The MATLAB `mod` function computes the remainder after division of one number by another, returning the result according to the formula `a = b*q + r`, where `r` is the remainder.
Here’s an example code snippet:
% Calculate the remainder of 10 divided by 3
remainder = mod(10, 3);
disp(remainder); % Output will be 1
Understanding Remainders in Mathematics
What is a Remainder?
A remainder is the amount left over after division when one number cannot be exactly divided by another. For instance, when you divide \( 10 \) by \( 3 \), the division results in \( 3 \) with a remainder of \( 1 \). The formula can be expressed as:
\[ \text{Dividend} = (\text{Divisor} \times \text{Quotient}) + \text{Remainder} \]
The significance of the remainder lies in its utility in various mathematical operations, especially in number theory, where it helps in understanding divisibility.
Importance of Remainders in Programming
In programming, remainders play a critical role in a variety of algorithms. For instance, they can help:
- Determine even or odd numbers: By checking if the remainder when divided by \( 2 \) is zero or not.
- Cycle through array indices: It enables you to loop back to the beginning after reaching the end of an array.
- Utilize modular arithmetic in cryptography and hash functions.
The concepts of remainders form the backbone of many computational tasks, making them essential for any programmer, especially those dealing with numerical calculations.
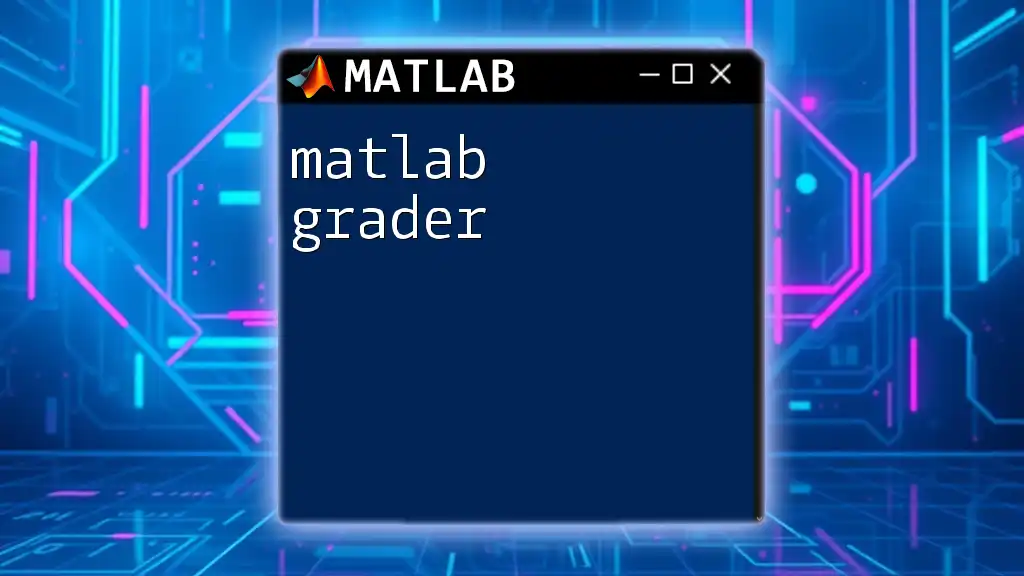
MATLAB Basics
Introduction to MATLAB
MATLAB is a high-performance language primarily used for technical computing, which includes analysis, visualization, and programming. Its ability to handle matrix operations and complex mathematical functions makes it a popular choice for scientists and engineers who need to manipulate numerical data.
Working with Basic Syntax
MATLAB's syntax is user-friendly, making commands straightforward to enter and execute. For instance, assignment can be done simply with:
a = 5;
b = 10;
This straightforward approach allows for rapid prototyping of mathematical ideas, especially when working with operations like obtaining remainders.
