The `assert` function in MATLAB is used to verify that a condition is true, and it produces an error if the condition evaluates to false, helping ensure that code behaves as expected.
assert(isnumeric(A), 'Input A must be a numeric array.');
What is `assert` in MATLAB?
The `assert` function in MATLAB is a crucial tool for ensuring that conditions in your code are met before proceeding with computations. It serves as a validation mechanism that helps confirm certain expectations about your variables or program state. By utilizing `assert`, programmers can catch errors early in the development process, significantly reducing debugging time and enhancing code reliability.
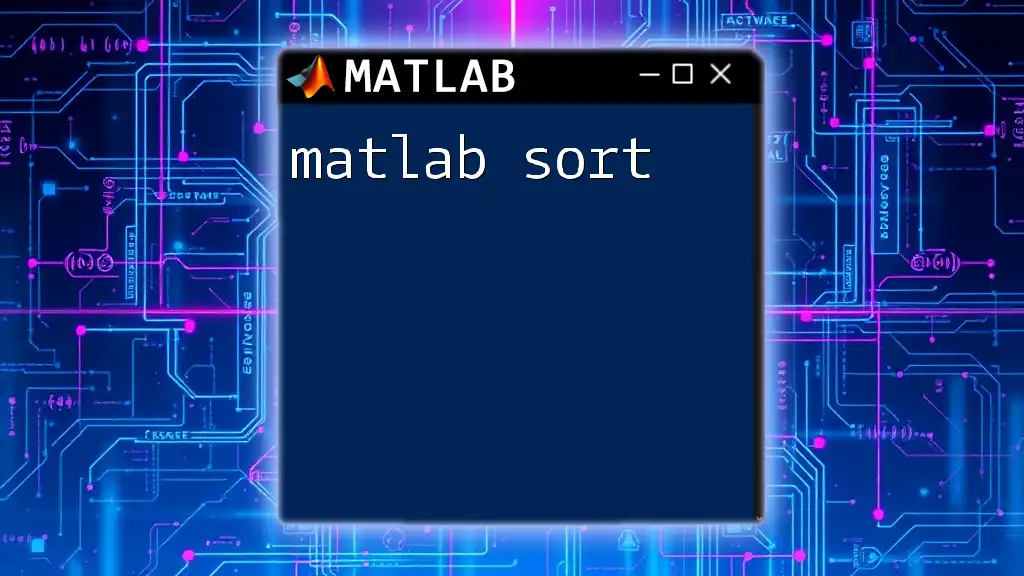
Why Use `assert`?
-
Ensuring Correctness of Conditions: By asserting conditions, you can ensure that your code behaves as intended. This not only helps in verifying logic but also provides a safety net against unexpected input or states.
-
Debugging Advantages: `assert` acts as a guardrail during code execution. When an assertion fails, it generates an error message that references the problematic condition, providing developers with valuable insight into what went wrong.
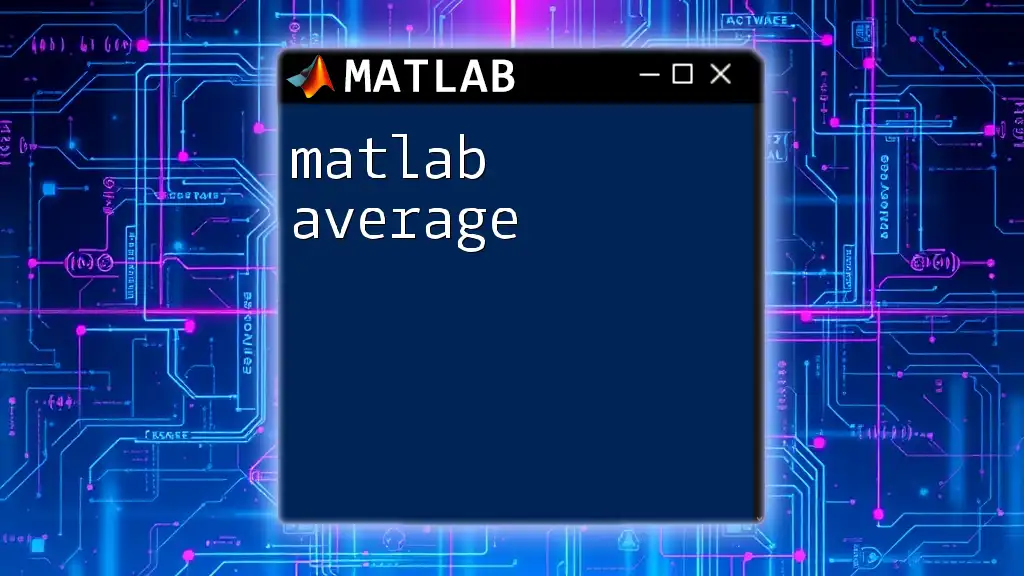
Syntax of `assert`
Basic Syntax
The basic structure of the `assert` function is straightforward, consisting of a condition to evaluate and an optional message that describes what went wrong if the assertion fails.
