In MATLAB, array indices are numerical values used to access or modify specific elements within an array, where indexing starts from 1 rather than 0, as seen in many other programming languages.
Here’s a simple example of how to use array indices:
% Create an array
A = [10, 20, 30, 40, 50];
% Access the third element
thirdElement = A(3); % Output will be 30
% Modify the second element
A(2) = 25; % A is now [10, 25, 30, 40, 50]
Understanding MATLAB Array Indices
What Are Array Indices?
Array indices in MATLAB are critical as they allow users to access and manipulate specific elements within arrays. Understanding how to work with these indices is essential for effective data manipulation, as it forms the foundation of matrix operations and programming in MATLAB.
Basic Concepts of MATLAB Arrays
Before diving deeper into array indices, it’s important to understand the types of arrays available in MATLAB. MATLAB primarily deals with:
- 1D Arrays: Also known as vectors, these are linear collections of values.
- 2D Arrays: These are essentially matrices with rows and columns.
- ND Arrays: Multi-dimensional arrays that can have three or more dimensions.
- Cell Arrays: Special types of arrays that can contain different types of elements.
- Structures: A way to store data with different types, including arrays.
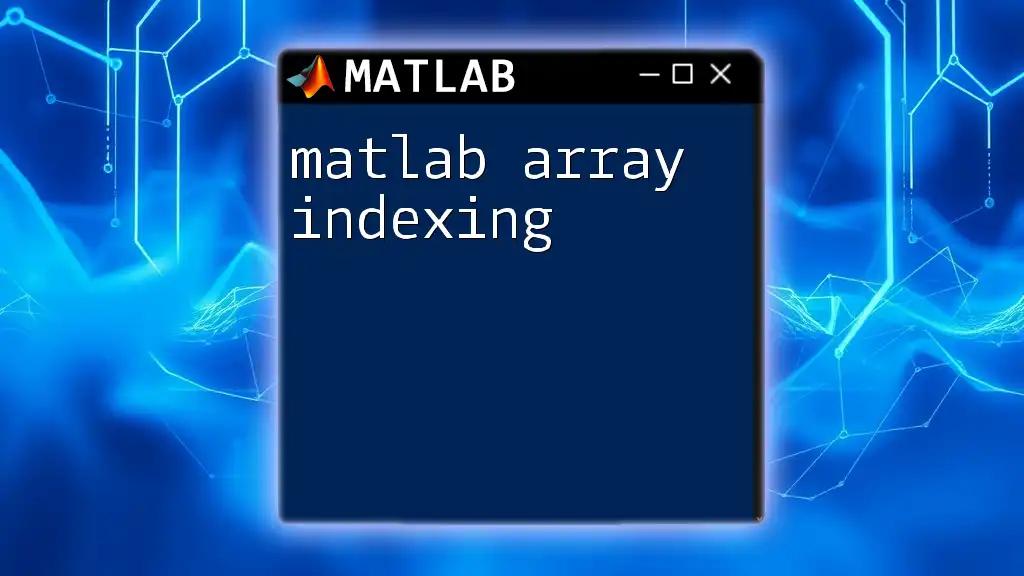
Accessing Array Elements
Single Element Access
Accessing a single element in a MATLAB array is a straightforward process. You simply specify the index of the element you want to retrieve. For example, if you have a 1D array `A`, accessing the third element would look like this:
A = [10, 20, 30, 40];
element = A(3); % Accessing the third element
In this example, `element` would hold the value `30`. It's essential to remember that MATLAB uses 1-based indexing, meaning that the first element is at index 1.
Accessing Multiple Elements
You can also access multiple elements from an array by providing a vector of indices. This capability is particularly useful when you need to select specific elements. For instance, consider the following example:
B = [5, 10, 15, 20, 25];
selectedElements = B([1, 4, 5]); % Accessing 1st, 4th, and 5th elements
Here, `selectedElements` will contain the values `[5, 20, 25]`.
