The transpose operation in MATLAB converts rows of a matrix into columns and vice versa, often denoted by an apostrophe (`'`) after the matrix name.
Here's a code snippet demonstrating how to transpose a matrix in MATLAB:
A = [1, 2, 3; 4, 5, 6]; % Original matrix
B = A'; % Transposed matrix
Understanding the Transpose of a Matrix
Definition of Transpose
In linear algebra, the transpose of a matrix is a new matrix whose rows are the columns of the original. In simple terms, if you have a matrix \( A \), the transpose is denoted as \( A^T \) or \( A' \). The transformation effectively flips the matrix over its diagonal, which means that the element at the position \( (i, j) \) in the original matrix becomes the element at position \( (j, i) \) in the transposed matrix.
Notation
Common notations used for transposing matrices are typically \( A^T \) or \( A' \). It is crucial to maintain consistency in these notations when performing calculations—especially when working with programming languages like MATLAB, where the operator and function differ slightly but produce the same result.
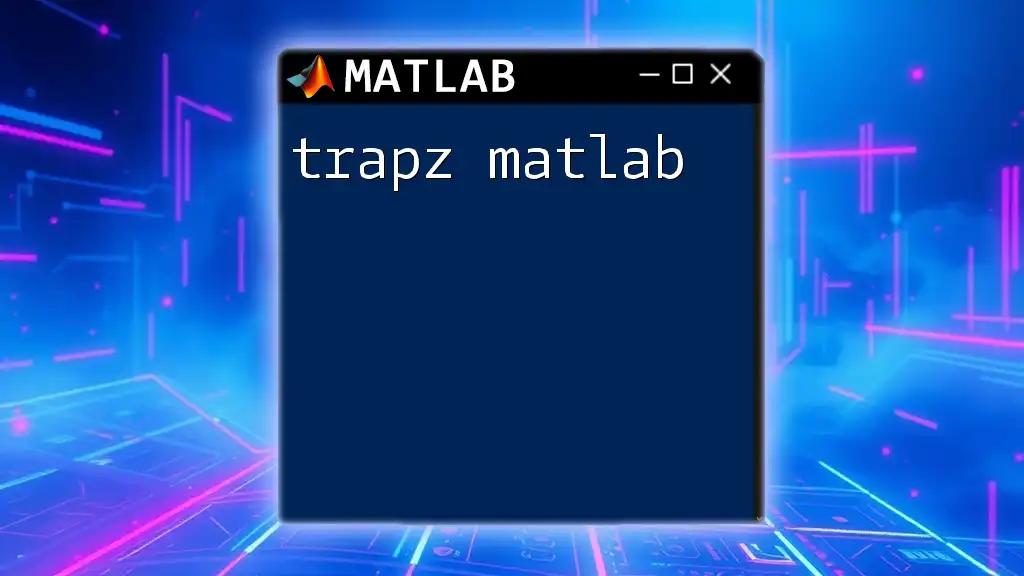
How to Transpose a Matrix in MATLAB
Using the Transpose Operator
In MATLAB, the simplest way to transpose a matrix is to use the transpose operator (' ). This operator can be applied directly to the matrix variable.
Example: Basic Transpose
A = [1, 2, 3; 4, 5, 6];
B = A'; % Transposes matrix A
In this example, the matrix \( A \) is transposed to produce matrix \( B \):
B = [1, 4;
2, 5;
3, 6];
Using the `transpose` Function
An alternative method to achieve the same result is by using the `transpose` function. This function can be particularly useful for clarity in operations involving multiple matrix manipulations or when building more complex algorithms.
Example: Using the `transpose` function
C = transpose(A); % Transposes matrix A
This will yield the same result as using the transpose operator, transforming matrix \( A \) into matrix \( C \):
C = [1, 4;
2, 5;
3, 6];
Comparison of Both Methods
Both the operator and the function accomplish the same transposition. However, while the operator is often more concise, the function may enhance readability, especially for those new to MATLAB or unfamiliar with the transpose operation. The choice between the two methods often comes down to preference and context.
