In MATLAB, the `diff` function calculates the difference between adjacent elements in an array, which can be useful for analyzing changes in data sets.
Here's a simple code snippet demonstrating the use of the `diff` function:
data = [1, 3, 6, 10]; % Example data
differences = diff(data); % Calculate the difference between adjacent elements
disp(differences); % Display the differences
Understanding the Importance of Differences
In the context of MATLAB, the term "difference" encompasses multiple meanings tied to mathematical concepts, numerical analysis, and algorithmic implementations. Understanding these differences is crucial, as they play a pivotal role in diverse applications such as data analysis, simulation, and mathematical modeling.
Differentiation—whether numerical or symbolic—is a fundamental process used throughout MATLAB for interpreting and manipulating data. From simple trend detection to complex simulations in engineering and science, differences help clarify changes over time, identify trends, and improve computational models.
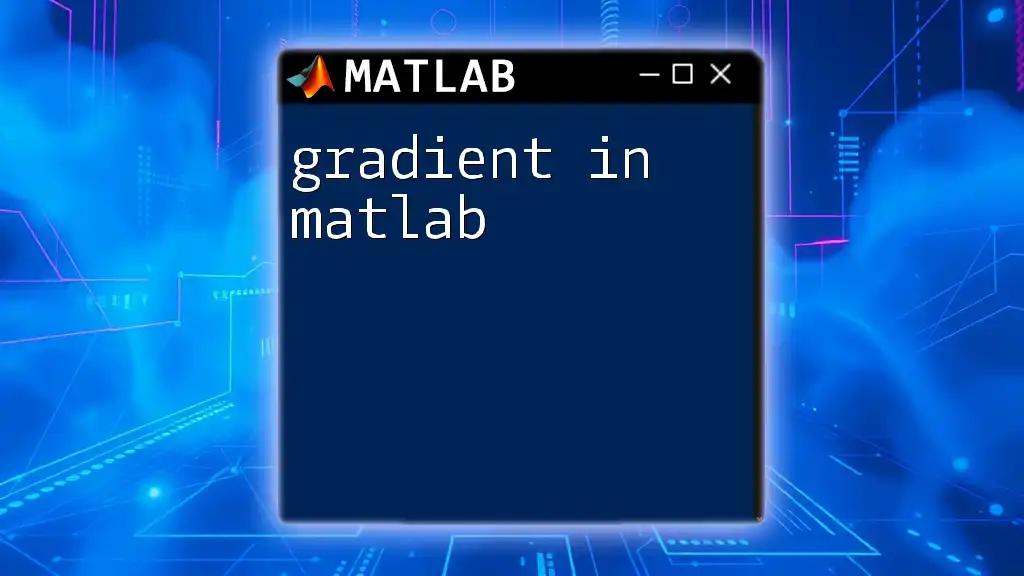
Types of Differences in MATLAB
Discrete Differences in MATLAB
Definition and Explanation
Discrete differences refer to the change in data values at distinct intervals or points. This type of difference is particularly useful in time series analysis, statistical computations, and any scenario where data points are sampled rather than continuous.
Using the `diff` Function
The built-in `diff` function is a powerful tool for calculating discrete differences in MATLAB. Its basic syntax is straightforward, enabling quick computation of first-order differences.
For example, consider an array:
A = [1, 2, 4, 7];
D = diff(A);
disp(D);
In this example, `D` will output:
1 2 3
This indicates that the differences between successive elements in `A` are 1, 2, and 3, respectively. This simple yet effective function allows users to explore data changes quickly.
Applications of Discrete Differences
Discrete differences find wide application in areas such as:
- Trend Analysis: Identifying upward or downward movements in data.
- Time Series Prediction: Evaluating historical data to forecast future values.
- Signal Processing: Analyzing and removing noise from sampled signals.
Continuous Differences in MATLAB
Definition and Explanation
Continuous differences refer to the concept of partial derivatives or rates of change in continuous mathematics. Understanding this form is essential in modeling natural phenomena and solving equations involving real-world scenarios.
