Join our MATLAB Debugger Webinar to gain essential skills in troubleshooting and optimizing your MATLAB code efficiently, using tools like breakpoints and the debug command to enhance your programming workflow.
Here's a quick example of using breakpoints in MATLAB:
% Set a breakpoint by clicking on the left margin next to the line number
result = myFunction(inputData); % This line will pause execution if a breakpoint is set
disp(result); % Output the result after debugging
What is the MATLAB Debugger?
The MATLAB Debugger is a powerful tool designed to help users identify and fix errors in their code efficiently. Debugging is an essential part of programming, as it involves not just finding bugs but understanding why they occur. By harnessing the capabilities of the debugger, users can significantly improve their coding skills and flow, leading to fewer errors and more robust applications.
Benefits of using the MATLAB Debugger include:
- Error Detection: Quickly identify and rectify bugs in your code, which can save a substantial amount of time.
- Performance Improvement: Optimize code execution through thorough examination and understanding of variable states and logic flow.
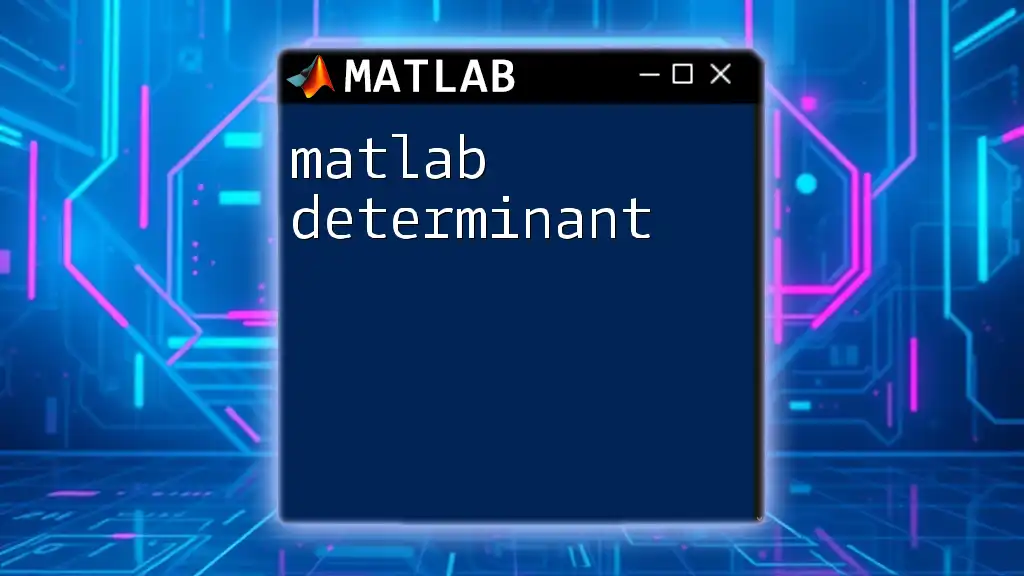
Getting Started with the MATLAB Debugger
Accessing the Debugger
To leverage the debugger effectively, you first need to know how to invoke it. You can start the debugger by entering the following command in the Command Window:
dbstop if error
This command tells MATLAB to stop execution whenever an error occurs, allowing you to inspect the state of your code at that moment.
Understanding Debugger Interfaces
When you start debugging in MATLAB, you'll interact with several key components:
- MATLAB Desktop Environment: This is the main interface, where you can view scripts, run commands, and monitor outputs.
- Debugging Windows:
- Command Window: The area where you can interact with MATLAB directly.
- Editor: Where you write and edit your scripts.
- Variable Editor: Displays the current values of variables.
- Call Stack Window: Shows the sequence of function calls that led to the current point in the code, facilitating easier tracking of how control flows through your program.
