The factorial function in MATLAB calculates the product of all positive integers up to a specified number, and can be easily implemented using the built-in `factorial` function.
result = factorial(5); % This will compute 5! which equals 120
Understanding the Factorial Function in MATLAB
What is a Factorial?
The factorial of a non-negative integer \( n \) is the product of all positive integers less than or equal to \( n \). Mathematically, it is represented as \( n! \). For example:
- \( 5! = 5 × 4 × 3 × 2 × 1 = 120 \)
Factorials have significant importance in various mathematical disciplines such as combinatorics, where they are pivotal in counting permutations and combinations.
The Role of MATLAB in Computing Factorials
MATLAB, short for Matrix Laboratory, is a high-level programming language primarily used for numerical and mathematical computations. It provides a robust environment for performing calculations efficiently, including the computation of factorials. The use of MATLAB for calculating factorials enhances productivity and reduces the possibility of human error.
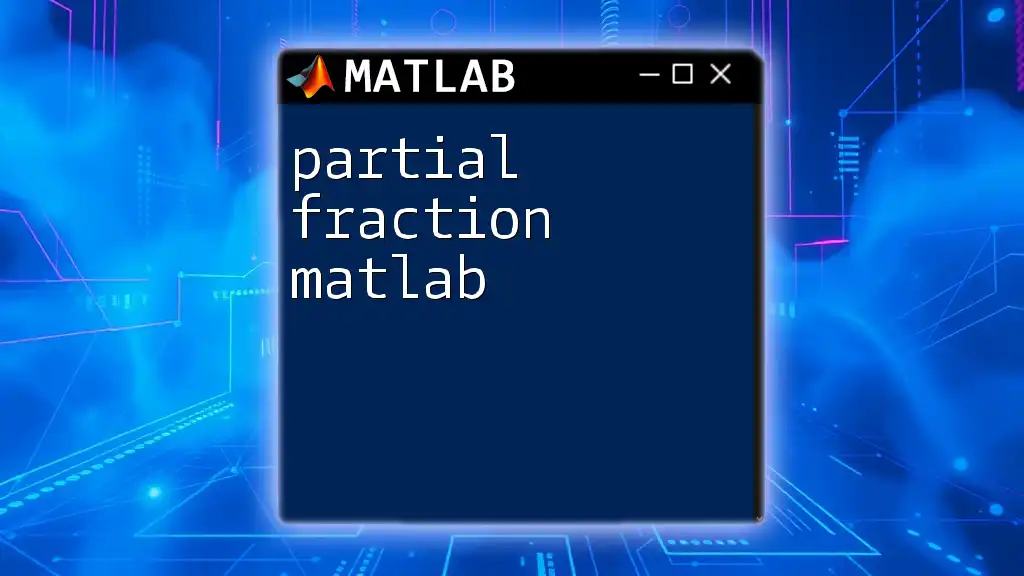
Using the Built-in Factorial Function in MATLAB
Syntax of the Factorial Function
MATLAB offers a straightforward function to compute the factorial of a number. The basic syntax is:
result = factorial(n)
In this syntax:
- Input: \( n \), a non-negative integer whose factorial you wish to calculate.
- Output: The factorial of \( n \).
For example, to calculate the factorial of 5, you would write:
result = factorial(5);
disp(result); % Output: 120
Calculating Factorials of Different Types
Single Integers
You can easily compute the factorial of a single integer using the built-in function. For instance:
result = factorial(3);
disp(result); % Output: 6
Vectors
MATLAB’s factorial function can also operate on arrays or vectors. Suppose you have a vector containing integers:
vec = [1, 2, 3];
results = factorial(vec);
disp(results); % Output: 1 2 6
Here, the factorial is calculated for each element in the vector individually.
Matrices
The factorial function can be applied element-wise to matrices, allowing you to compute factorials for each matrix entry. For example:
mat = [1, 2; 3, 4];
results = factorial(mat);
disp(results);
The output will be a matrix containing the factorials of the corresponding elements.
