MATLAB is a powerful tool for manipulating matrices, allowing users to easily perform operations such as addition, multiplication, and transposition with simple commands.
Here’s a basic example of how to create and manipulate a matrix in MATLAB:
% Create a 2x2 matrix and perform operations
A = [1, 2; 3, 4]; % Define a 2x2 matrix
B = A' % Transpose the matrix
C = A + B % Add A and its transpose
D = A * B % Multiply A by its transpose
Understanding Matrices in MATLAB
Definition of a Matrix
In MATLAB, a matrix is a two-dimensional array of numbers arranged in rows and columns. Matrices are fundamental in mathematics, engineering, and data analysis, forming the backbone of numerous numerical computations.
It's essential to distinguish between scalars, vectors, and matrices:
- Scalar: A single numerical value (e.g., `A = 5;`).
- Vector: A one-dimensional array, either a row vector (horizontal) or a column vector (vertical). Example:
rVec = [1 2 3]; cVec = [1; 2; 3];
- Matrix: A two-dimensional array, such as:
A = [1 2; 3 4];
Creating Matrices
Matrices in MATLAB can be created effortlessly using various methods.
Using the `[]` notation allows you to define matrices directly. For instance:
A = [1 2; 3 4];
This command creates a matrix with two rows and two columns.
Using Built-in Functions offers a more structured approach. Some crucial functions include:
-
`zeros`: Creates a matrix filled with zeros.
Z = zeros(3);
This generates a 3x3 matrix of zeros.
-
`ones`: Creates a matrix filled with ones.
O = ones(3, 4);
This results in a 3x4 matrix of ones.
-
`eye`: Creates an identity matrix.
I = eye(5);
An identity matrix has ones on the diagonal and zeros elsewhere.
Matrix Types
Understanding the different types of matrices is vital:
-
Row and Column Vectors: Vectors are matrices with only one dimension. Row vectors look like `[1 2 3]`, while a column vector appears as:
cVec = [1; 2; 3];
-
Square Matrices: These have an equal number of rows and columns (e.g., a 2x2 or 3x3 matrix).
-
Diagonal Matrices: These have non-zero elements only along the diagonal. Creating one can be accomplished as follows:
D = diag([1 2 3]);
-
Sparse Matrices: These contain many zeros and can be defined using the `sparse` function, which optimally allocates memory. Example:
S = sparse(1000,1000);
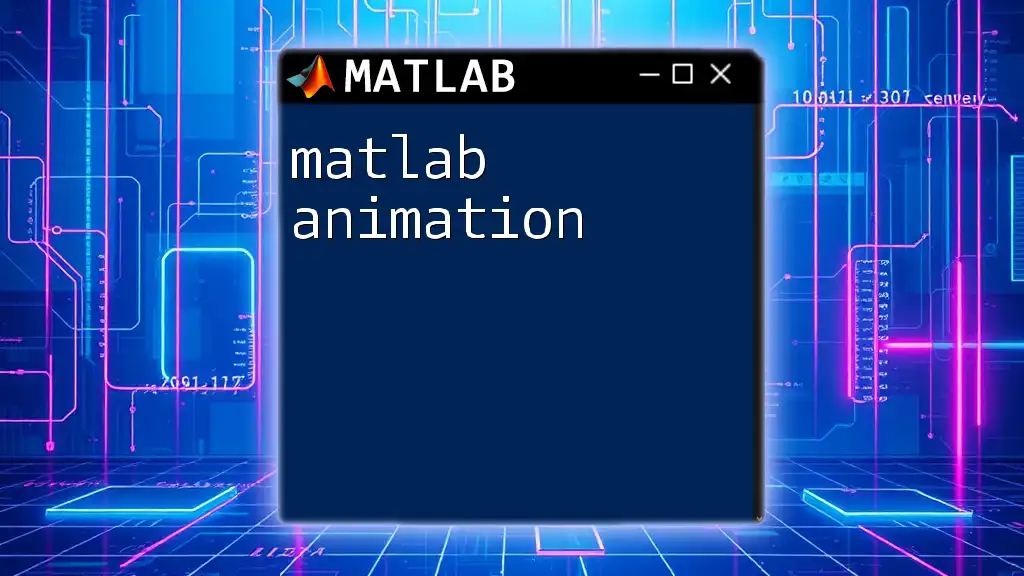
Manipulating Matrices in MATLAB
Accessing Elements
Indexing and Slicing in MATLAB allows you to access specific elements, rows, or columns of matrices. For example, to retrieve the element in the first row and second column of matrix `A`, you can execute:
element = A(1,2);
This will provide the value `2` from the previously defined matrix `A`.
