"Imaging MATLAB" refers to the process of visualizing and manipulating image data using MATLAB's built-in functions to enhance image processing capabilities.
Here’s a simple code snippet to read and display an image:
% Read an image
img = imread('example_image.jpg');
% Display the image
imshow(img);
Getting Started with MATLAB for Image Processing
Setting Up MATLAB
To begin your journey with imaging in MATLAB, first ensure you have MATLAB installed on your system. Download the latest version from the official MATLAB website, and follow the on-screen instructions to complete the installation. Once installed, familiarize yourself with the Image Processing Toolbox, which is specifically designed to help you work with images easily and efficiently.
Basic MATLAB Commands for Image Handling
MATLAB offers several fundamental commands for handling images that every beginner should learn.
- `imread()`: This command is essential for loading an image from your files into the MATLAB workspace.
- `imshow()`: Use this command to display the image in a figure window.
- `imwrite()`: Save images back to your disk using this command.
Here's a simple example to demonstrate these commands:
img = imread('example.jpg'); % Read an image file
imshow(img); % Display the image
imwrite(img, 'output.png'); % Write the image to a new file
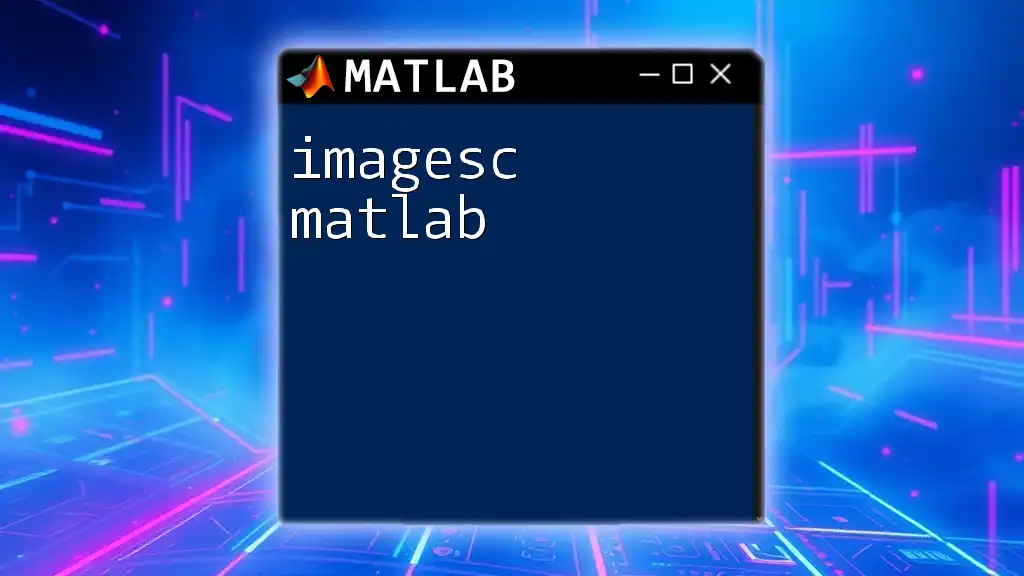
Understanding Image Data Types
Image Data Types in MATLAB
Understanding image data types is crucial as it affects your image processing tasks. MATLAB supports several image data types, including:
- `uint8`: This is the most common data type for images, where pixel values range from 0 to 255.
- `uint16`: Used for images that require a higher bit depth.
- `double`: This data type is useful for performing calculations or transformations on images.
The importance of data types lies in their impact on processing and displaying images. For instance, using `double` may yield different results when manipulating image intensities.
