In MATLAB, you can compute logarithms using the `log`, `log10`, and `log2` functions, where `log` returns the natural logarithm, `log10` returns the logarithm base 10, and `log2` returns the logarithm base 2.
% Example of computing logarithms in MATLAB
x = 10;
natural_log = log(x); % Natural logarithm of 10
log_base10 = log10(x); % Logarithm base 10 of 10
log_base2 = log2(x); % Logarithm base 2 of 10
Understanding Logarithms
What are Logarithms?
Logarithms are the mathematical inverses of exponentiation. They provide a way to express large numbers in a more manageable form and are critical in various scientific and engineering fields. Simply put, the logarithm log_b(a) answers the question: "To what exponent must we raise base b to obtain a?" For instance, if we have b = 10 and a = 100, then log_10(100) = 2 since 10^2 = 100.
Logarithms are particularly significant in analyses involving exponential growth or decay, making them invaluable in computations performed in MATLAB.
The Mathematical Backbone
Understanding the properties of logarithms can help you wield them effectively in MATLAB. Here are some key identities:
- Product Rule: \( \log_b(m \cdot n) = \log_b(m) + \log_b(n) \)
- Quotient Rule: \( \log_b\left(\frac{m}{n}\right) = \log_b(m) - \log_b(n) \)
- Power Rule: \( \log_b(m^n) = n \cdot \log_b(m) \)
These properties enable you to manipulate and simplify logarithmic expressions efficiently.
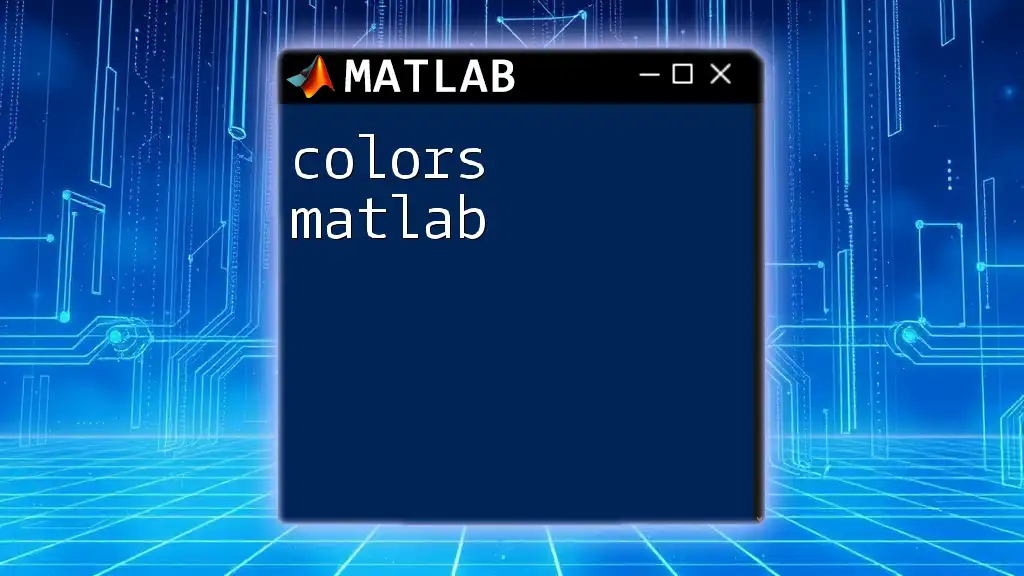
Logarithmic Functions in MATLAB
Introduction to MATLAB's Logarithmic Functions
MATLAB offers a suite of built-in functions to perform logarithmic calculations, making it easy for users to manipulate logarithmic values in various applications. These functions include log, log10, and log2, each catering to a specific base.
Common Logarithm Functions
Natural Logarithm: `log()`
The `log()` function computes the natural logarithm (base e) for an array of inputs. This function is often used due to the significance of e in mathematical modeling.
Syntax:
log(X)
Example:
x = [1, 2.718, 7.389];
natural_logs = log(x);
disp(natural_logs);
This snippet computes the natural logarithm of the values in x. The output would show the logarithmic values, emphasizing 1 has a logarithm of 0, while e (approximately 2.718) has a logarithm of 1, and e^2 (approximately 7.389) has a logarithm of 2.
Base-10 Logarithm: `log10()`
The `log10()` function is designed for computing logarithms to the base 10.
Syntax:
log10(X)
Example:
x = [1, 10, 100];
base10_logs = log10(x);
disp(base10_logs);
This example would yield logarithmic values of 1, 10, and 100, returning 0, 1, and 2, respectively, demonstrating the relationship of these numbers with base 10.
