Fast Fourier Transforms (FFT) in MATLAB allows you to efficiently compute the discrete Fourier transform (DFT) of a sequence, which is essential for analyzing frequency components in signals.
Here’s a simple code snippet using FFT in MATLAB:
% Example: Compute the FFT of a simple sine wave
Fs = 1000; % Sampling frequency
T = 1/Fs; % Sampling period
L = 1000; % Length of the signal
t = (0:L-1)*T; % Time vector
% Create a sine wave
f = 50; % Frequency of the sine wave
signal = 0.5*sin(2*pi*f*t);
% Compute the FFT
Y = fft(signal);
% Compute the two-sided spectrum
P2 = abs(Y/L);
P1 = P2(1:L/2+1);
P1(2:end-1) = 2*P1(2:end-1);
% Frequency axis
f = Fs*(0:(L/2))/L;
% Plotting the FFT
figure;
plot(f,P1);
title('Single-Sided Amplitude Spectrum of Signal');
xlabel('Frequency (f)');
ylabel('|P1(f)|');
Introduction to Fast Fourier Transforms (FFT) in MATLAB
Fast Fourier Transform (FFT) is a powerful algorithm used to compute the Discrete Fourier Transform (DFT) and its inverse efficiently. It’s fundamental in various fields including signal processing, image analysis, and audio processing. The primary benefit of using FFT over other techniques is the drastic reduction in computation required, making it an essential tool for analyzing frequency components of signals.
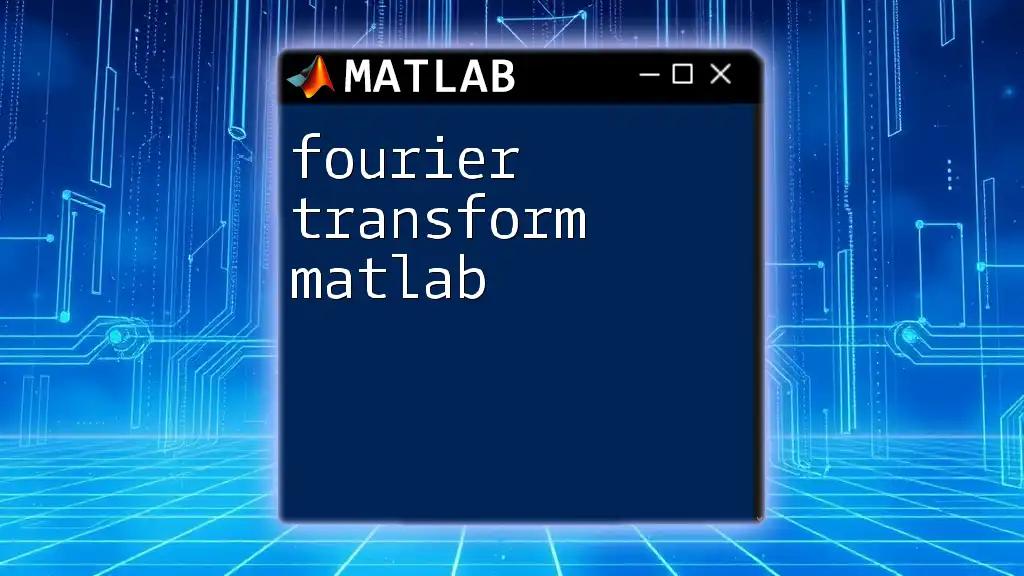
Basics of Fourier Transforms
What is a Fourier Transform?
The Fourier Transform is a mathematical operation that transforms a time-domain signal into its constituent frequencies. The significance of this transformation lies in its ability to reveal periodic patterns within a signal, thereby providing insight into its frequency content.
For continuous signals, the transformation is defined mathematically, however, when working with digital or discrete signals, the Discrete Fourier Transform is employed.
Continuous vs. Discrete Fourier Transform
The difference between Continuous and Discrete Fourier Transforms is significant, particularly in the context of digital systems. The Continuous Fourier Transform applies to continuous-time signals, while the Discrete Fourier Transform (DFT) applies to sequences of numbers, making it suitable for the processing of digital signals.
