In MATLAB, string formatting allows you to create formatted text output by embedding variables and controlling the display of numerical values within a string.
Here’s a simple code snippet demonstrating string formatting using the `sprintf` function:
name = 'Alice';
value = 42;
formattedString = sprintf('The value for %s is %d.', name, value);
disp(formattedString);
Understanding Strings in MATLAB
What is a String?
In MATLAB, a string is a sequence of characters used to represent text. Strings can be created using either character arrays or string arrays, and they play a crucial role in data manipulation, output display, and user interaction within MATLAB scripts and functions.
Creating Strings
Creating strings in MATLAB is straightforward. You can use double quotes for string arrays or single quotes for character arrays. The new `string()` function can also be employed to instantiate string arrays. For example:
myString1 = "Hello, World!"; % Using double quotes to create a string
myString2 = string('Hello, MATLAB!'); % Using the string() function
Both methods are widely used, but string arrays offer benefits in handling text data, such as automatic handling of character encodings and more intuitive manipulation methods.
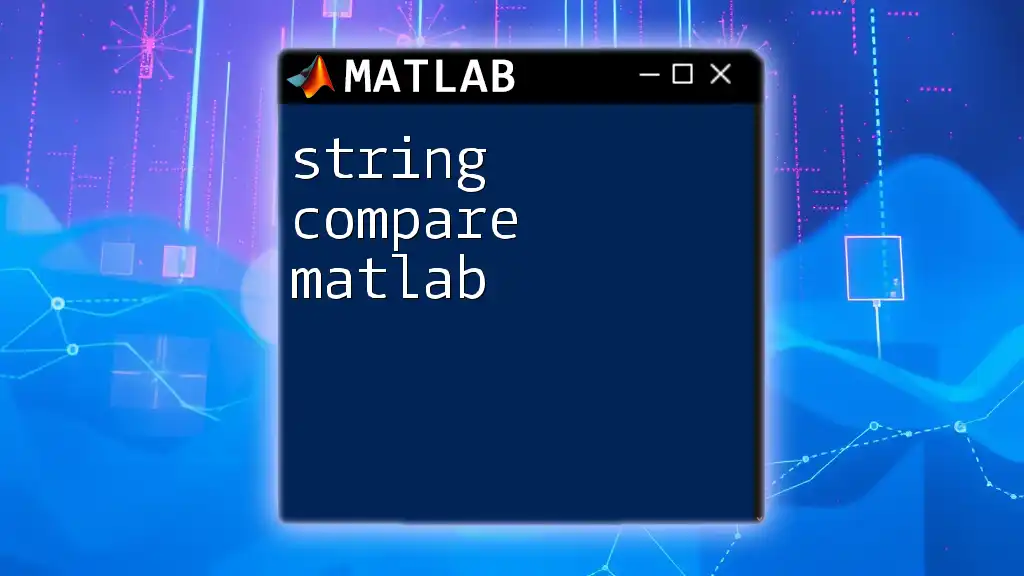
String Formatting Basics
What is String Formatting?
String formatting in MATLAB is essential for converting data into a human-readable format. It is used to create dynamic messages, format numeric outputs, or present data in a structured way. Effective string formatting enhances the clarity and usability of MATLAB outputs.
The `sprintf` Function
The `sprintf` function is one of the primary tools for string formatting in MATLAB. It generates a formatted string based on specified format specifiers without sending output to the console.
Syntax:
formattedString = sprintf(formatSpec, A, ...)
Here's an example that demonstrates how to format a string with numeric values:
formattedString = sprintf('Value: %.2f, Name: %s', 3.14159, 'Pi');
disp(formattedString); % Displays: Value: 3.14, Name: Pi
Explanation of format specifiers:
- `%.2f` specifies that the floating-point number should be displayed with two decimal points.
- `%s` is used for strings.
Common Format Specifiers
Understanding format specifiers is crucial for effective string formatting in MATLAB. Here’s a brief overview of commonly used specifiers:
- `%s`: Represents a string.
- `%d`: Represents an integer in decimal format.
- `%f`: Represents a floating-point number.
- `%x`: Represents an integer in hexadecimal format.
