In MATLAB, a figure is a window where graphical outputs such as plots and charts can be displayed, allowing users to visualize data effectively.
Here’s a simple code snippet to create a figure and plot a sine wave:
x = 0:0.01:2*pi; % Generate x values from 0 to 2π
y = sin(x); % Compute the sine of each x value
figure; % Create a new figure window
plot(x, y); % Plot the sine wave
title('Sine Wave'); % Add a title to the plot
xlabel('x'); % Label x-axis
ylabel('sin(x)'); % Label y-axis
What is a Figure in MATLAB?
A figure in MATLAB serves as a graphical window where visual representations of data are created. These figures are essential for interpreting complex datasets, allowing users to identify trends, outliers, and relationships through visual cues. Figures can take many forms, such as line plots, scatter plots, bar graphs, and histograms, each tailored to present data in the most effective way possible.
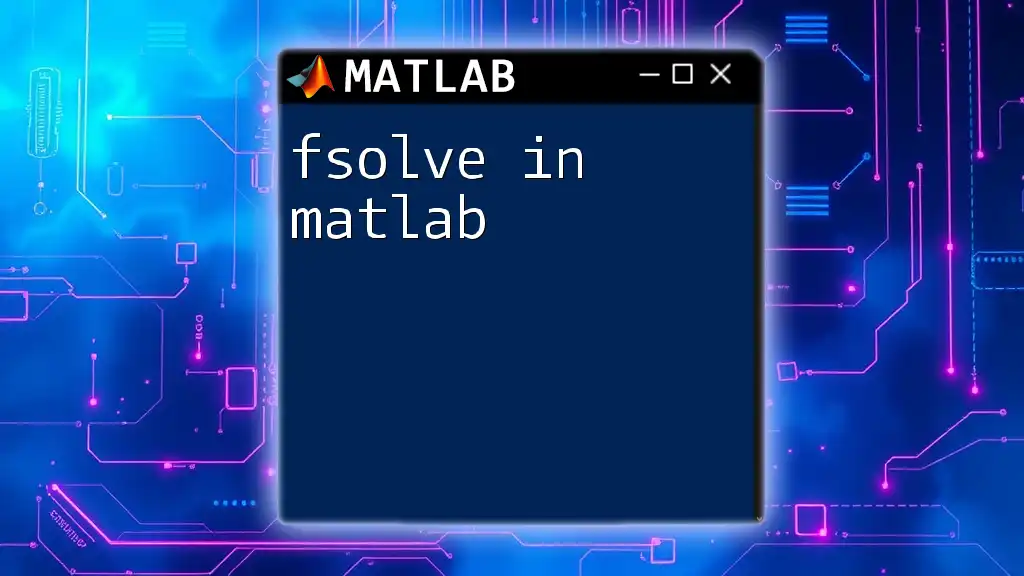
Creating a Figure
Opening a New Figure Window
To create a new figure window, use the `figure` command. This initializes a blank canvas for your graphical representations.
figure;
When this command is executed, a new figure window pops up, ready for your plotting commands. You can have multiple figure windows open simultaneously, with each serving as a unique workspace for your graphics.
Adding a Title to Your Figure
Titles are crucial for providing context to your figures. Use the `title` function to add descriptive text to your figure.
title('My First Figure Title');
Crafting effective titles can enhance understanding. Ensure your title succinctly describes the content and focus of the plot.
