A MATLAB transfer function is a mathematical representation of the relationship between the input and output of a linear time-invariant system, defined using the transfer function estimation capabilities of MATLAB.
Here’s a code snippet to create a basic transfer function in MATLAB:
% Define numerator and denominator coefficients
num = [1]; % Numerator coefficients
den = [1, 3, 2]; % Denominator coefficients
% Create the transfer function
sys = tf(num, den);
disp(sys); % Display the transfer function
What is a Transfer Function?
A transfer function is a mathematical representation that describes the relationship between the input and output of a linear time-invariant (LTI) system in the Laplace domain. It is expressed as the ratio of the Laplace transform of the output to the Laplace transform of the input, assuming all initial conditions are zero. Transfer functions are crucial in engineering fields, especially in control systems and signal processing, allowing engineers to analyze system dynamics and design controllers effectively.
Applications of Transfer Functions
Transfer functions have a variety of applications across multiple domains:
- Control Systems: They help in analyzing and designing stable control systems by providing insight into how the system behaves under various conditions.
- Signal Processing: Transfer functions are used in filters and digital signal processing to modify signal properties.
- Mechanical Systems: Engineers can predict the response of mechanical structures, like beams or bridges, to dynamic loads.
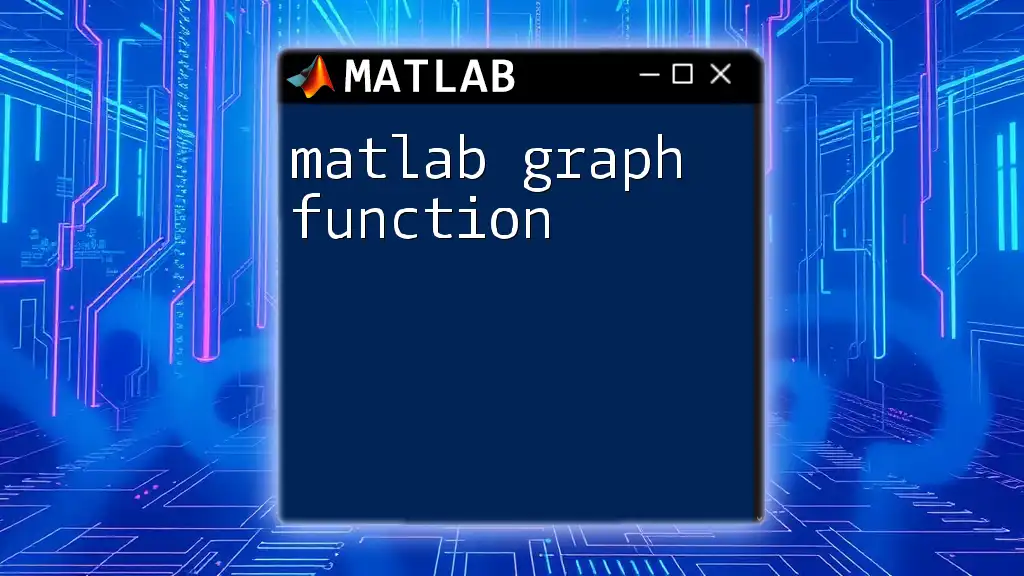
Basics of MATLAB for Control Systems
Getting Started with MATLAB
Before delving into transfer functions, it's essential to understand the MATLAB environment. MATLAB's rich set of functions and tools greatly simplifies the analysis and design of control systems. Its intuitive interface allows users to visualize data and models, making it an ideal choice for engineers.
Key Commands for Control Systems
To work with transfer functions in MATLAB, familiarize yourself with the following commands:
- `tf`: Defines a transfer function.
- `step`: Simulates the step response of the system.
- `impulse`: Simulates the impulse response.