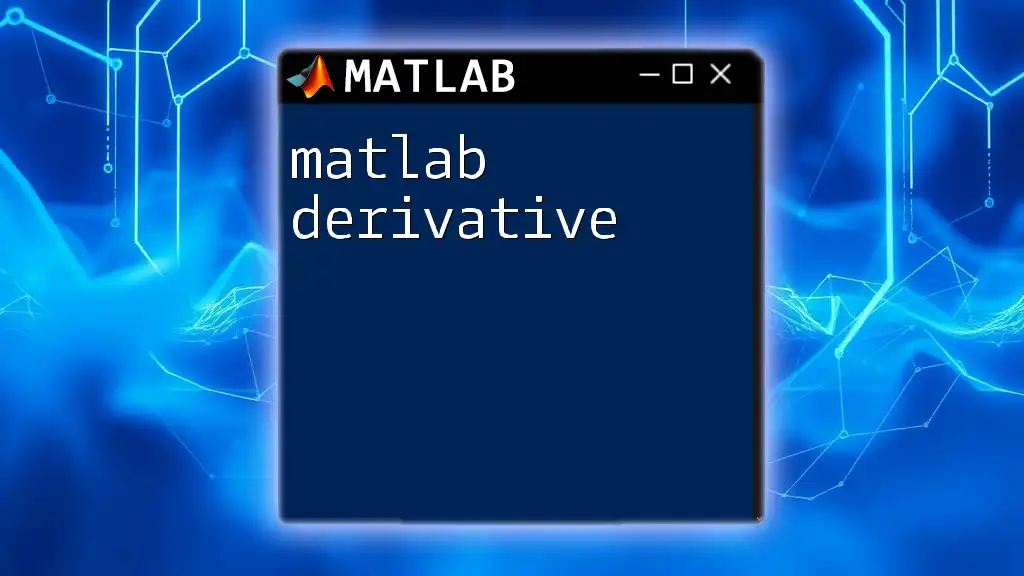
Key Debugging Tools and Features
Breakpoints
Breakpoints are markers you can set in your code that instruct the debugger to pause execution at specific locations. This is essential in examining your code in-depth. To set a breakpoint, use the following command:
dbstop at line_number
For example, setting a breakpoint at line 5 of your function can be done like this:
function myFunction()
dbstop at 5; % Breakpoint at line 5
% Function Code
end
When the script reaches line 5, execution will halt, and you can inspect variables and the flow of execution.
Step Execution
Once the debugger halts at a breakpoint, you can use step commands to navigate through your code:
- `dbstep`: Moves into the current line of code, allowing you to execute one line at a time and drop into any functions being called.
- `dbcont`: Continues code execution until the next breakpoint.
- `dbquit`: Exits the debugger entirely.
For example, you might want to step through your function to monitor the state of certain variables:
dbstep % Move to the next line of code
Examining Variables
During debugging, examining variable values is key. You can display variable values directly in the Command Window. For instance, to view the value of a variable, use:
disp(variable_name)
This helps you understand how the function's logic is impacting variable states.
Setting Conditional Breakpoints
Conditional breakpoints allow you to specify conditions under which a breakpoint should trigger. This further refines the effectiveness of your debugging sessions. The syntax for setting a conditional breakpoint is:
dbstop if variable_name == value
For example, you might only want to halt execution when a variable `x` reaches a value of `10`:
dbstop if x == 10
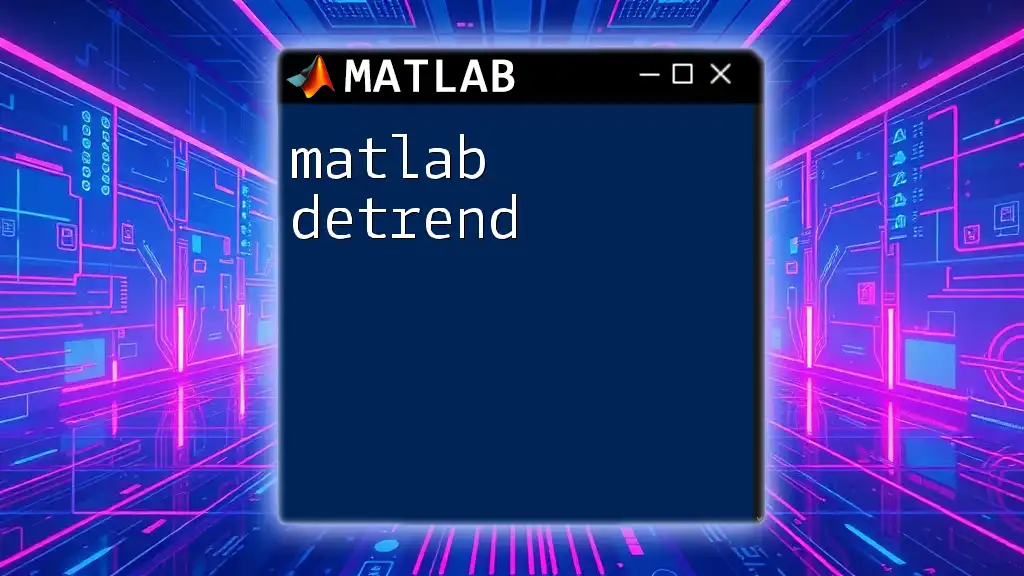
Debugging Best Practices
Common Debugging Techniques
-
Logical Checks: Ensure the logic in your code is correct by reviewing it step-by-step and verifying assumptions.
