In MATLAB, you can create a table to store data in a structured format using the `table` function, which allows for easy manipulation and analysis of related variables.
% Creating a table with sample data
Names = {'Alice'; 'Bob'; 'Charlie'};
Ages = [25; 30; 35];
Scores = [90; 85; 88];
T = table(Names, Ages, Scores);
Understanding Tables in MATLAB
What is a Table?
In MATLAB, a table is a data type that is specifically designed for managing and analyzing heterogeneous data. Unlike traditional data structures, such as arrays or cell arrays, tables allow you to work with variables that may contain different types of data (e.g., numeric, text, categorical). This makes tables particularly useful in data analysis and data visualization tasks where you often deal with mixed data types.
Key benefits of using tables include:
- Ease of data handling: Organize data in columns named after the variables, making your code easier to read and understand.
- Enhanced data manipulation: MATLAB provides numerous built-in functions for statistical analysis, filtering, and data exploration that work seamlessly with tables.
- Integrative data storage: Easy integration with visualization functions, allowing for rapid analysis and presentation of results.
Structure of a Table
Understanding the structure of a table is essential when you perform data manipulation.
Columns and Rows: Each table consists of rows and columns, with rows representing observations and columns representing variables. This tabular format makes data representation clear and straightforward.
Variable Names: It’s important to use consistent and meaningful names for your table’s columns. For example, a table containing data about students could have columns named `Name`, `Age`, and `Grade`.
Data Types: MATLAB tables support various data types, making them extremely flexible. You can store:
- Numeric data (e.g., integers and floats)
- Text data (character arrays or strings)
- Categorical data (e.g., limited set of values)
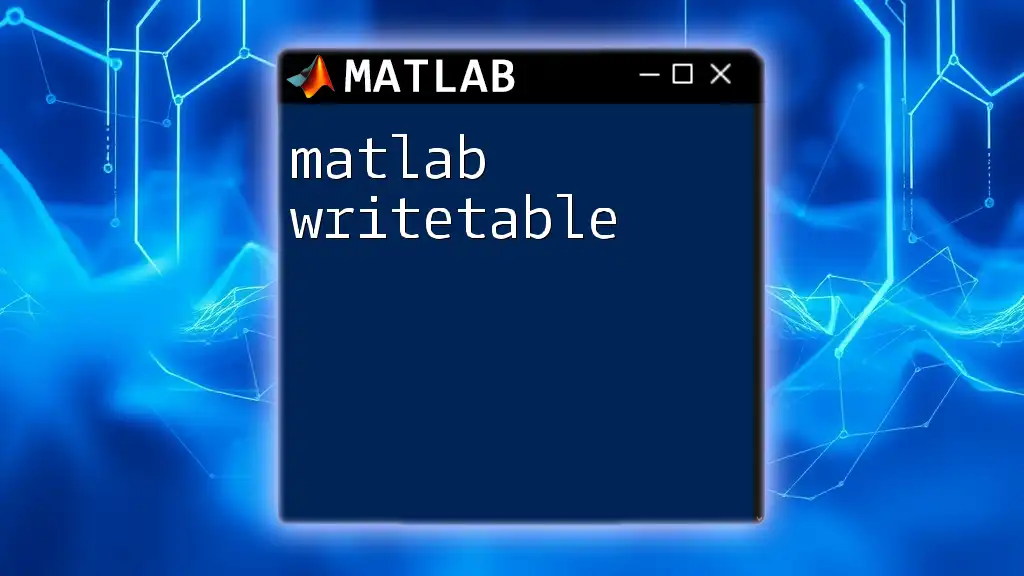
Creating a Table
Using the `table` Function
The primary way to create a table in MATLAB is by using the `table` function. Its syntax is intuitive and allows you to create tables quickly.
Here’s a simple example of creating a table:
% Create a basic table
names = {'John'; 'Alice'; 'Bob'};
ages = [28; 24; 30];
heights = [5.9; 5.5; 6.0];
myTable = table(names, ages, heights);
disp(myTable);
This code snippet creates a table named `myTable` with three columns: `names`, `ages`, and `heights`. You'll notice how the `table` function takes vectors as input and constructs the specification automatically.
