The `sortrows` function in MATLAB is used to sort the rows of a matrix or table based on one or more specified columns.
% Example of sorting a matrix by the second column
A = [3, 4; 1, 2; 5, 0];
sortedA = sortrows(A, 2);
What is `sortrows` in MATLAB?
`sortrows` is a built-in MATLAB function that allows users to sort the rows of a matrix or table based on one or more columns. This function is essential for organizing data, making analysis easier and more intuitive.
Syntax
The basic syntax of the `sortrows` function is as follows:
B = sortrows(A)
In this example:
- `A` is the input matrix or table that you want to sort.
- `B` will contain the sorted version of `A`.
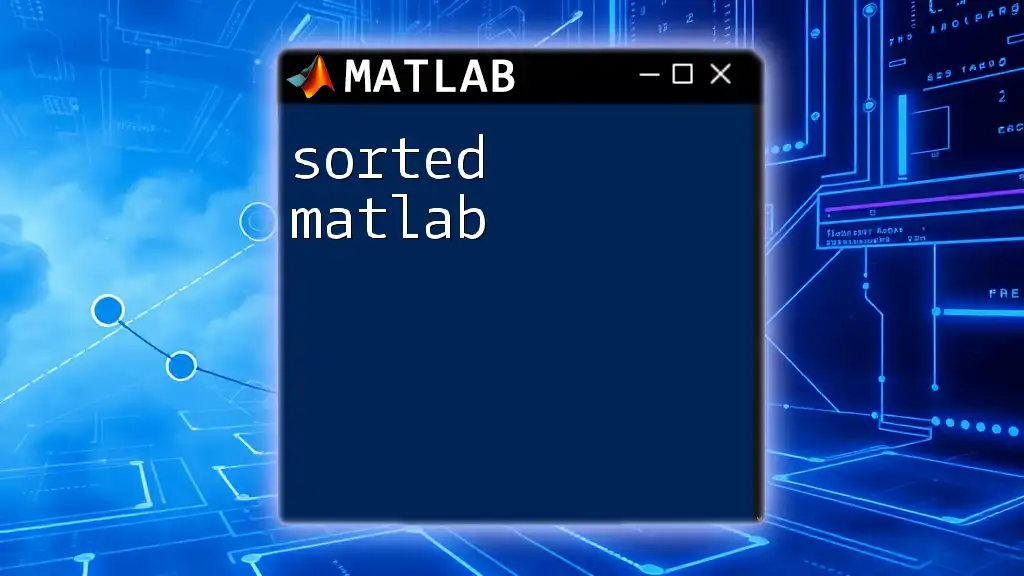
How `sortrows` Works
Sorting Rows Based on Multiple Columns
You may want to sort data based on multiple columns. The `sortrows` function can do this seamlessly.
For example, consider the following matrix:
A = [1, 2; 3, 1; 2, 3];
B = sortrows(A, [2, 1])
In this code:
- The argument `[2, 1]` indicates that the sorting will first be done by the second column and then by the first column.
- The resulting matrix `B` will be ordered according to these criteria, which allows users to prioritize one column's sorting over another.
Specifying Sort Order
In addition to sorting by multiple columns, MATLAB allows specifying the sort order for each column.
The syntax for this is:
B = sortrows(A, [1, 2], {'ascend', 'descend'})
Here:
- The first column is sorted in ascending order, while the second column is sorted in descending order. This combination offers significant flexibility and precision when working with complex datasets.
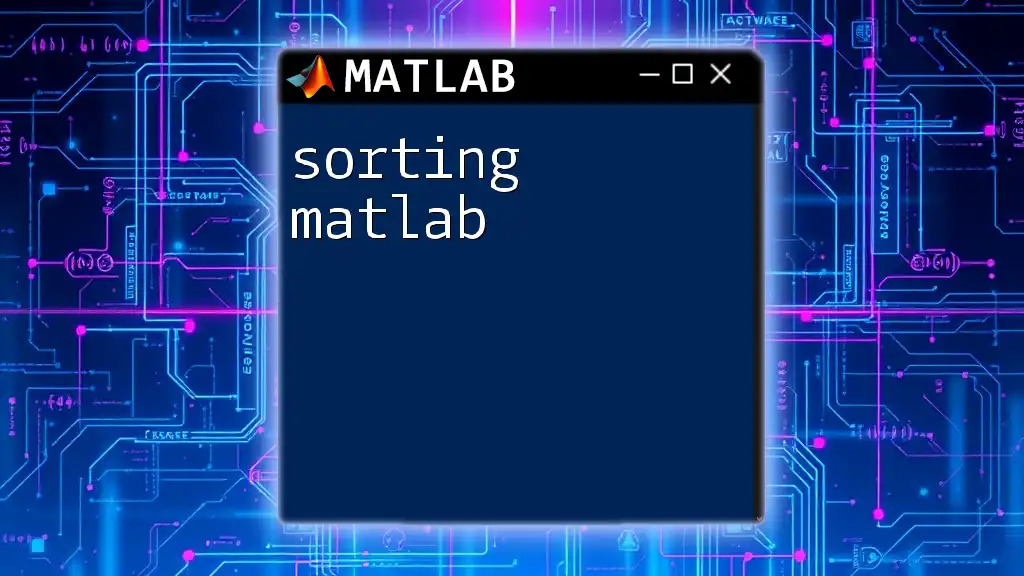
Practical Usage of `sortrows`
Sorting Tables
`sortrows` is particularly useful for sorting tables, which are more commonly used in MATLAB for handling datasets.
