The `rand` function in MATLAB generates uniformly distributed random numbers between 0 and 1, which can be useful for simulations and statistical sampling.
Here's a code snippet demonstrating its usage:
% Generate a 3x3 matrix of random numbers
randomMatrix = rand(3);
Understanding the Basics of rand Function
What is the rand Function?
The `rand` function in MATLAB is a built-in function that generates uniformly distributed random numbers in the interval \([0, 1)\). It is an essential tool for a variety of applications in programming, from simulations to probabilistic analysis. Understanding how to use `rand` effectively can greatly enhance your MATLAB programming skills, especially in fields involving statistical modeling, data analysis, and optimization.
Syntax of rand Function
The syntax for the `rand` function can be summarized as follows:
rand(size)
This function can be utilized in various ways to generate numbers of different dimensions:
- `rand(m, n)`: Generates an \( m \times n \) matrix.
- `rand([m n])`: An alternative syntax that achieves the same result by passing an array.
These variations allow you to create matrices of any size, which is particularly useful when your computations require different data structures.
Output Characteristics
The output of the `rand` function consists solely of floating-point numbers ranging from \( 0 \) to \( 1 \). Each call to the function produces a different set of numbers unless a seed is set with the `rng` function (discussed later). The values generated are ideal for simulations that require stochastic processes or random sampling methodologies.
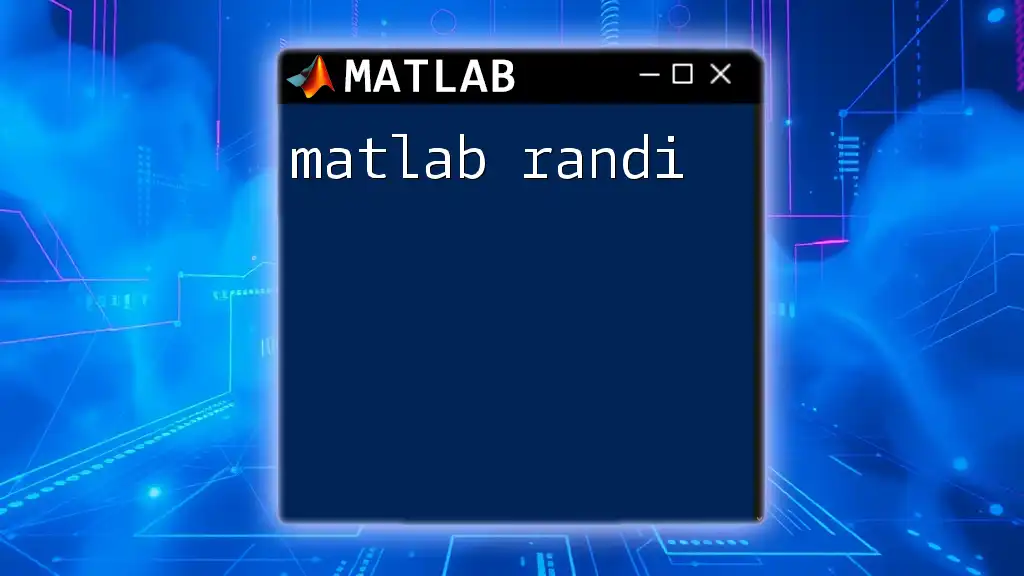
Generating Random Numbers
Generating a Single Random Number
To generate a single random number, simply call the `rand` function without arguments:
singleValue = rand();
This command assigns a random value between \( 0 \) and \( 1 \) to `singleValue`. Such a simple function is often used in scenarios where a single, unpredictable score is necessary.
Generating Vectors and Matrices of Random Numbers
Creating a Vector of Random Numbers
If you wish to generate a random vector, you can specify the desired size. For example, to create a \( 1 \times 5 \) vector of random numbers:
vector = rand(1, 5); % 1-by-5 vector
The variable `vector` will contain five random values between \( 0 \) and \( 1 \). This approach is beneficial in testing algorithms or for scenarios requiring sample data.
Creating Matrices
To create matrices, you can define the number of rows and columns directly. For instance, to generate a \( 3 \times 4 \) matrix:
matrix = rand(3, 4); % 3-by-4 matrix
This command generates a 3-row by 4-column matrix filled with random numbers, which can be utilized in various data analyses or visualizations.
