In MATLAB, you can customize the colors of your plots using predefined color names or RGB triplets, enhancing the visual appeal and clarity of your data representation.
Here's a simple code snippet demonstrating how to set the color of a plot:
x = 0:0.1:10; % Define x values
y = sin(x); % Compute sine of x
plot(x, y, 'Color', [0 0.5 1]); % Plot y with a custom RGB color
title('Sine Wave Plot');
xlabel('X-axis');
ylabel('Y-axis');
grid on;
Understanding Color Models
RGB Color Model
The RGB color model is a widely used system in digital displays, combining three primary colors: Red, Green, and Blue. MATLAB allows users to define colors using this model by specifying values between 0 and 1 for each color component.
To create specific colors, you simply define an RGB triplet. For example, to create a shade of purple, you might use an RGB triplet like `[0.5, 0, 0.5]`. Here's a simple code snippet demonstrating how to create a plot using an RGB color:
x = 1:10;
y = rand(1, 10);
plot(x, y, 'Color', [0.5, 0, 0.5]); % Plotting in purple
title('Line Plot in Purple');
Other Color Models
In addition to RGB, there are several other color models:
- CMYK (Cyan, Magenta, Yellow, Black): Primarily used in printing, this model emphasizes the mixing of inks.
- HSV (Hue, Saturation, Value): Useful for color selection, where hue represents color type, saturation describes color intensity, and value indicates brightness.
Understanding these models can assist in determining the right application for various colors in MATLAB graphics.
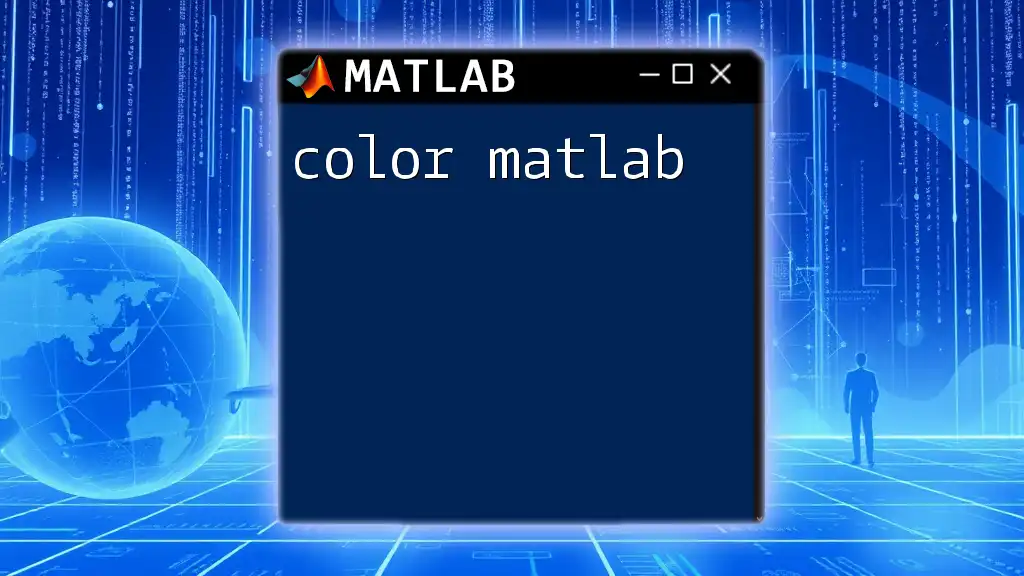
Using Built-in Color Names
Available Color Names in MATLAB
MATLAB has a variety of built-in color names that can simplify the process of color selection. Common names include:
- `'red'`
- `'green'`
- `'blue'`
- `'yellow'`
- `'cyan'`
- `'magenta'`
This feature allows you to create plots without explicitly defining RGB values. For instance, you can create a simple bar graph using built-in color names:
data = [5, 10, 15];
bar(data, 'FaceColor', 'green');
title('Bar Graph with Built-in Color Name');
Customizing Colors with Color Names
Combining multiple styles and colors is straightforward with built-in color names. You can customize elements in your visualizations by mixing colors and styles.