Modifying Matrices
Altering elements in matrices is straightforward. To change a specific value:
A(1,1) = 10;
This command updates the first element of matrix `A` to `10`.
You can also add or remove rows or columns efficiently. To append a new row, you could use:
A(end+1,:) = [5, 6];
This adds a new row to the end of matrix `A`.
Similarly, to add a column, you can do:
A(:,end+1) = [7; 8; 9];
To remove elements, use `A(1,:) = [];` to delete the first row or `A(:,1) = [];` to remove the first column.
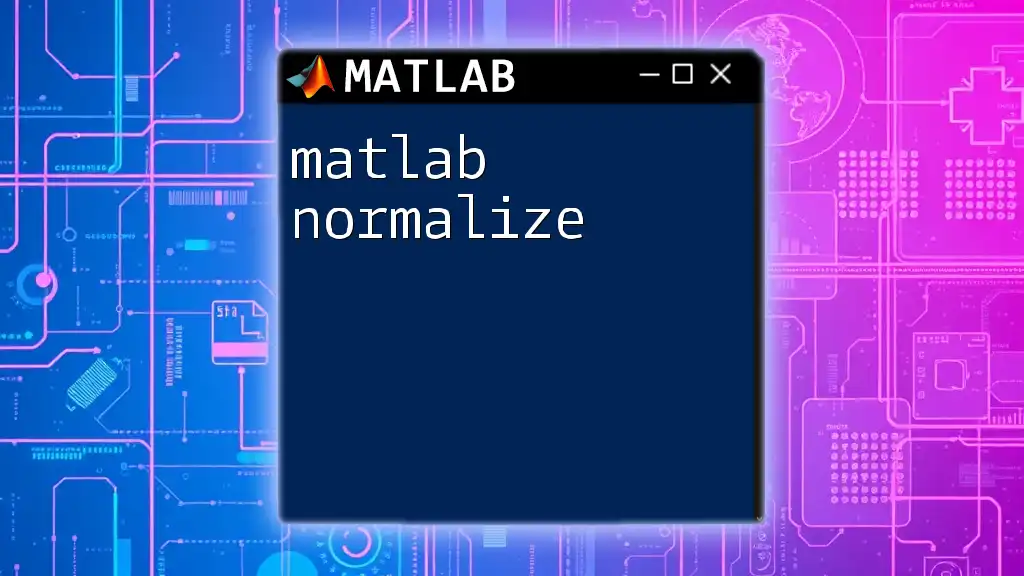
Matrix Operations
Basic Arithmetic Operations
In MATLAB, you can execute fundamental arithmetic operations with matrices seamlessly.
Addition and Subtraction: Matrices of the same size can be added or subtracted directly. For instance:
C = A + B;
Ensure that `A` and `B` have identical dimensions.
Multiplication: Understanding multiplication nuances is crucial.
-
Element-wise multiplication: Use the dot operator `.*`. Example:
C = A .* B;
This multiplies corresponding elements of matrices `A` and `B`.
-
Matrix multiplication: To perform standard matrix multiplication, use the `*` operator. Example:
C = A * B;
Note that inner dimensions must align; if `A` is `m x n`, then `B` must be `n x p`.
Special Matrix Functions
Certain functions provide advanced capabilities:
Transposing a Matrix: This flips a matrix over its diagonal. To transpose matrix `A`, simply use:
B = A';
This command switches rows with columns.
Finding the Determinant and Inverse: For square matrices, you can find the determinant and inverse easily. Use:
detA = det(A);
To calculate the determinant, and for the inverse matrix:
invA = inv(A);
Ensure `A` is non-singular (determinant not equal to zero) to compute the inverse.
