Feedback in MATLAB refers to the process of using the output of a system to influence its operation, often employed in control systems to maintain desired performance.
Here's a simple code snippet illustrating feedback in a control system using a proportional controller in MATLAB:
% Define system parameters
Kp = 1; % Proportional gain
setpoint = 5; % Desired value
current_value = 0; % Initial value
dt = 0.1; % Time step
% Simulate feedback control loop
for t = 0:dt:10
error = setpoint - current_value; % Calculate error
control_signal = Kp * error; % Calculate control signal
current_value = current_value + control_signal * dt; % Update current value
fprintf('Time: %.1f, Current Value: %.2f\n', t, current_value);
end
Understanding Feedback Theory
What is Feedback?
Feedback in control systems refers to the process of using a portion of the output of a system to influence its input. This mechanism can greatly alter a system's behavior, ensuring desired performance or maintaining stability. Feedback can be classified into two main types: positive feedback, which amplifies errors or deviations, and negative feedback, which counters them. Understanding these types is crucial, as they play distinct roles in system stability and performance.
Feedback Loops in Control Systems
In control systems, feedback loops are essential for achieving control objectives. A feedback loop typically consists of:
- Input: The initial signal or command sent to the system.
- Process: The operations that the system performs in response to the input.
- Output: The resulting actions or signals produced by the system.
- Feedback Path: The path through which the output is monitored and fed back to the input.
Open-loop systems operate without using feedback. They do not adjust their actions based on the output, making them simpler but less flexible. In contrast, closed-loop systems utilize feedback to make corrections based on the output, leading to enhanced stability and performance.
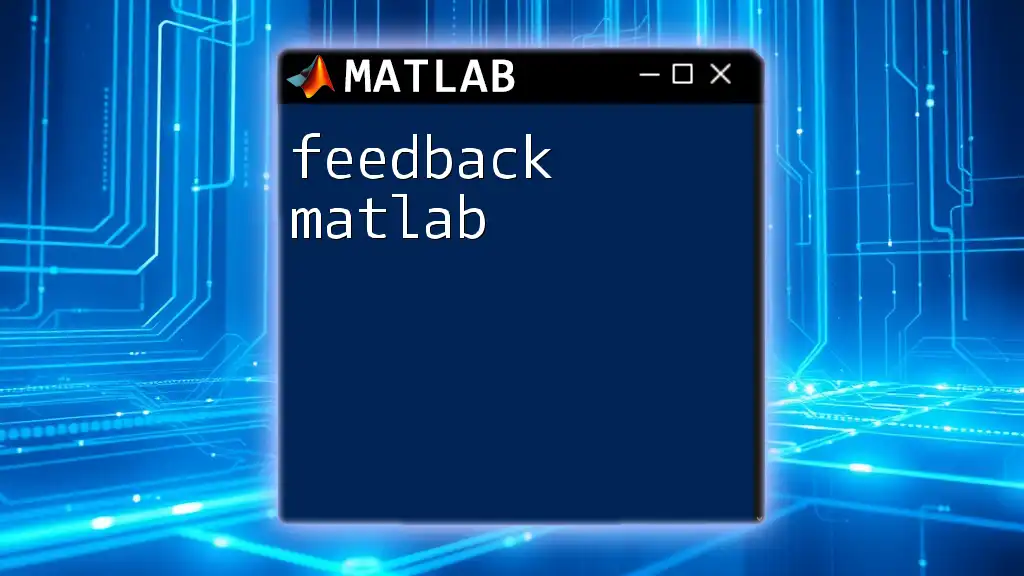
Setting Up Feedback in MATLAB
Essential MATLAB Commands for Feedback Systems
The core function for implementing feedback in MATLAB is the `feedback` function. This function allows you to create a closed-loop control system from a given transfer function. The basic syntax is:
closed_loop_sys = feedback(G, H)
Where `G` is the forward path transfer function, and `H` is the feedback transfer function.
Example Code Snippet for a Simple Feedback System:
To illustrate the concept, you can create a basic feedback system using the following code:
num = [1]; % Numerator for the system
den = [1, 10]; % Denominator for the system
sys = tf(num, den); % Continuous-time transfer function
closed_loop_sys = feedback(sys, 1); % Unity feedback
In this example, we define a simple first-order system with a transfer function and then apply unity feedback to produce a closed-loop system.
Creating a Simple Feedback Model
To build a feedback model step-by-step, follow these guidelines:
- Define Transfer Functions: Use the `tf` command to create the system's transfer function, as shown in the previous snippet.
