"Maximum MATLAB" focuses on teaching users how to effectively utilize MATLAB commands to achieve maximum efficiency and productivity in their coding endeavors. Here's a simple code snippet to find the maximum value in an array:
% Find the maximum value in an array
data = [3, 5, 2, 8, 6];
maxValue = max(data);
disp(['The maximum value is: ', num2str(maxValue)]);
Overview of MATLAB
What is MATLAB?
MATLAB is a high-performance programming language and interactive environment designed for numerical computing and visualization. It enables engineers and scientists to easily perform complex mathematical calculations, analyze data, and visualize results through an intuitive interface.
Why Use MATLAB?
Using MATLAB comes with several significant advantages:
- Ease of Use: The syntax is straightforward and designed for simplicity, making it accessible even to beginners.
- Extensive Libraries: MATLAB offers built-in functions and toolboxes for various applications, such as signal processing, image processing, and machine learning.
- Strong Community Support: The MATLAB community is vast, with numerous resources, forums, and user groups available to assist learners.
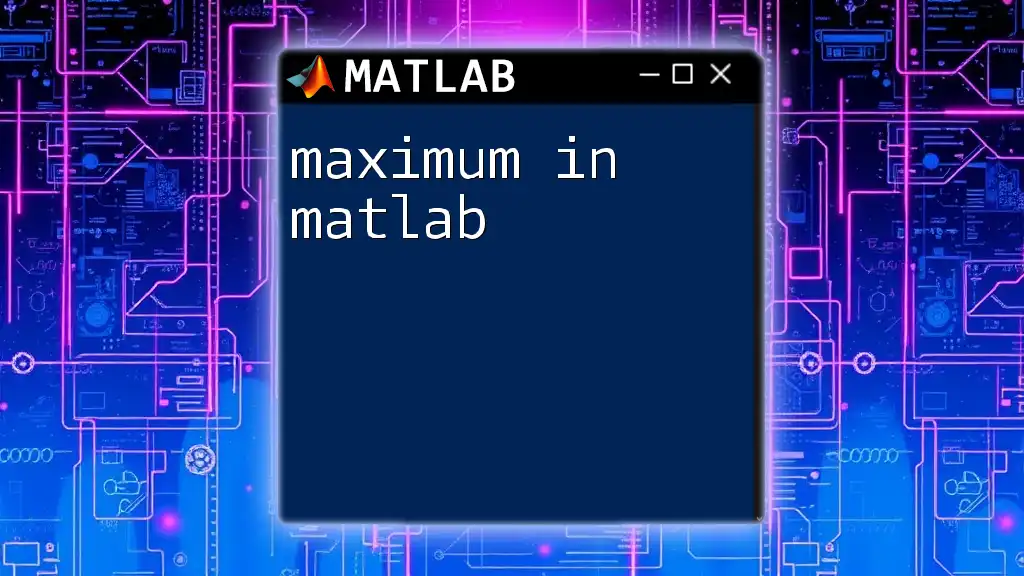
Getting Started with MATLAB
Installation and Setup
Installing MATLAB is simple. Ensure your system meets the required specifications, and visit the official MathWorks website to download the correct version for your operating system. Follow the on-screen instructions to install the software fully.
The MATLAB Environment
Upon launching MATLAB, you'll notice the environment features several key components:
- Command Window: Here, you can enter commands directly and receive instant feedback.
- Workspace: Displays all variables currently in use, allowing you to inspect and manage your data easily.
- Editor: A space for writing and debugging scripts, with useful tools for coding features such as syntax highlighting and error detection.