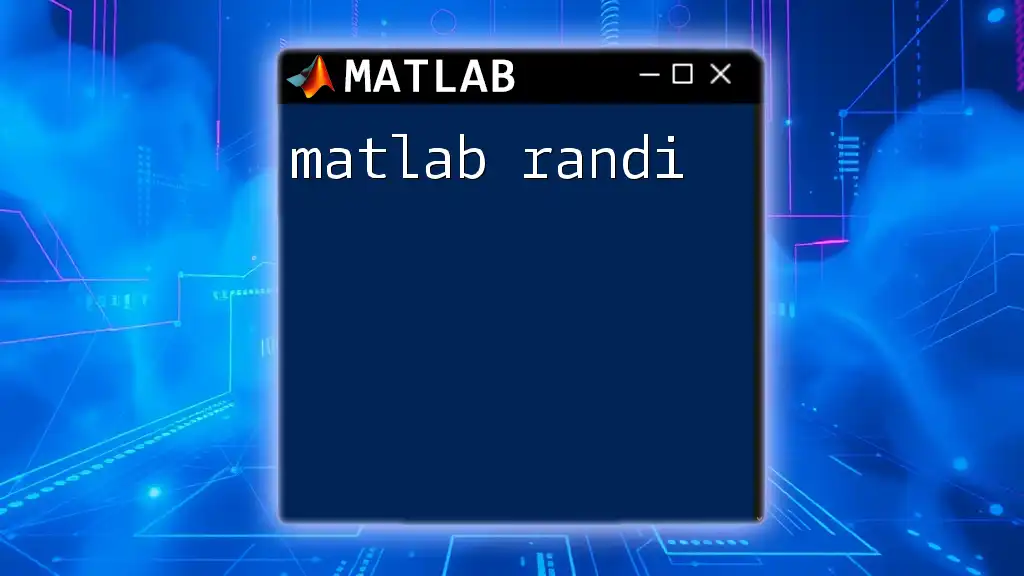
The Remainder Function in MATLAB
The `mod` Function
The `mod` function in MATLAB is specifically used to calculate the remainder after division. Its syntax is structured as follows:
y = mod(x, m)
Where \( x \) is the dividend, and \( m \) is the divisor.
Example Code Snippet:
x = 10;
m = 3;
remainder = mod(x, m); % This returns 1
fprintf("Remainder of %d divided by %d is %d\n", x, m, remainder);
In this snippet, when \( 10 \) is divided by \( 3 \), the output confirms that the remainder is indeed \( 1 \).
The `rem` Function
Conversely, MATLAB also provides another function called `rem`, which serves a similar purpose but behaves slightly differently, especially with negative numbers. The syntax is:
y = rem(x, m)
Example Code Snippet:
x = -10;
m = 3;
remainder = rem(x, m); % This returns -1
fprintf("Remainder of %d divided by %d using rem is %d\n", x, m, remainder);
In this case, \( -10 \) divided by \( 3 \) results in a different remainder of \( -1 \), showcasing how `rem` treats negative dividends differently than `mod`.
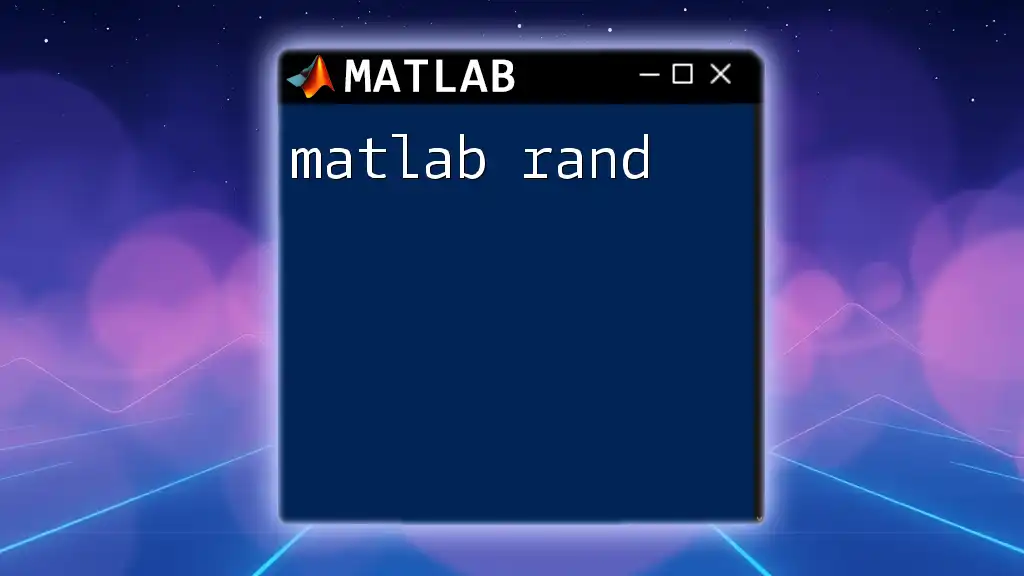
Comparing `mod` and `rem`
Key Differences
One key distinction between `mod` and `rem` is their handling of negative values:
- `mod` always returns a non-negative remainder when the divisor is positive.