Here’s a basic setup to get started with transfer functions:
% MATLAB Environment Setup
clear; clc; close all;
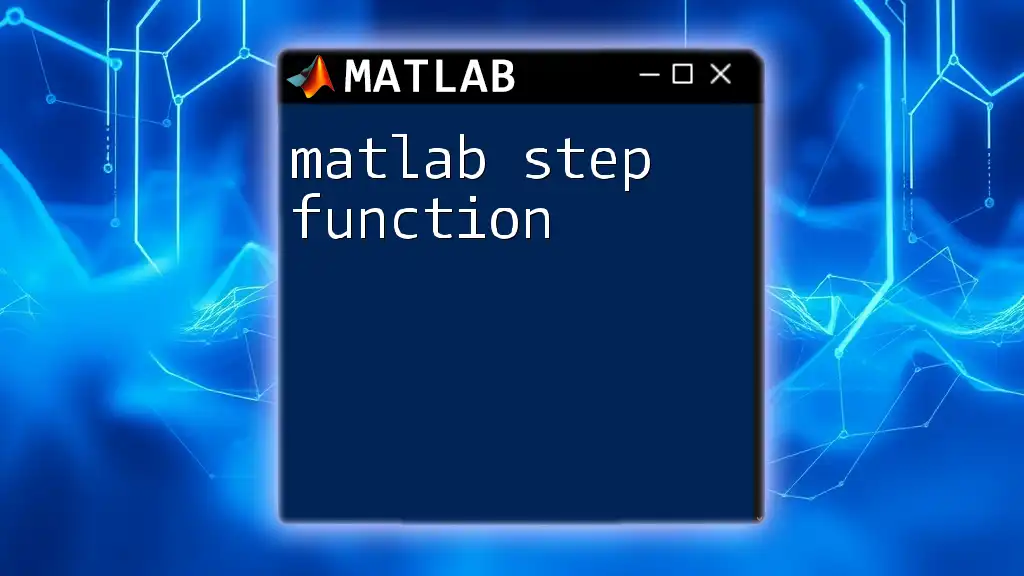
Creating Transfer Functions in MATLAB
Using the `tf` Command
The `tf` command in MATLAB is used to create a transfer function model. The general syntax is as follows:
sys = tf(N, D)
Where `N` is the numerator coefficients and `D` is the denominator coefficients of the transfer function.
Example: Creating a Simple Transfer Function
To define a transfer function \( H(s) = \frac{2s + 3}{s^2 + 4s + 5} \):
N = [2 3]; % Numerator coefficients
D = [1 4 5]; % Denominator coefficients
sys = tf(N, D);
Numerical and Symbolic Transfer Functions
Transfer functions can be expressed numerically using arrays or symbolically using the `syms` command. When you want to derive equations or analyze components on a symbolic level, symbolic representation is beneficial.
Here's how to define a symbolic transfer function:
syms s
H = (2*s + 3) / (s^2 + 4*s + 5);
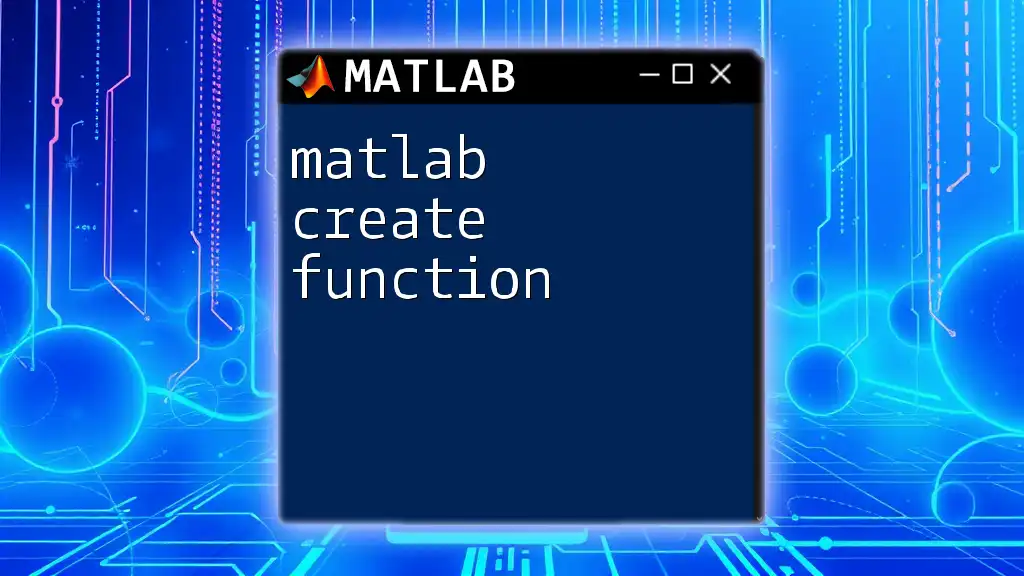
Analyzing Transfer Functions
Time Response Analysis
The time response of a system reflects how the output evolves over time in response to an input signal. In control systems, understanding time response is critical to determine system behavior.
