The MATLAB `norm` function computes the norm of a vector or matrix, which is a measure of its length or size in a given mathematical space.
Here's a code snippet demonstrating its use:
% Example of calculating the 2-norm (Euclidean norm) of a vector
v = [3, 4];
result = norm(v);
disp(result); % Displays: 5
What is a Norm?
Definition of a Norm
In mathematics, a norm is a function that assigns a positive length or size to vectors in a vector space. It provides a measure for quantifying the magnitude of vectors, giving insights into their properties and relationships. In various fields such as data science, engineering, and optimization, understanding norms is crucial for analyzing and interpreting data effectively.
Types of Norms
Different types of norms apply to various scenarios, each serving unique purposes:
-
L2 Norm (Euclidean Norm): This is perhaps the most common type of norm, defined mathematically as: \[ \|x\|2 = \sqrt{\sum{i=1}^{n} x_i^2} \] The L2 norm corresponds to the Euclidean distance in a Cartesian space and is particularly useful in least squares problems.
-
L1 Norm (Manhattan Norm): Defined as: \[ \|x\|1 = \sum{i=1}^{n} |x_i| \] The L1 norm calculates the sum of the absolute values of the components. This norm is often used in sparse modeling, where a smaller number of features is preferred.
-
Infinity Norm: Also known as the maximum norm, this can be expressed as: \[ \|x\|_{\infty} = \max_i |x_i| \] It assesses the maximum deviation among the components of a vector and finds applications in constraint satisfaction problems.
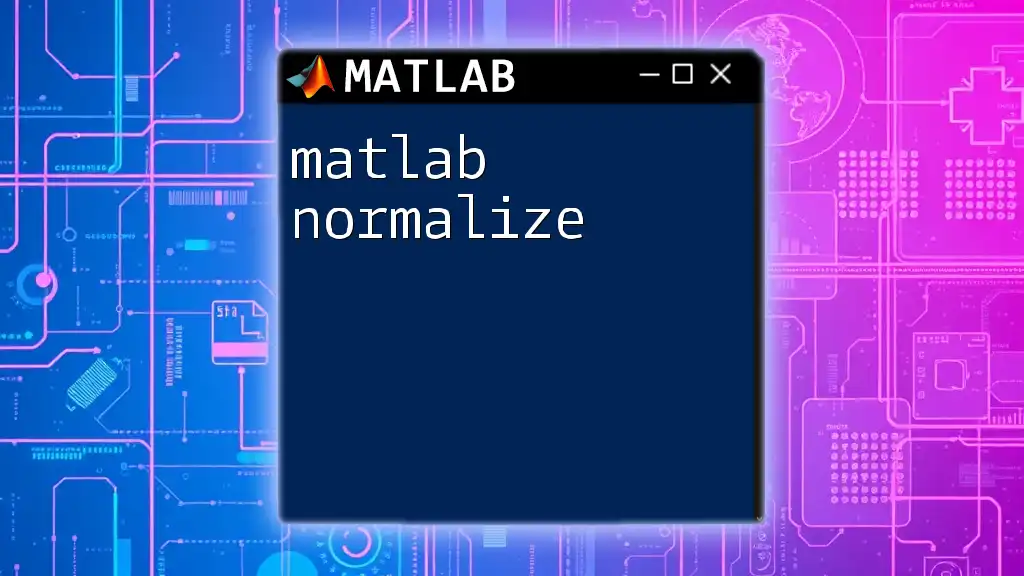
Using Norms in MATLAB
MATLAB Functions for Norms
In MATLAB, the `norm()` function is a powerful tool for calculating various norms. This function can take as arguments either a vector or a matrix and can compute the norm specified in a second argument:
A = [3; 4];
euclideanNorm = norm(A); % Computes the L2 norm
manhattanNorm = norm(A, 1); % Computes the L1 norm
infNorm = norm(A, Inf); % Computes the infinity norm
This simple syntax shows how easy it is to use MATLAB for calculating norms, making your data analysis both efficient and effective.
Calculating Different Types of Norms
L2 Norm Calculation
To calculate the L2 norm in MATLAB, you can use the `norm()` function without additional parameters. This will yield the Euclidean norm. Here’s how you can do it using both vector and matrix inputs:
vec = [1, 2, 3];
matrix = [1, 2; 3, 4];
disp(['L2 Norm of vector: ', num2str(norm(vec))]);
disp(['L2 Norm of matrix: ', num2str(norm(matrix))]);
Running this code will provide the L2 norms for both the vector and the matrix, illustrating how the dimension impacts the result.
