Indexing in MATLAB allows you to access or modify specific elements of an array or matrix using their indices, enabling efficient data manipulation.
Here's a simple code snippet demonstrating how to index a matrix:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a 3x3 matrix
element = A(2, 3); % Access the element in the 2nd row and 3rd column (result: 6)
A(1, 1) = 10; % Modify the element in the 1st row and 1st column (A becomes [10, 2, 3; 4, 5, 6; 7, 8, 9])
Understanding Indexing in MATLAB
What is Indexing?
Indexing refers to the method by which elements of arrays, matrices, and other data structures in MATLAB are accessed or modified. It plays a critical role in MATLAB programming as it enables efficient data retrieval and manipulation. Essentially, indexing allows users to specify which particular element of an array or matrix they wish to work with, making it a foundational skill in MATLAB.
Why Indexing Matters in MATLAB
Mastering the art of indexing in MATLAB is essential for several reasons. Performance is one of the most significant factors—efficiently accessing data can drastically reduce the computational time of your scripts. Additionally, effective indexing contributes to code clarity and simplicity, which in turn affects debugging and maintenance positively. When you can manipulate data quickly and clearly, your overall productivity increases.

Types of Indexing in MATLAB
Numeric Indexing
Numeric indexing is the most common method of accessing elements in MATLAB. It involves using positive integers to reference the position of the desired element within an array.
For example:
A = [10, 20, 30, 40, 50];
x = A(3); % Retrieves the third element, which is 30
In this code snippet, `A(3)` returns the third element of the array `A`, demonstrating how simply and effectively numeric indexing works.
Logical Indexing
Logical indexing allows users to index arrays based on conditions rather than specific elements. This method produces a new array by evaluating logical conditions.
For instance:
A = [1, 2, 3, 4, 5];
index = A > 3; % Creates a logical array: [0, 0, 0, 1, 1]
result = A(index); % Returns [4, 5]
Here, the condition `A > 3` generates a logical array that is true for elements greater than 3. The resulting new array contains only the elements meeting that condition. This indexing technique is particularly useful for filtering data.
Cell and Structure Arrays Indexing
Indexing is slightly different when working with cell arrays and structures in MATLAB.
For Cell Arrays: Cell arrays can hold data of varying types and sizes. Each cell can be indexed using curly braces `{}`.
C = {'apple', 'banana', 'cherry'};
fruit = C{2}; % Retrieves 'banana'
In this example, `C{2}` accesses the second cell of the cell array, showcasing how to retrieve non-numeric data.
For Structures: Structures allow you to group related data together. You can access the fields of a structure using the dot `.` notation.
S.name = 'John';
S.age = 30;
name = S.name; % Retrieves 'John'
This shows that using structures can provide clarity and organization, especially when dealing with complex datasets.
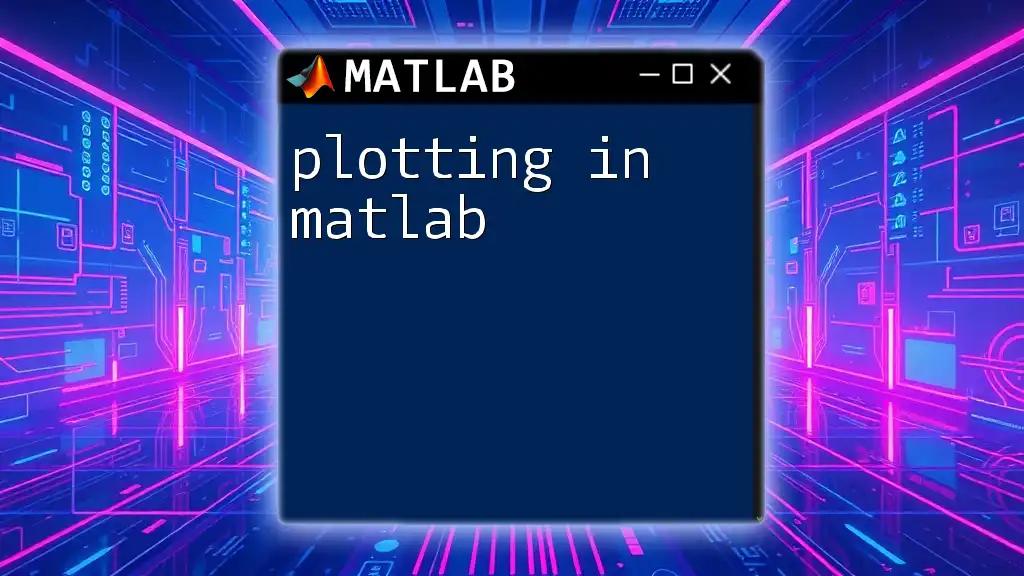
Advanced Indexing Techniques
Multi-dimensional Indexing
In MATLAB, you can work with data in more than two dimensions. Multi-dimensional indexing employs additional indices to specify data positions.