MATLAB Functions for Time Response
You can analyze the time response using:
- `step` for step responses.
- `impulse` for impulse responses.
- `lsim` for simulating an arbitrary input.
Example: Simulating a Step Response
To visualize the step response of the previously defined transfer function:
step(sys);
title('Step Response of the Transfer Function');
Frequency Response Analysis
Frequency response analysis helps understand how a system reacts to sinusoidal inputs of varying frequencies. This is vital for characterizing system stability and performance.
Using Bode, Nyquist, and Nichols Plots
MATLAB provides functions to create frequency response plots, such as:
- Bode Plot: Uses `bode`.
- Nyquist Plot: Uses `nyquist`.
- Nichols Plot: Uses `nichols`.
Example: Generating Bode Plots
To create a Bode plot of the transfer function:
bode(sys);
title('Bode Plot of the Transfer Function');
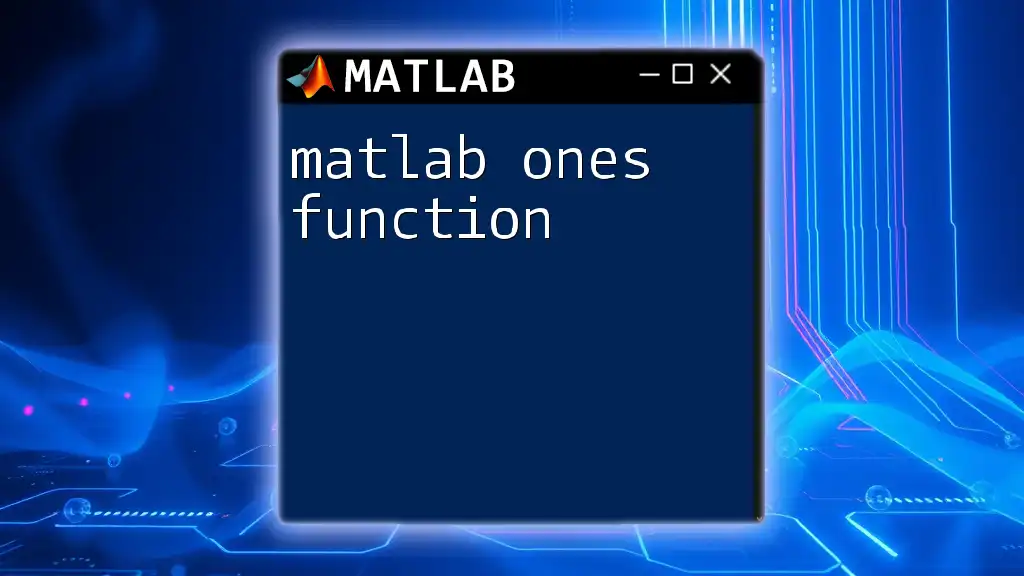
Modifying Transfer Functions
Feedback Systems
In control systems, feedback is essential for achieving desired performance. The `feedback` function in MATLAB allows creating closed-loop systems from open-loop transfer functions.
Example: Creating a Closed-Loop System
K = 1; % Gain
closed_loop_sys = feedback(K*sys, 1);
Cascade and Parallel Systems
Often, systems encounter series (cascade) or parallel configurations. MATLAB provides straightforward means to create these systems through addition and multiplication of transfer functions.
