The Fourier Transform in MATLAB allows you to transform a signal from the time domain to the frequency domain, enabling analysis of its frequency components.
Here’s a simple code snippet to compute the Fourier Transform of a signal:
t = 0:0.001:1; % Time vector from 0 to 1 second with a step of 0.001
signal = sin(2*pi*5*t); % Create a sine wave signal with a frequency of 5 Hz
Y = fft(signal); % Compute the Fourier Transform of the signal
f = (0:length(Y)-1)*1000/length(Y); % Frequency vector for plotting
plot(f, abs(Y)); % Plot the magnitude of the Fourier Transform
title('Fourier Transform of the Signal');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
Understanding the Concepts Behind Fourier Transform
Fourier Transform is a mathematical technique that transforms a time-domain signal into its frequency-domain representation. This is crucial within many fields, such as signal processing, image analysis, and even audio engineering, as it enables us to analyze the frequency content of signals.
Basic Principles of Fourier Transform
At its core, Fourier Transform allows us to switch between the time domain and frequency domain. Time domain refers to how a signal changes over time, while frequency domain illustrates the various frequency components present in the signal. Decomposing a signal into its frequency components allows for easier analysis and manipulation, whether for filtering, compression, or feature extraction.
Types of Fourier Transforms
Continuous Fourier Transform (CFT) is primarily used for continuous signals and is represented mathematically. It is essential for understanding signals without the limitation of discrete sampling.
Discrete Fourier Transform (DFT) applies to discrete signals and is crucial for digital signal processing. It uses sampled data points to compute the frequency spectrum, and it’s computed using the following relationship:
\[ X[k] = \sum_{n=0}^{N-1} x[n] \cdot e^{-j\frac{2\pi}{N}kn} \]
Fast Fourier Transform (FFT) is an efficient algorithm for calculating the DFT and is a cornerstone in computational mathematics. FFT significantly reduces the computational complexity, making it feasible to analyze large datasets.

Getting Started with MATLAB's Fourier Transform Functions
MATLAB Environment Overview
MATLAB provides an intuitive interface and a comprehensive set of functions for performing mathematical computations, including Fourier Transform. The Signal Processing Toolbox is particularly useful, containing tools for analyzing, visualizing, and designing signal processing algorithms.
Basic MATLAB Commands for Fourier Transform
MATLAB offers built-in functions for both fft() and ifft(), allowing easy execution of Fourier Transform procedures.
fft() function is the primary method used to compute the discrete Fourier Transform of a signal. Its syntax is straightforward, accepting a vector or matrix input. Here’s a brief example:
fs = 1000; % Sampling frequency
t = 0:1/fs:1-1/fs; % Time vector
x = sin(2*pi*50*t) + sin(2*pi*120*t); % Sample signal
% Perform FFT
Y = fft(x);
In this example, we generate a sample signal composed of two sine waves. By applying the fft() function, we compute its frequency representation.
The ifft() function reconstructs the original signal from its frequency components. Its syntax mirrors that of fft():
% Reconstruct the original signal
x_reconstructed = ifft(Y);
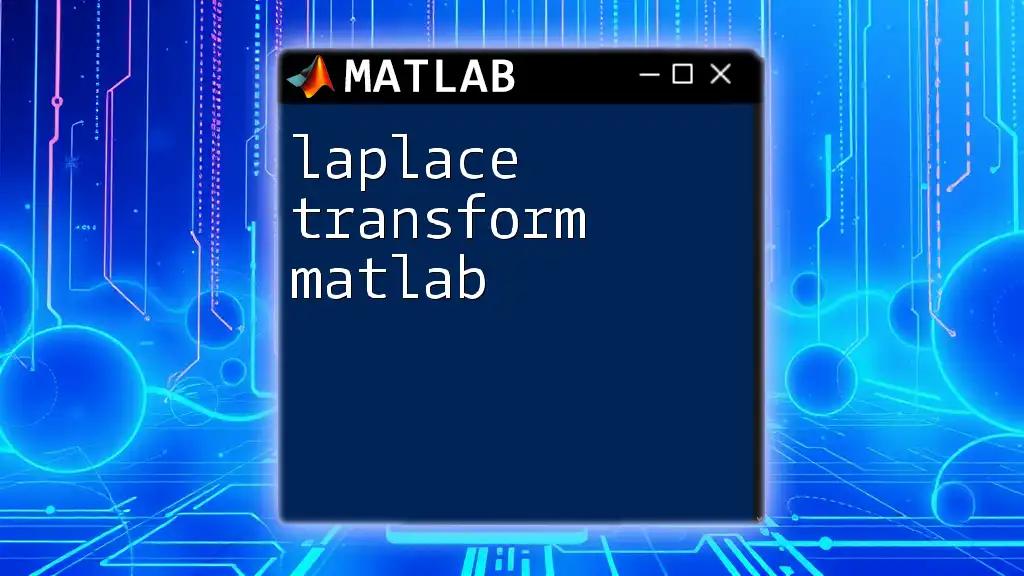
Analyzing Fourier Transform Results
Interpreting FFT Output
The output of the fft() function represents complex numbers that contain both magnitude and phase information about the signal. To extract this information, you can use the following MATLAB commands:
f = (0:length(Y)-1)*fs/length(Y); % Frequency vector
magnitude = abs(Y);
phase = angle(Y);
Magnitude represents the amplitude of each frequency component, while phase indicates the phase shift for each component. Both can be crucial for various applications such as filtering and audio processing.