x = linspace(0, 2*pi, 10);
y1 = sin(x);
y2 = cos(x);
plot(x, y1, 'Color', 'blue', 'LineStyle', '--'); % Dashed blue line for sine
hold on;
plot(x, y2, 'Color', 'red', 'LineStyle', '-'); % Solid red line for cosine
title('Sine and Cosine Functions');
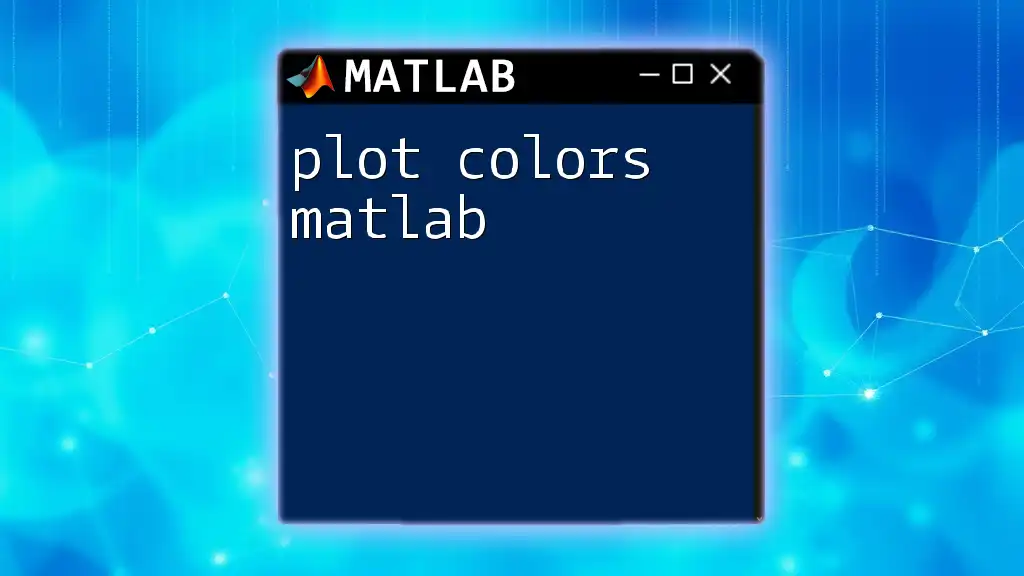
Creating Custom Colors
Specifying Colors Using RGB Triplet
Creating custom colors directly from RGB values gives you flexibility in your plots. You can craft unique colors that suit your data presentation needs.
Here's an example of creating a scatter plot using custom colors:
x = rand(1, 50);
y = rand(1, 50);
colors = [rand(1, 50); rand(1, 50); rand(1, 50)]'; % Random colors for points
scatter(x, y, 100, colors, 'filled'); % Scatter plot with random colors
title('Scatter Plot with Custom Colors');
Utilizing Hexadecimal Color Codes
Hexadecimal color codes are another way to define colors in MATLAB. The hex code is a six-digit representation, where the first two characters indicate red, the next two green, and the last two blue.