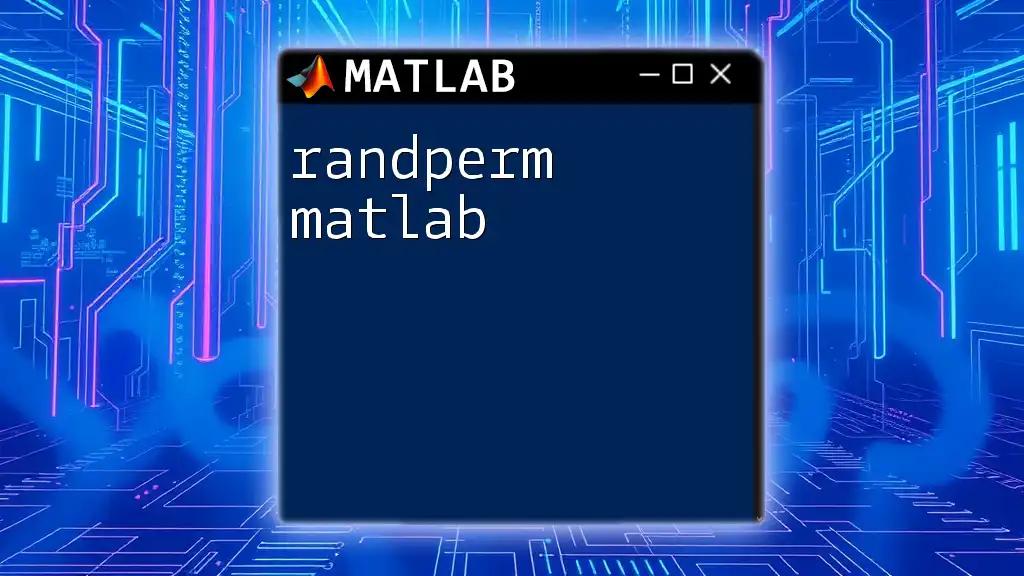
Characteristics of Transposed Matrices
Properties of Transposition
Transposing a matrix comes with several important properties:
- Symmetry: If you transpose a transposed matrix, you recover the original. This can be expressed mathematically as \( (A^T)^T = A \).
- Transpose of a Product: The transpose operator distributes over multiplication, such that \( (AB)^T = B^T A^T \). This property is vital in linear algebra and can be particularly useful for simplifying expressions involving matrix products.
Visualizing Transposition
Visual aids can significantly enhance understanding. Consider creating a graphical representation where you visualize matrix \( A \) and its transposed form \( B \). This not only provides insight into what happens during transposition but can also assist in reinforcing the underlying concepts.
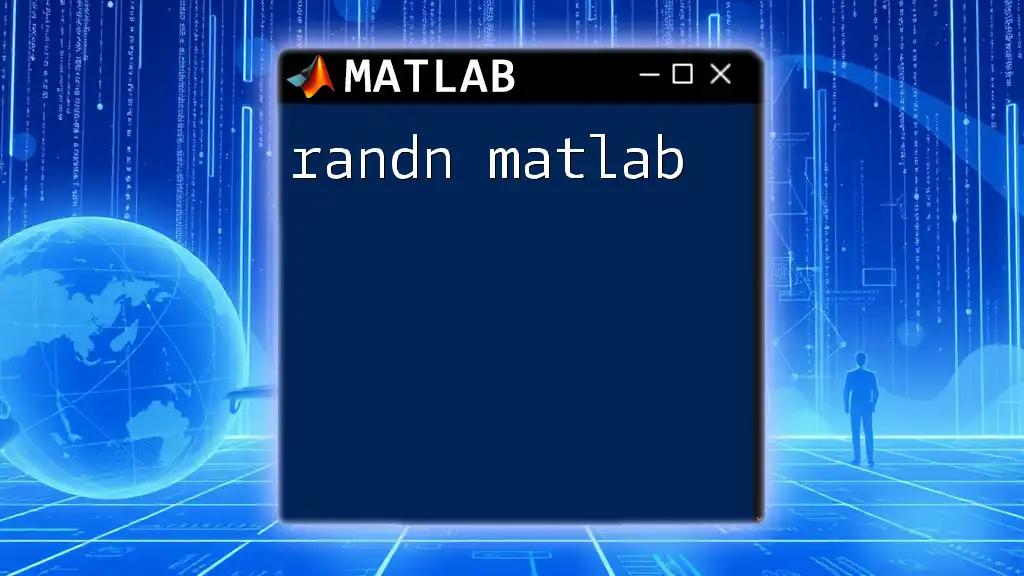
Advanced Applications of Transposition
Transpose in Linear Algebra
Transposition plays a critical role in various aspects of linear algebra, especially concerning eigenvalues and eigenvectors. When solving eigenvalue problems, transposed matrices can lead to symmetrical matrices, which simplify calculations.
