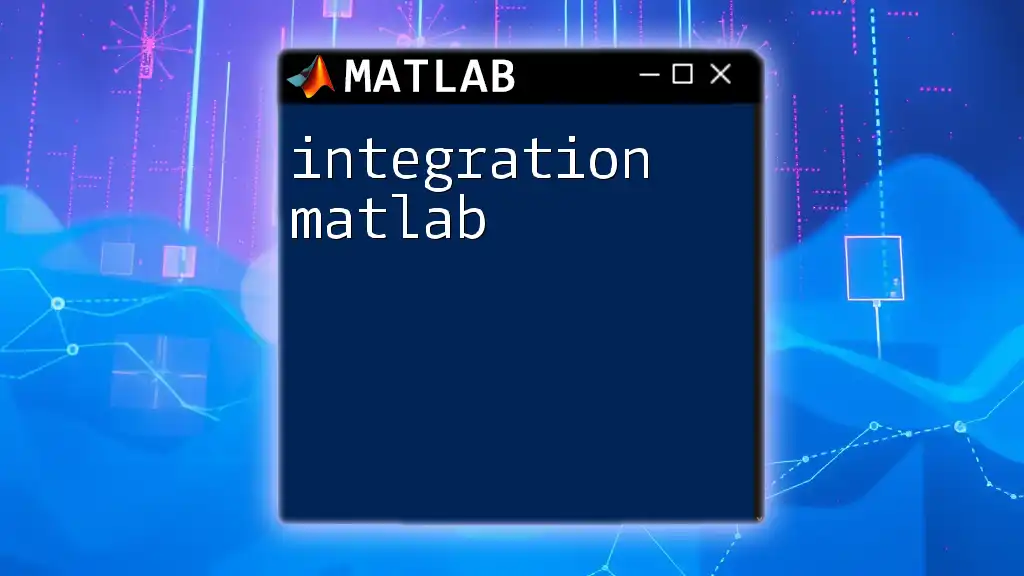
Automation and Scripting in Electronic MATLAB
Creating Scripts for Repetitive Tasks
Automation in MATLAB allows you to execute repetitive tasks efficiently. By writing scripts, you can streamline simulations and analyses, enabling faster results.
Example: Batch Processing of Data
Here’s how you can automate circuit analysis for various resistor values:
for R = [10, 20, 50]
H = 1/(R + 1/(s*L) + s*C); % New transfer function for each R
figure; % Open new figure
step(H); % Plot step response
title(['Step Response for R = ' num2str(R)]); % Set title
end
In this example, a loop iterates through different values of resistance, generating a new figure for each analysis. Automating such repetitive tasks can save valuable time and enhance productivity.
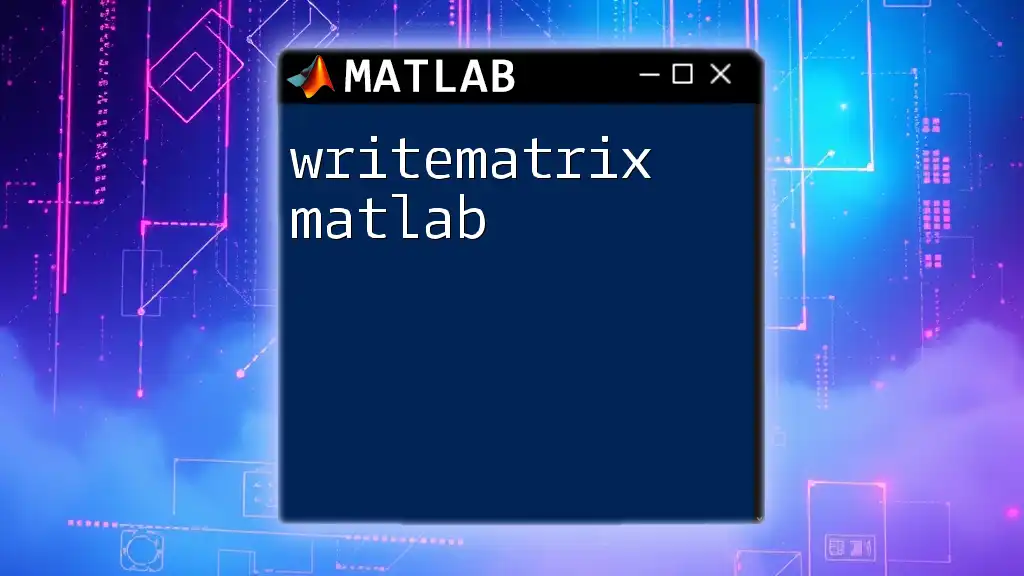
Advanced Topics in Electronic MATLAB
Integration with Hardware
Integrating MATLAB with hardware like Arduino or Raspberry Pi allows for real-world data analysis and control. This integration aids engineers in testing and implementing theories practically.
Example: Data Acquisition
Utilizing the Data Acquisition Toolbox, you can read data from various sensors seamlessly. Here’s an elementary example of how to gather data:
d = daq.createSession('ni'); % Create session for National Instruments
addAnalogInputChannel(d, 'Dev1', 'ai0', 'Voltage'); % Add voltage channel
data = d.startForeground(); % Collect data
plot(data); % Plot the acquired data
This simple code demonstrates how to set up a data acquisition session, read voltage data, and visualize it. Acquiring and analyzing real-time data enhances research and development in electronics.