Base-2 Logarithm: `log2()`
For computations involving binary systems, MATLAB provides the `log2()` function.
Syntax:
log2(X)
Example:
x = [1, 2, 4, 8];
base2_logs = log2(x);
disp(base2_logs);
The output would be 0, 1, 2, and 3, confirming that log_2(1) = 0, log_2(2) = 1, and so forth.
Using Custom Bases for Logarithms
Calculating logarithms with bases other than the standard types can be easily achieved through the change of base formula.
Formula: \[ \log_a(b) = \frac{\log(b)}{\log(a)} \]
Example:
b = 100;
a = 10;
custom_log = log(b) / log(a);
disp(custom_log);
In this case, the code calculates \( \log_{10}(100) \), yielding 2, as expected.
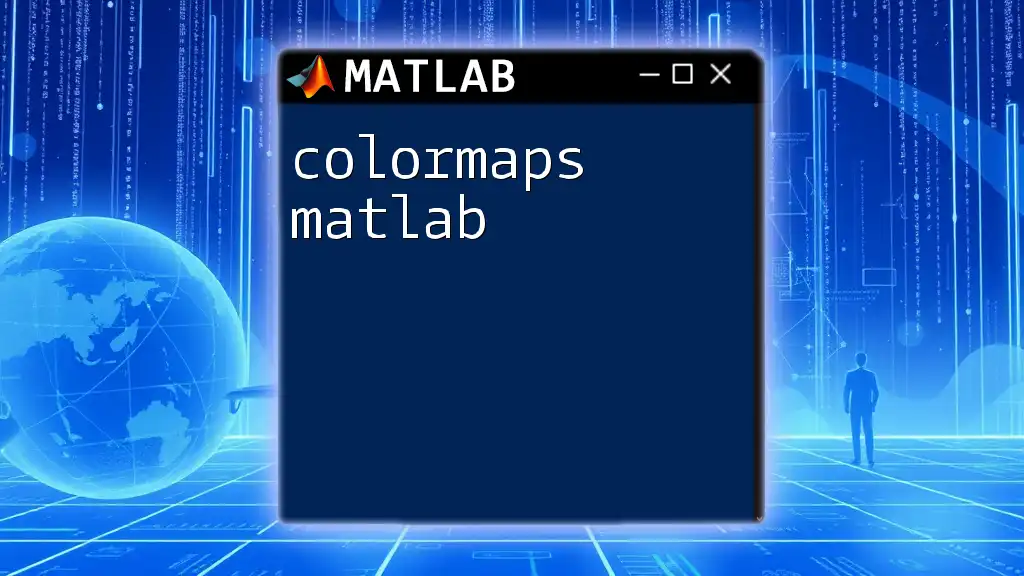
Practical Applications of Logarithms in MATLAB
Data Analysis
Logarithmic transformations are invaluable in data analysis, particularly for normalizing skewed datasets. Using logarithms can stabilize variance and make data conform more closely to a normal distribution, thus facilitating better statistical analysis.
Signal Processing
In audio signal processing, logarithmic scales allow for a more intuitive measurement of sound intensity. Normally, we express sound levels in decibels (dB), which use a logarithmic scale to relate sound intensity to a reference level.
Example: To calculate the decibel value, you can use the formula:
decibels = 10 * log10(intensity / reference_intensity);
This allows engineers to express dynamic audio levels compactly.