L1 Norm Calculation
Calculating the L1 norm in MATLAB requires specifying the second argument as 1:
disp(['L1 Norm of vector: ', num2str(norm(vec, 1))]);
disp(['L1 Norm of matrix: ', num2str(norm(matrix, 1))]);
This command will return the sum of the absolute values of the components, showcasing its applicability in scenarios where the focus is on the total deviation from zero, such as in sparse solutions.
Infinity Norm Calculation
To find the infinity norm, simply set the second argument to `Inf`:
disp(['Infinity Norm of vector: ', num2str(norm(vec, Inf))]);
disp(['Infinity Norm of matrix: ', num2str(norm(matrix, Inf))]);
These commands effectively retrieve the maximum element of the vector and matrix, useful in scenarios where you need to ensure no single component exceeds certain limits.
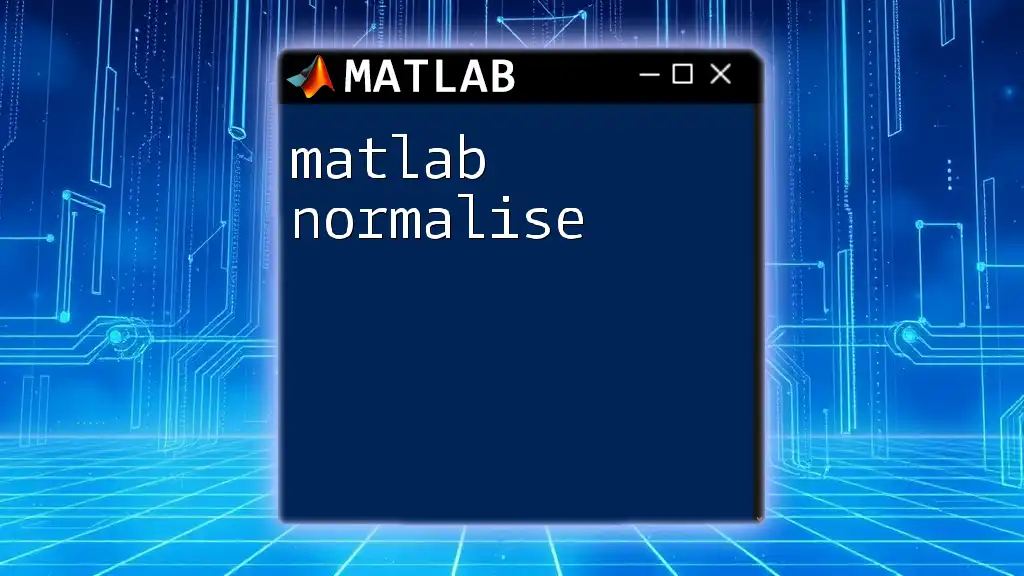
Applications of Norms in Various Fields
Data Analysis
Norms are foundational in data analysis, where they help quantify distances and similarities among data points. For example, in clustering algorithms, the L2 norm is often used to measure how closely data points group together. This is vital for understanding data distributions, identifying outliers, and interpreting complex data sets.
Machine Learning
In machine learning, norms play a crucial role in regularization techniques, which are essential for preventing overfitting. Regularization strategies such as Ridge regression (L2 regularization) and Lasso regression (L1 regularization) utilize norms to control the complexity of models. The choice between L1 and L2 norms can significantly impact model performance, where L1 encourages sparsity leading to simpler models while L2 retains all features with reduced impact from multi-collinearity.
Engineering
Within engineering, norms assist in solving systems of equations and optimization problems. For example, norms help measure how close an approximate solution is to the true solution, enabling engineers to create accurate models. Whether it’s minimizing error in signal processing or analyzing stability in control systems, having a firm grasp of norms is invaluable.