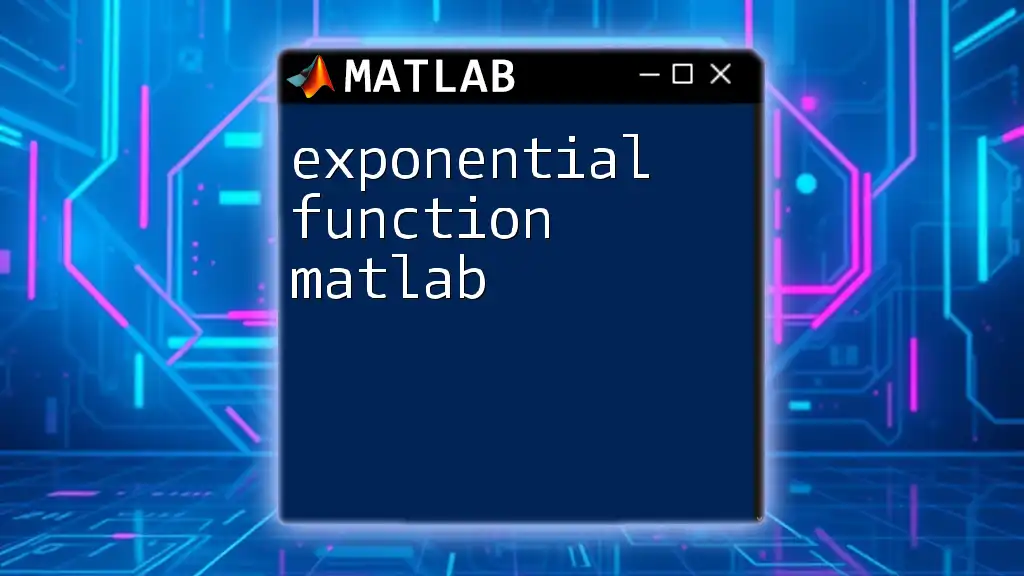
Custom Implementation of the Factorial Function
Why Create Your Own Factorial Function?
Although MATLAB provides a built-in factorial function, implementing your own can be a great exercise to deepen your understanding of programming concepts like recursion and iteration.
Writing a Factorial Function Using Recursion
Recursion is a programming technique where a function calls itself. Below is an example of a recursive function to compute the factorial of a number:
function result = factorialRecursive(n)
if n == 0
result = 1;
else
result = n * factorialRecursive(n-1);
end
end
In this implementation, the base case is \( n = 0 \), which returns 1, while for all other numbers, the function calls itself with \( n-1 \).
Writing a Factorial Function Using Iteration
An iterative approach to computing factorial avoids the overhead of recursive calls. Here’s how you can implement it:
function result = factorialIterative(n)
result = 1;
for i = 1:n
result = result * i;
end
end
In this example, the function initializes `result` to 1 and then multiplies it by each integer from 1 to \( n \).
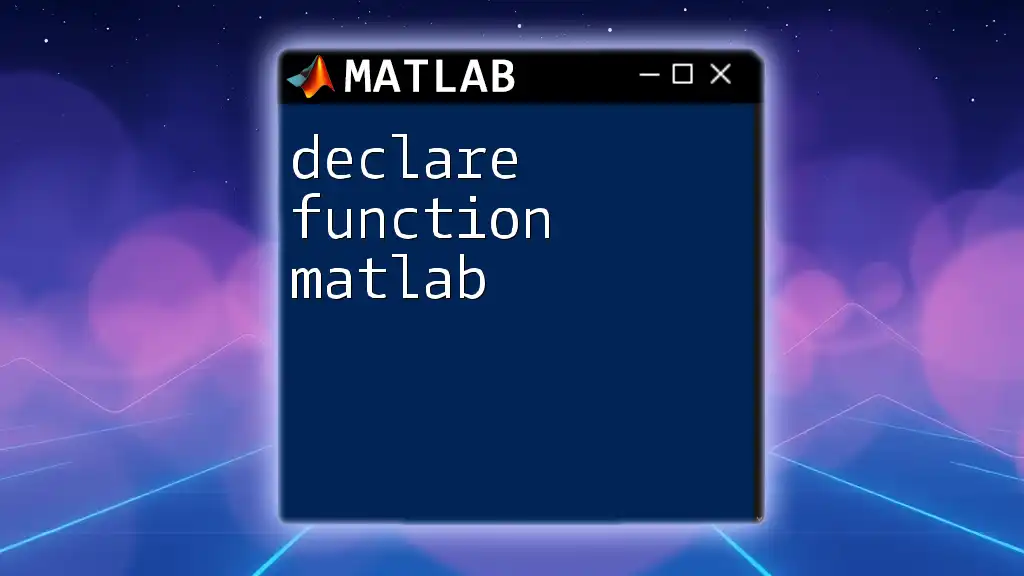
Applications of Factorial Function in MATLAB
Combinatorics and Probability
Factorials play a crucial role in combinatorial mathematics, particularly in calculating combinations and permutations. For example, to calculate the number of combinations of choosing \( r \) objects from \( n \) objects, you can use the formula:
\[ C(n, r) = \frac{n!}{r!(n-r)!} \]
Here is a MATLAB example to calculate combinations:
n = 5; % number of items
r = 3; % number of choices
combinations = factorial(n) / (factorial(r) * factorial(n-r));
disp(combinations);
Statistics and Data Analysis
In statistics, factorials are used in various functions, including the calculation of variance and standard deviation for discrete distributions, further highlighting their versatility and importance.
