The Laplace transform in MATLAB can be computed using the `laplace` function, which takes a symbolic expression or function as an input and returns its Laplace transform.
Here’s a simple example of how to use the `laplace` command in MATLAB:
syms t s
f = exp(-2*t); % Define the function f(t) = e^(-2t)
L = laplace(f, t, s); % Compute the Laplace transform L{f(t)}
disp(L); % Display the result
What is the Laplace Transform?
The Laplace Transform is a fundamental mathematical operation widely used in engineering, physics, and mathematics. It transforms a time-domain function, typically representing a signal or system response, into a complex frequency-domain representation. This operation simplifies many calculations related to linear time-invariant systems, making it easier to analyze and design control systems, electrical circuits, and mechanical systems.
Why Use MATLAB for Laplace Transform?
MATLAB offers significant advantages for conducting Laplace Transforms. It has a powerful Symbolic Math Toolbox that enables users to perform symbolic computations easily, allowing for cleaner representations and manipulations of mathematical constructs. With built-in functions such as `laplace` and `ilaplace`, you can find both the Laplace Transform and its inverse without cumbersome calculations, making it an ideal tool for both education and practical applications.
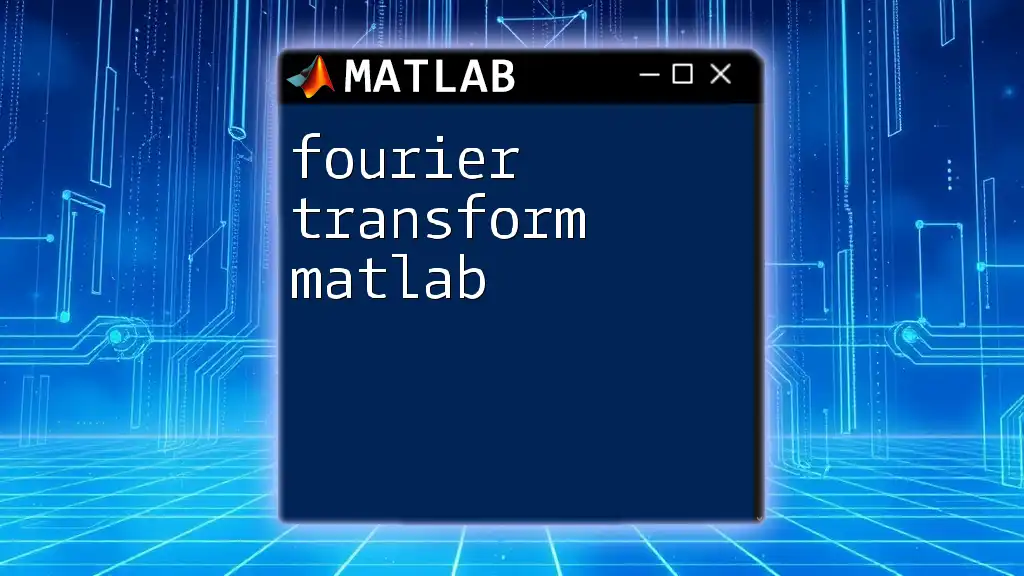
Basic Concepts of Laplace Transform
Mathematical Definition
The mathematical definition of the Laplace Transform is given by the following formula:
\[ L[f(t)] = F(s) = \int_{0}^{\infty} e^{-st} f(t) dt \]
In this formula:
- \( f(t) \) is the function in the time domain
- \( s \) is a complex number frequency parameter
- \( F(s) \) is the transformed function in the frequency domain
Properties of the Laplace Transform
Understanding the properties of the Laplace Transform is essential as they simplify many calculations.
-
Linearity: If \( a \) and \( b \) are constants, then: \[ L[af(t) + bg(t)] = aL[f(t)] + bL[g(t)] \] This means you can treat the transform of a sum as the sum of the transforms.
-
Time Shifting: If \( f(t) \) has a Laplace Transform \( L[f(t)] \), then: \[ L[f(t-a)u(t-a)] = e^{-as} F(s) \] This property allows you to shift functions in time domain.
