The MATLAB Fourier transformation allows users to convert a time-domain signal into its frequency-domain representation using the `fft` function, enabling analysis of the frequency components present in the signal.
Here's a simple code snippet demonstrating the use of the Fast Fourier Transform (FFT) in MATLAB:
% Define a sample signal
t = 0:0.001:1; % Time vector
signal = sin(2*pi*50*t) + sin(2*pi*120*t); % Sample signal with two frequencies
% Perform the Fourier Transform
Y = fft(signal);
% Compute the frequency axis
Fs = 1000; % Sampling frequency
f = (0:length(Y)-1)*Fs/length(Y); % Frequency vector
% Plot the results
plot(f, abs(Y));
title('Fourier Transform of the Signal');
xlabel('Frequency (Hz)');
ylabel('Magnitude');
xlim([0 200]); % Limit frequency range for clarity
Understanding the Mathematical Foundations
What is Fourier Transformation?
At its core, Fourier Transformation is a mathematical technique used to convert signals from the time domain to the frequency domain. This transformation reveals the hidden frequency components of a signal, allowing for analysis and manipulation in the frequency domain. Applications of Fourier Transformation span various fields, such as engineering, image processing, telecommunications, and audio analysis.
The Concept of Frequency
Frequency refers to the number of occurrences of a repeating event per unit time. In signal processing, understanding the frequency of a signal helps us analyze its behavior and characteristics. For instance, different musical notes correspond to different frequencies, making frequency analysis crucial in audio processing.
Mathematical Representation of Fourier Transform
The Fourier Transform can be expressed mathematically, allowing us to understand how signals can be decomposed into their constituent frequencies. The general equation for the Continuous Fourier Transform is:
$$ F(\omega) = \int_{-\infty}^{\infty} f(t) e^{-j\omega t} dt $$
Where:
- \( F(\omega) \) is the Fourier Transform of the function \( f(t) \).
- \( \omega \) represents the angular frequency.
- \( j \) is the imaginary unit.
In contrast, the Discrete Fourier Transform (DFT) applies to sequences of sampled data, and MATLAB provides the `fft` function to efficiently compute it.
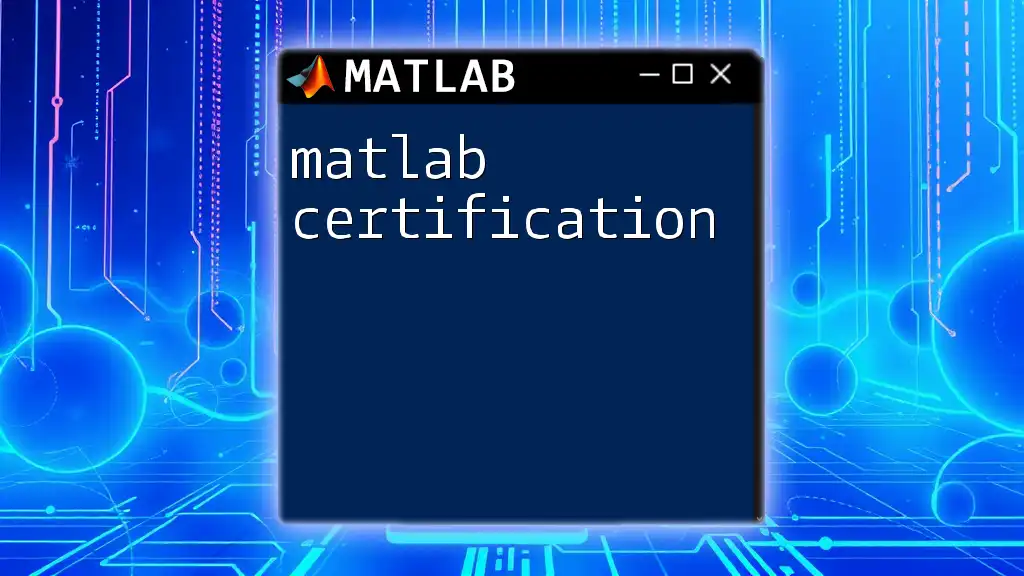
MATLAB Basics for Fourier Transform
Setting Up the Environment
Before diving into Fourier Transformation, ensure you have MATLAB installed on your system. Start MATLAB and familiarize yourself with basic commands such as:
clc; % Clear command window
clear; % Clear workspace variables
close all; % Close all figures
Key MATLAB Functions for Fourier Transformation
Several essential functions facilitate Fourier Transformation in MATLAB. Below are the key functions and their purposes:
- `fft`: Computes the Discrete Fourier Transform.
