Piecewise MATLAB allows users to define functions with multiple expressions, each applicable for a certain interval of the input variable, facilitating complex conditional calculations.
Here's a simple example:
f = @(x) piecewise(x < 0, -1, x == 0, 0, x > 0, 1);
Understanding Piecewise Functions
Definition and Concept
A piecewise function is a fundamental mathematical function that is defined by multiple sub-functions, each of which applies to a certain interval or condition. The importance of piecewise functions is vast, including applications across various domains such as engineering, economics, computer science, and more. They provide a flexible way to model complex behaviors that cannot be captured using a single expression.
Basic Structure
Typically, a piecewise function consists of segments defined by a condition that dictates how the function behaves in each interval. For example, a function may behave differently when its input is positive versus negative. Understanding how conditions work is crucial for properly defining piecewise functions and ensuring they accurately reflect the desired mathematical behavior.
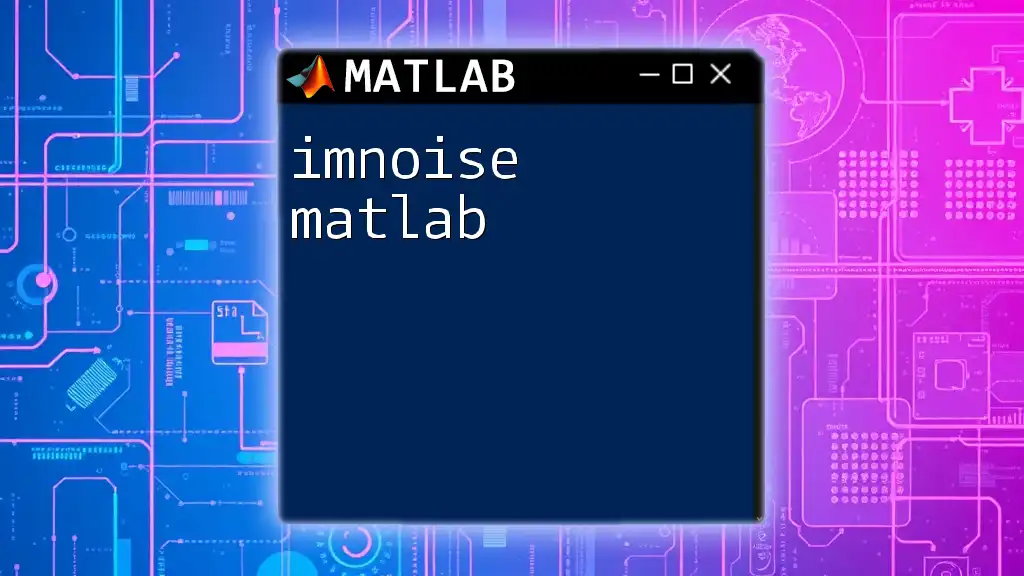
Implementing Piecewise Functions in MATLAB
Using `piecewise` Function
The easiest way to implement piecewise functions in MATLAB is through the `piecewise` function. This function is particularly user-friendly for symbolic calculations and can handle multiple conditions seamlessly.
Syntax and Basic Usage: To define a piecewise function in MATLAB, you can use the following syntax:
syms x
f = piecewise(condition1, value1, condition2, value2, ...);
Example of a Simple Piecewise Function:
syms x
f = piecewise(x < 0, -1, x == 0, 0, x > 0, 1);
In this example, the function `f` returns -1 for any `x` less than zero, 0 when `x` equals zero, and 1 for any `x` greater than zero. This illustrates how easy it is to specify conditions with clear corresponding outputs.
Using Logical Indexing
Logical indexing allows you to create piecewise functions by applying conditions directly to arrays. This method utilizes MATLAB’s capability to perform element-wise operations, making it efficient for large datasets.
Advantages of Using Logical Conditions:
- Clear and concise code
- Easily extensible for complex conditions
- Better performance on larger data sets
Example Code Snippet:
x = -5:0.1:5;
y = zeros(size(x)); % Initialize y with zeros
y(x < 0) = -1;
y(x == 0) = 0;
y(x > 0) = 1;
plot(x, y);
In this code, we initialize `y` with zeros and apply conditions to assign corresponding values based on intervals of `x`. This not only clarifies the implementation but also visually represents the function in a straightforward manner through plotting.
Using `if-else` Statements
While the `piecewise` function is effective, sometimes you may prefer the control that `if-else` statements provide, especially for more complex scenarios.
When to Use `if-else` for Piecewise Functions: Using `if-else` is beneficial if you plan to have more complicated logic or if your piecewise function requires nested conditions.