-
Frequency Shifting: The Laplace Transform can handle frequency shifts, such that: \[ L[e^{at} f(t)] = F(s-a) \]
-
Scaling in the Time Domain: Changing the time variable can be expressed as: \[ L[f(at)] = \frac{1}{a} F\left(\frac{s}{a}\right) \]
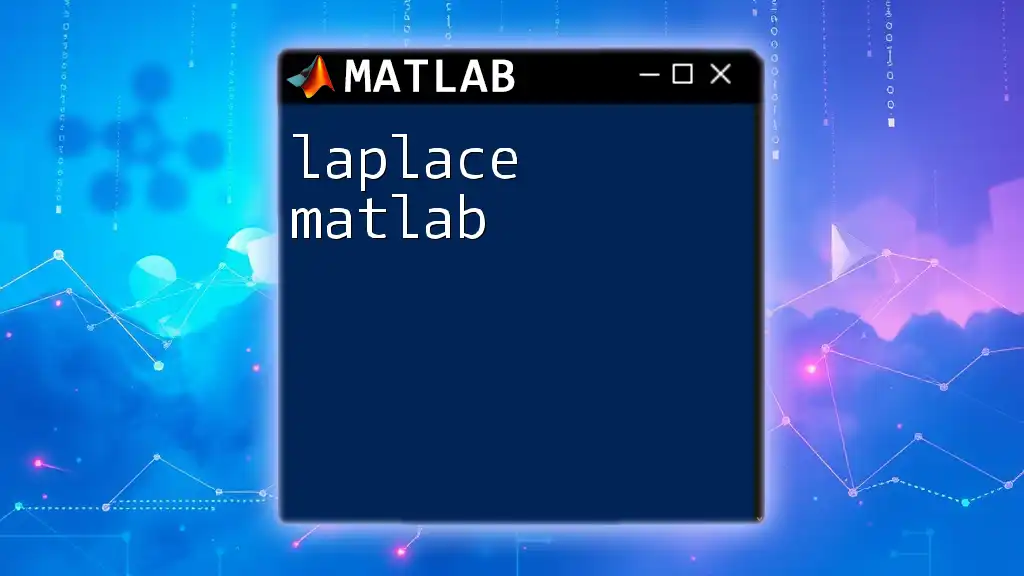
Getting Started with MATLAB
Installing MATLAB
To get started, download MATLAB from the MathWorks website and follow installation instructions. Ensure that you install the Symbolic Math Toolbox for optimal use of Laplace functionalities.
Setting up the Symbolic Toolbox
Before diving into the computations, it’s crucial to verify that the Symbolic Toolbox is installed. The following command checks its installation:
ver symbolic
Basic Commands Overview
Familiarize yourself with essential commands in MATLAB:
- `syms`: Used to declare symbolic variables.
- `laplace`: Calculates the Laplace Transform of a function.
- `ilaplace`: Computes the Inverse Laplace Transform.