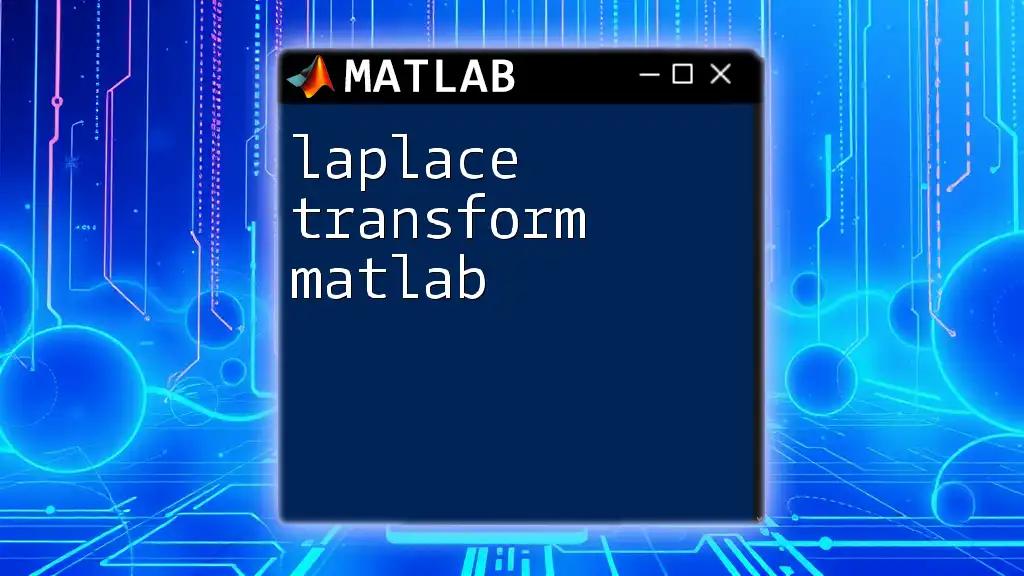
Introduction to MATLAB's FFT Function
Overview of MATLAB's FFT Implementation
MATLAB is an interactive environment designed for numerical computation, which makes it a preferred platform for implementing Fast Fourier Transforms. The FFT function simplifies the process of transforming data from the time domain to the frequency domain, providing a straightforward approach to complex computations.
Matlab Syntax for FFT
The basic syntax for the FFT function in MATLAB is:
Y = fft(X)
In this command, `X` is your input signal, and `Y` is the transformed output which contains complex numbers representing amplitude and phase information for each frequency component.
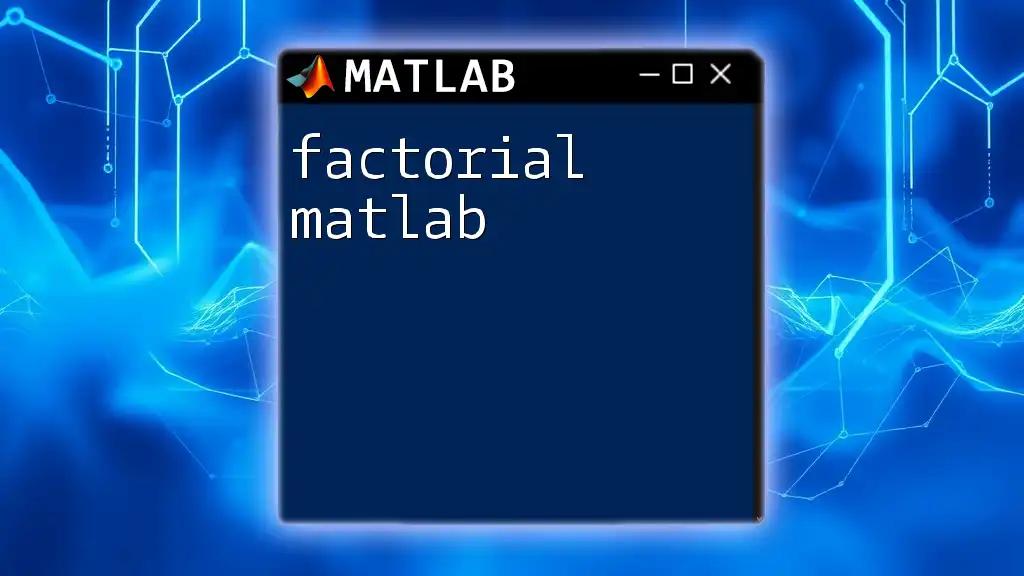
Step-by-Step Guide to Using FFT in MATLAB
Setting Up MATLAB Environment
Before executing FFT transformations, ensure that your MATLAB environment is properly configured. Typically, no special setup is required for basic functions, but ensure you have the necessary toolboxes installed for advanced features.