assert(condition, message)
Components of the Syntax
- condition: This is the logical expression that you expect to be true. If it evaluates to false, the assertion fails, and MATLAB throws an error.
- message: A brief description of the assertion's purpose. This message becomes the error output, aiding in debugging.
For example, you might assert that a variable `a` should equal `5`:
a = 5;
assert(a == 5, 'a must be equal to 5');
If `a` were not `5`, MATLAB would halt execution and print the message you provided.
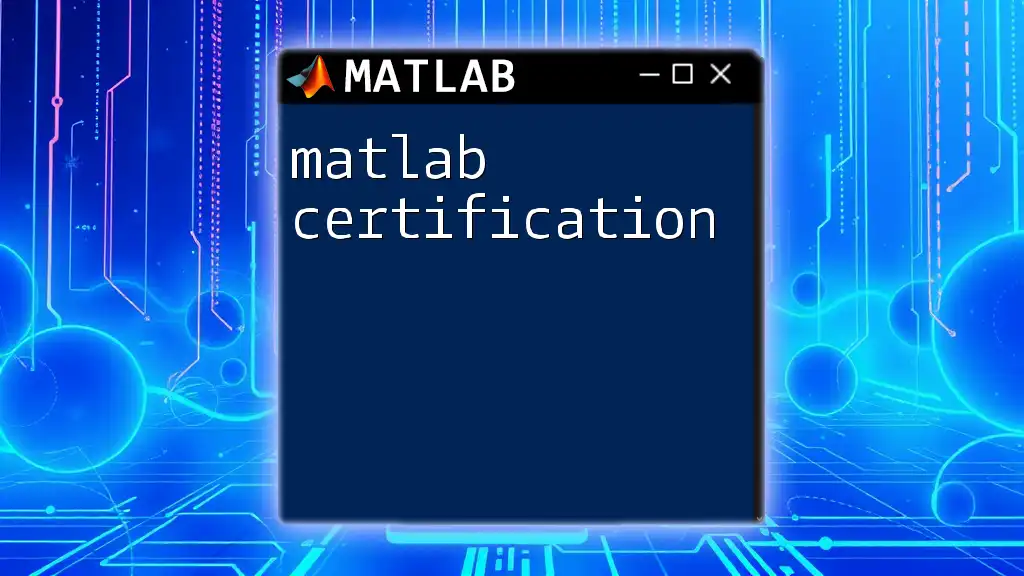
How `assert` Works
Evaluating Conditions
When `assert` is called, MATLAB evaluates the provided condition. If the condition is true, the program continues execution without interruption. However, if the condition is false, MATLAB stops execution and displays the specified error message.
Example Scenarios
Scenario 1: Variables and Mathematical Conditions
You can use `assert` to check values from mathematical comparisons. For instance:
x = 10;
y = 5;
assert(x > y, 'x must be greater than y');
In this example, since `x` is indeed greater than `y`, the assertion will pass without any issues. If you change `x` to a value less than or equal to `5`, the program will stop and produce the error message.
Scenario 2: Function Outputs
`assert` is also invaluable for validating outputs of functions. Here is how you can implement it:
function result = square(val)
assert(isnumeric(val), 'Input must be numeric');
result = val^2;
end
With this function, if the input `val` is anything other than a numeric type, the assertion will trigger, preventing further computation and alerting the user to the issue.