- `ifft`: Computes the Inverse Discrete Fourier Transform.
- `fftshift`: Shifts the zero-frequency component to the center of the spectrum.
- `fftfreq`: Generates frequency bins for plotting frequency spectrum.
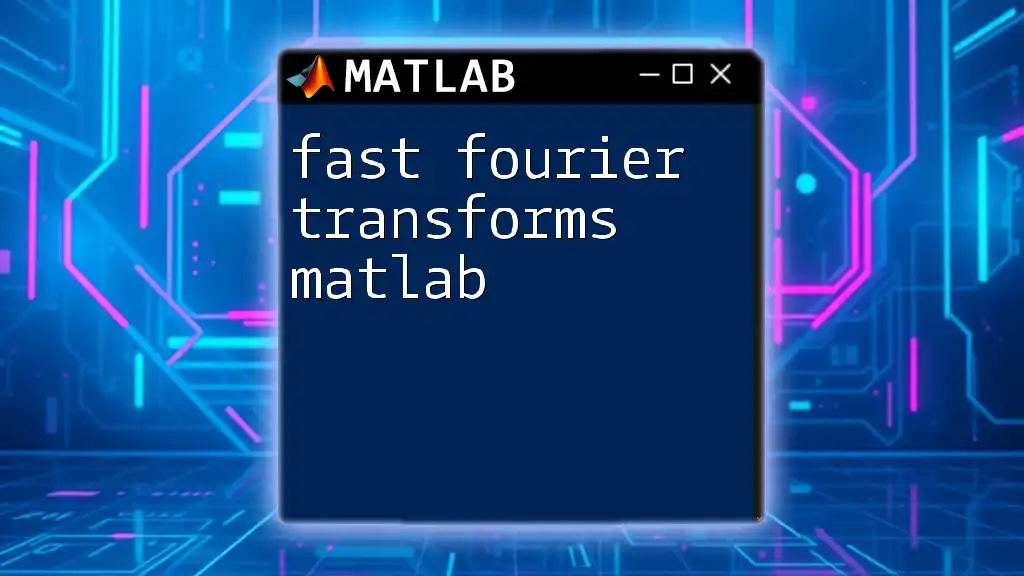
Performing Fourier Transformation in MATLAB
Step-by-Step Guide to DFT
Creating a Signal:
To begin, you can create a simple sine wave, which serves as a perfect example for learning Fourier Transformation. Here’s a basic MATLAB code snippet for generating a sine wave:
Fs = 1000; % Sampling frequency
t = 0:1/Fs:1-1/Fs; % Time vector
f = 5; % Frequency of the sine wave
signal = sin(2*pi*f*t); % Sine wave signal
plot(t, signal); % Plot the signal
title('Sine Wave Signal');
xlabel('Time (s)');
ylabel('Amplitude');
This code creates a sine wave of 5 Hz and plots it against time.
Applying the Fourier Transform:
To transform this time-domain signal into the frequency domain, use the `fft` function:
Y = fft(signal);
This operation converts your time-domain signal into its frequency components, allowing for further analysis.
Visualizing the Results:
To visualize the frequency spectrum, a plot of the magnitude spectrum is helpful. The following code calculates and displays the magnitude of the Fourier Transform:
f = Fs*(0:(length(Y)/2))/length(Y); % Frequency vector
P = abs(Y/length(signal)); % Normalize
plot(f,P(1:length(f))); % Magnitude Spectrum
title('Magnitude Spectrum');
xlabel('Frequency (f)');
ylabel('|P(f)|');
This code snippet generates a plot showing how the frequency components of the original signal are distributed.