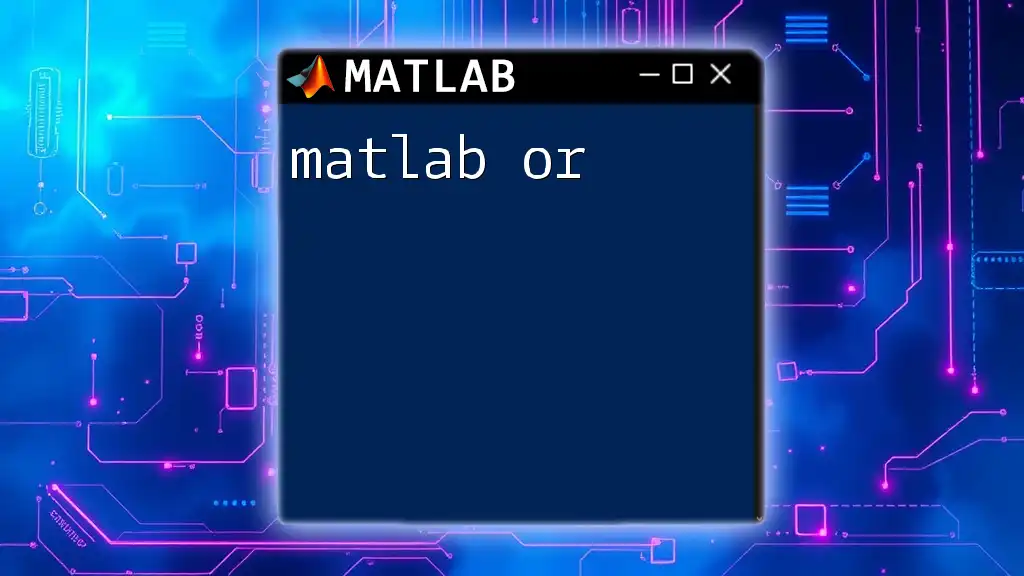
Best Practices for Using Norms in MATLAB
When working with norms in MATLAB, it's crucial to choose the right type based on the context of your problem. The L1 norm may offer benefits in a sparsity context, while the L2 norm can give a more stable metric against small perturbations. Be aware that each norm conveys different information, and interpreting results depends on understanding the underlying data structure.
Avoid common pitfalls such as neglecting the scale of data or failing to normalize inputs before applying algorithms that utilize norms. Understanding the context in which you apply norms will enhance your ability to draw meaningful conclusions from your analyses.
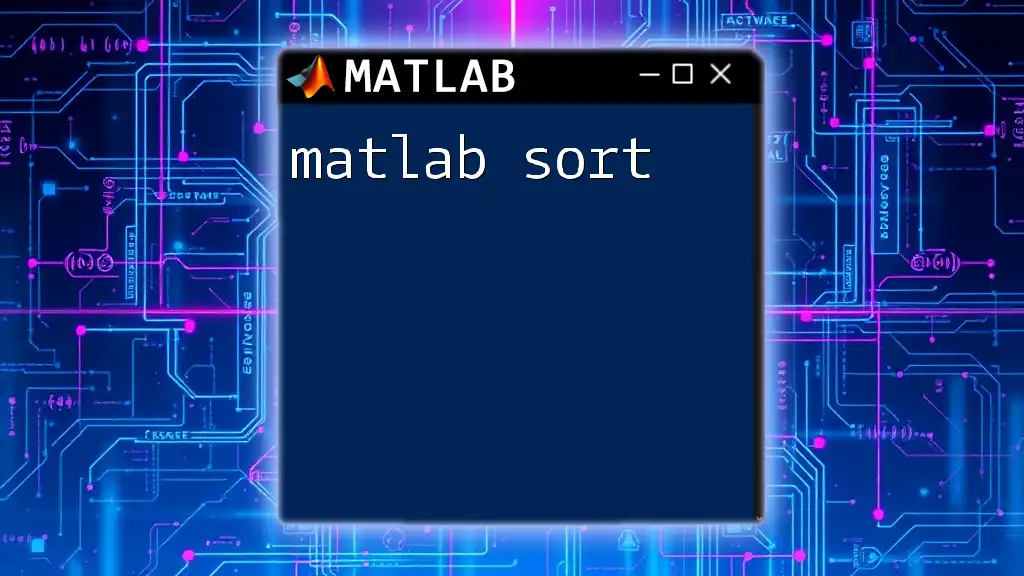
Real-World Example: Comparing Norms
Case Study
Consider a data analysis task where we wish to evaluate distances among data points in a high-dimensional space. By implementing the L1, L2, and Infinity norms, you can uncover different aspects of the data distribution. For instance, if you're using L1 norms, you may notice that certain features dominate the distance calculations, highlighting their importance. Conversely, the L2 norm could yield a more balanced perspective. In optimization problems, applying the Infinity norm may reveal critical constraints that need to be addressed before progressing.
This comparative analysis helps solidify your understanding of the implications of choosing one norm over another and demonstrates how adapting your approach can lead to better problem-solving strategies.
