MATLAB and Simulink are complementary tools used for numerical computation, algorithm development, and model-based design, enabling users to create complex simulations and analyze dynamic systems efficiently.
Here’s a simple code example that illustrates how to plot a sine wave in MATLAB:
x = 0:0.01:2*pi; % Generate values from 0 to 2π
y = sin(x); % Calculate the sine of each value
plot(x, y); % Create the plot
title('Sine Wave'); % Add a title to the plot
xlabel('X'); % Label the x-axis
ylabel('sin(X)'); % Label the y-axis
grid on; % Enable grid
Understanding the Basics of MATLAB
MATLAB Environment
MATLAB offers a unique environment tailored for mathematical computing. The interface primarily comprises several key components:
- Command Window: This is the main area where you enter commands and interact with MATLAB directly.
- Workspace: Displays all the variables you have created during your session, allowing you to track your data easily.
- Editor and Script Files: Here, you can write, edit, and save scripts (sequences of MATLAB commands) to be run later.
Getting started often involves familiarizing yourself with essential commands. You can utilize `help` to access documentation on any function, while `doc` provides a detailed overview. For example, entering a basic arithmetic operation like `5 + 3` in the Command Window will immediately yield `8`.
The handling of matrices and arrays is fundamental in MATLAB, which excels in this area. Here's how to define a matrix:
A = [1, 2; 3, 4];
This command creates a 2x2 matrix, showcasing MATLAB's intuitive syntax.
Basic MATLAB Syntax
Understanding variables and data types is critical. MATLAB supports several types, including numeric, character, and structure data types.
Control Structures are fundamental for programming logic. You can implement conditional statements using `if-else` syntax, like so:
x = 10;
if x > 5
disp('x is greater than 5');
else
disp('x is 5 or less');
end
For iteration, `for` and `while` loops are incredibly useful. Here’s an example of a `for` loop that iterates through an array:
for i = 1:5
disp(['Iteration: ', num2str(i)]);
end
You can also define functions to encapsulate logic. Below is a simple MATLAB function to compute the factorial of a number:
function f = factorial(n)
if n == 0
f = 1;
else
f = n * factorial(n - 1);
end
end
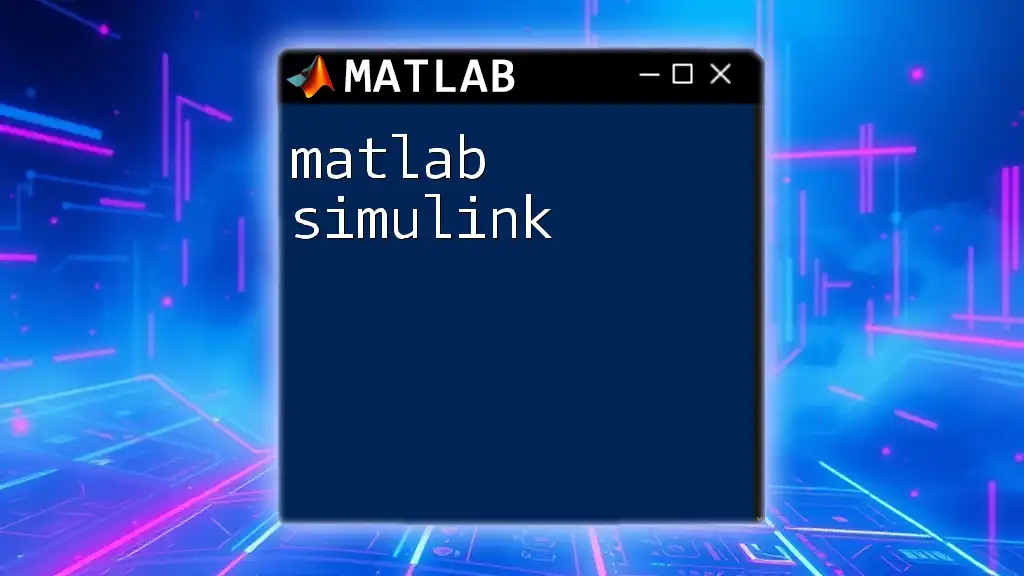
Diving Deeper: Advanced MATLAB Features
Plotting with MATLAB
Visualizing data is one of MATLAB’s powerful features. You can create 2D plots using the command `plot()` as follows:
x = 0:0.1:10; % Create an array from 0 to 10 with an interval of 0.1
y = sin(x); % Calculate sine of each value in x
plot(x, y); % Plot x versus y
title('Sine Wave');
xlabel('X-axis');
ylabel('Y-axis');
For 3D data visualization, you can use commands like `surf()` to create surface plots. Below is how you can visualize a 3D surface:
