A periodogram in MATLAB is a method used to estimate the power spectral density of a signal, providing insights into the frequency content of the data.
% Example of calculating and plotting a periodogram in MATLAB
x = randn(1, 1024); % Generate a random signal
periodogram(x) % Estimate and plot the power spectral density
Understanding the Periodogram
What is a Periodogram?
A periodogram is a technique used to estimate the power spectral density (PSD) of a signal. It provides insight into the frequency content of a time-domain signal, making it valuable for any application involving signal processing. The periodogram serves as a graphical representation that helps visualize how the power of a signal is distributed across various frequency bands.
Mathematically, the periodogram is derived from the Discrete Fourier Transform (DFT) of a signal, giving insight into both periodic and non-periodic signals across different applications, such as telecommunications, biomedical engineering, and finance.
The Discrete Fourier Transform (DFT)
The Discrete Fourier Transform is a mathematical transformation used in signal processing to convert a sequence of values into components of different frequencies. It underlies the principles of frequency spectrum analysis. Understanding DFT is essential because the periodogram leverages it to produce frequency-domain information from time-domain signals.
Here’s a simple code snippet that demonstrates how to compute the DFT of a signal in MATLAB:
% Define a sample signal
Fs = 1000; % Sampling frequency
t = 0:1/Fs:1-1/Fs; % Time vector
x = cos(2*pi*100*t) + randn(size(t)); % Sample signal (cosine + noise)
% Compute the DFT
N = length(x); % Length of the signal
f = (0:N-1)*(Fs/N); % Frequency vector
X = fft(x); % Apply Fourier Transform
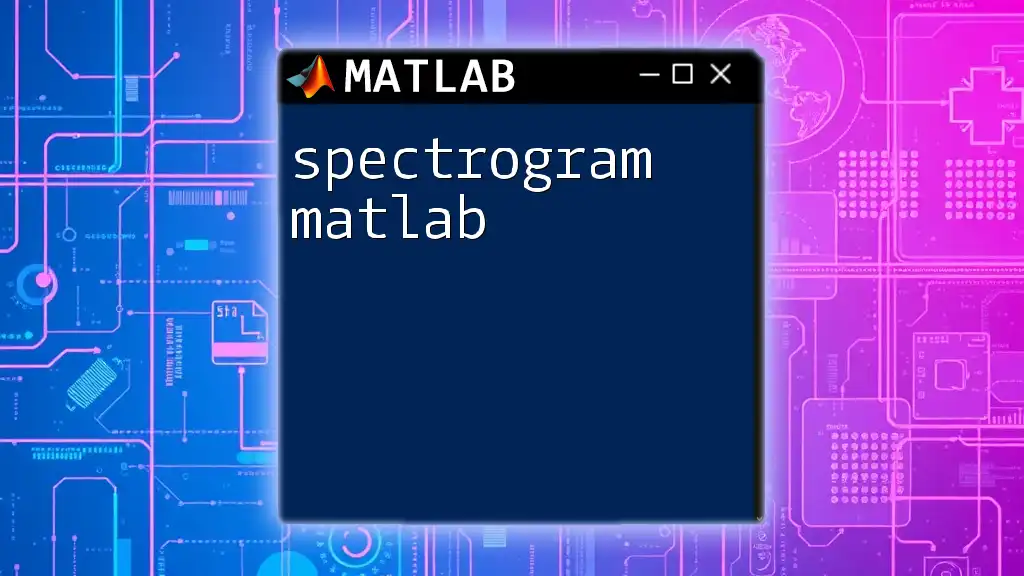
Periodogram in MATLAB
Built-in Functions
MATLAB provides several built-in functions for generating periodograms, with the most common being `periodogram` and `pwelch`. While the `periodogram` function gives an unbiased estimate of the spectral density, it can be noisy, particularly for short signals. In contrast, Welch's method, accessible through `pwelch`, divides the signal into overlapping segments, computes a periodogram for each segment, and then averages those results, resulting in a smoother estimate.
To compute a basic periodogram in MATLAB, consider the following example:
% Example of a simple periodogram
[Pxx, F] = periodogram(x, [], [], Fs); % Periodogram calculation
plot(F,10*log10(Pxx)); % Plotting the results in dB
title('Periodogram');
xlabel('Frequency (Hz)');
ylabel('Power/Frequency (dB/Hz)');
Understanding Output
The output of the `periodogram` function consists of two main components: Pxx and F. Here, Pxx is the estimated power spectral density (PSD) of the signal, while F represents the corresponding frequency values.