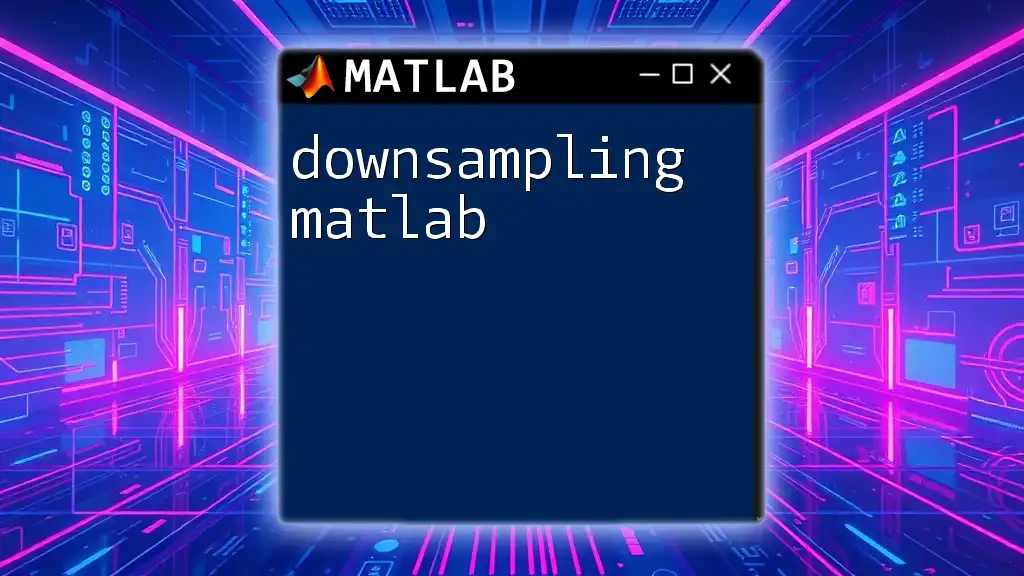
Image Manipulation Techniques
Basic Image Manipulation
Once you have your image loaded into MATLAB, you may want to modify it. Basic image manipulation techniques include:
- Resizing: Change the dimensions of an image without altering its aspect ratio.
- Cropping: Focus on a specific part of the image.
- Rotating: Alter the orientation of the image.
- Flipping: Reverse the image either horizontally or vertically.
Here’s how you can use these techniques:
resizedImg = imresize(img, 0.5); % Resize image to 50%
croppedImg = imcrop(img, [50 50 100 100]); % Crop the image
rotatedImg = imrotate(img, 90); % Rotate the image by 90 degrees
Color Space Conversion
In many imaging applications, converting between color spaces is necessary. Understanding RGB, HSV, and Grayscale color spaces is essential for effective image processing. You can convert images between these spaces easily:
hsvImg = rgb2hsv(img); % Convert RGB to HSV
grayImg = rgb2gray(img); % Convert RGB to Grayscale
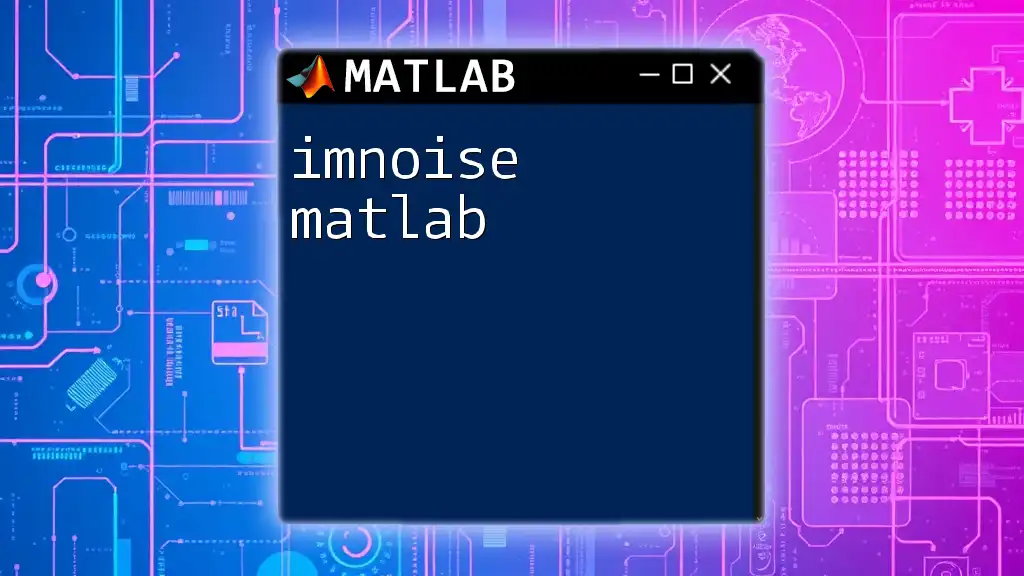
Advanced Image Processing Techniques
Filtering and Noise Reduction