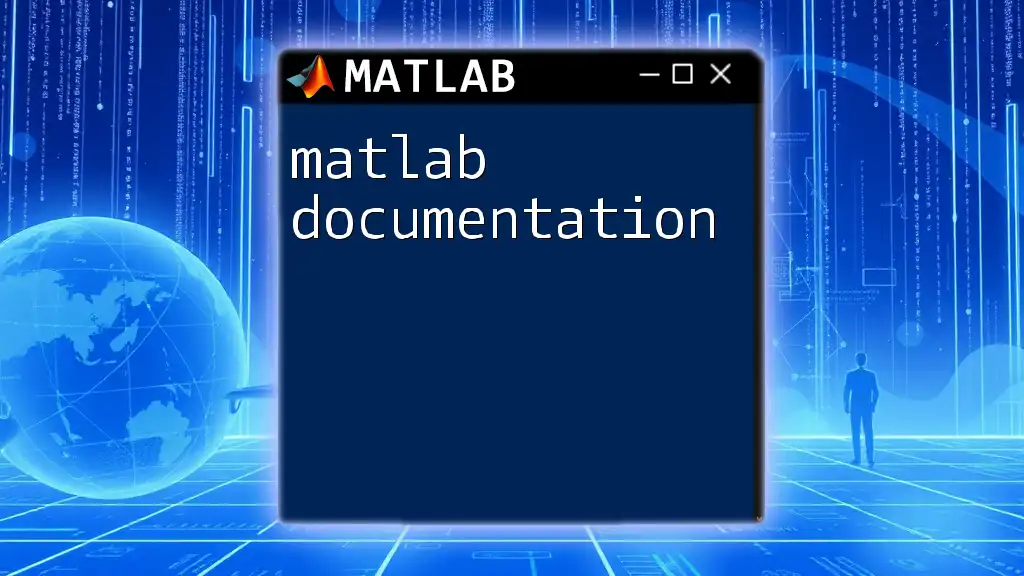
Analyzing Fourier Transform Results
Interpreting the Frequency Spectrum
Once you have the frequency spectrum, it’s essential to understand what the peaks represent. Peaks in the plotted amplitude spectrum correspond to frequencies that are prominent in the original signal. For instance, if your original signal was a 5 Hz sine wave, you should observe a distinct peak in the frequency spectrum at 5 Hz.
Common Issues and Troubleshooting
When using Fourier Transform, you might encounter several common issues, such as aliasing. Aliasing occurs when a signal is sampled below its Nyquist rate, leading to incorrect representations in the frequency domain. To avoid this, ensure that your sampling frequency is at least twice the highest frequency present in the signal.
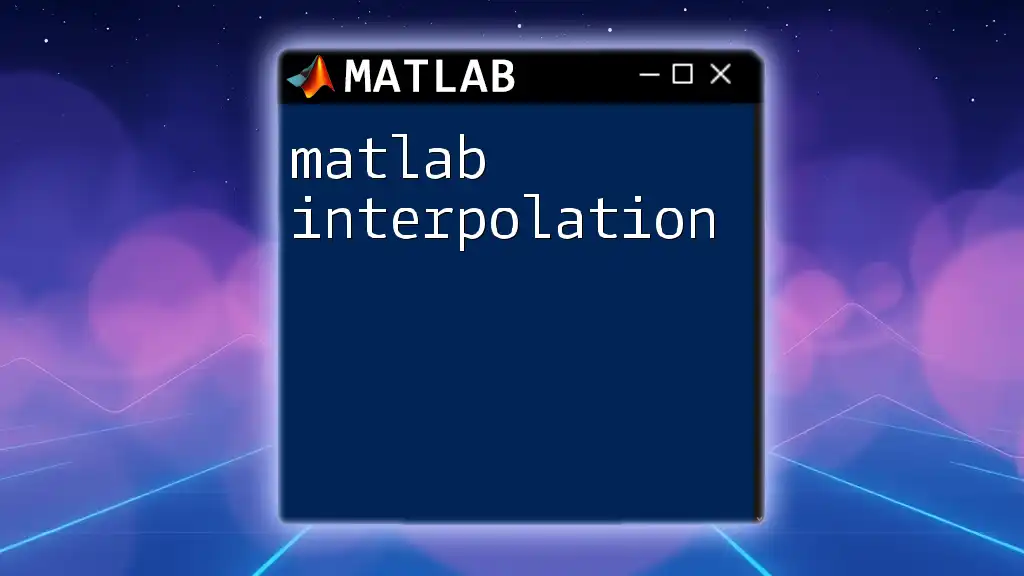
Advanced Fourier Transform Techniques
Fast Fourier Transform (FFT)
The Fast Fourier Transform is an algorithm to compute the Discrete Fourier Transform efficiently. Its primary advantage is speed, making it practical for large datasets. In MATLAB, using `fft` is essentially performing FFT, resulting in what was once a computationally intensive operation being executed in a fraction of the time.