[X, Y] = meshgrid(-5:0.5:5, -5:0.5:5);
Z = sin(sqrt(X.^2 + Y.^2));
surf(X, Y, Z);
title('3D Surface Plot');
xlabel('X-axis');
ylabel('Y-axis');
zlabel('Z-axis');
Customizing visualizations is equally important. Adding titles, labels, and legends enhances interpretability. Here's how you can customize a plot:
plot(x, y);
title('Sine Wave', 'FontSize', 14);
xlabel('Time (seconds)', 'FontWeight', 'bold');
ylabel('Amplitude', 'Color', 'r');
legend('sin(x)');
grid on;
Data Analysis and Statistical Functions
MATLAB excels in data analysis. You can perform basic statistical calculations easily. For instance, to find the mean, median, and mode of an array:
data = [1, 2, 3, 4, 4, 5, 6];
meanVal = mean(data); % Calculate mean
medianVal = median(data); % Calculate median
modeVal = mode(data); % Calculate mode
Visualizing this data through histograms can offer insights into the distribution:
histogram(data);
title('Data Distribution');
xlabel('Value');
ylabel('Frequency');
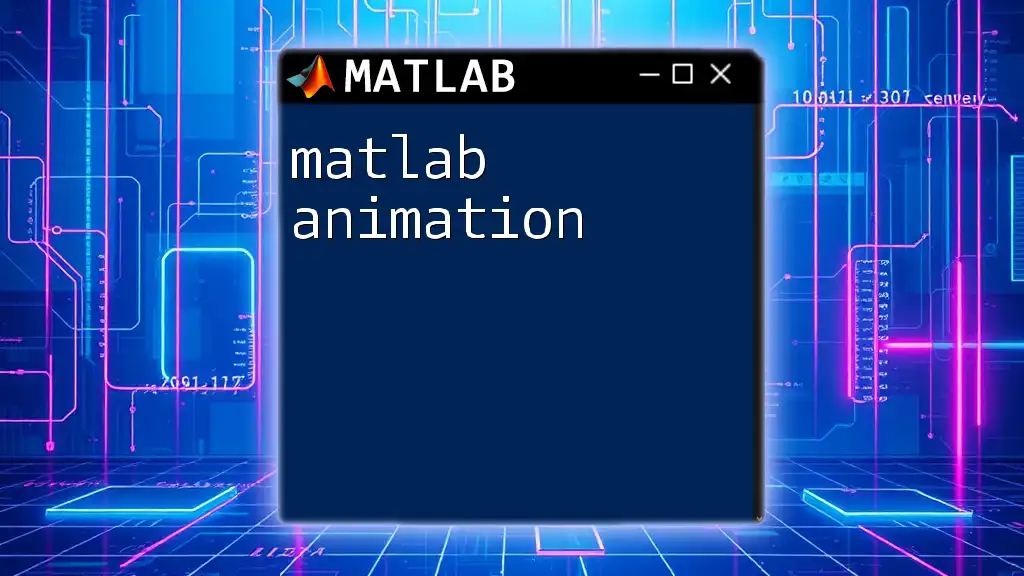
Introducing Simulink
Overview of Simulink
Simulink is an intuitive environment supporting graphical programming. It is predominantly used for modeling and simulating dynamic systems like control systems or signal processing. One of the major advantages of Simulink is its ability to integrate seamlessly with MATLAB, allowing users to leverage the strengths of both.
Building Models in Simulink
To get started with Simulink, simply launch it from MATLAB and create a new model. Familiarize yourself with blocks from various libraries that serve different functions. They can represent mathematical operations, logic conditions, transfer functions, and more.
Creating a Simple Model: As an example, let's model a basic RC circuit. In your Simulink model, you would integrate the following blocks:
- A step input to simulate a voltage input.
- An R (resistor) and C (capacitor) block connected in series.
- A scope block to observe output voltage across the capacitor.
Simulating Models in Simulink
Once your model is built, you can run simulations. Set up simulation parameters by navigating to the `Model Configuration Parameters` menu. To execute your model, simply hit the run button.
Interpreting simulation results requires viewing outputs through scope blocks which show voltage over time. Synchronizing Matlab commands can enhance your understanding, allowing for comparisons with theoretical results.
Customizing Simulink Models
Modifying block parameters tailors the functionality to your needs. Open any block settings to change values, or add a gain block to adjust signal amplitude.
Saving and Exporting Models is crucial. You can save your work in the `.slx` format, making it accessible to others. Additionally, you can export data for further analysis in MATLAB, allowing for an integrated workflow.