An example of using hex codes for color definition is as follows:
bar(data, 'FaceColor', '#FF5733'); % Using hex color for bar graph
title('Bar Graph with Hexadecimal Color');
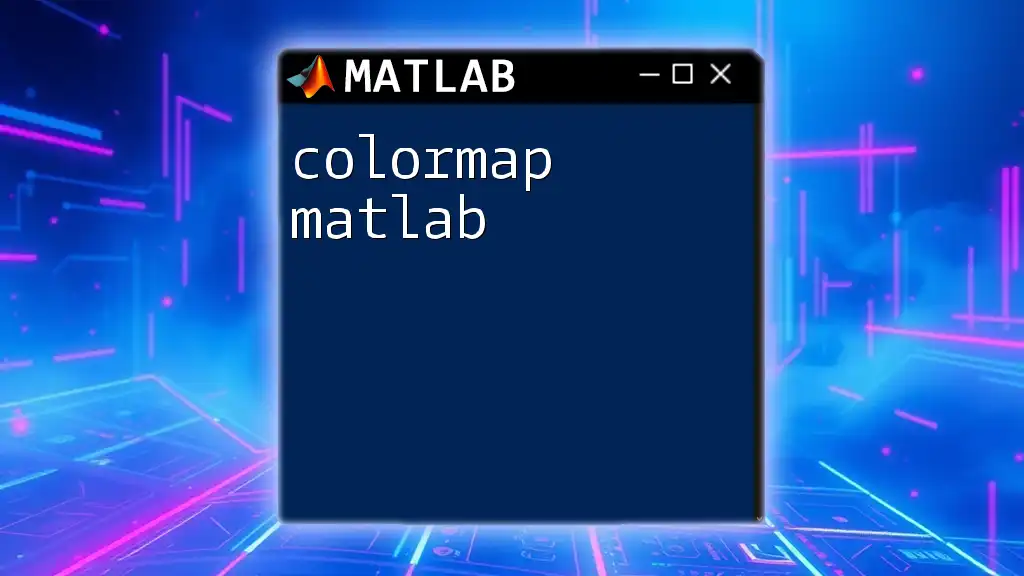
Colormaps in MATLAB
What is a Colormap?
A colormap is a matrix of colors used to map values in your data to colors in visualizations. MATLAB provides various built-in colormaps, essential for enhancing data visualizations, especially for images and surface plots.
Commonly Used Colormaps
Some well-known built-in colormaps in MATLAB include:
- 'parula': The default colormap; it provides a smooth transition of colors.
- 'jet': Known for high contrast, often used in scientific fields.
- 'hot': Features a gradient from black to red to yellow, representing heat.
To apply a colormap to a figure, use the `colormap` function. Here's a code snippet that applies the 'hot' colormap to a matrix:
data = peaks(30);
imagesc(data); % Display the data as an image
colormap('hot'); % Apply 'hot' colormap
colorbar; % Display color scale
title('Heatmap with Hot Colormap');
Creating Custom Colormaps
MATLAB allows you to create custom colormaps that suit your specific data requirements. A custom colormap can be defined as a matrix of RGB triplets.
The following example shows how to create and apply a custom colormap:
custom_cmap = [1 0 0; 0 1 0; 0 0 1]; % Red, Green, Blue
data = peaks(30);
imagesc(data);
colormap(custom_cmap); % Apply custom colormap
colorbar;
title('Heatmap with Custom Colormap');
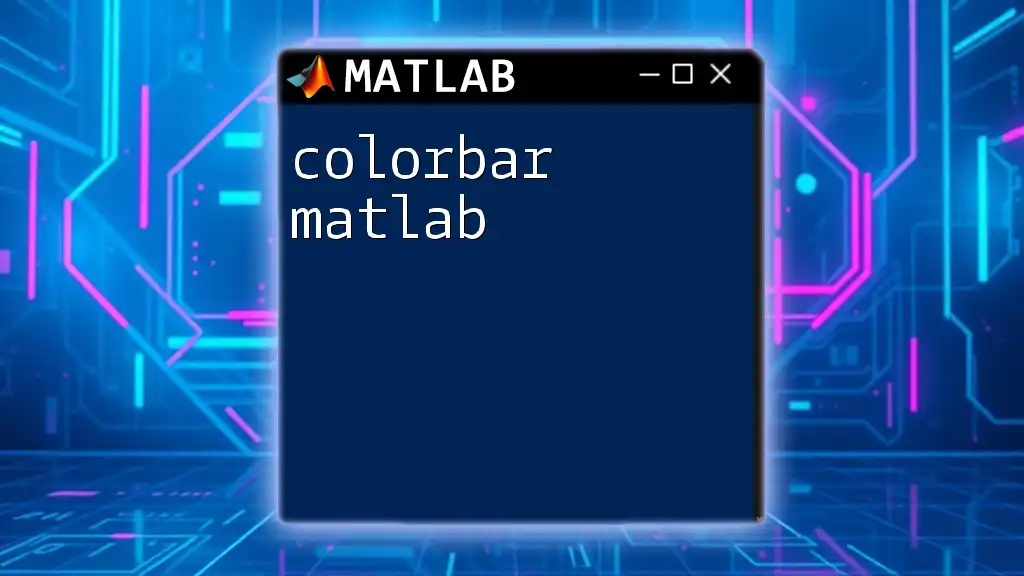
Color Properties in Graphical Objects
Setting Color Properties
MATLAB graphical objects have various properties that control their appearance, including color. Key properties include 'FaceColor' and 'EdgeColor', which define the interior and border colors of objects respectively.