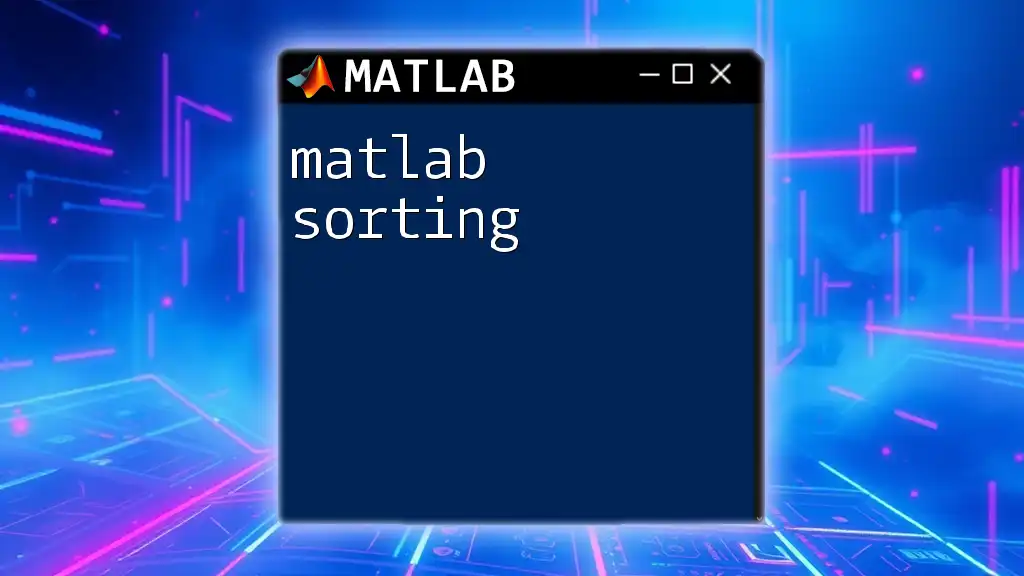
Common Use Cases for `assert`
Input Validation
One of the primary areas where `assert` shines is in input validation. It’s critical to ensure that the arguments passed to functions meet expected criteria. Consider the following example:
function divide(a, b)
assert(b ~= 0, 'Denominator must not be zero.');
disp(a/b);
end
Here, `assert` is used to prevent division by zero. If the second parameter `b` is found to be zero, MATLAB will stop execution and raise an informative error.
Checking Program State
You can use `assert` to verify the state of variables, especially when the state is crucial for the logic of your program. For example, you might check that two matrices are of the same size before performing an operation:
A = [1, 2, 3];
B = [4; 5; 6];
assert(all(size(A) == size(B)), 'A and B must be of the same size');
This assertion ensures that operations involving matrices `A` and `B` are valid, supporting clear and bug-free code.
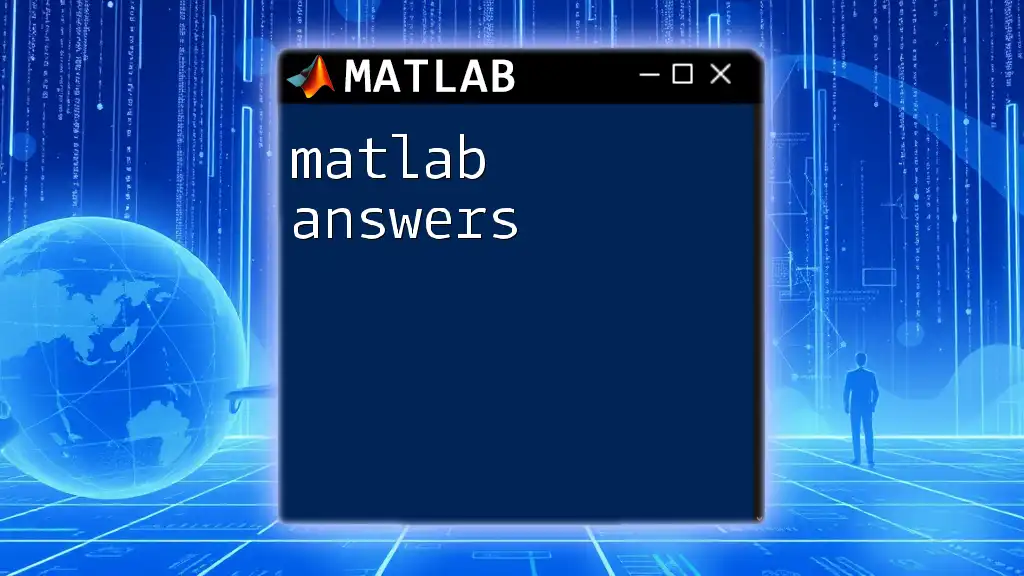
Best Practices When Using `assert`
Utilizing `assert` for Debugging
Using `assert` effectively during development can seamlessly identify logical errors. However, avoid using `assert` for production code, as it can lead to unintended terminations if conditions aren't met during normal operation. Rather, use it primarily for development and testing.
