In MATLAB, you can generate random numbers using the `rand`, `randi`, or `randn` functions, which provide uniformly distributed values, uniformly distributed integers, and normally distributed values, respectively.
Here’s a brief example of how to generate a random number between 0 and 1:
randNumber = rand; % Generates a random number between 0 and 1
Understanding Random Number Generation
What is Random Number Generation?
Random number generation is the process of producing a sequence of numbers that lack any predictable pattern. In programming and simulations, these numbers are critical for tasks ranging from statistical sampling to creating realistic models. In the context of MATLAB, random numbers play an essential role in various applications, including simulations, data analysis, and algorithm testing.
Types of Random Number Generators
In MATLAB, random numbers are generated using pseudorandom number generators (PRNGs). This means that the numbers are generated in a deterministic manner based on an initial seed value. While PRNGs are not truly random, they can produce sequences that approximate the properties of random numbers. In contrast, true random numbers are generated from inherently random physical processes.
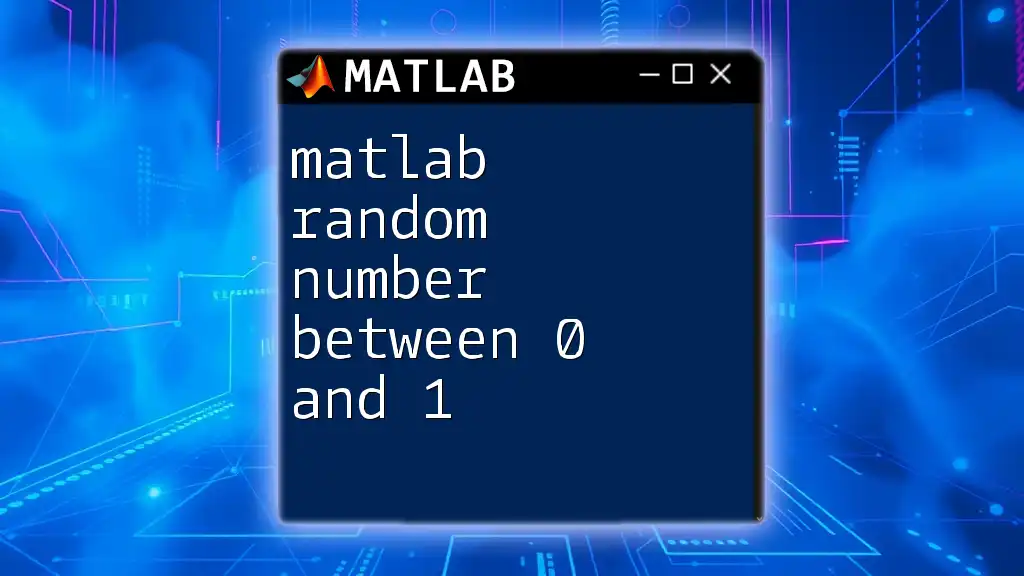
MATLAB's Random Number Functions
Basic Random Number Functions in MATLAB
MATLAB provides several built-in functions for generating random numbers:
- `rand`: Generates uniformly distributed random numbers in the interval (0, 1).
- `randn`: Produces normally distributed random numbers with a mean of 0 and a standard deviation of 1.
- `randi`: Generates uniformly distributed random integers within a specified range.
`rand` Function
Syntax and Usage
The `rand` function is used to create random numbers uniformly distributed between 0 and 1. The basic syntax is as follows:
A = rand(n);
This command generates an n x n matrix filled with random numbers. You can also specify dimensions as needed.
Examples
Creating a 2x3 matrix of random numbers can be accomplished with the following command:
B = rand(2, 3);
This will produce a matrix like:
0.8150 0.2785 0.9661
0.3894 0.5772 0.8480
Interpreting the output reveals that each value is a random decimal within the specified range.
`randn` Function
Syntax and Usage
To generate random numbers with a normal distribution, use the `randn` function:
C = randn(m);
Where `m` specifies the dimensions of the output matrix. The values generated will follow a standard normal distribution, characterized by a mean of 0 and a standard deviation of 1.
Examples
To create a 1x5 array of normally distributed random numbers, you would employ:
D = randn(1, 5);
The result will yield an array with numbers that have varying values, often centered around zero, demonstrating the properties of normal distribution perfectly.
`randi` Function
Syntax and Usage
To generate random integers, the `randi` function is particularly useful:
E = randi([low, high], size);
This allows you to specify a range `[low, high]` and the size of the resulting array.
Examples
For instance, to generate random integers between 1 and 10, use:
F = randi([1, 10], 1, 5);
This command produces a row vector of 5 random integers, which could look like:
3 8 1 4 10
This functionality can be particularly useful in simulations or scenarios where random selection is required, such as in gaming applications.
