In MATLAB, you can create a vector using square brackets, separating the elements with spaces or commas for a row vector, or semicolons for a column vector.
% Row vector
row_vector = [1, 2, 3, 4, 5];
% Column vector
column_vector = [1; 2; 3; 4; 5];
Understanding Vectors in MATLAB
Definition of a Vector
In the context of MATLAB, a vector is a one-dimensional array that can hold a sequence of numerical values. It can either be a row vector, which is a horizontal arrangement of elements, or a column vector, which is a vertical arrangement. Understanding the distinction between these two types is crucial for correctly utilizing vectors in your computations.
Types of Vectors
Row Vectors: These are defined using a space or a comma to separate elements and are placed within square brackets. A row vector is formed by listing values horizontally.
rowVec = [1, 2, 3, 4, 5]; % A simple row vector
Column Vectors: These vectors utilize semicolons to separate the individual elements. This format places values vertically.
colVec = [1; 2; 3; 4; 5]; % A simple column vector
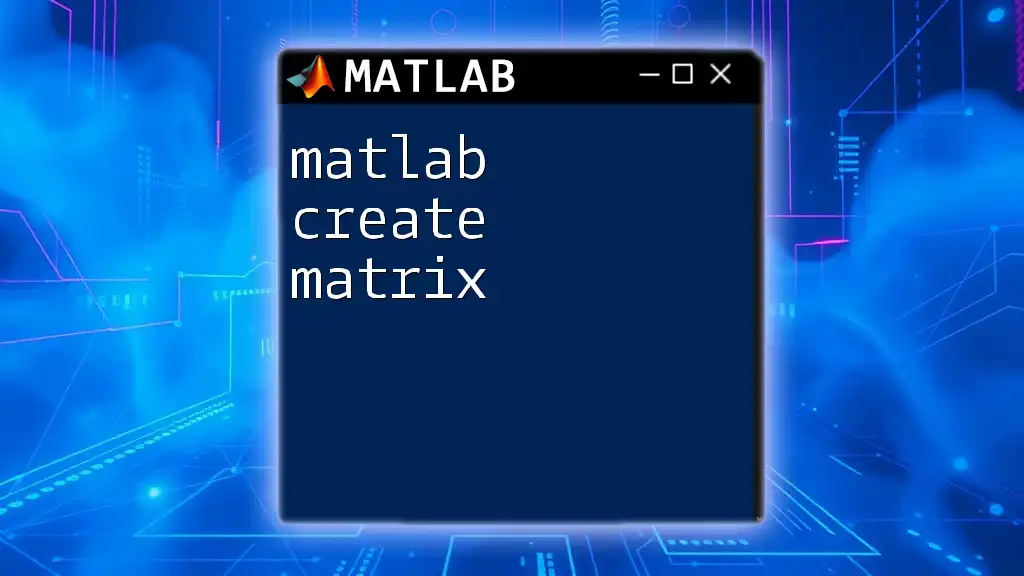
Creating Vectors in MATLAB
Creating Row Vectors
Using Square Brackets: The most straightforward method to create a row vector is by enclosing comma-separated values within square brackets.
rowVec1 = [1, 2, 3, 4, 5]; % An example of a row vector
Using the `linspace` Function: MATLAB offers the `linspace` function, which can generate vectors with equally spaced elements. The function accepts three parameters: the starting value, the ending value, and the number of points to generate.
rowVec2 = linspace(1, 10, 5); % Creates a vector with 5 linearly spaced points from 1 to 10
Creating Column Vectors
Using Square Brackets: Just like with row vectors, you can create a column vector by separating individual elements with semicolons within square brackets.
colVec1 = [1; 2; 3; 4; 5]; % An example of a column vector
Using the `linspace` Function: Creating a column vector with `linspace` is similar; however, to convert a generated row vector to a column vector, you can transpose it using the apostrophe operator.
colVec2 = linspace(1, 10, 5)'; % Transpose to convert row vector to column vector
Creating Vectors with Specific Increments
Using the Colon Operator: MATLAB's colon operator (:) is an efficient way to create vectors with specific increments. This operator takes the form `start:increment:end`, where `start` is the initial value, `increment` is the specified step size, and `end` is the final value.
vectorWithIncrements = 1:2:10; % Creates vector [1, 3, 5, 7, 9]
Combining `linspace` with Different Parameters: This method is useful when you need a specific number of points across a defined range, ensuring that the values are evenly distributed.