Specifying Row and Column Names
To further enhance the clarity of your data, you can assign custom row and column names.
Setting Row Names: You can specify row names like this:
rowNames = {'Person 1'; 'Person 2'; 'Person 3'};
myTable.Properties.RowNames = rowNames;
This assigns clear labels for each row, making your output more informative.
Setting Column Names: You can also specify column names when creating your table:
myTable = table(names, ages, heights, 'VariableNames', {'Name', 'Age', 'Height'});
In this example, the columns of `myTable` are now clearly labeled as `Name`, `Age`, and `Height`, promoting better readability in your dataset.
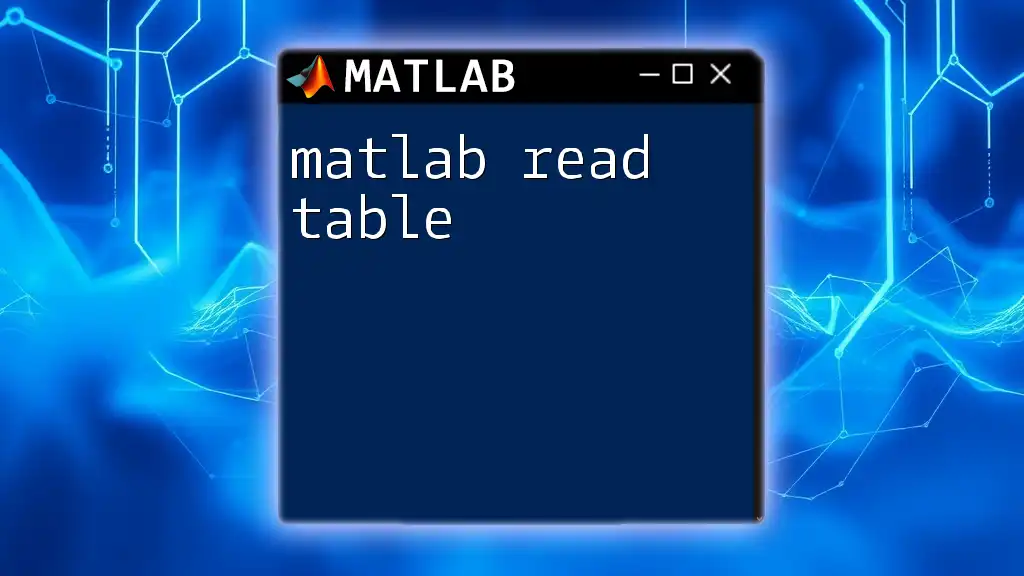
Modifying Tables
Adding New Variables
As your analysis progresses, you may need to add new columns to your table. For instance, if you wanted to include a weight for each person, you could do it seamlessly:
weights = [150; 135; 180];
myTable.Weight = weights;
Removing Variables
If you find that a certain variable is no longer necessary, removing it is straightforward. For example, to delete the `Weight` column from `myTable`, you can simply use:
myTable.Weight = []; % Remove the 'Weight' column
Modifying Existing Data
Access and modify data effortlessly. For example, if you need to update John's age, the following command can be utilized:
myTable.Age(1) = 29; % Change John's age
This manipulates the table directly, allowing for real-time updates.
