In MATLAB, the `linestyle` property allows you to customize the appearance of lines in a plot, such as changing them to solid, dashed, or dotted.
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
plot(x, y1, 'r--', x, y2, 'b:'); % 'r--' for red dashed line, 'b:' for blue dotted line
Understanding Line Styles in MATLAB
What is a Line Style?
A line style is a visual representation of data in plots that provides clarity and distinction between different data series. In MATLAB, various line styles such as solid, dashed, dotted, and dash-dot help in varying the appearance of plots, allowing the viewer to easily differentiate between multiple datasets. Line styles play a critical role in making visualizations more informative and engaging.
Default Line Styles in MATLAB
MATLAB provides a default set of line styles, which include:
- Solid Line (–): The default line style, ideal for presenting individual datasets clearly.
- Dashed Line (--): Useful for representing data that changes significantly, drawing attention to trends.
- Dotted Line (:): A less aggressive style suited for auxiliary data or subtle changes.
- Dash-Dot Line (-.): Combines the characteristics of dashed and dotted lines, providing a unique look for representing computed values.
To illustrate these default styles, consider the following code snippet:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
figure;
plot(x, y1, 'b', 'LineWidth', 2); % Solid line for sin
hold on;
plot(x, y2, 'r--', 'LineWidth', 2); % Dashed line for cos
legend('Sine', 'Cosine');
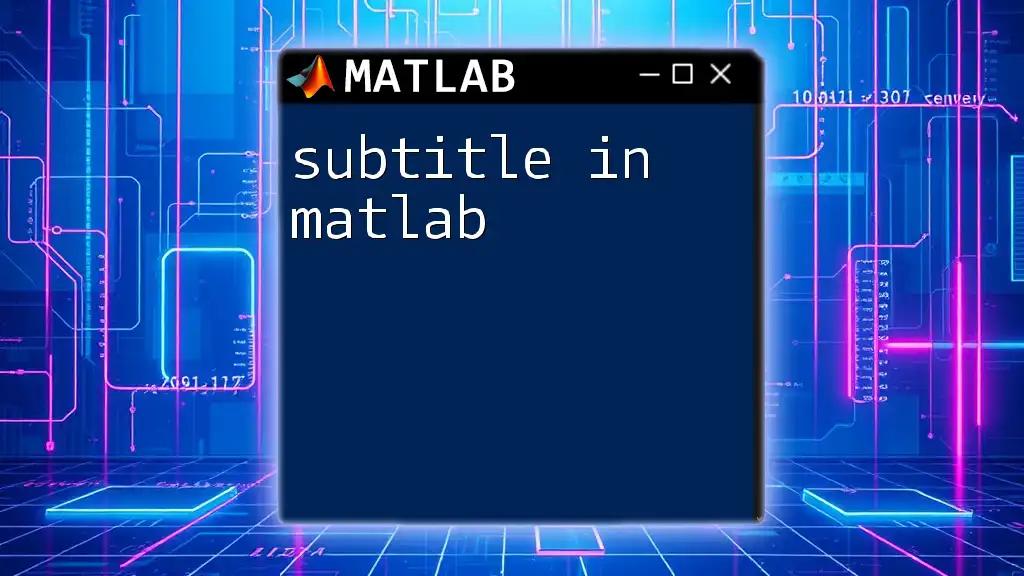
Customizing Line Styles
How to Set Line Styles in MATLAB
Setting line styles in MATLAB is straightforward using the `LineStyle` property. You can specify line styles directly within the plot function or modify them afterwards. The syntax typically involves using the property name followed by the desired style.
Here’s an example of setting line styles in a plot:
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
figure;
plot(x, y1, 'LineStyle', '--', 'Color', 'b', 'DisplayName', 'Sine');
hold on;
plot(x, y2, 'LineStyle', ':', 'Color', 'r', 'DisplayName', 'Cosine');
legend show;
Common Line Style Options
Solid Line
The solid line is used for the most important data points. It provides a clean and continuous representation.
x = 0:0.1:10;
y = exp(x);
figure;
plot(x, y, 'LineStyle', '-', 'Color', 'g');
title('Exponential Function');
Dashed Line
Dashed lines are perfect for indicating a change or a prediction. Here’s how to implement it:
x = 0:0.1:10;
y = log(x + 1);
figure;
plot(x, y, 'LineStyle', '--', 'Color', 'm');
title('Logarithmic Function');
Dotted Line
This style is effective for displaying additional reference data without overpowering the main content.