- Create the Closed-Loop System: Utilize the `feedback` function to construct the closed-loop system.
- Visualize the System: Plot the step response or other characteristics to understand the system better.
For a more recent example, consider a system defined as follows:
num = [2]; % Numerator for the system
den = [1, 5]; % Denominator for the system
sys = tf(num, den); % Create transfer function
closed_loop_sys = feedback(sys, 1); % Create closed-loop system
% Visualize the Step Response
step(closed_loop_sys);
title('Step Response of the Closed Loop System');
Analyzing Feedback Systems
Stability Analysis
Stability is a primary concern when designing feedback systems. Two significant methods to determine stability in MATLAB include the Root Locus Method and Bode Plots.
- Root Locus Method: This graphical technique allows you to visualize the location of closed-loop poles as system parameters vary. You can generate a root locus plot using:
rlocus(closed_loop_sys);
title('Root Locus of Closed Loop System');
This method helps in assessing how changes to system parameters impact stability.
- Bode Plots: These plots provide frequency response data, which is crucial for stability analysis. Use the `bode` function:
bode(closed_loop_sys);
title('Bode Plot of Closed Loop System');
These visualizations allow you to determine gain and phase margins, essential for ensuring system stability.
Time Response Analysis
Understanding how your system reacts over time is critical. The step response is one of the most common ways to evaluate this aspect. Using the `step` function, you can assess how well your system tracks a step input:
step(closed_loop_sys);
title('Step Response of Closed Loop System');
This plot illustrates the system's performance, demonstrating characteristics like rise time, settling time, and overshoot.
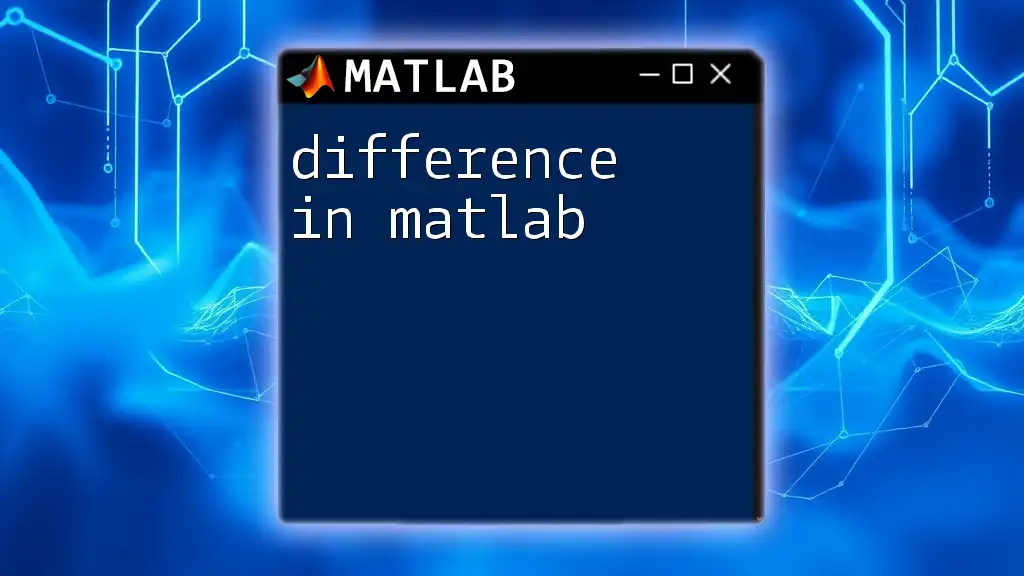
Advanced Feedback Techniques
Cascaded Feedback Systems
A cascaded feedback system involves multiple control elements in series, where the output of one is the input of the next. Such configurations are beneficial for complex systems requiring layered controls. Implement them in MATLAB by defining transfer functions for each stage and combining them with the `feedback` function.
PID Control in Feedback Systems
PID controllers (Proportional, Integral, Derivative) are among the most practical controllers used in feedback systems. They combine three control actions to enhance system performance. To create a PID controller in MATLAB, utilize the `pid` function:
Kp = 1; Ki = 1; Kd = 0.1; % Gain values
pid_controller = pid(Kp, Ki, Kd);
closed_loop_pid = feedback(pid_controller * sys, 1); % Feedback with PID controller
This code snippet creates a PID controller and incorporates it into the feedback loop, significantly improving the system response.
Adaptive Feedback Systems
Adaptive feedback systems dynamically adjust their parameters in response to changing conditions in real-time. Techniques utilize algorithms that modify gain values to suit system demands. MATLAB provides various tools, like System Identification and Control System Toolbox, for developing and testing adaptive control algorithms efficiently.
