In MATLAB, you can generate random values using the `rand` function, which produces uniformly distributed random numbers in the interval (0,1).
% Generate a 3x3 matrix of random values
randomMatrix = rand(3);
Understanding Random Values in MATLAB
What Are Random Values?
Random values play a crucial role in various applications within MATLAB. In mathematical terms, a random value is a numerical value generated in such a way that it cannot be perfectly predicted. This characteristic is especially important in fields such as statistics, data analysis, and algorithm development, where randomness can mimic real-world variability and uncertainty.
When using MATLAB, understanding the nature of randomness allows you to perform simulations, make statistical inferences, and conduct randomness-based testing of algorithms more effectively.
Types of Random Values
In MATLAB, you can generate several types of random values, each serving distinct purposes:
-
Uniform Distribution: This type of distribution generates numbers that are evenly distributed across a specified range. For example, the command `rand(m,n)` produces random numbers in the interval [0, 1]. Uniform distributions are particularly useful in simulations where you need equal chance for outcomes.
-
Normal Distribution: Also known as Gaussian distribution, normal distributions give more weight to values around a central mean, producing a bell-shaped curve. This is achieved using the command `randn(m,n)`, which generates random numbers from a standard normal distribution with a mean of 0 and a standard deviation of 1. Normal distributions are often used in data analysis when dealing with natural phenomena, where values tend to cluster around a mean.
-
Other Distributions: MATLAB supports various other distributions, such as Poisson, Binomial, and Exponential. Each of these distributions has unique characteristics that can be beneficial in specific scenarios. For instance, the `poissrnd(lambda)` command generates random numbers from a Poisson distribution with a specified parameter `lambda`.
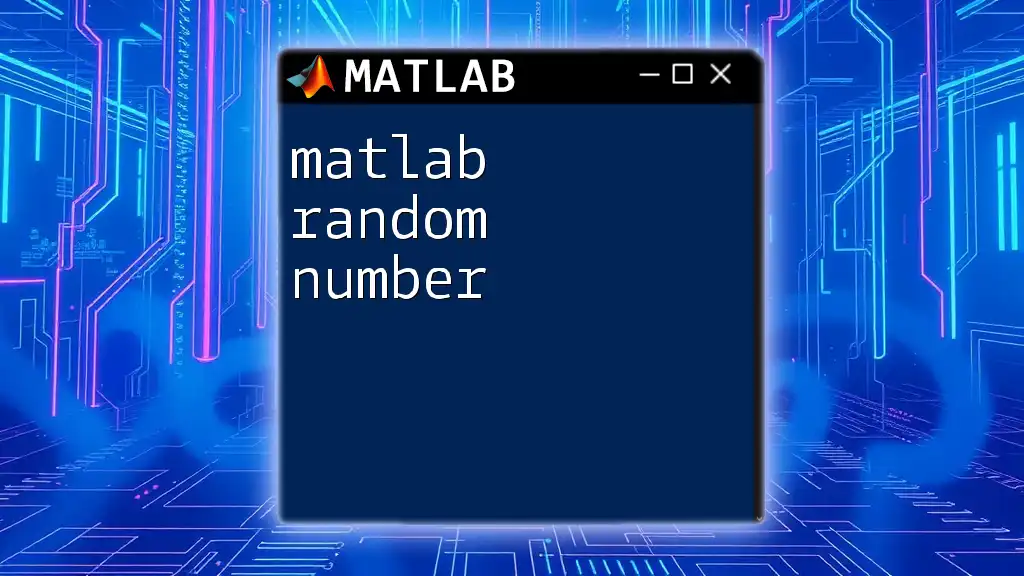
Generating Random Values in MATLAB
Basic Commands for Random Number Generation
MATLAB provides a set of straightforward commands to generate random values efficiently.