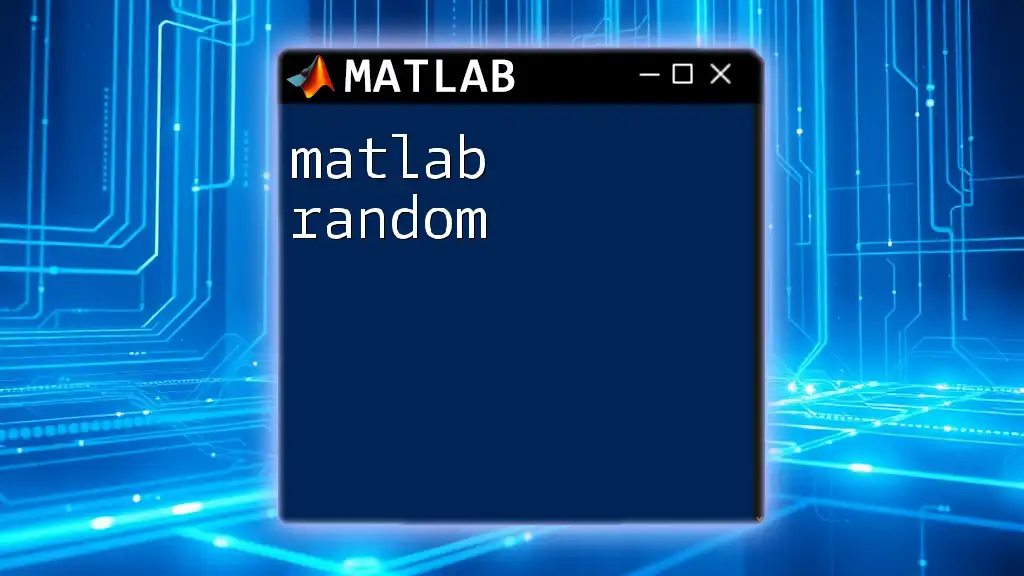
Customizing Random Number Generation
Specifying the Range of Values
Often, you may need random numbers that fall within a specific range. You can easily scale and shift the output from `rand`:
lower_bound = 10;
upper_bound = 20;
randomNumInRange = lower_bound + (upper_bound - lower_bound) * rand();
In this example, `randomNumInRange` will contain a value between 10 and 20. This is particularly useful in simulations where you need to control the constraints of your random samples, such as generating random heights or scores within given limits.
Seeding the Random Number Generator
To ensure that the random numbers generated are reproducible across different runs, you must set the seed for the random number generator:
rng(1); % Set seed for reproducibility
randomNumbersSeeded = rand(5);
By calling `rng(1)`, you ensure that every execution of your script produces the same sequence of random numbers. Reproducibility is crucial for debugging and results validation in any computational project.
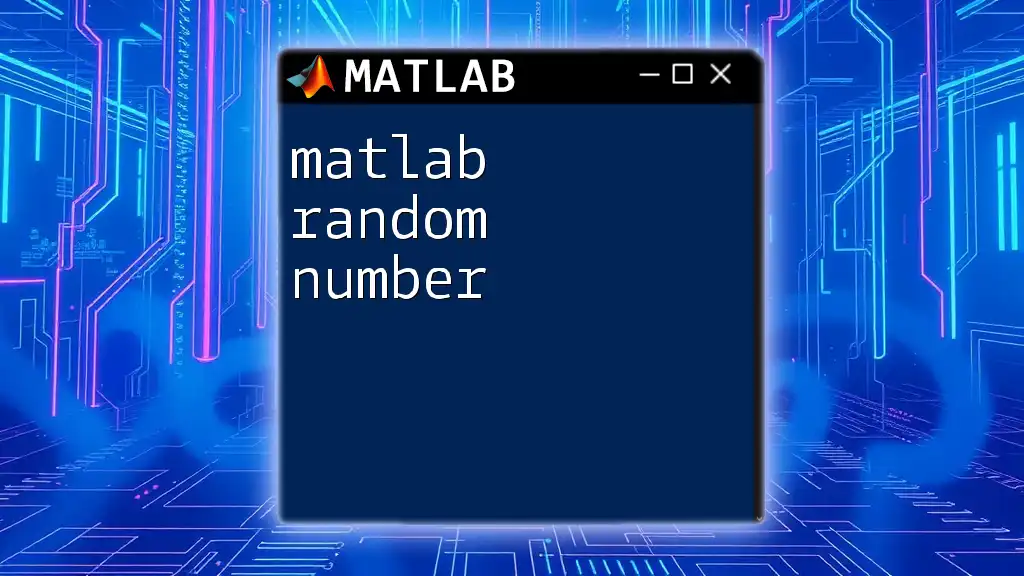
Applications of rand Function
Simulations
One of the primary applications of the `rand` function is in simulations, particularly Monte Carlo simulations. By generating random samples, you can approximate distributions or calculate integrals. For instance, simulating the outcome of a random experiment helps analyze the probabilities of different events occurring in complex systems.
Random Sampling
The `rand` function is extremely useful for generating random samples from a larger dataset. If you're interested in selecting random indices, consider the `randperm` function to ensure unique selections:
sample = randperm(10, 3); % Randomly select 3 unique numbers from 1 to 10
This command produces three unique random indices from the numbers \( 1 \) to \( 10 \), which can be applied in various statistical analyses or sampling methods.