Accessing Elements in a Two-Dimensional Array
When working with two-dimensional arrays, you need to specify both the row and column indices. For example, if you wanted to access the element at row 2, column 3 in a 2D array `C`, you would do it this way:
C = [1, 2, 3; 4, 5, 6; 7, 8, 9];
element = C(2, 3); % Accessing element at row 2, column 3
In this instance, `element` would be `6`, which is located at the intersection of the second row and third column.
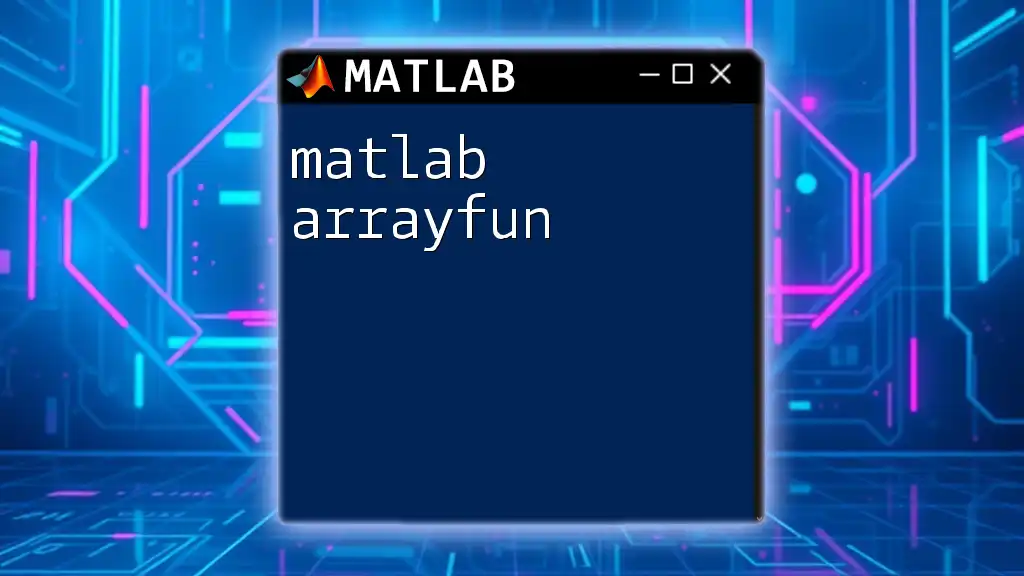
Advanced Indexing Techniques
Logical Indexing
One more powerful feature of MATLAB is logical indexing, where you create a logical array to index another array. This allows you to filter elements based on certain conditions. Here’s how you can achieve this:
D = [1, 2, 3, 4, 5];
logicalIndex = D > 3; % Creating a logical index
selectedElements = D(logicalIndex); % Selecting elements greater than 3
In this example, `selectedElements` would contain `[4, 5]`.
Linear Indexing
Linear indexing allows users to reference elements in a matrix as if they were in a one-dimensional array, using a single index that counts through the elements in column-major order. For instance:
E = [10, 20; 30, 40; 50, 60];
linearElement = E(5); % Accessing element by linear index
This command accesses the value `50`, which is the fifth element in the matrix when counted column by column.