Example with `if-else`:
x = -5:0.1:5;
y = zeros(size(x));
for i = 1:length(x)
if x(i) < 0
y(i) = -1;
elseif x(i) == 0
y(i) = 0;
else
y(i) = 1;
end
end
plot(x, y);
In this example, we iterate through each value in `x`, using an `if-else` structure to assign `y` values based on the conditions. This approach, while straightforward, highlights the flexibility of MATLAB when implementing piecewise functions.
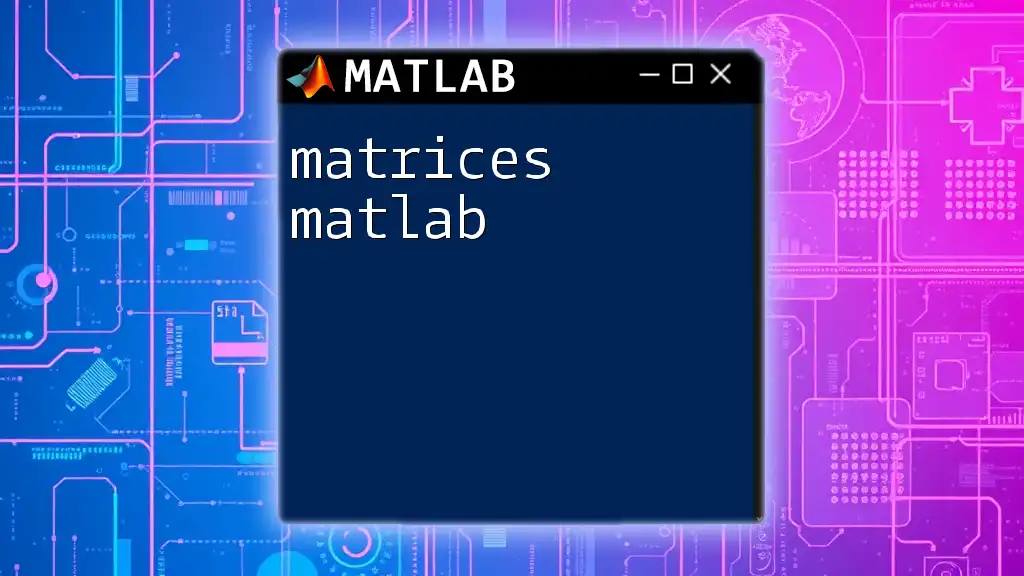
Visualizing Piecewise Functions
Plotting Piecewise Functions
Visualization is a powerful tool in understanding the behavior of mathematical functions, and piecewise functions are no exception. By plotting these functions, you provide a visual representation that can help elucidate their behavior across different intervals.
Why Visualization Matters: Visualizing piecewise functions allows you to grasp complex behavior patterns more intuitively, making it easier to identify transitions between different states.
Tips for Effective Plotting:
- Use grid lines to improve readability.
- Choose appropriate markers to highlight key points.
- Always label the x-axis and y-axis for clarity.
Example Plot of a Piecewise Function
x = -5:0.1:5;
y = zeros(size(x));
y(x < -2) = -1;
y(x >= -2 & x < 2) = 0;
y(x >= 2) = 1;
figure;
plot(x, y, 'LineWidth', 2);
xlabel('x-axis');
ylabel('y-axis');
title('Piecewise Function Visualization');
grid on;
This code demonstrates the visualization of a piecewise function segmented into three intervals. Each segment behaves differently, reinforcing the way MATLAB allows for complex representations with ordinary commands.