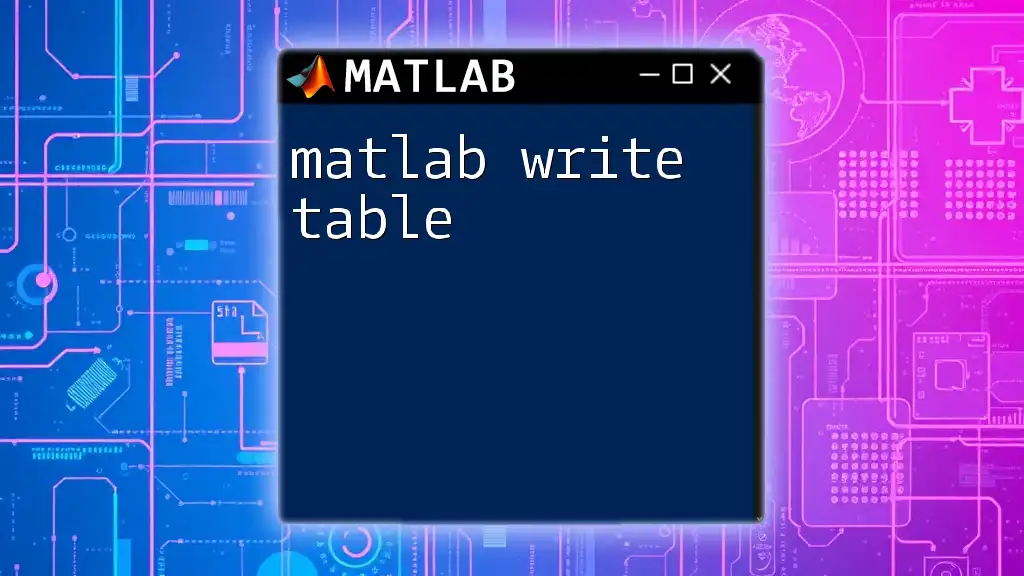
Accessing Data in Tables
Indexing with Row and Column Names
Accessing specific data in a table is made easy with named indexing. For example, to retrieve Alice's age, you can do so with:
ageOfAlice = myTable.Age(2); % Accessing Alice's age
This clear indexing strategy enhances the usability and readability of your code.
Logical Indexing
MATLAB provides powerful ways to filter data based on conditions. For instance, if you need to extract only the rows where individuals are adults (aged 21 and older), you can achieve this with logical indexing:
adultsTable = myTable(myTable.Age >= 21, :); % Filter adults
This command creates a new table, `adultsTable`, that only contains the rows meeting the specified criteria.
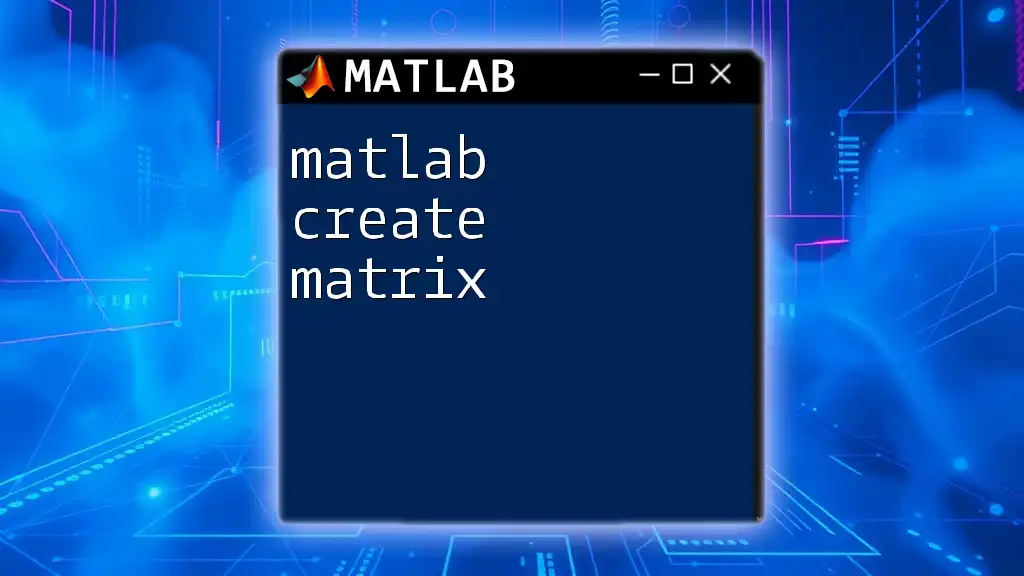
Useful Table Functions
Summary and Statistics
When working with tables, you might often want a quick overview of your data. For this, MATLAB provides the `summary()` function, which gives you insightful statistics about the variables present in your table.