For example, to declare a symbolic variable:
syms t s
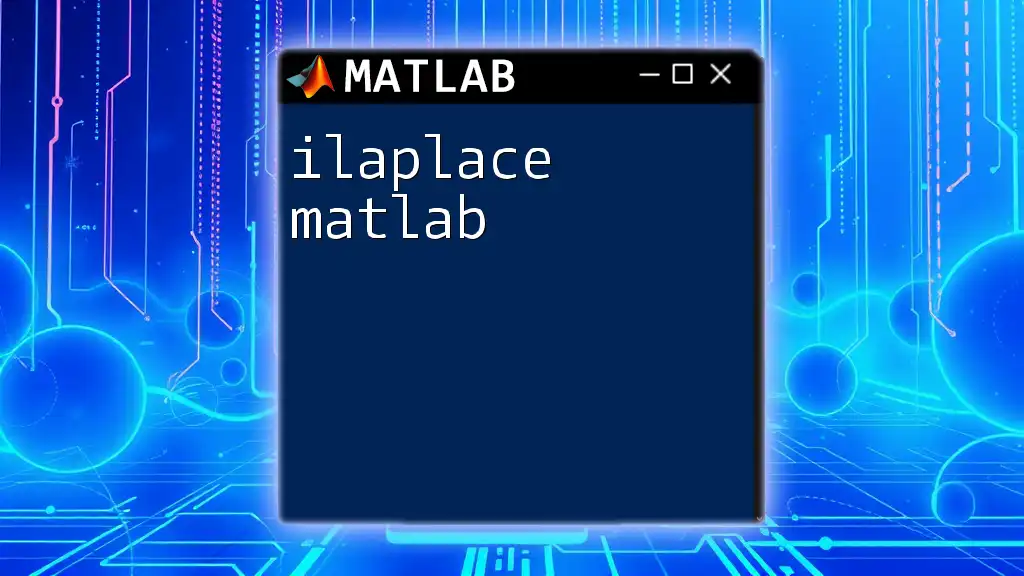
Performing Laplace Transforms in MATLAB
Using the `laplace` Command
The primary command for the Laplace Transform is `laplace`. Here’s how you can use it in its basic form:
syms t s
f = exp(-2*t);
L = laplace(f, t, s)
In this example, `exp(-2*t)` is transformed into the frequency domain.
Example: Laplace Transform of a Polynomial
Let’s consider finding the Laplace Transform of a polynomial function. Using:
syms t s
f = t^2 + 3*t + 5;
L = laplace(f, t, s)
MATLAB returns the transform \( \frac{2}{s^3} + \frac{3}{s^2} + \frac{5}{s} \).
Handling Piecewise Functions
Defining piecewise functions in MATLAB can be achieved using the `piecewise` function. Here is an example for finding its Laplace Transform:
f = piecewise(t < 1, 0, t < 2, t, t >= 2, 1);
L = laplace(f, t, s)
This snippet handles multiple segments of a function, providing a meaningful output in the frequency domain.
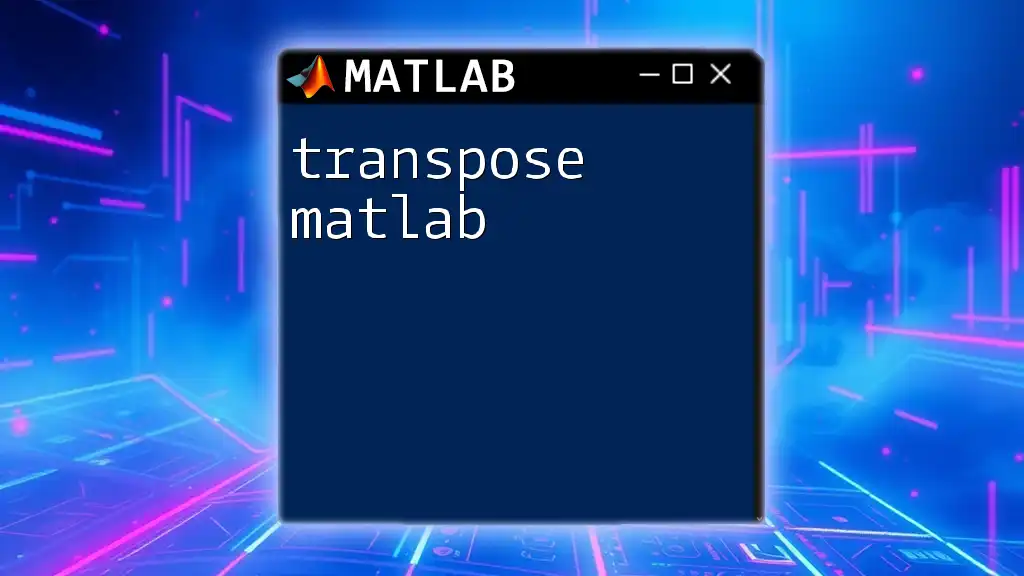
Inverse Laplace Transform in MATLAB
Using the `ilaplace` Command
To perform an inverse operation, the `ilaplace` command is utilized. Here's a basic example of its use:
L = 1/(s^2 + 1);
f = ilaplace(L, s, t)
This takes the frequency-domain function and finds its time-domain equivalent.