Here's an example demonstrating how to explicitly set these properties for a patch object (a filled polygon):
x = [0 1 1 0];
y = [0 0 1 1];
patch(x, y, 'FaceColor', 'cyan', 'EdgeColor', 'blue'); % Patch with specified face and edge colors
title('Colored Patch with Edge and Face Colors');
Transparency and Color
In addition to color, transparency can be achieved using the alpha value, which ranges from 0 (fully transparent) to 1 (fully opaque). This can enhance visual clarity in overlapping plots.
hold on;
fill(x, y, 'red', 'FaceAlpha', 0.5); % Semi-transparent red patch
title('Patch with Transparency');
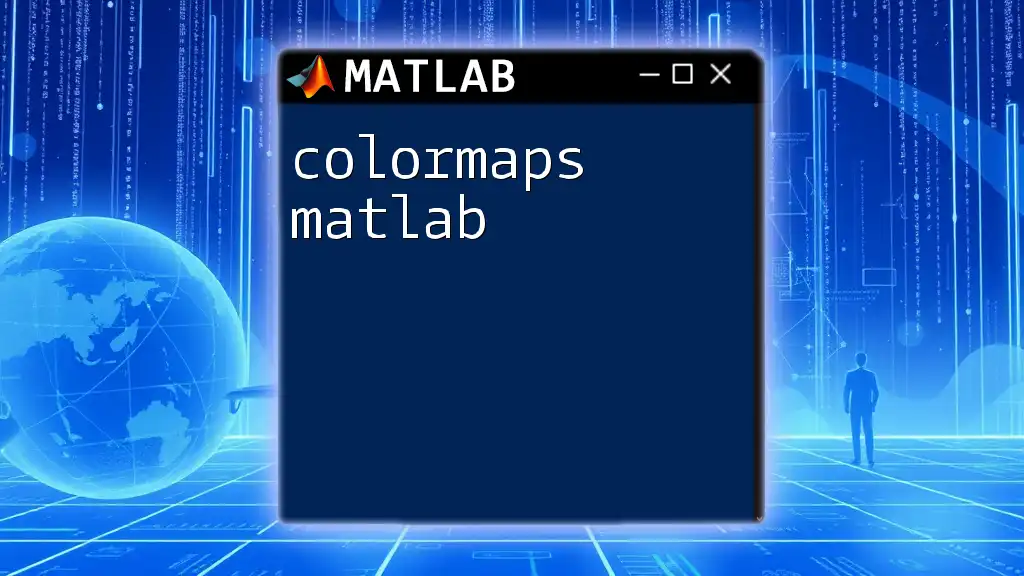
Best Practices for Color Usage
Accessibility Considerations
When choosing colors for visualizations, contrast and accessibility are critical. Make sure that colors are distinguishable for all viewers, including those with color vision deficiencies. Using more accessible color palettes can improve the interpretability of your plots.
Psychological Impact of Colors
Colors are not just aesthetic; they carry psychological implications. For instance, red can evoke urgency, while blue typically conveys calmness. Thoughtfully selecting colors based on the nature of your data can impact viewer perception and response.
