In MATLAB, the term "infinity" is used to represent an infinitely large number, which can be utilized in calculations or comparisons, and you can denote it using the `Inf` keyword.
% Example of using Inf in MATLAB
result = 10 / Inf; % This will yield 0
Understanding Infinity in MATLAB
What is Infinity?
In mathematics, infinity refers to an unbounded quantity that is greater than any real number. In MATLAB, infinity is represented using the `inf` keyword, and it distinguishes between positive infinity (`+inf`) and negative infinity (`-inf`). Understanding how MATLAB treats infinity is fundamental for numerical computations as it can significantly affect the results of arithmetic and logical operations.
Use Cases for Infinity
Infinity plays a critical role in various scenarios:
- Handling Limits in Functions: In calculus, approaching infinity often helps in evaluating limits and understanding function behavior.
- Number Representation in Coding: MATLAB uses infinity to represent results that exceed the largest finite numbers to prevent overflow errors.
- Avoiding Overflow Errors: By using infinity, MATLAB gracefully handles calculations that would ordinarily result in errors due to exceedingly large numbers.
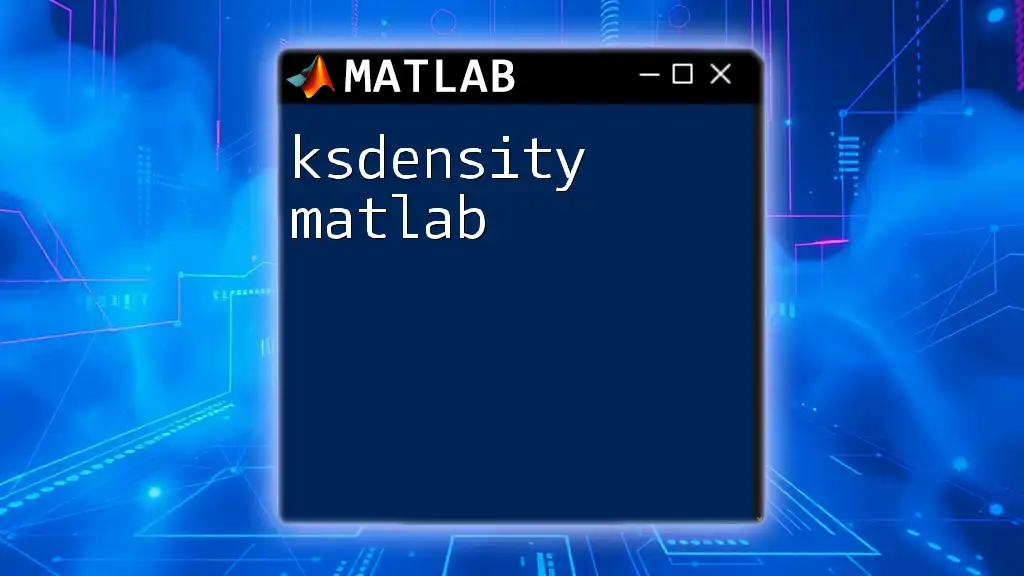
Working with Infinity in MATLAB
Creating Infinite Values
To create infinite values in MATLAB, simply use the `inf` keyword. This keyword signifies positive infinity, while the negative infinity can be represented as `-inf`. Here are simple commands for creating such values:
posInf = inf; % Positive Infinity
negInf = -inf; % Negative Infinity
Checking for Infinity
To check if a number is infinite, MATLAB provides the `isinf` function. This function returns `true` if the input is either positive or negative infinity. Here’s an example:
myValue = 1/0; % This creates positive infinity
isInfinite = isinf(myValue); % Returns true
Understanding this check is crucial, especially when operating within loops or conditional statements to manage infinite results effectively.