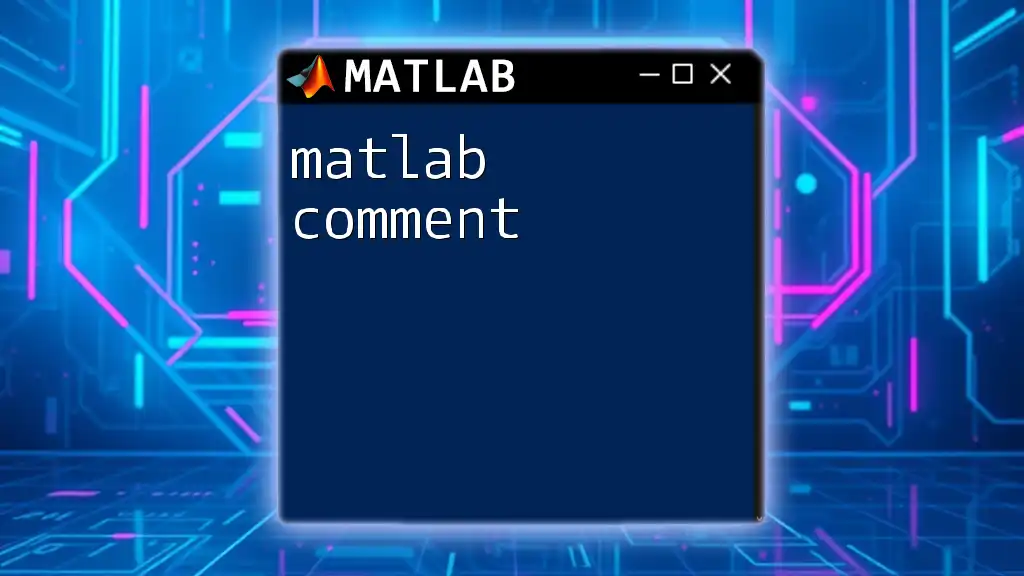
Conclusion
Grasping the concept of the MATLAB norm and its applications will significantly enhance your capabilities in data analysis, machine learning, and even engineering tasks. By practicing with various norms and understanding their implications, you’ll be well-equipped to tackle complex problems in your field.
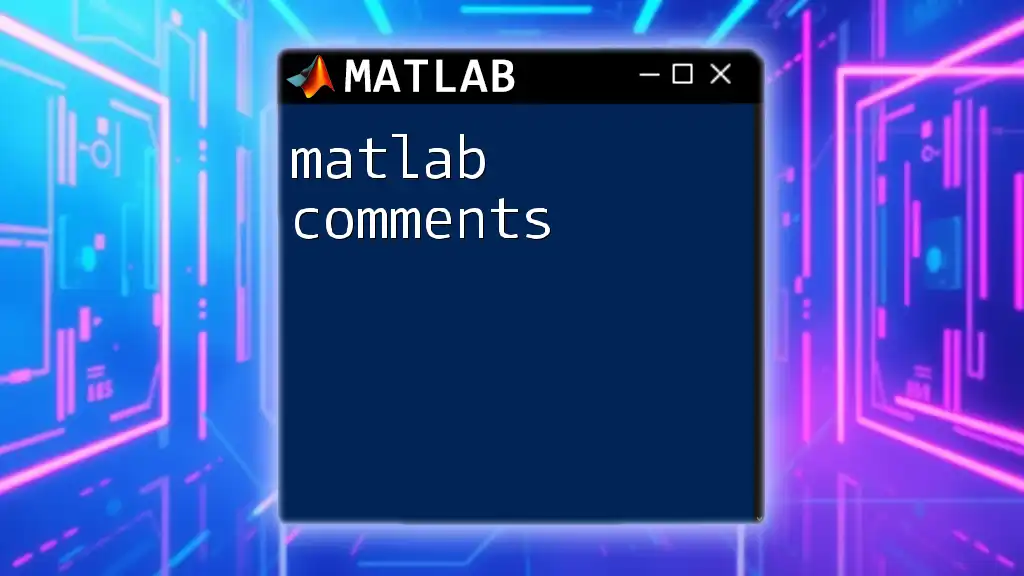
Further Resources
For those looking to deepen their knowledge of norms and their application in MATLAB, consider exploring the MATLAB documentation, online coding platforms, and related courses that focus extensively on these concepts. Engaging with these resources will provide clarity and reinforce your learning, ensuring you master the use of norms in MATLAB.