Writing Clear Messages
When crafting error messages for your assertions, clarity is key. The messages should be descriptive yet concise, allowing the developer to quickly understand what went wrong. For instance, use specifics rather than vague messages:
Good example: `assert(a > 0, 'Variable a must be positive for calculation.')`
Poor example: `assert(a > 0, 'Error at input.')`
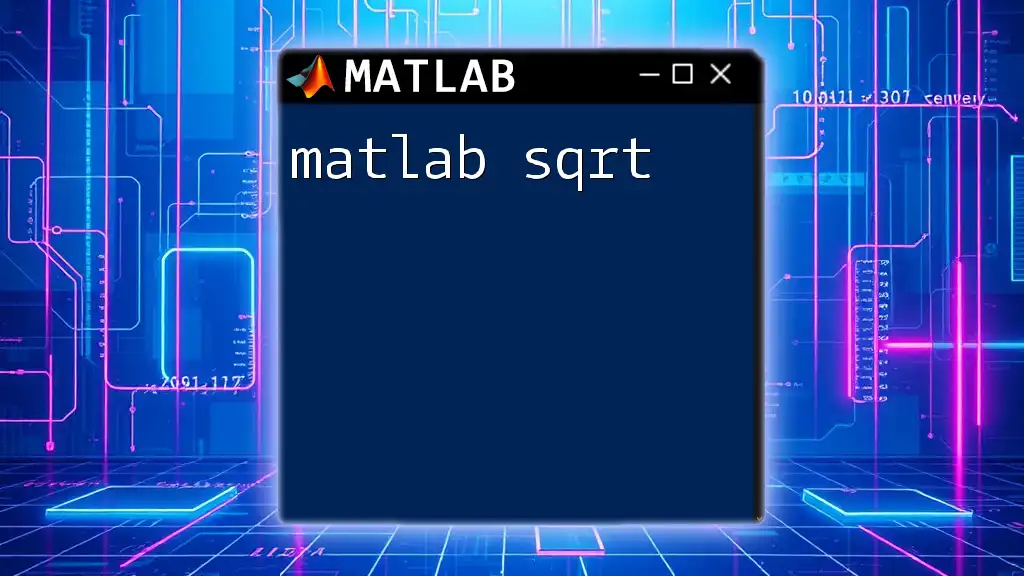
Limitations of Using `assert`
Considerations for Deployment
While `assert` is invaluable during development, it’s important to recognize that the function can be stripped out in optimized code. If too many assertions run in production, they can adversely affect performance and lead to inefficient execution.
Alternatives to `assert`
In situations where you require more control over error handling, consider using `try-catch` blocks. These constructs allow for managing error scenarios without abruptly halting the program.
try
divide(10, 0);
catch ME
disp(ME.message);
end
This method gives you the flexibility to handle errors gracefully without using assertions.