Using Symbolic Math Toolbox for Continuous Differences
For continuous differentiation, MATLAB provides the Symbolic Math Toolbox. This enables users to define mathematical functions symbolically and compute their derivatives.
For example:
syms x;
f = x^2 + 3*x + 2;
df = diff(f, x);
disp(df);
This code snippet computes the derivative of the function \( f(x) = x^2 + 3x + 2 \), resulting in:
2*x + 3
This output showcases how derivatives convey slopes of functions at any point on the curve, crucial in assessing behavior in physics and engineering.
Numerical Approximation of Continuous Differences
When dealing with empirical data, continuous differences can be approximated using numerical methods, particularly finite difference techniques.
Here's an example of how to compute a numerical derivative using differences:
x = 0:0.1:10;
y = sin(x);
dydx = diff(y) ./ diff(x);
disp(dydx);
This code snippet calculates the derivative of the sine function based on discrete points. The result will provide an approximation of the derivative \( \cos(x) \) at each interval.

Practical Examples and Applications
Example 1: Analyzing Data Trends
To illustrate the application of differences, consider a dataset representing observed values over time. Such data might look like this:
time = [1, 2, 3, 4, 5];
data = [10, 20, 15, 25, 30];
To visualize the differences:
plot(time, data, '-o', time(1:end-1), diff(data), '-x');
legend('Original Data', 'Differences');
title('Data Trend Analysis');
This plot enables quick visual inspection of changes in the dataset, showcasing how the differences reveal trends over the specified timeframe.
Example 2: Signal Processing
In signal processing, differences play a crucial role in filtering noise from signals. By applying the `filter` function, users can effectively manage data to extract significant features while minimizing errors introduced by noise.
Consider a scenario where you have data representing noise-superimposed signals. By applying first differences, users can identify abrupt changes, suggesting signal transitions or errors needing correction.
Example 3: Numerical Methods in Engineering
In engineering disciplines, understanding differences is indispensable for numerical simulations of structures and thermal analysis. Finite difference methods often serve as the backbone for solving differential equations, providing approximations in conditions where analytical solutions are infeasible.
For example, simulating heat distribution across a material involves assuming initial conditions and iterating through the heat equation, employing differences at each step.
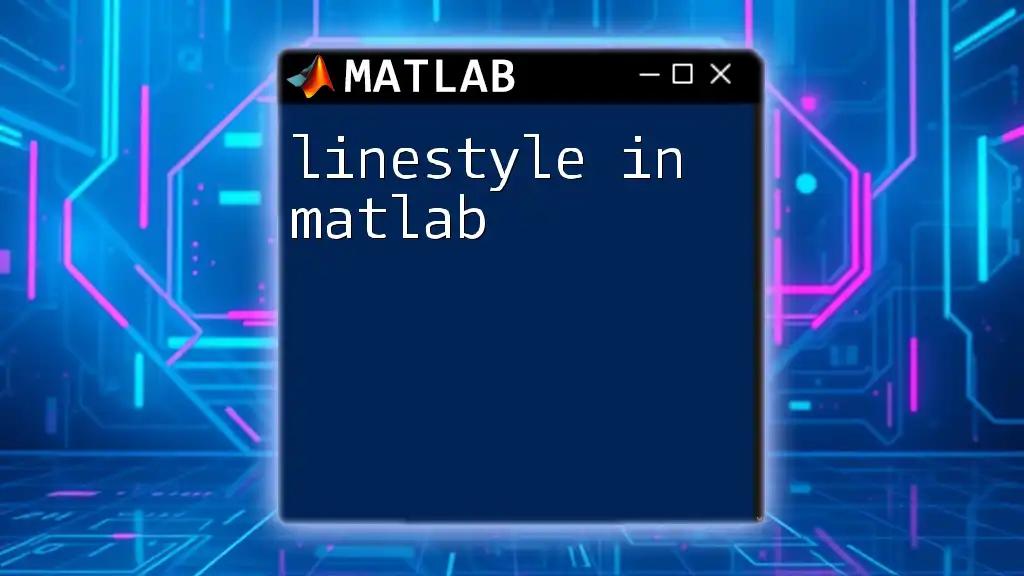
Advanced Techniques
Higher-Order Differences
Definition and Explanation
Higher-order differences extend the concept of first-order differences to examine changes over multiple intervals. This advancement can expose deeper insights in data trends or smooth out fluctuations, which is particularly beneficial for predictive modeling.