It's crucial to understand that the values in Pxx can vary widely; peaks in this output indicate dominant frequencies, whereas lower values point to noise or unimportant frequency components.
Interpreting Results
Interpreting the results from the periodogram involves identifying significant peaks in the plot. These peaks represent essential frequency components of your signal. For example, if you plot the periodogram of a sine wave mixed with noise, you should observe a peak at the sine wave's frequency. Consequently, proficient interpretation requires experience and familiarity with the signal being analyzed.
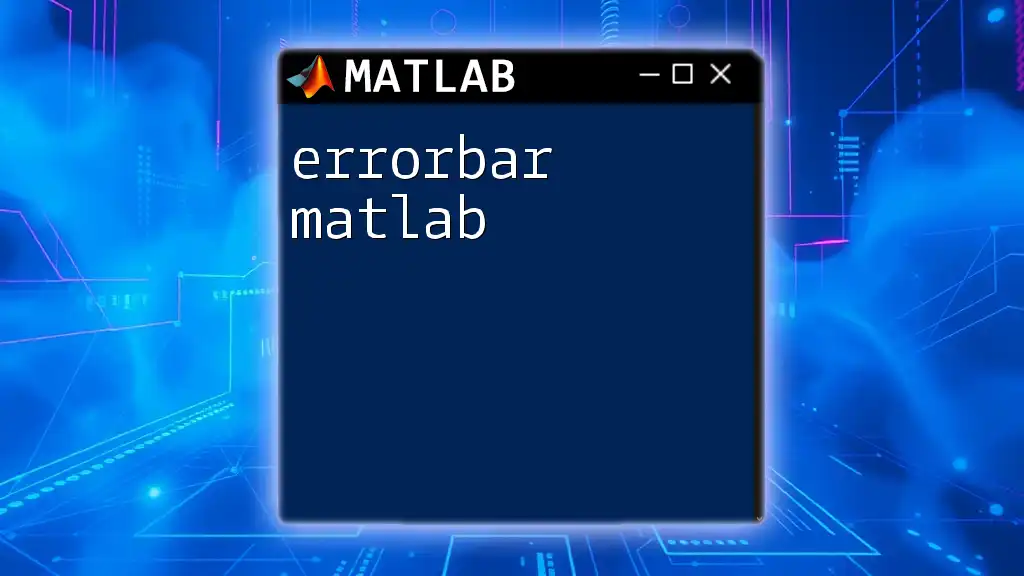
Using Windowing Functions
Importance of Windowing
Windowing is a technique applied to mitigate the effects of spectral leakage when estimating power spectral density. Without windowing, the abrupt edges of a finite-length signal can manifest as spurious frequency components in the estimation.
By employing windowing functions (such as Hamming, Hanning, or Blackman), one can smooth the signal edges, resulting in a more accurate periodogram. It is particularly important for analyzing real-world signals, which often contain noise and abrupt discontinuities.
Implementing Window Functions in MATLAB
Here’s how to apply a window function in MATLAB:
% Applying a Hamming window
window = hamming(length(x));
[Pxx_windowed, F] = periodogram(x .* window, [], [], Fs); % Periodogram with windowing
In this example, the signal x is multiplied by a Hamming window before computing the periodogram, resulting in less leakage and improved frequency estimation.
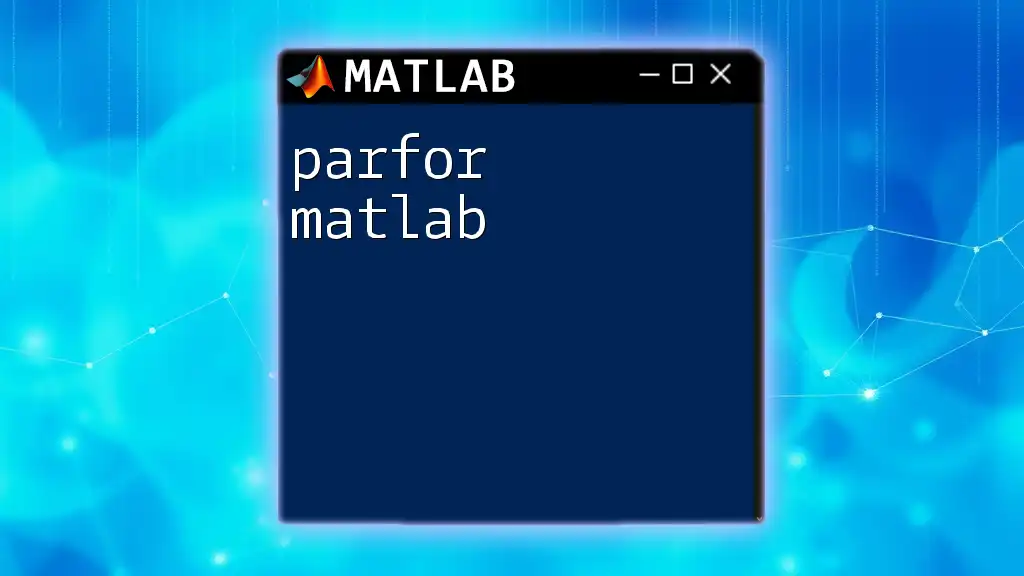
Advanced Periodogram Techniques
Welch’s Method
Welch's method is an excellent technique for reducing variance in periodogram estimates. By dividing the data into overlapping segments, it computes a periodogram for each and averages the results, leading to improved stability in the PSD estimate.
