The area under a curve in MATLAB can be calculated using the `trapz` function, which implements the trapezoidal rule to approximate the integral of a set of data points.
Here’s a code snippet to demonstrate this:
x = [0, 1, 2, 3, 4]; % x-coordinates
y = [1, 3, 2, 5, 4]; % y-coordinates
area = trapz(x, y); % Calculate area under the curve
disp(area); % Display the result
Understanding Area Under Curve Concepts
Definition of Area Under Curve
The Area Under Curve (AUC) can be mathematically defined through the concept of integrals. Specifically, it represents the integral of a function F(x) over an interval [a, b]. This can be expressed as:
\[ AUC = \int_{a}^{b} F(x) \, dx \]
This definition plays a critical role in multiple fields, including statistics and engineering, as it provides a numerical measure of the total accumulation of a value represented by the function over a specified range.
Graphical Interpretation
Visualizing AUC typically involves plotting a function on a Cartesian plane. The area beneath the curve from point a to point b represents the AUC. When observing different shapes of curves, you will notice that the area can vary significantly.
For instance, a linear function generates a triangle below the curve. In contrast, a quadratic function resembles a parabolic shape, resulting in a different area size. Understanding these graphical representations is vital when calculating and interpreting AUC.
Applications of AUC
The AUC has extensive applications across various domains. Some key areas include:
- Data Analysis: In analyzing datasets, AUC helps quantify total production, consumption, or other accumulative measures.
- Finance: AUC methods are used for option pricing and evaluating financial metrics.
- Machine Learning: The AUC is pivotal in evaluating the performance of classification models, particularly when assessing the Receiver Operating Characteristic (ROC) curve.
In machine learning, for example, a higher AUC indicates a better model performance in distinguishing between classes.
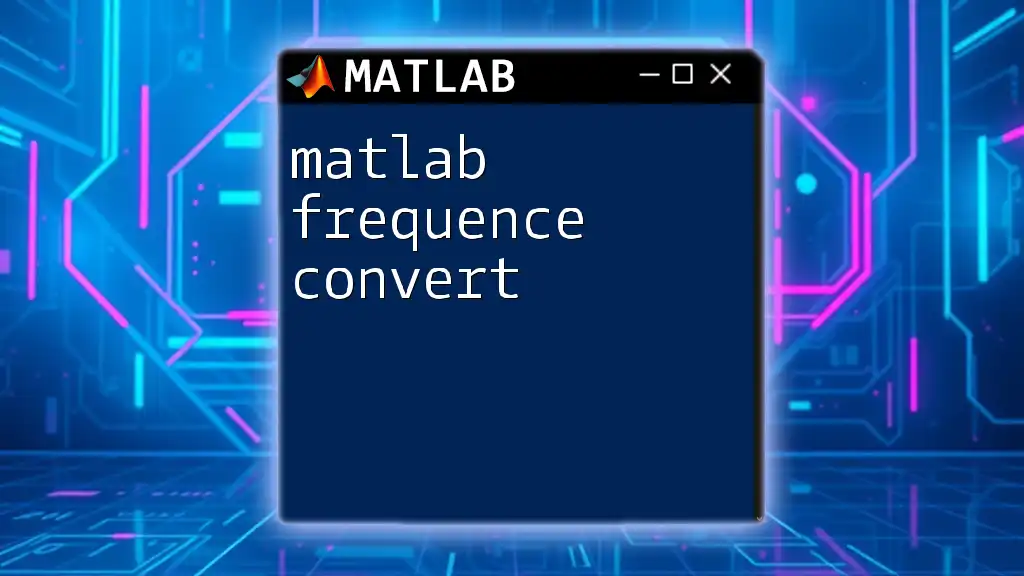
MATLAB Commands for Calculating AUC
Fundamental MATLAB Functions
MATLAB is equipped with powerful functions specifically designed for numerical analysis, among which `trapz()` and `integral()` are essential for calculating AUC.
Using `trapz()` Function
The `trapz()` function computes the integral of discrete data points using the trapezoidal rule.
Basic Syntax and Structure The general structure for using `trapz()` in MATLAB is:
AUC = trapz(X, Y)
Here, X represents the independent variable (e.g., time), and Y represents the dependent variable (e.g., observed data).
Example Code Snippet
x = [0 1 2 3];
y = [0 1 4 9];
AUC = trapz(x, y);
fprintf('Area under curve: %.2f\n', AUC);
Step-by-Step Explanation of Example
- The array `x` contains values for the independent variable.
- The array `y` holds corresponding observed values of the dependent variable.
- The `trapz()` function applies the trapezoidal rule to compute the area, resulting in AUC, which is displayed with a formatted message.