Applications of FFT in Signal Processing
FFT is widely used in various signal processing applications, such as filtering, spectral analysis, and audio processing. Here’s a simple example illustrating how easily FFT can be applied to real-world audio signals.
Inverse Fourier Transform
To convert back to the time domain from the frequency domain, you would use the Inverse Fourier Transform, achievable through the `ifft` function. The following example demonstrates how to reconstruct a time-domain signal:
reconstructed_signal = ifft(Y);
plot(t, reconstructed_signal);
title('Reconstructed Signal');
xlabel('Time (s)');
ylabel('Amplitude');
This step is vital when modifications are made in the frequency domain.
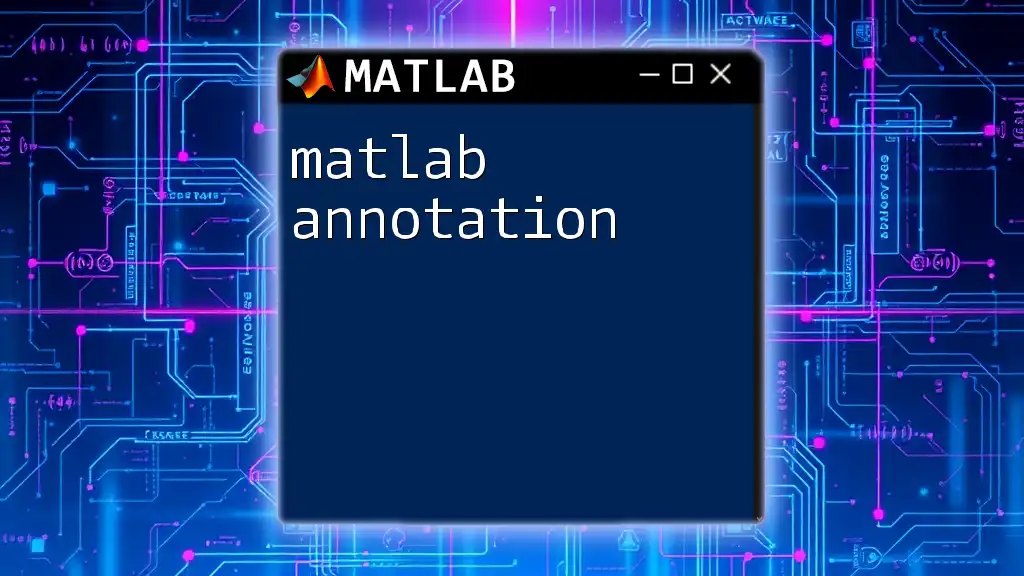
Practical Applications of Fourier Transformation
Signal Analysis
The realm of signal analysis heavily relies on Fourier Transformation. In audio processing, for instance, Fourier Transform is essential for analyzing sound waves, which can be further manipulated for noise reduction or tone adjustment.
Case Studies
Consider a case study where researchers analyzed seismic data using Fourier Transform to detect anomalies in underground vibrations. By transforming the time-series seismic data into frequency components, they identified unusual patterns indicative of geological activity. Such methods underscore the real-world utility of MATLAB Fourier Transformation.