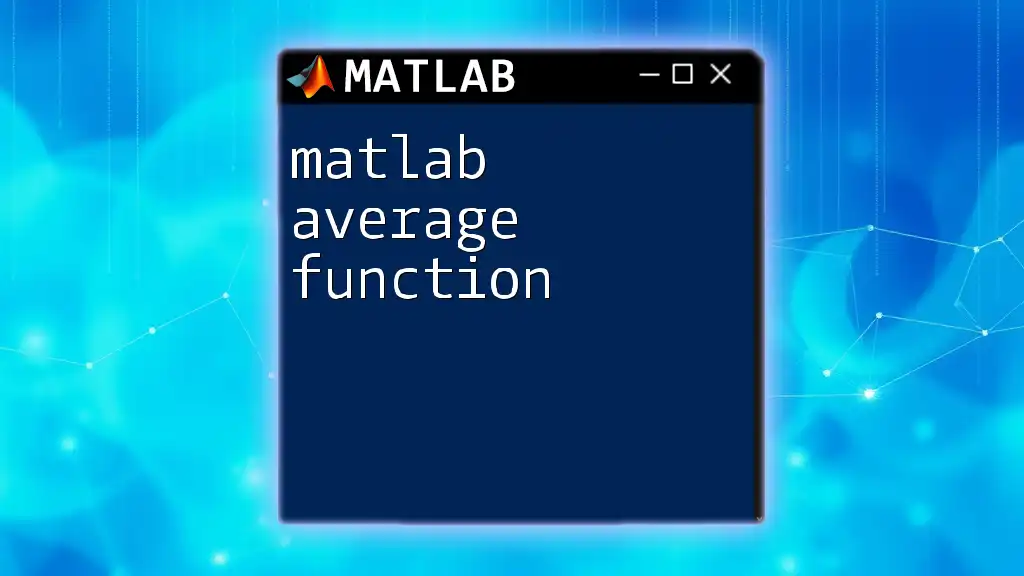
Conclusion
In summary, the `assert` function in MATLAB is a powerful tool for ensuring that your code is safe and behaves as expected. By performing critical checks on your variables and functions, you significantly enhance the reliability of your programs.
As you continue your journey in mastering MATLAB, consider incorporating `assert` into your coding standards for enhanced validation. Keep practicing and stay aware of other validation techniques for a comprehensive understanding of error handling in MATLAB.
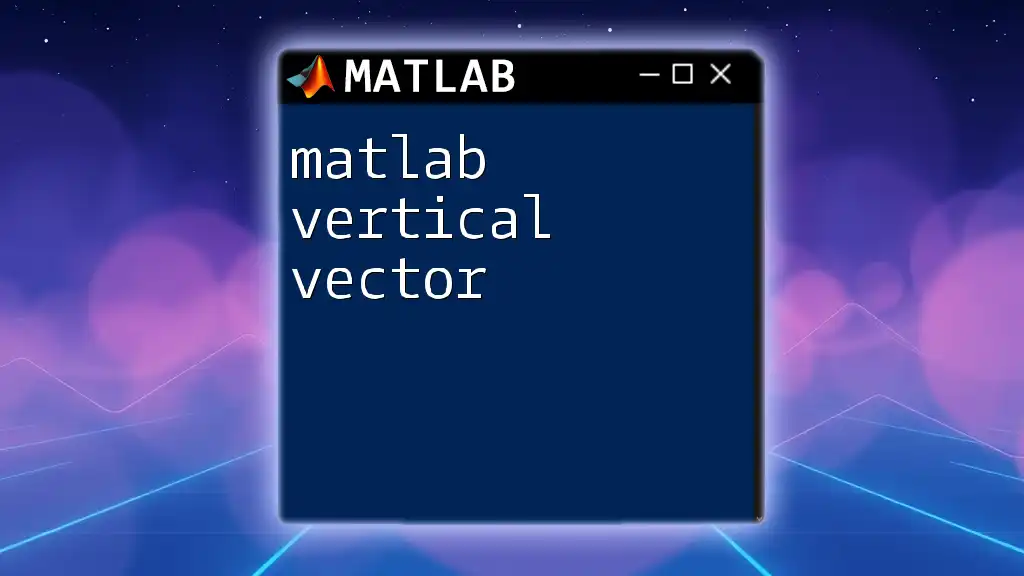
Additional Resources
For further reading and mastery of `assert` and other MATLAB functions, refer to the official MATLAB documentation and explore coding tutorials that can deepen your understanding of MATLAB's validation and error handling capabilities.