The `datetime` function in MATLAB creates an array of date and time values, allowing for easy manipulation and formatting of temporal data.
Here's a simple code snippet to create a datetime object for the current date and time:
currentDateTime = datetime('now');
Understanding Datetime
What is Datetime?
The datetime object in MATLAB is a powerful tool designed to represent dates and times efficiently. Unlike traditional date formats that can often lead to confusion and inconsistency, the datetime class provides a unified structure that facilitates easier manipulation and analysis of temporal data.
One of its fundamental benefits is its ability to handle a wide range of formats and its flexibility in representing dates and times accurately, including support for varying time zones.
Key Features of the Datetime Class
Time Zone Support
Understanding time zones is crucial, especially when working with global data. The datetime class in MATLAB allows you to specify time zones directly. This feature is invaluable for accurate data representation and analysis across different regions.
Precision and Granularity
Datetime objects support high-resolution timestamps, accommodating sub-second precision. This feature is particularly important for data-intensive applications that require high accuracy, such as scientific simulations or real-time data logging.
Formatting and Display
MATLAB allows users to define how datetime objects are displayed through custom formatting options. This means you can present dates and times in the way that best suits your needs, producing clear and user-friendly output.
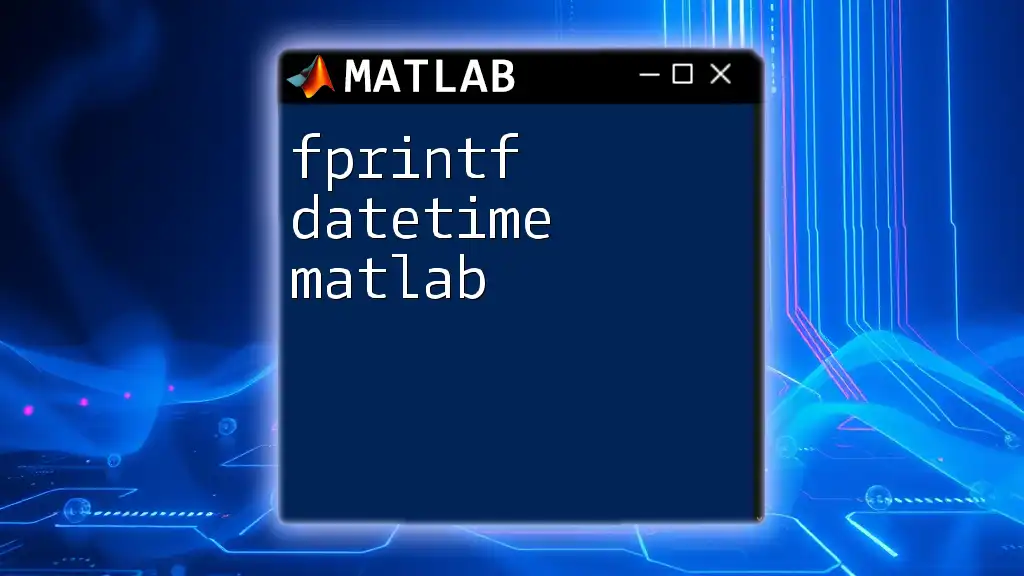
Creating Datetime Objects
Using the `datetime` Function
The `datetime` function is the cornerstone for creating datetime objects. It can be invoked using various parameters.
For example:
dt1 = datetime('now'); % This captures the current date and time.
dt2 = datetime(2023, 10, 1); % This creates an object for October 1, 2023.
By leveraging the `datetime` function, you can easily synthesize accurate temporal information tailored to your requirements.
Creating Datetime from Strings
Datetime objects can also be created from character vectors and string arrays using specified input formats. This is particularly useful for importing dates from external text files.
For instance:
dt3 = datetime('01-Oct-2023', 'InputFormat', 'dd-MMM-yyyy'); % String formatted date
The flexibility in input formats allows for smooth integration with various data sources.
Construction from Individual Components
You can build datetime objects from their individual components, such as year, month, day, hour, minute, and second. This method provides an intuitive way of specifying exact times.