Visualization of Frequency Spectrum
Visualizing the frequency spectrum is essential for understanding the data and diagnosing issues. You can use MATLAB’s plotting capabilities to illustrate the original signal and its frequency representation:
% Plot the original and reconstructed signals
subplot(2, 1, 1), plot(t, x), title('Original Signal');
subplot(2, 1, 2), plot(t, x_reconstructed), title('Reconstructed Signal');
% Plot the frequency spectrum
figure;
plot(f, magnitude);
title('Magnitude Spectrum');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
This will provide insights into how well the signal has been reconstructed after employing the Fourier Transform.
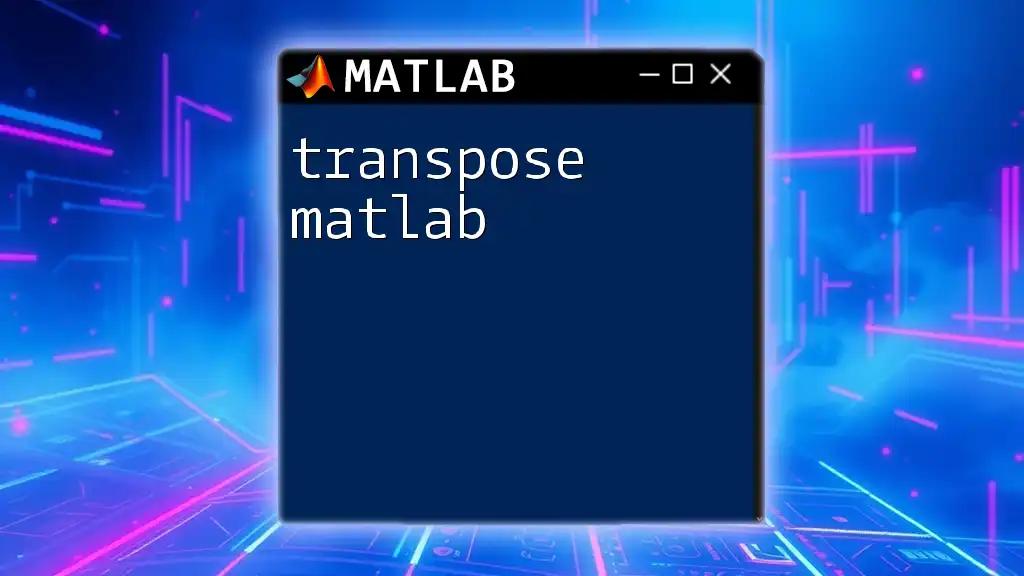
Advanced Techniques in Fourier Transform
Windowing and its Effects
Windowing functions are used to mitigate the leakage effect in Fourier Transform. A Hamming or Hanning window can be applied before performing the FFT to improve frequency resolution.
window = hamming(length(x));
x_windowed = x .* window';
Y_windowed = fft(x_windowed);
Using a window ensures that you analyze only a part of the signal over a defined period, making your frequency representation more accurate.
Spectrograms and Time-Frequency Analysis
A spectrogram is a powerful tool that shows how the frequency spectrum varies over time, providing a visual representation of the signal's frequency content. MATLAB makes it easy to generate a spectrogram:
spectrogram(x, 256, [], [], fs, 'yaxis');
title('Spectrogram');
The spectrogram is especially useful in applications like audio processing, where frequency content changes dynamically.