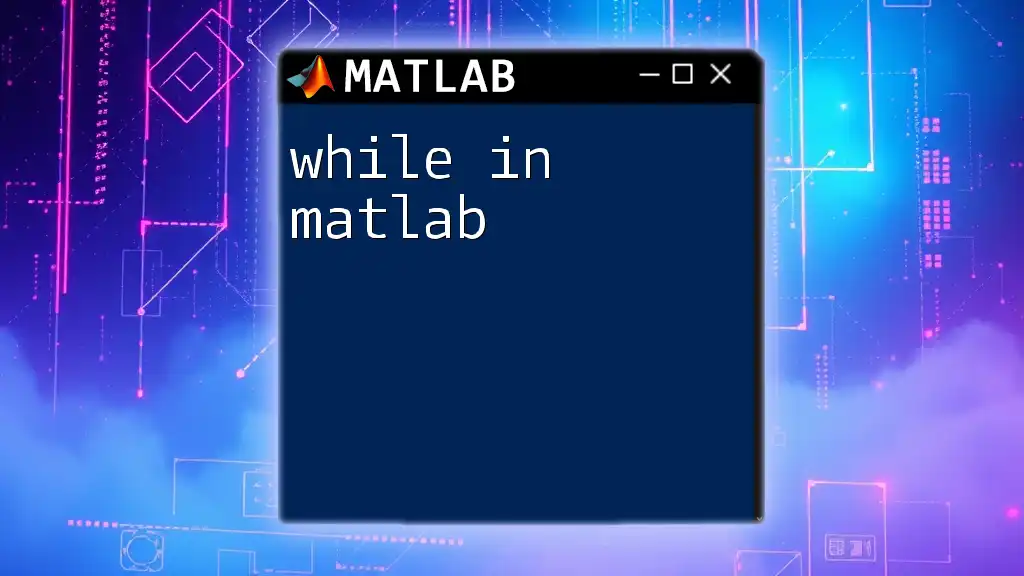
Types of Figures in MATLAB
2D Line Plots
Line plots are one of the most common ways to visualize continuous data. They are particularly useful for showcasing trends over time or other continuous variables.
Here's how to create a simple sine wave line plot:
x = 0:0.1:10;
y = sin(x);
plot(x,y);
title('Sine Wave');
xlabel('X values');
ylabel('Y values');
In this example, the `plot` function generates a smooth curve representing the sine of x values, while the `xlabel` and `ylabel` functions label the x-axis and y-axis respectively.
Bar Graphs
Bar graphs are ideal for comparing categorical data. They visually emphasize differences among various categories.
To create a bar graph in MATLAB, use the following example:
categories = {'Category A', 'Category B', 'Category C'};
values = [5, 10, 15];
bar(values);
set(gca, 'XTickLabel', categories);
title('Bar Graph Example');
In this snippet, `set(gca, 'XTickLabel', categories)` ensures that each bar corresponds correctly to its category, improving clarity.
Scatter Plots
Scatter plots are particularly effective for visualizing the relationship between two continuous variables. They reveal correlations and distributions clearly.
Here's an example of a scatter plot:
x = rand(1, 100);
y = rand(1, 100);
scatter(x, y);
title('Random Scatter Plot');
xlabel('X Random Values');
ylabel('Y Random Values');
This snippet generates random x and y values and plots them, providing a visual representation of their distribution in a two-dimensional space.
Histograms
Histograms are used to represent the distribution of data points. They help illustrate the underlying frequency distribution of a set of continuous data.
To create a histogram, you can use the following code:
data = randn(1, 1000);
histogram(data);
title('Histogram of Random Data');
In this example, `randn` generates random numbers from a standard normal distribution, which are then displayed in a histogram, allowing for an insightful view of the data distribution.