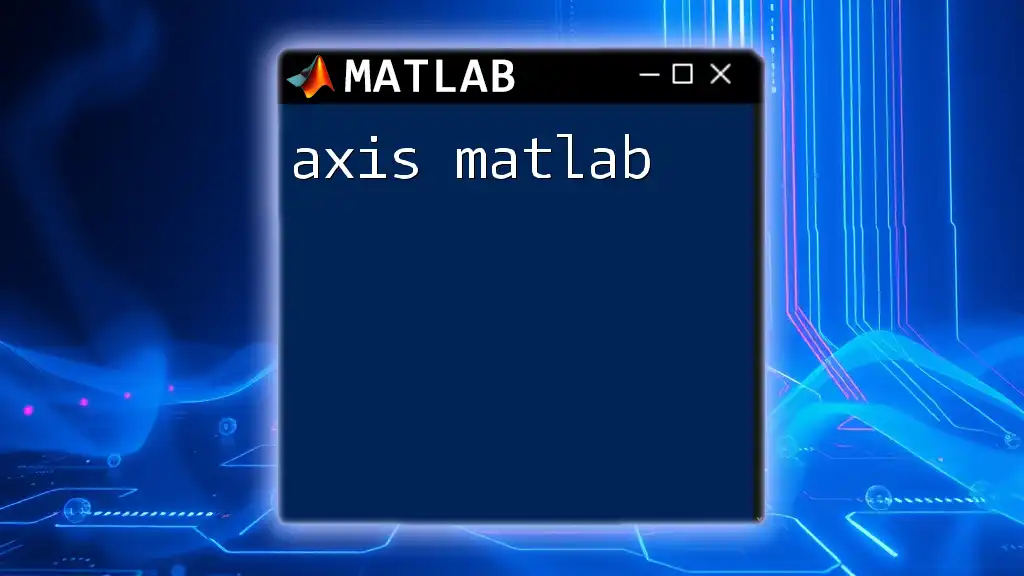
Essential MATLAB Commands
Variables and Data Types
Creating Variables
In MATLAB, you can create a variable by assigning a value to it using the equals sign. For instance:
a = 10;
This line assigns the value 10 to the variable a.
Common Data Types
MATLAB supports several data types, including:
- Numeric: For numbers, such as integers or decimals.
- Character: To represent strings of text.
- Logical: True or false values.
Here are examples of each:
x = 5; % Numeric
name = 'Sam'; % Character
status = true; % Logical
Basic Operations
Arithmetic Operations
MATLAB supports standard arithmetic operations. For example:
result = (5 + 3) * 2; % result equals 16
This line demonstrates addition and multiplication in a single equation.
Relational and Logical Operations
You can also perform comparisons using relational operators, such as:
is_greater = (a > 5); % returns true if a is greater than 5
Logical operations (AND, OR, NOT) can easily be performed as well, making MATLAB powerful for condition checks.
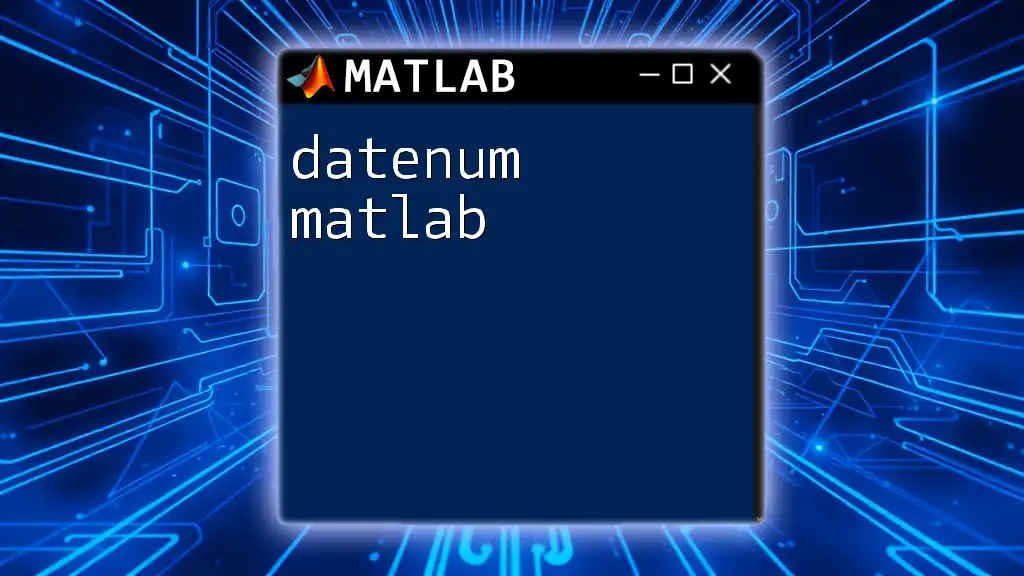
Advanced MATLAB Features
Functions
Creating Functions
You can define your own functions in MATLAB, which promotes code reusability and organization. For example:
function result = addNumbers(a, b)
result = a + b;
end
This function, `addNumbers`, takes two inputs and returns their sum.