Example with explanations:
num = 255;
fprintf('Decimal: %d, Hexadecimal: %x\n', num, num); % Displays Decimal: 255, Hexadecimal: ff
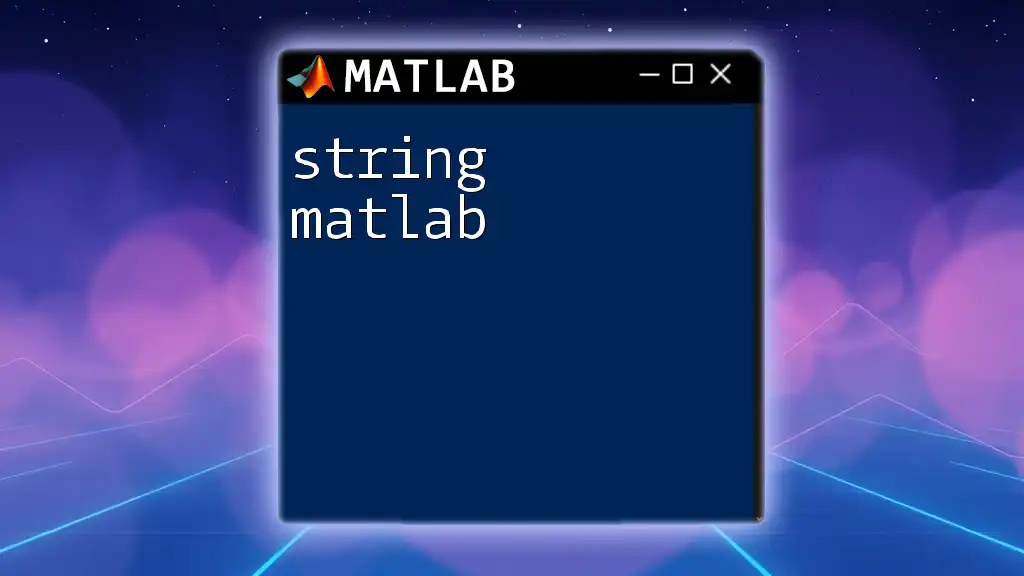
Advanced String Formatting Techniques
Using `fprintf` for Formatted Output
The `fprintf` function is similar to `sprintf`, but it directly outputs formatted data to the command window or a file. Use `fprintf` when immediate display of results is desired.
fprintf('Hello %s!\n', 'MATLAB'); % Displays: Hello MATLAB!
String Concatenation
Concatenating strings efficiently is essential in MATLAB for building dynamic messages. You can use various methods to concatenate strings, including:
-
Using square brackets:
fullString = [myString1, ' ', myString2]; % Combines two strings with a space
-
Using the `strcat()` function:
firstName = "John"; lastName = "Doe"; fullName = strcat(firstName, ' ', lastName); disp(fullName); % Displays: John Doe
Using `compose` for Complex Formatting
For more complex formatting scenarios, the `compose` function is a versatile choice. It is capable of generating multiple formatted strings from arrays of different sizes.
For example:
names = ["Alice", "Bob"];
scores = [95, 85];
results = compose('Student: %s, Score: %d', names, scores);
disp(results);
This code outputs two formatted strings in one go, showcasing the power of array-based formatting.
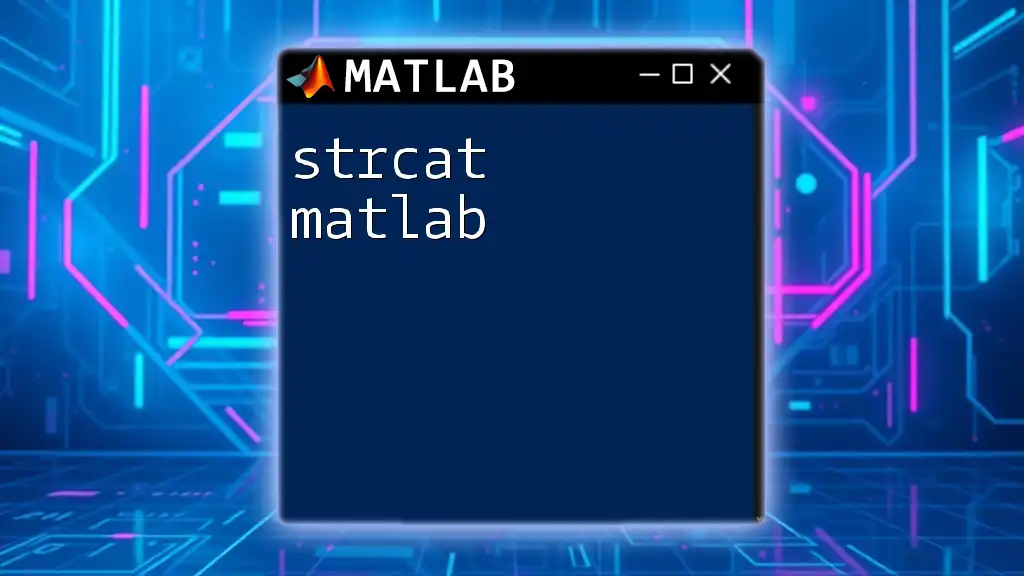
Formatting Numeric Values
Controlling Decimal Precision
It's important to control the precision of numeric outputs to maintain clarity. By specifying the precision in format specifiers, you can easily present numbers to the desired number of decimal places.