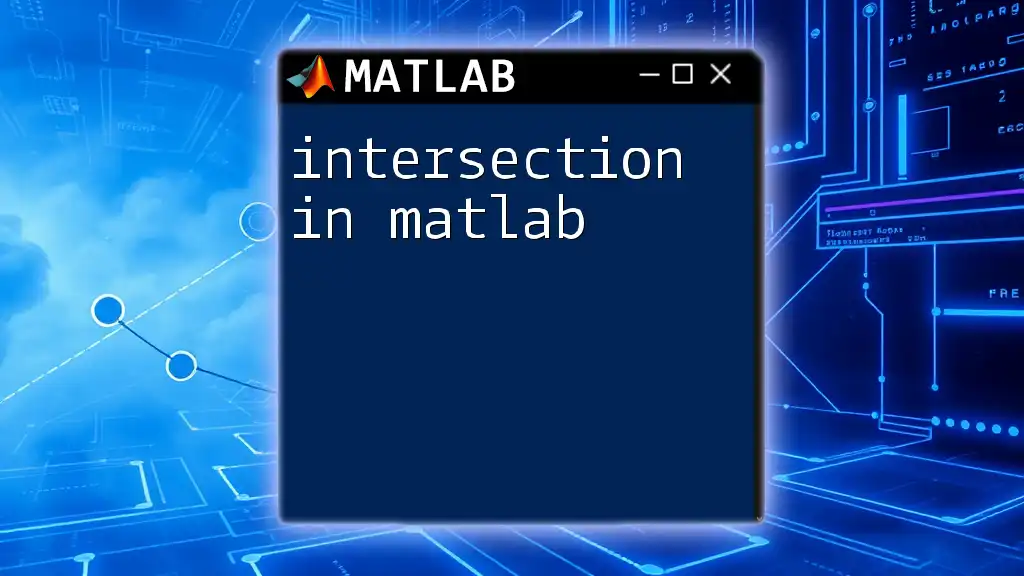
Conclusion
Summary of Key Takeaways
Our exploration of electronic MATLAB covered essential circuit analysis, signal processing, control systems, automation, and hardware integration. Each area provides vital insights for engineers aspiring to master MATLAB's capabilities in electronics.
Future of Electronic MATLAB
As technology advances, the role of MATLAB in electronics continues to grow. Emerging trends such as machine learning applications, real-time data processing, and Internet of Things (IoT) implementations promise exciting developments.
Call to Action
Now that you have a robust understanding of electronic MATLAB, it's time to experiment! Dive into MATLAB, try the examples outlined, and begin developing your skills in this powerful tool that significantly enhances electronic problem-solving.
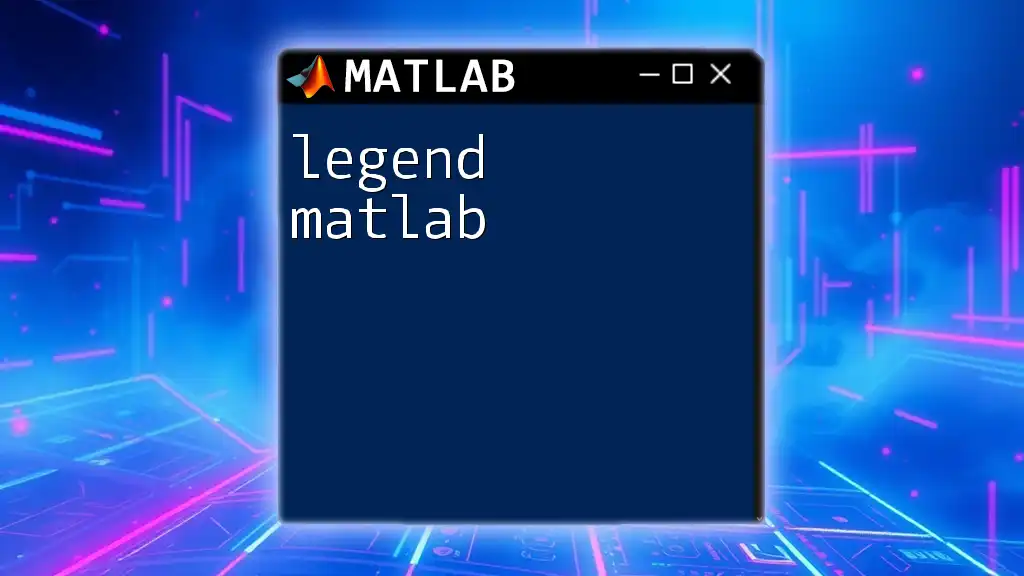
Additional Resources
Books, Tutorials, and Online Courses
For those eager to learn more, consider exploring recommended resources such as textbooks focused on MATLAB in electronics, online tutorials, and courses that provide hands-on projects.
Community and Forums for MATLAB Users
Join community forums like MATLAB Central to connect with other users, share experiences, and seek assistance. Engaging with fellow enthusiasts can accelerate your learning and keep you informed about the latest trends in electronic MATLAB.