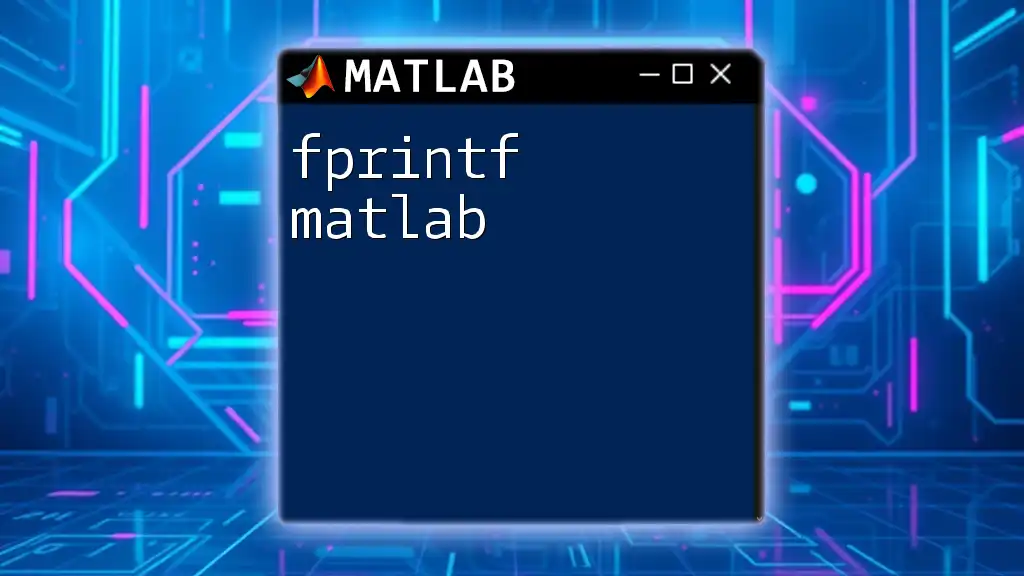
Mathematical Operations with Infinity
Arithmetic with Infinity
MATLAB follows specific mathematical rules when conducting arithmetic operations involving infinity. For instance:
-
Addition and infinity: Any finite number added to positive infinity results in positive infinity:
result1 = inf + 100; % Returns inf
-
Multiplication and infinity: Multiplying a finite number by positive infinity yields positive infinity:
result2 = inf * 2; % Returns inf
Conversely, negative infinity behaves similarly, but it can lead to different results, such as when adding a finite number:
result3 = -inf + 100; % Returns -inf
Infinity in Mathematical Functions
Infinity also interacts with several common mathematical functions in MATLAB. Here are a few examples:
- The exponential function:
expInf = exp(inf); % Yields inf
- Taking the logarithm of infinity:
logInf = log(inf); % Yields inf
- Trigonometric function behavior with infinity, for instance:
sinInf = sin(inf); % Yields NaN
This illustrates how different functions can either yield infinity or result in "Not a Number" (NaN), highlighting the importance of understanding the context in which you use infinity.
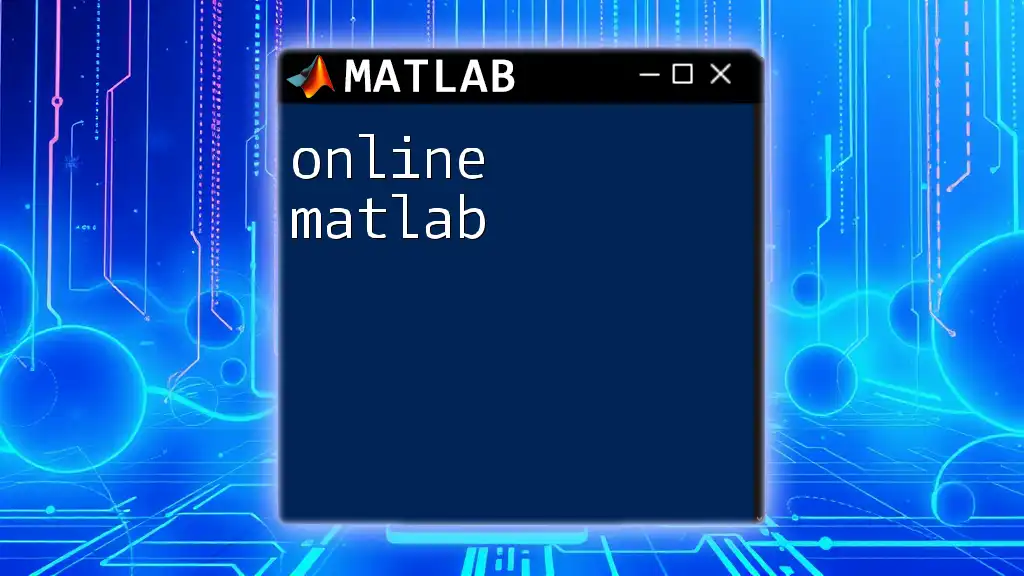
Visualizing Infinity in MATLAB
Plots that Involve Infinity
When visualizing functions that include infinite values, MATLAB can construct plots that effectively demonstrate these features. To create visualization for functions that approach infinity, consider the following example:
x = -10:0.1:10;
y = 1 ./ x; % This will create asymptotes
plot(x, y);
ylim([-10, 10]); % Setting limits to visualize behavior around infinity
Example: Plotting the Function Approaching Infinity
To visualize a function that illustrates limits approaching infinity, you can follow these steps:
f = @(x) 1./(x.^2);
fplot(f, [-10, 10]);
title('Behavior of f(x) = 1/x^2 as x approaches infinity');
xlabel('x');
ylabel('f(x)');
grid on;
This plot shows how the function behaves as it nears infinity, serving as a strong visual aid in understanding the concept of limits and infinity in mathematics.
