The `gradient` function in MATLAB computes the numerical gradient of a matrix or vector, returning the rate of change of the data elements along each dimension.
% Example of using the gradient function in MATLAB
% Define a vector
y = [1, 2, 4, 7, 11];
% Calculate the gradient
g = gradient(y);
disp(g);
Understanding the Gradient
What is a Gradient?
A gradient represents the direction and rate of change of a function. In mathematical terms, it is a vector that points in the direction of the steepest ascent of a function. The magnitude of the gradient indicates how steep that ascent is.
Mathematical Representation
The gradient of a scalar function \( f(x, y, z, \ldots) \) is mathematically defined using partial derivatives as follows:
\[ \nabla f = \left( \frac{\partial f}{\partial x}, \frac{\partial f}{\partial y}, \frac{\partial f}{\partial z}, \ldots \right) \]
Each component of the gradient vector consists of the partial derivative of the function with respect to a specific variable.
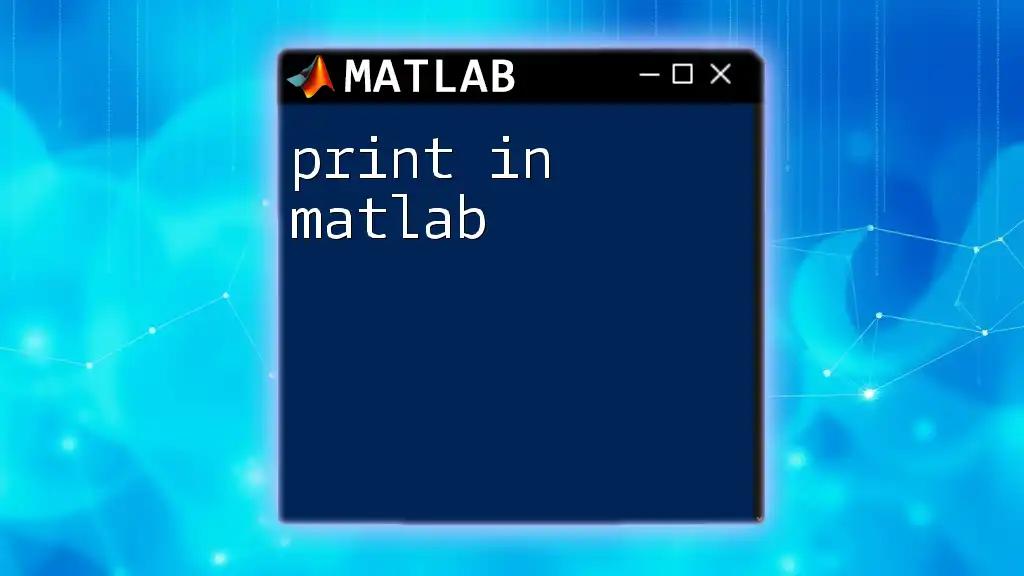
The Gradient Command in MATLAB
Overview of the `gradient` Function
In MATLAB, the `gradient` function provides a straightforward way to calculate the gradient of a function based on its discrete values. This is particularly useful in engineering and scientific applications where functions are often represented as data points.
The syntax for the `gradient` function can be summarized as follows:
[Gx, Gy] = gradient(F)
Here, \( F \) is a matrix representing the values of a function, and \( Gx \) and \( Gy \) are the gradient outputs in the x and y directions, respectively.
Input and Output
- Input: The `gradient` function can accept one-dimensional arrays or two-dimensional matrices. 1D arrays yield a single gradient vector, while 2D matrices return two gradient matrices corresponding to each dimension.
- Output: The output consists of gradient vectors that show how the function value changes in various directions. For a matrix input, you will receive two matrices representing the gradients along each axis.