For example:
B = rand(3,4,2); % Creates a 3D array with random values
x = B(2,3,1); % Accesses a specific element in the 3D array
Here, `B(2,3,1)` retrieves an element from the second row, third column of the first slice. Understanding multi-dimensional indexing is crucial for manipulating complex datasets effectively.
Using the `end` Keyword for Indexing
The `end` keyword in MATLAB is a powerful feature that refers to the last index of an array. This can simplify code and enhance its readability.
Consider this example:
A = [1, 2, 3, 4, 5];
lastElement = A(end); % Retrieves the last element, which is 5
By using `A(end)`, you avoid hard-coding the array’s length, making your code more adaptable and robust against changes in array size.
Slicing Arrays
Slicing is a specific indexing technique that allows you to select a contiguous block of data from an array. This method can differ from standard indexing in its approach—rather than accessing individual elements, you access a range.
For instance:
A = [1, 2, 3, 4, 5];
subArray = A(2:4); % Retrieves [2, 3, 4]
In this example, `A(2:4)` extracts the elements from the second to the fourth position in the array. Knowing how to slice arrays effectively can make data manipulation much easier.
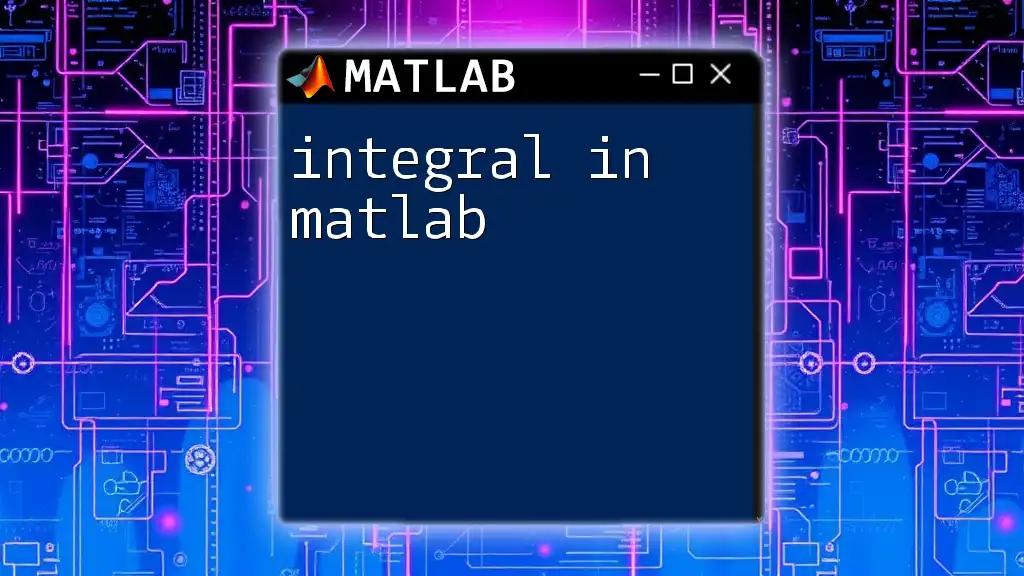
Best Practices for Indexing in MATLAB
Performance Considerations
While indexing in MATLAB is versatile, it’s important to consider the trade-offs between readability and performance. For instance, using logical indexing may be less efficient than numeric indexing for extremely large datasets, especially in loops. Always measure performance while working on significant datasets, and select the indexing technique that strikes a balance between speed and clarity.
Readability and Maintainability
Clarity shouldn't be sacrificed for brevity. To maintain readability, consider commenting your code, using descriptive variable names, and structuring your code logically. This practice is essential when your scripts require maintenance or are shared with colleagues.