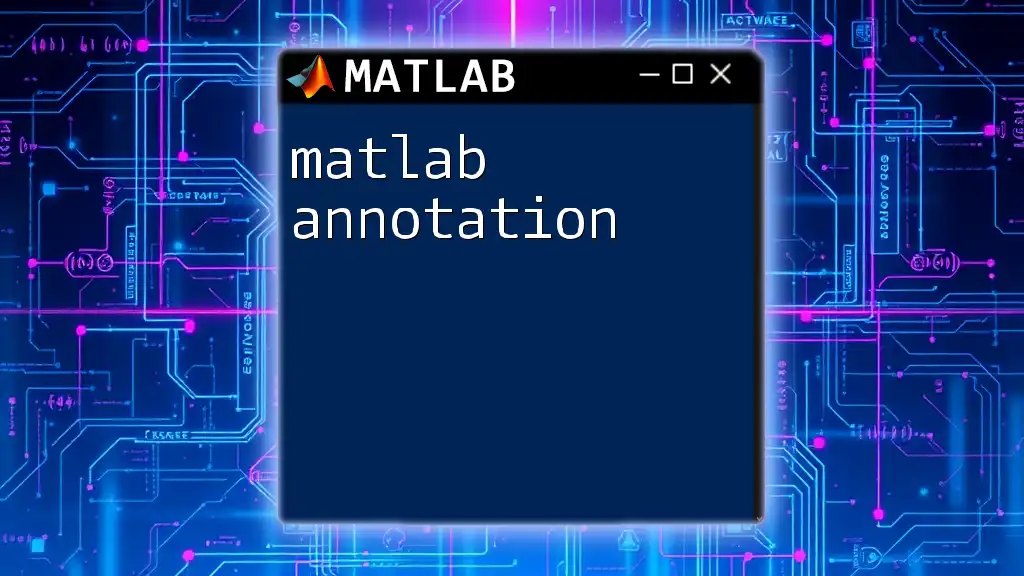
Advanced Matrix Techniques
Solving Linear Equations
Matlab excels in solving linear equations represented in matrix form. You may express the equation \(Ax = b\) and use the backslash operator for solutions:
x = A\b;
This will find the vector \(x\) that satisfies the equation.
Matrix Factorizations
LU Decomposition is useful for simplifying matrix equations. Obtain the LU decomposition of `A` by executing:
[L,U,P] = lu(A);
Here, `L` is a lower triangular matrix, `U` is upper triangular, and `P` is the permutation matrix, if necessary.
Singular Value Decomposition (SVD) allows you to decompose any matrix into three matrices:
[U, S, V] = svd(A);
SVD is extensively used in data analysis, including dimensionality reduction techniques like PCA (Principal Component Analysis).
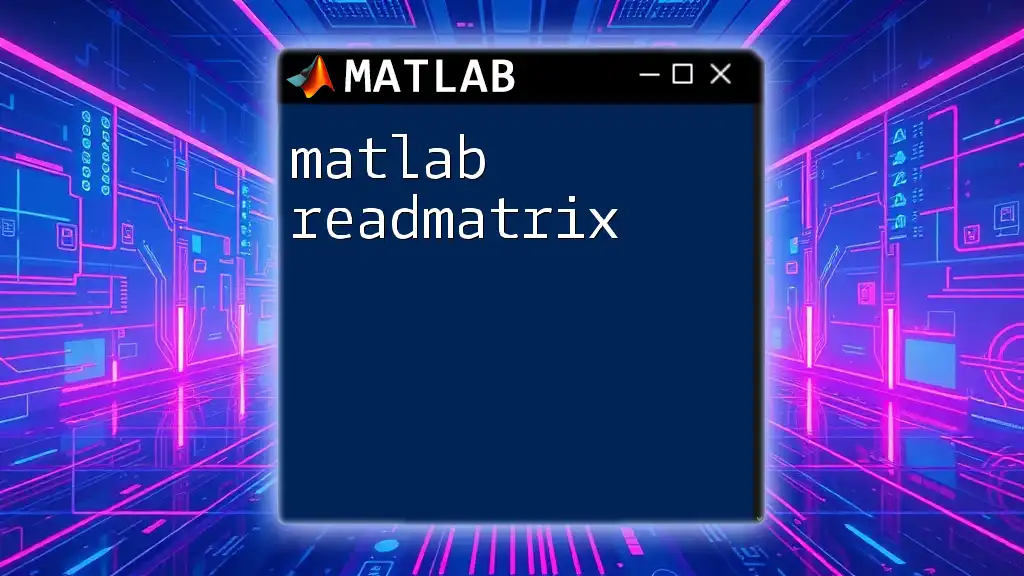
Visualizing Matrices
Visual representations can help comprehend matrix structures and data better. Using `imagesc` allows you to visualize a matrix with color coding:
imagesc(A);
colorbar;
Employing colormaps helps give insights into how various matrix elements relate to one another visually.
