To calculate the average of an array in MATLAB, you can use the `mean` function, which quickly computes the arithmetic mean of the elements in the provided array.
Here's a sample code snippet:
data = [1, 2, 3, 4, 5];
average_value = mean(data);
disp(average_value);
Understanding Averages
Types of Averages
When working with data analysis, different methods to calculate averages play a crucial role. Three common types of averages are:
- Mean: The arithmetic average, computed by adding all numbers together and dividing by the count.
- Median: The middle value in a sorted dataset, used especially to mitigate the impact of outliers.
- Mode: The most frequently occurring value in a dataset, highlighting popular trends or repeated measurements.
Understanding when to use each type of average is essential. For instance, the mean is helpful in a normal distribution but can be misleading if the data contains outliers. In contrast, the median provides a better central tendency measure when outliers are present.
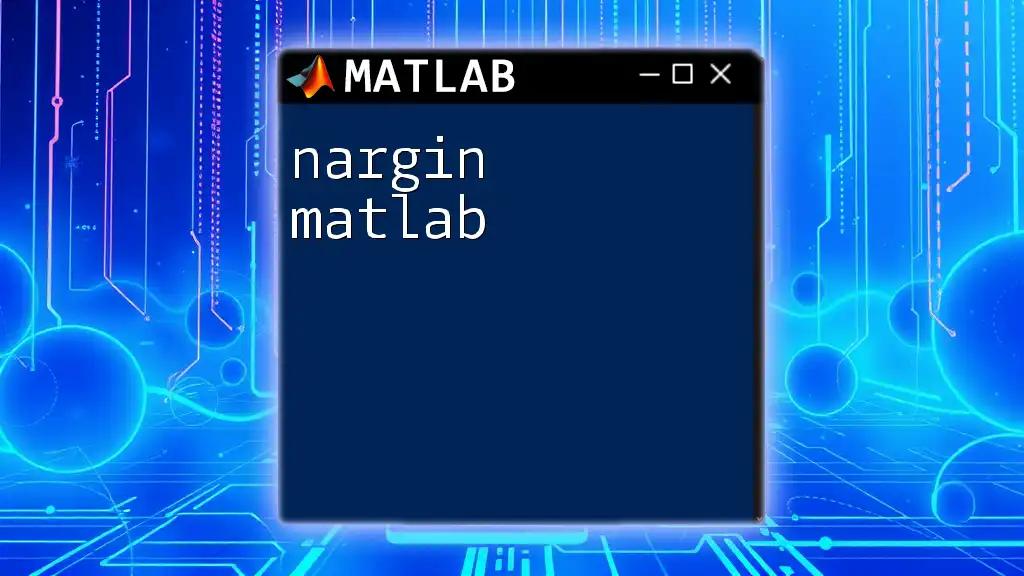
Getting Started with MATLAB
Overview of MATLAB Environment
Before diving into specific commands, familiarize yourself with the MATLAB environment. The interface consists of:
- Command Window: Where you execute commands interactively.
- Script Editor: For writing and saving scripts that contain multiple lines of code.
- Workspace: A view of all current variables.
To create a new script, navigate to the MATLAB interface and open the Script Editor, where you can write and test your commands.
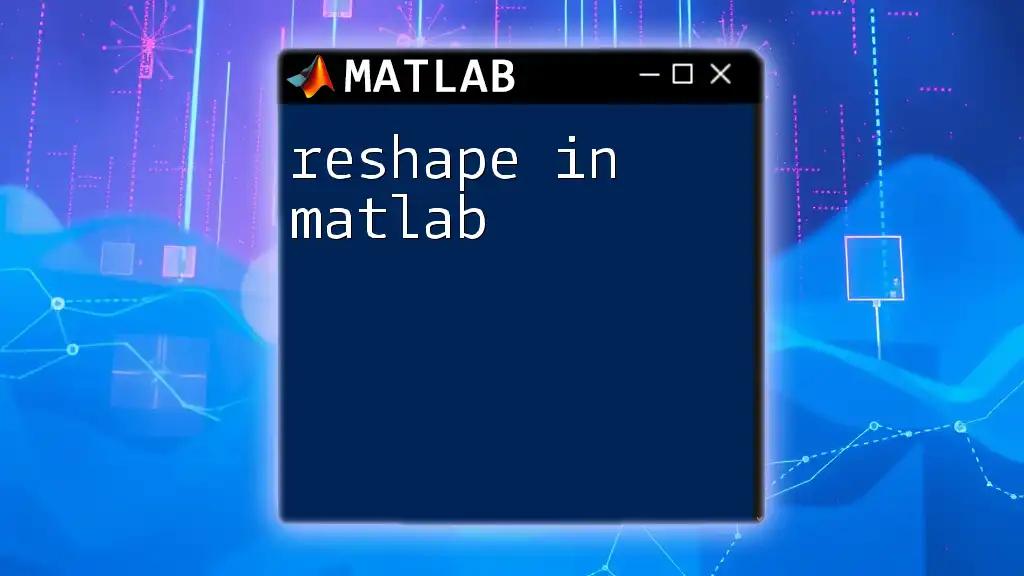
Mathematical Representation of Average
Mean Calculation
The mean is mathematically represented as:
\[ \text{Mean} = \frac{\sum_{i=1}^{n} x_i}{n} \]
where \( x_i \) represents each value in the dataset and \( n \) is the number of data points. The mean is significant because it provides a single measure of central tendency that summarizes a dataset efficiently.