Additionally, you can calculate averages or other statistical measures easily. For example, to find the mean age of individuals in `myTable`, you can use:
meanAge = mean(myTable.Age);
Joining Tables
Combining multiple tables can enhance your data analysis. MATLAB offers various functions such as `innerjoin` and `outerjoin` for this purpose. Here’s an example demonstrating how you can join tables:
otherTable = table({'John'; 'Bob'}, [1; 2], 'VariableNames', {'Name', 'ID'});
joinedTable = innerjoin(myTable, otherTable, 'Keys', 'Name');
This snippet creates a new table, `joinedTable`, resulting from an inner join between `myTable` and `otherTable`, merging them based on the `Name` key.
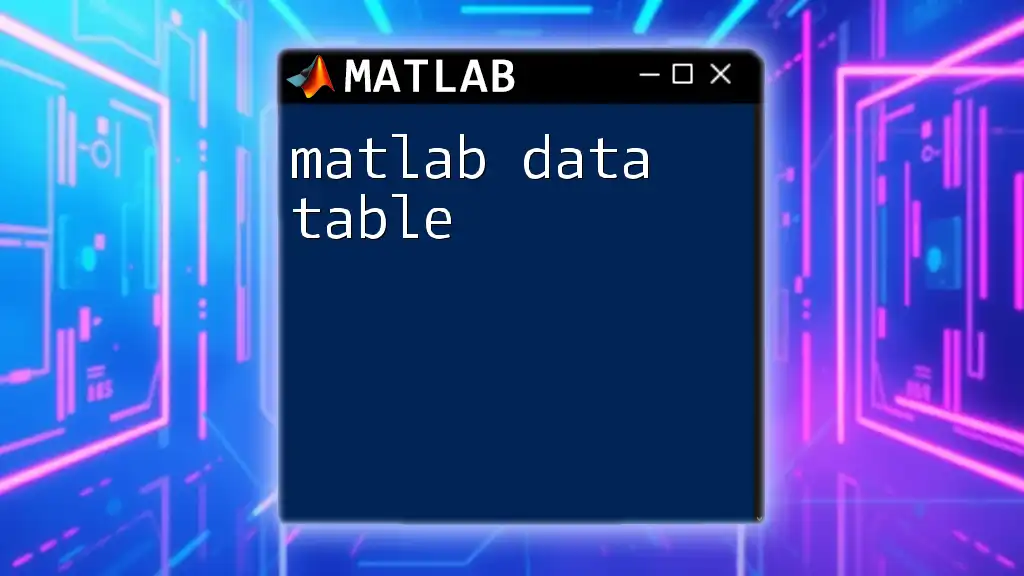
Conclusion
In this comprehensive guide on how to matlab create table, we explored the definition, structure, and various functionalities of tables in MATLAB.
From creating and modifying tables to accessing and filtering data, you've seen how tables simplify data management tasks, making them an indispensable tool for any MATLAB user. With a wealth of built-in functions and a clear indexing system, leveraging tables enhances your efficiency in data analysis significantly.
As you continue to explore the functionalities of MATLAB tables, I encourage you to try the examples provided and build your own data manipulations. With practice, you'll become increasingly adept at navigating and utilizing tables for your specific analytical needs.