x = pi/4:0.1:pi/2;
y = tan(x);
figure;
plot(x, y, 'LineStyle', ':', 'Color', 'c');
title('Tangent Function');
Dash-Dot Line
Use dash-dot for representing complex data interactions, creating a balanced view.
x = 0:0.1:10;
y = sin(x) + 0.5;
figure;
plot(x, y, 'LineStyle', '-.', 'Color', 'k');
title('Modified Sine Function');
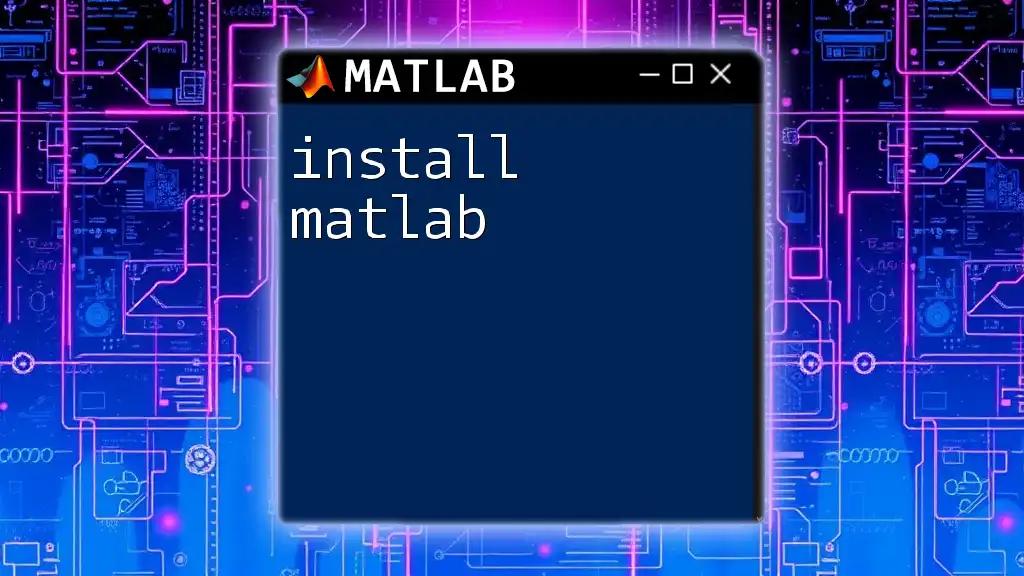
Combining Line Styles with Other Properties
Line Width and Color
Enhancing line styles with different colors and line widths adds another layer of emphasis. The importance lies in balancing visibility and aesthetics, ensuring plots are legible.
Here’s an example demonstrating these combinations:
x = 0:0.1:10;
y = x.^2;
figure;
plot(x, y, 'LineStyle', '-.', 'LineWidth', 2, 'Color', [0.1 0.6 0.8]);
title('Custom Line with Width and Color');
xlabel('X-axis');
ylabel('Y-axis');
Markers and Line Styles
Integrating markers with line styles enhances data visualization by allowing viewers to easily identify critical points along the line.
Consider the following example that utilizes both line style and markers:
x = 0:0.1:10;
y = sin(x);
figure;
plot(x, y, 'o', 'LineStyle', '--', 'Color', 'g', 'MarkerSize', 10);
title('Sine Function with Markers');
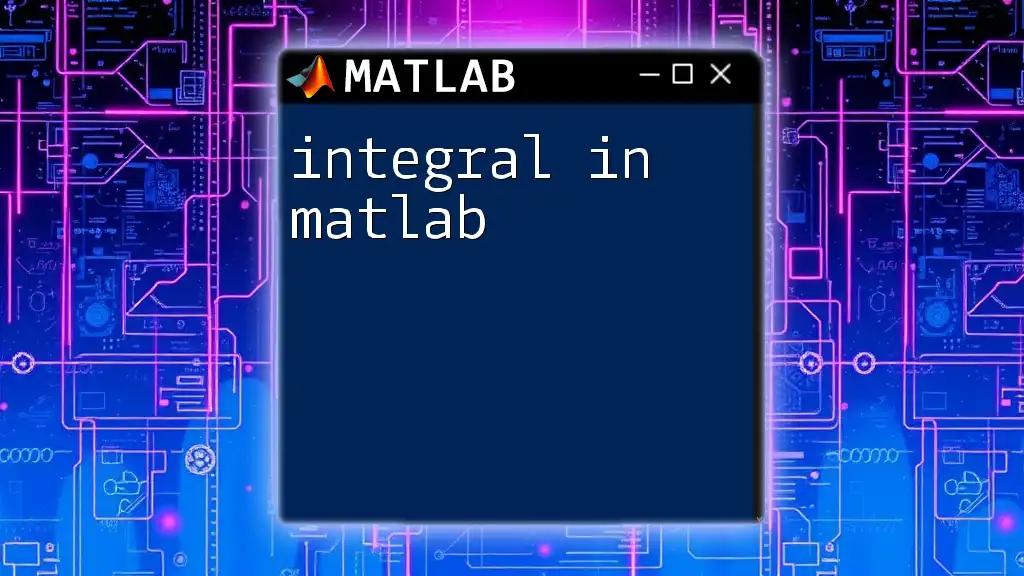
Advanced Customization Techniques
Using Custom Line Styles
Creating custom line styles allows for unique data representation. You can define a specific line style using a combination of characters to achieve your desired effect.