Example: Inverse Laplace Transform of a Common Function
Consider this operation:
L = 1/(s^2 + 2*s + 1);
f = ilaplace(L, s, t)
This code provides a clear means of recovering the original function, emphasizing the utility of MATLAB in both forward and reverse transforms.
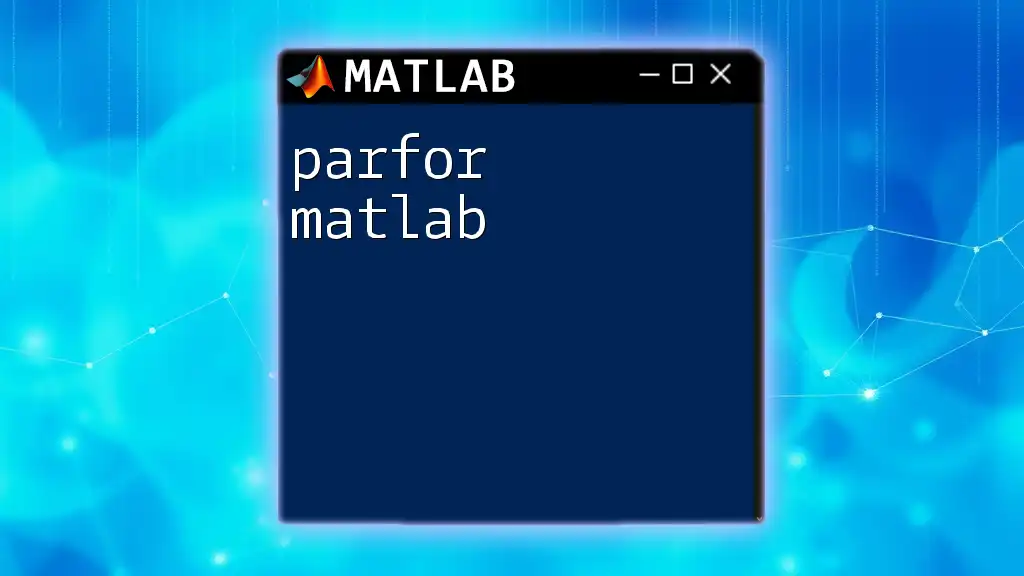
Applications of Laplace Transform in Control Systems
System Response Analysis
In control systems analysis, Laplace transforms help describe the system's behavior in various dynamic conditions. You can convert differential equations into algebraic equations, making solving system responses more manageable.
MATLAB Example: Transfer Function Creation
Creating a transfer function in MATLAB is also straightforward. The `tf` command is used as follows:
num = [1]; % numerator
den = [1, 3, 2]; % denominator
sys = tf(num, den)
In this example, a transfer function representing a second-order system is created, providing insights into the system’s dynamics.
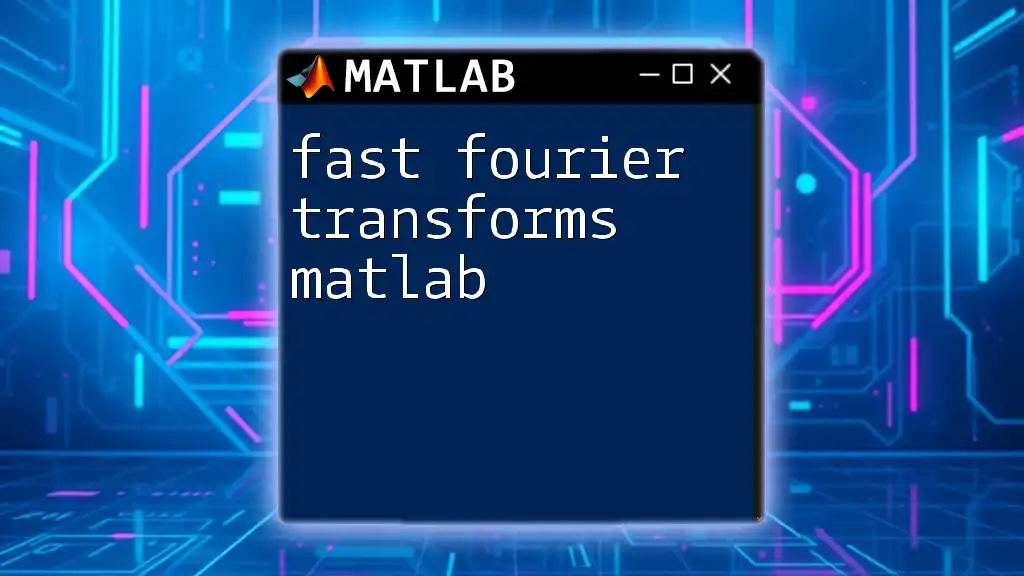
Plotting Laplace Transforms Results
Understanding Function Behavior
Visualization plays a crucial role in interpreting Laplace Transform results. Using MATLAB, you can visualize the output functions clearly Using `fplot` or similar functions aids in this process. For example:
f = ilaplace(L, s, t);
fplot(f, [0, 10])
xlabel('Time (s)')
ylabel('f(t)')
title('Response of the function over time')
This command plots the function over a specified time interval, allowing for an analytical view of the system response.