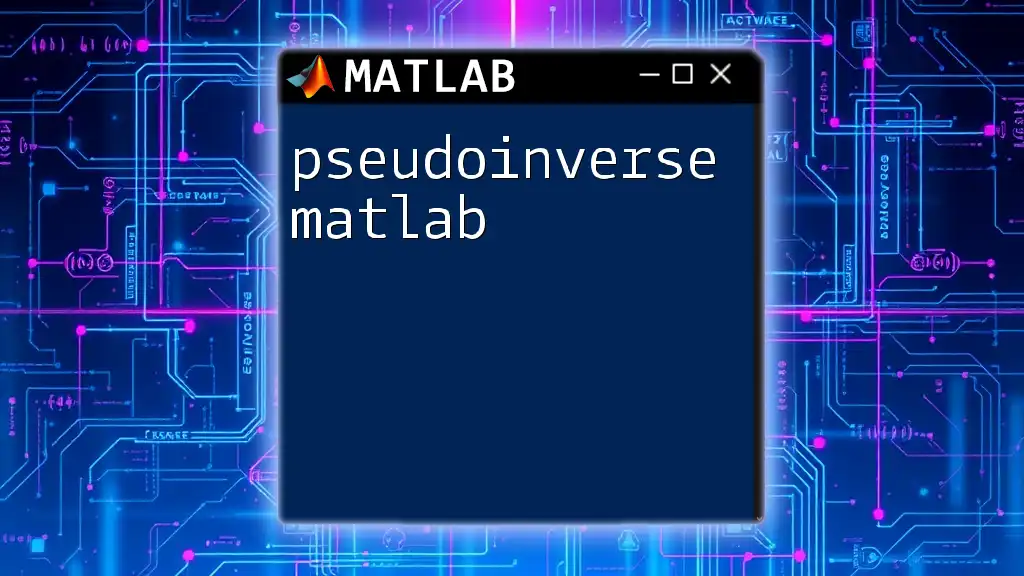
Applications of Piecewise Functions in MATLAB
Real-World Examples
Piecewise functions have varied applications across multiple fields:
-
Engineering: In materials science, piecewise functions often model stress-strain relationships where the material behaves linearly under small stress and changes properties when the stress exceeds a certain threshold.
-
Economics: Supply and demand curves frequently adopt piecewise functions to represent step changes in pricing structures.
-
Signal Processing: In this field, switching functions that define system behavior can be modeled with piecewise functions.
Example Code Snippet for an Application:
t = linspace(0, 10, 100);
signal = piecewise(t < 5, sin(t), t >= 5, sin(t) + 2);
plot(t, signal);
title('Piecewise Signal Example');
xlabel('Time (s)');
ylabel('Amplitude');
This snippet models a signal that behaves as a sine wave for the first five seconds but shifts upward by 2 units for later intervals. This exemplifies how piecewise functions can represent changes in systems over time.
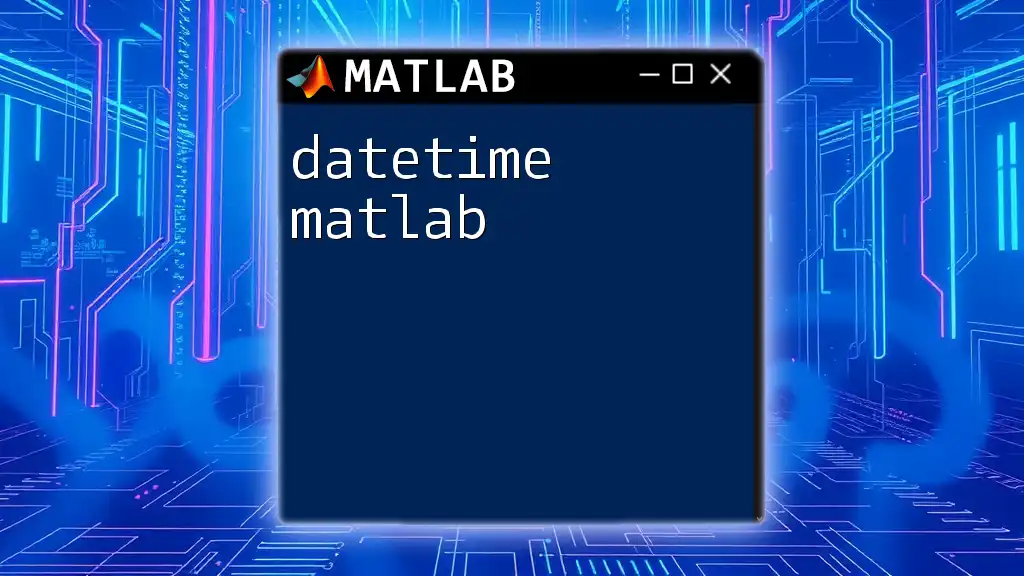
Common Mistakes to Avoid
Common Errors with Piecewise Functions
Despite their utility, piecewise functions can lead to errors if not implemented correctly:
- Forgetting to Specify Conditions: Always ensure that conditions define the behavior comprehensively.
- Handling Edge Cases Poorly: Pay attention to how values at boundary conditions are treated—this can greatly affect outcomes.
- Neglecting to Test All Intervals: Validating each segment of the function through testing is crucial for accuracy.
How to Troubleshoot
If you encounter issues when working with piecewise functions, consider employing debugging techniques. Use `disp()` and `fprintf()` statements to trace values and ensure conditions are being reached as expected.