piString = sprintf('Value of Pi: %.4f', pi); % Displays: Value of Pi: 3.1416
Formatting Percentages and Scientific Notation
Formatting percentages and numbers in scientific notation is also straightforward. Adjusting specifiers allows you to easily manipulate how numbers appear.
For example, to display a probability as a percentage:
prob = 0.001234;
fprintf('Probability: %.2f%%\n', prob * 100); % Displays: Probability: 0.12%
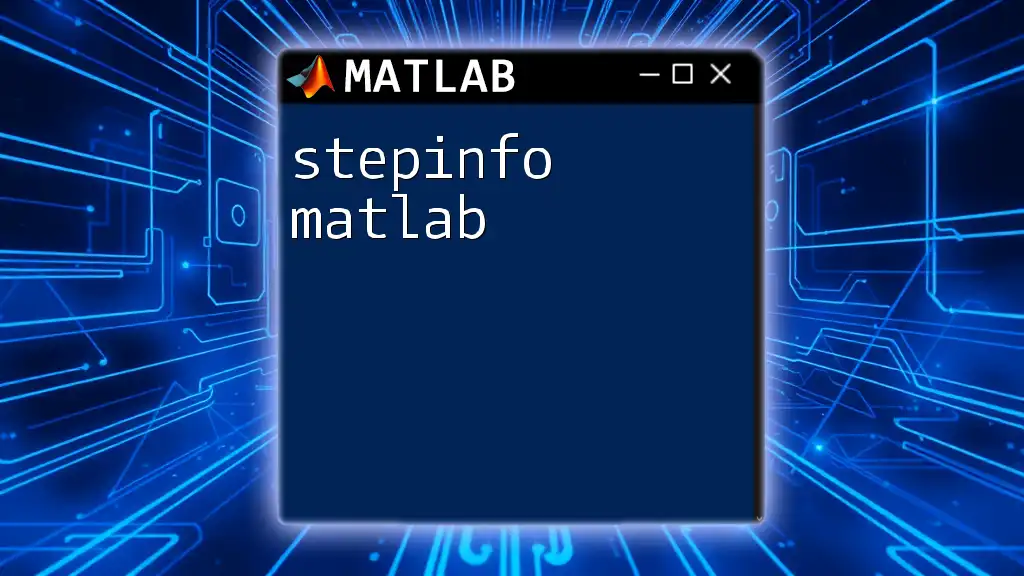
Working with Character Arrays
Differences Between Strings and Character Arrays
While strings and character arrays may seem similar, they have key differences. Character arrays are arrays of individual characters, whereas string arrays are a more modern, flexible way to handle text data in MATLAB. Transitioning to string arrays can simplify many operations.