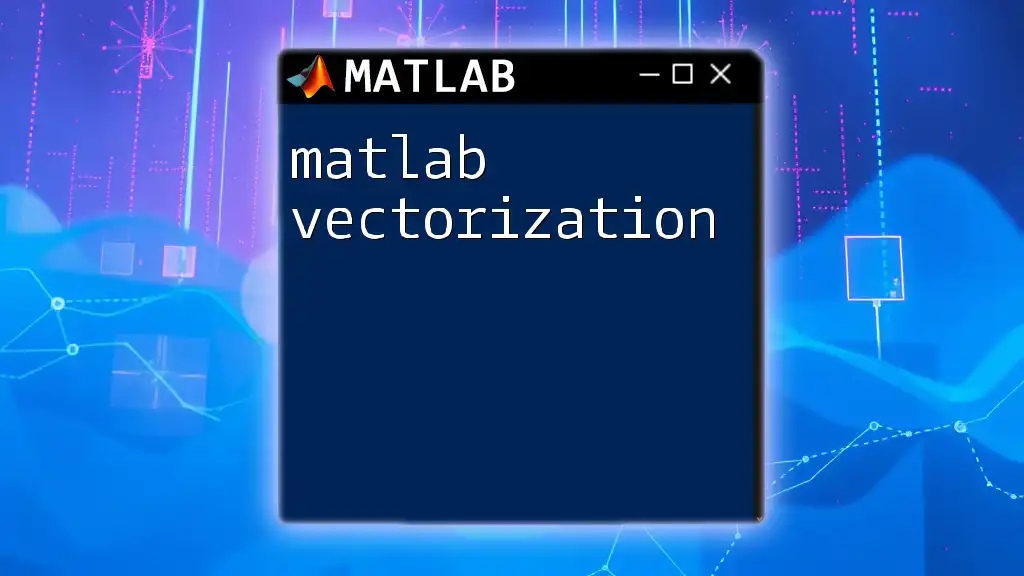
Conclusion
In summary, the MATLAB Fourier Transformation is an indispensable tool in both academic and applied science settings. From understanding the fundamentals behind frequency and mathematical representation to applying FFT and reconstructing signals, mastering this technique opens up a plethora of analytical possibilities. With practice and exploration, you can harness the power of Fourier Transform to analyze and manipulate data in ways that were previously unimaginable.
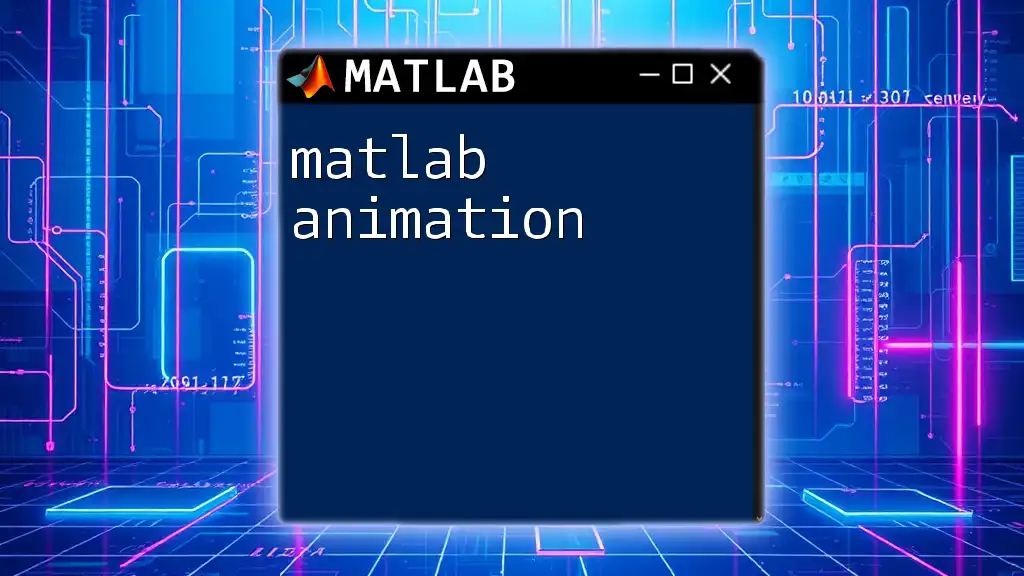
FAQs
Common Questions about MATLAB Fourier Transformation
For the novice and advanced users alike, understanding common queries regarding MATLAB Fourier Transformation can deepen your practical knowledge, enabling you to avoid pitfalls and enhance your proficiency in MATLAB signal processing.
By exploring the rich potential of Fourier Transformation, you can elevate your analytical skills and apply them across various domains with confidence and expertise.