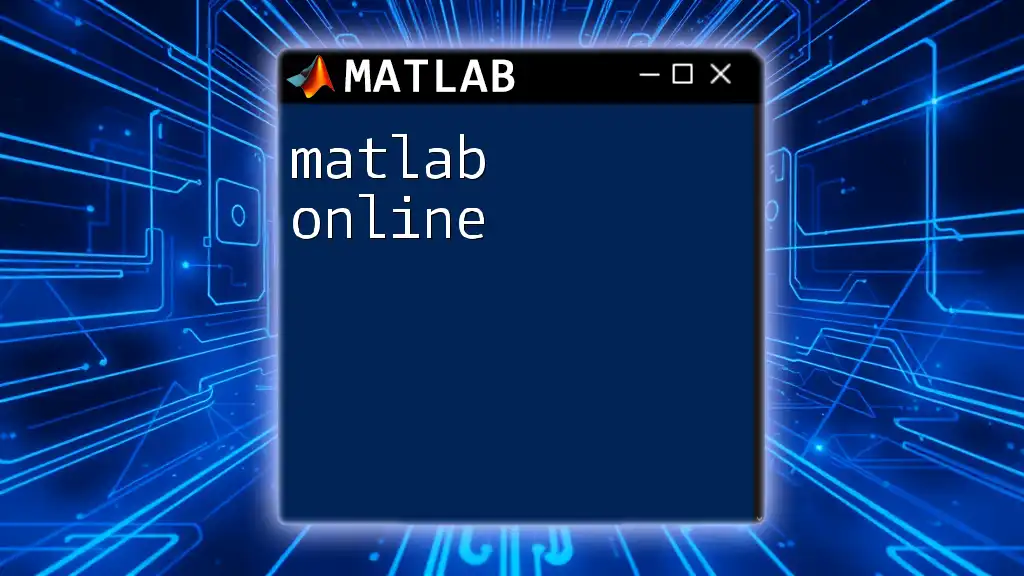
Integration of MATLAB and Simulink
Data Exchange Between MATLAB and Simulink
Data exchange between MATLAB and Simulink opens up a plethora of functionalities. By utilizing MATLAB scripts, you can define input values for your Simulink models effortlessly. For example:
% Define parameters in MATLAB
R = 1000; % Resistor value in ohms
C = 0.001; % Capacitor value in farads
sim('your_simulink_model'); % Run the Simulink model
Extracting simulation results is just as easy. You might want to visualize the output by plotting the results using MATLAB. This seamless interaction enhances debugging and analysis efficiency.
Benefits of Using MATLAB and Simulink Together
The combination of MATLAB and Simulink provides enhanced simulation capabilities. It significantly accelerates the prototyping and testing of algorithms, which is crucial in engineering projects. This integration creates a more efficient workflow, making them indispensable tools in various industries.
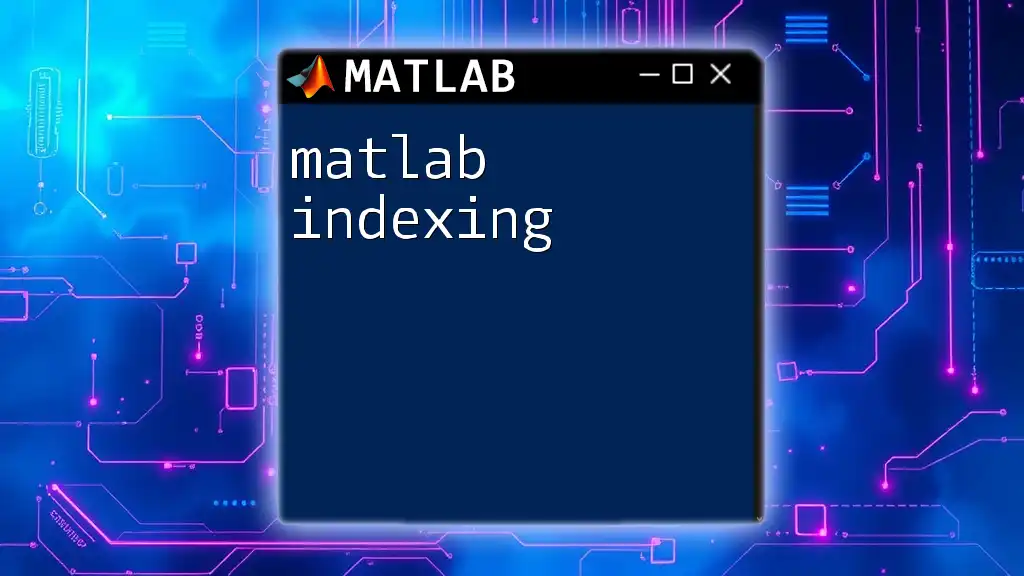
Tips and Best Practices in MATLAB and Simulink
Writing Efficient Code
Efficiency in MATLAB can be achieved through vectorization. Rather than using loops, apply operations to entire arrays. This method optimizes performance while simplifying code.