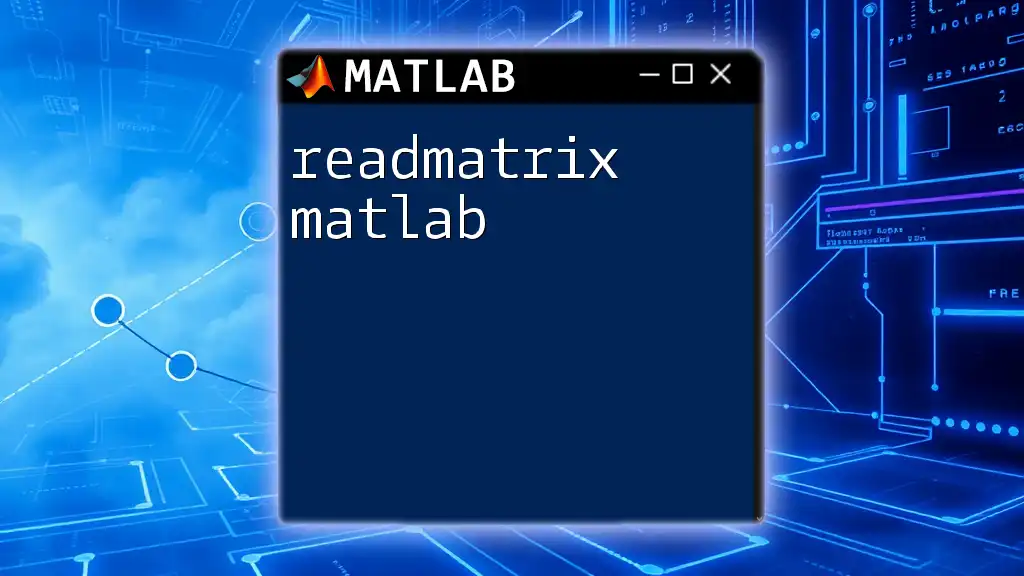
Practical Applications of Feedback in MATLAB
Feedback mechanisms are extensively used across various fields, from robotics to aerospace engineering. For instance, a drone's stability relies heavily on feedback loops to maintain altitude and navigate effectively. By simulating these scenarios in MATLAB, engineers can extrapolate data and design robust systems.
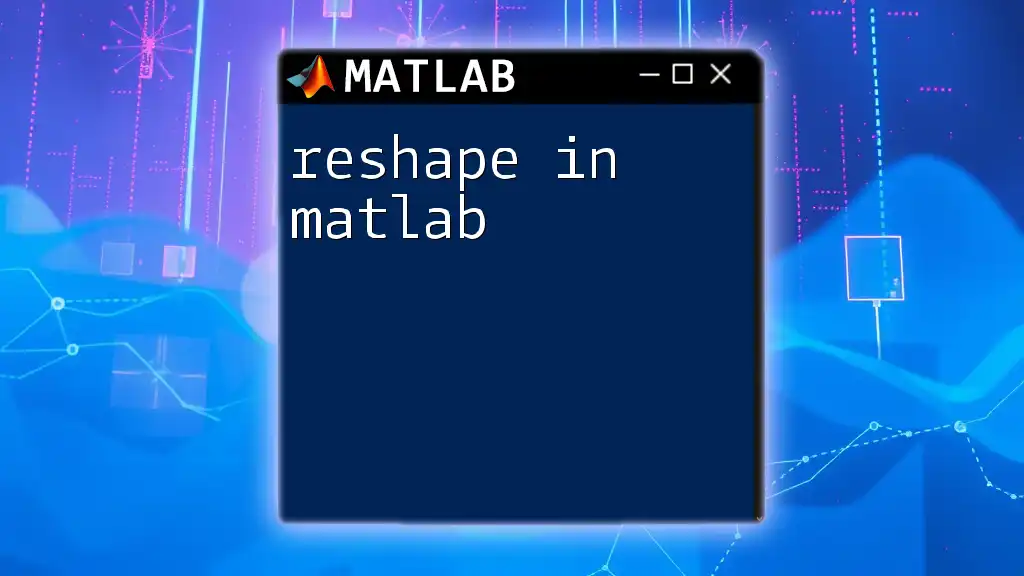
Debugging Feedback Systems in MATLAB
Common Issues and Solutions
When working with feedback systems, several challenges may arise:
- Incorrect Transfer Function Definition: Ensure you correctly define your numerator and denominator.