Preparing Your Data
Your data plays a crucial role in the effectiveness of FFT analysis. Suitable data types include time-series signals and image matrices.
For example, you can generate a sample signal comprised of multiple sine waves:
t = 0:0.001:1; % Time vector
x = sin(2*pi*50*t) + sin(2*pi*120*t); % Sample signal (sum of sinusoids)
Performing FFT on Your Data
With your signal ready, you can apply the FFT function directly.
Perform the FFT on the prepared signal `x` as follows:
Y = fft(x);
Understanding Output
The output `Y` is a complex array wherein each element corresponds to a frequency component of the input signal. To decipher this output effectively, you need to understand that:
- The magnitude of each complex number represents the strength of that frequency component.
- The phase can be derived using the `angle` function, which can provide further insights into the behavior of the signal.
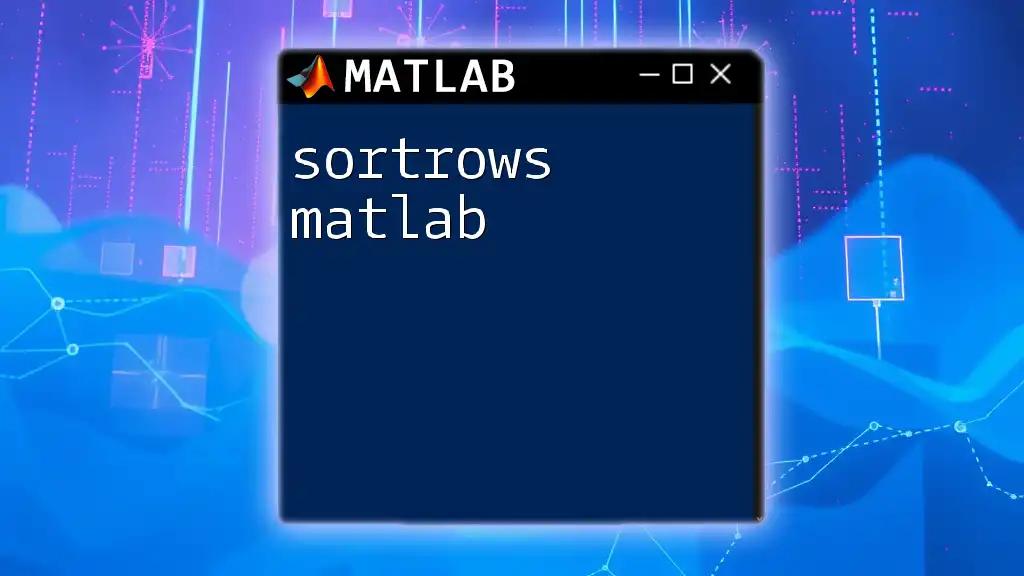
Visualizing FFT Results
Plotting the Frequency Spectrum
Visual representation of the FFT results is crucial as it allows for easy identification of the frequency components of the signal. Using the following code, you can plot the magnitude of the FFT output:
f = (0:length(Y)-1)*(1000/length(Y)); % Frequency vector
plot(f, abs(Y)); % Magnitude spectrum
title('Frequency Spectrum');
xlabel('Frequency (Hz)');
ylabel('|Y(f)|');
This plot will help you visualize which frequencies are present in your original signal and their respective amplitudes, allowing for easy identification of dominant frequencies.