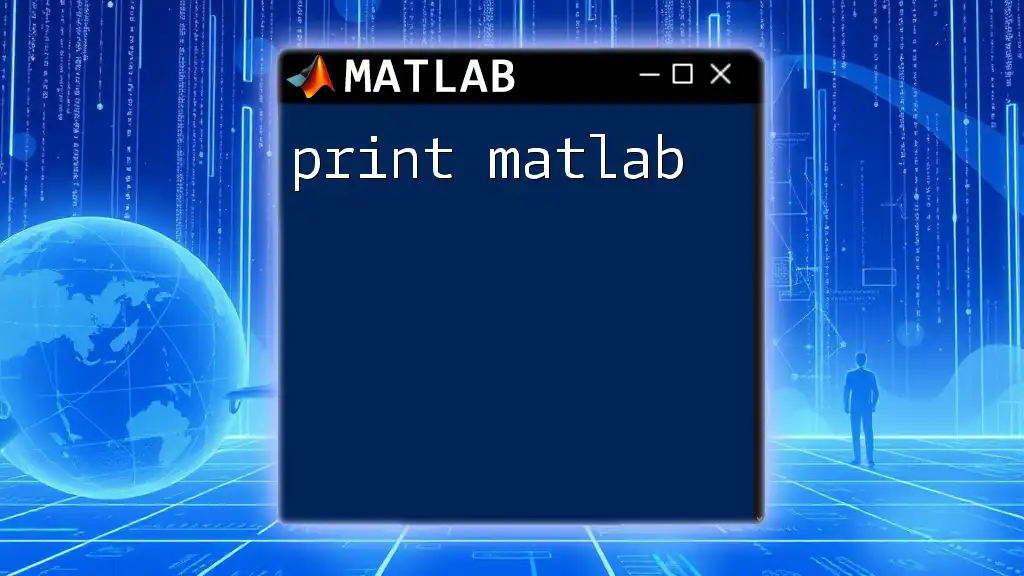
Common Errors Related to Infinity
Errors and Warnings
While working with infinity in MATLAB, certain operations can trigger errors or warnings. For instance, division by zero would typically result in positive or negative infinity, which sometimes isn't desired in computations.
Tips to Avoid Common Pitfalls
To avoid common pitfalls with infinity:
- Always check for infinite values before proceeding with operations.
- Use the `isinf` function liberally to safeguard against erroneous conditions.
- Normalize inputs or setting boundaries where necessary can help manage how infinity affects computations.
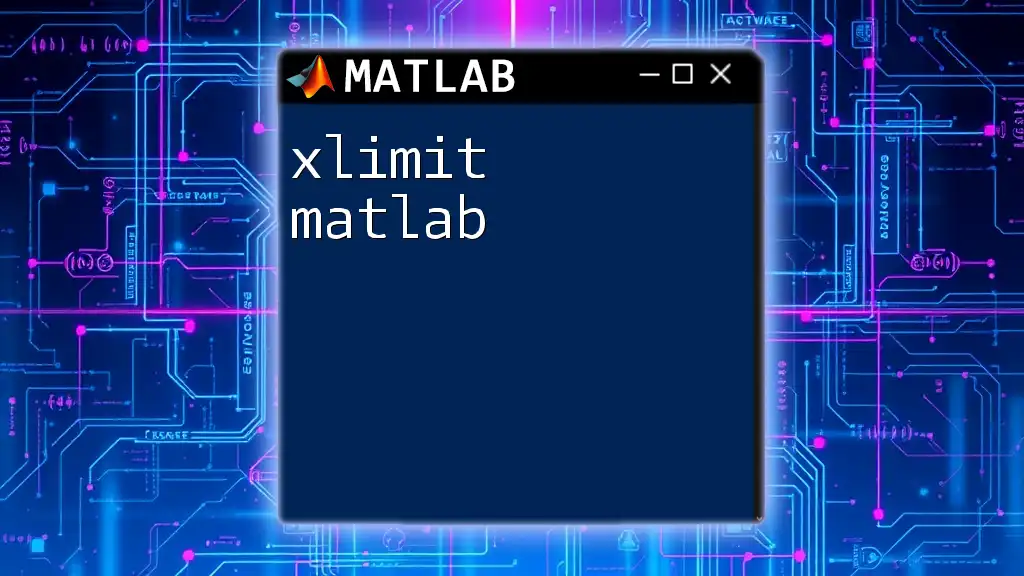
Conclusion
Understanding "infinity in MATLAB" is crucial for anyone looking to harness the full potential of mathematical functions and numerical computations. By grasping how MATLAB treats infinity, you can avoid common errors and conduct more reliable mathematical modeling. Encourage yourself to explore the inherent power of infinity through the examples provided, as well as experiment with your functions to gain a solid understanding of this profound concept.