- Stability Problems: Utilize the root locus and Bode plots to track down instability in your closed-loop system.
- Time Delays: Consider implementing compensators or filters to manage delays that deteriorate system performance.
Use debugging strategies such as systematically breaking down your system into simpler components, validating each part's functionality before proceeding to more complex integrations.
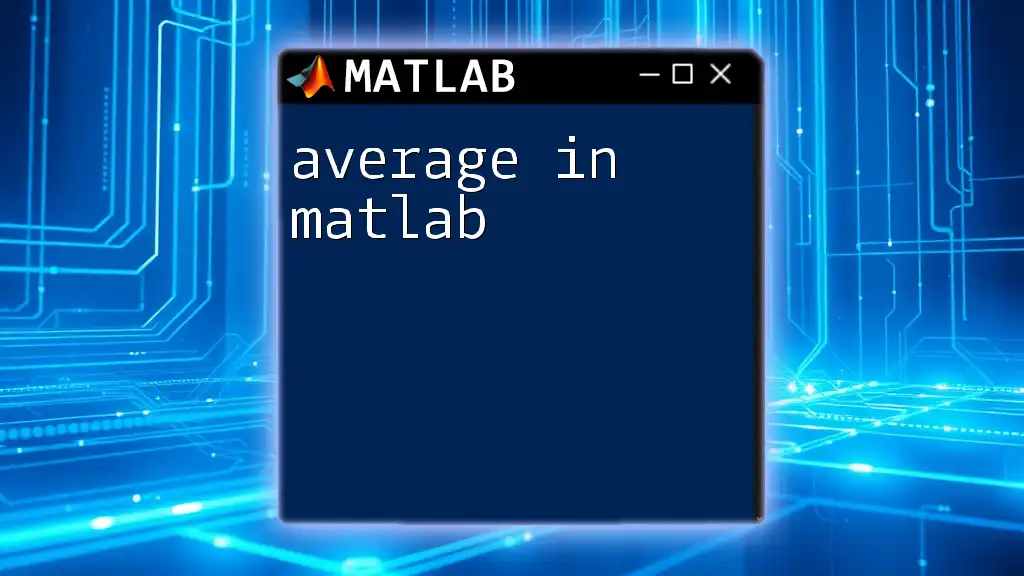
Conclusion
Feedback in MATLAB is at the heart of system control, enabling robust designs and simulations. Mastering feedback principles enhances your control systems’ design and analysis, making it essential for aspiring engineers and programmers to grasp.
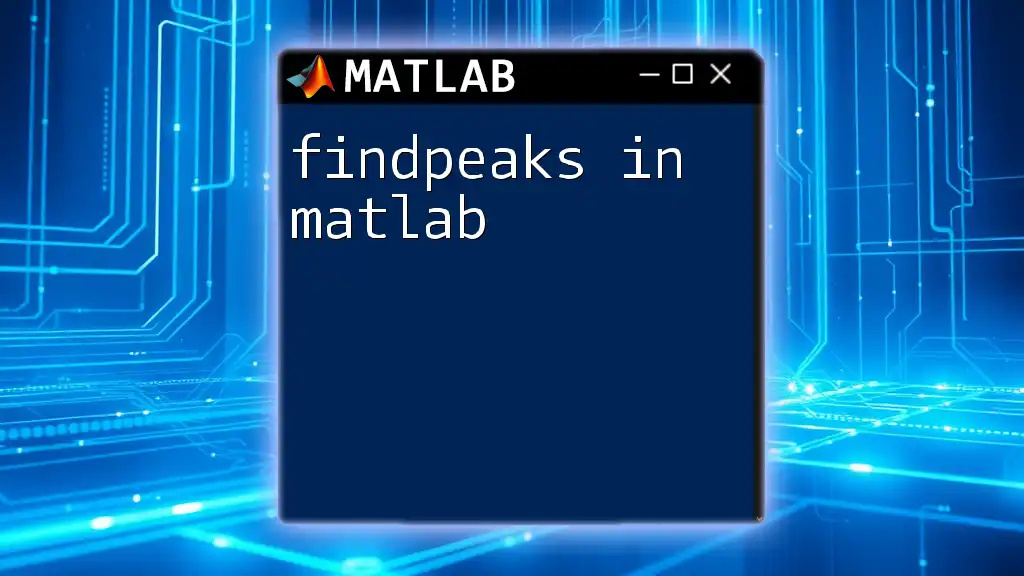
Additional Resources
To deepen your understanding, consider exploring MATLAB documentation, online forums, and literature focused on control systems. They provide valuable knowledge on advanced topics and best practices.