Identifying Key Frequencies
To identify dominant frequencies within your FFT output, you may implement peak-finding algorithms, which can be achieved using basic logic in MATLAB to explore the maxima within the magnitude spectrum.
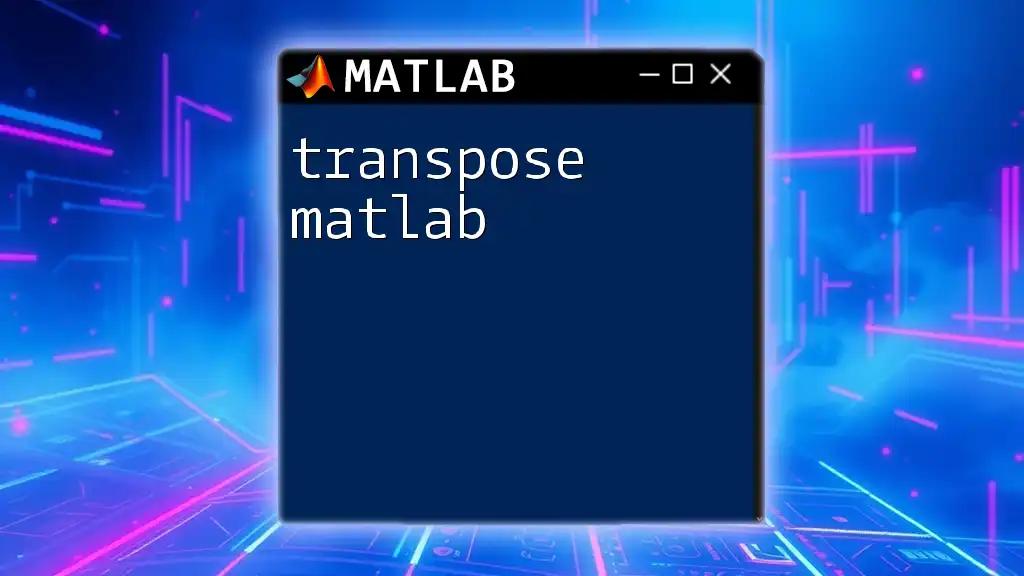
Advanced FFT Techniques in MATLAB
Zero-padding and Its Importance
Zero-padding is a technique where additional zeros are appended to the time-domain signal before computing the FFT. This process increases frequency resolution, enabling you to get a clearer spectral representation.
For example, you might choose to zero-pad your signal as follows:
X = fft(x, 2048); % Zero-padding to get more frequency resolution
Using the FFT for Signal Filtering
FFT can also be utilized to design filters, such as low-pass and high-pass filters. By transforming the signal to the frequency domain, modifying the frequency components, and then applying the Inverse FFT, you can effectively filter out unwanted frequency ranges.
Y_filtered = Y; % Create a copy of the FFT result
Y_filtered(f > 100) = 0; % Low-pass filter example (removing frequencies above 100 Hz)
x_filtered = ifft(Y_filtered); % Inverse FFT to get the time domain signal
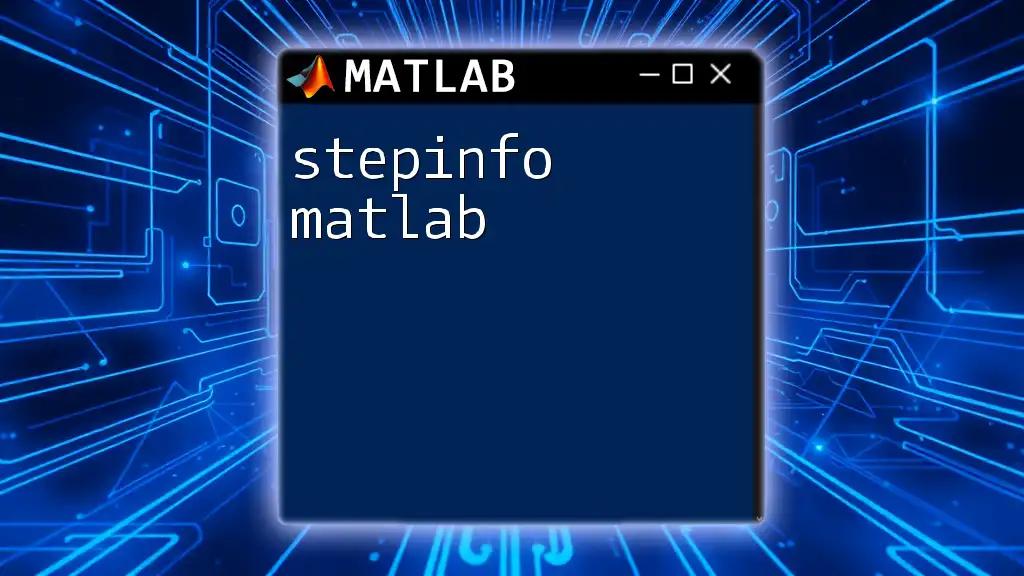
Common Pitfalls and Troubleshooting
Interpreting Non-physical Results