Using `integral()` Function
The `integral()` function provides a way to compute a definite integral of a function. This function supports symbolic integration, making it versatile for continuous functions.
Basic Syntax and Integration Techniques The syntax for using `integral()` is as follows:
AUC = integral(F, a, b)
Where F is a function handle, and a and b are the limits of integration.
Example Code Snippet
f = @(x) x.^2; % Define the function
AUC = integral(f, 0, 3); % Compute integral from 0 to 3
fprintf('Area under curve: %.2f\n', AUC);
Illustrative Example In this example, the function being integrated is \( f(x) = x^2 \). The computed AUC corresponds to the area under this curve from 0 to 3. By executing this code, MATLAB will provide the AUC value, showcasing the power of numerical integration.
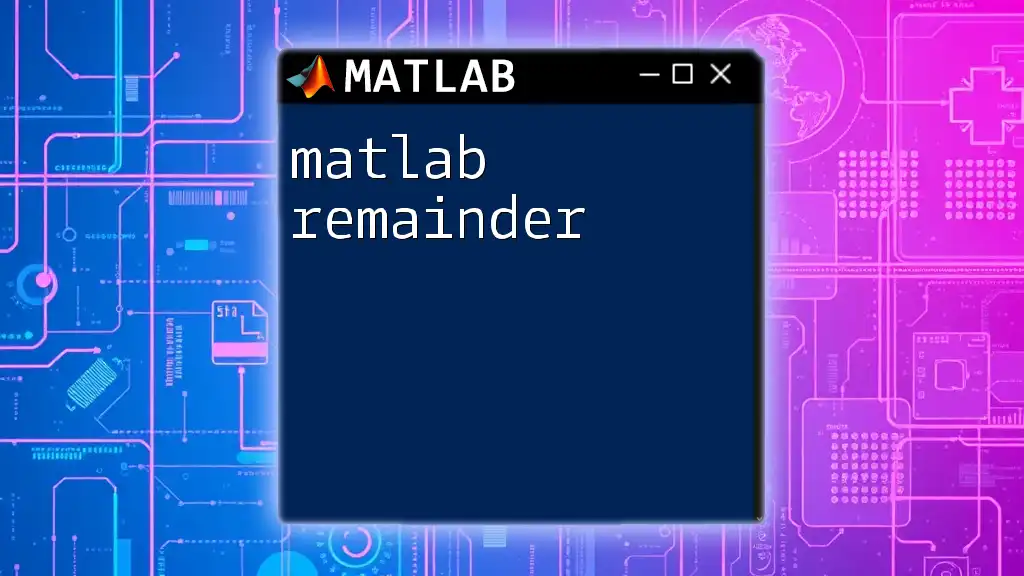
Advanced Techniques
AUC for Discrete Data
When dealing with empirical datasets, it’s essential to process the data appropriately to enable the calculation of AUC.
Handling Empirical Data Sets Often, data will not be perfectly continuous, and thus utilizing discrete points is crucial. Here’s how you can calculate AUC from empirical data:
data = [1.0, 2.5, 3.3, 4.1, 5.0];
time = [0, 1, 2, 3, 4];
AUC = trapz(time, data);
Visualization To better understand the AUC from empirical data, it is useful to plot the data points along with the computed area:
plot(time, data, '-o');
hold on;
area(time, data, 'FaceColor', 'cyan', 'FaceAlpha', 0.5);
hold off;
title('Area Under Curve Visualization');
This will illustrate the area under the curve in a visually appealing manner, enhancing understanding of how AUC is used with real-world datasets.
Comparing Curves
In practical scenarios, you may need to compare the AUC among multiple datasets or curves.
Multiple Curves and Their AUCs Using the `trapz()` function, you can compute AUC for multiple curves efficiently:
x1 = [0 1 2 3];
y1 = [0 1 4 9];
x2 = [0 1 2 3];
y2 = [0 0.5 2 8];
AUC1 = trapz(x1, y1);
AUC2 = trapz(x2, y2);
fprintf('AUC1: %.2f, AUC2: %.2f\n', AUC1, AUC2);
Visual Comparison using MATLAB To create overlapping plots for better comparison, you can plot both curves on the same axis. By visually analyzing the curves along with their respective AUCs, you can draw conclusions about which dataset accumulates more – a critical skill in data analysis.
