The `any` function in MATLAB checks whether any elements of an array are non-zero or true, returning a logical true or false accordingly.
% Example of using the 'any' function
A = [0 0 1 0];
result = any(A); % result will be true (1) since there is at least one non-zero element
Understanding Logical Conditions
What are Logical Operators?
In MATLAB, logical operators are essential for performing logical operations on arrays and matrices. The primary logical operators include:
- `&&` (logical AND)
- `||` (logical OR)
- `~` (logical NOT)
These operators allow you to evaluate conditions and make decisions based on the results. For instance, if you're analyzing data measurements, you might want to assess whether the conditions meet specific thresholds. Combining these operators lets you create complex logical expressions that guide your data analysis effectively.
Importance of Logical Operations
Logical operations are pivotal in data manipulation, particularly in fields like engineering, data science, and statistical analysis. They enable you to filter data, apply conditions, and perform actions based on the results. For example, you may need to identify certain entries in a dataset that exceed a defined threshold or check the integrity of your measurements.
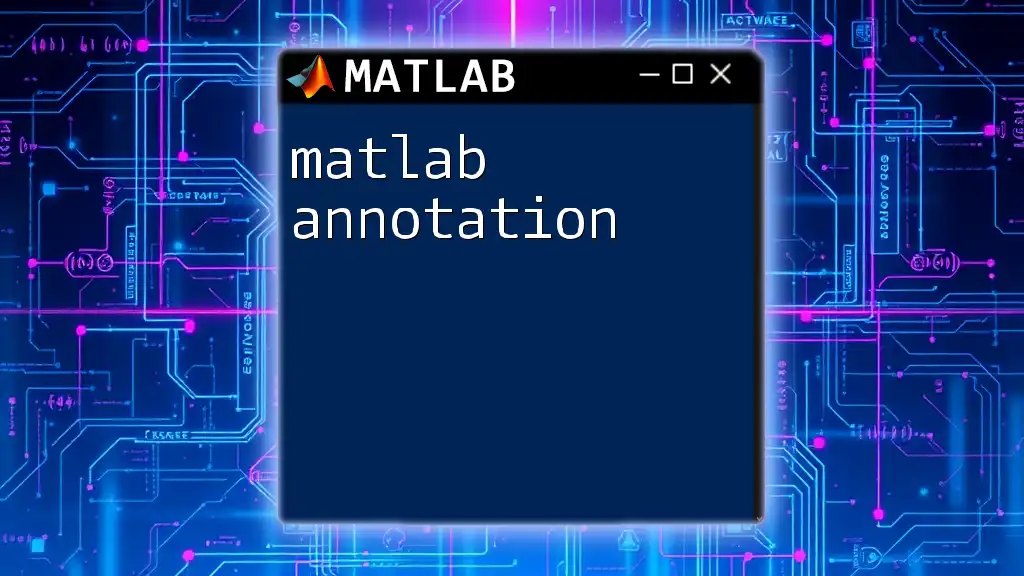
Introduction to the `any` Function
Definition of `any`
The `any` function in MATLAB is designed to assess whether at least one element of an array meets a specified logical condition. The basic syntax is:
result = any(X, dim)
Where:
- `X` refers to the input array (which can be a vector or matrix).
- `dim` specifies the dimension along which to operate (1 for columns, 2 for rows). If omitted, MATLAB defaults to operating along the first dimension (columns).
How `any` Works
When using `any`, the function evaluates the elements in the specified dimension and checks for truthiness (considering non-zero values in a numerical context as true). If any element meets the criteria, `any` returns `true` (1); otherwise, it returns `false` (0).