vectorWithLinspace = linspace(0, 1, 5); % 5 points between 0 and 1
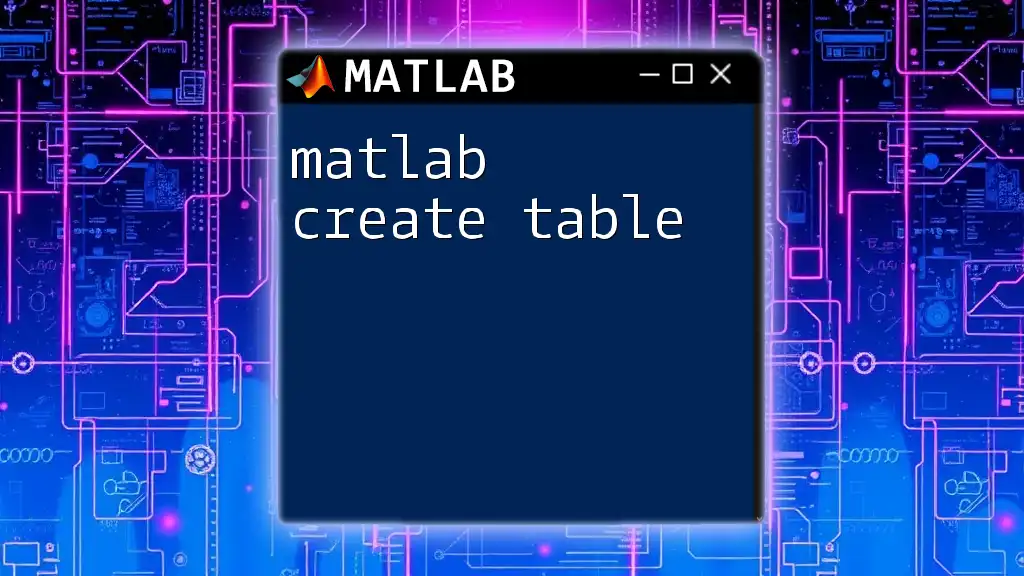
Vector Manipulation and Operations
Accessing Elements of Vectors
Accessing specific elements within a vector is simple. You simply specify the index of the element you wish to retrieve using parentheses. Remember that MATLAB indices start at 1.
element = rowVec1(3); % Access the 3rd element of rowVec1
Modifying Vectors
Changing values in a vector is straightforward. By using the index, you can assign a new value to a specific position within the vector.
rowVec1(3) = 10; % Change the 3rd element value to 10
Concatenating Vectors
MATLAB allows for easy combination of vectors. You can concatenate row and column vectors, provided you transpose them properly to match dimensions.
newRowVec = [rowVec1, colVec1']; % Concatenating a row and a column vector
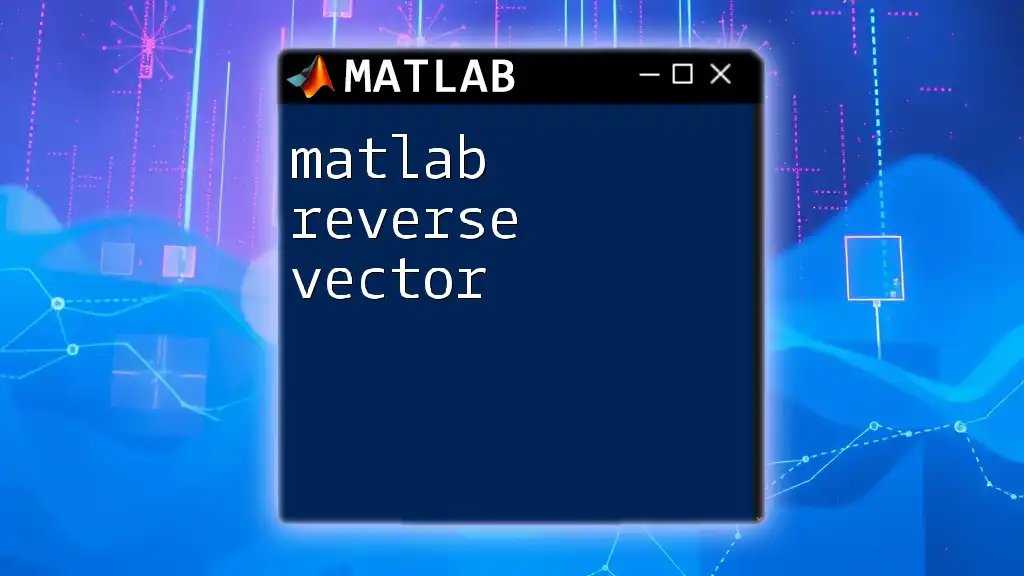
Advanced Vector Creation Techniques
Using Functions to Generate Vectors
Creating custom functions can significantly streamline vector generation tailored to your needs. For instance, a functions like this demonstrates how to encapsulate vector generation logic:
function vec = myVectorGenerator(startVal, endVal, numElements)
vec = linspace(startVal, endVal, numElements);
end
Vectorization Advantages
MATLAB optimizes vectorized operations for performance. Instead of relying on loops, utilize operations that can be performed on entire vectors. This can greatly enhance code execution speed.