Basic Example
A basic example of calculating the gradient using MATLAB could look like this:
% Example: Basic 1D Gradient Calculation
x = [1, 2, 3, 4, 5];
y = [10, 20, 10, 40, 50];
[gx, gy] = gradient(y, x);
disp(gx);
In this snippet, the gradient of the vector y with respect to x is computed. The output gx reflects the rate of change of y with respect to x.
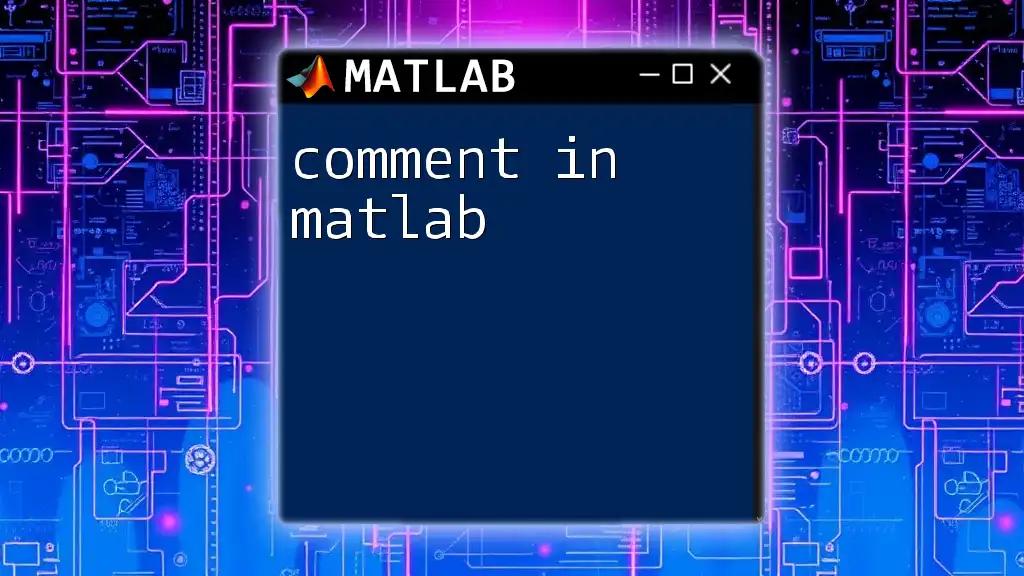
Applying the Gradient in MATLAB
Gradients of 2D Functions
Calculating the gradient of two-dimensional functions is essential for understanding how changes occur across a plane. Here’s how you can work with 2D data:
% Example: Gradient of a 2D matrix
[X, Y] = meshgrid(-5:0.1:5, -5:0.1:5);
Z = X.^2 + Y.^2; % Function
[Gx, Gy] = gradient(Z);
surf(X, Y, Z); % 3D surface plot
In this example, we create a mesh grid for values ranging from -5 to 5. The function \( Z = X^2 + Y^2 \) describes a simple paraboloid. The gradient matrices \( Gx \) and \( Gy \) reveal how steep the surface is at every point, which can be visualized using a 3D surface plot.
Gradient Magnitude and Direction
Calculating the gradient's magnitude provides insight into the "steepness" of the field, while the direction indicates where the function is increasing most rapidly. You can calculate the magnitude of the gradient using:
% Example: Gradient Magnitude
Gmag = sqrt(Gx.^2 + Gy.^2);
This calculates the Euclidean norm of the gradient vectors. The magnitude shows how strong the directional change is. In many applications, understanding both the magnitude and direction is crucial for interpreting results meaningfully.

Practical Applications of Gradients
Optimization Problems
Gradients play a vital role in numerous optimization algorithms, particularly in methods like gradient descent. Gradient descent utilizes the gradient to find the function's minimum (or maximum) efficiently. Here’s a simple implementation of gradient descent:
% Example: Simple Gradient Descent
f = @(x) (x - 3).^2; % Objective function
x0 = 0; % Starting point
learning_rate = 0.1; % Learning rate
for i = 1:10
grad = 2 * (x0 - 3); % Gradient calculation
x0 = x0 - learning_rate * grad; % Update step
end
disp(x0);
In this example, we find the minimum of the function \( f(x) = (x - 3)^2 \). By iteratively updating the variable x0 using the gradient, we can find the point where the function achieves its lowest value.