Line Style Properties in Multiple Plots
In more complex visualizations, applying different line styles to multiple datasets enables clear comparison. This functionality is crucial in analyzing relationships between functions.
x = 0:0.1:10;
y1 = sin(x);
y2 = cos(x);
y3 = sin(2*x);
figure;
plot(x, y1, '--', 'Color', 'b', 'DisplayName', 'Sine');
hold on;
plot(x, y2, ':', 'Color', 'r', 'DisplayName', 'Cosine');
plot(x, y3, '-.', 'Color', 'k', 'DisplayName', 'Sine (2x)');
legend show;
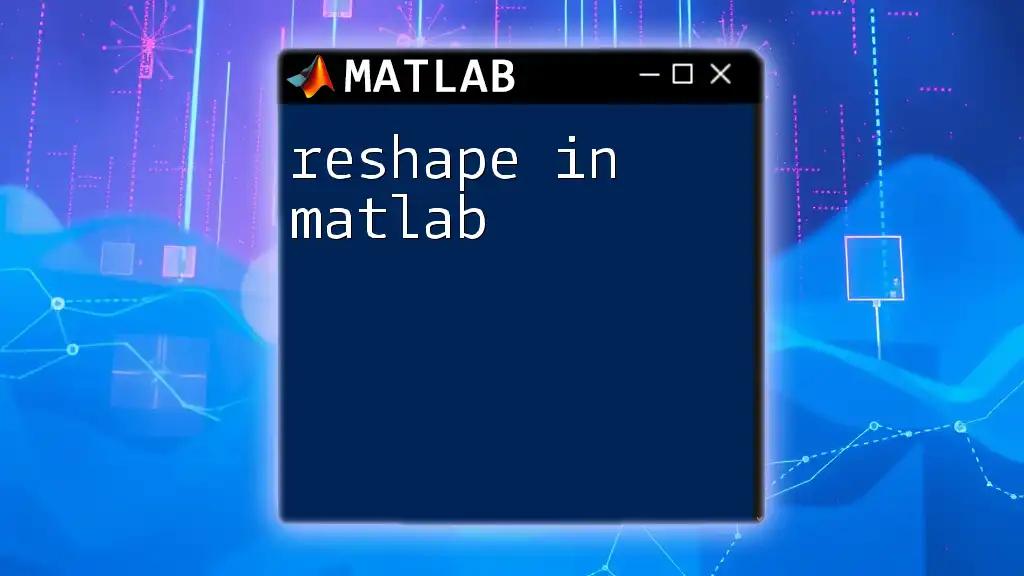
Practical Applications of Line Styles
Distinguishing Data Series
Distinct line styles are crucial for comparative data analysis. When presenting different datasets, using a mix of line styles draws focus on each data series, allowing for better insights.
Visualizing Error Bars with Line Styles
Error bars are an excellent way to indicate variability in your data. By adjusting the line style of both your main data line and the error bars, you enhance comprehension.