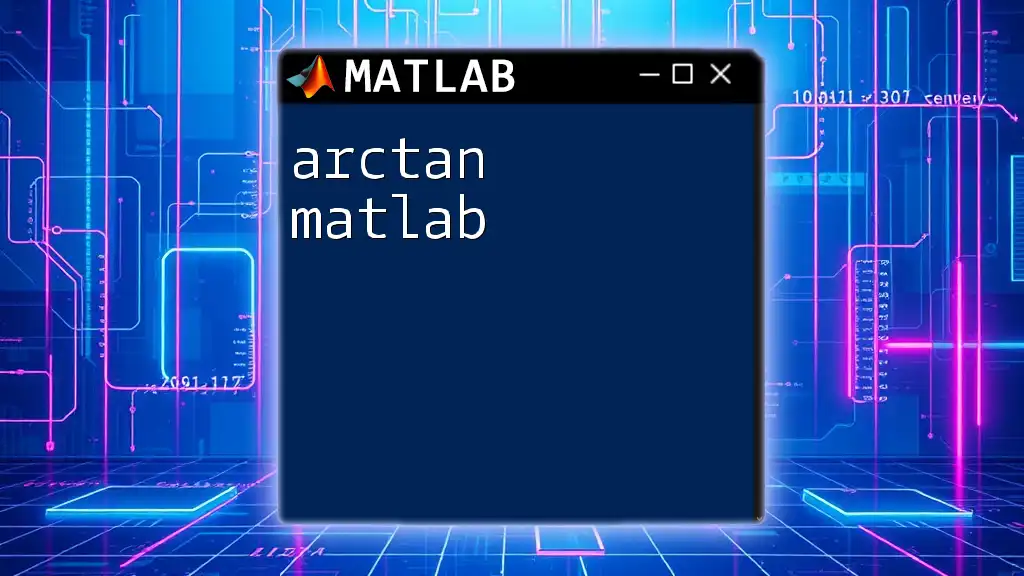
Best Practices for Using Laplace Transform in MATLAB
Tips for Efficient Coding
To ensure a smooth workflow, consider organizing your scripts and functions logically. Write comments and document your code thoroughly. This practice promotes better collaboration and understanding, especially for complex operations.
Debugging Common Errors
Common errors often arise from syntax issues or misuse of commands. Always double-check your function forms and ensure that symbols are correctly defined before executing commands. Utilizing MATLAB’s built-in debugging tools can significantly streamline troubleshooting efforts.