Often, images can contain noise. Applying filters is a common technique for smoothing images. MATLAB provides various filtering options, such as Gaussian filters, which help in noise reduction:
filteredImg = imgaussfilt(img, 2); % Apply Gaussian filter with a standard deviation of 2
Edge Detection and Enhancement
Edge detection is a pivotal step in many image processing applications, allowing you to identify boundaries within images. MATLAB supports several edge detection methods, including Sobel and Canny algorithms:
edges = edge(rgb2gray(img), 'Canny'); % Apply Canny edge detection
Morphological Image Processing
Morphological operations are used to process shapes or structures within an image. Common operations include dilation and erosion:
se = strel('disk', 5); % Create a structuring element
dilatedImg = imdilate(grayImg, se); % Perform dilation
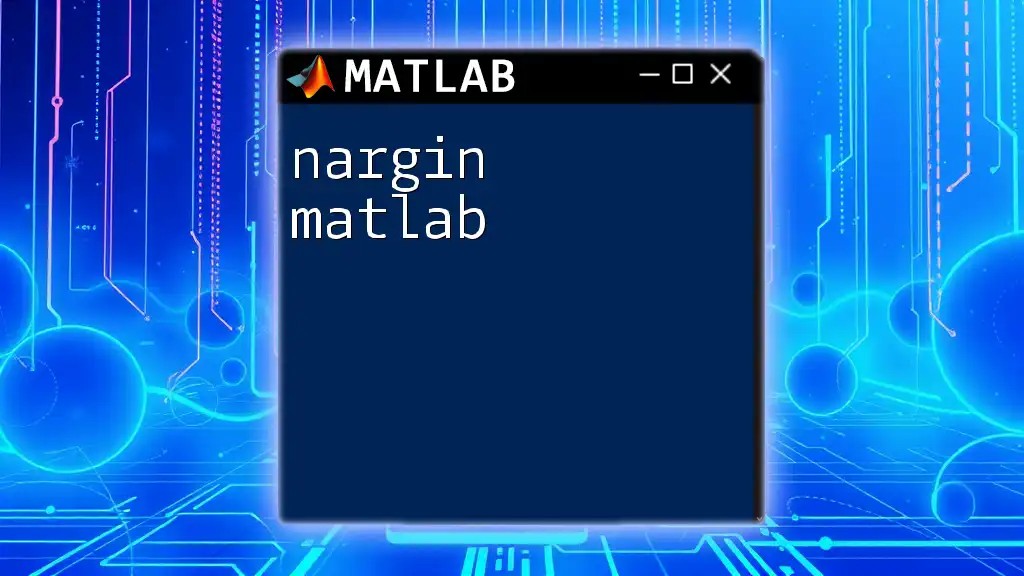
Analyzing Images
Image Statistics and Measurement
MATLAB offers tools for analyzing images, providing insights into image properties such as area and bounding boxes. This is especially useful for segmenting images and understanding shapes:
stats = regionprops('table', bwlabel(edges), 'Area', 'BoundingBox'); % Analyze properties of labeled regions