Built-in Functions
MATLAB offers a variety of built-in functions that simplify common tasks. For example, compute the mean of a dataset with:
average = mean([1, 2, 3, 4, 5]); % returns 3
Plotting in MATLAB
Types of Plots
MATLAB excels in visualization capabilities, allowing you to create a wide range of plots. Common types include 2D plots, 3D plots, and histograms.
Example of 2D Plotting
To create a simple 2D plot, you can use the following code:
x = 0:0.01:2*pi; % Create a vector from 0 to 2π
y = sin(x); % Calculate the sine of each x value
plot(x, y); % Plot x vs y
title('Sine Wave'); % Add title
xlabel('x'); % Label x-axis
ylabel('sin(x)');% Label y-axis
This demonstrates how quickly you can visualize mathematical functions in MATLAB.
Working with Matrices
Matrix Creation and Manipulation
Matrices form the backbone of MATLAB. You can create and manipulate matrices straightforwardly. For example:
A = [1, 2, 3; 4, 5, 6; 7, 8, 9]; % Create a 3x3 matrix
element = A(2,3); % Access element at 2nd row, 3rd column
In this example, `A` is a matrix, and you can access its elements using indices.
Matrix Operations
MATLAB simplifies matrix operations like addition, multiplication, and transposition. For example, if you multiply two matrices, you can easily achieve it with:
B = [1, 2; 3, 4];
C = A * B; % Matrix multiplication
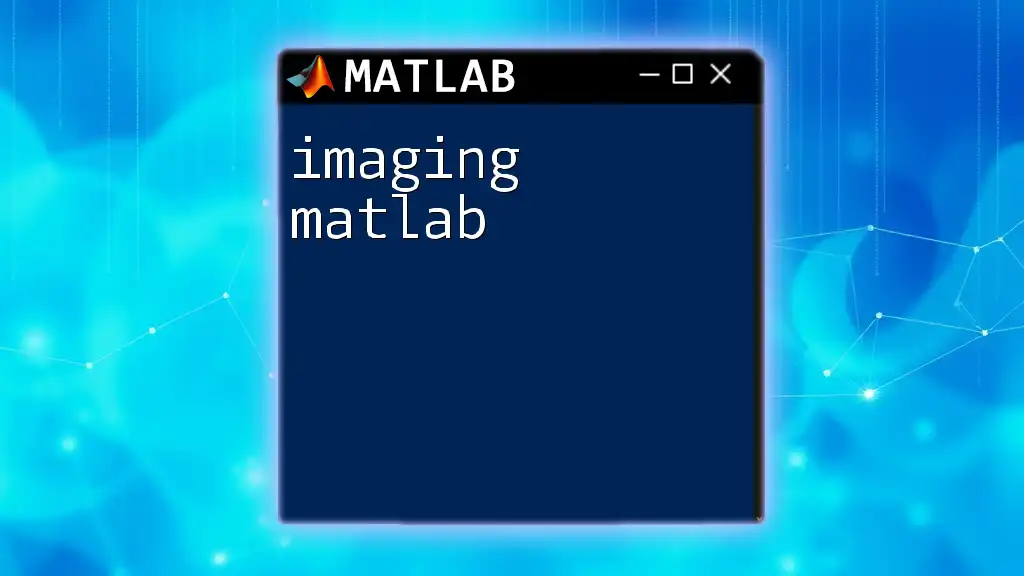
Error Handling and Debugging
Understanding Errors in MATLAB
Errors will inevitably occur while coding. Familiarizing yourself with common types of errors, such as syntax errors or index out of bounds, will save you time in troubleshooting. Always read the error messages—MATLAB provides helpful hints for addressing issues.