Image Processing
In the realm of image processing, gradients are instrumental in edge detection, helping to identify significant changes in pixel intensity. A basic edge detection implementation might look like this:
% Example: Edge Detection
img = imread('image.jpg');
gray_img = rgb2gray(img);
[Gx, Gy] = gradient(double(gray_img));
edges = sqrt(Gx.^2 + Gy.^2);
imshow(edges, []);
This code reads an image and converts it to grayscale. The gradient of the image is then computed with respect to the pixel intensity values, allowing edges to be identified and visualized.
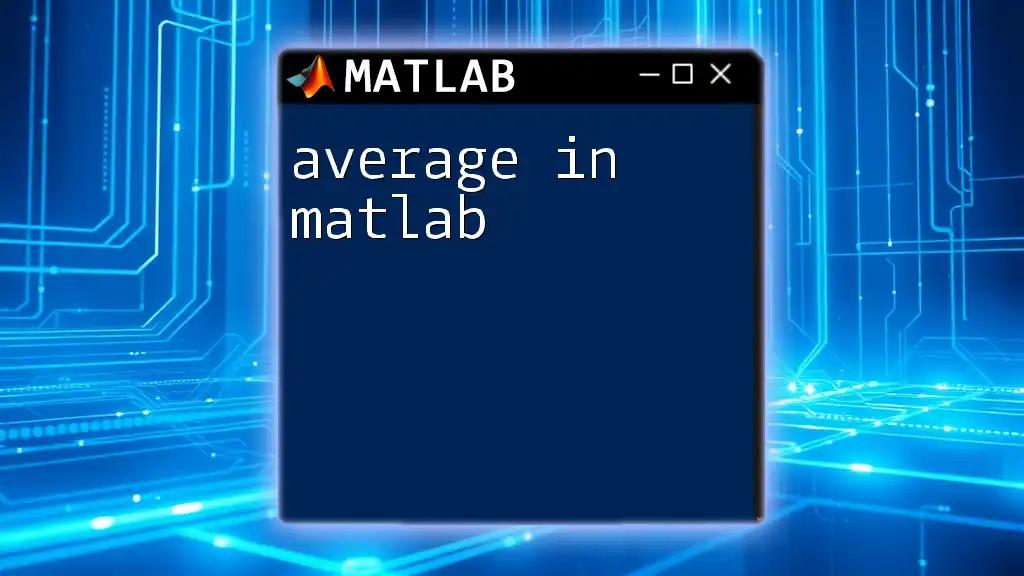
Best Practices for Using Gradients in MATLAB
Tips for Efficient Coding
- Vectorization: Avoid loops. MATLAB is optimized for vector and matrix operations, so using built-in functions like `gradient` is typically faster.
- Memory Management: Be mindful of memory usage, especially when handling large datasets. Preallocating matrices can mitigate unnecessary memory allocation overhead.
Advanced Techniques
For a deeper analysis, consider exploring higher-order derivatives or applying gradient calculations in more complex multi-dimensional spaces. These techniques can be particularly valuable in machine learning and data modeling.