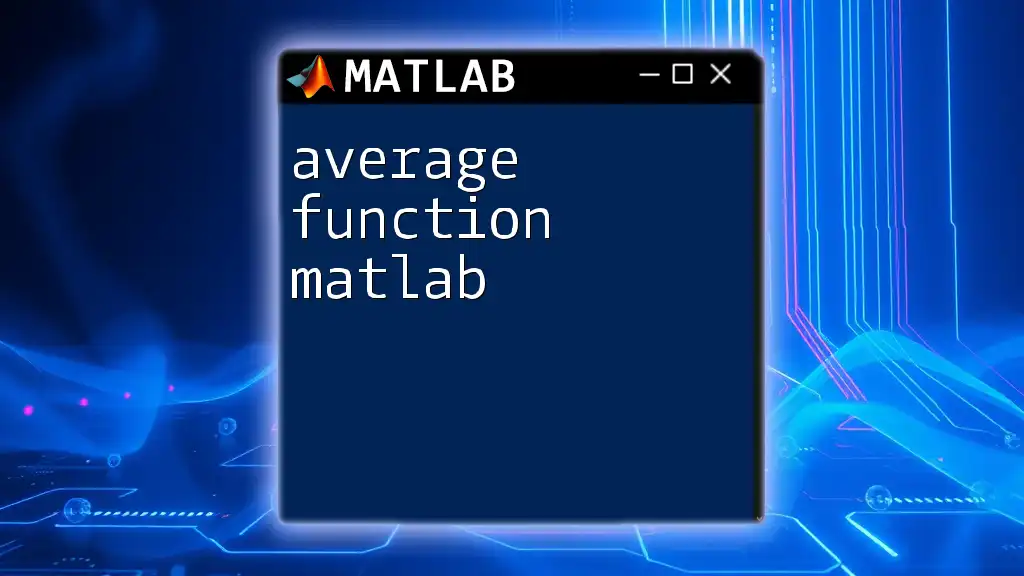
Common Errors and Troubleshooting
Handling Input Errors
When using the built-in factorial function, it’s critical to ensure that the input is a non-negative integer. Attempting to compute the factorial of a negative number or a non-integer will result in errors. Here is a simple check to prevent such errors:
if n < 0 || mod(n, 1) ~= 0
error('Input must be a non-negative integer');
end
Performance Considerations
Computing factorials of large numbers can lead to performance issues due to the rapid growth of the factorial function. For very large integers, consider using the `prod` function to compute the factorial by multiplying all integers up to \( n \):
largeFactorial = prod(1:n);
This method can provide a more efficient way to calculate larger factorials without hitting memory limits.
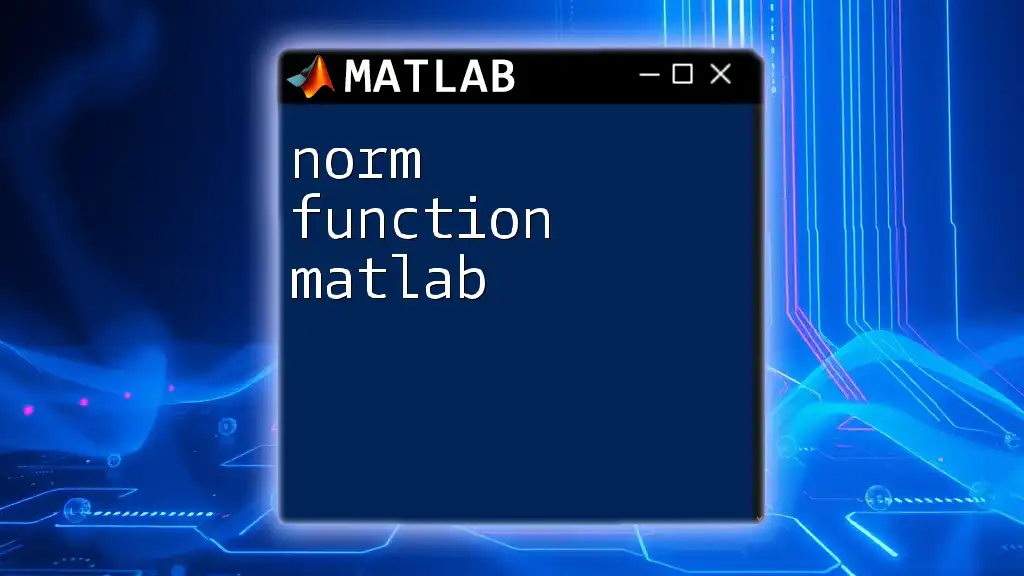
Conclusion
Recap of Key Points
In summary, the factorial function in MATLAB is a powerful tool for both beginners and advanced users, enabling efficient computation for a variety of mathematical applications. You can utilize the built-in function for simple calculations, or implement your own recursive or iterative functions for deeper learning.
Encouragement to Experiment
We encourage readers to explore different methods of computing factorials in MATLAB, whether through built-in functions or custom implementations. Experiment with vectors, matrices, and incorporate error handling as you go!
Call to Action
Stay tuned for more engaging MATLAB tutorials and insights to enhance your programming skills. Happy coding!