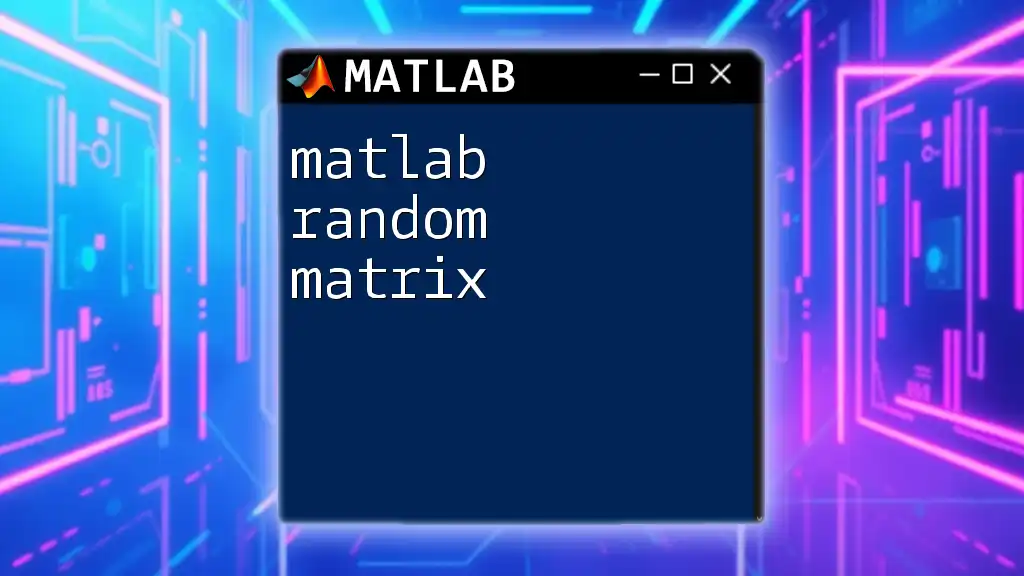
Setting Random Number Seeds
Reproducibility in Random Number Generation
Setting a seed for your random number generator is crucial for reproducibility. This means that every time you run a script with the same seed, you will generate the same sequence of random numbers. To set a seed, use the `rng` function:
rng(seed);
Examples
For example, you might want to generate a controlled sequence as follows:
rng(42); % Setting the seed
G1 = rand(1, 5);
rng(42); % Resetting the seed
G2 = rand(1, 5); % G1 and G2 will be identical
Here, both `G1` and `G2` will yield the same random numbers, which is invaluable for testing and debugging.
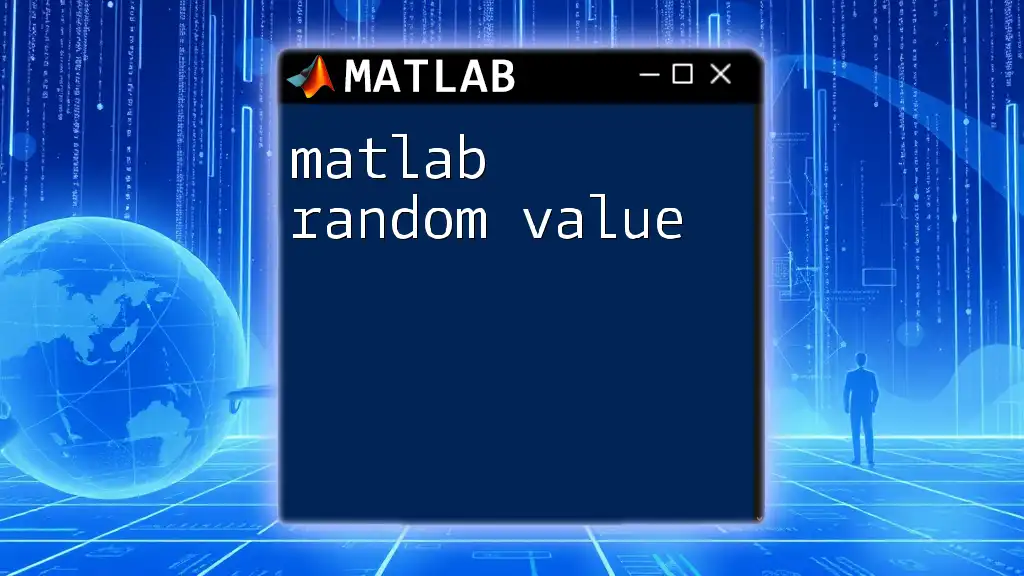
Advanced Random Number Techniques
Generating Random Samples from Custom Distributions
In more sophisticated applications, you may desire random samples from non-standard distributions. MATLAB allows you to draw samples based on weighted probabilities. This can be achieved using the `datasample` function:
p = [0.1, 0.3, 0.6]; % Example probabilities
outcomes = [1, 2, 3];
sample = datasample(outcomes, 100, 'Weights', p);
In this example, the variable `sample` will reflect the outcomes according to the specified weights.
Multidimensional Random Number Generation
If you need random numbers in higher dimensions, you can specify the size of the matrix as follows:
H = rand(3, 4); % Generates a 3x4 matrix of random numbers
This visualization can be particularly useful in multidimensional data analysis and simulations.