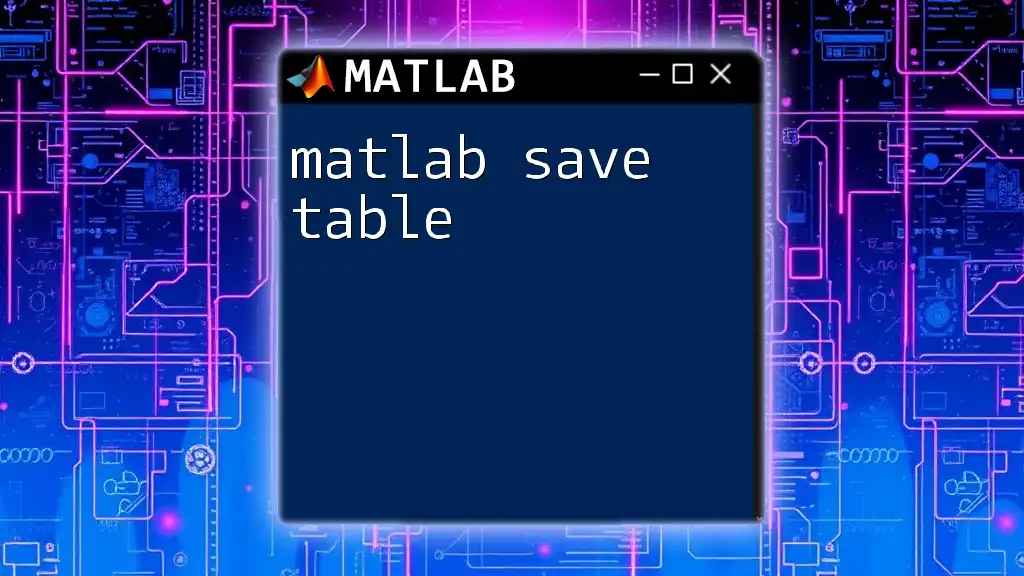
Additional Resources
For those seeking further insights and practices, make sure to explore the official MATLAB documentation on tables, which offers extensive guidance and applications. Additionally, consider tackling suggested exercises for hands-on experience to solidify your understanding of data structures in MATLAB.
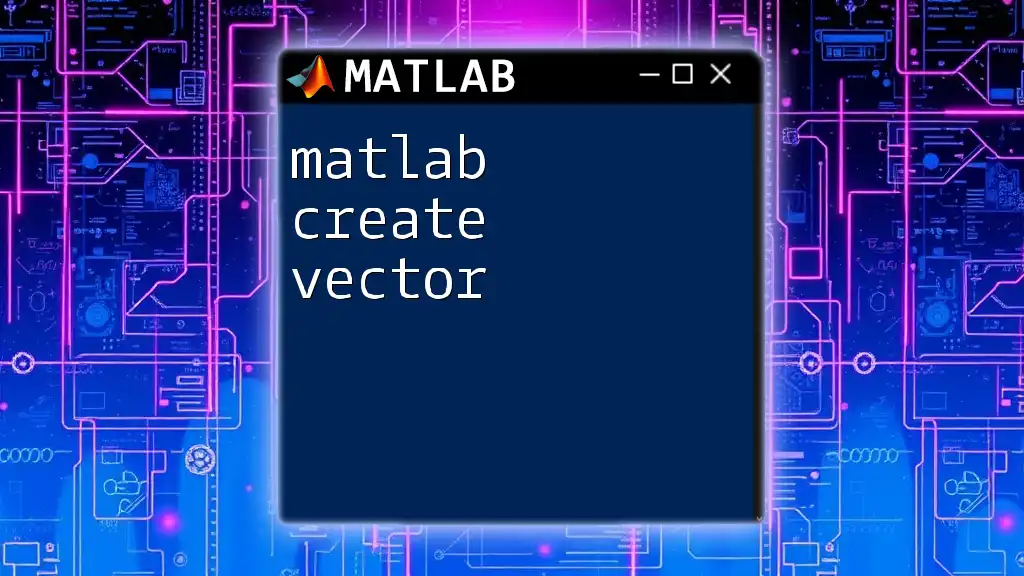
FAQs
Common Questions Regarding MATLAB Tables
-
What are the advantages of using tables in MATLAB?
Tables provide an intuitive structure for managing heterogeneous data, allowing for easy manipulation and analysis. -
How can I convert a table to another data type?
You can convert tables to arrays using the `table2array` function for numeric data or various other methods based on your requirements. -
Can tables hold mixed data types?
Yes, tables can store a variety of data types across different columns, including numbers, text, and categorical data, making them highly versatile.