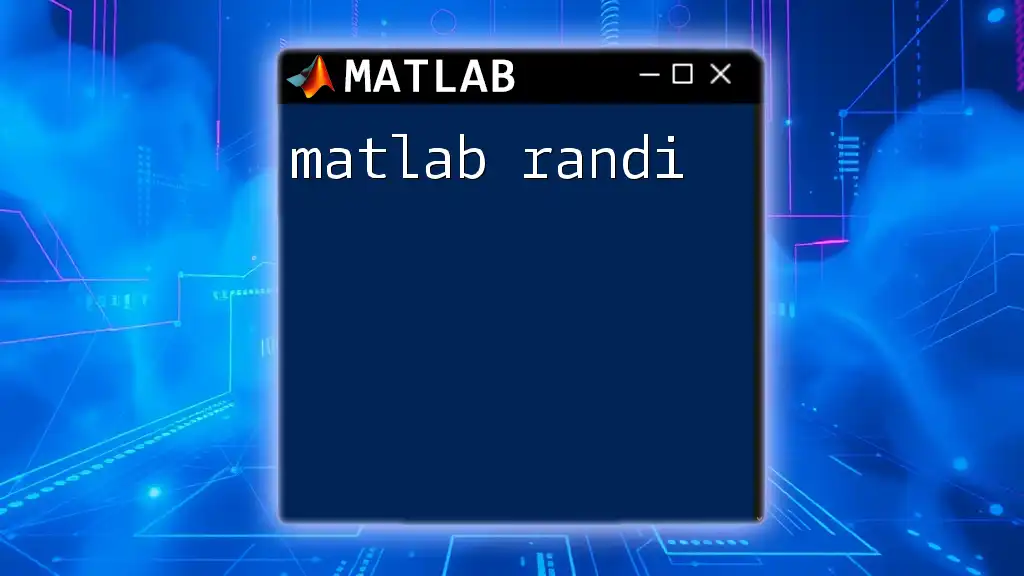
Modifying Array Elements
Changing Element Values
Once you have accessed an element, you can also modify its value. This is particularly straightforward. Here’s an example where you change an element in a matrix:
F = [1, 2; 3, 4];
F(2, 1) = 99; % Changing the value at row 2, column 1
Now, `F` will be updated to:
[1, 2;
99, 4]
Adding and Removing Elements
You can manage array size dynamically in MATLAB. For instance, if you want to append an element to an array, you can do so with:
G = [1, 2, 3];
G(end + 1) = 4; % Appending 4 to the end of the array
After executing this code, `G` will have the value `[1, 2, 3, 4]`.
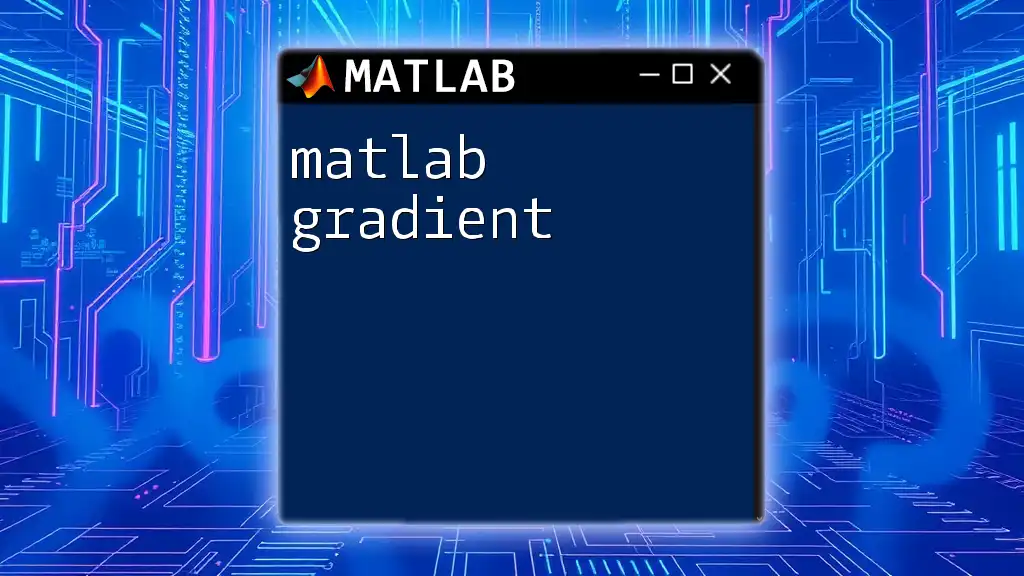
Array Slicing
Understanding Array Slicing
Array slicing allows you to extract a specific section of an array or matrix. For example, if you wish to extract a subset from a 1D array `H`, you can do it as follows:
H = [1, 2, 3, 4, 5];
slicedArray = H(2:4); % Extracting elements from index 2 to 4
After this operation, `slicedArray` will contain `[2, 3, 4]`.