For example, instead of using a for-loop to perform an operation on each element:
for i = 1:length(rowVec)
rowVec(i) = rowVec(i) * 2; % Multiplying each element by 2
end
You can achieve the same result with vectorized operations:
rowVec = rowVec * 2; % Efficiently multiplies the vector by 2
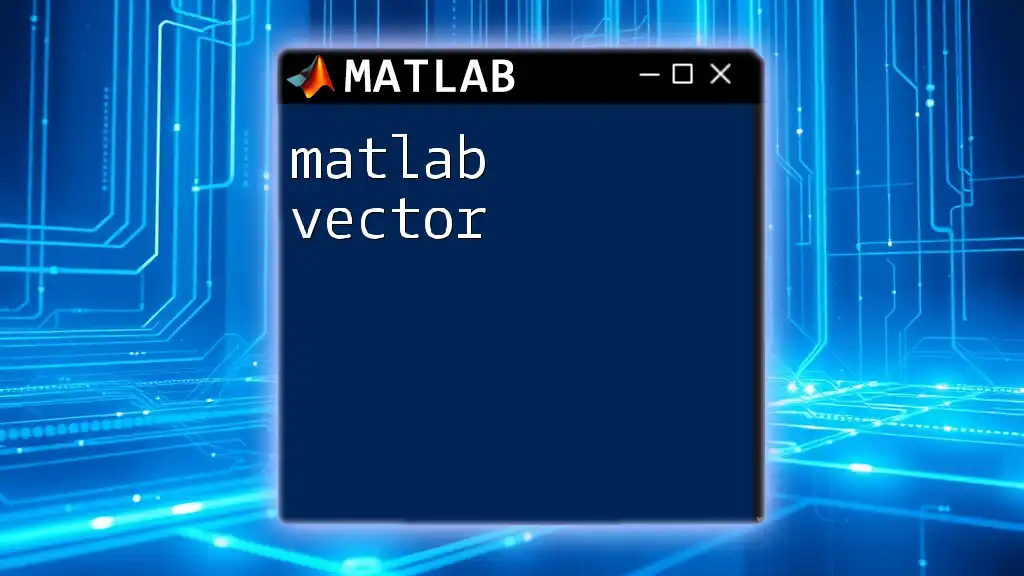
Common Mistakes When Creating Vectors
When creating vectors, there are several common pitfalls that you should be aware of. One frequent mistake is a mismatch between row and column dimensions during concatenation, which results in an error.
To avoid these issues:
- Always check your dimensions before concatenation.
- Be cautious with the use of parentheses and the distinction between row and column vectors.
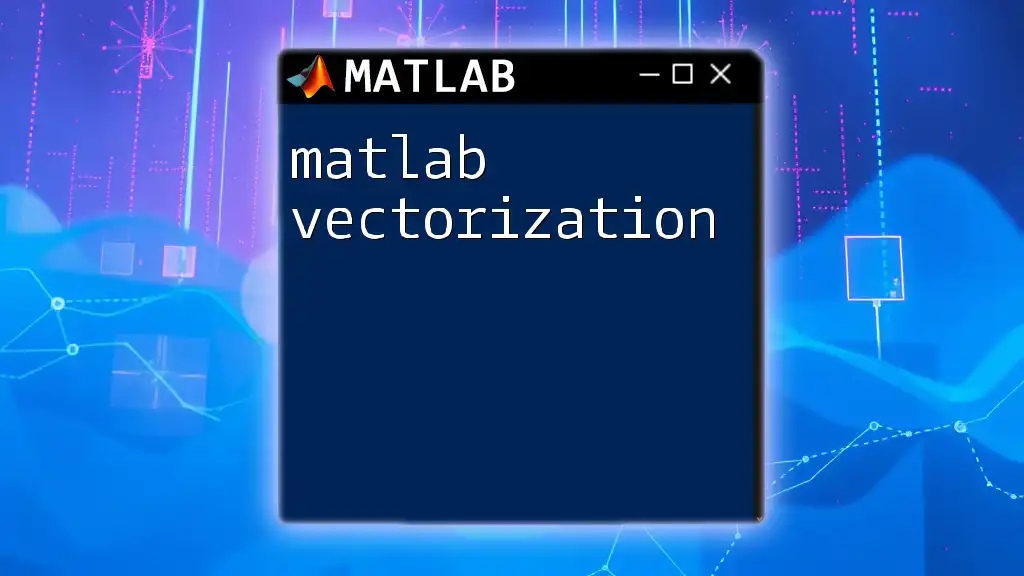
Conclusion
Creating vectors in MATLAB is a fundamental skill that enhances your programming capabilities. From basic creation methods to advanced manipulation and optimization through vectorization, understanding how to matlab create vector enables you to unlock the full power of MATLAB for your mathematical and computational needs. Regular practice with the commands and concepts discussed will ensure a solid grasp of vector operations. For further learning, consider exploring the extensive MATLAB documentation and online resources dedicated to MATLAB programming.