Feature Detection
Feature detection allows you to identify key points in your images, which is vital for further processing like object detection. By utilizing keypoint detectors such as SURF:
points = detectSURFFeatures(grayImg); % Detect SURF features in the grayscale image
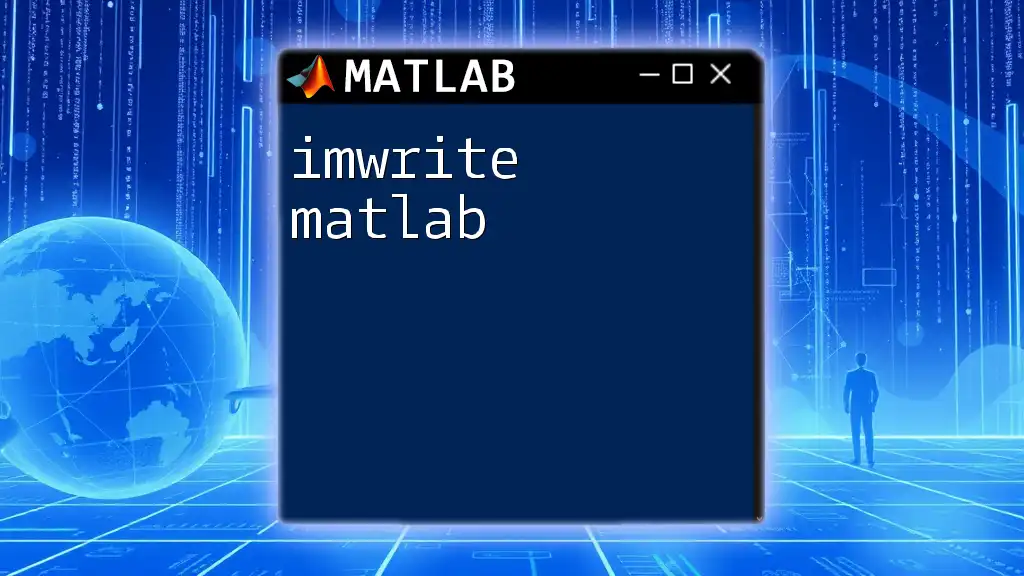
Visualizing Image Data
Creating Plots and Visualizations
To analyze an image effectively, visualizing the data is key. You can create histograms or visualize pixel intensity distributions using the imhist function:
imhist(grayImg); % Display the histogram of the grayscale image
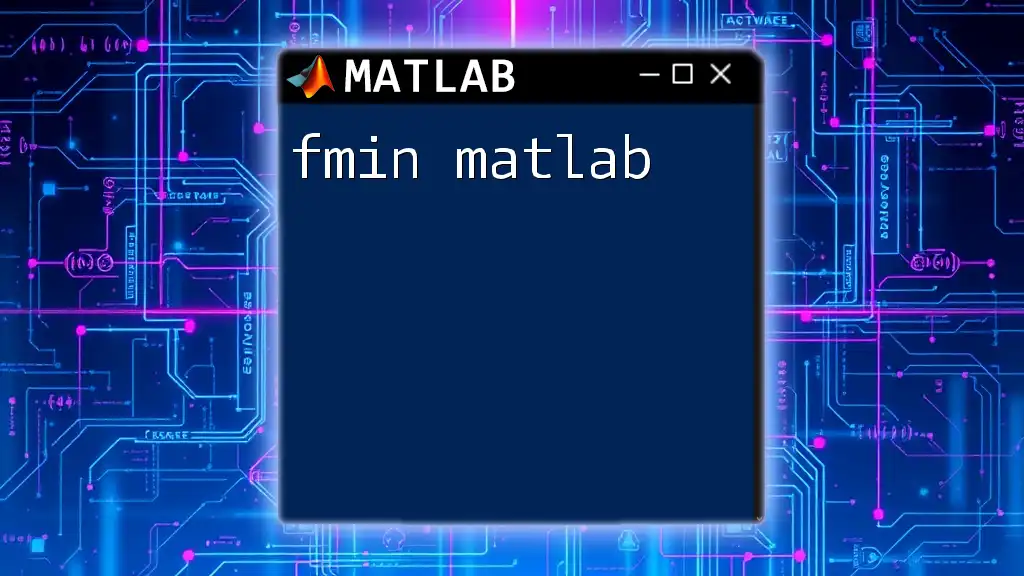
Common Applications of Image Processing in MATLAB
Medical Imaging
Imaging MATLAB is heavily used in the medical field, particularly for analyzing MRI and CT scans, helping in diagnostics and treatment planning.
Object Detection and Recognition
MATLAB provides robust framework support for detecting and recognizing objects, a feature utilized in autonomous vehicles, security systems, and more.
Image Restoration Techniques
Various techniques in MATLAB help to restore images, including inpainting for removing unwanted objects and deblurring for sharpening images.