Here’s a simple conceptual demonstration:
x = 0:0.1:10;
y = sin(x);
errors = 0.1 * rand(1, length(x));
figure;
errorbar(x, y, errors, 'LineStyle', '--', 'Color', 'b');
title('Sine Function with Error Bars');
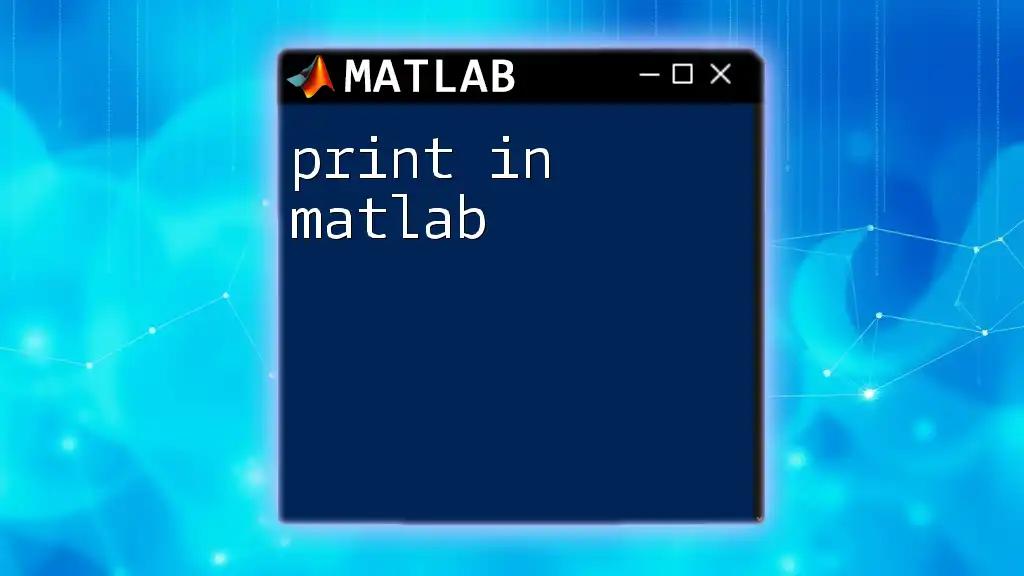
Conclusion
Understanding and using different line styles in MATLAB empowers you to create visually compelling plots that enhance data interpretation. Whether you're presenting complex datasets or simple functions, a well-chosen line style can significantly impact the clarity and effectiveness of your visualizations. Embrace the customization options available and experiment freely with your plots to achieve the best representation of your data.
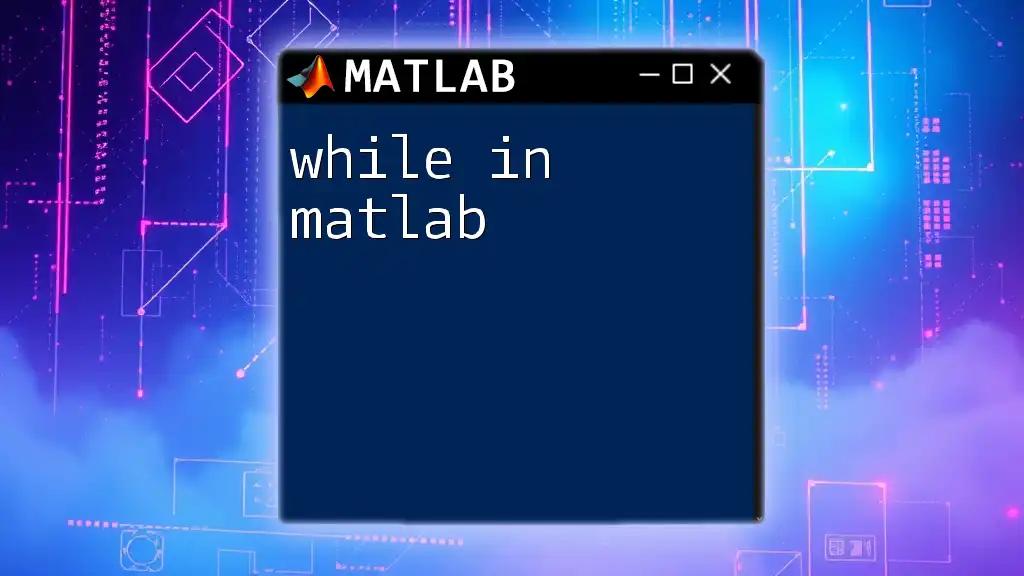
Additional Resources
For further exploration, refer to the official MATLAB documentation. Explore tutorials and online courses to deepen your knowledge and proficiency in MATLAB plotting techniques for superior data visualization.