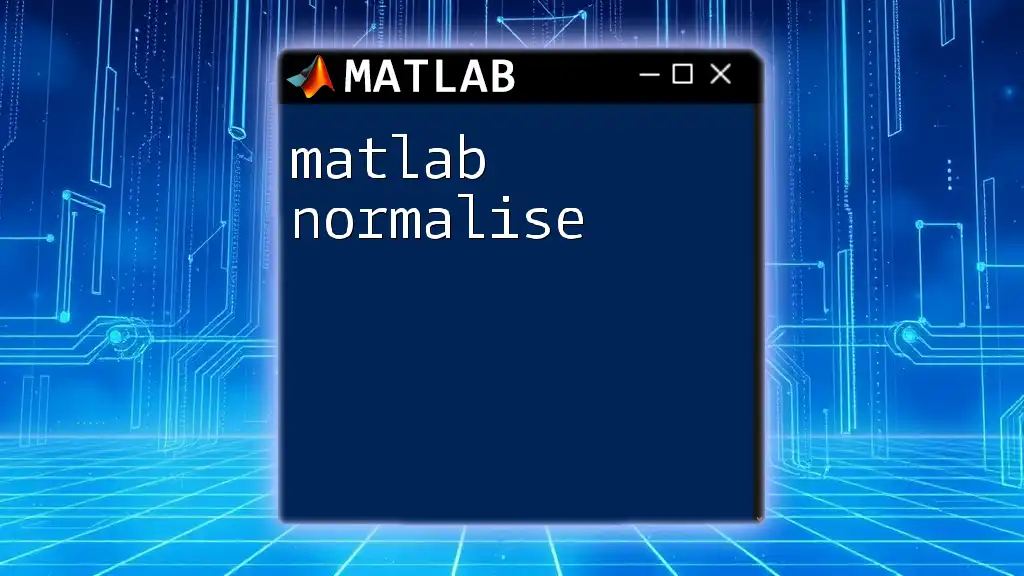
Tips and Best Practices
When working with matrices in MATLAB, it’s essential to keep a few best practices in mind:
- Always write clean and efficient code. Using functions like `preallocate` can save time and memory when dealing with larger matrices.
- Familiarize yourself with MATLAB's built-in documentation and use the help command (`help function_name`) to learn more about specific functions and features.
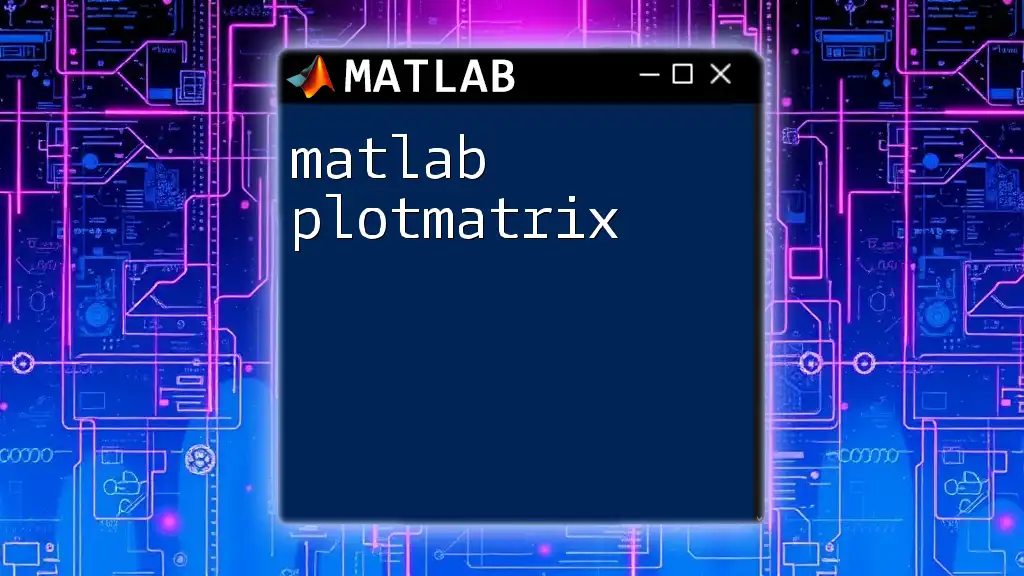
Conclusion
This guide on MATLAB and matrices serves as a fundamental starting point for understanding how to work with matrices effectively. By mastering the creation, manipulation, and operations on matrices, you can harness the full power of MATLAB for a variety of applications, from numerical analysis to advanced engineering problems.
Call to Action
If you're eager to delve deeper into the world of MATLAB, consider joining our training sessions, where we’ll explore practical applications and best practices for leveraging MATLAB in your projects. Stay tuned for our upcoming webinars focusing on MATLAB matrices and other valuable topics!