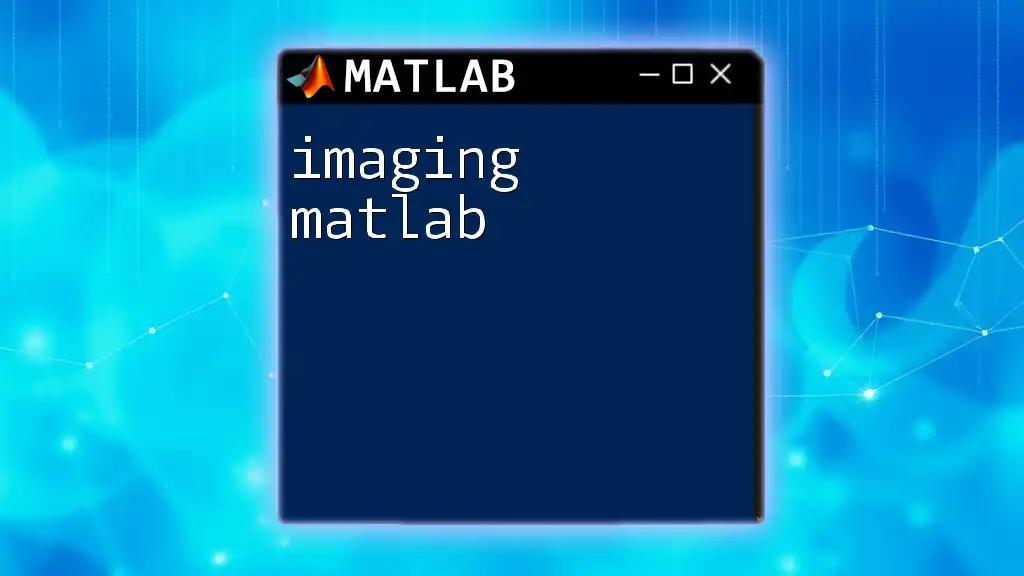
Common Pitfalls in Indexing
Off-by-One Errors
One of the most common errors in programming is the off-by-one error, which occurs when developers mistakenly reference the wrong index. Since MATLAB uses one-based indexing, a common mistake is to assume zero-based indexing, leading to unexpected results.
Working with Empty Arrays
Understanding how to handle empty arrays is pivotal in MATLAB. Indexing into an empty array will yield errors and unexpected behavior.
A = [];
index = A(1); % This will produce an error!
This error highlights the need to always check if your data structures contain elements before attempting to access them.
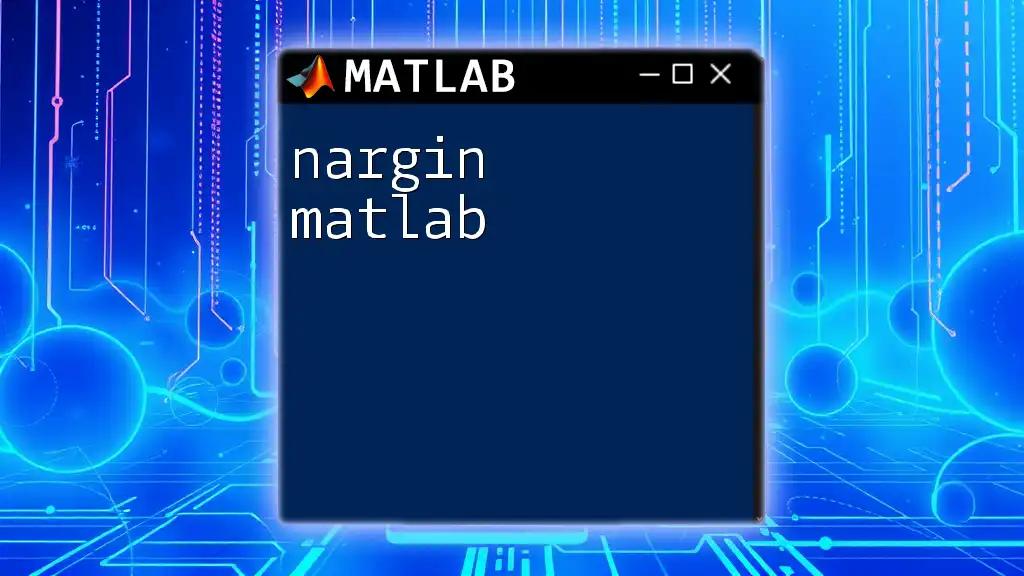
Conclusion
In summary, indexing in MATLAB is a fundamental concept that underlies much of the language's data manipulation capabilities. Understanding various indexing techniques—like numeric, logical, and multi-dimensional indexing—enables users to write more efficient, clear, and maintainable code. By adhering to best practices and avoiding common pitfalls, anyone can become proficient in utilizing indexing to maximize their MATLAB experience.
Resources for Further Learning
To expand your knowledge on indexing in MATLAB, consider exploring additional resources such as online courses, official MATLAB documentation, and community forums. Regular practice in applying what you've learned will solidify your skills, paving the way for more advanced MATLAB programming.
Call to Action
Start integrating these concepts into your MATLAB workflow today! Follow our company for more tips on using MATLAB commands quickly and concisely. Happy indexing!