"To simplify MATLAB usage, we focus on providing clear, concise commands and examples that enable users to quickly grasp essential functionalities without getting bogged down in complexity."
Here's a simple code snippet demonstrating how to create a basic plot in MATLAB:
x = 0:0.1:10; % Generate values from 0 to 10 with an increment of 0.1
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Plot y against x
xlabel('x values'); % Label for x-axis
ylabel('sin(x)'); % Label for y-axis
title('Sine Function'); % Title of the plot
grid on; % Turn on the grid
The Basics of MATLAB Commands
Understanding MATLAB Syntax
MATLAB commands are structured into a clear and concise syntax, comprising an action or function followed by specific arguments. Understanding this syntax is key to simplifying MATLAB usage. For instance, a simple arithmetic operation like adding two numbers can be expressed as:
result = 5 + 3;
This straightforward command assigns the sum of 5 and 3 to the variable `result`, showcasing how MATLAB allows for immediate calculations with minimal syntax.
Variables and Data Types
Defining Variables
Creating variables in MATLAB is as easy as specifying a name followed by an assignment operator (`=`). This flexibility enables users to manage data effortlessly. For example:
a = 10; % Assigning a scalar
b = [1, 2, 3, 4]; % Assigning a vector
Common Data Types in MATLAB
MATLAB supports several data types that are fundamental to its operation:
- Double: Default numeric type for real numbers.
- Integer: Used for whole numbers, categorized into different types (e.g., `int8`, `int16`).
- Char: Represents single characters or strings of text.
- Logical: Boolean type, true or false.
A practical example assigning different data types can look like this:
number = 42; % Double
charArray = 'Hello, MATLAB'; % Char
isTrue = true; % Logical
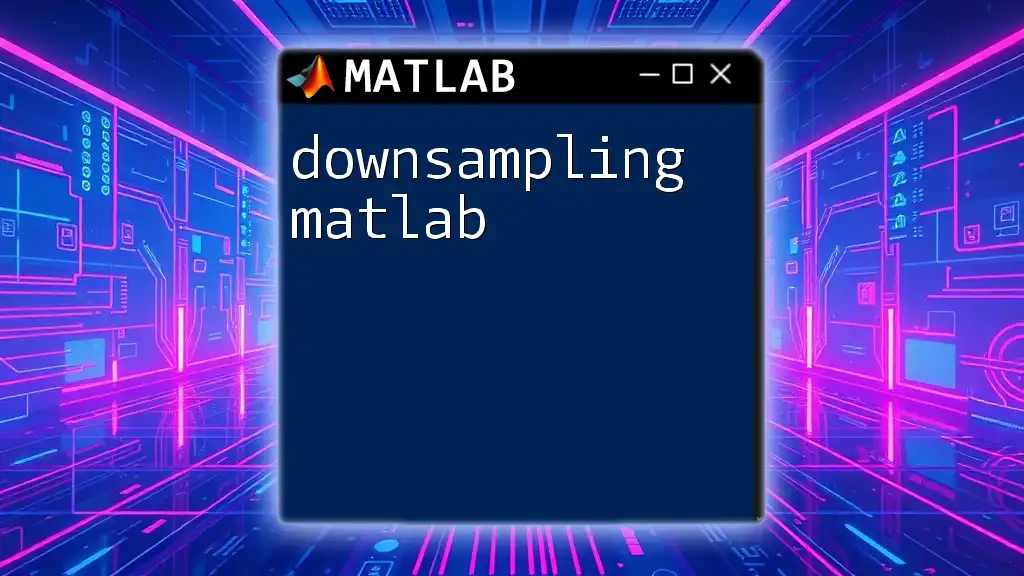
Simplification Techniques in MATLAB
Using Built-in Functions
MATLAB is renowned for its extensive library of built-in functions designed to perform common tasks while eliminating the need for lengthy code. These functions provide a shorthand method to achieve complex calculations. For example, instead of manually calculating an average, one can simply use the `mean()` function:
data = [10, 20, 30, 40];
average = mean(data); % Calculates the mean directly
Using built-in functions not only simplifies the code but also enhances readability and reduces potential errors.
Utilizing Scripts and Functions
Creating Scripts for Repetitive Tasks
Scripting in MATLAB allows users to automate repetitive processes, thus simplifying MATLAB interactions significantly. By encapsulating code into script files, one can execute complex sequences of commands with a single call, saving time and reducing error potential.