Multi-Dimensional Slicing
You can also slice multi-dimensional arrays. For example, to extract rows and columns from a 2D matrix, you can use:
I = [1, 2, 3; 4, 5, 6; 7, 8, 9];
subMatrix = I(1:2, 2:3); % Extracting a sub-matrix
This results in `subMatrix` holding the values:
[2, 3;
5, 6]
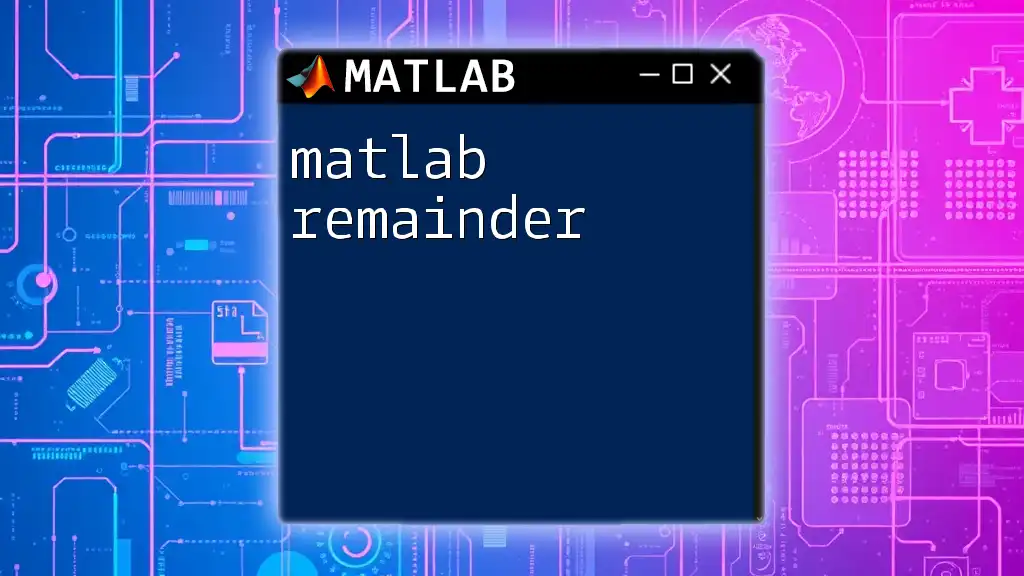
Special Indexing Techniques
Using the `end` Keyword
The `end` keyword in MATLAB is a useful feature for referencing the last element of an array. For example, if you want to access the last element in an array `J`, you can do:
J = [3, 6, 9, 12];
lastElement = J(end); % Accessing the last element
Here, `lastElement` will hold the value `12`, the last element of the array.
Indexing with `find` Function
The `find` function provides another dimension for indexing, helping you locate indices that meet specific conditions. For instance, to find indices of all non-zero elements in an array `K`, you could do:
K = [0, 2, 3, 0, 5];
indices = find(K); % Finding non-zero indices
The `indices` variable will contain `[2, 3, 5]`, indicating the positions of the non-zero elements in the array.