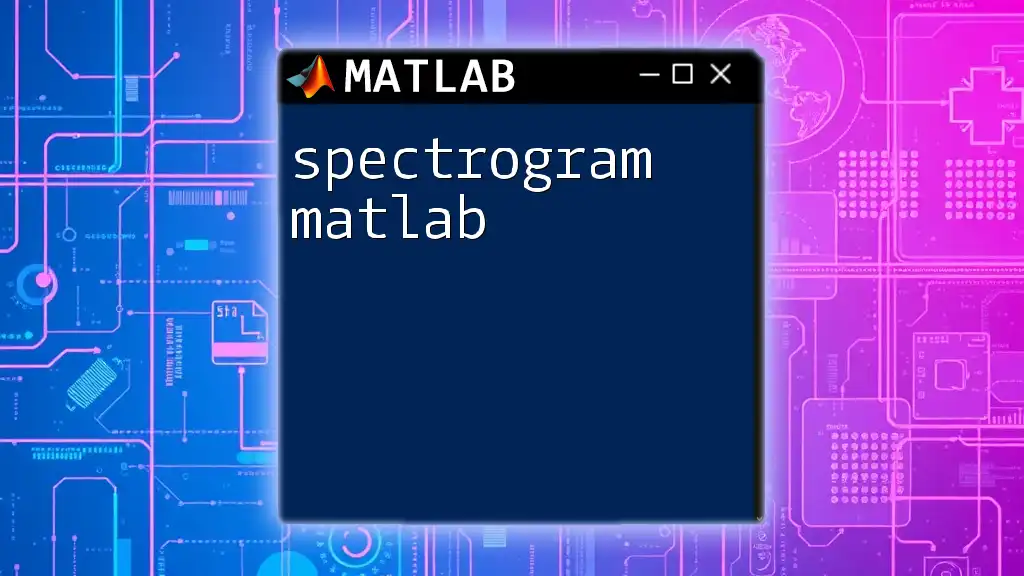
Conclusion
In conclusion, mastering the Laplace Transform in MATLAB provides powerful tools for engineers and scientists alike. From performing algebraic transformations to analyzing complex systems, MATLAB simplifies mathematical processing. By becoming proficient in these commands and concepts, you can leverage MATLAB to tackle a wide array of practical problems in engineering and beyond.
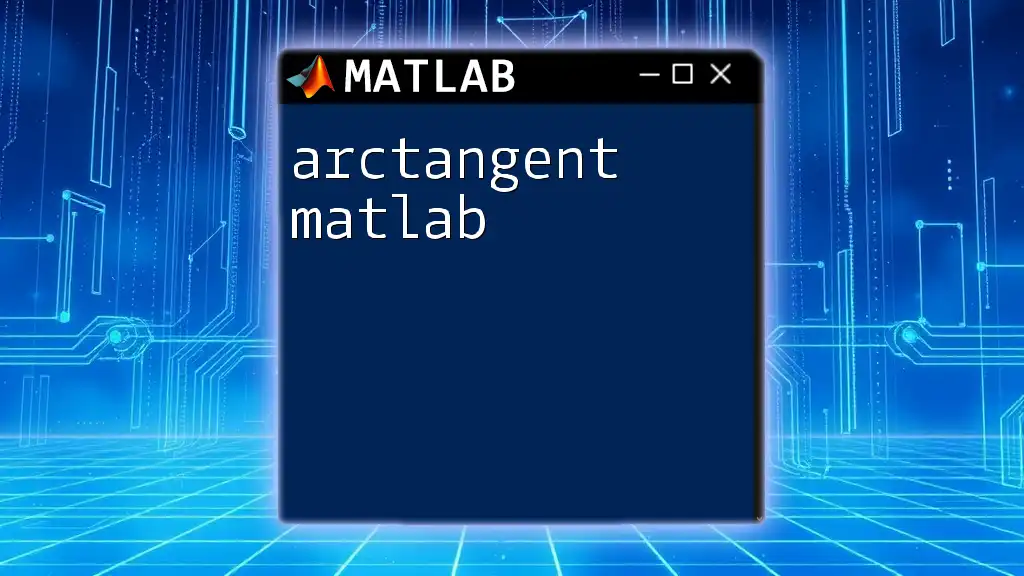
Additional Resources
For further guidance, refer to MATLAB's official documentation on symbolic math and Laplace transforms to deepen your understanding. Engaging with online communities and forums can also enhance your learning experience, allowing you to share insights and seek help when needed.