Here’s a simple example of a script that generates a plot:
% myPlotScript.m
x = 0:0.1:10; % Generate x values
y = sin(x); % Compute y values
plot(x, y); % Create the plot
title('Sine Wave'); % Set title
xlabel('X-axis'); % Label x-axis
ylabel('Y-axis'); % Label y-axis
Creating Custom Functions
Custom functions can be written to further encapsulate specific tasks, leading to even more concise code and reusability. A quick overview of creating a custom function to sum an array can be portrayed as follows:
function total = sumArray(arr)
total = sum(arr); % Use built-in sum function
end
This function allows users to sum any array with a simple call, promoting code reuse and clarity.
Vectorization
What is Vectorization?
Vectorization refers to the process of converting iterative operations into vector-based operations, significantly improving computational efficiency. It is a core concept in MATLAB that enables quicker execution times and simplified code.
Examples of Vectorization in MATLAB
To illustrate, consider the difference between using a loop and vectorization:
Traditional Method Using Loops:
sum = 0;
for i = 1:length(arr)
sum = sum + arr(i);
end
Vectorized Method:
sum = sum(arr); % Direct summation using a built-in function
By eliminating explicit loops, vectorized operations lead to cleaner, faster code, making the task of simplifying MATLAB more effective.
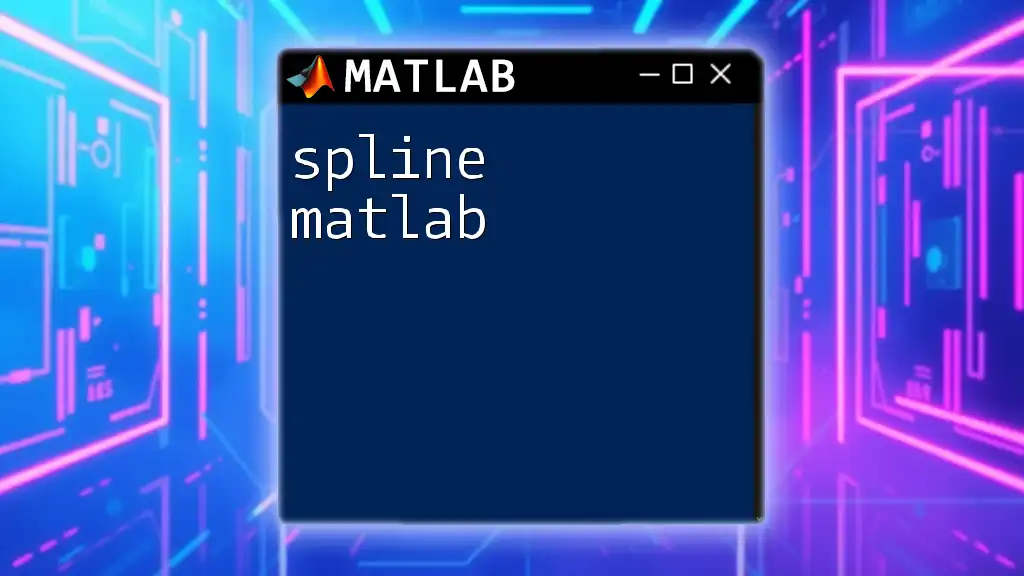
Tips for Writing Clear and Concise MATLAB Code
Code Organization and Readability
Organizing your code well and maintaining readability is critical. Comments are an essential part of this practice. They provide clarity to your code, enabling others (or you at a later time) to understand the logic and purpose behind each section.
For example:
% Calculate the mean of an array
data = [1, 2, 3, 4, 5];
averageValue = mean(data); % Mean calculation
Indentation and Formatting
Proper indentation plays a vital role in enhancing code readability. Consistent formatting makes it easier to identify sections of code and control structures, such as loops and conditionals. For instance:
if averageValue > 3
disp('Above average');
else
disp('Below average');
end
Using Help and Documentation
MATLAB comes equipped with rich help documentation, which can be leveraged to clarify uses of functions and commands. Using the `help` or `doc` commands can lead users toward a better understanding:
help mean % Displays detailed information about the mean function
This practice ensures that users can quickly access vital information, thus simplifying the learning curve.