To implement Welch's method in MATLAB, you can use the following code snippet:
% Using Welch's method for power spectral density estimation
[Pxx_welch, F_welch] = pwelch(x, window, [], [], Fs);
plot(F_welch, 10*log10(Pxx_welch));
title('Welch Periodogram');
Multi-Taper Method
The multi-taper method is another advanced approach for spectral estimation, offering improved time-frequency resolution. It utilizes several orthogonal tapers to minimize bias in spectral estimates, making it especially useful for analyzing complex signals. This technique is more advanced and is best suited for users who need highly accurate time-frequency localization.
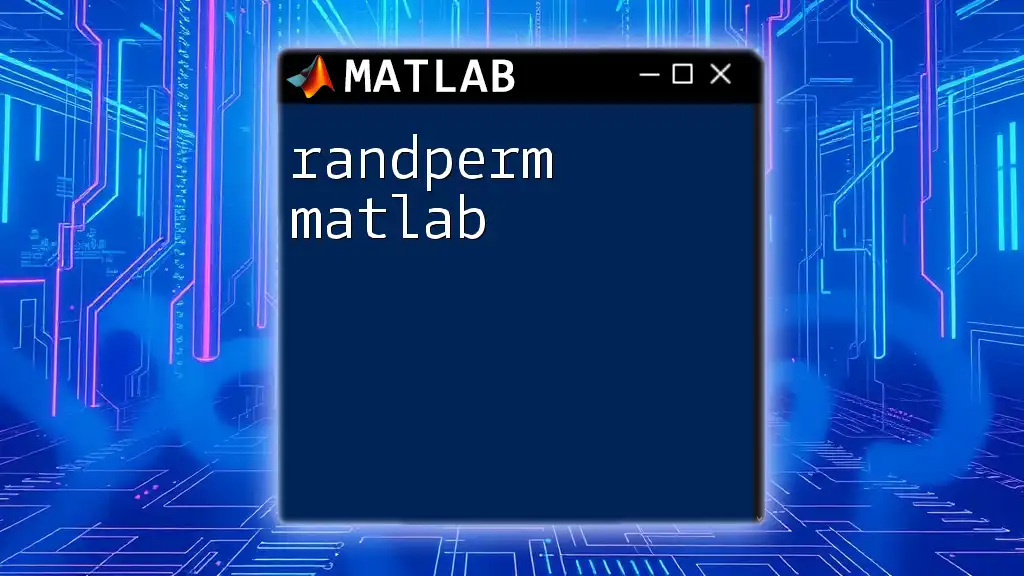
Case Studies and Practical Applications
Example 1: Analyzing Audio Signals
A practical example of using periodograms in MATLAB can be found in audio analysis. By applying a periodogram to audio samples, one can identify the dominant frequencies, which is vital for sound engineering and music analysis. For instance, after reading an audio file, you can apply the following code:
[x, Fs] = audioread('audioFile.wav'); % Read the audio file
[Pxx_audio, F_audio] = periodogram(x, [], [], Fs); % Compute periodogram
Example 2: Financial Time Series
In finance, periodograms can be utilized to analyze stock price fluctuations over time. By applying spectral analysis, traders can identify cyclical patterns or anomalies in financial data. An example of processing stock price data would look like this:
data = load('stockPriceData.mat'); % Load the financial data
[Pxx_finance, F_finance] = periodogram(data.price, [], [], data.samplingRate); % Compute periodogram
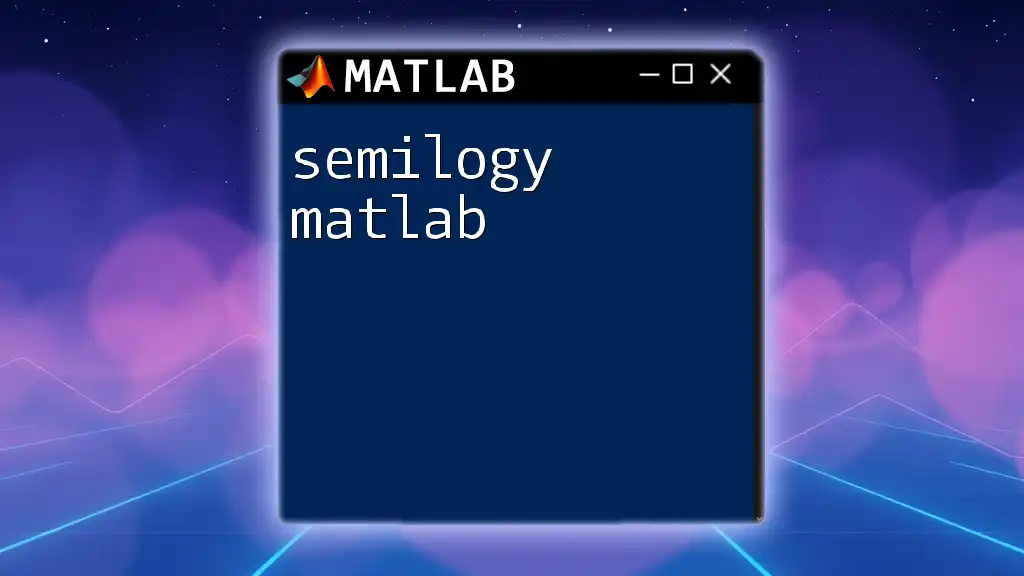
Troubleshooting Common Issues
Noisy Data
For signals affected by noise, using windowing and averaging techniques (like Welch's method) significantly improves the periodogram’s accuracy. Moreover, performing pre-processing steps, such as filtering, may also help reduce noise before periodogram analysis.
Insufficient Data Length
To ensure accurate periodogram estimation, it is recommended that your data length is sufficient. If the length is short, consider using window functions and Welch's method to provide better estimates. Increasing sample size, if possible, will yield more reliable results.
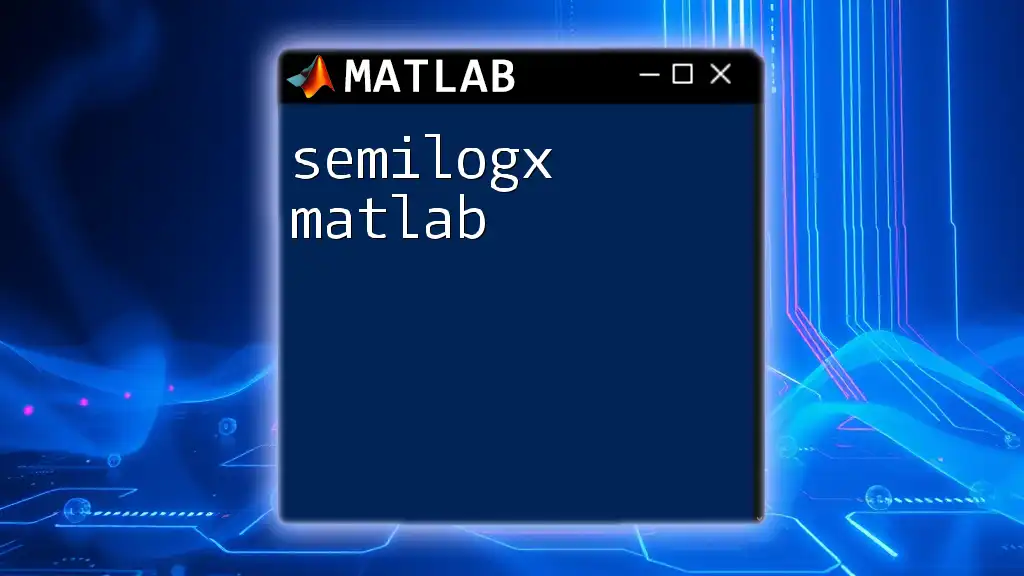
Conclusion
The periodogram is an invaluable tool in MATLAB for analyzing the frequency content of signals. By understanding how to implement this technique effectively—utilizing built-in functions, addressing issues with windowing, and applying advanced techniques like Welch’s method—you will enhance your capabilities in signal processing. With this knowledge, you can uncover deeper insights into the behavior of your signals, whether in audio processing, finance, or any field requiring frequency analysis. Embrace these techniques and explore further to master the art of periodogram analysis in MATLAB.