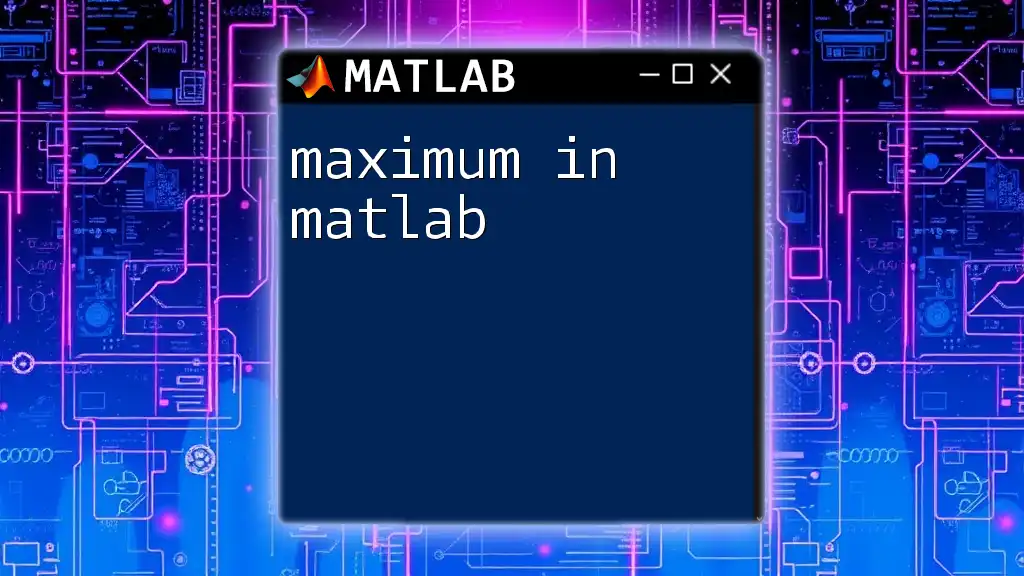
Customizing Figures
Changing Figure Properties
MATLAB allows you to modify various properties of figures, such as color and size, to enhance their appearance and readability.
For instance, to create a figure with specific background color and size, you can use:
fig = figure('Color','w','Position',[100,100,800,600]);
This command creates a white figure with specified dimensions, enhancing visibility and aesthetic appeal.
Adding Legends
Legends provide essential information about different data series in a plot. To create a legend, you can utilize the following code:
plot(x, y, 'r', 'DisplayName', 'Sine Wave');
legend show;
Here, the `'DisplayName'` property adds a label to the plot, which is then presented in the legend, making it easier to distinguish between multiple datasets.
Annotating Figures
Annotations in figures enhance understanding, drawing attention to specific data points or trends.
For example, you can add a text annotation with an arrow as follows:
annotation('textarrow',[0.5 0.5],[0.6 0.8],'String','Peak');
This command inserts a text arrow pointing to a designated location in your figure, helping to highlight significant features in your data visualization.
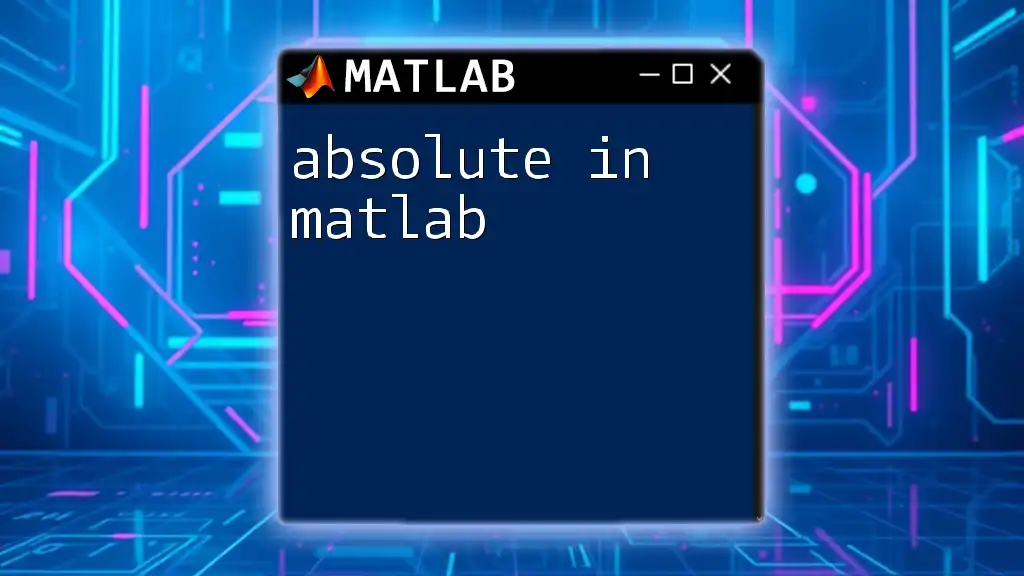
Saving and Exporting Figures
To preserve your work, MATLAB provides functionality for saving figures in various formats. You can save your figure using the `saveas` command, as shown below:
saveas(gcf, 'myFigure.png');
The `gcf` function gets the current figure, which you can then export in formats like PNG, JPG, or even PDF, allowing for easy sharing or printing of your visual results.