-
Narrative Tracing: Document your thought process alongside your code using comments. This narrative can help you backtrack when errors occur.
-
Incremental Testing: Test smaller sections of code before scaling up to the entire program. This can catch issues early.
Creating Effective Debugging Workflows
-
Combining Functions: Write modular code by breaking your program into functions. This division allows for easier troubleshooting since individual components can be tested separately.
-
Version Control: Implement version control (like Git) to help manage changes. Well-maintained versions can simplify reverting to previous states when errors are encountered.
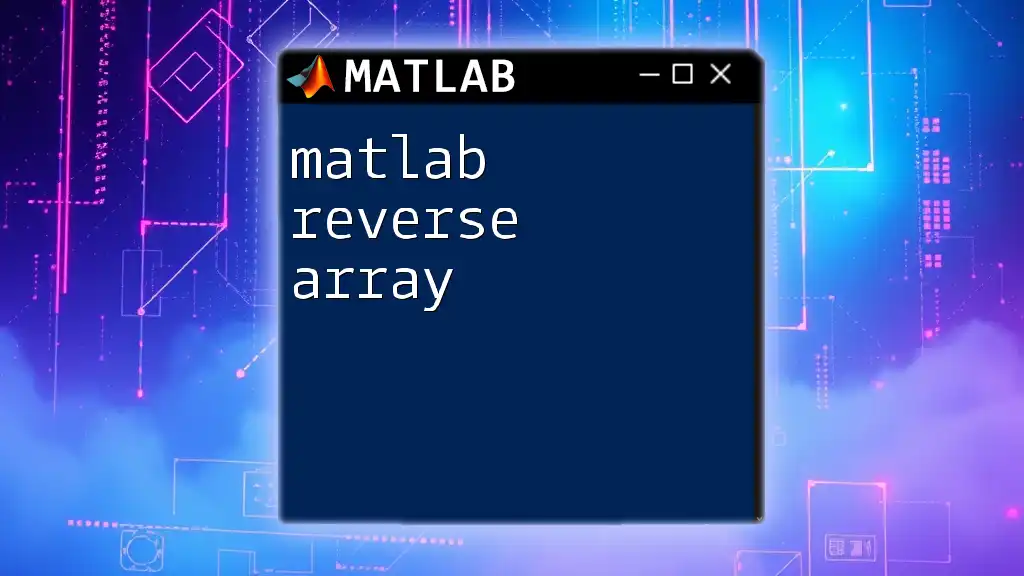
Advanced Debugging Techniques
Using `dbstatus`
The command `dbstatus` is a useful tool that allows users to check which breakpoints are currently active. This command outputs a list of all breakpoints, providing clarity on your debugging environment:
dbstatus % Display active breakpoints
Leveraging MATLAB’s Built-in Functions
MATLAB’s built-in functions, such as `try/catch`, can gracefully handle errors without compromising other parts of the program. This method can be particularly effective when dealing with potentially erroneous code blocks. An example of using `try/catch` is as follows:
try
% Code that may error