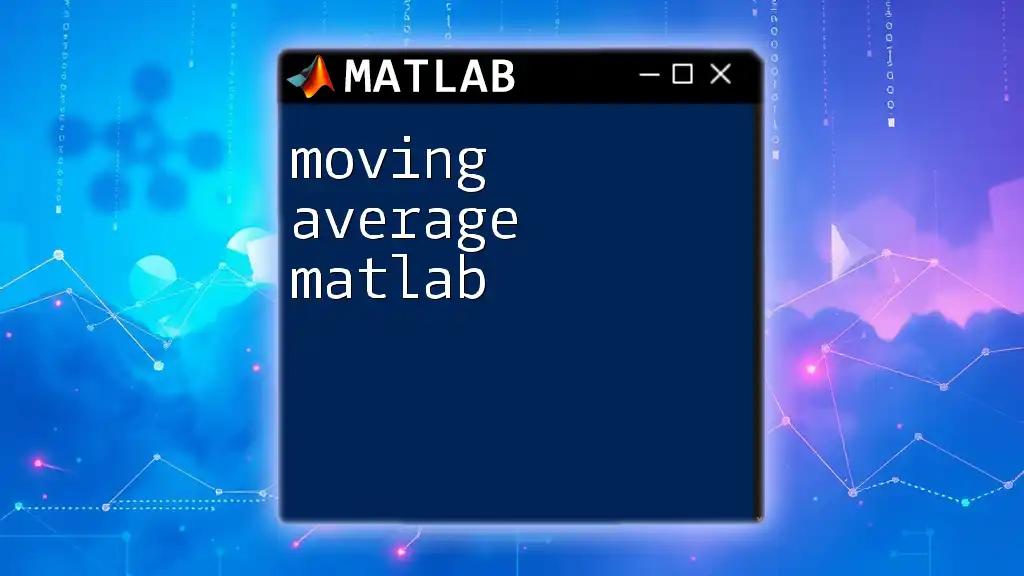
Calculating the Mean in MATLAB
Basic Mean Calculation
To compute the mean in MATLAB, you can use the built-in `mean()` function. This function simplifies the process drastically.
Consider the following example:
data = [3, 7, 5, 2, 8];
average = mean(data);
disp(average);
In this code snippet, we define an array `data`, calculate its mean using `mean(data)`, and display the result. Here, the mean is \(5.0\), which represents the central value of the dataset.
Mean of Rows and Columns
When dealing with matrices, you can compute the mean for rows or columns by specifying dimensions in the `mean()` function.
Here’s how you can do it:
matrixData = [1, 2, 3; 4, 5, 6];
rowAverage = mean(matrixData, 2);
columnAverage = mean(matrixData, 1);
disp(rowAverage);
disp(columnAverage);
In this snippet, `mean(matrixData, 2)` calculates the mean for each row, while `mean(matrixData, 1)` computes the mean for each column. This is essential when analyzing multi-dimensional data.