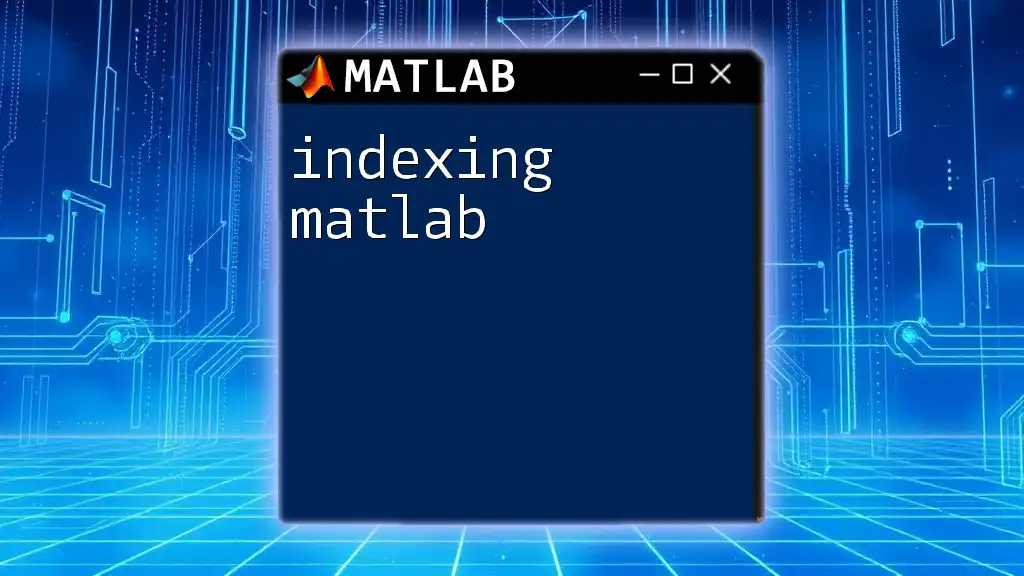
Troubleshooting Common Issues
When working with imaging in MATLAB, you may encounter some common errors. Here is a brief overview:
- File Not Found: Ensure the file path is correctly specified for image reading.
- Data Type Mismatches: Verify that you're using appropriate data types for your images, especially during calculations.
Keep troubleshooting techniques handy, and don’t hesitate to refer to the MATLAB documentation for assistance.
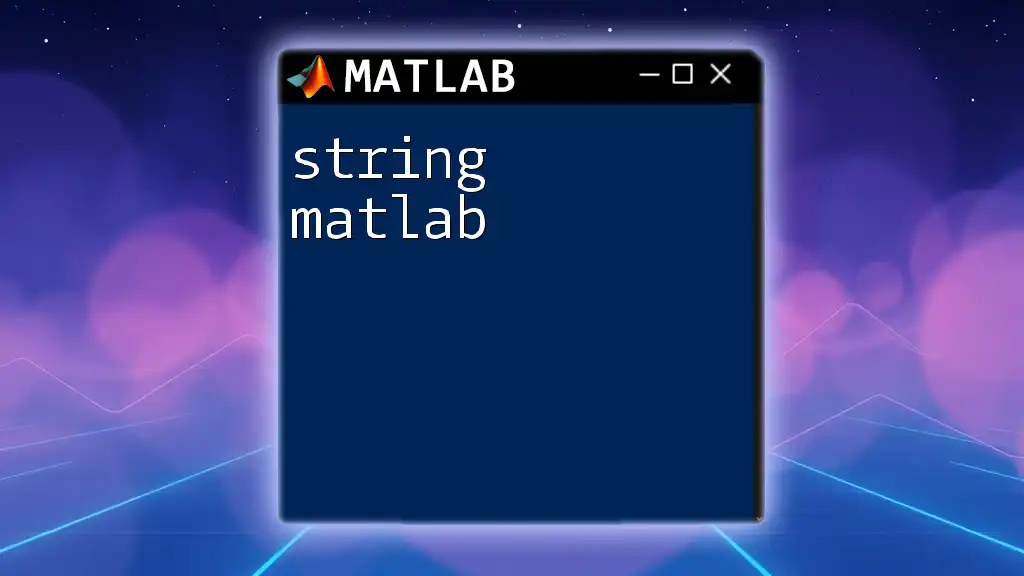
Conclusion
In summary, mastering imaging in MATLAB involves understanding pivotal concepts, commands, and techniques for effective image processing. From basic image handling to advanced manipulation and analysis techniques, MATLAB offers a powerful capability for any aspiring image processing expert. For those looking to dive deeper into this fascinating field, don't hesitate to explore additional resources and practice regularly. A wealth of learning awaits you in the world of imaging MATLAB!