Random Noise Generation
Adding randomness to datasets, particularly in time-series data or signal processing, can create more realistic models. Here’s an example of how to add white noise to a sine wave signal:
signal = sin(0:0.01:2*pi);
noisySignal = signal + 0.1 * rand(1, length(signal)); % Adding noise to a sine wave
In this case, `noisySignal` will mimic a sine wave, but with added random fluctuations, allowing for better testing of algorithms under various conditions.
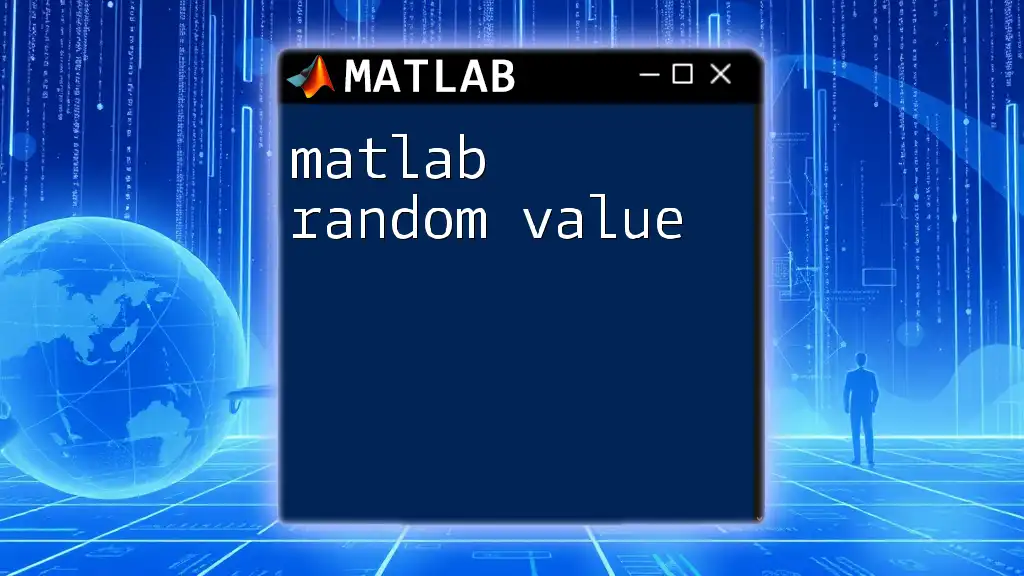
Common Mistakes and Troubleshooting
Common Errors with rand
As with any function, experienced users may encounter pitfalls. One common error involves misconceptions about matrix dimensions. If you request a matrix but accidentally input incorrect dimensions, MATLAB will throw an error, or produce unexpected results.
Performance Considerations
When generating random numbers, it's essential to consider whether you need a large array or if smaller groups will suffice. Creating an unnecessarily large sample can slow down your operations. It is wise to optimize your commands according to the requirements of your task, ensuring an efficient use of system resources.
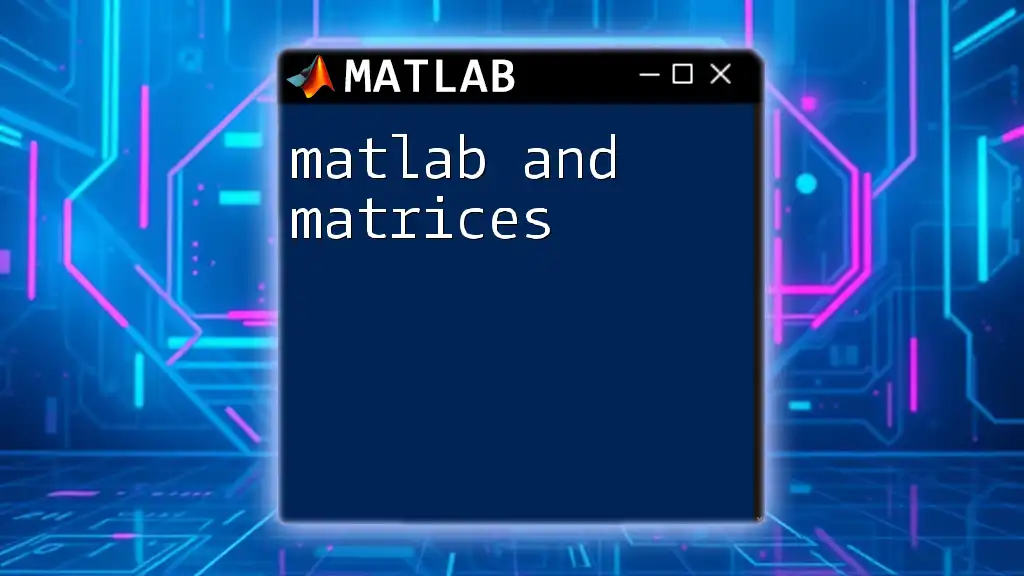
Conclusion
The `rand` function in MATLAB is an indispensable tool for generating random numbers, with numerous applications in simulations, statistical analysis, and algorithm testing. Mastering this function allows you to enhance your programming capabilities and opens doors to more sophisticated analyses. Don't hesitate to explore the broader functions available in MATLAB for random number generation to further elevate your work.