Visualizing Random Numbers
To easily visualize the distribution of random numbers, you can create scatter plots, which can be helpful for understanding random distributions:
scatter(rand(1, 100), rand(1, 100));
xlabel('Random X');
ylabel('Random Y');
title('Scatter Plot of Random Numbers');
This code will visualize the randomness of the generated points, providing insight into their distribution across a 2D plane.
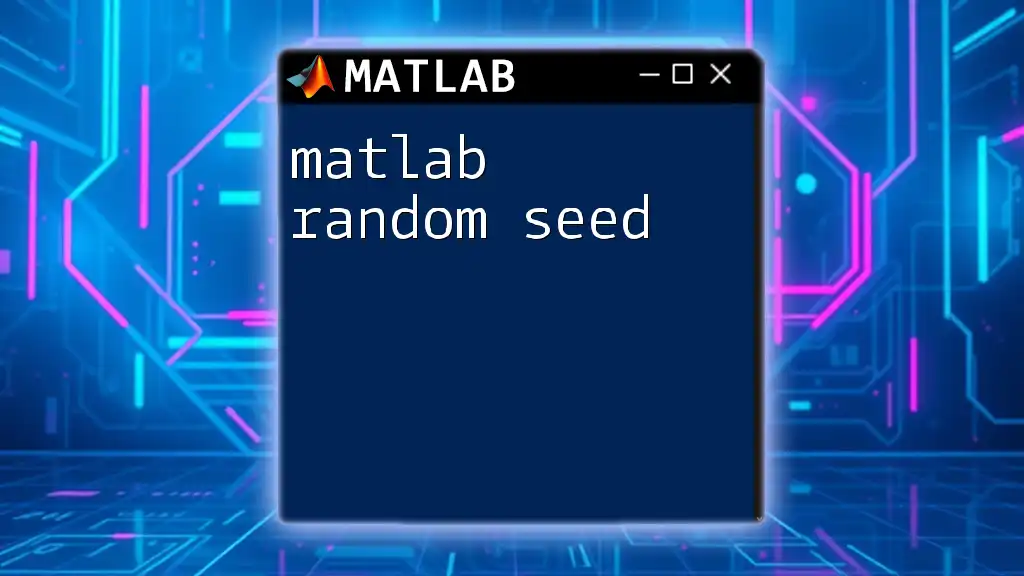
Conclusion
In summary, understanding the generation of random numbers in MATLAB through functions like `rand`, `randn`, and `randi` is fundamental for a wide range of applications. This guide has highlighted key functions, established the importance of reproducibility, and delved into advanced techniques for custom distributions and multidimensional data. Practicing these methods will enhance your mastery of random number manipulation in MATLAB, opening doors to more complex simulations and data analyses.
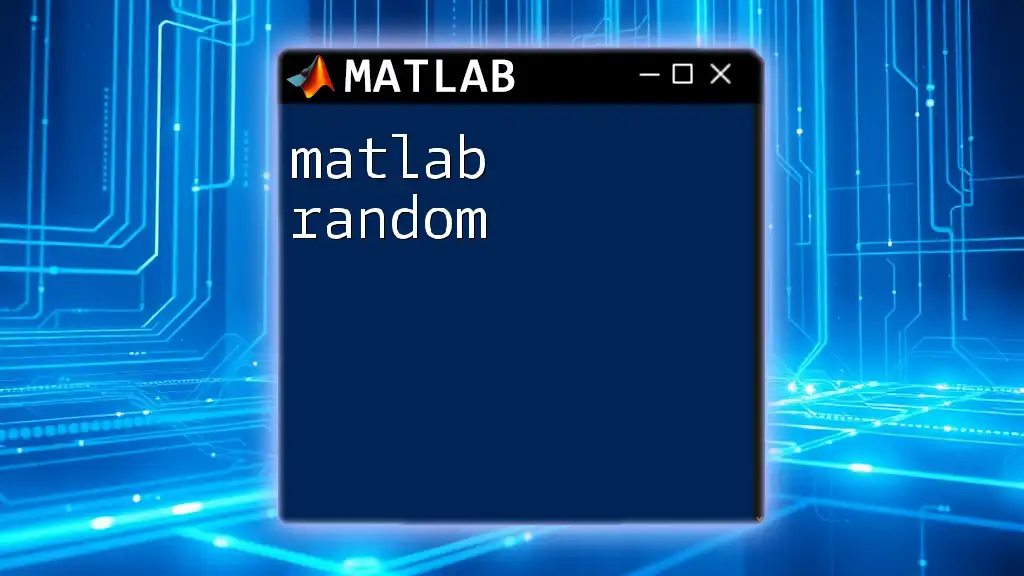
Additional Resources
For further exploration, the official MATLAB documentation on random number generation can provide deeper insights, and various online tutorials may assist in reinforcing your understanding. Don't hesitate to explore and experiment with these functions to become proficient in using MATLAB random numbers.