For instance, the eigenvalues of matrix \( A \) and its transpose \( A^T \) will be the same. Thus, if you're computing eigenvalues in MATLAB, transposed matrices can be instrumental.
Use in Data Manipulation
In data analysis, you frequently need to manipulate datasets. The transposition of matrices is particularly handy when reshaping data. For example, if you have a matrix that represents data where rows correspond to observations and columns to variables, transposing can help you restructure this data effectively.
Example: Data reshaping using transpose
data = rand(3, 5); % Create a 3x5 matrix
reshaped_data = data'; % Reshape to 5x3 matrix
In this example, the transposed matrix can be utilized when preparing data for analysis, making it more efficient to work with in subsequent operations.
Transpose and Complex Matrices
When working with complex matrices, it is crucial to distinguish between the standard transpose and the conjugate transpose. The conjugate transpose (denoted as \( C^H \) or \( C^* \)) not only transposes the matrix but also takes the complex conjugate of each element.
Example: Transposing Complex Matrices
C = [1+2i, 3+4i; 5+6i, 7+8i];
D = C'; % Standard transpose
E = C'; % Conjugate transpose
This distinction is pivotal in fields like quantum mechanics and signal processing, where complex matrices are prevalent.
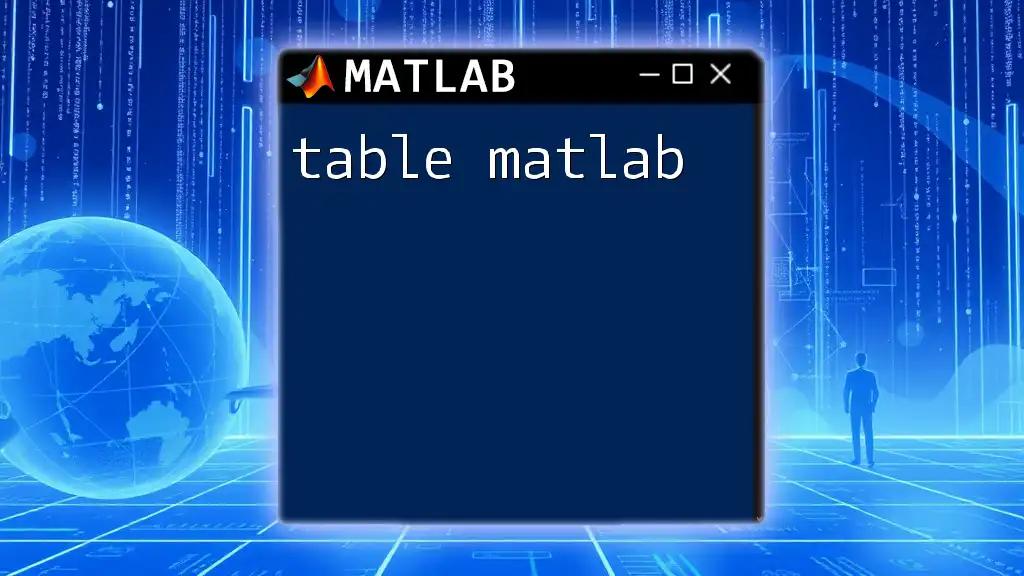
Tips and Best Practices
Common Mistakes to Avoid
A frequent source of confusion arises when the dimensions of matrices are not compatible for certain operations after transposition. Always ensure that you account for dimensions when performing calculations to avoid unexpected errors.
Performance Considerations
When dealing with large matrices, transposing can become computationally intensive. Best practices involve working on smaller subsets of data and carefully structuring your code to minimize the number of transpositions, thereby optimizing performance.