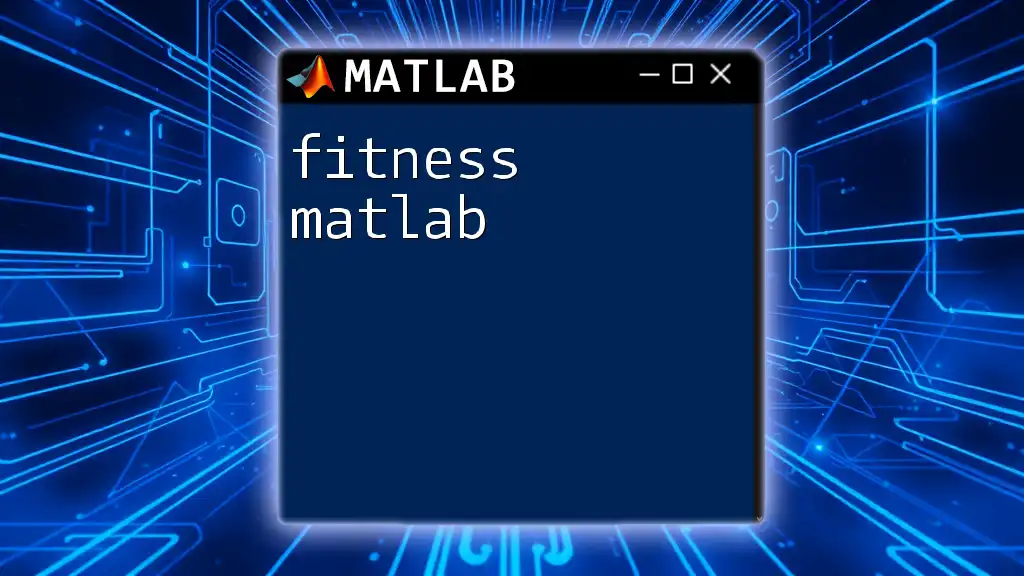
Conclusion
The ability to define and utilize piecewise functions in MATLAB opens up numerous possibilities for modeling complex systems and behaviors. By mastering the implementation techniques discussed, you can leverage the power of piecewise functions in your own projects. Don't hesitate to experiment with these instructions and continue exploring the fascinating world of piecewise functions!
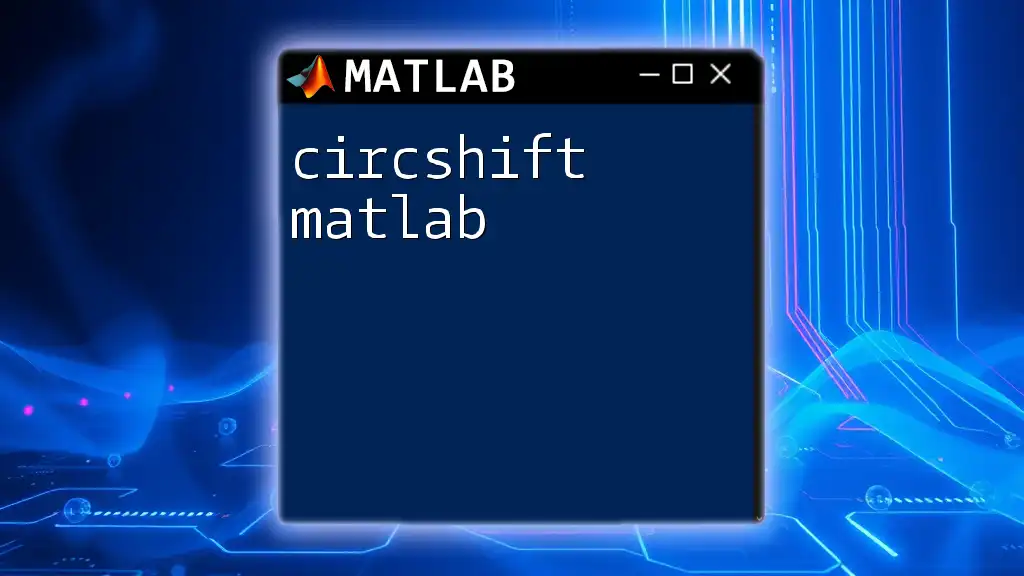
Additional Resources
For deeper insight into piecewise functions and MATLAB's capabilities, consider consulting:
- MATLAB documentation for the `piecewise` function and related functions.
- Online courses that focus specifically on MATLAB applications.
- Community forums like MATLAB Central for shared knowledge and troubleshooting advice.