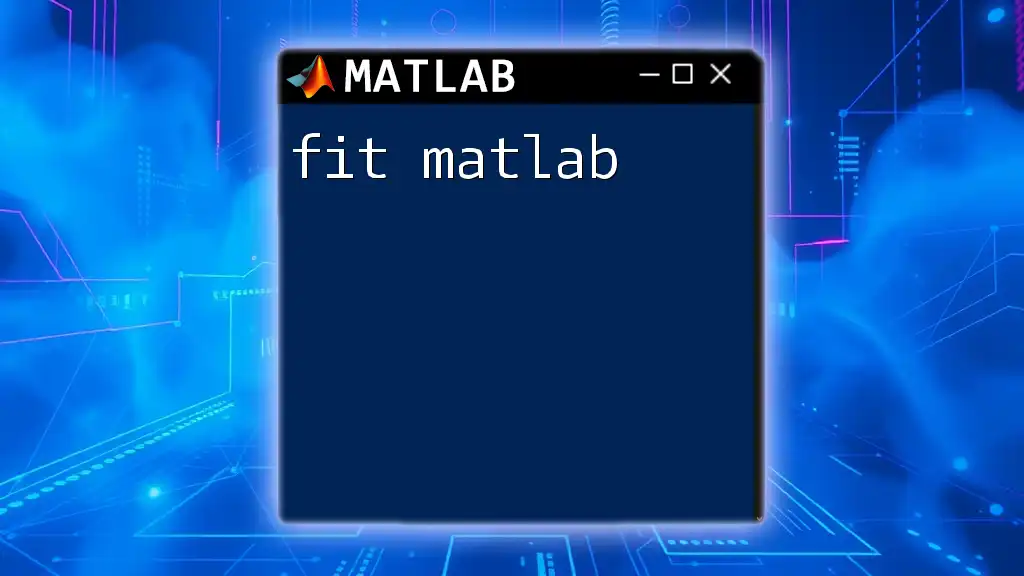
Additional Resources
For more detailed study, you may refer to MATLAB's official documentation on infinity and numerical representation, along with additional tutorials and reading materials to broaden your knowledge.
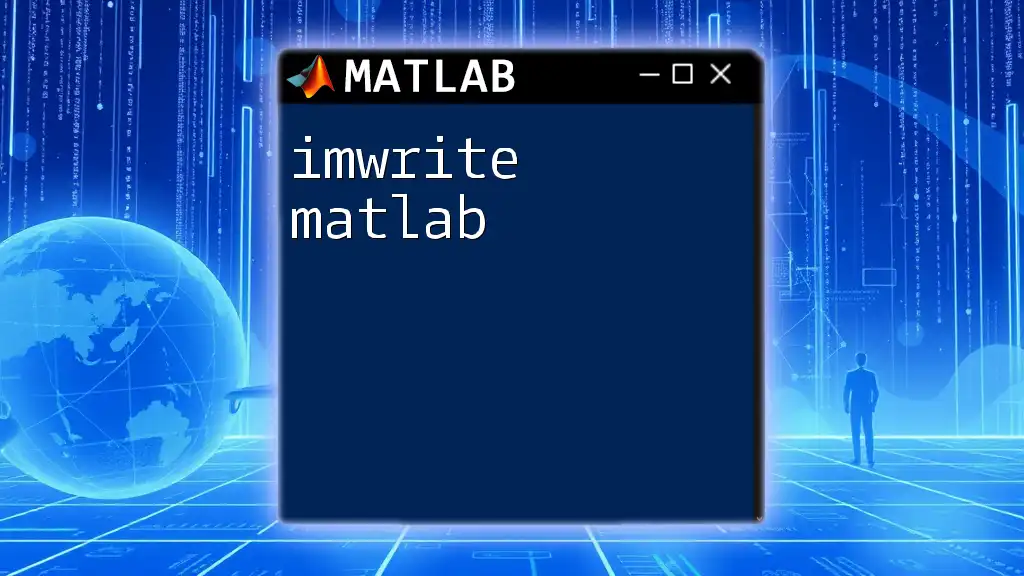
FAQs about Infinity in MATLAB
-
Can I use infinity in arrays? Yes, you can assign `inf` or `-inf` within arrays to represent unbounded limits.
-
How do I handle functions that return infinity? Use conditional checks with `isinf` and plan how to process or truncate results as needed to maintain desired outputs.