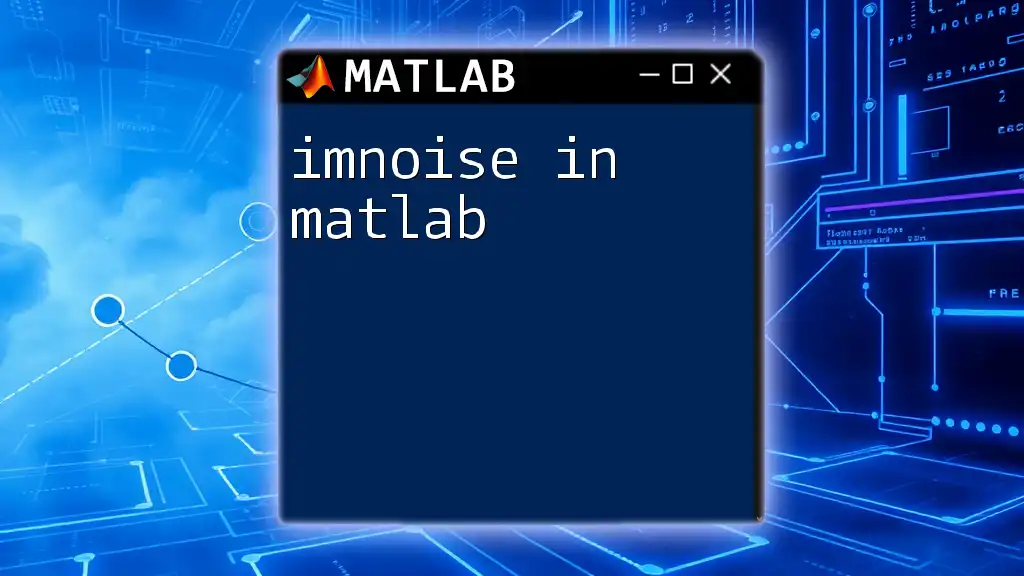
Common Mistakes and Troubleshooting
Issues with Figure Overlapping
A common issue when creating multiple figures is overcrowding in the figure window. To prevent this, use the `hold on` and `hold off` commands.
hold on;
plot(anotherX, anotherY);
hold off;
This ensures that when you plot additional data, it adds to the existing figure without overwriting previous plots.
Handling Data Errors
Errors often arise when data types or dimensions do not match. Understanding the types of data can help you diagnose problems quickly. Always ensure that you prepare the data correctly for the specific plot type you are using.