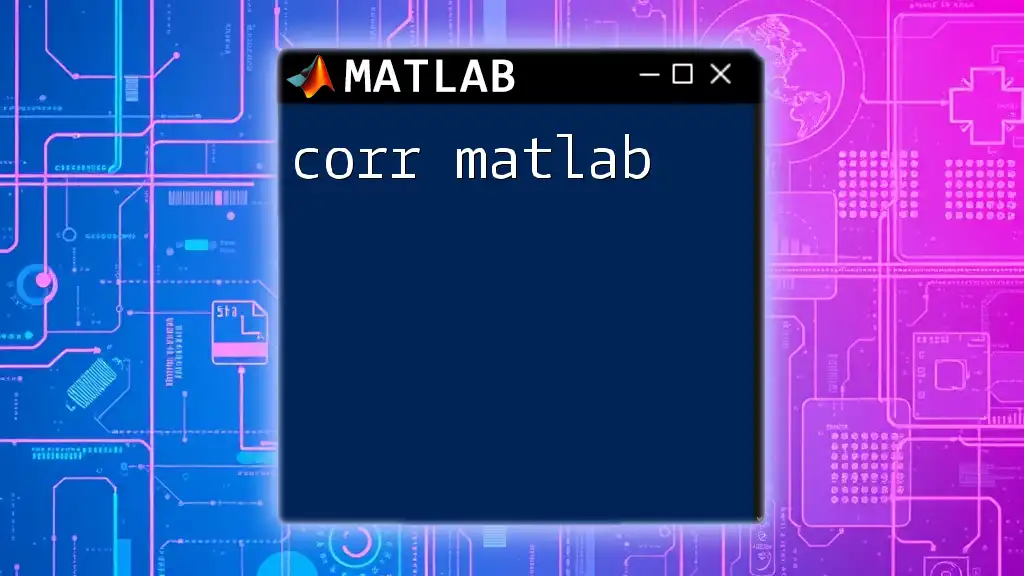
Conclusion
Incorporating color effectively is fundamental in creating engaging and informative visualizations in MATLAB. By understanding the various color models, leveraging built-in color names, and experimenting with custom colors and colormaps, you can enhance both the clarity and appeal of your data presentations. Take the time to explore and experiment with these features, as they can significantly improve how your data is communicated.
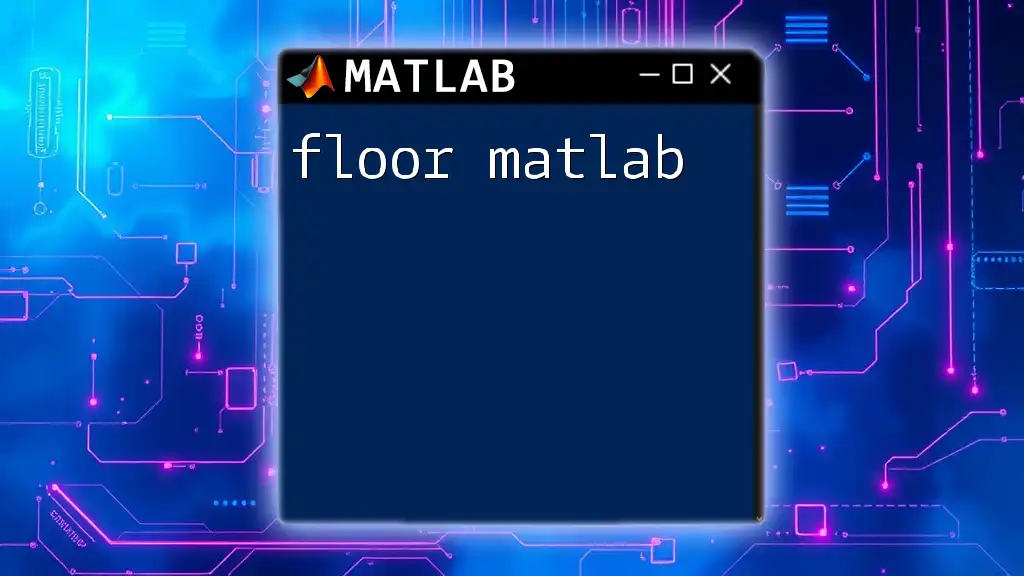
Additional Resources
For further exploration, consult the [MATLAB documentation on color handling](https://www.mathworks.com/help/matlab/ref/colormap.html) and consider various tutorials and courses that can provide deeper insights into the effective use of color in your applications.