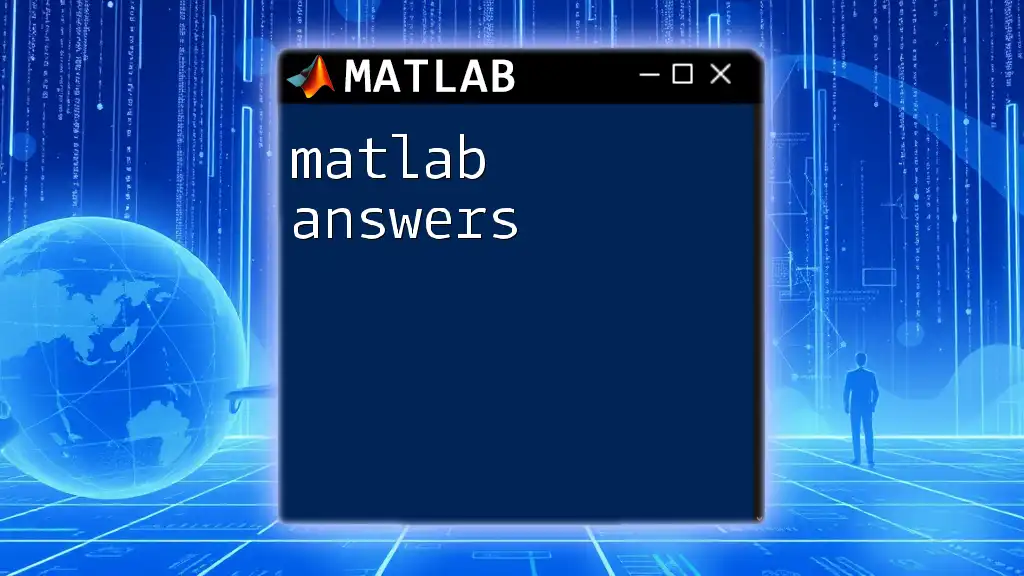
Using `any` with Vectors and Matrices
Checking for True Values in Vectors
Example 1: Simple Boolean Vector
v = [0, 0, 1, 0];
result = any(v);
In this example, `result` will be `true` because the vector `v` contains a `1`, which is interpreted as `true`. This demonstrates how `any` efficiently checks for the presence of true values in a Boolean vector.
Example 2: Logical Vector
v2 = [false, false, true, false];
result2 = any(v2);
In this case, `result2` will also be `true`, confirming that `any` successfully identifies that at least one element in `v2` is `true`. This functionality is crucial for applications needing logical assessments.
Handling Matrices with `any`
Example 3: 2D Matrix Check
M = [0 0 0; 0 1 0; 0 0 0];
result3 = any(M);
Here, `result3` will return `[0 1 0]`, showcasing that the second column contains at least one true value. By default, `any` processes along the columns, making it useful for data structured in tabular formats.
Example 4: Using `any` Across Specific Dimensions
result4 = any(M, 2);
With this command, `result4` returns a column vector `[0; 1; 0]`, which indicates that only the second row contains a true value. This flexibility allows users to check conditions across various dimensions based on their analytical needs.
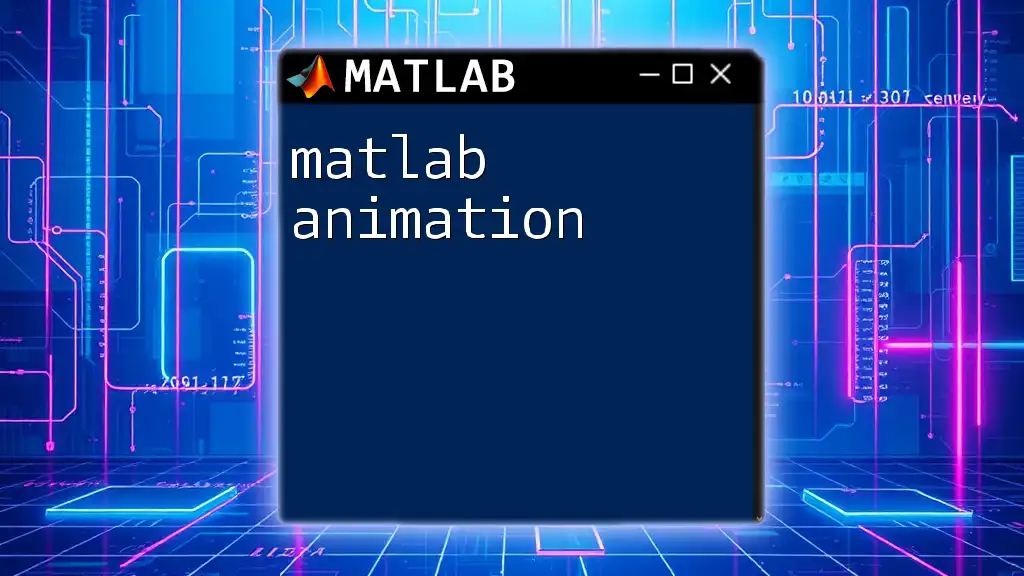
Combining `any` with Other Functions
Filtering Data Using `any`
Example 5: Conditional Filtering
data = rand(5); % Random 5x5 matrix
filtered_data = data(any(data > 0.5, 2), :);
In this example, `data > 0.5` creates a logical matrix that identifies which elements exceed `0.5`. Applying `any` along the rows filters the dataset, returning only those rows with at least one value greater than `0.5`. This practical use of `any` enhances data exploration and validation.