Code Example for Cascade System
sys1 = tf([1], [1 2]); % First Transfer Function
sys2 = tf([1], [1 1]); % Second Transfer Function
cascade_sys = sys1 * sys2; % Cascading
Code Example for Parallel System
parallel_sys = sys1 + sys2; % Parallel Combination
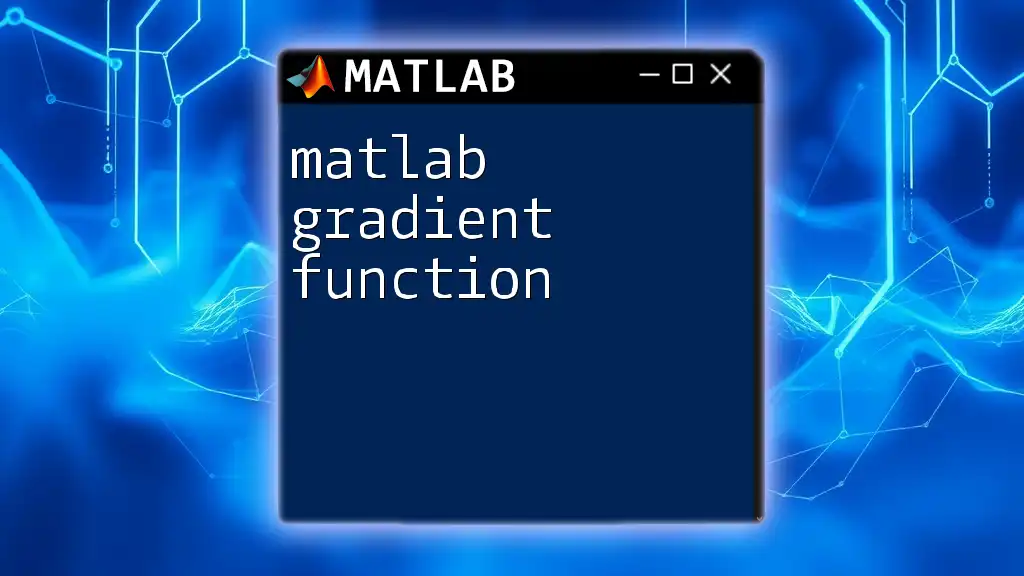
Stability Analysis of Transfer Functions
Understanding System Stability
Stability in control systems refers to the ability of a system to return to a steady state after a disturbance. Stability can be determined using methods such as the Routh-Hurwitz criterion or Nyquist stability criterion.
Applying Stability Criteria in MATLAB
To assess the stability of a transfer function, you can use:
- `pole`: Returns the pole locations.
- `zero`: Returns the zero locations.
- `margin`: Computes gain and phase margins.
Example: Checking for Stability
poles = pole(sys);
disp('Pole Locations:');
disp(poles);
If any poles have a positive real part, the system is unstable.
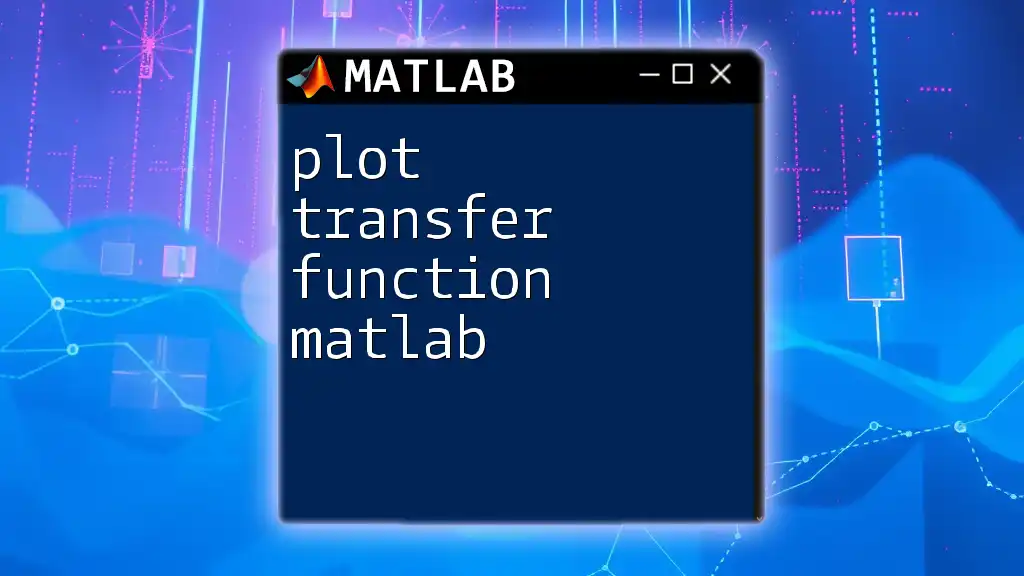
Designing Controllers with Transfer Functions
PID Controllers and Transfer Functions
PID controllers are widely used in industrial control systems. They combine proportional, integral, and derivative control actions to enhance system performance.