For example:
dt4 = datetime(2023, 10, 1, 12, 30, 0); % Representing October 1, 2023, at 12:30 PM
This approach gives you granular control over creating datetime objects.
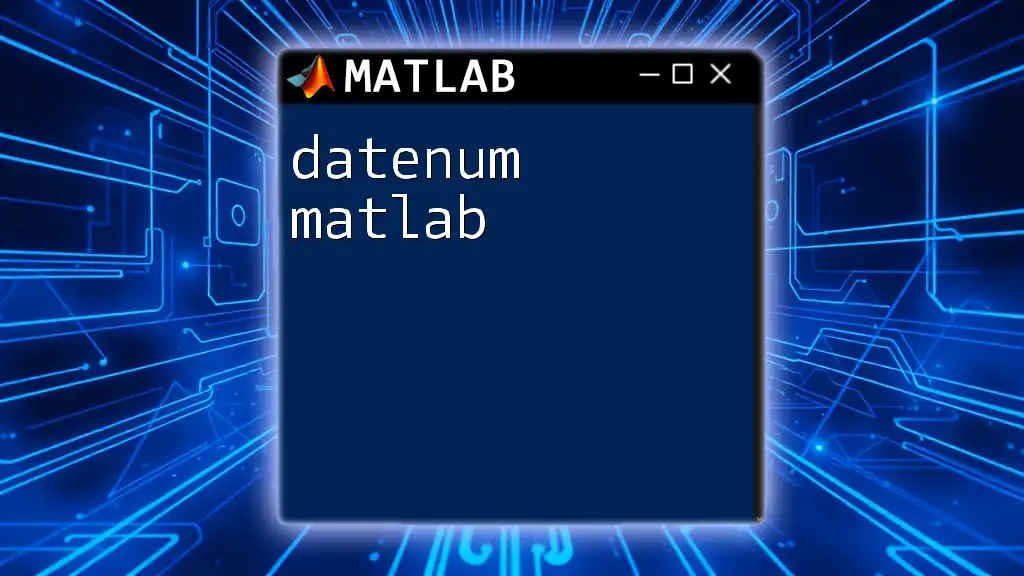
Manipulating Datetime Objects
Adding and Subtracting Time
Manipulating datetime objects is straightforward and powerful. You can easily add or subtract durations using the `years`, `months`, `days`, `hours`, `minutes`, and `seconds` functions.
For instance:
dt5 = dt1 + days(10); % Adding 10 days to the current datetime
This ability to manipulate dates and times seamlessly enhances the functionality of your data analysis.
Comparing Datetime Values
Datetime objects can be compared using standard relational operators. This allows for logical evaluations based on temporal data.
For example:
if dt3 > dt2
disp('dt3 is after dt2');
end
Such comparisons are essential when sorting datasets or conducting what-if analyses.
Finding Differences Between Dates
The `between` function enables users to calculate the duration between two datetime values easily. This functionality is particularly beneficial in applications requiring duration assessment.
Example of usage:
duration = between(dt2, dt3, 'Days'); % Calculates the number of days between two dates
This capability aids in analytic tasks, such as determining time spans and intervals.