Debugging Techniques
To debug effectively, use breakpoints in the MATLAB editor. You can pause execution to analyze variable values and program flow. Utilize the interactive variables inspector in the workspace for a comprehensive view of your data.
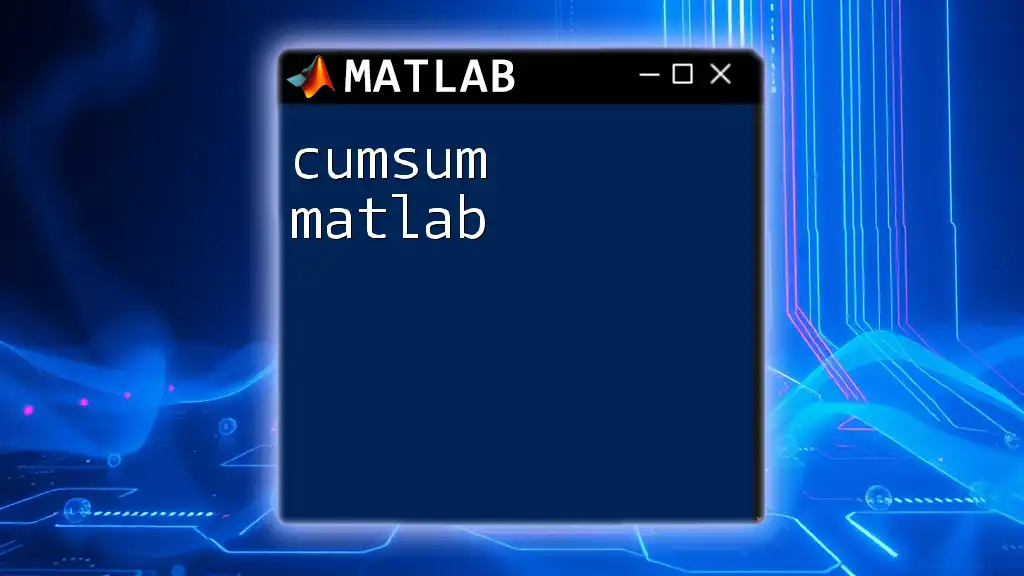
Best Practices for Writing MATLAB Code
Code Readability
Writing clear code is crucial. Use comments liberally to clarify complex sections, explaining your logic and making your code easier for others (and yourself later) to read and understand. For example:
% This function calculates the factorial of a number
function result = factorial(n)
if n == 0
result = 1; % Base case
else
result = n * factorial(n - 1); % Recursive call
end
end
Efficient Coding Techniques
Whenever possible, opt for vectorization instead of loops. MATLAB excels at handling arrays, and vectorized operations yield better performance. Consider this comparison:
Using a for-loop:
result = 0;
for i = 1:100
result = result + i;
end
Versus vectorization:
result = sum(1:100); % Significantly faster
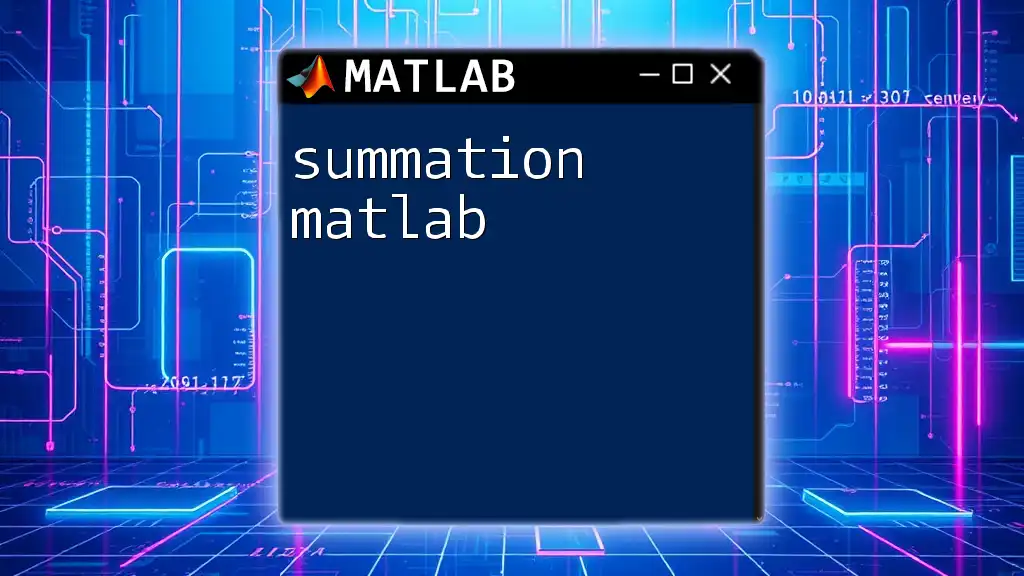
Resources for Learning More
Online Tutorials and MOOCs
To enhance your MATLAB skills, numerous online platforms offer tutorials and MOOCs. Websites like Coursera and edX provide structured courses that cater to all levels, from beginner to advanced.