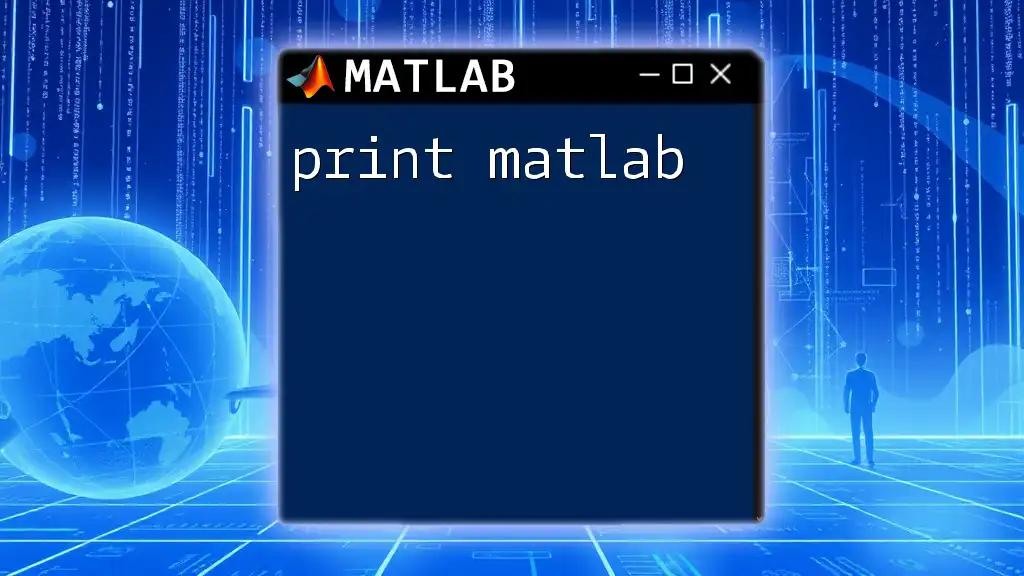
Conclusion
Understanding gradients is foundational in various fields, including engineering, physics, and machine learning. The ability to compute and interpret gradients in MATLAB enhances analytical capabilities and drives innovative solutions to complex problems.
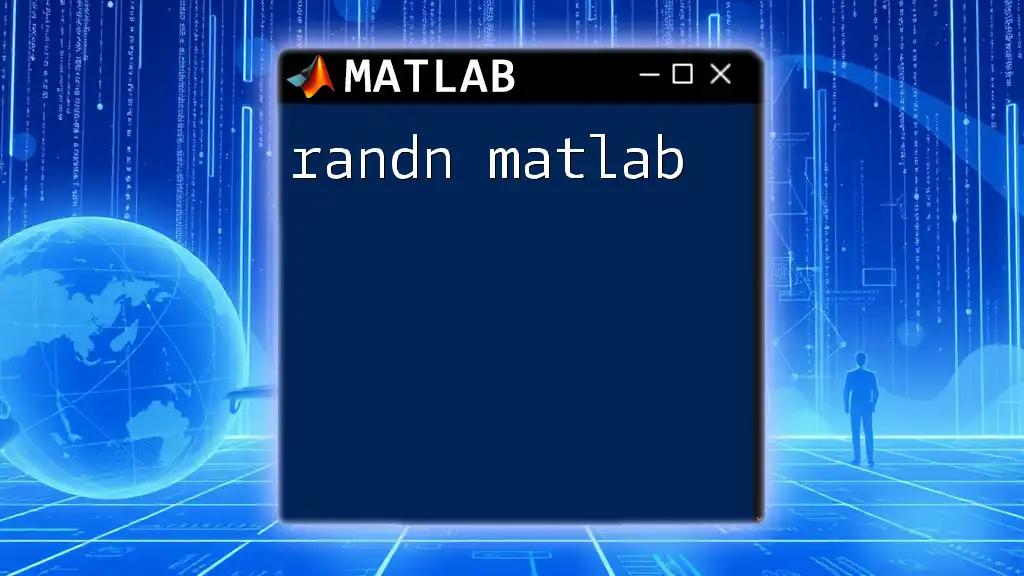
Further Resources
For more in-depth learning, refer to the official MATLAB documentation on the `gradient` function. Additionally, consider exploring textbooks related to numerical methods, optimization, and mathematical modeling that emphasize the application of gradients. Engaging with community forums can also provide invaluable insights and answers to specific questions you may encounter during your learning journey.