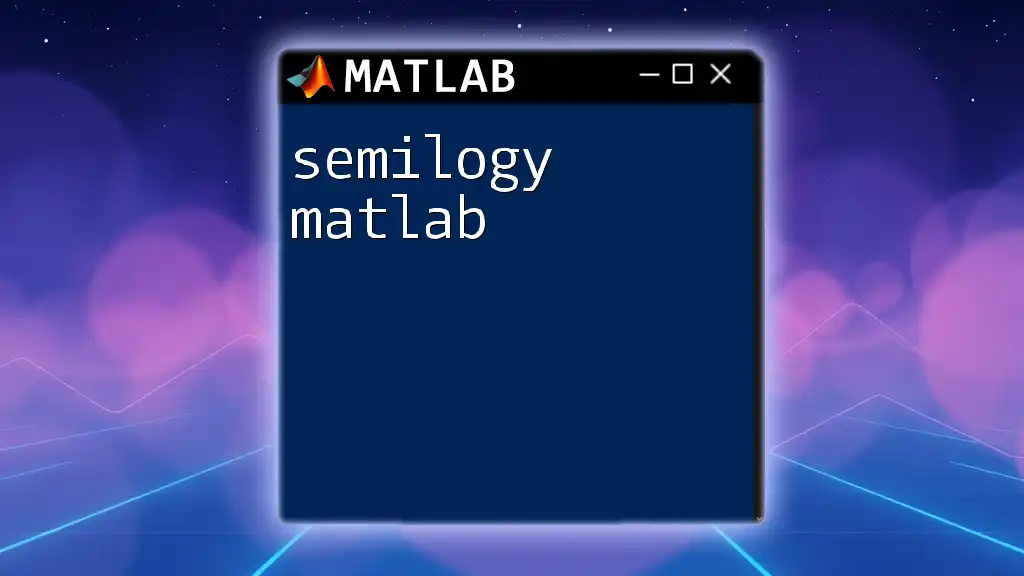
Interactive Programming with MATLAB
Using the Command Window Efficiently
The command window is a powerful feature of MATLAB that allows for quick computations and tests. Utilizing shortcuts such as command history can dramatically speed up workflow. Additionally, interactive plotting allows users to visualize data instantly, enhancing the understanding of results.
x = 0:0.1:10;
y = cos(x);
plot(x, y); % Interactive plot of cosine function
Debugging Simplified
Effective debugging strategies are crucial for any programmer. MATLAB offers several debugging tools that can simplify this process:
- Display Variables: Use `disp()` to output variable values at critical points.
- Breakpoints: Set breakpoints to pause execution and inspect variables at runtime.
breakpoint; % Set a breakpoint in your script to pause for inspection
By employing these techniques, users can quickly identify and rectify errors, which further streamlines the development process.
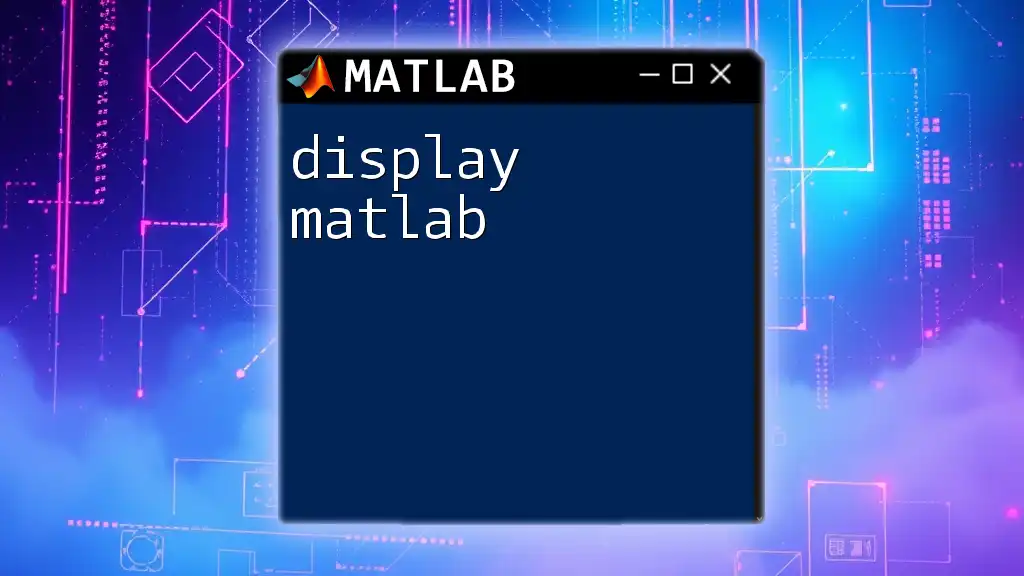
Advanced Simplification Techniques
MATLAB Toolboxes
MATLAB's rich ecosystem includes a variety of specialized toolboxes tailored for specific domains such as Image Processing and Signal Processing. These toolboxes contain pre-built functions that simplify complex tasks, allowing users to focus on their analysis rather than implementing exhaustive algorithms.
For instance, using the Image Processing toolbox for filtering images can reduce elaborate code to a single command:
filtered_image = imfilter(original_image, filter_kernel); % Simple image filtering
GUI Development for Enhanced User Experience
Creating Graphical User Interfaces (GUIs) in MATLAB can significantly enhance user experiences. For applications that require user interaction, GUIs simplify procedures by providing intuitive interfaces.