catch exception
disp(exception.message) % Handle the error gracefully
end
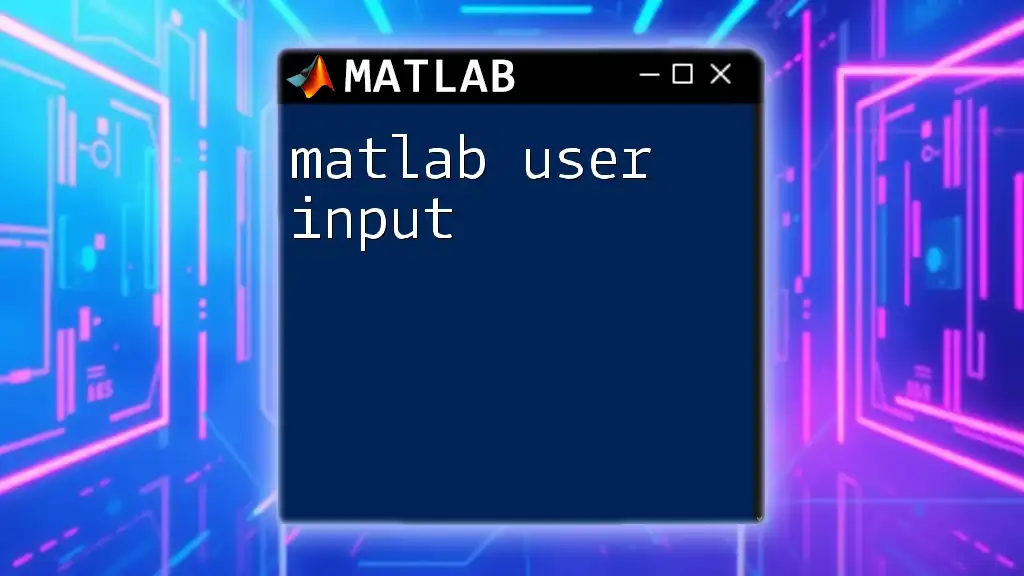
Resources for Continuing Education
For those looking to deepen their understanding of debugging in MATLAB, several resources can be immensely beneficial:
-
Recommended Reading: The official MATLAB documentation provides extensive tutorials and reference material.
-
Online Forums and Community Support: Platforms such as MATLAB Central are great for engaging with other users and finding solutions to common problems.
-
Links to Additional Webinars and Tutorials: Look out for future webinars that can provide live demonstrations, interactive learning experiences, and real-time Q&A sessions.
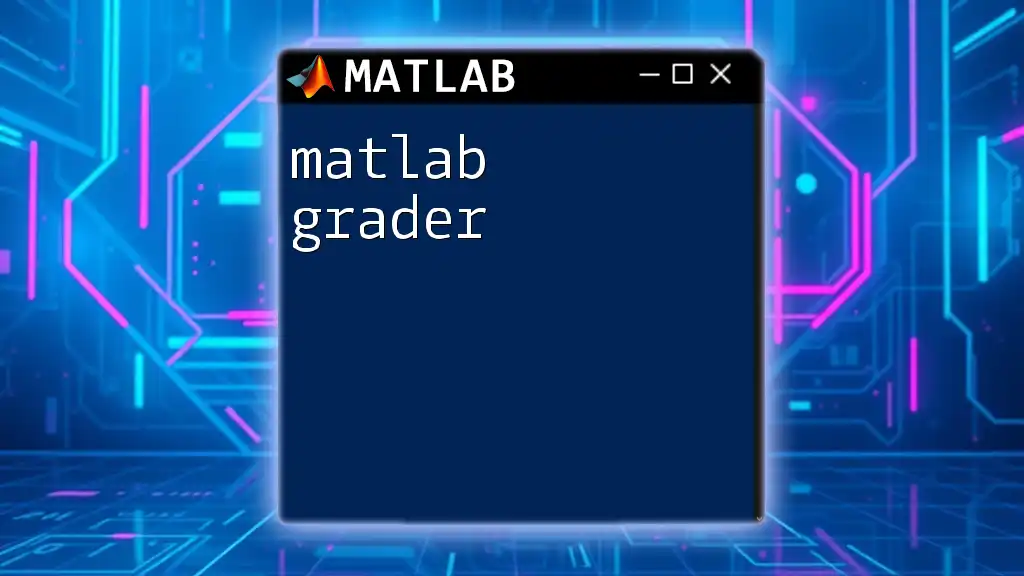
Conclusion
In conclusion, understanding and utilizing the MATLAB Debugger is vital for anyone looking to improve their programming efficiency and effectiveness. The tools and techniques covered in this guide are essential for both novice and experienced users alike.
By practicing regularly with debugging tools, you will foster a deeper understanding of your code's behavior, ultimately leading to enhanced programming skills. Don't miss out on the opportunity to join our MATLAB Debugger Webinar for a more interactive and thorough exploration of these concepts!