Consider the following table:
T = table([1; 2; 3], [2; 1; 3], 'VariableNames', {'Column1', 'Column2'});
sortedTable = sortrows(T, 'Column1')
In this example:
- The table `T` consists of two columns.
- The command sorts the table based on `Column1`. This showcases how user-friendly MATLAB's table handling can be when combined with functions like `sortrows`.
Handling NaN Values
When working with datasets, encountering NaN (Not-a-Number) values is common. The `sortrows` function has built-in functionalities to handle such cases, usually placing NaNs at the end of the sorted data by default.
For instance:
A = [1, NaN; 3, 1; 2, 3];
B = sortrows(A)
Here:
- The sorted result will automatically adjust for the NaN value, ensuring the remaining numbers are ordered correctly while pushing NaNs to the end. Understanding how to manage NaN entries significantly enhances data integrity in analysis.
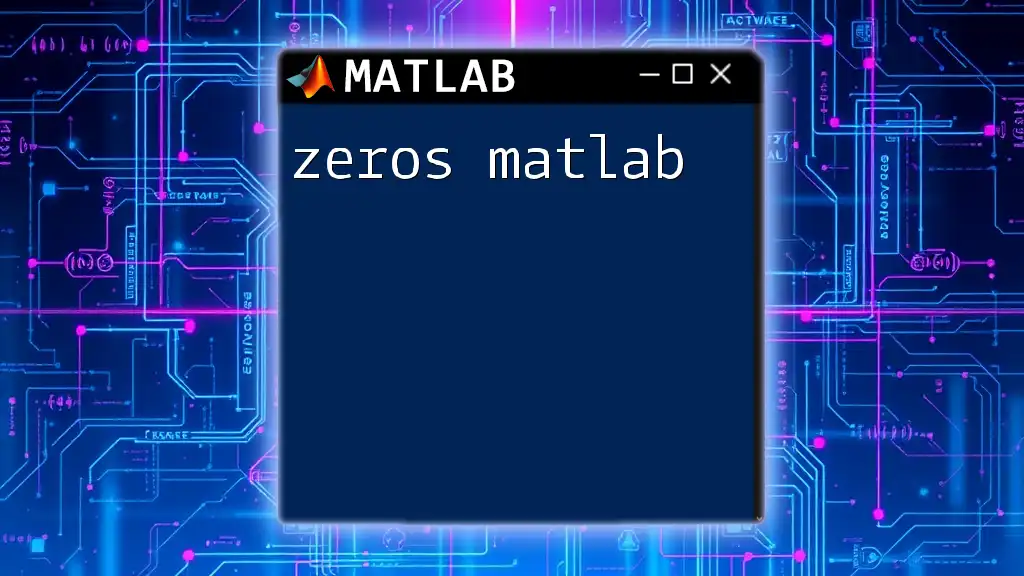
Advanced Sorting with `sortrows`
Custom Sorting Algorithms
For complex sorting challenges, you can implement custom sorting criteria using `sortrows`.
An example is as follows:
A = [1, 2; 3, 1; 2, 3];
B = sortrows(A, @(x) x(1)*2)
Here, `@(x) x(1)*2` defines a custom sorting function based on the first column multiplied by two. This level of customization allows users to tailor the sorting mechanism to their specific needs.
Using Logical Indexing for Sort
Combining `sortrows` with logical indexing offers additional filtering layers before sorting.
Consider this example:
A = [1, 2; 3, 1; 2, 3];
idx = A(:,2) > 1;
B = sortrows(A(idx, :))
In this case:
- The logical condition `A(:,2) > 1` filters the rows where the second column values are greater than 1.
- The subsequent `sortrows` on this filtered dataset ensures that only relevant data is sorted. This powerful combination can help narrow down analysis to specific groups within your dataset.