Implementing Controllers in MATLAB
The `pid` command in MATLAB allows users to model a PID controller conveniently. Here's an example of designing a simple PID controller:
Kp = 2; Ki = 1; Kd = 0.5; % PID parameters
pid_controller = pid(Kp, Ki, Kd);
closed_loop_pid_sys = feedback(pid_controller * sys, 1);
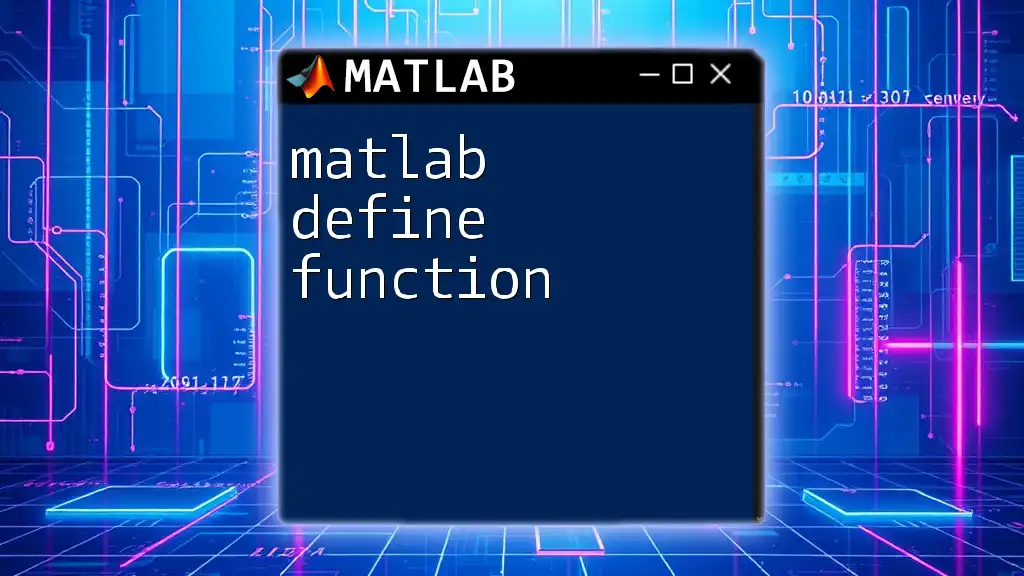
Case Studies and Practical Examples
Real-World Case Study
Suppose we analyze a temperature control system using a given transfer function. By applying step and impulse responses in MATLAB, we derive system behavior insights that can inform design improvements and operational adjustments.
Common Pitfalls and Best Practices
When using MATLAB transfer functions, avoid the following common mistakes:
- Forgetting to define all coefficients: Ensure that all numerator and denominator coefficients are included.
- Neglecting time-domain analysis: Understanding both time and frequency responses is crucial for system performance.