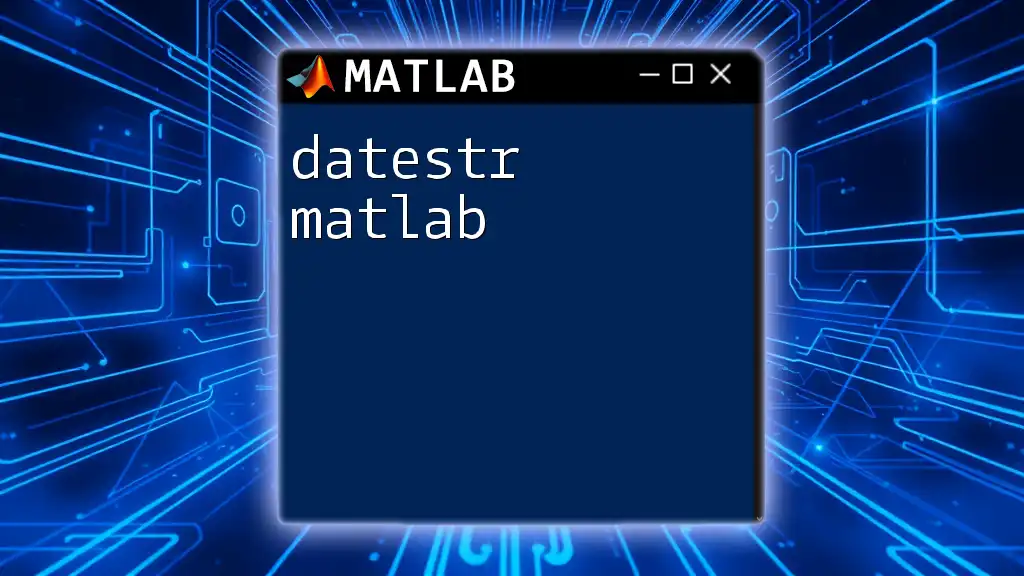
Formatting Datetime Output
Display Formats
MATLAB provides a variety of predefined formats for displaying datetime objects, including short and long date formats. You can easily change the display style based on your audience's needs.
For example:
dt6 = datetime('01/10/2023'); % Default format
dt6.Format = 'dd-MMM-yyyy'; % Custom format
Changing formats provides clarity when presenting dates in analysis reports or visualizations.
Custom Formatting
For scenarios requiring more specific display formats, MATLAB permits in-depth customization. You can define your own format string to tailor the presentation of dates.
For instance, creating a complex format might look like:
dt7 = datetime('2023-10-01 12:30:00');
dt7.Format = 'EEEE, dd MMMM yyyy HH:mm:ss'; % Produces a full textual representation
This feature is invaluable when you need to present datetime information in a user-friendly manner.
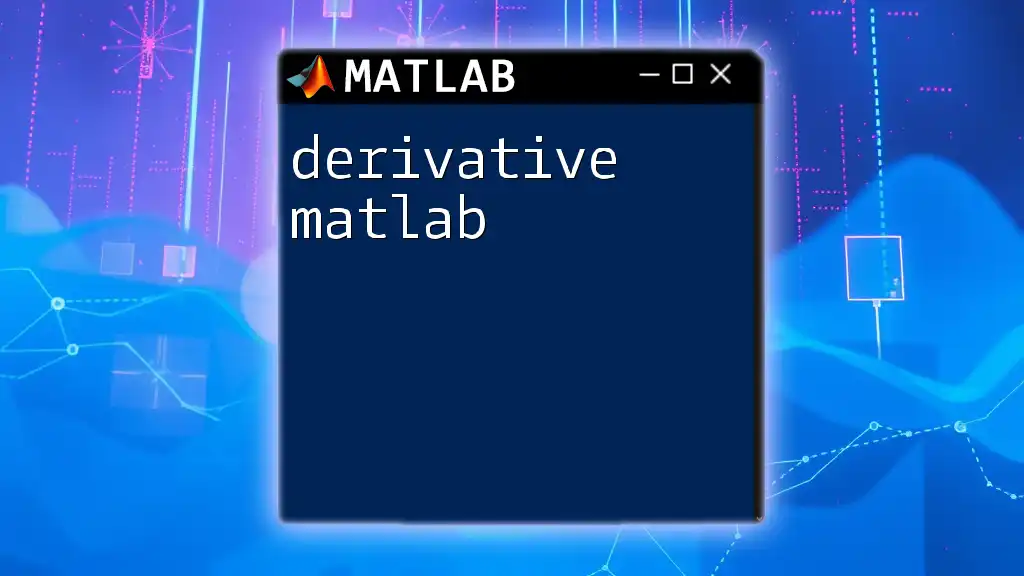
Converting Between Datetime and Other Types
Datetime to String Conversion
When outputting datetime objects, converting them to strings can enhance readability. This can be accomplished using the `datestr` function.
For example:
dateStr = datestr(dt7);
This conversion can streamline reporting and sharing data that involves datetime objects.