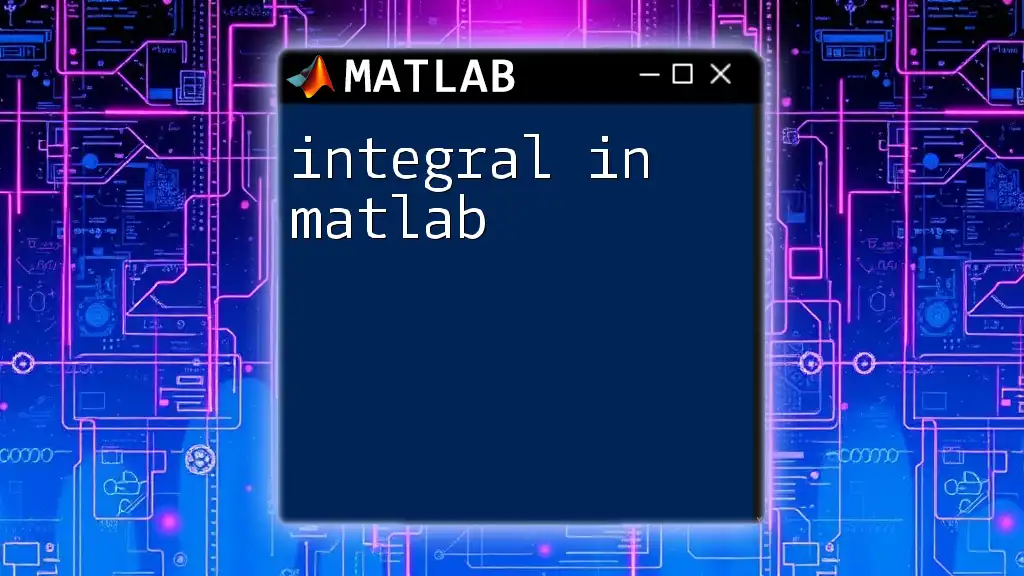
Call to Action
Now that you have a comprehensive understanding of feedback in MATLAB, it's your turn to experiment with creating and analyzing feedback systems. Sign up for our courses or tutorials to gain hands-on experience and mastery over this essential topic.
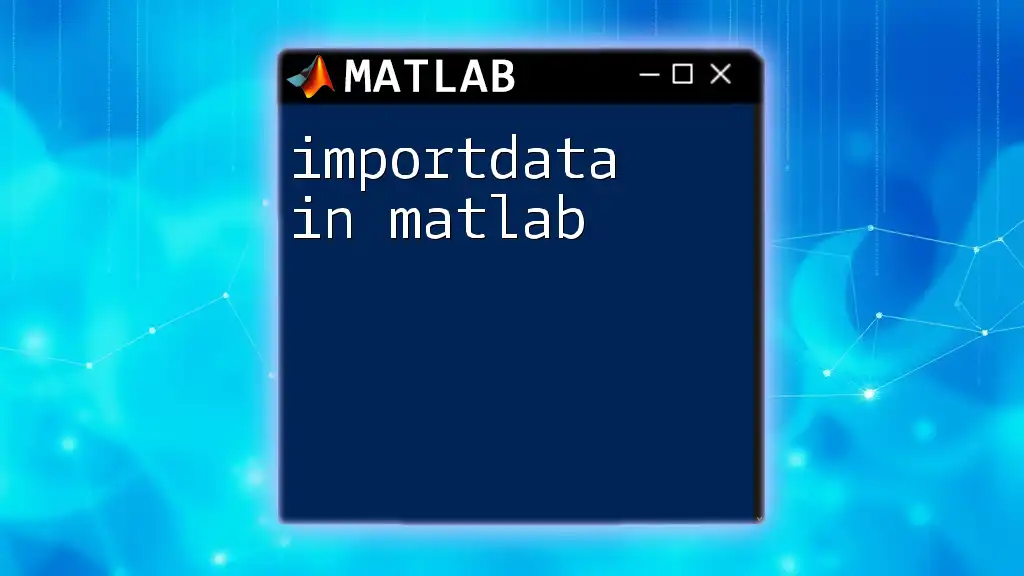
Appendix
Glossary of Terms
- Feedback: Process of returning a portion of the output to the input to influence a system's behavior.
- Open-loop System: A control system that does not utilize feedback.
- Closed-loop System: A control system that uses feedback to adjust its operations.
- PID Controller: A control loop feedback mechanism widely used in industrial control systems.