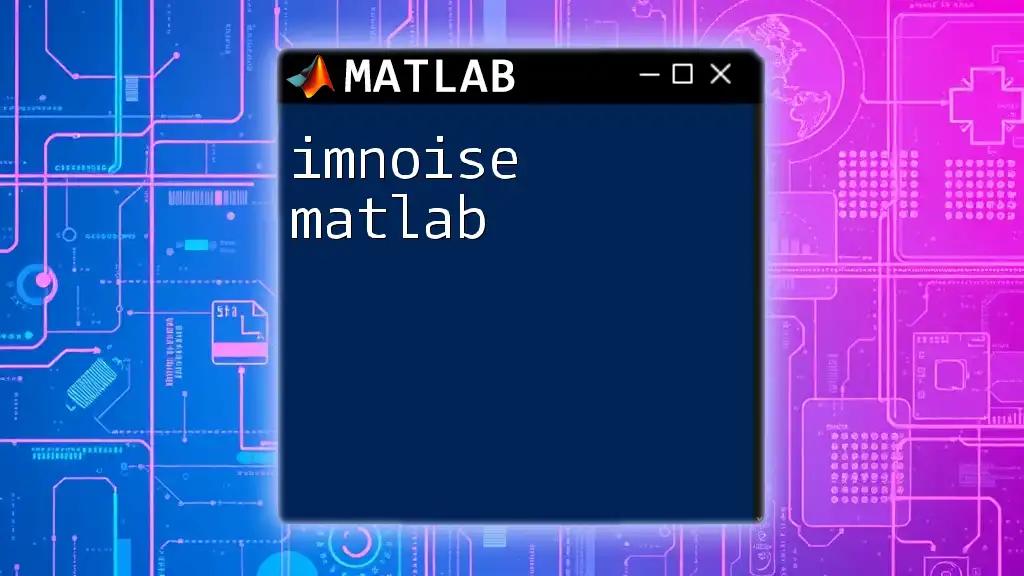
Conclusion
Mastering the concept of transpose in MATLAB is essential for both beginners and advanced users alike. With the power to reshape matrices and reveal new dimensions in data analysis, understanding how to effectively use the transpose operator and function can enhance your programming skillset.
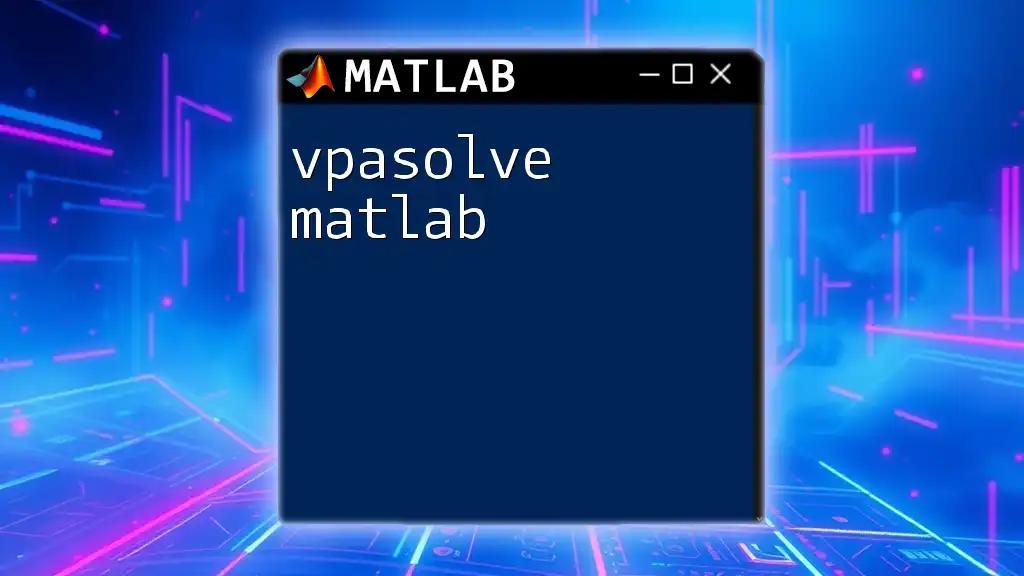
Additional Resources
Explore MATLAB's official documentation for deeper insights into matrix operations. Numerous online courses and tutorials provide further enrichment and practical exercises, enabling you to gain hands-on experience with these concepts. Practicing with real datasets will catapult your learning and application skills to the next level.