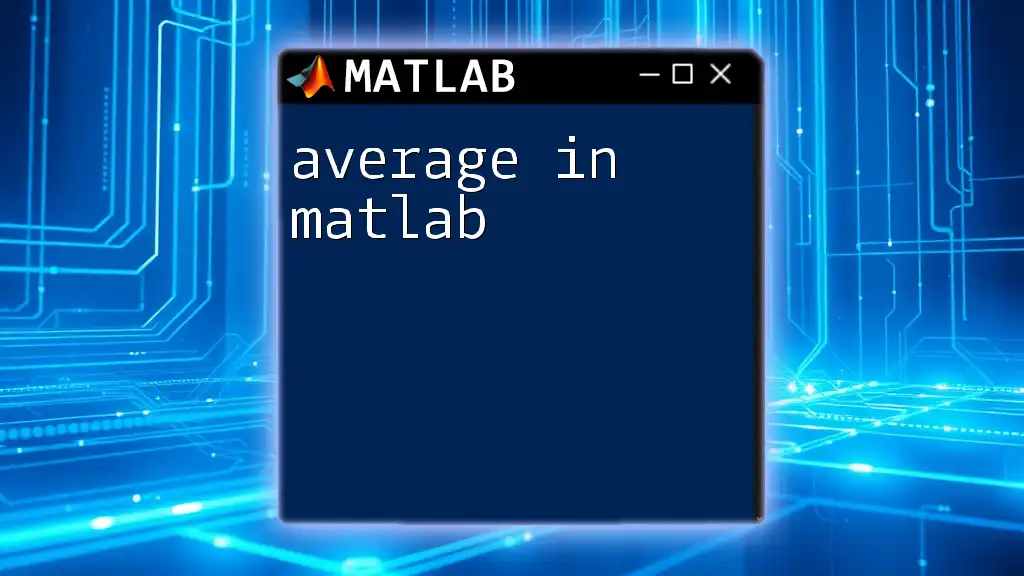
Conclusion
In summary, understanding how to effectively use figures in MATLAB is essential for visual data analysis. Mastering the creation and customization of various types of figures will significantly enhance your data interpretation capabilities. Encouraging practice will reinforce these concepts and lead to more insightful visuals in your MATLAB projects.
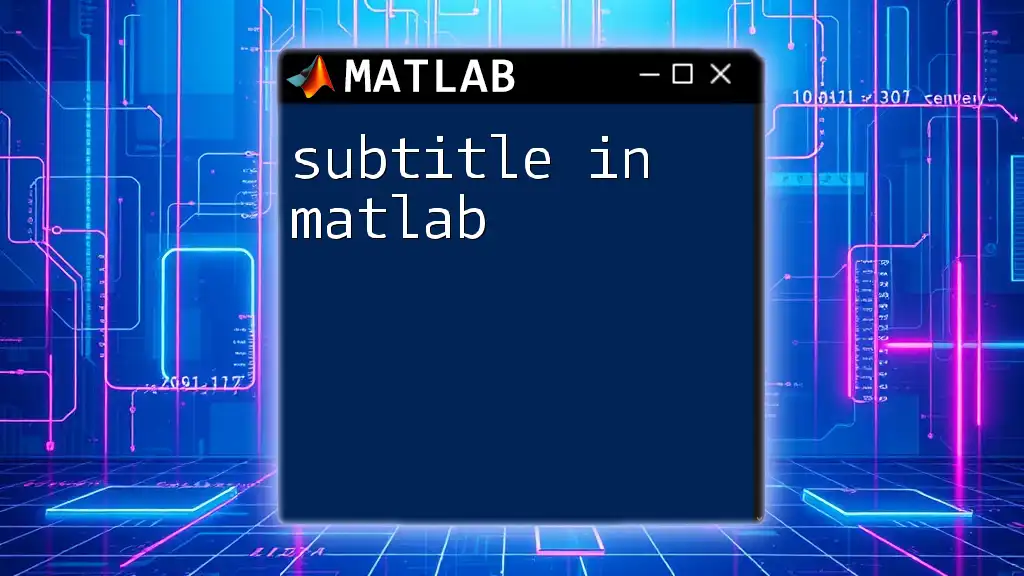
Additional Resources
For further learning, explore the [MathWorks MATLAB Documentation](https://www.mathworks.com/help/matlab/) for detailed insights into figure creation and customization. Additionally, consider enrolling in courses and tutorials specifically designed to enhance your MATLAB skills, focusing on effective data visualization techniques.