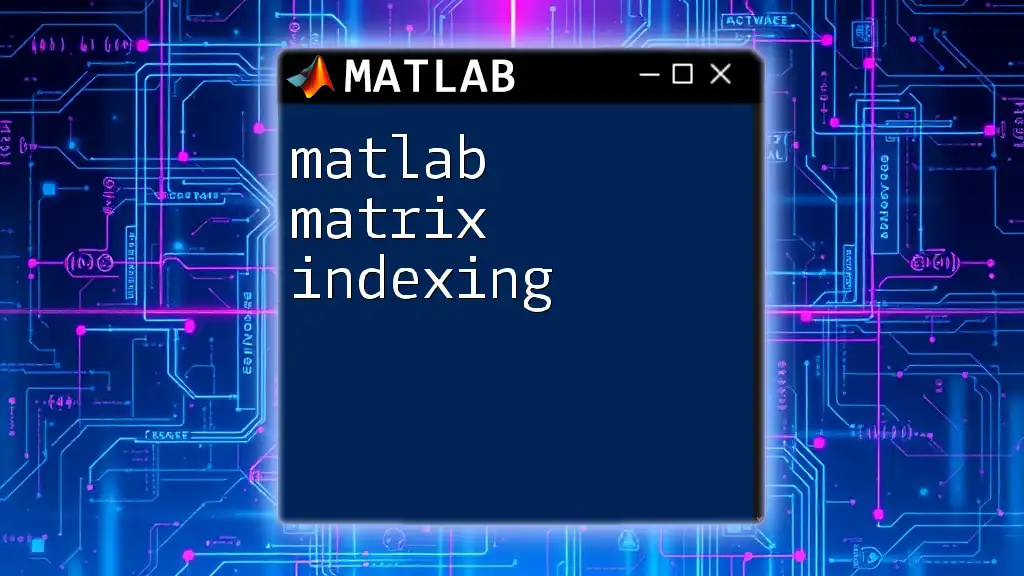
Best Practices for Using Array Indices
Common Pitfalls to Avoid
When working with MATLAB array indices, avoid common mistakes such as:
- Forgetting that MATLAB uses 1-based indexing (not 0-based).
- Miscounting when using ranges, which can lead to off-by-one errors.
- Not checking the dimensions of arrays before trying to index them can lead to runtime errors.
Optimizing Performance
For large datasets, efficient indexing can significantly speed up your code. Here are some best practices:
- Preallocate arrays before filling them to improve performance.
- Utilize vectorized operations instead of loops when possible.
- Make use of logical indexing and built-in functions which are typically optimized.
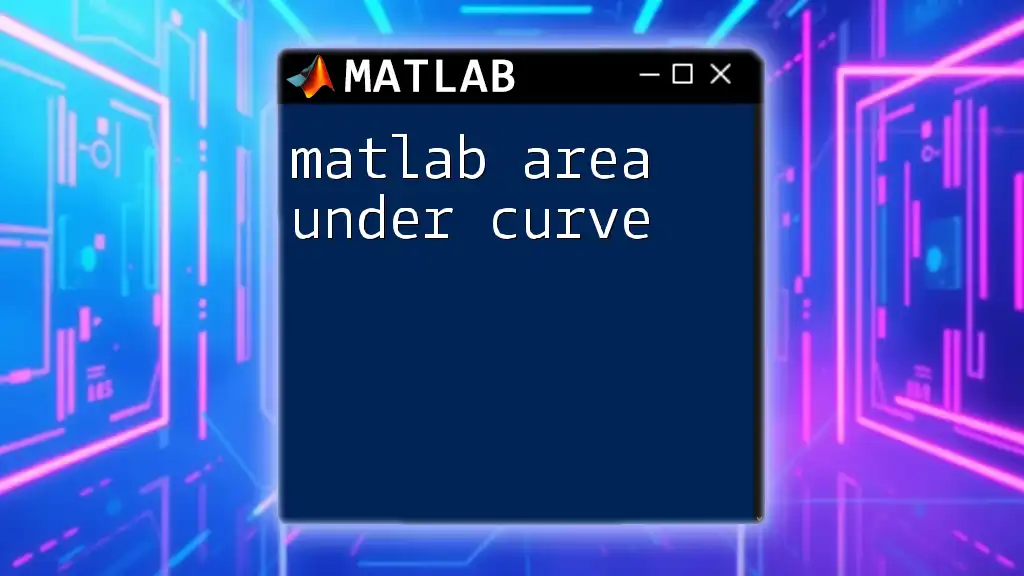
Conclusion
Understanding MATLAB array indices is foundational for anyone working in this powerful programming language. With clear knowledge of how to access, modify, and manage elements of arrays, you can extend your capability significantly in data manipulation and programming. Experiment with the techniques discussed, and you will soon become adept at using MATLAB array indices effectively.