Using `any` in If Statements
Example 6: Conditional Execute
if any(v)
disp('At least one true value found.');
end
This snippet demonstrates how `any` can assess conditions within control flow structures. The `if` statement checks whether `any` element of `v` is true, then executes the command to display the message. This application is crucial for effective programming logic.
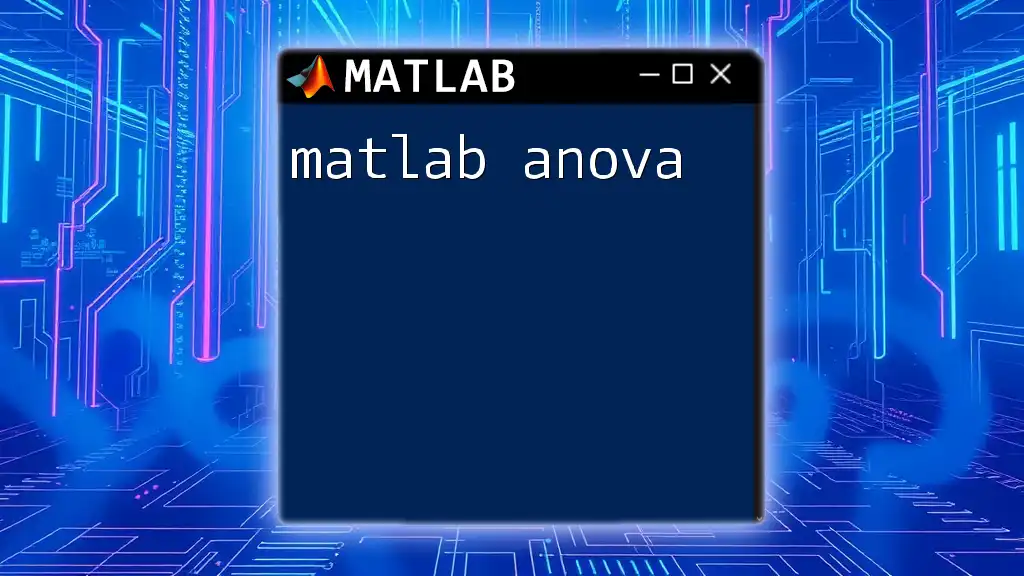
Common Pitfalls and Best Practices
Avoiding Misuse of `any`
While `any` is a powerful tool, it’s important to avoid common pitfalls, such as:
- Data Types: Ensure the input data is appropriate for logical evaluation. Non-logical data types (like strings) could lead to misleading results.
- Empty Arrays: Using `any` on empty arrays can yield unintuitive results. Always check your data before applying `any` to avoid unexpected outcomes.
Performance Considerations
When working with large datasets, remember that efficiency is crucial. The `any` function is generally optimized for performance, but consider using it judiciously. Whenever possible, minimize the size of the data being analyzed, especially in multidimensional arrays, to enhance execution speed.