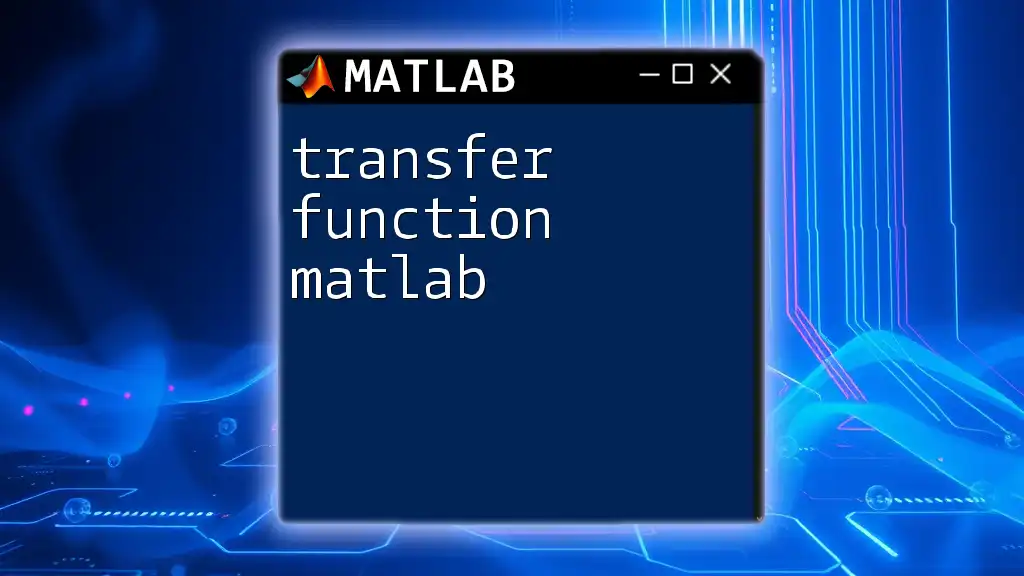
Conclusion
MATLAB transfer functions are a powerful tool for engineers and scientists in designing and analyzing dynamic systems. By understanding transfer functions and employing MATLAB effectively, users can enhance system performance and stability. Emphasizing continuous learning and practice will pave the way for mastering control systems and MATLAB applications.
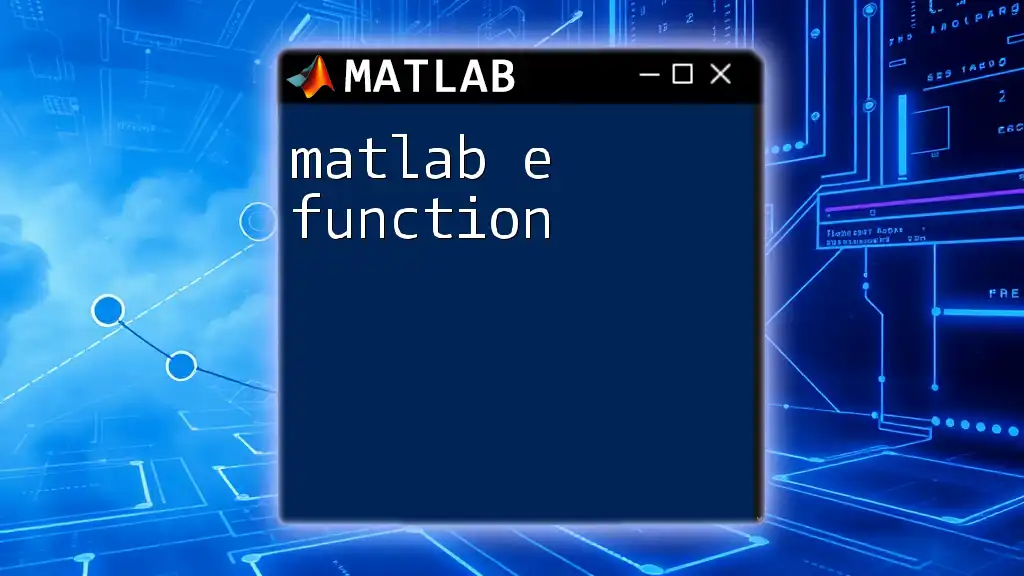
Additional Resources
Recommended Reading
Explore textbooks and online courses that specifically focus on control systems and MATLAB applications. This reading will deepen your knowledge and skills beyond the basics.
Community and Support
Engaging with online forums and user communities can provide insights, troubleshooting support, and collaborative learning opportunities as you continue your MATLAB journey.