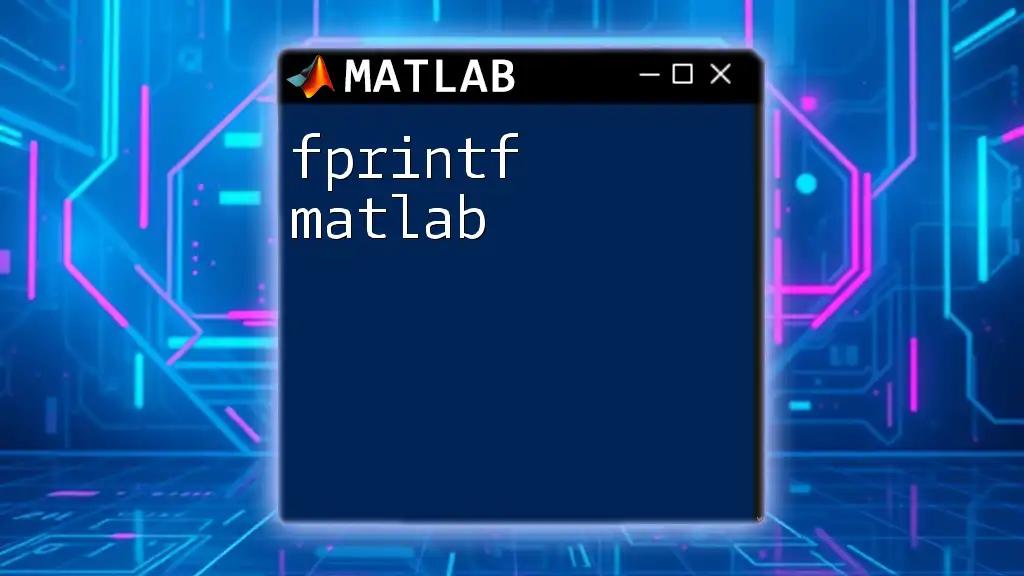
Common Applications of Fourier Transform in MATLAB
Filtering Signals
One common application of the Fourier Transform is filtering signals in the frequency domain. You can design a low-pass or high-pass filter by manipulating the magnitude spectrum. For example, zeroing out frequency components above a certain threshold can create a simple low-pass filter.
Image Processing Applications
Fourier Transform is also used extensively in image processing for filtering and enhancement. The fft2() function allows for two-dimensional Fourier Transform on images.
img = imread('example_image.png');
img_fft = fft2(img);
img_fft_filtered = img_fft; % Example: simple manipulation
img_reconstructed = ifft2(img_fft_filtered);
By manipulating the frequency components of the image, you can achieve various effects such as blurring or sharpening.
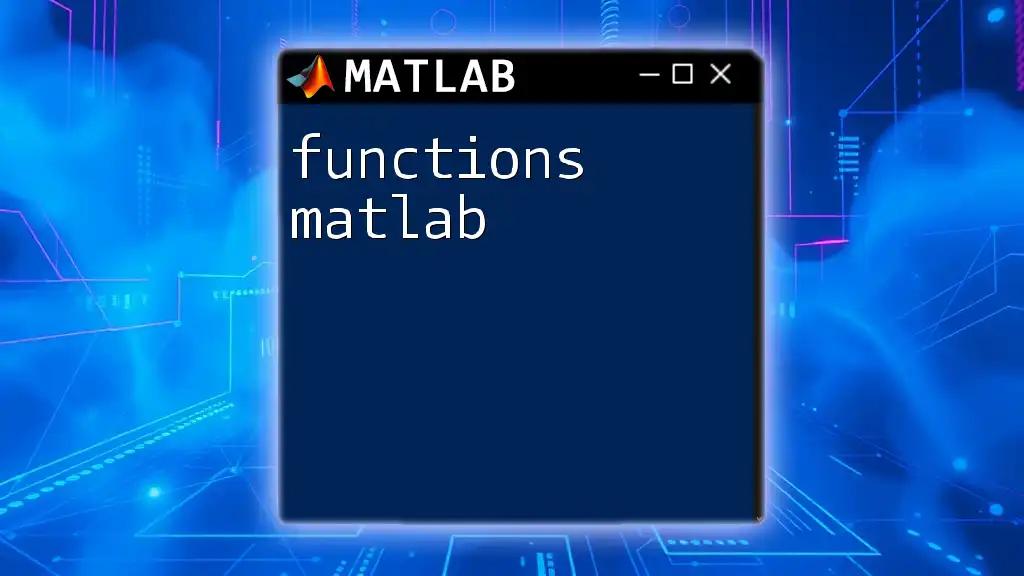
Troubleshooting Common Issues
Common Pitfalls in FFT Implementation
When performing FFT, it is essential to be mindful of the concepts like aliasing and windowing effects. Ensure your sampling frequency is at least twice the highest frequency component of your signal (Nyquist Theorem) to prevent aliasing. Windowing can reduce artifacts known as spectral leakage.
Tips for Optimizing Performance
For large datasets, consider using FFT algorithms and procedures that minimize computational overhead. Utilizing fft() on vectorized operations instead of loops can drastically improve execution time.