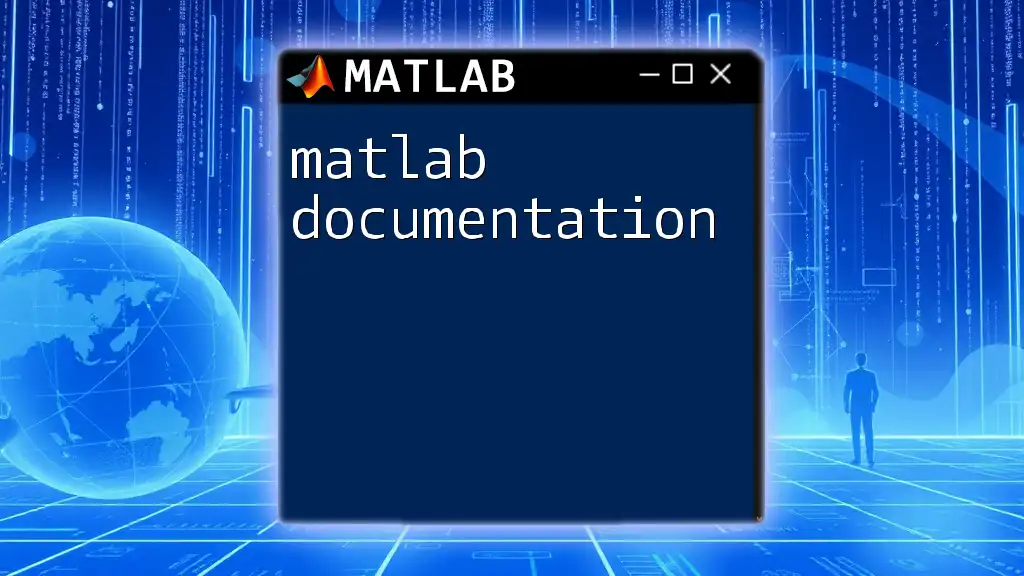
FAQs Section
What is the difference between a breakpoint and a watchpoint?
A breakpoint pauses the execution of your code at specified locations, whereas a watchpoint monitors the value of a variable, triggering the debugger only when that value changes.
Can I debug multiple files at once?
Yes, MATLAB allows debugging across multiple files, enabling you to open and step through the code from various functions or scripts seamlessly.
What common mistakes should I avoid while debugging?
Some common pitfalls include ignoring error messages, not utilizing breakpoints effectively, and failing to document your thought process during the debugging session.
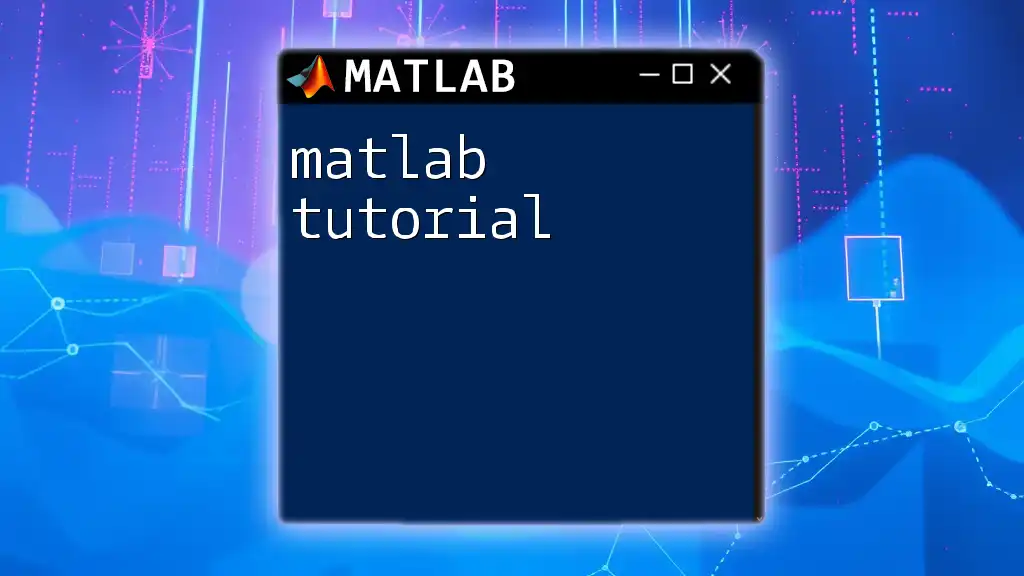
Call to Action
Join us for the upcoming MATLAB Debugger Webinar! This event promises to enhance your understanding of debugging with live demonstrations, tips from experts, and an interactive Q&A session. Register now to secure your spot and take your MATLAB skills to the next level!