When employing FFT, non-physical results may sometimes occur due to issues such as aliasing, where higher frequencies distort the output. Awareness and understanding of the Nyquist theorem can help mitigate this.
Performance Issues with Large Data Sets
When dealing with large datasets, performance issues may arise due to the computational complexity of the FFT algorithm. It's essential to monitor and optimize your code by utilizing built-in functions and minimizing unnecessary computations where possible.
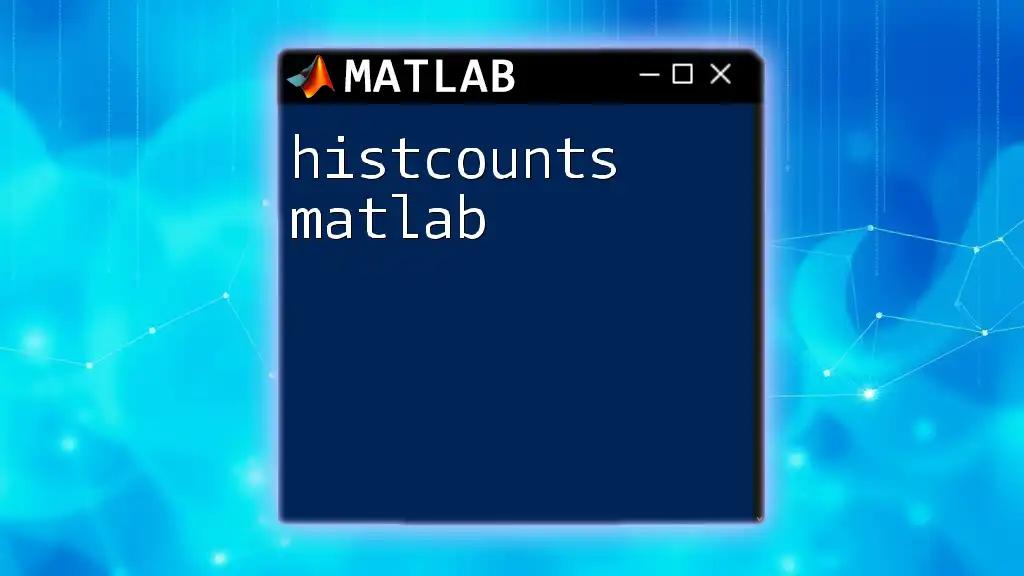
Conclusion
MATLAB's implementation of Fast Fourier Transforms provides a robust platform for analyzing signals in the frequency domain. Understanding how to effectively prepare data, execute FFT, visualize results, and troubleshoot common issues will equip you to use this powerful tool to its fullest potential. As you explore the realm of fast Fourier transforms in MATLAB, practice with various signals and datasets to enhance your skills and knowledge in this indispensable area of digital signal processing.
For further learning, consider exploring the wealth of resources available through the MATLAB documentation, online courses, and textbooks dedicated to signal processing techniques. With a solid foundation in FFT, you can tackle more complex challenges in your future projects.