Converting Strings Back to Datetime
You can also revert strings back to datetime objects, allowing for seamless integration with string-based data sources.
For example:
dt8 = datetime(dateStr); % Converts string back to datetime object
This feature supports data flow between your MATLAB environment and external datasets.
Datetime to Numeric Values
Converting datetime to serial date numbers can be helpful for calculations requiring floating-point arithmetic.
For example:
serialNum = datenum(dt3); % Converts datetime to a serial date number
This numeric approach allows for additional mathematical operations where necessary.
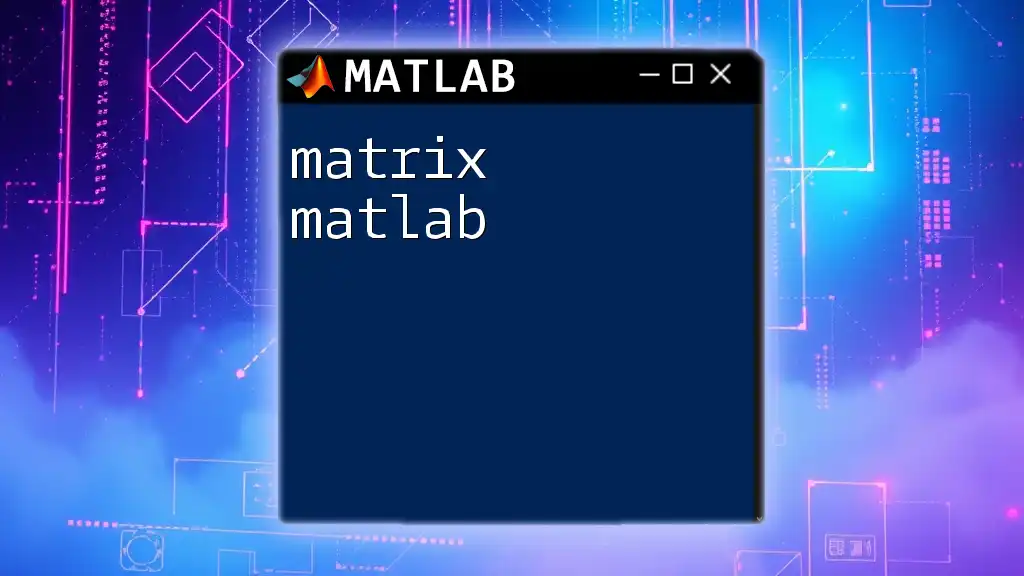
Practical Applications of Datetime in MATLAB
Data Analysis
The significance of datetime becomes evident in data analysis, especially for time-series data. MATLAB’s powerful plotting functions can visualize data over time efficiently.
Here’s a simple example of plotting:
time = datetime(2023, 10, 1) + days(0:29); % Generating a range of dates
data = rand(1, 30); % Example data
plot(time, data); % Plotting the data against the datetime
By leveraging datetime objects, analysts can glean insights from trends and patterns over time.
Scheduling Tasks
In addition to analysis, datetime objects can facilitate scheduling tasks and logging event occurrences. Assigning a timestamp for each action can provide a comprehensive log for audits or performance tracking.
For instance, logging when an event occurs can look like:
eventTime = datetime('now'); % Capture timestamp for an event
This capability enables better project management and accountability.