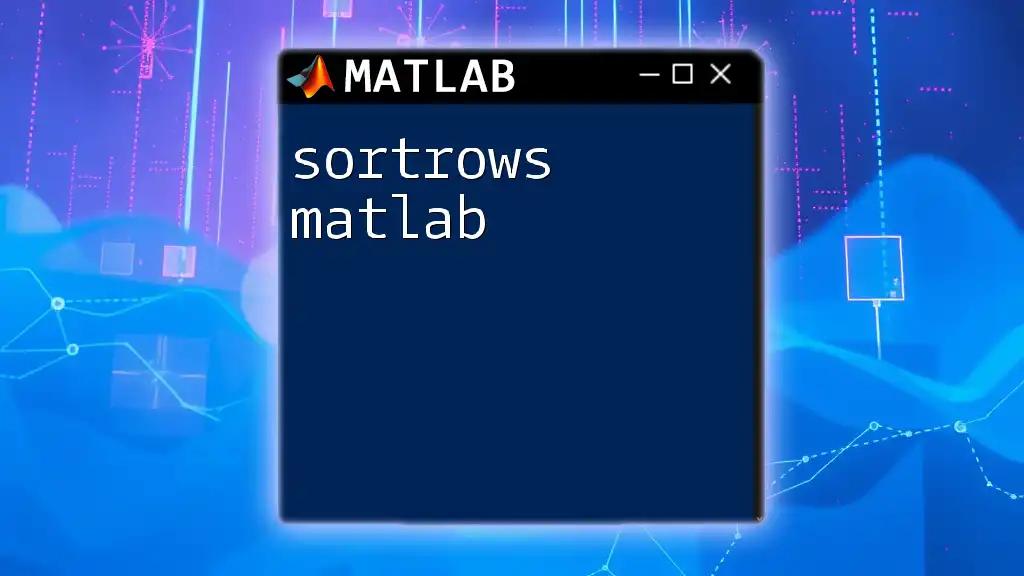
Conclusion
Fourier Transform is a transformative technique that enables a plethora of applications in signal and image processing. By leveraging MATLAB's powerful tools, you can efficiently implement Fourier analysis and visualization.
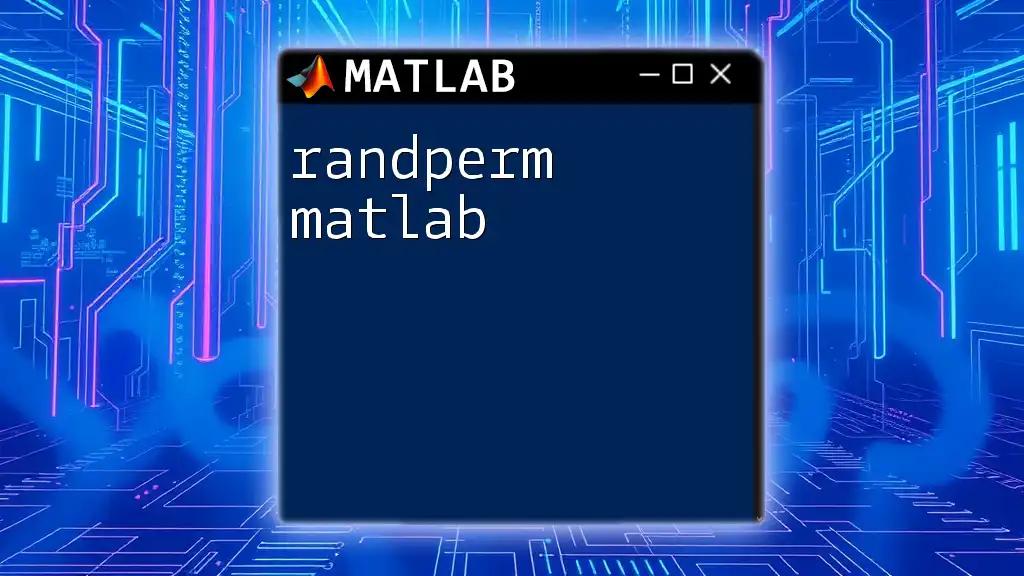
FAQs
What is the difference between FFT and DFT?
The Discrete Fourier Transform (DFT) computes the frequency spectrum of a discrete signal, while the Fast Fourier Transform (FFT) is a computationally efficient algorithm to calculate the DFT, significantly reducing the processing time needed for large datasets.
How can I visualize the phase information from FFT?
The phase information can be visualized by plotting the `angle(Y)` values against the associated frequencies, providing insights into phase shifts present in the signal.
Are there any limitations to using Fourier Transform in MATLAB?
Fourier Transform assumes that signals are periodic. Non-periodic signals can lead to inaccuracies if not windowed correctly. Additionally, FFT may not yield the most effective results for signals containing sharp transients or discontinuities.