Developing a basic GUI might involve using code similar to:
% Basic GUI creation
hFig = uifigure;
hButton = uibutton(hFig, 'Text', 'Click Me');
This simplicity allows users to interact with their programming more directly and intuitively.
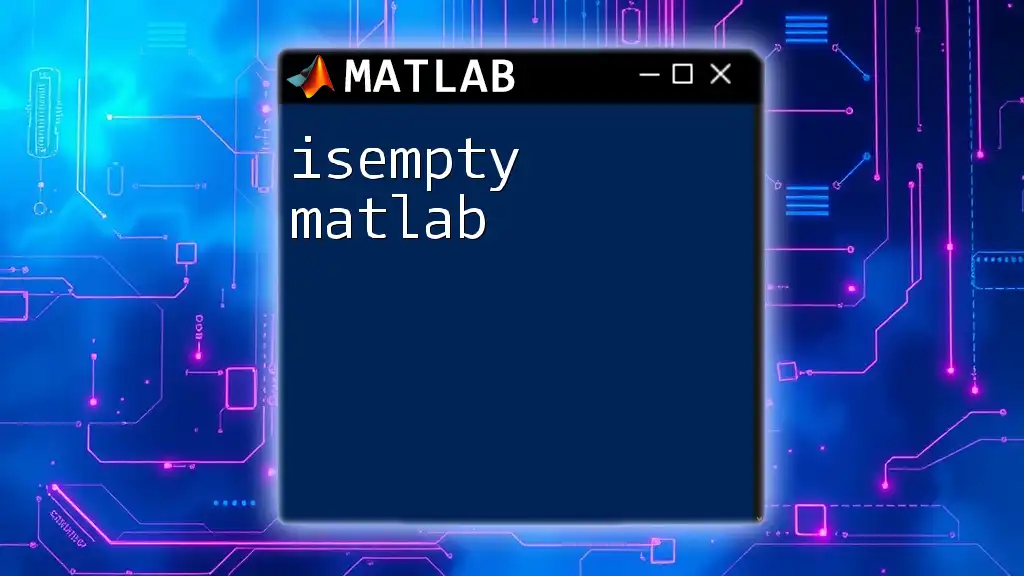
Conclusion
In summary, simplifying MATLAB can elevate not just your coding experience, but also the overall efficacy of your programming efforts. By leveraging built-in functions, mastering scripting, and employing vectorization techniques, users can significantly reduce complexity. Encourage a practice-oriented approach, integrating these simplification strategies into everyday tasks and projects to become a more proficient MATLAB user.
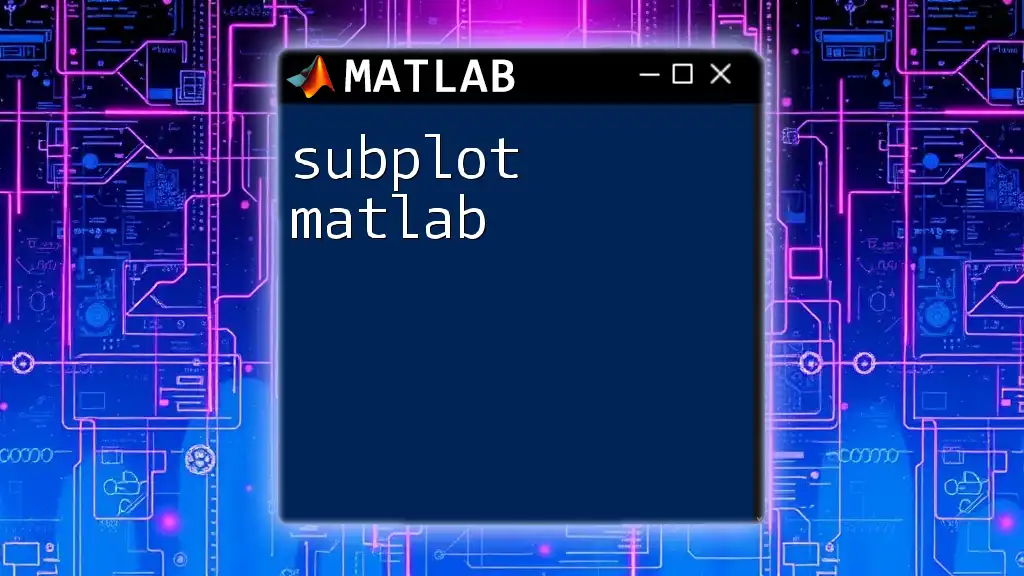
Call to Action
If you're eager to delve deeper into MATLAB and learn more about simplifying MATLAB, join our learning community today! Explore resources, enroll in courses, and transform your approach to MATLAB programming for enhanced efficiency and clarity.