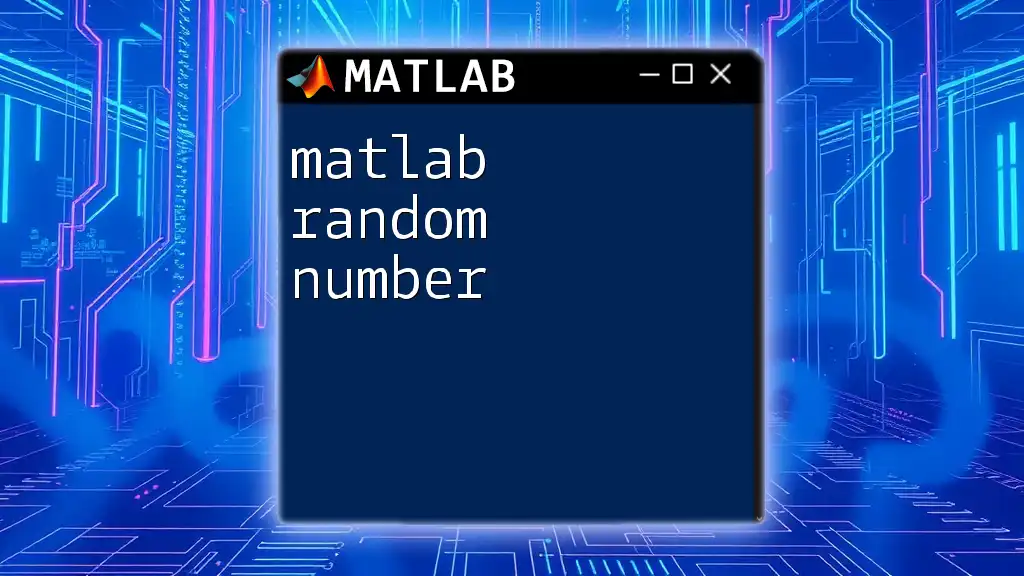
Common Errors and Troubleshooting
When calculating AUC in MATLAB, users may encounter various errors. Common issues include:
- Inconsistent Sizes: Ensure that the vectors for independent and dependent variables are of the same length. MATLAB will throw an error if they do not match.
- NaN Values: If your data contains non-numeric entries or NaN values, consider cleaning the data before processing it. Use the `isnan()` function to identify such values.
- Integration Limits: Ensure that the integration limits are set correctly; any misalignment can lead to inaccurate results.
To prevent and debug errors, carefully review your code and the associated data inputs.
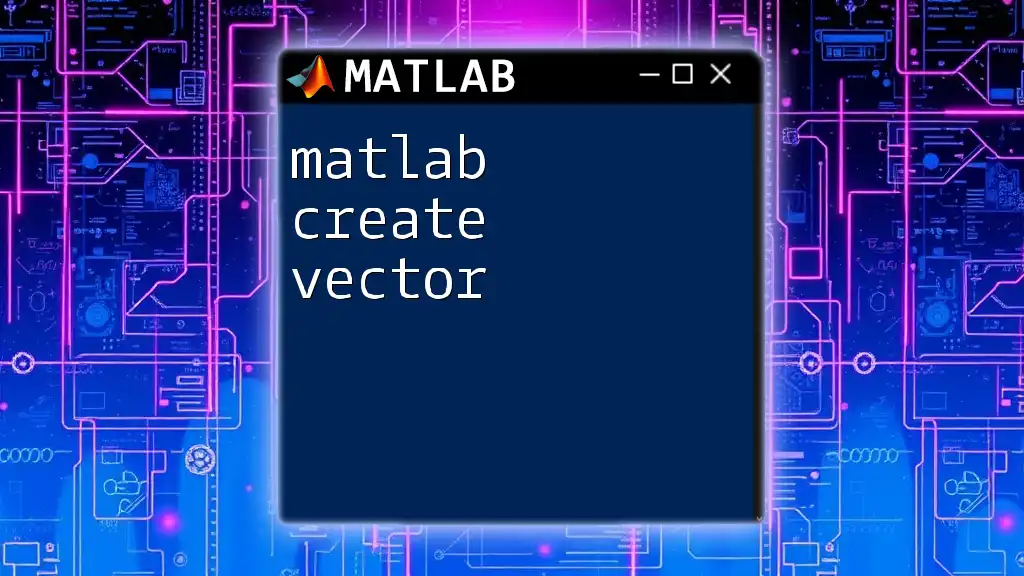
Conclusion
The significance of MATLAB Area Under Curve is profound across numerous fields, enhancing data analysis and model evaluation. By mastering the `trapz()` and `integral()` functions, users can efficiently compute AUC and gain meaningful insights from their data.
Aspiring MATLAB users are encouraged to explore further into MATLAB’s capabilities, delving into additional resources to deepen their understanding and practical skills in numerical analysis and function evaluation.