Using Loop Structures to Calculate Higher-Order Differences
Implementing higher-order differences often requires iterations through data. Consider calculating the second difference:
A = [1, 2, 4, 7, 11];
first_diff = diff(A);
second_diff = diff(first_diff);
disp(second_diff);
This will yield the second order differences, providing insight into the acceleration of changes—an extremely valuable metric in various analytical contexts, including physics and finance.
Difference Equations in MATLAB
What are Difference Equations?
Difference equations express relationships between discrete time points. Utilizing these equations allows mathematicians and engineers to model systems based on recursions, similar to ordinary differential equations for continuous cases.
Solving Difference Equations
MATLAB's ability to handle difference equations makes it powerful for simulations. For instance, consider a simple first-order difference equation:
n = 0:10;
y(1) = 1; % Initial condition
for i = 2:length(n)
y(i) = 0.5 * y(i-1) + 1; % Example difference equation
end
disp(y);
This implementation showcases how the output evolves based on prior values, providing a dynamic understanding of system behaviors over time.
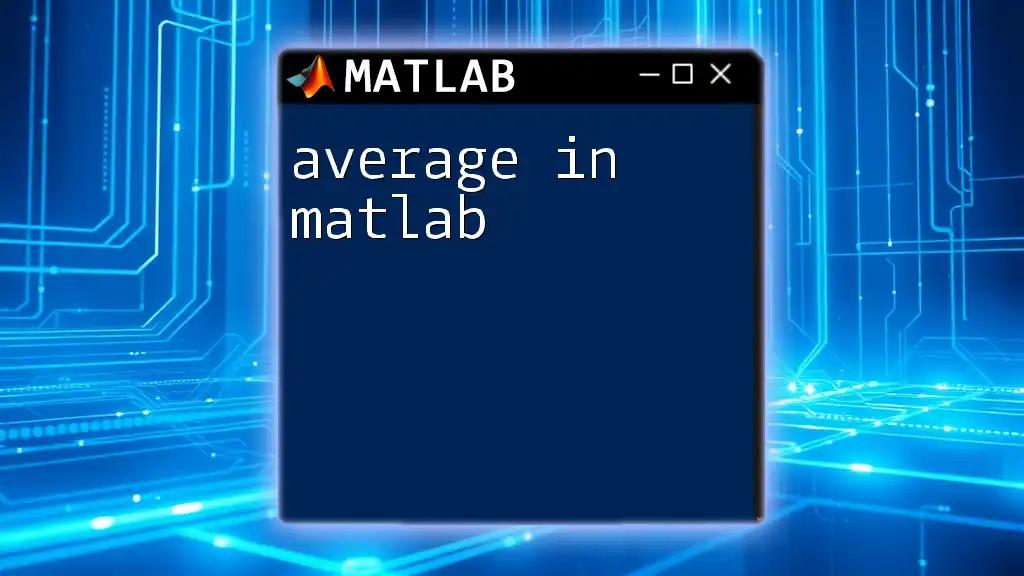
Conclusion
In summary, differences in MATLAB offer vital roles across multiple domains, from statistical analysis and engineering simulations to financial modeling. Mastering the different commands, such as `diff`, as well as understanding continuous differences through symbolic math tools, enables users to produce meaningful insights from their data.
As you delve deeper into MATLAB’s capabilities, explore advanced techniques that can leverage these differences further. The potential for powerful applications, whether in simple data analysis or robust simulations, encourages continued exploration and learning within the MATLAB ecosystem.