Best Practices for Simulink Models
Keeping models organized is crucial. Break down complex systems into sub-systems to improve manageability. Utilize annotation blocks to add descriptive comments, ensuring readability for anyone who views your model.
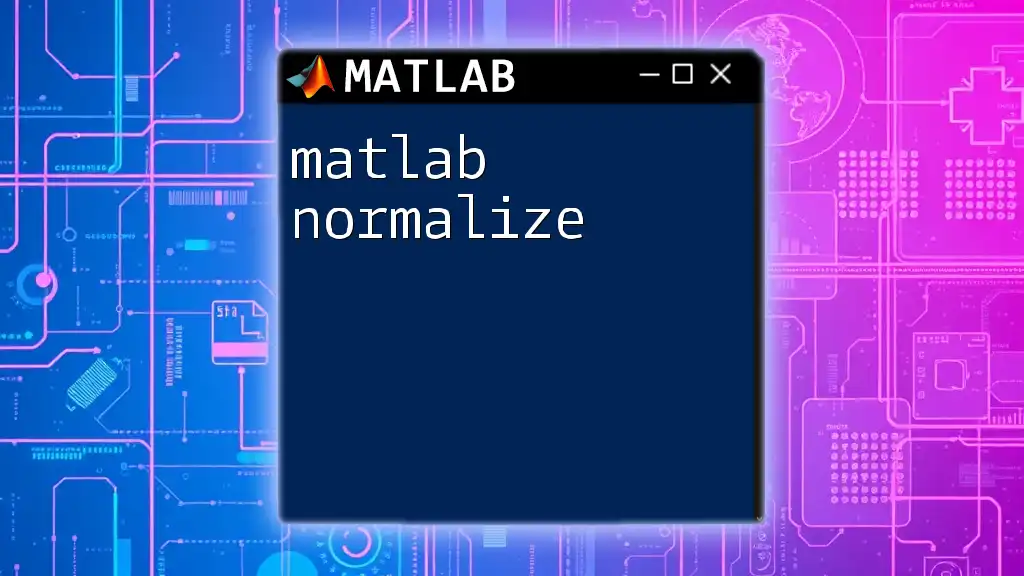
Conclusion
This comprehensive guide on MATLAB and Simulink highlights their functionalities and applications. Both tools are essential for engineers and scientists alike, providing powerful solutions for mathematical modeling, simulation, and data analysis. Becoming well-versed in these tools is a critical step toward mastering modern engineering practices. Explore further, experiment, and you'll rapidly enhance your skills in this domain!
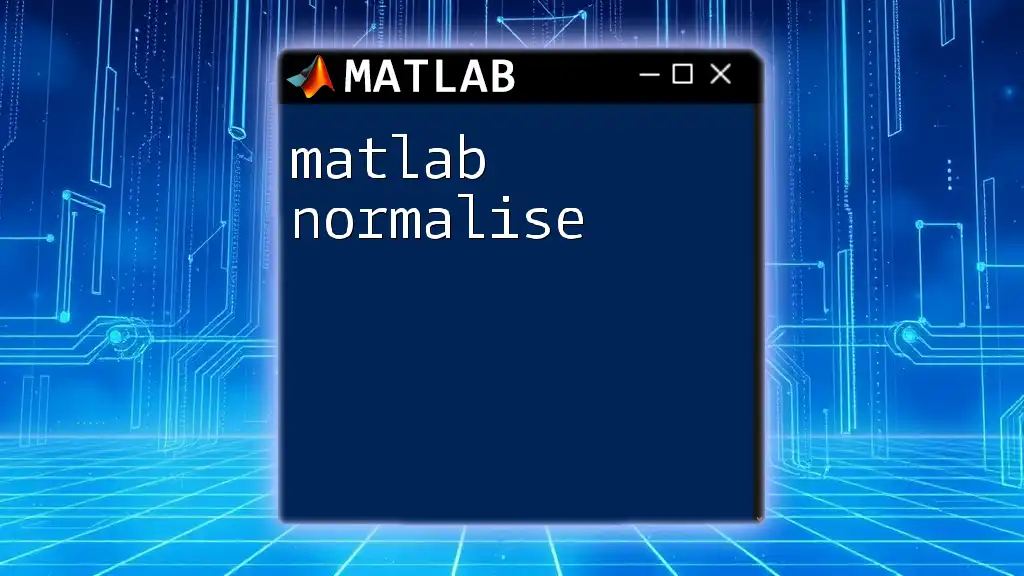
Additional Resources
As you continue your journey in mastering MATLAB and Simulink, consider engaging with recommended books, websites, and forums such as MATLAB Central and Stack Overflow for valuable insights and community support.