The `rand` Function
The `rand` function is the most commonly used to create random values uniformly distributed in the interval [0, 1]. Its syntax is simple:
r = rand(m,n)
For example, if you want to generate a 3x2 matrix of random numbers, you can use the following code:
% Generate a 3x2 matrix of random numbers
randomMatrix = rand(3, 2);
The `randi` Function
If you need random integers, the `randi` function is the go-to. This function generates uniformly distributed random integers within specified bounds. The basic syntax is as follows:
r = randi(imax, m, n)
To create a 1x5 vector of random integers ranging from 1 to 10, use:
% Generate a 1x5 vector of random integers between 1 and 10
randomIntegers = randi(10, 1, 5);
The `randn` Function
When you need random values from a normal distribution, use the `randn` function, which has a syntax similar to `rand`:
r = randn(m,n)
To generate a 4x4 matrix of random numbers from a standard normal distribution, you can write:
% Generate a 4x4 matrix of random numbers from a standard normal distribution
randomGaussianMatrix = randn(4, 4);
Specifying Ranges and Dimensions
MATLAB also enables you to create random numbers within specific ranges and dimensions.
Creating Random Numbers Within a Specific Range
To generate random numbers within a specified range, manipulate the output of `rand`. For example, to generate random numbers in the range [5, 10]:
% Generate random numbers in the range [5, 10]
randomRange = 5 + (10-5) * rand(1, 5);
This code effectively scales the output of the `rand` function to fit the range from 5 to 10.
Generating Multi-Dimensional Random Values
The ability to generate random values across multiple dimensions can significantly enhance data modeling. To create a 3D matrix filled with random numbers, you can utilize:
% Generate a 3D matrix of random numbers
random3D = rand(3, 3, 3);
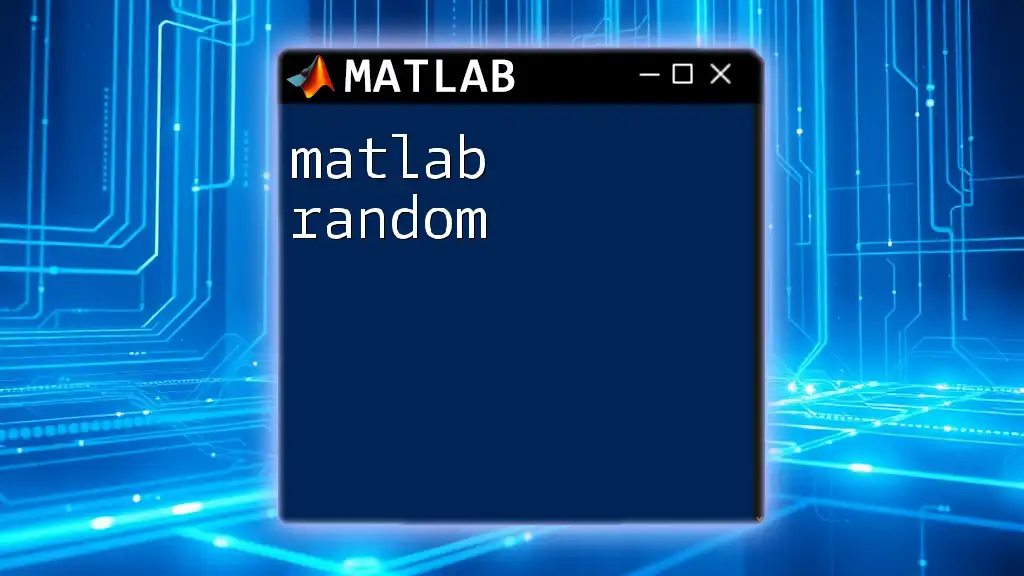
Practical Applications of Random Values in MATLAB
Simulations
Random values in MATLAB are extensively used for simulations, particularly in the context of Monte Carlo simulations. These simulations use randomness to model complex systems and evaluate probabilities. Here’s how you can implement a Monte Carlo simulation to estimate the value of π:
% Monte Carlo simulation for estimating pi
n = 100000; % Total number of random samples
x = rand(n, 1);
y = rand(n, 1);
inCircle = (x.^2 + y.^2) <= 1; % Identify points inside the unit circle
piEstimate = 4 * sum(inCircle) / n; % Estimate pi
This method randomizes point generation within a unit square and checks how many fall within the quarter circle to estimate π.
Data Analysis
Random sampling is essential in data analysis to extract representative samples from larger datasets. The `datasample` function can help achieve this. For example, to randomly sample 10 observations from a dataset named `data`:
% Randomly sample from a dataset
dataSample = datasample(data, 10);
This ensures your analysis remains unbiased and reflectively represents the population.