Books and Documentation
The official MathWorks documentation is a treasure trove of detailed information. Additionally, consider reading books specifically focused on MATLAB applications in your field of interest, whether it's engineering, science, or finance.
MATLAB Community and Forums
Engaging with the MATLAB community can significantly boost your learning experience. Participate in MATLAB Central, stack overflow discussions related to MATLAB, and local user groups to ask questions and share insights.
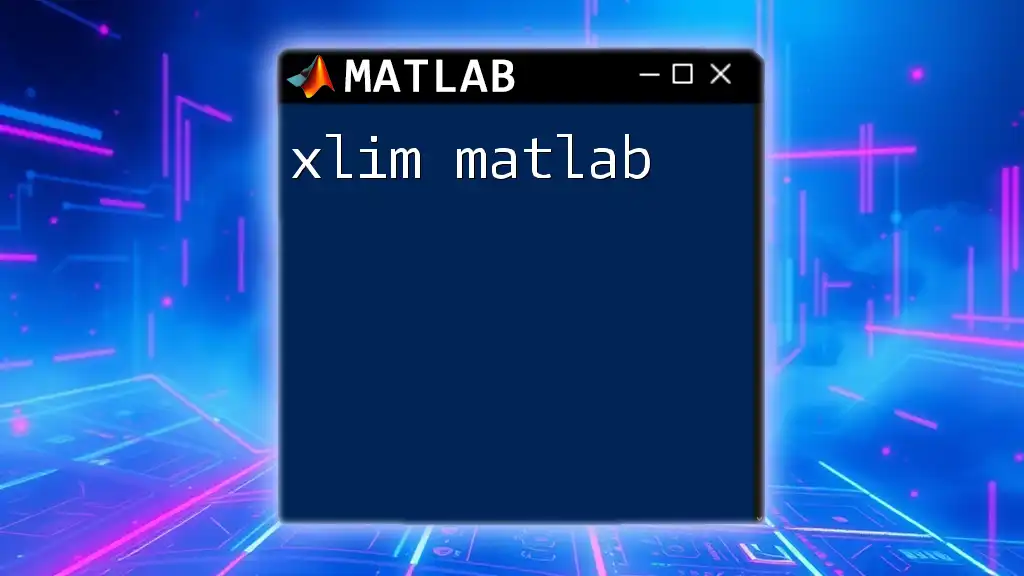
Conclusion
Summary of Key Points
In this guide, we explored the maximum MATLAB experience, covering everything from basic commands and functions to advanced matrix operations and plotting capabilities. We emphasized the importance of error handling, debugging techniques, and best coding practices to enhance your programming efficiency.
Encouragement for Further Learning
MATLAB's capabilities are vast and powerful. By investing time into mastering these tools, you open doors to endless opportunities in programming, data analysis, engineering calculations, and much more. Continue exploring and pushing the boundaries of what you can accomplish with MATLAB!