Solutions of Equations
Logarithmic functions are also crucial when solving equations that involve exponential terms. For instance, if you're trying to solve an equation like \( 2^x = 16 \), applying logarithms can simplify the process: \[ x = \log_2(16) \]
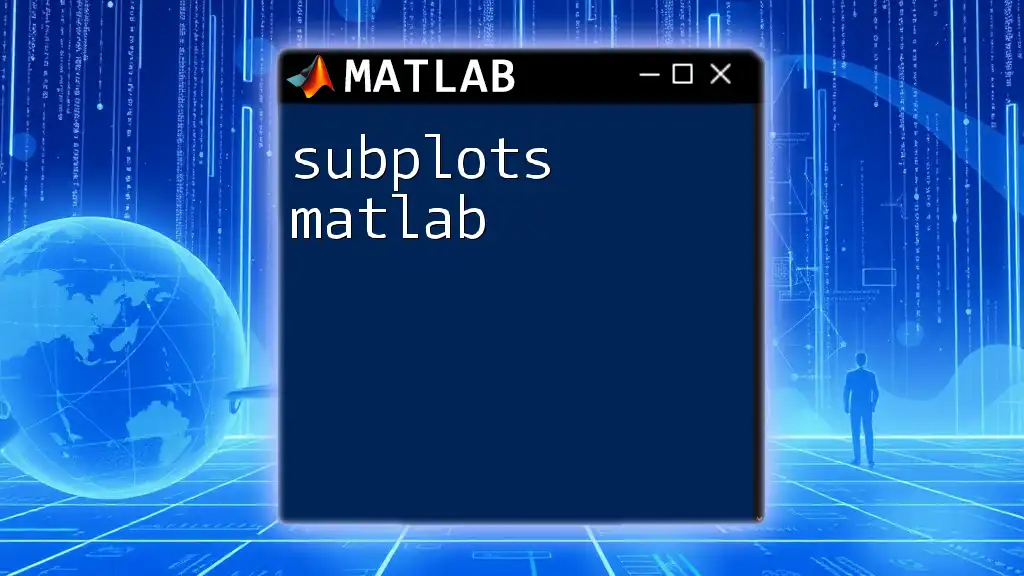
Visualization of Logarithmic Functions
Plotting Logarithmic Functions
MATLAB provides the capability to plot logarithmic functions, offering insights into their behavior. For example, you can plot the natural logarithm function with the following code:
x = linspace(0.1, 10, 100);
y = log(x);
plot(x, y);
title('Plot of y = log(x)')
xlabel('x')
ylabel('y')
grid on
This code snippet will generate a plot displaying how the logarithm function behaves across a specified range of values.
Logarithmic Scales in Graphs
Sometimes, it’s useful to display data on logarithmic scales to better visualize relationships. MATLAB makes this easy with functions like `semilogx()` and `semilogy()` for creating logarithmic axes.
Example: To use logarithmic scales on the y-axis:
x = linspace(1, 100, 100);
y = x.^2;
semilogy(x, y);
title('Semi-logarithmic Plot of y = x^2')
xlabel('x')
ylabel('log(y)')
grid on
The resulting plot showcases the quadratic relationship while compressing the y-axis for clearer visualization.
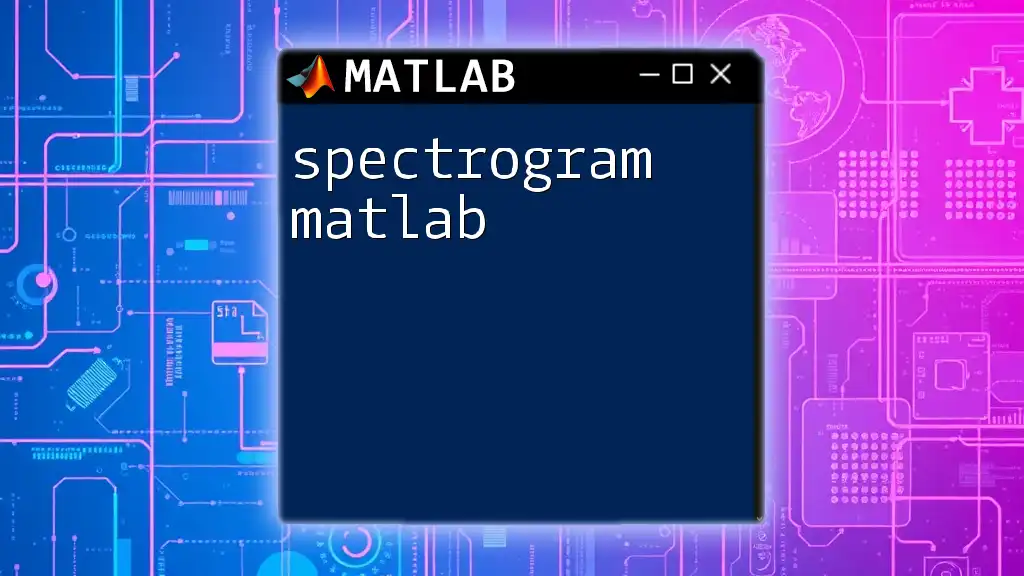
Conclusion
Logarithmic functions in MATLAB open up a realm of possibilities for data analysis, equation solving, and visualization. Mastering commands like `log()`, `log10()`, and `log2()` equips users to handle various mathematical tasks efficiently. We encourage you to explore and practice these functions to deepen your understanding of logarithms in MATLAB. Harnessing their power will elevate your computational skills and enhance your analytical capabilities.
Additional Resources
To further your understanding of logarithmic functions and their application in MATLAB, consider consulting the official MATLAB documentation or engaging with hands-on exercises for practical experience.