Algorithm Testing
Another significant application of MATLAB random values is in algorithm testing. Random values can serve as test inputs to validate the robustness and performance of an algorithm. For instance, generating random test cases can expose edge-case scenarios not initially considered, enhancing the reliability of your software.
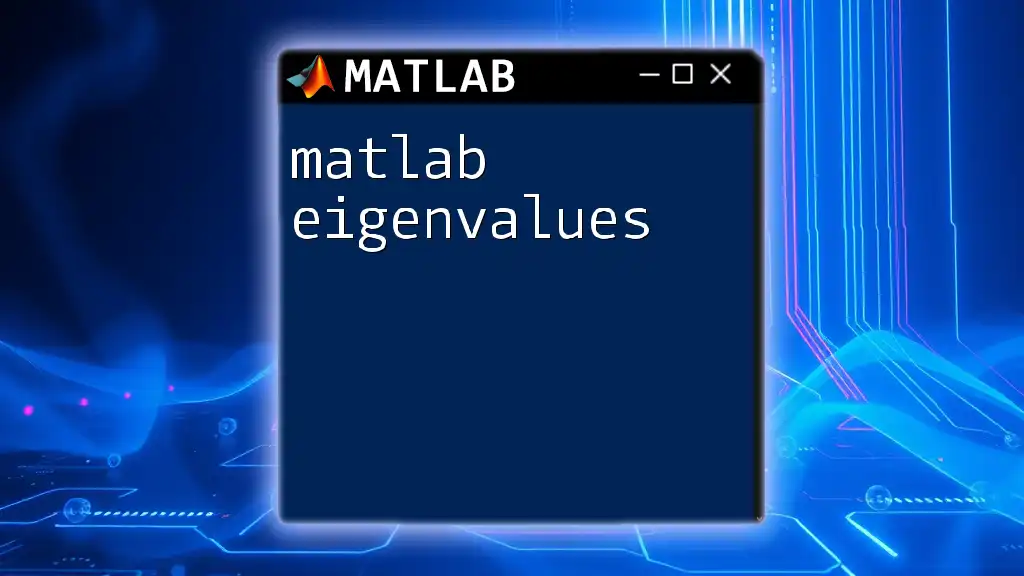
Visualizing Random Values
Visual representation of random data helps in grasping distribution behavior. MATLAB offers various plotting functions to visualize the generation and distribution of random values.
Plotting Random Data
Creating visualizations can give immediate insight into the behavior of the generated random numbers. To visualize a uniform distribution, you might consider using a histogram:
% Visualizing a uniform distribution
data = rand(1000, 1);
histogram(data, 30);
title('Uniform Distribution');
The histogram will display the uniform distribution over a specified number of bins, helping to illustrate how random numbers are uniformly spread.
Comparing Distributions
You can also compare different types of distributions visually. For example, overlaying a uniform and a normal distribution can clarify their distinct characteristics:
% Plotting uniform and normal distributions
x = linspace(-4, 4, 100);
yUniform = unifpdf(x, -1, 1);
yNormal = normpdf(x, 0, 1);
plot(x, yUniform, 'r', x, yNormal, 'b');
legend('Uniform', 'Normal');
title('Comparison of Random Distributions');
The resulting plot will illustrate the fundamental differences between the distributions visually, aiding in understanding their applications.