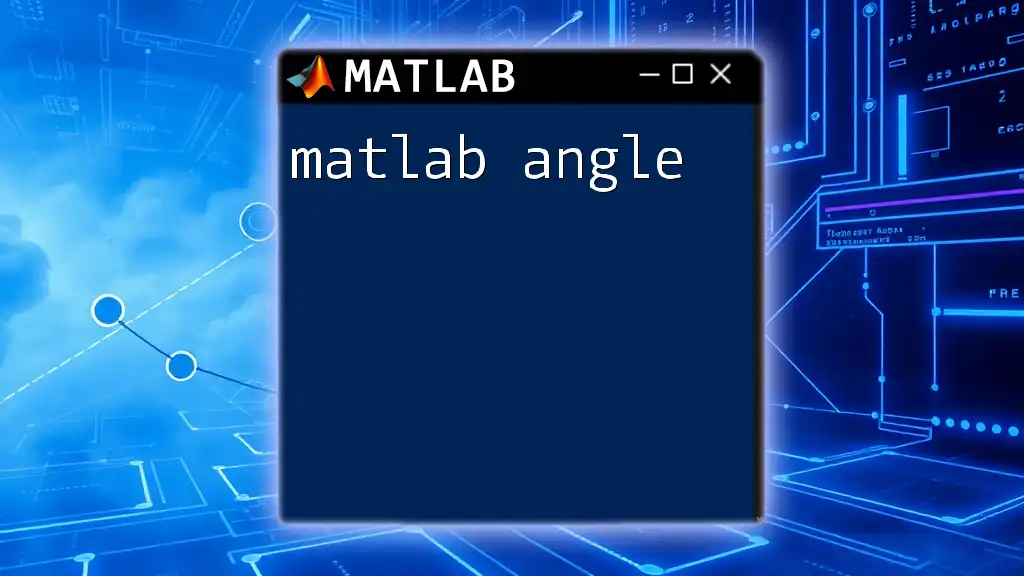
Conclusion
The `any` function in MATLAB is a versatile tool for assessing logical conditions across arrays and matrices. By integrating `any` effectively, you can streamline data analysis, filter datasets, and create robust programming solutions. When utilizing `any`, remember to consider data types and dimensions carefully to harness its full potential.
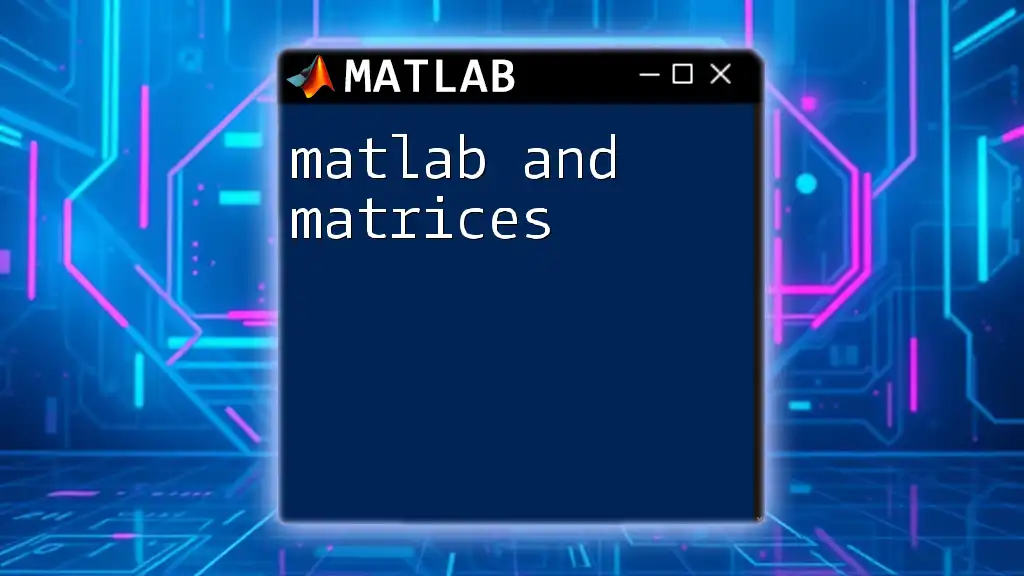
Additional Resources
For further exploration of the `any` function and logical operations in MATLAB, consider reviewing the official MATLAB documentation. Engaging with practice exercises will also deepen your understanding of this vital programming feature.