- `rem` may return a negative remainder when the dividend is negative.
This difference can significantly affect the outcomes in programs where signed numbers are involved.
When to Use Which?
Choosing the appropriate function depends on the specific requirements of your operation:
- Use `mod` when you require a non-negative result, such as in positional calculations or cyclic applications.
- Use `rem` when the sign of the answer is important, such as in mathematical contexts that require a consistent treatment of negative numbers.
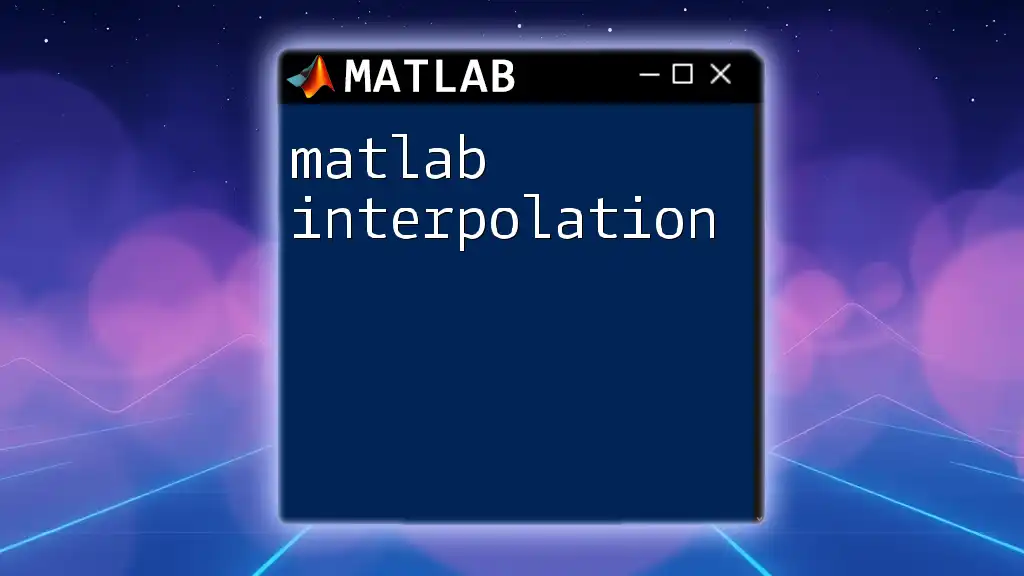
Practical Examples of Remainder Calculations
Simple Division Examples
Let's dive into some simple calculations to illustrate both functions:
% Example for positive integers
x1 = 42;
m1 = 5;
fprintf("mod(%d, %d) = %d\n", x1, m1, mod(x1, m1)); % Output: 2
fprintf("rem(%d, %d) = %d\n", x1, m1, rem(x1, m1)); % Output: 2
Here, both functions produce the same result for non-negative inputs. This suggests that the choice of method may not matter in this context.
Remainders with Arrays
MATLAB excels in handling arrays, allowing users to apply functions across multiple values seamlessly:
A = [10, 20, 30, 40];
m = 6;
remainder_mod = mod(A, m);
fprintf("Remainders using mod: %s\n", mat2str(remainder_mod));
This snippet calculates the remainders of elements in array \( A \) when divided by \( 6 \), enabling a quick overview without needing to loop manually.