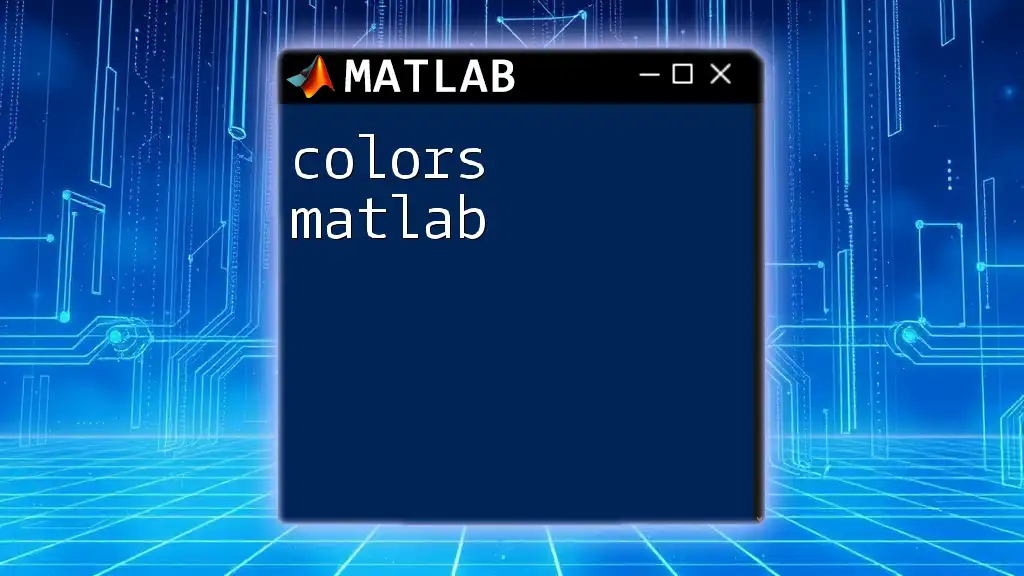
Common Mistakes and Troubleshooting
Common Pitfalls
While `sortrows` is straightforward, users often make mistakes such as:
- Forgetting to specify sort orders when dealing with multiple columns, which can lead to unexpected results.
- Ignoring NaN values, which can affect the integrity of the sorted output.
Error Messages
Be prepared to encounter certain error messages like "Index exceeds matrix dimensions". This usually arises from trying to access columns that don't exist in the dataset. Always ensure your input size matches the expected dimensions.
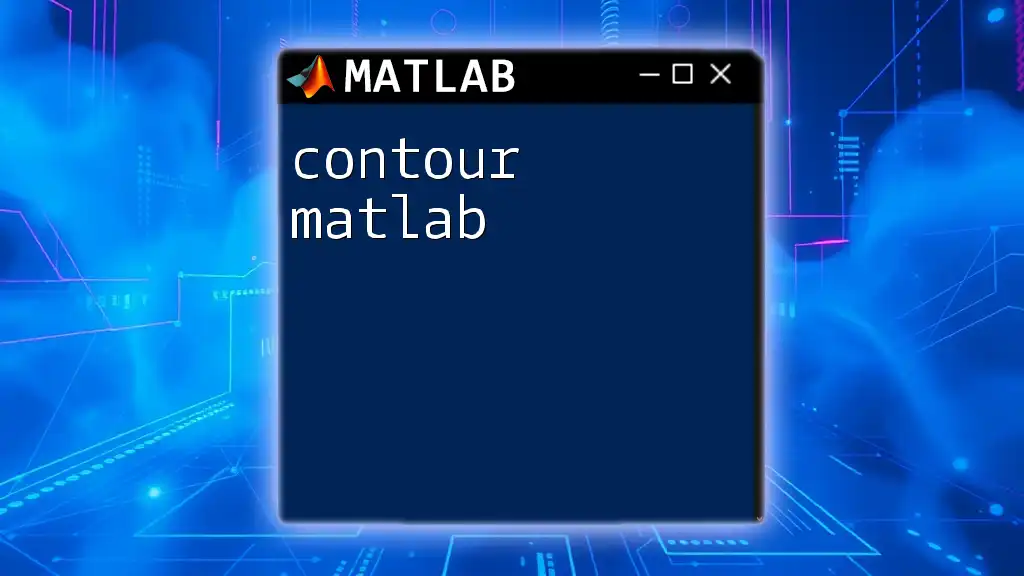
Conclusion
In summary, the `sortrows` MATLAB function is a robust tool for effectively organizing and sorting datasets. Mastering this command will undoubtedly enhance your efficiency and accuracy in data analysis.
By experimenting with the examples and code provided, you can become proficient in sorting rows to suit your analysis needs and improve your overall MATLAB skills.