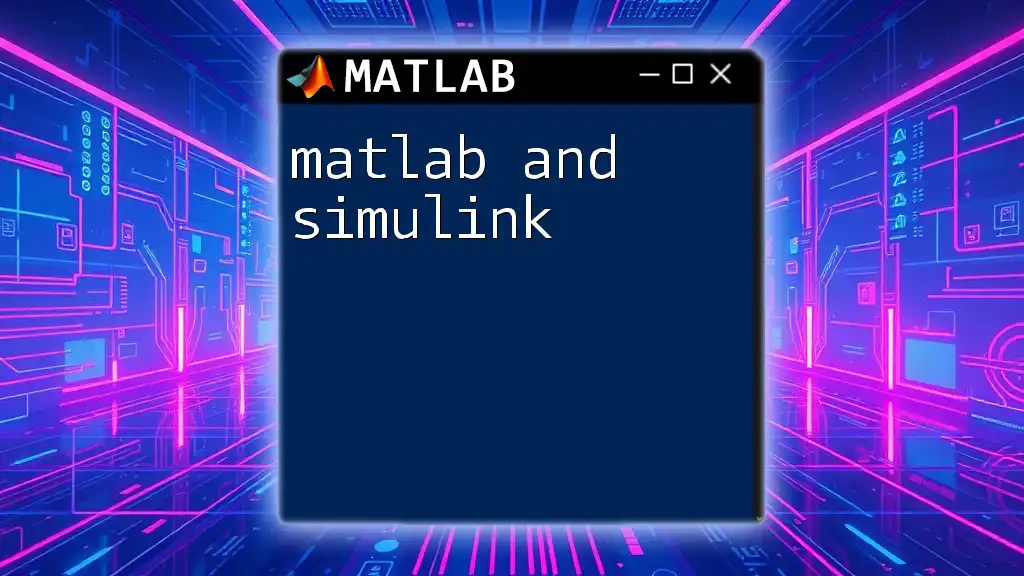
Additional Resources
For those who wish to dive further, refer to the [official MATLAB documentation for rand](https://www.mathworks.com/help/matlab/ref/rand.html). There are many tutorials available online, along with courses designed to deepen your understanding. Engage with MATLAB community forums to share experiences and learn from others in the field.
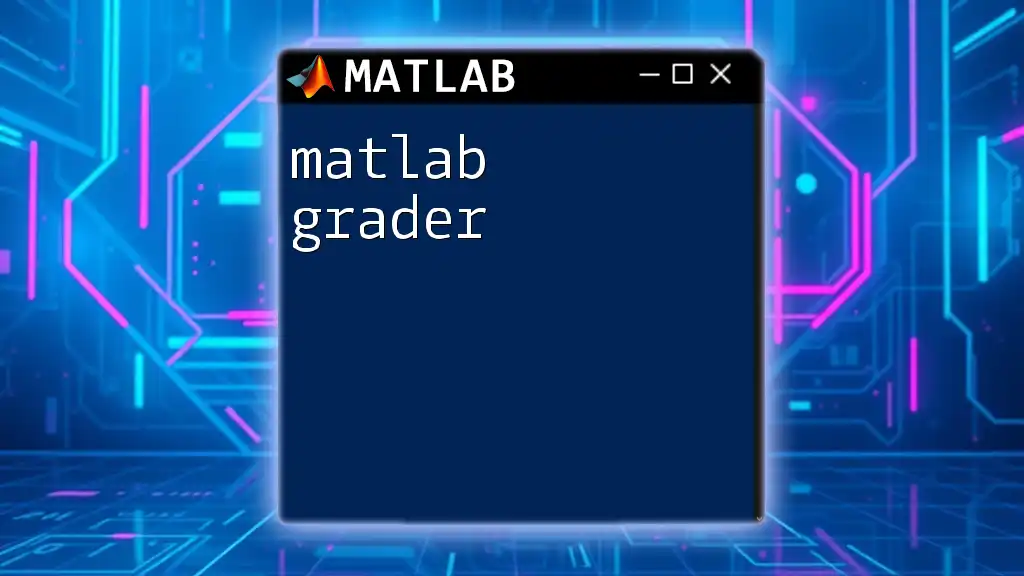
Call to Action
Try running the code snippets provided throughout this guide in your MATLAB environment. Experiment with generating random numbers tailored to your needs and explore advanced features by signing up for our comprehensive courses focused on MATLAB programming!