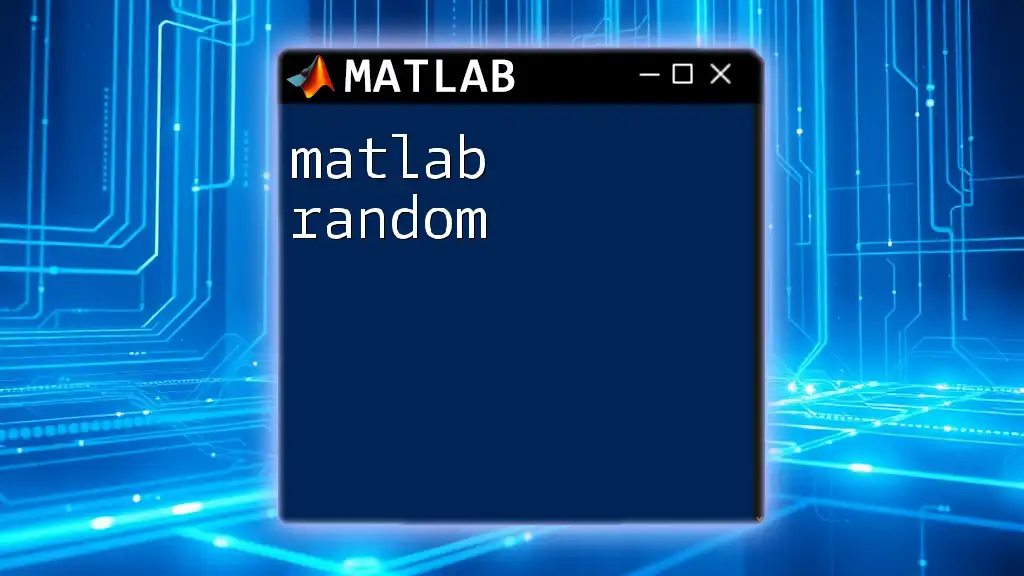
Common Pitfalls and How to Avoid Them
Understanding Edge Cases
Common mistakes arise primarily due to the treatment of negative values. For example, using `mod(-10, 3)` yields \( 2 \) while `rem(-10, 3)` yields \( -1 \). Always be cognizant of how your specific use case interacts with negative numbers to prevent logical errors.
Best Practices in Using `mod` and `rem`
To maintain clarity in code, strive for readability when selecting functions. Use comments to explain your choice if you're handling negative numbers, ensuring your intentions are as clear as possible to anyone reading your code later.
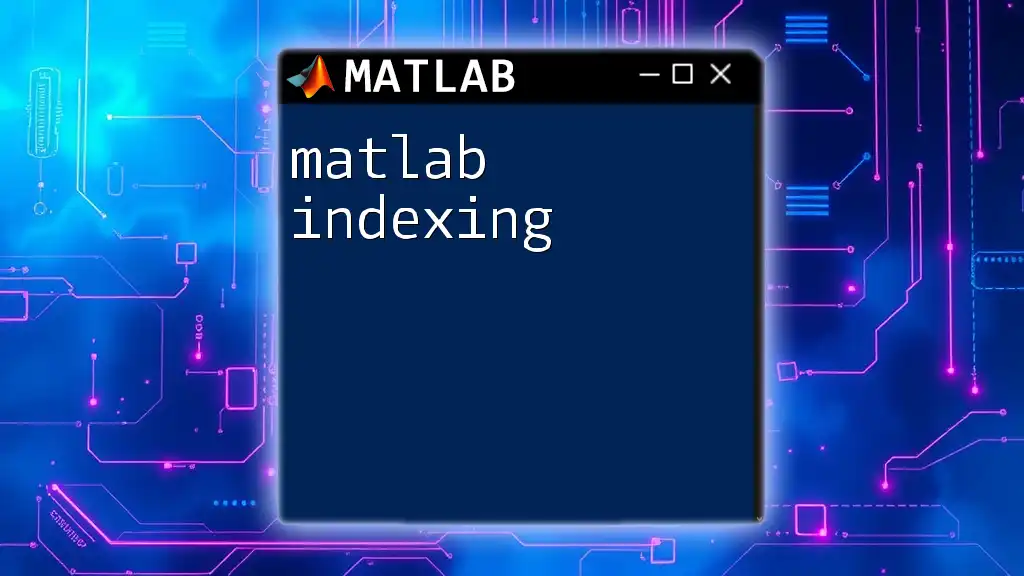
Advanced Applications
Remainders in Algorithms
Remainders are fundamentally important in numerous algorithms:
- Sorting: Remainders can determine positioning during bucket sort.
- Searching: Hash functions often rely on modulus calculations to evenly distribute data for rapid access.
For example, if you're working on a hash table where values are swiftly accessed based on keys, using a simple `mod` operation can greatly enhance efficiency.
Using Remainders in Statistical Analysis
In statistical contexts, remainders can assist in segmenting data. For instance, if you are categorizing event data into bins based on periodic intervals, utilizing modulo operations can streamline the process of assigning each data point to the appropriate bucket.