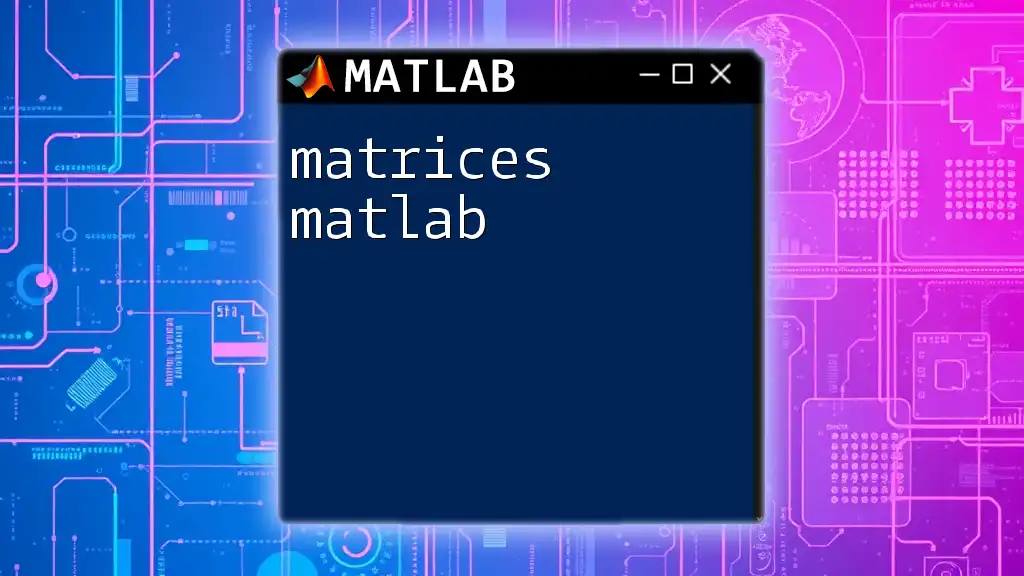
Conclusion
Understanding the datetime functionality in MATLAB empowers users to manage temporal data effectively. With its comprehensive set of functionalities, including creation, manipulation, formatting, and conversion, datetime transforms the handling of time-related data. As you explore working with datetime objects, you will begin to realize their immense potential in your data analysis tasks. Whether you are capturing the current time, analyzing time-series data, or scheduling tasks, the datetime class provides the support you need. Embrace the flexibility of datetime to elevate your MATLAB experience to new heights.
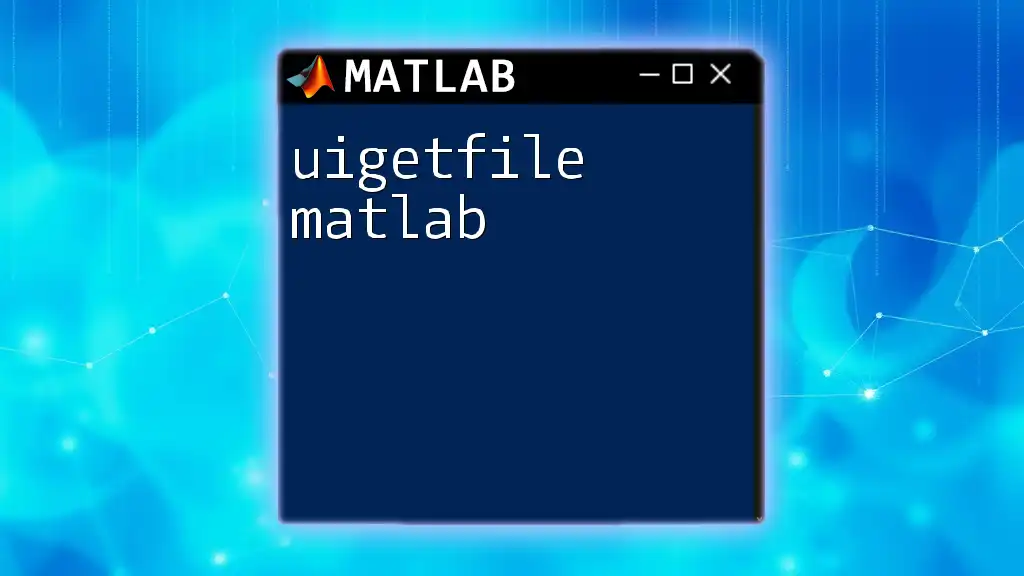
Additional Resources
For further exploration of datetime functionality, consider reviewing the [official MATLAB documentation](https://www.mathworks.com/help/matlab/ref/datetime.html). Continuous practice and exploration will deepen your understanding and enhance your skills in utilizing MATLAB for various applications efficiently. Contact us if you're interested in training or further resources to increase your proficiency in MATLAB, especially in areas involving datetime and beyond.