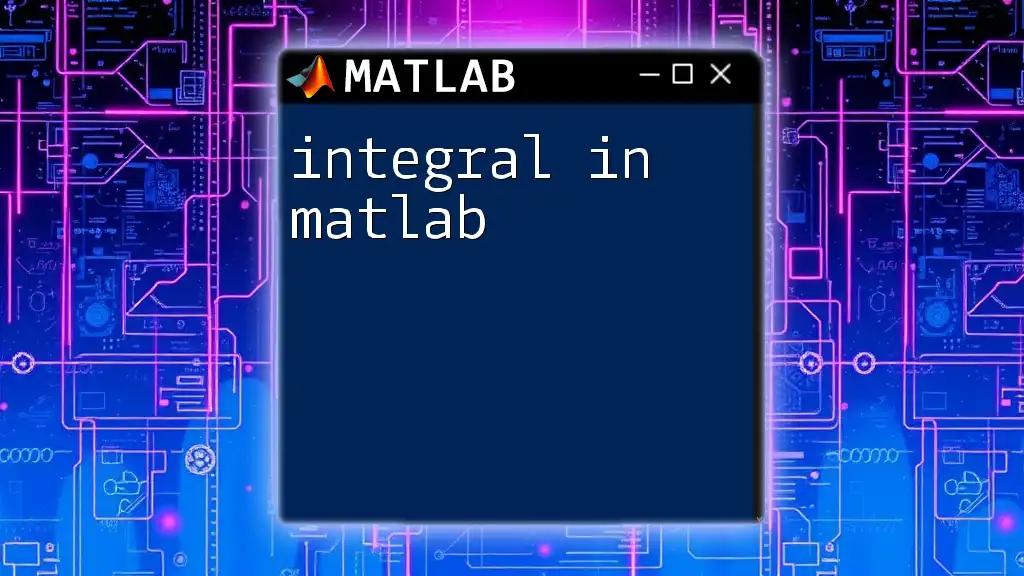
Advanced Mean Calculations
Weighted Mean
Sometimes, not all observations contribute equally to the average. In such cases, the weighted mean is a better measure. The weighted mean is calculated as:
\[ \text{Weighted Mean} = \frac{\sum_{i=1}^{n} (x_i \cdot w_i)}{\sum_{i=1}^{n} w_i} \]
where \( w_i \) is the weight assigned to each observation. The MATLAB implementation can be as follows:
values = [3, 5, 8];
weights = [0.1, 0.3, 0.6];
weightedAverage = sum(values .* weights) / sum(weights);
disp(weightedAverage);
In this example, the weighted mean accounts for the different influences of each data point, providing a more accurate reflection of the dataset's central tendency when weights are applied.
Handling Missing Data
Handling NaN (Not a Number) values is critical when calculating averages. Ignoring NaN values helps in avoiding data corruption. The `mean()` function provides an option to skip NaN values easily:
dataWithNaN = [1, 2, NaN, 4];
averageIgnoringNaN = mean(dataWithNaN, 'omitnan');
disp(averageIgnoringNaN);
By specifying `'omitnan'`, the mean is computed based on the available data only, resulting in a robust average that reflects the dataset accurately.

Calculating Median in MATLAB
The median is another essential measure of central tendency, especially in skewed distributions. Using MATLAB's `median()` function is straightforward.
For example:
data = [1, 3, 3, NaN, 4, 5];
medValue = median(data, 'omitnan');
disp(medValue);
This snippet calculates the median while skipping any NaN values, ensuring a correct representation of the dataset's center.
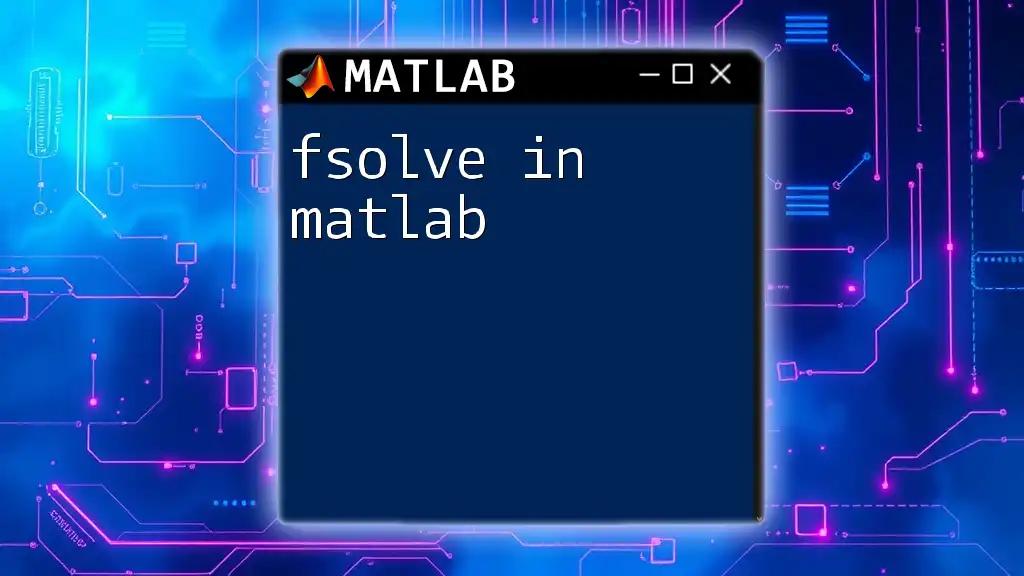
Calculating Mode in MATLAB
To find out the most common data point in your dataset, MATLAB offers the `mode()` function.
Here's how you can use it:
data = [1, 1, 2, 3, 4, 4, 4];
modeValue = mode(data);
disp(modeValue);
In this code snippet, the mode is determined effectively, revealing the most frequently occurring number in the dataset, which can be invaluable for categorical data analysis.