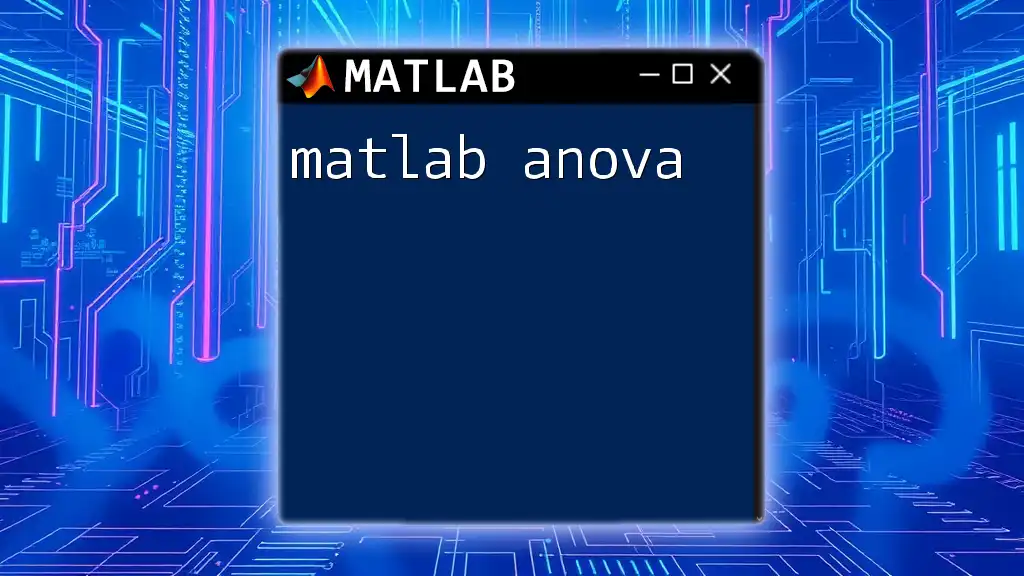
Common Pitfalls and Troubleshooting
Ensuring True Randomness
In MATLAB, it’s essential to be aware that random number generators are pseudo-random. If reproducibility of results is a requirement, use the `rng` function to set the random seed. This will ensure that your random numbers are the same every time you run your code:
% Set the random seed for reproducibility
rng(1);
randomValues = rand(1, 10);
Understanding Output
It's important to correctly interpret what the randomly generated values represent. Common misconceptions can lead to faulty analyses. Always validate the randomness by plotting or examining the outputs statistically to avoid biased conclusions.
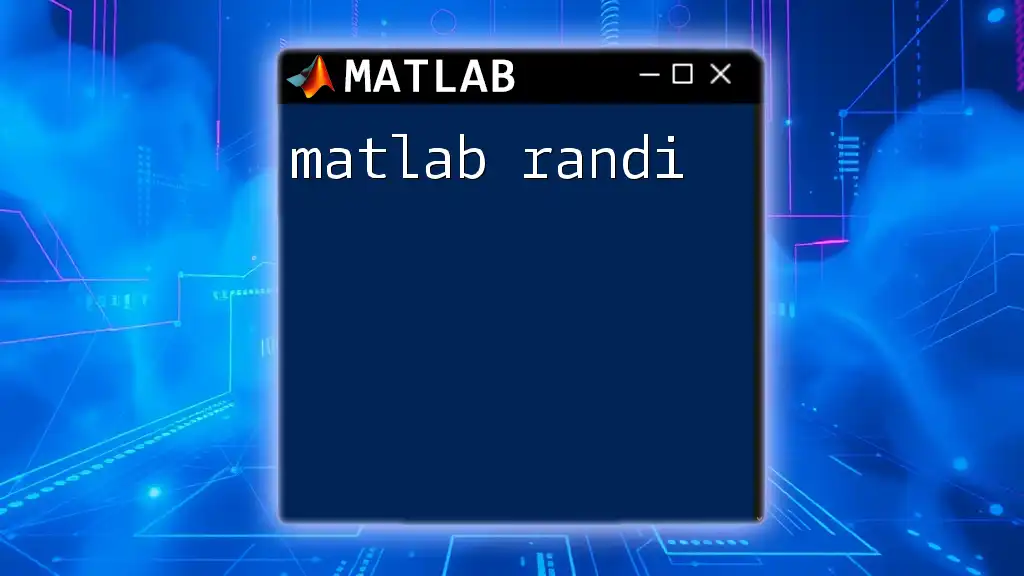
Conclusion
In summary, understanding the concept of MATLAB random values and their applications can greatly enhance your capabilities in simulations, data analysis, and algorithm testing. MATLAB’s diverse range of functions for generating random numbers provides the flexibility needed to apply randomness effectively across various fields.
By practicing these commands and exploring their applications, you’ll be better equipped to leverage randomness in your projects and analyses with confidence.