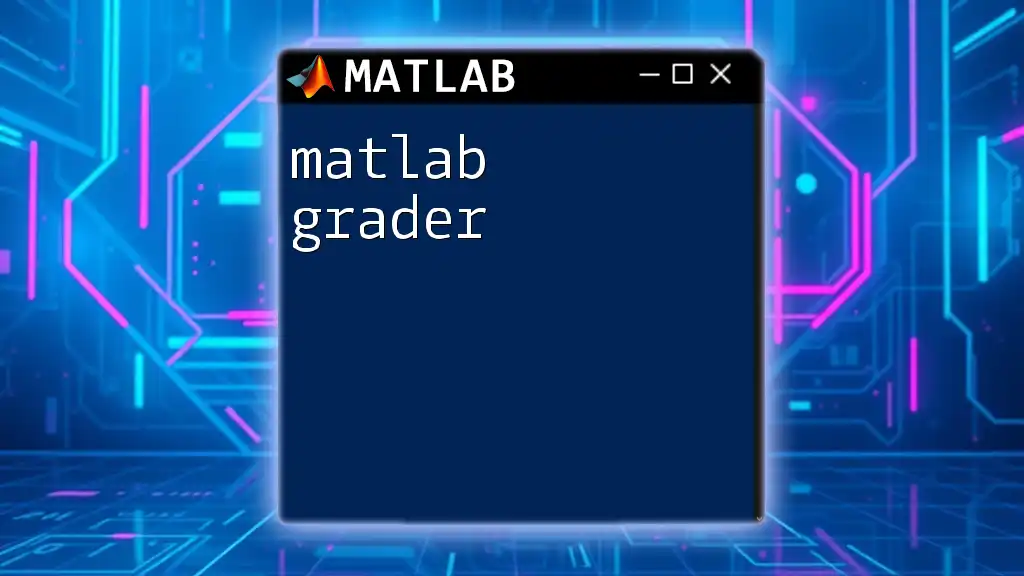
Additional Resources
For further learning, refer to the official MATLAB documentation, which offers extensive tutorials and examples. Additionally, consider exploring reputable books and online courses that focus on MATLAB programming and array manipulation.
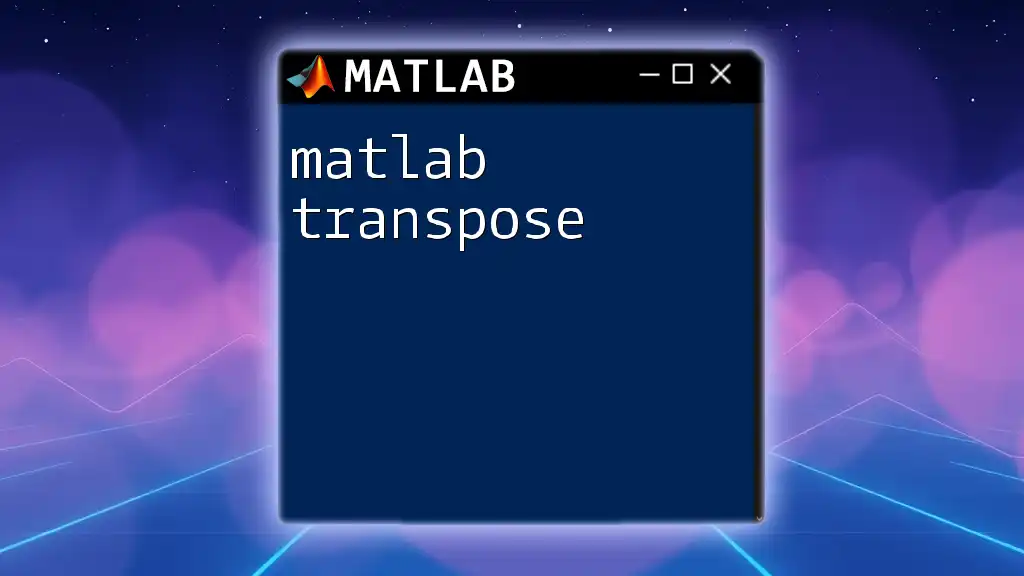
Call to Action
Stay tuned for more posts focused on MATLAB commands and tips, designed to enhance your programming skills and efficiency in using MATLAB!