Formatting Character Arrays
Despite the differences, you can format character arrays using similar techniques as with string arrays. Here's how you can format character arrays:
charArray = 'Sample: %d';
formattedCharArray = sprintf(charArray, 10);
disp(formattedCharArray); % Displays: Sample: 10
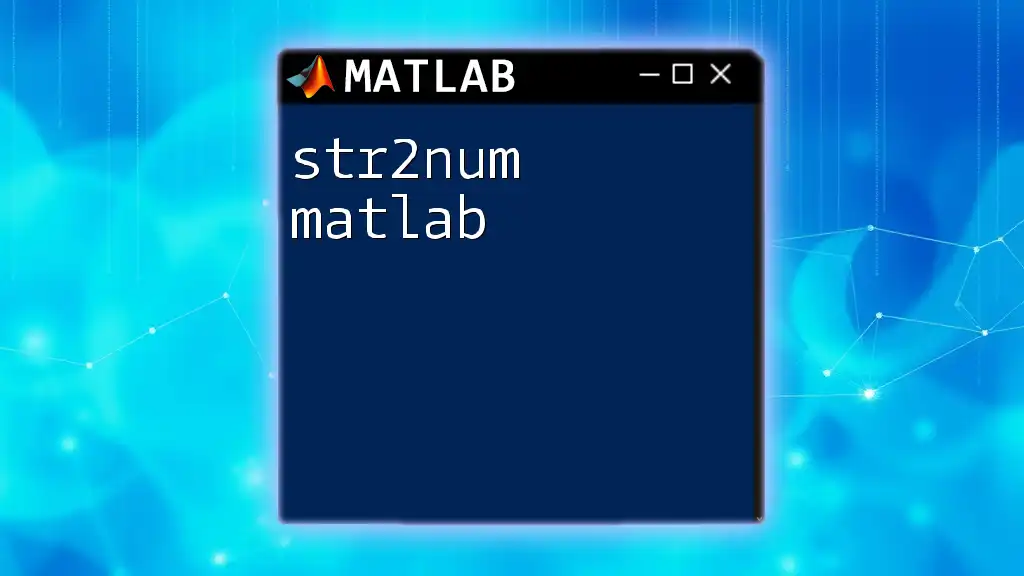
Best Practices for String Formatting
Readability and Maintenance
To ensure the readability of your code, strive to keep formatted strings simple and logically structured. Avoid excessively lengthy format specifiers and complicated expressions that may confuse readers.
Avoiding Common Pitfalls
One common mistake is neglecting to match format specifiers with the provided data types. This can lead to runtime errors or unexpected results. Always double-check that your specified formats align with the actual data types being formatted.
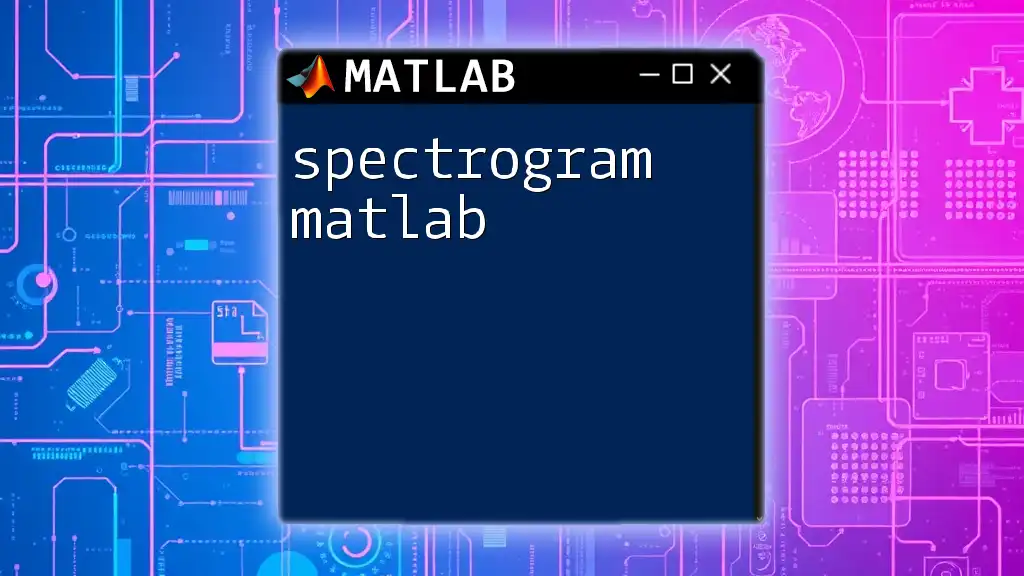
Conclusion
String formatting in MATLAB is a vital skill that enhances the clarity and efficiency of your programming. The use of functions like `sprintf`, `fprintf`, and `compose`, combined with a solid understanding of format specifiers, allows you to present data effectively.
Take the time to practice string formatting, experiment with different functions, and implement what you learn in your projects. Engaging with the community can also provide additional insights and tips for mastering string formatting in MATLAB. For further study, consult MATLAB's official documentation and explore examples that resonate with your specific needs.