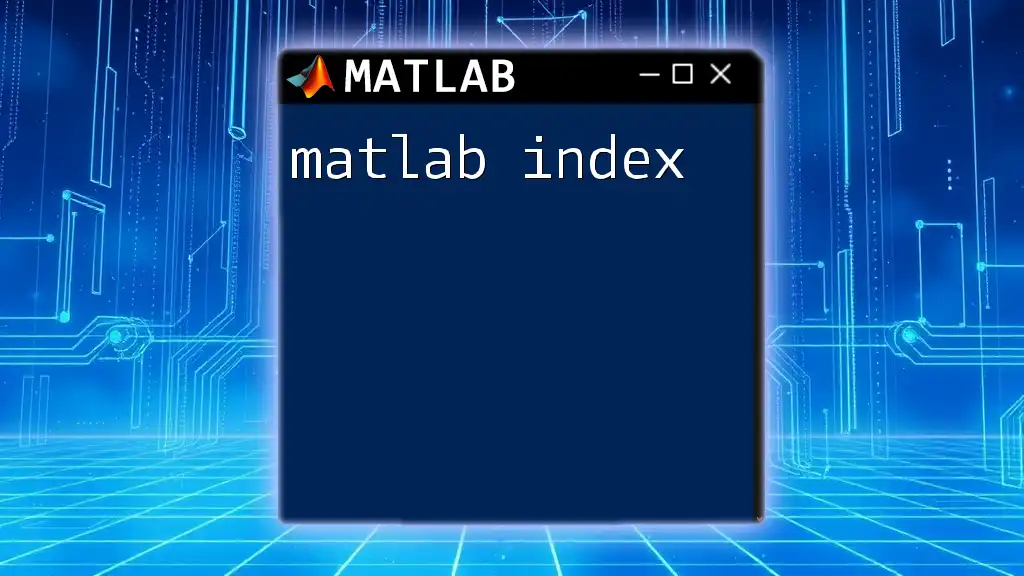
Conclusion
Summary of Key Points
Understanding the MATLAB remainder functions — `mod` and `rem` — is essential for performing reliable calculations. The differences in handling positive and negative numbers can significantly impact results, especially in practical applications.
Further Learning Resources
To deepen your understanding, consider exploring the official MATLAB documentation, online courses, and interactive programming environments where you can practice using the `mod` and `rem` functions in different contexts.
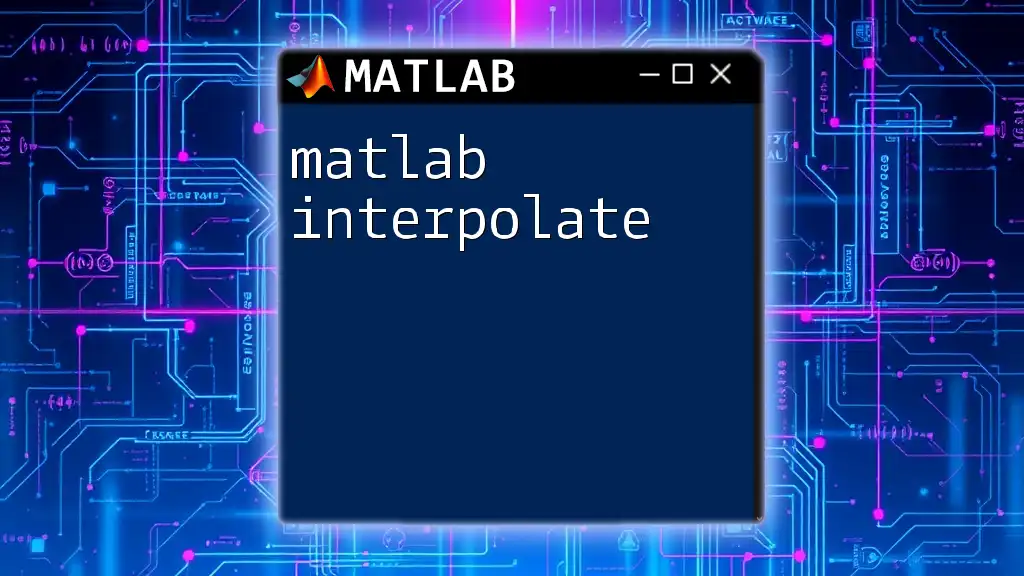
Call to Action
Ready to sharpen your MATLAB skills? Dive into practical exercises using the remainder functions. Experiment with varying inputs and see how these functions behave differently. Your journey to mastering MATLAB begins now!