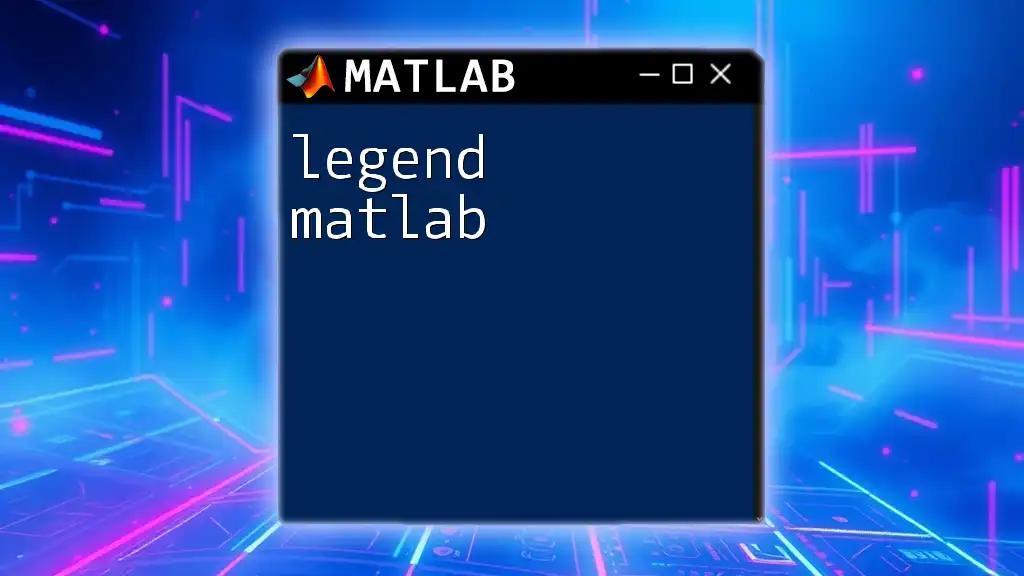
Visualizing Average Values
Visualizing the data alongside average values can enhance understanding and impact decision-making. Graphical representation gives insights into trends and distributions clearly.
An example of plotting averages using a bar chart is as follows:
data = [5, 2, 3, 8, 1];
avgValue = mean(data);
bar(data);
hold on;
yline(avgValue, '--r', 'Average');
hold off;
In this example, the bar chart displays the dataset, while the average is overlaid as a dotted red line, facilitating immediate recognition of how the data compares to the average value.
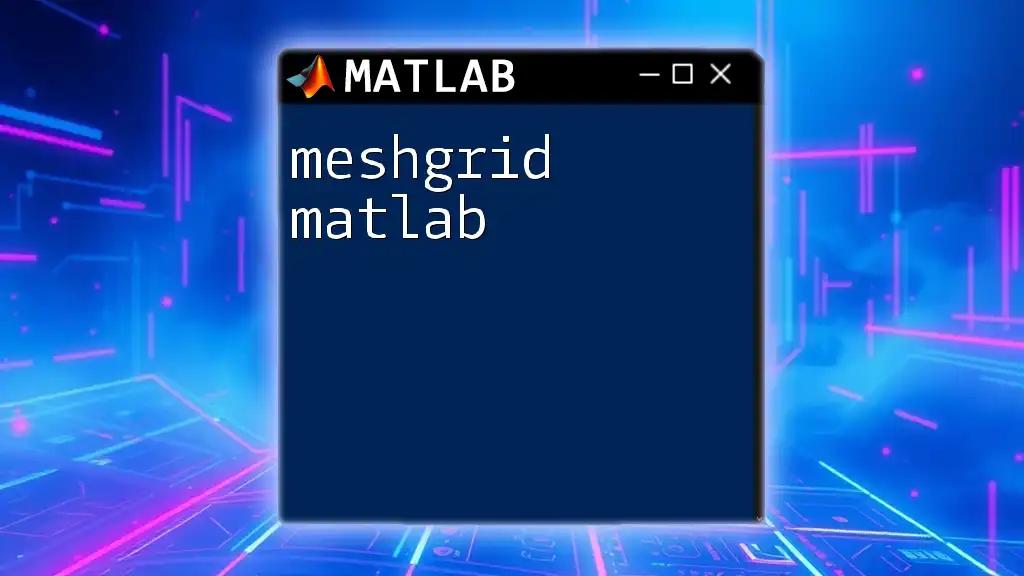
Conclusion
In summary, computing the average in MATLAB is straightforward with built-in functions such as `mean()`, `median()`, and `mode()`. Each of these functions provides unique insights into your dataset, tailored for various applications.
Practicing these commands in different scenarios will solidify your understanding and empower you to achieve proficient data analysis results in MATLAB. Explore further learning resources and engage with communities to enhance your skills in this invaluable tool for data analytics.